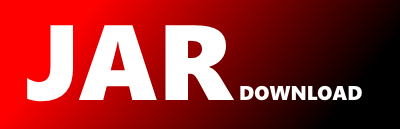
com.amazonaws.services.securitylake.AmazonSecurityLakeAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-securitylake Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.securitylake;
import javax.annotation.Generated;
import com.amazonaws.services.securitylake.model.*;
/**
* Interface for accessing Amazon Security Lake asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.securitylake.AbstractAmazonSecurityLakeAsync} instead.
*
*
*
*
* Amazon Security Lake is in preview release. Your use of the Security Lake preview is subject to Section 2 of the Amazon Web Services Service Terms("Betas and Previews").
*
*
*
* Amazon Security Lake is a fully managed security data lake service. You can use Security Lake to automatically
* centralize security data from cloud, on-premises, and custom sources into a data lake that's stored in your Amazon
* Web Servicesaccount. Amazon Web Services Organizations is an account management service that lets you consolidate
* multiple Amazon Web Services accounts into an organization that you create and centrally manage. With Organizations,
* you can create member accounts and invite existing accounts to join your organization. Security Lake helps you
* analyze security data for a more complete understanding of your security posture across the entire organization. It
* can also help you improve the protection of your workloads, applications, and data.
*
*
* The data lake is backed by Amazon Simple Storage Service (Amazon S3) buckets, and you retain ownership over your
* data.
*
*
* Amazon Security Lake integrates with CloudTrail, a service that provides a record of actions taken by a user, role,
* or an Amazon Web Services service in Security Lake CloudTrail captures API calls for Security Lake as events. The
* calls captured include calls from the Security Lake console and code calls to the Security Lake API operations. If
* you create a trail, you can enable continuous delivery of CloudTrail events to an Amazon S3 bucket, including events
* for Security Lake. If you don't configure a trail, you can still view the most recent events in the CloudTrail
* console in Event history. Using the information collected by CloudTrail you can determine the request that was made
* to Security Lake, the IP address from which the request was made, who made the request, when it was made, and
* additional details. To learn more about Security Lake information in CloudTrail, see the Amazon Security Lake
* User Guide.
*
*
* Security Lake automates the collection of security-related log and event data from integrated Amazon Web Services and
* third-party services. It also helps you manage the lifecycle of data with customizable retention and replication
* settings. Security Lake converts ingested data into Apache Parquet format and a standard open-source schema called
* the Open Cybersecurity Schema Framework (OCSF).
*
*
* Other Amazon Web Services and third-party services can subscribe to the data that's stored in Security Lake for
* incident response and security data analytics.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonSecurityLakeAsync extends AmazonSecurityLake {
/**
*
* Adds a natively supported Amazon Web Service as an Amazon Security Lake source. Enables source types for member
* accounts in required Amazon Web Services Regions, based on the parameters you specify. You can choose any source
* type in any Region for either accounts that are part of a trusted organization or standalone accounts. At least
* one of the three dimensions is a mandatory input to this API. However, you can supply any combination of the
* three dimensions to this API.
*
*
* By default, a dimension refers to the entire set. When you don't provide a dimension, Security Lake assumes that
* the missing dimension refers to the entire set. This is overridden when you supply any one of the inputs. For
* instance, when you do not specify members, the API enables all Security Lake member accounts for all sources.
* Similarly, when you do not specify Regions, Security Lake is enabled for all the Regions where Security Lake is
* available as a service.
*
*
* You can use this API only to enable natively supported Amazon Web Services as a source. Use
* CreateCustomLogSource
to enable data collection from a custom source.
*
*
* @param createAwsLogSourceRequest
* @return A Java Future containing the result of the CreateAwsLogSource operation returned by the service.
* @sample AmazonSecurityLakeAsync.CreateAwsLogSource
* @see AWS API Documentation
*/
java.util.concurrent.Future createAwsLogSourceAsync(CreateAwsLogSourceRequest createAwsLogSourceRequest);
/**
*
* Adds a natively supported Amazon Web Service as an Amazon Security Lake source. Enables source types for member
* accounts in required Amazon Web Services Regions, based on the parameters you specify. You can choose any source
* type in any Region for either accounts that are part of a trusted organization or standalone accounts. At least
* one of the three dimensions is a mandatory input to this API. However, you can supply any combination of the
* three dimensions to this API.
