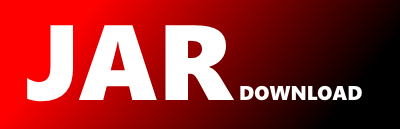
com.amazonaws.services.servermigration.model.AppSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-servermigration Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.servermigration.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about the application.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AppSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique ID of the application.
*
*/
private String appId;
/**
*
* The ID of the application.
*
*/
private String importedAppId;
/**
*
* The name of the application.
*
*/
private String name;
/**
*
* The description of the application.
*
*/
private String description;
/**
*
* Status of the application.
*
*/
private String status;
/**
*
* A message related to the status of the application
*
*/
private String statusMessage;
/**
*
* Status of the replication configuration.
*
*/
private String replicationConfigurationStatus;
/**
*
* The replication status of the application.
*
*/
private String replicationStatus;
/**
*
* A message related to the replication status of the application.
*
*/
private String replicationStatusMessage;
/**
*
* The timestamp of the application's most recent successful replication.
*
*/
private java.util.Date latestReplicationTime;
/**
*
* Status of the launch configuration.
*
*/
private String launchConfigurationStatus;
/**
*
* The launch status of the application.
*
*/
private String launchStatus;
/**
*
* A message related to the launch status of the application.
*
*/
private String launchStatusMessage;
/**
*
* Details about the latest launch of the application.
*
*/
private LaunchDetails launchDetails;
/**
*
* The creation time of the application.
*
*/
private java.util.Date creationTime;
/**
*
* The last modified time of the application.
*
*/
private java.util.Date lastModified;
/**
*
* The name of the service role in the customer's account used by Server Migration Service.
*
*/
private String roleName;
/**
*
* The number of server groups present in the application.
*
*/
private Integer totalServerGroups;
/**
*
* The number of servers present in the application.
*
*/
private Integer totalServers;
/**
*
* The unique ID of the application.
*
*
* @param appId
* The unique ID of the application.
*/
public void setAppId(String appId) {
this.appId = appId;
}
/**
*
* The unique ID of the application.
*
*
* @return The unique ID of the application.
*/
public String getAppId() {
return this.appId;
}
/**
*
* The unique ID of the application.
*
*
* @param appId
* The unique ID of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withAppId(String appId) {
setAppId(appId);
return this;
}
/**
*
* The ID of the application.
*
*
* @param importedAppId
* The ID of the application.
*/
public void setImportedAppId(String importedAppId) {
this.importedAppId = importedAppId;
}
/**
*
* The ID of the application.
*
*
* @return The ID of the application.
*/
public String getImportedAppId() {
return this.importedAppId;
}
/**
*
* The ID of the application.
*
*
* @param importedAppId
* The ID of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withImportedAppId(String importedAppId) {
setImportedAppId(importedAppId);
return this;
}
/**
*
* The name of the application.
*
*
* @param name
* The name of the application.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the application.
*
*
* @return The name of the application.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the application.
*
*
* @param name
* The name of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withName(String name) {
setName(name);
return this;
}
/**
*
* The description of the application.
*
*
* @param description
* The description of the application.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the application.
*
*
* @return The description of the application.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the application.
*
*
* @param description
* The description of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Status of the application.
*
*
* @param status
* Status of the application.
* @see AppStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Status of the application.
*
*
* @return Status of the application.
* @see AppStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Status of the application.
*
*
* @param status
* Status of the application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppStatus
*/
public AppSummary withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Status of the application.
*
*
* @param status
* Status of the application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppStatus
*/
public AppSummary withStatus(AppStatus status) {
this.status = status.toString();
return this;
}
/**
*
* A message related to the status of the application
*
*
* @param statusMessage
* A message related to the status of the application
*/
public void setStatusMessage(String statusMessage) {
this.statusMessage = statusMessage;
}
/**
*
* A message related to the status of the application
*
*
* @return A message related to the status of the application
*/
public String getStatusMessage() {
return this.statusMessage;
}
/**
*
* A message related to the status of the application
*
*
* @param statusMessage
* A message related to the status of the application
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withStatusMessage(String statusMessage) {
setStatusMessage(statusMessage);
return this;
}
/**
*
* Status of the replication configuration.
