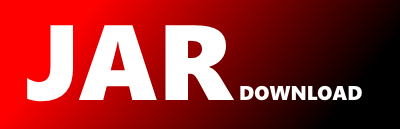
com.amazonaws.services.serverlessapplicationrepository.model.CreateApplicationResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-serverlessapplicationrepository Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.serverlessapplicationrepository.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateApplicationResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The application Amazon Resource Name (ARN).
*
*/
private String applicationId;
/**
*
* The name of the author publishing the app.
*
*
* Minimum length=1. Maximum length=127.
*
*
* Pattern "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
*/
private String author;
/**
*
* The date and time this resource was created.
*
*/
private String creationTime;
/**
*
* The description of the application.
*
*
* Minimum length=1. Maximum length=256
*
*/
private String description;
/**
*
* A URL with more information about the application, for example the location of your GitHub repository for the
* application.
*
*/
private String homePageUrl;
/**
*
* Whether the author of this application has been verified. This means means that AWS has made a good faith review,
* as a reasonable and prudent service provider, of the information provided by the requester and has confirmed that
* the requester's identity is as claimed.
*
*/
private Boolean isVerifiedAuthor;
/**
*
* Labels to improve discovery of apps in search results.
*
*
* Minimum length=1. Maximum length=127. Maximum number of labels: 10
*
*
* Pattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
*/
private java.util.List labels;
/**
*
* A link to a license file of the app that matches the spdxLicenseID value of your application.
*
*
* Maximum size 5 MB
*
*/
private String licenseUrl;
/**
*
* The name of the application.
*
*
* Minimum length=1. Maximum length=140
*
*
* Pattern: "[a-zA-Z0-9\\-]+";
*
*/
private String name;
/**
*
* A link to the readme file in Markdown language that contains a more detailed description of the application and
* how it works.
*
*
* Maximum size 5 MB
*
*/
private String readmeUrl;
/**
*
* A valid identifier from https://spdx.org/licenses/.
*
*/
private String spdxLicenseId;
/**
*
* The URL to the public profile of a verified author. This URL is submitted by the author.
*
*/
private String verifiedAuthorUrl;
/**
*
* Version information about the application.
*
*/
private Version version;
/**
*
* The application Amazon Resource Name (ARN).
*
*
* @param applicationId
* The application Amazon Resource Name (ARN).
*/
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
/**
*
* The application Amazon Resource Name (ARN).
*
*
* @return The application Amazon Resource Name (ARN).
*/
public String getApplicationId() {
return this.applicationId;
}
/**
*
* The application Amazon Resource Name (ARN).
*
*
* @param applicationId
* The application Amazon Resource Name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withApplicationId(String applicationId) {
setApplicationId(applicationId);
return this;
}
/**
*
* The name of the author publishing the app.
*
*
* Minimum length=1. Maximum length=127.
*
*
* Pattern "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
*
* @param author
* The name of the author publishing the app.
*
* Minimum length=1. Maximum length=127.
*
*
* Pattern "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*/
public void setAuthor(String author) {
this.author = author;
}
/**
*
* The name of the author publishing the app.
*
*
* Minimum length=1. Maximum length=127.
*
*
* Pattern "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
*
* @return The name of the author publishing the app.
*
* Minimum length=1. Maximum length=127.
*
*
* Pattern "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*/
public String getAuthor() {
return this.author;
}
/**
*
* The name of the author publishing the app.
*
*
* Minimum length=1. Maximum length=127.
*
*
* Pattern "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
*
* @param author
* The name of the author publishing the app.
*
* Minimum length=1. Maximum length=127.
*
*
* Pattern "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withAuthor(String author) {
setAuthor(author);
return this;
}
/**
*
* The date and time this resource was created.
*
*
* @param creationTime
* The date and time this resource was created.
*/
public void setCreationTime(String creationTime) {
this.creationTime = creationTime;
}
/**
*
* The date and time this resource was created.
*
*
* @return The date and time this resource was created.
*/
public String getCreationTime() {
return this.creationTime;
}
/**
*
* The date and time this resource was created.
*
*
* @param creationTime
* The date and time this resource was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withCreationTime(String creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The description of the application.
*
*
* Minimum length=1. Maximum length=256
*
*
* @param description
* The description of the application.
*
* Minimum length=1. Maximum length=256
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the application.
*
*
* Minimum length=1. Maximum length=256
*
*
* @return The description of the application.
*
* Minimum length=1. Maximum length=256
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the application.
*
*
* Minimum length=1. Maximum length=256
*
*
* @param description
* The description of the application.
*
* Minimum length=1. Maximum length=256
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* A URL with more information about the application, for example the location of your GitHub repository for the
* application.
*
*
* @param homePageUrl
* A URL with more information about the application, for example the location of your GitHub repository for
* the application.