*
*
* By default, a dimension refers to the entire set. When you don't provide a dimension, Security Lake assumes that
* the missing dimension refers to the entire set. This is overridden when you supply any one of the inputs. For
* instance, when you do not specify members, the API enables all Security Lake member accounts for all sources.
* Similarly, when you do not specify Regions, Security Lake is enabled for all the Regions where Security Lake is
* available as a service.
*
*
* You can use this API only to enable natively supported Amazon Web Services as a source. Use
* CreateCustomLogSource
to enable data collection from a custom source.
*
*
* @param createAwsLogSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAwsLogSource operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.CreateAwsLogSource
* @see AWS API Documentation
*/
java.util.concurrent.Future createAwsLogSourceAsync(CreateAwsLogSourceRequest createAwsLogSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a third-party custom source in Amazon Security Lake, from the Amazon Web Services Region where you want to
* create a custom source. Security Lake can collect logs and events from third-party custom sources. After creating
* the appropriate IAM role to invoke Glue crawler, use this API to add a custom source name in Security Lake. This
* operation creates a partition in the Amazon S3 bucket for Security Lake as the target location for log files from
* the custom source in addition to an associated Glue table and an Glue crawler.
*
*
* @param createCustomLogSourceRequest
* @return A Java Future containing the result of the CreateCustomLogSource operation returned by the service.
* @sample AmazonSecurityLakeAsync.CreateCustomLogSource
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomLogSourceAsync(CreateCustomLogSourceRequest createCustomLogSourceRequest);
/**
*
* Adds a third-party custom source in Amazon Security Lake, from the Amazon Web Services Region where you want to
* create a custom source. Security Lake can collect logs and events from third-party custom sources. After creating
* the appropriate IAM role to invoke Glue crawler, use this API to add a custom source name in Security Lake. This
* operation creates a partition in the Amazon S3 bucket for Security Lake as the target location for log files from
* the custom source in addition to an associated Glue table and an Glue crawler.
*
*
* @param createCustomLogSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomLogSource operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.CreateCustomLogSource
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomLogSourceAsync(CreateCustomLogSourceRequest createCustomLogSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initializes an Amazon Security Lake instance with the provided (or default) configuration. You can enable
* Security Lake in Amazon Web Services Regions with customized settings before enabling log collection in Regions.
* You can either use the enableAll
parameter to specify all Regions or specify the Regions where you
* want to enable Security Lake. To specify particular Regions, use the Regions
parameter and then
* configure these Regions using the configurations
parameter. If you have already enabled Security
* Lake in a Region when you call this command, the command will update the Region if you provide new configuration
* parameters. If you have not already enabled Security Lake in the Region when you call this API, it will set up
* the data lake in the Region with the specified configurations.
*
*
* When you enable Security Lake, it starts ingesting security data after the CreateAwsLogSource
call.
* This includes ingesting security data from sources, storing data, and making data accessible to subscribers.
* Security Lake also enables all the existing settings and resources that it stores or maintains for your Amazon
* Web Services account in the current Region, including security log and event data. For more information, see the
* Amazon Security
* Lake User Guide.
*
*
* @param createDatalakeRequest
* @return A Java Future containing the result of the CreateDatalake operation returned by the service.
* @sample AmazonSecurityLakeAsync.CreateDatalake
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDatalakeAsync(CreateDatalakeRequest createDatalakeRequest);
/**
*
* Initializes an Amazon Security Lake instance with the provided (or default) configuration. You can enable
* Security Lake in Amazon Web Services Regions with customized settings before enabling log collection in Regions.
* You can either use the enableAll
parameter to specify all Regions or specify the Regions where you
* want to enable Security Lake. To specify particular Regions, use the Regions
parameter and then
* configure these Regions using the configurations
parameter. If you have already enabled Security
* Lake in a Region when you call this command, the command will update the Region if you provide new configuration
* parameters. If you have not already enabled Security Lake in the Region when you call this API, it will set up
* the data lake in the Region with the specified configurations.
*
*
* When you enable Security Lake, it starts ingesting security data after the CreateAwsLogSource
call.
* This includes ingesting security data from sources, storing data, and making data accessible to subscribers.
* Security Lake also enables all the existing settings and resources that it stores or maintains for your Amazon
* Web Services account in the current Region, including security log and event data. For more information, see the
* Amazon Security
* Lake User Guide.
*
*
* @param createDatalakeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDatalake operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.CreateDatalake
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDatalakeAsync(CreateDatalakeRequest createDatalakeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Automatically enables Amazon Security Lake for new member accounts in your organization. Security Lake is not
* automatically enabled for any existing member accounts in your organization.
