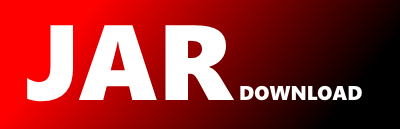
com.amazonaws.services.servicecatalog.AWSServiceCatalogAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-servicecatalog Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.servicecatalog;
import javax.annotation.Generated;
import com.amazonaws.services.servicecatalog.model.*;
/**
* Interface for accessing AWS Service Catalog asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.servicecatalog.AbstractAWSServiceCatalogAsync} instead.
*
*
* Service Catalog
*
* Service Catalog enables organizations to create and manage
* catalogs of IT services that are approved for Amazon Web Services. To get the most out of this documentation, you
* should be familiar with the terminology discussed in Service Catalog
* Concepts.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSServiceCatalogAsync extends AWSServiceCatalog {
/**
*
* Accepts an offer to share the specified portfolio.
*
*
* @param acceptPortfolioShareRequest
* @return A Java Future containing the result of the AcceptPortfolioShare operation returned by the service.
* @sample AWSServiceCatalogAsync.AcceptPortfolioShare
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptPortfolioShareAsync(AcceptPortfolioShareRequest acceptPortfolioShareRequest);
/**
*
* Accepts an offer to share the specified portfolio.
*
*
* @param acceptPortfolioShareRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptPortfolioShare operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.AcceptPortfolioShare
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptPortfolioShareAsync(AcceptPortfolioShareRequest acceptPortfolioShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified budget with the specified resource.
*
*
* @param associateBudgetWithResourceRequest
* @return A Java Future containing the result of the AssociateBudgetWithResource operation returned by the service.
* @sample AWSServiceCatalogAsync.AssociateBudgetWithResource
* @see AWS API Documentation
*/
java.util.concurrent.Future associateBudgetWithResourceAsync(
AssociateBudgetWithResourceRequest associateBudgetWithResourceRequest);
/**
*
* Associates the specified budget with the specified resource.
*
*
* @param associateBudgetWithResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateBudgetWithResource operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.AssociateBudgetWithResource
* @see AWS API Documentation
*/
java.util.concurrent.Future associateBudgetWithResourceAsync(
AssociateBudgetWithResourceRequest associateBudgetWithResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified principal ARN with the specified portfolio.
*
*
* If you share the portfolio with principal name sharing enabled, the PrincipalARN
association is
* included in the share.
*
*
* The PortfolioID
, PrincipalARN
, and PrincipalType
parameters are required.
*
*
* You can associate a maximum of 10 Principals with a portfolio using PrincipalType
as
* IAM_PATTERN
.
*
*
*
* When you associate a principal with portfolio, a potential privilege escalation path may occur when that
* portfolio is then shared with other accounts. For a user in a recipient account who is not an Service
* Catalog Admin, but still has the ability to create Principals (Users/Groups/Roles), that user could create a role
* that matches a principal name association for the portfolio. Although this user may not know which principal
* names are associated through Service Catalog, they may be able to guess the user. If this potential escalation
* path is a concern, then Service Catalog recommends using PrincipalType
as IAM
. With
* this configuration, the PrincipalARN
must already exist in the recipient account before it can be
* associated.
*
*
*
* @param associatePrincipalWithPortfolioRequest
* @return A Java Future containing the result of the AssociatePrincipalWithPortfolio operation returned by the
* service.
* @sample AWSServiceCatalogAsync.AssociatePrincipalWithPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future associatePrincipalWithPortfolioAsync(
AssociatePrincipalWithPortfolioRequest associatePrincipalWithPortfolioRequest);
/**
*
* Associates the specified principal ARN with the specified portfolio.
*
*
* If you share the portfolio with principal name sharing enabled, the PrincipalARN
association is
* included in the share.
*
*
* The PortfolioID
, PrincipalARN
, and PrincipalType
parameters are required.
*
*
* You can associate a maximum of 10 Principals with a portfolio using PrincipalType
as
* IAM_PATTERN
.