*/
public void setHomePageUrl(String homePageUrl) {
this.homePageUrl = homePageUrl;
}
/**
*
* A URL with more information about the application, for example the location of your GitHub repository for the
* application.
*
*
* @return A URL with more information about the application, for example the location of your GitHub repository for
* the application.
*/
public String getHomePageUrl() {
return this.homePageUrl;
}
/**
*
* A URL with more information about the application, for example the location of your GitHub repository for the
* application.
*
*
* @param homePageUrl
* A URL with more information about the application, for example the location of your GitHub repository for
* the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withHomePageUrl(String homePageUrl) {
setHomePageUrl(homePageUrl);
return this;
}
/**
*
* Whether the author of this application has been verified. This means means that AWS has made a good faith review,
* as a reasonable and prudent service provider, of the information provided by the requester and has confirmed that
* the requester's identity is as claimed.
*
*
* @param isVerifiedAuthor
* Whether the author of this application has been verified. This means means that AWS has made a good faith
* review, as a reasonable and prudent service provider, of the information provided by the requester and has
* confirmed that the requester's identity is as claimed.
*/
public void setIsVerifiedAuthor(Boolean isVerifiedAuthor) {
this.isVerifiedAuthor = isVerifiedAuthor;
}
/**
*
* Whether the author of this application has been verified. This means means that AWS has made a good faith review,
* as a reasonable and prudent service provider, of the information provided by the requester and has confirmed that
* the requester's identity is as claimed.
*
*
* @return Whether the author of this application has been verified. This means means that AWS has made a good faith
* review, as a reasonable and prudent service provider, of the information provided by the requester and
* has confirmed that the requester's identity is as claimed.
*/
public Boolean getIsVerifiedAuthor() {
return this.isVerifiedAuthor;
}
/**
*
* Whether the author of this application has been verified. This means means that AWS has made a good faith review,
* as a reasonable and prudent service provider, of the information provided by the requester and has confirmed that
* the requester's identity is as claimed.
*
*
* @param isVerifiedAuthor
* Whether the author of this application has been verified. This means means that AWS has made a good faith
* review, as a reasonable and prudent service provider, of the information provided by the requester and has
* confirmed that the requester's identity is as claimed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withIsVerifiedAuthor(Boolean isVerifiedAuthor) {
setIsVerifiedAuthor(isVerifiedAuthor);
return this;
}
/**
*
* Whether the author of this application has been verified. This means means that AWS has made a good faith review,
* as a reasonable and prudent service provider, of the information provided by the requester and has confirmed that
* the requester's identity is as claimed.
*
*
* @return Whether the author of this application has been verified. This means means that AWS has made a good faith
* review, as a reasonable and prudent service provider, of the information provided by the requester and
* has confirmed that the requester's identity is as claimed.
*/
public Boolean isVerifiedAuthor() {
return this.isVerifiedAuthor;
}
/**
*
* Labels to improve discovery of apps in search results.
*
*
* Minimum length=1. Maximum length=127. Maximum number of labels: 10
*
*
* Pattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
*
* @return Labels to improve discovery of apps in search results.
*
* Minimum length=1. Maximum length=127. Maximum number of labels: 10
*
*
* Pattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*/
public java.util.List getLabels() {
return labels;
}
/**
*
* Labels to improve discovery of apps in search results.
*
*
* Minimum length=1. Maximum length=127. Maximum number of labels: 10
*
*
* Pattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
*
* @param labels
* Labels to improve discovery of apps in search results.
*
* Minimum length=1. Maximum length=127. Maximum number of labels: 10
*
*
* Pattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*/
public void setLabels(java.util.Collection labels) {
if (labels == null) {
this.labels = null;
return;
}
this.labels = new java.util.ArrayList(labels);
}
/**
*
* Labels to improve discovery of apps in search results.
*
*
* Minimum length=1. Maximum length=127. Maximum number of labels: 10
*
*
* Pattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLabels(java.util.Collection)} or {@link #withLabels(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param labels
* Labels to improve discovery of apps in search results.
*
* Minimum length=1. Maximum length=127. Maximum number of labels: 10
*
*
* Pattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withLabels(String... labels) {
if (this.labels == null) {
setLabels(new java.util.ArrayList(labels.length));
}
for (String ele : labels) {
this.labels.add(ele);
}
return this;
}
/**
*
* Labels to improve discovery of apps in search results.
*
*
* Minimum length=1. Maximum length=127. Maximum number of labels: 10
*
*
* Pattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
*
* @param labels
* Labels to improve discovery of apps in search results.
*
* Minimum length=1. Maximum length=127. Maximum number of labels: 10
*
*
* Pattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withLabels(java.util.Collection labels) {
setLabels(labels);
return this;
}
/**
*
* A link to a license file of the app that matches the spdxLicenseID value of your application.