*
*
* @param createDatalakeAutoEnableRequest
* @return A Java Future containing the result of the CreateDatalakeAutoEnable operation returned by the service.
* @sample AmazonSecurityLakeAsync.CreateDatalakeAutoEnable
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatalakeAutoEnableAsync(CreateDatalakeAutoEnableRequest createDatalakeAutoEnableRequest);
/**
*
* Automatically enables Amazon Security Lake for new member accounts in your organization. Security Lake is not
* automatically enabled for any existing member accounts in your organization.
*
*
* @param createDatalakeAutoEnableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDatalakeAutoEnable operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.CreateDatalakeAutoEnable
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatalakeAutoEnableAsync(CreateDatalakeAutoEnableRequest createDatalakeAutoEnableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Designates the Amazon Security Lake delegated administrator account for the organization. This API can only be
* called by the organization management account. The organization management account cannot be the delegated
* administrator account.
*
*
* @param createDatalakeDelegatedAdminRequest
* @return A Java Future containing the result of the CreateDatalakeDelegatedAdmin operation returned by the
* service.
* @sample AmazonSecurityLakeAsync.CreateDatalakeDelegatedAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatalakeDelegatedAdminAsync(
CreateDatalakeDelegatedAdminRequest createDatalakeDelegatedAdminRequest);
/**
*
* Designates the Amazon Security Lake delegated administrator account for the organization. This API can only be
* called by the organization management account. The organization management account cannot be the delegated
* administrator account.
*
*
* @param createDatalakeDelegatedAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDatalakeDelegatedAdmin operation returned by the
* service.
* @sample AmazonSecurityLakeAsyncHandler.CreateDatalakeDelegatedAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatalakeDelegatedAdminAsync(
CreateDatalakeDelegatedAdminRequest createDatalakeDelegatedAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the specified notification subscription in Amazon Security Lake for the organization you specify.
*
*
* @param createDatalakeExceptionsSubscriptionRequest
* @return A Java Future containing the result of the CreateDatalakeExceptionsSubscription operation returned by the
* service.
* @sample AmazonSecurityLakeAsync.CreateDatalakeExceptionsSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatalakeExceptionsSubscriptionAsync(
CreateDatalakeExceptionsSubscriptionRequest createDatalakeExceptionsSubscriptionRequest);
/**
*
* Creates the specified notification subscription in Amazon Security Lake for the organization you specify.
*
*
* @param createDatalakeExceptionsSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDatalakeExceptionsSubscription operation returned by the
* service.
* @sample AmazonSecurityLakeAsyncHandler.CreateDatalakeExceptionsSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createDatalakeExceptionsSubscriptionAsync(
CreateDatalakeExceptionsSubscriptionRequest createDatalakeExceptionsSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a subscription permission for accounts that are already enabled in Amazon Security Lake. You can create a
* subscriber with access to data in the current Amazon Web Services Region.
*
*
* @param createSubscriberRequest
* @return A Java Future containing the result of the CreateSubscriber operation returned by the service.
* @sample AmazonSecurityLakeAsync.CreateSubscriber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSubscriberAsync(CreateSubscriberRequest createSubscriberRequest);
/**
*
* Creates a subscription permission for accounts that are already enabled in Amazon Security Lake. You can create a
* subscriber with access to data in the current Amazon Web Services Region.
*
*
* @param createSubscriberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSubscriber operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.CreateSubscriber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSubscriberAsync(CreateSubscriberRequest createSubscriberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Notifies the subscriber when new data is written to the data lake for the sources that the subscriber consumes in
* Security Lake.
*
*
* @param createSubscriptionNotificationConfigurationRequest
* @return A Java Future containing the result of the CreateSubscriptionNotificationConfiguration operation returned
* by the service.
* @sample AmazonSecurityLakeAsync.CreateSubscriptionNotificationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createSubscriptionNotificationConfigurationAsync(
CreateSubscriptionNotificationConfigurationRequest createSubscriptionNotificationConfigurationRequest);
/**
*
* Notifies the subscriber when new data is written to the data lake for the sources that the subscriber consumes in
* Security Lake.
*
*
* @param createSubscriptionNotificationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSubscriptionNotificationConfiguration operation returned
* by the service.
* @sample AmazonSecurityLakeAsyncHandler.CreateSubscriptionNotificationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createSubscriptionNotificationConfigurationAsync(
CreateSubscriptionNotificationConfigurationRequest createSubscriptionNotificationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a natively supported Amazon Web Service as an Amazon Security Lake source. When you remove the source,
* Security Lake stops collecting data from that source, and subscribers can no longer consume new data from the
* source. Subscribers can still consume data that Security Lake collected from the source before disablement.