*
*
* @param replicationConfigurationStatus
* Status of the replication configuration.
* @see AppReplicationConfigurationStatus
*/
public void setReplicationConfigurationStatus(String replicationConfigurationStatus) {
this.replicationConfigurationStatus = replicationConfigurationStatus;
}
/**
*
* Status of the replication configuration.
*
*
* @return Status of the replication configuration.
* @see AppReplicationConfigurationStatus
*/
public String getReplicationConfigurationStatus() {
return this.replicationConfigurationStatus;
}
/**
*
* Status of the replication configuration.
*
*
* @param replicationConfigurationStatus
* Status of the replication configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppReplicationConfigurationStatus
*/
public AppSummary withReplicationConfigurationStatus(String replicationConfigurationStatus) {
setReplicationConfigurationStatus(replicationConfigurationStatus);
return this;
}
/**
*
* Status of the replication configuration.
*
*
* @param replicationConfigurationStatus
* Status of the replication configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppReplicationConfigurationStatus
*/
public AppSummary withReplicationConfigurationStatus(AppReplicationConfigurationStatus replicationConfigurationStatus) {
this.replicationConfigurationStatus = replicationConfigurationStatus.toString();
return this;
}
/**
*
* The replication status of the application.
*
*
* @param replicationStatus
* The replication status of the application.
* @see AppReplicationStatus
*/
public void setReplicationStatus(String replicationStatus) {
this.replicationStatus = replicationStatus;
}
/**
*
* The replication status of the application.
*
*
* @return The replication status of the application.
* @see AppReplicationStatus
*/
public String getReplicationStatus() {
return this.replicationStatus;
}
/**
*
* The replication status of the application.
*
*
* @param replicationStatus
* The replication status of the application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppReplicationStatus
*/
public AppSummary withReplicationStatus(String replicationStatus) {
setReplicationStatus(replicationStatus);
return this;
}
/**
*
* The replication status of the application.
*
*
* @param replicationStatus
* The replication status of the application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppReplicationStatus
*/
public AppSummary withReplicationStatus(AppReplicationStatus replicationStatus) {
this.replicationStatus = replicationStatus.toString();
return this;
}
/**
*
* A message related to the replication status of the application.
*
*
* @param replicationStatusMessage
* A message related to the replication status of the application.
*/
public void setReplicationStatusMessage(String replicationStatusMessage) {
this.replicationStatusMessage = replicationStatusMessage;
}
/**
*
* A message related to the replication status of the application.
*
*
* @return A message related to the replication status of the application.
*/
public String getReplicationStatusMessage() {
return this.replicationStatusMessage;
}
/**
*
* A message related to the replication status of the application.
*
*
* @param replicationStatusMessage
* A message related to the replication status of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withReplicationStatusMessage(String replicationStatusMessage) {
setReplicationStatusMessage(replicationStatusMessage);
return this;
}
/**
*
* The timestamp of the application's most recent successful replication.
*
*
* @param latestReplicationTime
* The timestamp of the application's most recent successful replication.
*/
public void setLatestReplicationTime(java.util.Date latestReplicationTime) {
this.latestReplicationTime = latestReplicationTime;
}
/**
*
* The timestamp of the application's most recent successful replication.
*
*
* @return The timestamp of the application's most recent successful replication.
*/
public java.util.Date getLatestReplicationTime() {
return this.latestReplicationTime;
}
/**
*
* The timestamp of the application's most recent successful replication.
*
*
* @param latestReplicationTime
* The timestamp of the application's most recent successful replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withLatestReplicationTime(java.util.Date latestReplicationTime) {
setLatestReplicationTime(latestReplicationTime);
return this;
}
/**
*
* Status of the launch configuration.
*
*
* @param launchConfigurationStatus
* Status of the launch configuration.
* @see AppLaunchConfigurationStatus
*/
public void setLaunchConfigurationStatus(String launchConfigurationStatus) {
this.launchConfigurationStatus = launchConfigurationStatus;
}
/**
*
* Status of the launch configuration.
*
*
* @return Status of the launch configuration.
