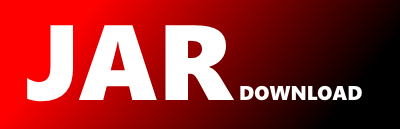
com.amazonaws.services.servermigration.AWSServerMigration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-servermigration Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.servermigration;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.servermigration.model.*;
/**
* Interface for accessing SMS.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.servermigration.AbstractAWSServerMigration} instead.
*
*
*
*
* Product update
*
*
* We recommend Amazon Web Services Application Migration
* Service (Amazon Web Services MGN) as the primary migration service for lift-and-shift migrations. If Amazon Web
* Services MGN is unavailable in a specific Amazon Web Services Region, you can use the Server Migration Service APIs
* through March 2023.
*
*
*
* Server Migration Service (Server Migration Service) makes it easier and faster for you to migrate your on-premises
* workloads to Amazon Web Services. To learn more about Server Migration Service, see the following resources:
*
*
* -
*
*
* -
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSServerMigration {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "sms";
/**
* Overrides the default endpoint for this client ("sms.us-east-1.amazonaws.com"). Callers can use this method to
* control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "sms.us-east-1.amazonaws.com") or a full URL, including the protocol
* (ex: "sms.us-east-1.amazonaws.com"). If the protocol is not specified here, the default protocol from this
* client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "sms.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "sms.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSServerMigration#setEndpoint(String)}, sets the regional endpoint for this client's
* service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Creates an application. An application consists of one or more server groups. Each server group contain one or
* more servers.
*
*
* @param createAppRequest
* @return Result of the CreateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.CreateApp
* @see AWS API
* Documentation
*/
CreateAppResult createApp(CreateAppRequest createAppRequest);
/**
*
* Creates a replication job. The replication job schedules periodic replication runs to replicate your server to
* Amazon Web Services. Each replication run creates an Amazon Machine Image (AMI).
*
*
* @param createReplicationJobRequest
* @return Result of the CreateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ServerCannotBeReplicatedException
* The specified server cannot be replicated.
* @throws ReplicationJobAlreadyExistsException
* The specified replication job already exists.
* @throws NoConnectorsAvailableException
* There are no connectors available.
* @throws InternalErrorException
* An internal error occurred.
* @throws TemporarilyUnavailableException
* The service is temporarily unavailable.
* @sample AWSServerMigration.CreateReplicationJob
* @see AWS API
* Documentation
*/
CreateReplicationJobResult createReplicationJob(CreateReplicationJobRequest createReplicationJobRequest);
/**
*
* Deletes the specified application. Optionally deletes the launched stack associated with the application and all
* Server Migration Service replication jobs for servers in the application.
*
*
* @param deleteAppRequest
* @return Result of the DeleteApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.DeleteApp
* @see AWS API
* Documentation
*/
DeleteAppResult deleteApp(DeleteAppRequest deleteAppRequest);
/**
*
* Deletes the launch configuration for the specified application.
*
*
* @param deleteAppLaunchConfigurationRequest
* @return Result of the DeleteAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.DeleteAppLaunchConfiguration
* @see AWS API Documentation
*/
DeleteAppLaunchConfigurationResult deleteAppLaunchConfiguration(DeleteAppLaunchConfigurationRequest deleteAppLaunchConfigurationRequest);
/**
*
* Deletes the replication configuration for the specified application.
*
*
* @param deleteAppReplicationConfigurationRequest
* @return Result of the DeleteAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.DeleteAppReplicationConfiguration
* @see AWS API Documentation
*/
DeleteAppReplicationConfigurationResult deleteAppReplicationConfiguration(DeleteAppReplicationConfigurationRequest deleteAppReplicationConfigurationRequest);
/**
*
* Deletes the validation configuration for the specified application.
*
*
* @param deleteAppValidationConfigurationRequest
* @return Result of the DeleteAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.DeleteAppValidationConfiguration
* @see AWS API Documentation
*/
DeleteAppValidationConfigurationResult deleteAppValidationConfiguration(DeleteAppValidationConfigurationRequest deleteAppValidationConfigurationRequest);
/**
*
* Deletes the specified replication job.
*
*
* After you delete a replication job, there are no further replication runs. Amazon Web Services deletes the
* contents of the Amazon S3 bucket used to store Server Migration Service artifacts. The AMIs created by the
* replication runs are not deleted.
*
*
* @param deleteReplicationJobRequest
* @return Result of the DeleteReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ReplicationJobNotFoundException
* The specified replication job does not exist.
* @sample AWSServerMigration.DeleteReplicationJob
* @see AWS API
* Documentation
*/
DeleteReplicationJobResult deleteReplicationJob(DeleteReplicationJobRequest deleteReplicationJobRequest);
/**
*
* Deletes all servers from your server catalog.
*
*
* @param deleteServerCatalogRequest
* @return Result of the DeleteServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @sample AWSServerMigration.DeleteServerCatalog
* @see AWS API
* Documentation
*/
DeleteServerCatalogResult deleteServerCatalog(DeleteServerCatalogRequest deleteServerCatalogRequest);
/**
*
* Disassociates the specified connector from Server Migration Service.
