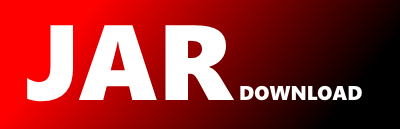
com.amazonaws.services.securitylake.model.CreateAwsLogSourceRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-securitylake Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.securitylake.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAwsLogSourceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Enables data collection from specific Amazon Web Services sources in all specific accounts and specific Regions.
*
*/
private java.util.Map>> enableAllDimensions;
/**
*
* Enables data collection from all Amazon Web Services sources in specific accounts or Regions.
*
*/
private java.util.List enableSingleDimension;
/**
*
* Enables data collection from specific Amazon Web Services sources in specific accounts or Regions.
*
*/
private java.util.Map> enableTwoDimensions;
/**
*
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member account.
*
*/
private java.util.List inputOrder;
/**
*
* Enables data collection from specific Amazon Web Services sources in all specific accounts and specific Regions.
*
*
* @return Enables data collection from specific Amazon Web Services sources in all specific accounts and specific
* Regions.
*/
public java.util.Map>> getEnableAllDimensions() {
return enableAllDimensions;
}
/**
*
* Enables data collection from specific Amazon Web Services sources in all specific accounts and specific Regions.
*
*
* @param enableAllDimensions
* Enables data collection from specific Amazon Web Services sources in all specific accounts and specific
* Regions.
*/
public void setEnableAllDimensions(java.util.Map>> enableAllDimensions) {
this.enableAllDimensions = enableAllDimensions;
}
/**
*
* Enables data collection from specific Amazon Web Services sources in all specific accounts and specific Regions.
*
*
* @param enableAllDimensions
* Enables data collection from specific Amazon Web Services sources in all specific accounts and specific
* Regions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAwsLogSourceRequest withEnableAllDimensions(java.util.Map>> enableAllDimensions) {
setEnableAllDimensions(enableAllDimensions);
return this;
}
/**
* Add a single EnableAllDimensions entry
*
* @see CreateAwsLogSourceRequest#withEnableAllDimensions
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateAwsLogSourceRequest addEnableAllDimensionsEntry(String key, java.util.Map> value) {
if (null == this.enableAllDimensions) {
this.enableAllDimensions = new java.util.HashMap>>();
}
if (this.enableAllDimensions.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.enableAllDimensions.put(key, value);
return this;
}
/**
* Removes all the entries added into EnableAllDimensions.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAwsLogSourceRequest clearEnableAllDimensionsEntries() {
this.enableAllDimensions = null;
return this;
}
/**
*
* Enables data collection from all Amazon Web Services sources in specific accounts or Regions.
*
*
* @return Enables data collection from all Amazon Web Services sources in specific accounts or Regions.
*/
public java.util.List getEnableSingleDimension() {
return enableSingleDimension;
}
/**
*
* Enables data collection from all Amazon Web Services sources in specific accounts or Regions.
*
*
* @param enableSingleDimension
* Enables data collection from all Amazon Web Services sources in specific accounts or Regions.
*/
public void setEnableSingleDimension(java.util.Collection enableSingleDimension) {
if (enableSingleDimension == null) {
this.enableSingleDimension = null;
return;
}
this.enableSingleDimension = new java.util.ArrayList(enableSingleDimension);
}
/**
*
* Enables data collection from all Amazon Web Services sources in specific accounts or Regions.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEnableSingleDimension(java.util.Collection)} or
* {@link #withEnableSingleDimension(java.util.Collection)} if you want to override the existing values.
*
*
* @param enableSingleDimension
* Enables data collection from all Amazon Web Services sources in specific accounts or Regions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAwsLogSourceRequest withEnableSingleDimension(String... enableSingleDimension) {
if (this.enableSingleDimension == null) {
setEnableSingleDimension(new java.util.ArrayList(enableSingleDimension.length));
}
for (String ele : enableSingleDimension) {
this.enableSingleDimension.add(ele);
}
return this;
}
/**
*
* Enables data collection from all Amazon Web Services sources in specific accounts or Regions.
*
*
* @param enableSingleDimension
* Enables data collection from all Amazon Web Services sources in specific accounts or Regions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAwsLogSourceRequest withEnableSingleDimension(java.util.Collection enableSingleDimension) {
setEnableSingleDimension(enableSingleDimension);
return this;
}
/**
*
* Enables data collection from specific Amazon Web Services sources in specific accounts or Regions.
*
*
* @return Enables data collection from specific Amazon Web Services sources in specific accounts or Regions.
*/
public java.util.Map> getEnableTwoDimensions() {
return enableTwoDimensions;
}
/**
*
* Enables data collection from specific Amazon Web Services sources in specific accounts or Regions.
*
*
* @param enableTwoDimensions
* Enables data collection from specific Amazon Web Services sources in specific accounts or Regions.