*
*
* Maximum size 5 MB
*
*
* @param licenseUrl
* A link to a license file of the app that matches the spdxLicenseID value of your application.
*
* Maximum size 5 MB
*/
public void setLicenseUrl(String licenseUrl) {
this.licenseUrl = licenseUrl;
}
/**
*
* A link to a license file of the app that matches the spdxLicenseID value of your application.
*
*
* Maximum size 5 MB
*
*
* @return A link to a license file of the app that matches the spdxLicenseID value of your application.
*
* Maximum size 5 MB
*/
public String getLicenseUrl() {
return this.licenseUrl;
}
/**
*
* A link to a license file of the app that matches the spdxLicenseID value of your application.
*
*
* Maximum size 5 MB
*
*
* @param licenseUrl
* A link to a license file of the app that matches the spdxLicenseID value of your application.
*
* Maximum size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withLicenseUrl(String licenseUrl) {
setLicenseUrl(licenseUrl);
return this;
}
/**
*
* The name of the application.
*
*
* Minimum length=1. Maximum length=140
*
*
* Pattern: "[a-zA-Z0-9\\-]+";
*
*
* @param name
* The name of the application.
*
* Minimum length=1. Maximum length=140
*
*
* Pattern: "[a-zA-Z0-9\\-]+";
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the application.
*
*
* Minimum length=1. Maximum length=140
*
*
* Pattern: "[a-zA-Z0-9\\-]+";
*
*
* @return The name of the application.
*
* Minimum length=1. Maximum length=140
*
*
* Pattern: "[a-zA-Z0-9\\-]+";
*/
public String getName() {
return this.name;
}
/**
*
* The name of the application.
*
*
* Minimum length=1. Maximum length=140
*
*
* Pattern: "[a-zA-Z0-9\\-]+";
*
*
* @param name
* The name of the application.
*
* Minimum length=1. Maximum length=140
*
*
* Pattern: "[a-zA-Z0-9\\-]+";
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withName(String name) {
setName(name);
return this;
}
/**
*
* A link to the readme file in Markdown language that contains a more detailed description of the application and
* how it works.
*
*
* Maximum size 5 MB
*
*
* @param readmeUrl
* A link to the readme file in Markdown language that contains a more detailed description of the
* application and how it works.
*
* Maximum size 5 MB
*/
public void setReadmeUrl(String readmeUrl) {
this.readmeUrl = readmeUrl;
}
/**
*
* A link to the readme file in Markdown language that contains a more detailed description of the application and
* how it works.
*
*
* Maximum size 5 MB
*
*
* @return A link to the readme file in Markdown language that contains a more detailed description of the
* application and how it works.
*
* Maximum size 5 MB
*/
public String getReadmeUrl() {
return this.readmeUrl;
}
/**
*
* A link to the readme file in Markdown language that contains a more detailed description of the application and
* how it works.
*
*
* Maximum size 5 MB
*
*
* @param readmeUrl
* A link to the readme file in Markdown language that contains a more detailed description of the
* application and how it works.
*
* Maximum size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withReadmeUrl(String readmeUrl) {
setReadmeUrl(readmeUrl);
return this;
}
/**
*
* A valid identifier from https://spdx.org/licenses/.
*
*
* @param spdxLicenseId
* A valid identifier from https://spdx.org/licenses/.
*/
public void setSpdxLicenseId(String spdxLicenseId) {
this.spdxLicenseId = spdxLicenseId;
}
/**
*
* A valid identifier from https://spdx.org/licenses/.
*
*
* @return A valid identifier from https://spdx.org/licenses/.
*/
public String getSpdxLicenseId() {
return this.spdxLicenseId;
}
/**
*
* A valid identifier from https://spdx.org/licenses/.
*
*
* @param spdxLicenseId
* A valid identifier from https://spdx.org/licenses/.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withSpdxLicenseId(String spdxLicenseId) {
setSpdxLicenseId(spdxLicenseId);
return this;
}
/**
*
* The URL to the public profile of a verified author. This URL is submitted by the author.
*
*
* @param verifiedAuthorUrl
* The URL to the public profile of a verified author. This URL is submitted by the author.
*/
public void setVerifiedAuthorUrl(String verifiedAuthorUrl) {
this.verifiedAuthorUrl = verifiedAuthorUrl;
}
/**
*
* The URL to the public profile of a verified author. This URL is submitted by the author.
*
*
* @return The URL to the public profile of a verified author. This URL is submitted by the author.
*/
public String getVerifiedAuthorUrl() {
return this.verifiedAuthorUrl;
}
/**
*
* The URL to the public profile of a verified author. This URL is submitted by the author.