*
*
* You can choose any source type in any Amazon Web Services Region for either accounts that are part of a trusted
* organization or standalone accounts. At least one of the three dimensions is a mandatory input to this API.
* However, you can supply any combination of the three dimensions to this API.
*
*
* By default, a dimension refers to the entire set. This is overridden when you supply any one of the inputs. For
* instance, when you do not specify members, the API disables all Security Lake member accounts for sources.
* Similarly, when you do not specify Regions, Security Lake is disabled for all the Regions where Security Lake is
* available as a service.
*
*
* When you don't provide a dimension, Security Lake assumes that the missing dimension refers to the entire set.
* For example, if you don't provide specific accounts, the API applies to the entire set of accounts in your
* organization.
*
*
* @param deleteAwsLogSourceRequest
* @return A Java Future containing the result of the DeleteAwsLogSource operation returned by the service.
* @sample AmazonSecurityLakeAsync.DeleteAwsLogSource
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAwsLogSourceAsync(DeleteAwsLogSourceRequest deleteAwsLogSourceRequest);
/**
*
* Removes a natively supported Amazon Web Service as an Amazon Security Lake source. When you remove the source,
* Security Lake stops collecting data from that source, and subscribers can no longer consume new data from the
* source. Subscribers can still consume data that Security Lake collected from the source before disablement.
*
*
* You can choose any source type in any Amazon Web Services Region for either accounts that are part of a trusted
* organization or standalone accounts. At least one of the three dimensions is a mandatory input to this API.
* However, you can supply any combination of the three dimensions to this API.
*
*
* By default, a dimension refers to the entire set. This is overridden when you supply any one of the inputs. For
* instance, when you do not specify members, the API disables all Security Lake member accounts for sources.
* Similarly, when you do not specify Regions, Security Lake is disabled for all the Regions where Security Lake is
* available as a service.
*
*
* When you don't provide a dimension, Security Lake assumes that the missing dimension refers to the entire set.
* For example, if you don't provide specific accounts, the API applies to the entire set of accounts in your
* organization.
*
*
* @param deleteAwsLogSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAwsLogSource operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.DeleteAwsLogSource
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAwsLogSourceAsync(DeleteAwsLogSourceRequest deleteAwsLogSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a custom log source from Amazon Security Lake.
*
*
* @param deleteCustomLogSourceRequest
* @return A Java Future containing the result of the DeleteCustomLogSource operation returned by the service.
* @sample AmazonSecurityLakeAsync.DeleteCustomLogSource
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomLogSourceAsync(DeleteCustomLogSourceRequest deleteCustomLogSourceRequest);
/**
*
* Removes a custom log source from Amazon Security Lake.
*
*
* @param deleteCustomLogSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCustomLogSource operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.DeleteCustomLogSource
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomLogSourceAsync(DeleteCustomLogSourceRequest deleteCustomLogSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* When you delete Amazon Security Lake from your account, Security Lake is disabled in all Amazon Web Services
* Regions. Also, this API automatically takes steps to remove the account from Security Lake .
*
*
* This operation disables security data collection from sources, deletes data stored, and stops making data
* accessible to subscribers. Security Lake also deletes all the existing settings and resources that it stores or
* maintains for your Amazon Web Services account in the current Region, including security log and event data. The
* DeleteDatalake
operation does not delete the Amazon S3 bucket, which is owned by your Amazon Web
* Services account. For more information, see the Amazon Security Lake
* User Guide.
*
*
* @param deleteDatalakeRequest
* @return A Java Future containing the result of the DeleteDatalake operation returned by the service.
* @sample AmazonSecurityLakeAsync.DeleteDatalake
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDatalakeAsync(DeleteDatalakeRequest deleteDatalakeRequest);
/**
*
* When you delete Amazon Security Lake from your account, Security Lake is disabled in all Amazon Web Services
* Regions. Also, this API automatically takes steps to remove the account from Security Lake .
*
*
* This operation disables security data collection from sources, deletes data stored, and stops making data
* accessible to subscribers. Security Lake also deletes all the existing settings and resources that it stores or
* maintains for your Amazon Web Services account in the current Region, including security log and event data. The
* DeleteDatalake
operation does not delete the Amazon S3 bucket, which is owned by your Amazon Web
* Services account. For more information, see the Amazon Security Lake
* User Guide.