*
*
*
* When you associate a principal with portfolio, a potential privilege escalation path may occur when that
* portfolio is then shared with other accounts. For a user in a recipient account who is not an Service
* Catalog Admin, but still has the ability to create Principals (Users/Groups/Roles), that user could create a role
* that matches a principal name association for the portfolio. Although this user may not know which principal
* names are associated through Service Catalog, they may be able to guess the user. If this potential escalation
* path is a concern, then Service Catalog recommends using PrincipalType
as IAM
. With
* this configuration, the PrincipalARN
must already exist in the recipient account before it can be
* associated.
*
*
*
* @param associatePrincipalWithPortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociatePrincipalWithPortfolio operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.AssociatePrincipalWithPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future associatePrincipalWithPortfolioAsync(
AssociatePrincipalWithPortfolioRequest associatePrincipalWithPortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified product with the specified portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param associateProductWithPortfolioRequest
* @return A Java Future containing the result of the AssociateProductWithPortfolio operation returned by the
* service.
* @sample AWSServiceCatalogAsync.AssociateProductWithPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future associateProductWithPortfolioAsync(
AssociateProductWithPortfolioRequest associateProductWithPortfolioRequest);
/**
*
* Associates the specified product with the specified portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param associateProductWithPortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateProductWithPortfolio operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.AssociateProductWithPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future associateProductWithPortfolioAsync(
AssociateProductWithPortfolioRequest associateProductWithPortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a self-service action with a provisioning artifact.
*
*
* @param associateServiceActionWithProvisioningArtifactRequest
* @return A Java Future containing the result of the AssociateServiceActionWithProvisioningArtifact operation
* returned by the service.
* @sample AWSServiceCatalogAsync.AssociateServiceActionWithProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future associateServiceActionWithProvisioningArtifactAsync(
AssociateServiceActionWithProvisioningArtifactRequest associateServiceActionWithProvisioningArtifactRequest);
/**
*
* Associates a self-service action with a provisioning artifact.
*
*
* @param associateServiceActionWithProvisioningArtifactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateServiceActionWithProvisioningArtifact operation
* returned by the service.
* @sample AWSServiceCatalogAsyncHandler.AssociateServiceActionWithProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future associateServiceActionWithProvisioningArtifactAsync(
AssociateServiceActionWithProvisioningArtifactRequest associateServiceActionWithProvisioningArtifactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate the specified TagOption with the specified portfolio or product.
*
*
* @param associateTagOptionWithResourceRequest
* @return A Java Future containing the result of the AssociateTagOptionWithResource operation returned by the
* service.
* @sample AWSServiceCatalogAsync.AssociateTagOptionWithResource
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTagOptionWithResourceAsync(
AssociateTagOptionWithResourceRequest associateTagOptionWithResourceRequest);
/**
*
* Associate the specified TagOption with the specified portfolio or product.
*
*
* @param associateTagOptionWithResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateTagOptionWithResource operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.AssociateTagOptionWithResource
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTagOptionWithResourceAsync(
AssociateTagOptionWithResourceRequest associateTagOptionWithResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates multiple self-service actions with provisioning artifacts.
*
*
* @param batchAssociateServiceActionWithProvisioningArtifactRequest
* @return A Java Future containing the result of the BatchAssociateServiceActionWithProvisioningArtifact operation
* returned by the service.
* @sample AWSServiceCatalogAsync.BatchAssociateServiceActionWithProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAssociateServiceActionWithProvisioningArtifactAsync(
BatchAssociateServiceActionWithProvisioningArtifactRequest batchAssociateServiceActionWithProvisioningArtifactRequest);
/**
*
* Associates multiple self-service actions with provisioning artifacts.
*
*
* @param batchAssociateServiceActionWithProvisioningArtifactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchAssociateServiceActionWithProvisioningArtifact operation
* returned by the service.
* @sample AWSServiceCatalogAsyncHandler.BatchAssociateServiceActionWithProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAssociateServiceActionWithProvisioningArtifactAsync(
BatchAssociateServiceActionWithProvisioningArtifactRequest batchAssociateServiceActionWithProvisioningArtifactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a batch of self-service actions from the specified provisioning artifact.