*
*
* @param verifiedAuthorUrl
* The URL to the public profile of a verified author. This URL is submitted by the author.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withVerifiedAuthorUrl(String verifiedAuthorUrl) {
setVerifiedAuthorUrl(verifiedAuthorUrl);
return this;
}
/**
*
* Version information about the application.
*
*
* @param version
* Version information about the application.
*/
public void setVersion(Version version) {
this.version = version;
}
/**
*
* Version information about the application.
*
*
* @return Version information about the application.
*/
public Version getVersion() {
return this.version;
}
/**
*
* Version information about the application.
*
*
* @param version
* Version information about the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationResult withVersion(Version version) {
setVersion(version);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getApplicationId() != null)
sb.append("ApplicationId: ").append(getApplicationId()).append(",");
if (getAuthor() != null)
sb.append("Author: ").append(getAuthor()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getHomePageUrl() != null)
sb.append("HomePageUrl: ").append(getHomePageUrl()).append(",");
if (getIsVerifiedAuthor() != null)
sb.append("IsVerifiedAuthor: ").append(getIsVerifiedAuthor()).append(",");
if (getLabels() != null)
sb.append("Labels: ").append(getLabels()).append(",");
if (getLicenseUrl() != null)
sb.append("LicenseUrl: ").append(getLicenseUrl()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getReadmeUrl() != null)
sb.append("ReadmeUrl: ").append(getReadmeUrl()).append(",");
if (getSpdxLicenseId() != null)
sb.append("SpdxLicenseId: ").append(getSpdxLicenseId()).append(",");
if (getVerifiedAuthorUrl() != null)
sb.append("VerifiedAuthorUrl: ").append(getVerifiedAuthorUrl()).append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateApplicationResult == false)
return false;
CreateApplicationResult other = (CreateApplicationResult) obj;
if (other.getApplicationId() == null ^ this.getApplicationId() == null)
return false;
if (other.getApplicationId() != null && other.getApplicationId().equals(this.getApplicationId()) == false)
return false;
if (other.getAuthor() == null ^ this.getAuthor() == null)
return false;
if (other.getAuthor() != null && other.getAuthor().equals(this.getAuthor()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getHomePageUrl() == null ^ this.getHomePageUrl() == null)
return false;
if (other.getHomePageUrl() != null && other.getHomePageUrl().equals(this.getHomePageUrl()) == false)
return false;
if (other.getIsVerifiedAuthor() == null ^ this.getIsVerifiedAuthor() == null)
return false;
if (other.getIsVerifiedAuthor() != null && other.getIsVerifiedAuthor().equals(this.getIsVerifiedAuthor()) == false)
return false;
if (other.getLabels() == null ^ this.getLabels() == null)
return false;
if (other.getLabels() != null && other.getLabels().equals(this.getLabels()) == false)
return false;
if (other.getLicenseUrl() == null ^ this.getLicenseUrl() == null)
return false;
if (other.getLicenseUrl() != null && other.getLicenseUrl().equals(this.getLicenseUrl()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getReadmeUrl() == null ^ this.getReadmeUrl() == null)
return false;
if (other.getReadmeUrl() != null && other.getReadmeUrl().equals(this.getReadmeUrl()) == false)
return false;
if (other.getSpdxLicenseId() == null ^ this.getSpdxLicenseId() == null)
return false;
if (other.getSpdxLicenseId() != null && other.getSpdxLicenseId().equals(this.getSpdxLicenseId()) == false)
return false;
if (other.getVerifiedAuthorUrl() == null ^ this.getVerifiedAuthorUrl() == null)
return false;
if (other.getVerifiedAuthorUrl() != null && other.getVerifiedAuthorUrl().equals(this.getVerifiedAuthorUrl()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getApplicationId() == null) ? 0 : getApplicationId().hashCode());
hashCode = prime * hashCode + ((getAuthor() == null) ? 0 : getAuthor().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getHomePageUrl() == null) ? 0 : getHomePageUrl().hashCode());
hashCode = prime * hashCode + ((getIsVerifiedAuthor() == null) ? 0 : getIsVerifiedAuthor().hashCode());
hashCode = prime * hashCode + ((getLabels() == null) ? 0 : getLabels().hashCode());
hashCode = prime * hashCode + ((getLicenseUrl() == null) ? 0 : getLicenseUrl().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getReadmeUrl() == null) ? 0 : getReadmeUrl().hashCode());
hashCode = prime * hashCode + ((getSpdxLicenseId() == null) ? 0 : getSpdxLicenseId().hashCode());
hashCode = prime * hashCode + ((getVerifiedAuthorUrl() == null) ? 0 : getVerifiedAuthorUrl().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
return hashCode;
}
@Override
public CreateApplicationResult clone() {
try {
return (CreateApplicationResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}