*
*
* @param deleteDatalakeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDatalake operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.DeleteDatalake
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDatalakeAsync(DeleteDatalakeRequest deleteDatalakeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Automatically deletes Amazon Security Lake to stop collecting security data. When you delete Amazon Security Lake
* from your account, Security Lake is disabled in all Regions. Also, this API automatically takes steps to remove
* the account from Security Lake .
*
*
* This operation disables security data collection from sources, deletes data stored, and stops making data
* accessible to subscribers. Security Lake also deletes all the existing settings and resources that it stores or
* maintains for your Amazon Web Services account in the current Region, including security log and event data. The
* DeleteDatalake
operation does not delete the Amazon S3 bucket, which is owned by your Amazon Web
* Services account. For more information, see the Amazon Security Lake
* User Guide.
*
*
* @param deleteDatalakeAutoEnableRequest
* @return A Java Future containing the result of the DeleteDatalakeAutoEnable operation returned by the service.
* @sample AmazonSecurityLakeAsync.DeleteDatalakeAutoEnable
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatalakeAutoEnableAsync(DeleteDatalakeAutoEnableRequest deleteDatalakeAutoEnableRequest);
/**
*
* Automatically deletes Amazon Security Lake to stop collecting security data. When you delete Amazon Security Lake
* from your account, Security Lake is disabled in all Regions. Also, this API automatically takes steps to remove
* the account from Security Lake .
*
*
* This operation disables security data collection from sources, deletes data stored, and stops making data
* accessible to subscribers. Security Lake also deletes all the existing settings and resources that it stores or
* maintains for your Amazon Web Services account in the current Region, including security log and event data. The
* DeleteDatalake
operation does not delete the Amazon S3 bucket, which is owned by your Amazon Web
* Services account. For more information, see the Amazon Security Lake
* User Guide.
*
*
* @param deleteDatalakeAutoEnableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDatalakeAutoEnable operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.DeleteDatalakeAutoEnable
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatalakeAutoEnableAsync(DeleteDatalakeAutoEnableRequest deleteDatalakeAutoEnableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the Amazon Security Lake delegated administrator account for the organization. This API can only be
* called by the organization management account. The organization management account cannot be the delegated
* administrator account.
*
*
* @param deleteDatalakeDelegatedAdminRequest
* @return A Java Future containing the result of the DeleteDatalakeDelegatedAdmin operation returned by the
* service.
* @sample AmazonSecurityLakeAsync.DeleteDatalakeDelegatedAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatalakeDelegatedAdminAsync(
DeleteDatalakeDelegatedAdminRequest deleteDatalakeDelegatedAdminRequest);
/**
*
* Deletes the Amazon Security Lake delegated administrator account for the organization. This API can only be
* called by the organization management account. The organization management account cannot be the delegated
* administrator account.
*
*
* @param deleteDatalakeDelegatedAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDatalakeDelegatedAdmin operation returned by the
* service.
* @sample AmazonSecurityLakeAsyncHandler.DeleteDatalakeDelegatedAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatalakeDelegatedAdminAsync(
DeleteDatalakeDelegatedAdminRequest deleteDatalakeDelegatedAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified notification subscription in Amazon Security Lake for the organization you specify.
*
*
* @param deleteDatalakeExceptionsSubscriptionRequest
* @return A Java Future containing the result of the DeleteDatalakeExceptionsSubscription operation returned by the
* service.
* @sample AmazonSecurityLakeAsync.DeleteDatalakeExceptionsSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatalakeExceptionsSubscriptionAsync(
DeleteDatalakeExceptionsSubscriptionRequest deleteDatalakeExceptionsSubscriptionRequest);
/**
*
* Deletes the specified notification subscription in Amazon Security Lake for the organization you specify.
*
*
* @param deleteDatalakeExceptionsSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDatalakeExceptionsSubscription operation returned by the
* service.
* @sample AmazonSecurityLakeAsyncHandler.DeleteDatalakeExceptionsSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDatalakeExceptionsSubscriptionAsync(
DeleteDatalakeExceptionsSubscriptionRequest deleteDatalakeExceptionsSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the subscription permission for accounts that are already enabled in Amazon Security Lake. You can delete
* a subscriber and remove access to data in the current Amazon Web Services Region.
*
*
* @param deleteSubscriberRequest
* @return A Java Future containing the result of the DeleteSubscriber operation returned by the service.