*
*
* @param batchDisassociateServiceActionFromProvisioningArtifactRequest
* @return A Java Future containing the result of the BatchDisassociateServiceActionFromProvisioningArtifact
* operation returned by the service.
* @sample AWSServiceCatalogAsync.BatchDisassociateServiceActionFromProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisassociateServiceActionFromProvisioningArtifactAsync(
BatchDisassociateServiceActionFromProvisioningArtifactRequest batchDisassociateServiceActionFromProvisioningArtifactRequest);
/**
*
* Disassociates a batch of self-service actions from the specified provisioning artifact.
*
*
* @param batchDisassociateServiceActionFromProvisioningArtifactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDisassociateServiceActionFromProvisioningArtifact
* operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.BatchDisassociateServiceActionFromProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisassociateServiceActionFromProvisioningArtifactAsync(
BatchDisassociateServiceActionFromProvisioningArtifactRequest batchDisassociateServiceActionFromProvisioningArtifactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified source product to the specified target product or a new product.
*
*
* You can copy a product to the same account or another account. You can copy a product to the same Region or
* another Region. If you copy a product to another account, you must first share the product in a portfolio using
* CreatePortfolioShare.
*
*
* This operation is performed asynchronously. To track the progress of the operation, use
* DescribeCopyProductStatus.
*
*
* @param copyProductRequest
* @return A Java Future containing the result of the CopyProduct operation returned by the service.
* @sample AWSServiceCatalogAsync.CopyProduct
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyProductAsync(CopyProductRequest copyProductRequest);
/**
*
* Copies the specified source product to the specified target product or a new product.
*
*
* You can copy a product to the same account or another account. You can copy a product to the same Region or
* another Region. If you copy a product to another account, you must first share the product in a portfolio using
* CreatePortfolioShare.
*
*
* This operation is performed asynchronously. To track the progress of the operation, use
* DescribeCopyProductStatus.
*
*
* @param copyProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyProduct operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.CopyProduct
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyProductAsync(CopyProductRequest copyProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a constraint.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param createConstraintRequest
* @return A Java Future containing the result of the CreateConstraint operation returned by the service.
* @sample AWSServiceCatalogAsync.CreateConstraint
* @see AWS API Documentation
*/
java.util.concurrent.Future createConstraintAsync(CreateConstraintRequest createConstraintRequest);
/**
*
* Creates a constraint.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param createConstraintRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConstraint operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.CreateConstraint
* @see AWS API Documentation
*/
java.util.concurrent.Future createConstraintAsync(CreateConstraintRequest createConstraintRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param createPortfolioRequest
* @return A Java Future containing the result of the CreatePortfolio operation returned by the service.
* @sample AWSServiceCatalogAsync.CreatePortfolio
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createPortfolioAsync(CreatePortfolioRequest createPortfolioRequest);
/**
*
* Creates a portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param createPortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePortfolio operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.CreatePortfolio
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createPortfolioAsync(CreatePortfolioRequest createPortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Shares the specified portfolio with the specified account or organization node. Shares to an organization node
* can only be created by the management account of an organization or by a delegated administrator. You can share
* portfolios to an organization, an organizational unit, or a specific account.
*
*
* Note that if a delegated admin is de-registered, they can no longer create portfolio shares.
*
*
* AWSOrganizationsAccess
must be enabled in order to create a portfolio share to an organization node.
*
*
* You can't share a shared resource, including portfolios that contain a shared product.
*
*
* If the portfolio share with the specified account or organization node already exists, this action will have no
* effect and will not return an error. To update an existing share, you must use the
* UpdatePortfolioShare
API instead.
*
*
*
* When you associate a principal with portfolio, a potential privilege escalation path may occur when that
* portfolio is then shared with other accounts. For a user in a recipient account who is not an Service
* Catalog Admin, but still has the ability to create Principals (Users/Groups/Roles), that user could create a role
* that matches a principal name association for the portfolio. Although this user may not know which principal
* names are associated through Service Catalog, they may be able to guess the user. If this potential escalation
* path is a concern, then Service Catalog recommends using PrincipalType
as IAM
. With
* this configuration, the PrincipalARN
must already exist in the recipient account before it can be
* associated.