* @see AppLaunchConfigurationStatus
*/
public String getLaunchConfigurationStatus() {
return this.launchConfigurationStatus;
}
/**
*
* Status of the launch configuration.
*
*
* @param launchConfigurationStatus
* Status of the launch configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppLaunchConfigurationStatus
*/
public AppSummary withLaunchConfigurationStatus(String launchConfigurationStatus) {
setLaunchConfigurationStatus(launchConfigurationStatus);
return this;
}
/**
*
* Status of the launch configuration.
*
*
* @param launchConfigurationStatus
* Status of the launch configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppLaunchConfigurationStatus
*/
public AppSummary withLaunchConfigurationStatus(AppLaunchConfigurationStatus launchConfigurationStatus) {
this.launchConfigurationStatus = launchConfigurationStatus.toString();
return this;
}
/**
*
* The launch status of the application.
*
*
* @param launchStatus
* The launch status of the application.
* @see AppLaunchStatus
*/
public void setLaunchStatus(String launchStatus) {
this.launchStatus = launchStatus;
}
/**
*
* The launch status of the application.
*
*
* @return The launch status of the application.
* @see AppLaunchStatus
*/
public String getLaunchStatus() {
return this.launchStatus;
}
/**
*
* The launch status of the application.
*
*
* @param launchStatus
* The launch status of the application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppLaunchStatus
*/
public AppSummary withLaunchStatus(String launchStatus) {
setLaunchStatus(launchStatus);
return this;
}
/**
*
* The launch status of the application.
*
*
* @param launchStatus
* The launch status of the application.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppLaunchStatus
*/
public AppSummary withLaunchStatus(AppLaunchStatus launchStatus) {
this.launchStatus = launchStatus.toString();
return this;
}
/**
*
* A message related to the launch status of the application.
*
*
* @param launchStatusMessage
* A message related to the launch status of the application.
*/
public void setLaunchStatusMessage(String launchStatusMessage) {
this.launchStatusMessage = launchStatusMessage;
}
/**
*
* A message related to the launch status of the application.
*
*
* @return A message related to the launch status of the application.
*/
public String getLaunchStatusMessage() {
return this.launchStatusMessage;
}
/**
*
* A message related to the launch status of the application.
*
*
* @param launchStatusMessage
* A message related to the launch status of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withLaunchStatusMessage(String launchStatusMessage) {
setLaunchStatusMessage(launchStatusMessage);
return this;
}
/**
*
* Details about the latest launch of the application.
*
*
* @param launchDetails
* Details about the latest launch of the application.
*/
public void setLaunchDetails(LaunchDetails launchDetails) {
this.launchDetails = launchDetails;
}
/**
*
* Details about the latest launch of the application.
*
*
* @return Details about the latest launch of the application.
*/
public LaunchDetails getLaunchDetails() {
return this.launchDetails;
}
/**
*
* Details about the latest launch of the application.
*
*
* @param launchDetails
* Details about the latest launch of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withLaunchDetails(LaunchDetails launchDetails) {
setLaunchDetails(launchDetails);
return this;
}
/**
*
* The creation time of the application.
*
*
* @param creationTime
* The creation time of the application.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The creation time of the application.
*
*
* @return The creation time of the application.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The creation time of the application.
*
*
* @param creationTime
* The creation time of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The last modified time of the application.
*
*
* @param lastModified
* The last modified time of the application.
*/
public void setLastModified(java.util.Date lastModified) {
this.lastModified = lastModified;
}
/**
*
* The last modified time of the application.
*
*
* @return The last modified time of the application.
*/
public java.util.Date getLastModified() {
return this.lastModified;
}
/**
*
* The last modified time of the application.
*
*
* @param lastModified
* The last modified time of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withLastModified(java.util.Date lastModified) {
setLastModified(lastModified);
return this;
}
/**
*
* The name of the service role in the customer's account used by Server Migration Service.
*
*
* @param roleName
* The name of the service role in the customer's account used by Server Migration Service.
*/
public void setRoleName(String roleName) {
this.roleName = roleName;
}
/**
*
* The name of the service role in the customer's account used by Server Migration Service.
*
*
* @return The name of the service role in the customer's account used by Server Migration Service.