*
*
* After you disassociate a connector, it is no longer available to support replication jobs.
*
*
* @param disassociateConnectorRequest
* @return Result of the DisassociateConnector operation returned by the service.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @sample AWSServerMigration.DisassociateConnector
* @see AWS API
* Documentation
*/
DisassociateConnectorResult disassociateConnector(DisassociateConnectorRequest disassociateConnectorRequest);
/**
*
* Generates a target change set for a currently launched stack and writes it to an Amazon S3 object in the
* customer’s Amazon S3 bucket.
*
*
* @param generateChangeSetRequest
* @return Result of the GenerateChangeSet operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.GenerateChangeSet
* @see AWS API
* Documentation
*/
GenerateChangeSetResult generateChangeSet(GenerateChangeSetRequest generateChangeSetRequest);
/**
*
* Generates an CloudFormation template based on the current launch configuration and writes it to an Amazon S3
* object in the customer’s Amazon S3 bucket.
*
*
* @param generateTemplateRequest
* @return Result of the GenerateTemplate operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.GenerateTemplate
* @see AWS API
* Documentation
*/
GenerateTemplateResult generateTemplate(GenerateTemplateRequest generateTemplateRequest);
/**
*
* Retrieve information about the specified application.
*
*
* @param getAppRequest
* @return Result of the GetApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.GetApp
* @see AWS API
* Documentation
*/
GetAppResult getApp(GetAppRequest getAppRequest);
/**
*
* Retrieves the application launch configuration associated with the specified application.
*
*
* @param getAppLaunchConfigurationRequest
* @return Result of the GetAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.GetAppLaunchConfiguration
* @see AWS
* API Documentation
*/
GetAppLaunchConfigurationResult getAppLaunchConfiguration(GetAppLaunchConfigurationRequest getAppLaunchConfigurationRequest);
/**
*
* Retrieves the application replication configuration associated with the specified application.
*
*
* @param getAppReplicationConfigurationRequest
* @return Result of the GetAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.GetAppReplicationConfiguration
* @see AWS API Documentation
*/
GetAppReplicationConfigurationResult getAppReplicationConfiguration(GetAppReplicationConfigurationRequest getAppReplicationConfigurationRequest);
/**
*
* Retrieves information about a configuration for validating an application.
*
*
* @param getAppValidationConfigurationRequest
* @return Result of the GetAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.GetAppValidationConfiguration
* @see AWS API Documentation
*/
GetAppValidationConfigurationResult getAppValidationConfiguration(GetAppValidationConfigurationRequest getAppValidationConfigurationRequest);
/**
*
* Retrieves output from validating an application.
*
*
* @param getAppValidationOutputRequest
* @return Result of the GetAppValidationOutput operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.GetAppValidationOutput
* @see AWS API
* Documentation
*/
GetAppValidationOutputResult getAppValidationOutput(GetAppValidationOutputRequest getAppValidationOutputRequest);
/**
*
* Describes the connectors registered with the Server Migration Service.
*
*
* @param getConnectorsRequest
* @return Result of the GetConnectors operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @sample AWSServerMigration.GetConnectors
* @see AWS API
* Documentation
*/
GetConnectorsResult getConnectors(GetConnectorsRequest getConnectorsRequest);
/**
*
* Describes the specified replication job or all of your replication jobs.
*
*
* @param getReplicationJobsRequest
* @return Result of the GetReplicationJobs operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @sample AWSServerMigration.GetReplicationJobs
* @see AWS API
* Documentation
*/
GetReplicationJobsResult getReplicationJobs(GetReplicationJobsRequest getReplicationJobsRequest);
/**
*
* Describes the replication runs for the specified replication job.
*
*
* @param getReplicationRunsRequest
* @return Result of the GetReplicationRuns operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @sample AWSServerMigration.GetReplicationRuns
* @see AWS API
* Documentation
*/
GetReplicationRunsResult getReplicationRuns(GetReplicationRunsRequest getReplicationRunsRequest);
/**
*
* Describes the servers in your server catalog.
*
*
* Before you can describe your servers, you must import them using ImportServerCatalog.
*
*
* @param getServersRequest
* @return Result of the GetServers operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @sample AWSServerMigration.GetServers
* @see AWS API
* Documentation
*/
GetServersResult getServers(GetServersRequest getServersRequest);
/**
*
* Allows application import from Migration Hub.
*
*
* @param importAppCatalogRequest
* @return Result of the ImportAppCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.ImportAppCatalog
* @see AWS API
* Documentation
*/
ImportAppCatalogResult importAppCatalog(ImportAppCatalogRequest importAppCatalogRequest);
/**
*
* Gathers a complete list of on-premises servers. Connectors must be installed and monitoring all servers to
* import.
*
*
* This call returns immediately, but might take additional time to retrieve all the servers.
*
*
* @param importServerCatalogRequest
* @return Result of the ImportServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws NoConnectorsAvailableException
* There are no connectors available.
* @sample AWSServerMigration.ImportServerCatalog
* @see AWS API
* Documentation
*/
ImportServerCatalogResult importServerCatalog(ImportServerCatalogRequest importServerCatalogRequest);
/**
*
* Launches the specified application as a stack in CloudFormation.