*
*
*
* @param createPortfolioShareRequest
* @return A Java Future containing the result of the CreatePortfolioShare operation returned by the service.
* @sample AWSServiceCatalogAsync.CreatePortfolioShare
* @see AWS API Documentation
*/
java.util.concurrent.Future createPortfolioShareAsync(CreatePortfolioShareRequest createPortfolioShareRequest);
/**
*
* Shares the specified portfolio with the specified account or organization node. Shares to an organization node
* can only be created by the management account of an organization or by a delegated administrator. You can share
* portfolios to an organization, an organizational unit, or a specific account.
*
*
* Note that if a delegated admin is de-registered, they can no longer create portfolio shares.
*
*
* AWSOrganizationsAccess
must be enabled in order to create a portfolio share to an organization node.
*
*
* You can't share a shared resource, including portfolios that contain a shared product.
*
*
* If the portfolio share with the specified account or organization node already exists, this action will have no
* effect and will not return an error. To update an existing share, you must use the
* UpdatePortfolioShare
API instead.
*
*
*
* When you associate a principal with portfolio, a potential privilege escalation path may occur when that
* portfolio is then shared with other accounts. For a user in a recipient account who is not an Service
* Catalog Admin, but still has the ability to create Principals (Users/Groups/Roles), that user could create a role
* that matches a principal name association for the portfolio. Although this user may not know which principal
* names are associated through Service Catalog, they may be able to guess the user. If this potential escalation
* path is a concern, then Service Catalog recommends using PrincipalType
as IAM
. With
* this configuration, the PrincipalARN
must already exist in the recipient account before it can be
* associated.
*
*
*
* @param createPortfolioShareRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePortfolioShare operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.CreatePortfolioShare
* @see AWS API Documentation
*/
java.util.concurrent.Future createPortfolioShareAsync(CreatePortfolioShareRequest createPortfolioShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a product.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* The user or role that performs this operation must have the cloudformation:GetTemplate
IAM policy
* permission. This policy permission is required when using the ImportFromPhysicalId
template source
* in the information data section.
*
*
* @param createProductRequest
* @return A Java Future containing the result of the CreateProduct operation returned by the service.
* @sample AWSServiceCatalogAsync.CreateProduct
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createProductAsync(CreateProductRequest createProductRequest);
/**
*
* Creates a product.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* The user or role that performs this operation must have the cloudformation:GetTemplate
IAM policy
* permission. This policy permission is required when using the ImportFromPhysicalId
template source
* in the information data section.
*
*
* @param createProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProduct operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.CreateProduct
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createProductAsync(CreateProductRequest createProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a plan.
*
*
* A plan includes the list of resources to be created (when provisioning a new product) or modified (when updating
* a provisioned product) when the plan is executed.
*
*
* You can create one plan for each provisioned product. To create a plan for an existing provisioned product, the
* product status must be AVAILABLE or TAINTED.
*
*
* To view the resource changes in the change set, use DescribeProvisionedProductPlan. To create or modify
* the provisioned product, use ExecuteProvisionedProductPlan.
*
*
* @param createProvisionedProductPlanRequest
* @return A Java Future containing the result of the CreateProvisionedProductPlan operation returned by the
* service.
* @sample AWSServiceCatalogAsync.CreateProvisionedProductPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future createProvisionedProductPlanAsync(
CreateProvisionedProductPlanRequest createProvisionedProductPlanRequest);
/**
*
* Creates a plan.
*
*
* A plan includes the list of resources to be created (when provisioning a new product) or modified (when updating
* a provisioned product) when the plan is executed.
*
*
* You can create one plan for each provisioned product. To create a plan for an existing provisioned product, the
* product status must be AVAILABLE or TAINTED.