*/
public String getRoleName() {
return this.roleName;
}
/**
*
* The name of the service role in the customer's account used by Server Migration Service.
*
*
* @param roleName
* The name of the service role in the customer's account used by Server Migration Service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withRoleName(String roleName) {
setRoleName(roleName);
return this;
}
/**
*
* The number of server groups present in the application.
*
*
* @param totalServerGroups
* The number of server groups present in the application.
*/
public void setTotalServerGroups(Integer totalServerGroups) {
this.totalServerGroups = totalServerGroups;
}
/**
*
* The number of server groups present in the application.
*
*
* @return The number of server groups present in the application.
*/
public Integer getTotalServerGroups() {
return this.totalServerGroups;
}
/**
*
* The number of server groups present in the application.
*
*
* @param totalServerGroups
* The number of server groups present in the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withTotalServerGroups(Integer totalServerGroups) {
setTotalServerGroups(totalServerGroups);
return this;
}
/**
*
* The number of servers present in the application.
*
*
* @param totalServers
* The number of servers present in the application.
*/
public void setTotalServers(Integer totalServers) {
this.totalServers = totalServers;
}
/**
*
* The number of servers present in the application.
*
*
* @return The number of servers present in the application.
*/
public Integer getTotalServers() {
return this.totalServers;
}
/**
*
* The number of servers present in the application.
*
*
* @param totalServers
* The number of servers present in the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AppSummary withTotalServers(Integer totalServers) {
setTotalServers(totalServers);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAppId() != null)
sb.append("AppId: ").append(getAppId()).append(",");
if (getImportedAppId() != null)
sb.append("ImportedAppId: ").append(getImportedAppId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusMessage() != null)
sb.append("StatusMessage: ").append(getStatusMessage()).append(",");
if (getReplicationConfigurationStatus() != null)
sb.append("ReplicationConfigurationStatus: ").append(getReplicationConfigurationStatus()).append(",");
if (getReplicationStatus() != null)
sb.append("ReplicationStatus: ").append(getReplicationStatus()).append(",");
if (getReplicationStatusMessage() != null)
sb.append("ReplicationStatusMessage: ").append(getReplicationStatusMessage()).append(",");
if (getLatestReplicationTime() != null)
sb.append("LatestReplicationTime: ").append(getLatestReplicationTime()).append(",");
if (getLaunchConfigurationStatus() != null)
sb.append("LaunchConfigurationStatus: ").append(getLaunchConfigurationStatus()).append(",");
if (getLaunchStatus() != null)
sb.append("LaunchStatus: ").append(getLaunchStatus()).append(",");
if (getLaunchStatusMessage() != null)
sb.append("LaunchStatusMessage: ").append(getLaunchStatusMessage()).append(",");
if (getLaunchDetails() != null)
sb.append("LaunchDetails: ").append(getLaunchDetails()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getLastModified() != null)
sb.append("LastModified: ").append(getLastModified()).append(",");
if (getRoleName() != null)
sb.append("RoleName: ").append(getRoleName()).append(",");
if (getTotalServerGroups() != null)
sb.append("TotalServerGroups: ").append(getTotalServerGroups()).append(",");
if (getTotalServers() != null)
sb.append("TotalServers: ").append(getTotalServers());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AppSummary == false)
return false;
AppSummary other = (AppSummary) obj;
if (other.getAppId() == null ^ this.getAppId() == null)
return false;
if (other.getAppId() != null && other.getAppId().equals(this.getAppId()) == false)
return false;
if (other.getImportedAppId() == null ^ this.getImportedAppId() == null)
return false;
if (other.getImportedAppId() != null && other.getImportedAppId().equals(this.getImportedAppId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusMessage() == null ^ this.getStatusMessage() == null)
return false;
if (other.getStatusMessage() != null && other.getStatusMessage().equals(this.getStatusMessage()) == false)
return false;
if (other.getReplicationConfigurationStatus() == null ^ this.getReplicationConfigurationStatus() == null)
return false;
