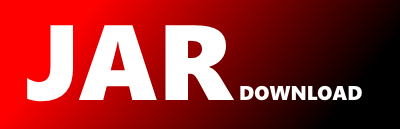
com.amazonaws.services.serverlessapplicationrepository.AWSServerlessApplicationRepositoryAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-serverlessapplicationrepository Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.serverlessapplicationrepository;
import javax.annotation.Generated;
import com.amazonaws.services.serverlessapplicationrepository.model.*;
/**
* Interface for accessing AWSServerlessApplicationRepository asynchronously. Each asynchronous method will return a
* Java Future object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be
* used to receive notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.serverlessapplicationrepository.AbstractAWSServerlessApplicationRepositoryAsync}
* instead.
*
*
*
* The AWS Serverless Application Repository makes it easy for developers and enterprises to quickly find and deploy
* serverless applications in the AWS Cloud. For more information about serverless applications, see Serverless
* Computing and Applications on the AWS website.
*
*
* The AWS Serverless Application Repository is deeply integrated with the AWS Lambda console, so that developers of all
* levels can get started with serverless computing without needing to learn anything new. You can use category keywords
* to browse for applications such as web and mobile backends, data processing applications, or chatbots. You can also
* search for applications by name, publisher, or event source. To use an application, you simply choose it, configure
* any required fields, and deploy it with a few clicks.
*
*
* You can also easily publish applications, sharing them publicly with the community at large, or privately within your
* team or across your organization. To publish a serverless application (or app), you can use the AWS Management
* Console, AWS Command Line Interface (AWS CLI), or AWS SDKs to upload the code. Along with the code, you upload a
* simple manifest file, also known as the AWS Serverless Application Model (AWS SAM) template. For more information
* about AWS SAM, see AWS Serverless Application Model (AWS SAM) on the AWS Labs GitHub repository.
*
*
* The AWS Serverless Application Repository Developer Guide contains more information about the two developer
* experiences available:
*
*
* -
*
* Consuming Applications – Browse for applications and view information about them, including source code and readme
* files. Also install, configure, and deploy applications of your choosing.
*
*
* Publishing Applications – Configure and upload applications to make them available to other developers, and publish
* new versions of applications.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSServerlessApplicationRepositoryAsync extends AWSServerlessApplicationRepository {
/**
*
* Creates an application, optionally including an AWS SAM file to create the first application version in the same
* call.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.CreateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest);
/**
*
* Creates an application, optionally including an AWS SAM file to create the first application version in the same
* call.
*
*
* @param createApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.CreateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an application version.
*
*
* @param createApplicationVersionRequest
* @return A Java Future containing the result of the CreateApplicationVersion operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.CreateApplicationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationVersionAsync(CreateApplicationVersionRequest createApplicationVersionRequest);
/**
*
* Creates an application version.
*
*
* @param createApplicationVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApplicationVersion operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.CreateApplicationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationVersionAsync(CreateApplicationVersionRequest createApplicationVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an AWS CloudFormation change set for the given application.
*
*
* @param createCloudFormationChangeSetRequest
* @return A Java Future containing the result of the CreateCloudFormationChangeSet operation returned by the
* service.
* @sample AWSServerlessApplicationRepositoryAsync.CreateCloudFormationChangeSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createCloudFormationChangeSetAsync(
CreateCloudFormationChangeSetRequest createCloudFormationChangeSetRequest);
/**
*
* Creates an AWS CloudFormation change set for the given application.
*
*
* @param createCloudFormationChangeSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCloudFormationChangeSet operation returned by the
* service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.CreateCloudFormationChangeSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createCloudFormationChangeSetAsync(
CreateCloudFormationChangeSetRequest createCloudFormationChangeSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an AWS CloudFormation template.
*
*
* @param createCloudFormationTemplateRequest
* @return A Java Future containing the result of the CreateCloudFormationTemplate operation returned by the
* service.
* @sample AWSServerlessApplicationRepositoryAsync.CreateCloudFormationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createCloudFormationTemplateAsync(
CreateCloudFormationTemplateRequest createCloudFormationTemplateRequest);
/**
*
* Creates an AWS CloudFormation template.
*
*
* @param createCloudFormationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCloudFormationTemplate operation returned by the
* service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.CreateCloudFormationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createCloudFormationTemplateAsync(
CreateCloudFormationTemplateRequest createCloudFormationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified application.
*
*
* @param deleteApplicationRequest
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.DeleteApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Deletes the specified application.
*
*
* @param deleteApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.DeleteApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the specified application.
*
*
* @param getApplicationRequest
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.GetApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getApplicationAsync(GetApplicationRequest getApplicationRequest);
/**
*
* Gets the specified application.
*
*
* @param getApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.GetApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getApplicationAsync(GetApplicationRequest getApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the policy for the application.
*
*
* @param getApplicationPolicyRequest
* @return A Java Future containing the result of the GetApplicationPolicy operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.GetApplicationPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getApplicationPolicyAsync(GetApplicationPolicyRequest getApplicationPolicyRequest);
/**
*
* Retrieves the policy for the application.
*
*
* @param getApplicationPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetApplicationPolicy operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.GetApplicationPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getApplicationPolicyAsync(GetApplicationPolicyRequest getApplicationPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the specified AWS CloudFormation template.
*
*
* @param getCloudFormationTemplateRequest
* @return A Java Future containing the result of the GetCloudFormationTemplate operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.GetCloudFormationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFormationTemplateAsync(
GetCloudFormationTemplateRequest getCloudFormationTemplateRequest);
/**
*
* Gets the specified AWS CloudFormation template.
*
*
* @param getCloudFormationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCloudFormationTemplate operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.GetCloudFormationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getCloudFormationTemplateAsync(
GetCloudFormationTemplateRequest getCloudFormationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the list of applications nested in the containing application.
*
*
* @param listApplicationDependenciesRequest
* @return A Java Future containing the result of the ListApplicationDependencies operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.ListApplicationDependencies
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationDependenciesAsync(
ListApplicationDependenciesRequest listApplicationDependenciesRequest);
/**
*
* Retrieves the list of applications nested in the containing application.
*
*
* @param listApplicationDependenciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplicationDependencies operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.ListApplicationDependencies
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationDependenciesAsync(
ListApplicationDependenciesRequest listApplicationDependenciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists versions for the specified application.
*
*
* @param listApplicationVersionsRequest
* @return A Java Future containing the result of the ListApplicationVersions operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.ListApplicationVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationVersionsAsync(ListApplicationVersionsRequest listApplicationVersionsRequest);
/**
*
* Lists versions for the specified application.
*
*
* @param listApplicationVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplicationVersions operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.ListApplicationVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationVersionsAsync(ListApplicationVersionsRequest listApplicationVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists applications owned by the requester.
*
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.ListApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest);
/**
*
* Lists applications owned by the requester.
*
*
* @param listApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.ListApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the permission policy for an application. For the list of actions supported for this operation, see Application Permissions .
*
*
* @param putApplicationPolicyRequest
* @return A Java Future containing the result of the PutApplicationPolicy operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.PutApplicationPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putApplicationPolicyAsync(PutApplicationPolicyRequest putApplicationPolicyRequest);
/**
*
* Sets the permission policy for an application. For the list of actions supported for this operation, see Application Permissions .
*
*
* @param putApplicationPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutApplicationPolicy operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.PutApplicationPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putApplicationPolicyAsync(PutApplicationPolicyRequest putApplicationPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified application.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsync.UpdateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Updates the specified application.
*
*
* @param updateApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AWSServerlessApplicationRepositoryAsyncHandler.UpdateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}