*
*
* To view the resource changes in the change set, use DescribeProvisionedProductPlan. To create or modify
* the provisioned product, use ExecuteProvisionedProductPlan.
*
*
* @param createProvisionedProductPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProvisionedProductPlan operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.CreateProvisionedProductPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future createProvisionedProductPlanAsync(
CreateProvisionedProductPlanRequest createProvisionedProductPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a provisioning artifact (also known as a version) for the specified product.
*
*
* You cannot create a provisioning artifact for a product that was shared with you.
*
*
* The user or role that performs this operation must have the cloudformation:GetTemplate
IAM policy
* permission. This policy permission is required when using the ImportFromPhysicalId
template source
* in the information data section.
*
*
* @param createProvisioningArtifactRequest
* @return A Java Future containing the result of the CreateProvisioningArtifact operation returned by the service.
* @sample AWSServiceCatalogAsync.CreateProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future createProvisioningArtifactAsync(
CreateProvisioningArtifactRequest createProvisioningArtifactRequest);
/**
*
* Creates a provisioning artifact (also known as a version) for the specified product.
*
*
* You cannot create a provisioning artifact for a product that was shared with you.
*
*
* The user or role that performs this operation must have the cloudformation:GetTemplate
IAM policy
* permission. This policy permission is required when using the ImportFromPhysicalId
template source
* in the information data section.
*
*
* @param createProvisioningArtifactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProvisioningArtifact operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.CreateProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future createProvisioningArtifactAsync(
CreateProvisioningArtifactRequest createProvisioningArtifactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a self-service action.
*
*
* @param createServiceActionRequest
* @return A Java Future containing the result of the CreateServiceAction operation returned by the service.
* @sample AWSServiceCatalogAsync.CreateServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future createServiceActionAsync(CreateServiceActionRequest createServiceActionRequest);
/**
*
* Creates a self-service action.
*
*
* @param createServiceActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateServiceAction operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.CreateServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future createServiceActionAsync(CreateServiceActionRequest createServiceActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a TagOption.
*
*
* @param createTagOptionRequest
* @return A Java Future containing the result of the CreateTagOption operation returned by the service.
* @sample AWSServiceCatalogAsync.CreateTagOption
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTagOptionAsync(CreateTagOptionRequest createTagOptionRequest);
/**
*
* Creates a TagOption.
*
*
* @param createTagOptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTagOption operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.CreateTagOption
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTagOptionAsync(CreateTagOptionRequest createTagOptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified constraint.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param deleteConstraintRequest
* @return A Java Future containing the result of the DeleteConstraint operation returned by the service.
* @sample AWSServiceCatalogAsync.DeleteConstraint
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConstraintAsync(DeleteConstraintRequest deleteConstraintRequest);
/**
*
* Deletes the specified constraint.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param deleteConstraintRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConstraint operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DeleteConstraint
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConstraintAsync(DeleteConstraintRequest deleteConstraintRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified portfolio.
*
*
* You cannot delete a portfolio if it was shared with you or if it has associated products, users, constraints, or
* shared accounts.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param deletePortfolioRequest
* @return A Java Future containing the result of the DeletePortfolio operation returned by the service.
* @sample AWSServiceCatalogAsync.DeletePortfolio
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deletePortfolioAsync(DeletePortfolioRequest deletePortfolioRequest);
/**
*
* Deletes the specified portfolio.
*
*
* You cannot delete a portfolio if it was shared with you or if it has associated products, users, constraints, or
* shared accounts.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param deletePortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePortfolio operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DeletePortfolio
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deletePortfolioAsync(DeletePortfolioRequest deletePortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops sharing the specified portfolio with the specified account or organization node. Shares to an organization
* node can only be deleted by the management account of an organization or by a delegated administrator.
*
*
* Note that if a delegated admin is de-registered, portfolio shares created from that account are removed.
*
*
* @param deletePortfolioShareRequest
* @return A Java Future containing the result of the DeletePortfolioShare operation returned by the service.