if (other.getReplicationConfigurationStatus() != null
&& other.getReplicationConfigurationStatus().equals(this.getReplicationConfigurationStatus()) == false)
return false;
if (other.getReplicationStatus() == null ^ this.getReplicationStatus() == null)
return false;
if (other.getReplicationStatus() != null && other.getReplicationStatus().equals(this.getReplicationStatus()) == false)
return false;
if (other.getReplicationStatusMessage() == null ^ this.getReplicationStatusMessage() == null)
return false;
if (other.getReplicationStatusMessage() != null && other.getReplicationStatusMessage().equals(this.getReplicationStatusMessage()) == false)
return false;
if (other.getLatestReplicationTime() == null ^ this.getLatestReplicationTime() == null)
return false;
if (other.getLatestReplicationTime() != null && other.getLatestReplicationTime().equals(this.getLatestReplicationTime()) == false)
return false;
if (other.getLaunchConfigurationStatus() == null ^ this.getLaunchConfigurationStatus() == null)
return false;
if (other.getLaunchConfigurationStatus() != null && other.getLaunchConfigurationStatus().equals(this.getLaunchConfigurationStatus()) == false)
return false;
if (other.getLaunchStatus() == null ^ this.getLaunchStatus() == null)
return false;
if (other.getLaunchStatus() != null && other.getLaunchStatus().equals(this.getLaunchStatus()) == false)
return false;
if (other.getLaunchStatusMessage() == null ^ this.getLaunchStatusMessage() == null)
return false;
if (other.getLaunchStatusMessage() != null && other.getLaunchStatusMessage().equals(this.getLaunchStatusMessage()) == false)
return false;
if (other.getLaunchDetails() == null ^ this.getLaunchDetails() == null)
return false;
if (other.getLaunchDetails() != null && other.getLaunchDetails().equals(this.getLaunchDetails()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getLastModified() == null ^ this.getLastModified() == null)
return false;
if (other.getLastModified() != null && other.getLastModified().equals(this.getLastModified()) == false)
return false;
if (other.getRoleName() == null ^ this.getRoleName() == null)
return false;
if (other.getRoleName() != null && other.getRoleName().equals(this.getRoleName()) == false)
return false;
if (other.getTotalServerGroups() == null ^ this.getTotalServerGroups() == null)
return false;
if (other.getTotalServerGroups() != null && other.getTotalServerGroups().equals(this.getTotalServerGroups()) == false)
return false;
if (other.getTotalServers() == null ^ this.getTotalServers() == null)
return false;
if (other.getTotalServers() != null && other.getTotalServers().equals(this.getTotalServers()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAppId() == null) ? 0 : getAppId().hashCode());
hashCode = prime * hashCode + ((getImportedAppId() == null) ? 0 : getImportedAppId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusMessage() == null) ? 0 : getStatusMessage().hashCode());
hashCode = prime * hashCode + ((getReplicationConfigurationStatus() == null) ? 0 : getReplicationConfigurationStatus().hashCode());
hashCode = prime * hashCode + ((getReplicationStatus() == null) ? 0 : getReplicationStatus().hashCode());
hashCode = prime * hashCode + ((getReplicationStatusMessage() == null) ? 0 : getReplicationStatusMessage().hashCode());
hashCode = prime * hashCode + ((getLatestReplicationTime() == null) ? 0 : getLatestReplicationTime().hashCode());
hashCode = prime * hashCode + ((getLaunchConfigurationStatus() == null) ? 0 : getLaunchConfigurationStatus().hashCode());
hashCode = prime * hashCode + ((getLaunchStatus() == null) ? 0 : getLaunchStatus().hashCode());
hashCode = prime * hashCode + ((getLaunchStatusMessage() == null) ? 0 : getLaunchStatusMessage().hashCode());
hashCode = prime * hashCode + ((getLaunchDetails() == null) ? 0 : getLaunchDetails().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getLastModified() == null) ? 0 : getLastModified().hashCode());
hashCode = prime * hashCode + ((getRoleName() == null) ? 0 : getRoleName().hashCode());
hashCode = prime * hashCode + ((getTotalServerGroups() == null) ? 0 : getTotalServerGroups().hashCode());
hashCode = prime * hashCode + ((getTotalServers() == null) ? 0 : getTotalServers().hashCode());
return hashCode;
}
@Override
public AppSummary clone() {
try {
return (AppSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.servermigration.model.transform.AppSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}