* @sample AmazonSecurityLakeAsync.DeleteSubscriber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteSubscriberAsync(DeleteSubscriberRequest deleteSubscriberRequest);
/**
*
* Deletes the subscription permission for accounts that are already enabled in Amazon Security Lake. You can delete
* a subscriber and remove access to data in the current Amazon Web Services Region.
*
*
* @param deleteSubscriberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSubscriber operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.DeleteSubscriber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteSubscriberAsync(DeleteSubscriberRequest deleteSubscriberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified notification subscription in Amazon Security Lake for the organization you specify.
*
*
* @param deleteSubscriptionNotificationConfigurationRequest
* @return A Java Future containing the result of the DeleteSubscriptionNotificationConfiguration operation returned
* by the service.
* @sample AmazonSecurityLakeAsync.DeleteSubscriptionNotificationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSubscriptionNotificationConfigurationAsync(
DeleteSubscriptionNotificationConfigurationRequest deleteSubscriptionNotificationConfigurationRequest);
/**
*
* Deletes the specified notification subscription in Amazon Security Lake for the organization you specify.
*
*
* @param deleteSubscriptionNotificationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSubscriptionNotificationConfiguration operation returned
* by the service.
* @sample AmazonSecurityLakeAsyncHandler.DeleteSubscriptionNotificationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSubscriptionNotificationConfigurationAsync(
DeleteSubscriptionNotificationConfigurationRequest deleteSubscriptionNotificationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the Amazon Security Lake configuration object for the specified Amazon Web Services account ID. You can
* use the GetDatalake
API to know whether Security Lake is enabled for the current Region. This API
* does not take input parameters.
*
*
* @param getDatalakeRequest
* @return A Java Future containing the result of the GetDatalake operation returned by the service.
* @sample AmazonSecurityLakeAsync.GetDatalake
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDatalakeAsync(GetDatalakeRequest getDatalakeRequest);
/**
*
* Retrieves the Amazon Security Lake configuration object for the specified Amazon Web Services account ID. You can
* use the GetDatalake
API to know whether Security Lake is enabled for the current Region. This API
* does not take input parameters.
*
*
* @param getDatalakeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDatalake operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.GetDatalake
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDatalakeAsync(GetDatalakeRequest getDatalakeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the configuration that will be automatically set up for accounts added to the organization after the
* organization has onboarded to Amazon Security Lake. This API does not take input parameters.
*
*
* @param getDatalakeAutoEnableRequest
* @return A Java Future containing the result of the GetDatalakeAutoEnable operation returned by the service.
* @sample AmazonSecurityLakeAsync.GetDatalakeAutoEnable
* @see AWS API Documentation
*/
java.util.concurrent.Future getDatalakeAutoEnableAsync(GetDatalakeAutoEnableRequest getDatalakeAutoEnableRequest);
/**
*
* Retrieves the configuration that will be automatically set up for accounts added to the organization after the
* organization has onboarded to Amazon Security Lake. This API does not take input parameters.
*
*
* @param getDatalakeAutoEnableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDatalakeAutoEnable operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.GetDatalakeAutoEnable
* @see AWS API Documentation
*/
java.util.concurrent.Future getDatalakeAutoEnableAsync(GetDatalakeAutoEnableRequest getDatalakeAutoEnableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the expiration period and time-to-live (TTL) for which the exception message will remain. Exceptions
* are stored by default, for 2 weeks from when a record was created in Amazon Security Lake. This API does not take
* input parameters.
*
*
* @param getDatalakeExceptionsExpiryRequest
* @return A Java Future containing the result of the GetDatalakeExceptionsExpiry operation returned by the service.
* @sample AmazonSecurityLakeAsync.GetDatalakeExceptionsExpiry
* @see AWS API Documentation
*/
java.util.concurrent.Future getDatalakeExceptionsExpiryAsync(
GetDatalakeExceptionsExpiryRequest getDatalakeExceptionsExpiryRequest);
/**
*
* Retrieves the expiration period and time-to-live (TTL) for which the exception message will remain. Exceptions
* are stored by default, for 2 weeks from when a record was created in Amazon Security Lake. This API does not take
* input parameters.
*
*
* @param getDatalakeExceptionsExpiryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDatalakeExceptionsExpiry operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.GetDatalakeExceptionsExpiry
* @see AWS API Documentation
*/
java.util.concurrent.Future getDatalakeExceptionsExpiryAsync(
GetDatalakeExceptionsExpiryRequest getDatalakeExceptionsExpiryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the details of exception notifications for the account in Amazon Security Lake.