*/
public void setEnableTwoDimensions(java.util.Map> enableTwoDimensions) {
this.enableTwoDimensions = enableTwoDimensions;
}
/**
*
* Enables data collection from specific Amazon Web Services sources in specific accounts or Regions.
*
*
* @param enableTwoDimensions
* Enables data collection from specific Amazon Web Services sources in specific accounts or Regions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAwsLogSourceRequest withEnableTwoDimensions(java.util.Map> enableTwoDimensions) {
setEnableTwoDimensions(enableTwoDimensions);
return this;
}
/**
* Add a single EnableTwoDimensions entry
*
* @see CreateAwsLogSourceRequest#withEnableTwoDimensions
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateAwsLogSourceRequest addEnableTwoDimensionsEntry(String key, java.util.List value) {
if (null == this.enableTwoDimensions) {
this.enableTwoDimensions = new java.util.HashMap>();
}
if (this.enableTwoDimensions.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.enableTwoDimensions.put(key, value);
return this;
}
/**
* Removes all the entries added into EnableTwoDimensions.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAwsLogSourceRequest clearEnableTwoDimensionsEntries() {
this.enableTwoDimensions = null;
return this;
}
/**
*
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member account.
*
*
* @return Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member
* account.
* @see Dimension
*/
public java.util.List getInputOrder() {
return inputOrder;
}
/**
*
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member account.
*
*
* @param inputOrder
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member
* account.
* @see Dimension
*/
public void setInputOrder(java.util.Collection inputOrder) {
if (inputOrder == null) {
this.inputOrder = null;
return;
}
this.inputOrder = new java.util.ArrayList(inputOrder);
}
/**
*
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member account.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInputOrder(java.util.Collection)} or {@link #withInputOrder(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param inputOrder
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member
* account.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Dimension
*/
public CreateAwsLogSourceRequest withInputOrder(String... inputOrder) {
if (this.inputOrder == null) {
setInputOrder(new java.util.ArrayList(inputOrder.length));
}
for (String ele : inputOrder) {
this.inputOrder.add(ele);
}
return this;
}
/**
*
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member account.
*
*
* @param inputOrder
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member
* account.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Dimension
*/
public CreateAwsLogSourceRequest withInputOrder(java.util.Collection inputOrder) {
setInputOrder(inputOrder);
return this;
}
/**
*
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member account.
*
*
* @param inputOrder
* Specifies the input order to enable dimensions in Security Lake, namely Region, source type, and member
* account.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Dimension
*/
public CreateAwsLogSourceRequest withInputOrder(Dimension... inputOrder) {
java.util.ArrayList inputOrderCopy = new java.util.ArrayList(inputOrder.length);
for (Dimension value : inputOrder) {
inputOrderCopy.add(value.toString());
}
if (getInputOrder() == null) {
setInputOrder(inputOrderCopy);
} else {
getInputOrder().addAll(inputOrderCopy);
}
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEnableAllDimensions() != null)
sb.append("EnableAllDimensions: ").append(getEnableAllDimensions()).append(",");
if (getEnableSingleDimension() != null)
sb.append("EnableSingleDimension: ").append(getEnableSingleDimension()).append(",");
if (getEnableTwoDimensions() != null)
sb.append("EnableTwoDimensions: ").append(getEnableTwoDimensions()).append(",");
if (getInputOrder() != null)
sb.append("InputOrder: ").append(getInputOrder());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAwsLogSourceRequest == false)
return false;
CreateAwsLogSourceRequest other = (CreateAwsLogSourceRequest) obj;
if (other.getEnableAllDimensions() == null ^ this.getEnableAllDimensions() == null)
return false;
if (other.getEnableAllDimensions() != null && other.getEnableAllDimensions().equals(this.getEnableAllDimensions()) == false)
return false;
if (other.getEnableSingleDimension() == null ^ this.getEnableSingleDimension() == null)
return false;
if (other.getEnableSingleDimension() != null && other.getEnableSingleDimension().equals(this.getEnableSingleDimension()) == false)
return false;
if (other.getEnableTwoDimensions() == null ^ this.getEnableTwoDimensions() == null)
return false;
if (other.getEnableTwoDimensions() != null && other.getEnableTwoDimensions().equals(this.getEnableTwoDimensions()) == false)
return false;
if (other.getInputOrder() == null ^ this.getInputOrder() == null)
return false;
if (other.getInputOrder() != null && other.getInputOrder().equals(this.getInputOrder()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEnableAllDimensions() == null) ? 0 : getEnableAllDimensions().hashCode());
hashCode = prime * hashCode + ((getEnableSingleDimension() == null) ? 0 : getEnableSingleDimension().hashCode());
hashCode = prime * hashCode + ((getEnableTwoDimensions() == null) ? 0 : getEnableTwoDimensions().hashCode());
hashCode = prime * hashCode + ((getInputOrder() == null) ? 0 : getInputOrder().hashCode());
return hashCode;
}
@Override
public CreateAwsLogSourceRequest clone() {
return (CreateAwsLogSourceRequest) super.clone();
}
}