*
*
* @param launchAppRequest
* @return Result of the LaunchApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.LaunchApp
* @see AWS API
* Documentation
*/
LaunchAppResult launchApp(LaunchAppRequest launchAppRequest);
/**
*
* Retrieves summaries for all applications.
*
*
* @param listAppsRequest
* @return Result of the ListApps operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.ListApps
* @see AWS API
* Documentation
*/
ListAppsResult listApps(ListAppsRequest listAppsRequest);
/**
*
* Provides information to Server Migration Service about whether application validation is successful.
*
*
* @param notifyAppValidationOutputRequest
* @return Result of the NotifyAppValidationOutput operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.NotifyAppValidationOutput
* @see AWS
* API Documentation
*/
NotifyAppValidationOutputResult notifyAppValidationOutput(NotifyAppValidationOutputRequest notifyAppValidationOutputRequest);
/**
*
* Creates or updates the launch configuration for the specified application.
*
*
* @param putAppLaunchConfigurationRequest
* @return Result of the PutAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.PutAppLaunchConfiguration
* @see AWS
* API Documentation
*/
PutAppLaunchConfigurationResult putAppLaunchConfiguration(PutAppLaunchConfigurationRequest putAppLaunchConfigurationRequest);
/**
*
* Creates or updates the replication configuration for the specified application.
*
*
* @param putAppReplicationConfigurationRequest
* @return Result of the PutAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.PutAppReplicationConfiguration
* @see AWS API Documentation
*/
PutAppReplicationConfigurationResult putAppReplicationConfiguration(PutAppReplicationConfigurationRequest putAppReplicationConfigurationRequest);
/**
*
* Creates or updates a validation configuration for the specified application.
*
*
* @param putAppValidationConfigurationRequest
* @return Result of the PutAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.PutAppValidationConfiguration
* @see AWS API Documentation
*/
PutAppValidationConfigurationResult putAppValidationConfiguration(PutAppValidationConfigurationRequest putAppValidationConfigurationRequest);
/**
*
* Starts replicating the specified application by creating replication jobs for each server in the application.
*
*
* @param startAppReplicationRequest
* @return Result of the StartAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.StartAppReplication
* @see AWS API
* Documentation
*/
StartAppReplicationResult startAppReplication(StartAppReplicationRequest startAppReplicationRequest);
/**
*
* Starts an on-demand replication run for the specified application.
*
*
* @param startOnDemandAppReplicationRequest
* @return Result of the StartOnDemandAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.StartOnDemandAppReplication
* @see AWS API Documentation
*/
StartOnDemandAppReplicationResult startOnDemandAppReplication(StartOnDemandAppReplicationRequest startOnDemandAppReplicationRequest);
/**
*
* Starts an on-demand replication run for the specified replication job. This replication run starts immediately.
* This replication run is in addition to the ones already scheduled.
*
*
* There is a limit on the number of on-demand replications runs that you can request in a 24-hour period.
*
*
* @param startOnDemandReplicationRunRequest
* @return Result of the StartOnDemandReplicationRun operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ReplicationRunLimitExceededException
* You have exceeded the number of on-demand replication runs you can request in a 24-hour period.
* @throws DryRunOperationException
* The user has the required permissions, so the request would have succeeded, but a dry run was performed.
* @sample AWSServerMigration.StartOnDemandReplicationRun
* @see AWS API Documentation
*/
StartOnDemandReplicationRunResult startOnDemandReplicationRun(StartOnDemandReplicationRunRequest startOnDemandReplicationRunRequest);
/**
*
* Stops replicating the specified application by deleting the replication job for each server in the application.
*
*
* @param stopAppReplicationRequest
* @return Result of the StopAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.StopAppReplication
* @see AWS API
* Documentation
*/
StopAppReplicationResult stopAppReplication(StopAppReplicationRequest stopAppReplicationRequest);
/**
*
* Terminates the stack for the specified application.
*
*
* @param terminateAppRequest
* @return Result of the TerminateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.TerminateApp
* @see AWS API
* Documentation
*/
TerminateAppResult terminateApp(TerminateAppRequest terminateAppRequest);
/**
*
* Updates the specified application.
*
*
* @param updateAppRequest
* @return Result of the UpdateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @sample AWSServerMigration.UpdateApp
* @see AWS API
* Documentation
*/
UpdateAppResult updateApp(UpdateAppRequest updateAppRequest);
/**
*
* Updates the specified settings for the specified replication job.
*
*
* @param updateReplicationJobRequest
* @return Result of the UpdateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws ServerCannotBeReplicatedException
* The specified server cannot be replicated.
* @throws ReplicationJobNotFoundException
* The specified replication job does not exist.
* @throws InternalErrorException
* An internal error occurred.
* @throws TemporarilyUnavailableException
* The service is temporarily unavailable.
* @sample AWSServerMigration.UpdateReplicationJob
* @see AWS API
* Documentation
*/
UpdateReplicationJobResult updateReplicationJob(UpdateReplicationJobRequest updateReplicationJobRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}