*
*
* @param getDatalakeExceptionsSubscriptionRequest
* @return A Java Future containing the result of the GetDatalakeExceptionsSubscription operation returned by the
* service.
* @sample AmazonSecurityLakeAsync.GetDatalakeExceptionsSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future getDatalakeExceptionsSubscriptionAsync(
GetDatalakeExceptionsSubscriptionRequest getDatalakeExceptionsSubscriptionRequest);
/**
*
* Retrieves the details of exception notifications for the account in Amazon Security Lake.
*
*
* @param getDatalakeExceptionsSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDatalakeExceptionsSubscription operation returned by the
* service.
* @sample AmazonSecurityLakeAsyncHandler.GetDatalakeExceptionsSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future getDatalakeExceptionsSubscriptionAsync(
GetDatalakeExceptionsSubscriptionRequest getDatalakeExceptionsSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a snapshot of the current Region, including whether Amazon Security Lake is enabled for those accounts
* and which sources Security Lake is collecting data from.
*
*
* @param getDatalakeStatusRequest
* @return A Java Future containing the result of the GetDatalakeStatus operation returned by the service.
* @sample AmazonSecurityLakeAsync.GetDatalakeStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDatalakeStatusAsync(GetDatalakeStatusRequest getDatalakeStatusRequest);
/**
*
* Retrieves a snapshot of the current Region, including whether Amazon Security Lake is enabled for those accounts
* and which sources Security Lake is collecting data from.
*
*
* @param getDatalakeStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDatalakeStatus operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.GetDatalakeStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDatalakeStatusAsync(GetDatalakeStatusRequest getDatalakeStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the subscription information for the specified subscription ID. You can get information about a
* specific subscriber.
*
*
* @param getSubscriberRequest
* @return A Java Future containing the result of the GetSubscriber operation returned by the service.
* @sample AmazonSecurityLakeAsync.GetSubscriber
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSubscriberAsync(GetSubscriberRequest getSubscriberRequest);
/**
*
* Retrieves the subscription information for the specified subscription ID. You can get information about a
* specific subscriber.
*
*
* @param getSubscriberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSubscriber operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.GetSubscriber
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSubscriberAsync(GetSubscriberRequest getSubscriberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Amazon Security Lake exceptions that you can use to find the source of problems and fix them.
*
*
* @param listDatalakeExceptionsRequest
* @return A Java Future containing the result of the ListDatalakeExceptions operation returned by the service.
* @sample AmazonSecurityLakeAsync.ListDatalakeExceptions
* @see AWS API Documentation
*/
java.util.concurrent.Future listDatalakeExceptionsAsync(ListDatalakeExceptionsRequest listDatalakeExceptionsRequest);
/**
*
* Lists the Amazon Security Lake exceptions that you can use to find the source of problems and fix them.
*
*
* @param listDatalakeExceptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDatalakeExceptions operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.ListDatalakeExceptions
* @see AWS API Documentation
*/
java.util.concurrent.Future listDatalakeExceptionsAsync(ListDatalakeExceptionsRequest listDatalakeExceptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the log sources in the current Amazon Web Services Region.
*
*
* @param listLogSourcesRequest
* @return A Java Future containing the result of the ListLogSources operation returned by the service.
* @sample AmazonSecurityLakeAsync.ListLogSources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLogSourcesAsync(ListLogSourcesRequest listLogSourcesRequest);
/**
*
* Retrieves the log sources in the current Amazon Web Services Region.
*
*
* @param listLogSourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLogSources operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.ListLogSources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLogSourcesAsync(ListLogSourcesRequest listLogSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all subscribers for the specific Amazon Security Lake account ID. You can retrieve a list of subscriptions
* associated with a specific organization or Amazon Web Services account.
*
*
* @param listSubscribersRequest
* @return A Java Future containing the result of the ListSubscribers operation returned by the service.
* @sample AmazonSecurityLakeAsync.ListSubscribers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSubscribersAsync(ListSubscribersRequest listSubscribersRequest);
/**
*
* List all subscribers for the specific Amazon Security Lake account ID. You can retrieve a list of subscriptions
* associated with a specific organization or Amazon Web Services account.
*
*
* @param listSubscribersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSubscribers operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.ListSubscribers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSubscribersAsync(ListSubscribersRequest listSubscribersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specifies where to store your security data and for how long. You can add a rollup Region to consolidate data
* from multiple Amazon Web Services Regions.
*
*
* @param updateDatalakeRequest
* @return A Java Future containing the result of the UpdateDatalake operation returned by the service.
* @sample AmazonSecurityLakeAsync.UpdateDatalake
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDatalakeAsync(UpdateDatalakeRequest updateDatalakeRequest);
/**
*
* Specifies where to store your security data and for how long. You can add a rollup Region to consolidate data
* from multiple Amazon Web Services Regions.
*
*
* @param updateDatalakeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDatalake operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.UpdateDatalake
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDatalakeAsync(UpdateDatalakeRequest updateDatalakeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update the expiration period for the exception message to your preferred time, and control the time-to-live (TTL)
* for the exception message to remain. Exceptions are stored by default for 2 weeks from when a record was created
* in Amazon Security Lake.
*
*
* @param updateDatalakeExceptionsExpiryRequest
* @return A Java Future containing the result of the UpdateDatalakeExceptionsExpiry operation returned by the
* service.
* @sample AmazonSecurityLakeAsync.UpdateDatalakeExceptionsExpiry
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDatalakeExceptionsExpiryAsync(
UpdateDatalakeExceptionsExpiryRequest updateDatalakeExceptionsExpiryRequest);
/**
*
* Update the expiration period for the exception message to your preferred time, and control the time-to-live (TTL)
* for the exception message to remain. Exceptions are stored by default for 2 weeks from when a record was created
* in Amazon Security Lake.
*
*
* @param updateDatalakeExceptionsExpiryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDatalakeExceptionsExpiry operation returned by the
* service.
* @sample AmazonSecurityLakeAsyncHandler.UpdateDatalakeExceptionsExpiry
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDatalakeExceptionsExpiryAsync(
UpdateDatalakeExceptionsExpiryRequest updateDatalakeExceptionsExpiryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified notification subscription in Amazon Security Lake for the organization you specify.
*
*
* @param updateDatalakeExceptionsSubscriptionRequest
* @return A Java Future containing the result of the UpdateDatalakeExceptionsSubscription operation returned by the
* service.
* @sample AmazonSecurityLakeAsync.UpdateDatalakeExceptionsSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDatalakeExceptionsSubscriptionAsync(
UpdateDatalakeExceptionsSubscriptionRequest updateDatalakeExceptionsSubscriptionRequest);
/**
*
* Updates the specified notification subscription in Amazon Security Lake for the organization you specify.
*
*
* @param updateDatalakeExceptionsSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDatalakeExceptionsSubscription operation returned by the
* service.
* @sample AmazonSecurityLakeAsyncHandler.UpdateDatalakeExceptionsSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDatalakeExceptionsSubscriptionAsync(
UpdateDatalakeExceptionsSubscriptionRequest updateDatalakeExceptionsSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing subscription for the given Amazon Security Lake account ID. You can update a subscriber by
* changing the sources that the subscriber consumes data from.
*
*
* @param updateSubscriberRequest
* @return A Java Future containing the result of the UpdateSubscriber operation returned by the service.
* @sample AmazonSecurityLakeAsync.UpdateSubscriber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateSubscriberAsync(UpdateSubscriberRequest updateSubscriberRequest);
/**
*
* Updates an existing subscription for the given Amazon Security Lake account ID. You can update a subscriber by
* changing the sources that the subscriber consumes data from.
*
*
* @param updateSubscriberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSubscriber operation returned by the service.
* @sample AmazonSecurityLakeAsyncHandler.UpdateSubscriber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateSubscriberAsync(UpdateSubscriberRequest updateSubscriberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new subscription notification or adds the existing subscription notification setting for the specified
* subscription ID.
*
*
* @param updateSubscriptionNotificationConfigurationRequest
* @return A Java Future containing the result of the UpdateSubscriptionNotificationConfiguration operation returned
* by the service.
* @sample AmazonSecurityLakeAsync.UpdateSubscriptionNotificationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSubscriptionNotificationConfigurationAsync(
UpdateSubscriptionNotificationConfigurationRequest updateSubscriptionNotificationConfigurationRequest);
/**
*
* Creates a new subscription notification or adds the existing subscription notification setting for the specified
* subscription ID.
*
*
* @param updateSubscriptionNotificationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSubscriptionNotificationConfiguration operation returned
* by the service.
* @sample AmazonSecurityLakeAsyncHandler.UpdateSubscriptionNotificationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSubscriptionNotificationConfigurationAsync(
UpdateSubscriptionNotificationConfigurationRequest updateSubscriptionNotificationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}