* @sample AWSServiceCatalogAsync.DeletePortfolioShare
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePortfolioShareAsync(DeletePortfolioShareRequest deletePortfolioShareRequest);
/**
*
* Stops sharing the specified portfolio with the specified account or organization node. Shares to an organization
* node can only be deleted by the management account of an organization or by a delegated administrator.
*
*
* Note that if a delegated admin is de-registered, portfolio shares created from that account are removed.
*
*
* @param deletePortfolioShareRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePortfolioShare operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DeletePortfolioShare
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePortfolioShareAsync(DeletePortfolioShareRequest deletePortfolioShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified product.
*
*
* You cannot delete a product if it was shared with you or is associated with a portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param deleteProductRequest
* @return A Java Future containing the result of the DeleteProduct operation returned by the service.
* @sample AWSServiceCatalogAsync.DeleteProduct
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteProductAsync(DeleteProductRequest deleteProductRequest);
/**
*
* Deletes the specified product.
*
*
* You cannot delete a product if it was shared with you or is associated with a portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param deleteProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProduct operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DeleteProduct
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteProductAsync(DeleteProductRequest deleteProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified plan.
*
*
* @param deleteProvisionedProductPlanRequest
* @return A Java Future containing the result of the DeleteProvisionedProductPlan operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DeleteProvisionedProductPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProvisionedProductPlanAsync(
DeleteProvisionedProductPlanRequest deleteProvisionedProductPlanRequest);
/**
*
* Deletes the specified plan.
*
*
* @param deleteProvisionedProductPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProvisionedProductPlan operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DeleteProvisionedProductPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProvisionedProductPlanAsync(
DeleteProvisionedProductPlanRequest deleteProvisionedProductPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified provisioning artifact (also known as a version) for the specified product.
*
*
* You cannot delete a provisioning artifact associated with a product that was shared with you. You cannot delete
* the last provisioning artifact for a product, because a product must have at least one provisioning artifact.
*
*
* @param deleteProvisioningArtifactRequest
* @return A Java Future containing the result of the DeleteProvisioningArtifact operation returned by the service.
* @sample AWSServiceCatalogAsync.DeleteProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProvisioningArtifactAsync(
DeleteProvisioningArtifactRequest deleteProvisioningArtifactRequest);
/**
*
* Deletes the specified provisioning artifact (also known as a version) for the specified product.
*
*
* You cannot delete a provisioning artifact associated with a product that was shared with you. You cannot delete
* the last provisioning artifact for a product, because a product must have at least one provisioning artifact.
*
*
* @param deleteProvisioningArtifactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProvisioningArtifact operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DeleteProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProvisioningArtifactAsync(
DeleteProvisioningArtifactRequest deleteProvisioningArtifactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a self-service action.
*
*
* @param deleteServiceActionRequest
* @return A Java Future containing the result of the DeleteServiceAction operation returned by the service.
* @sample AWSServiceCatalogAsync.DeleteServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteServiceActionAsync(DeleteServiceActionRequest deleteServiceActionRequest);
/**
*
* Deletes a self-service action.
*
*
* @param deleteServiceActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteServiceAction operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DeleteServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteServiceActionAsync(DeleteServiceActionRequest deleteServiceActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified TagOption.
*
*
* You cannot delete a TagOption if it is associated with a product or portfolio.
*
*
* @param deleteTagOptionRequest
* @return A Java Future containing the result of the DeleteTagOption operation returned by the service.
* @sample AWSServiceCatalogAsync.DeleteTagOption
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTagOptionAsync(DeleteTagOptionRequest deleteTagOptionRequest);
/**
*
* Deletes the specified TagOption.
*
*
* You cannot delete a TagOption if it is associated with a product or portfolio.
*
*
* @param deleteTagOptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTagOption operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DeleteTagOption
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTagOptionAsync(DeleteTagOptionRequest deleteTagOptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified constraint.
*
*
* @param describeConstraintRequest
* @return A Java Future containing the result of the DescribeConstraint operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribeConstraint
* @see AWS API Documentation
*/
java.util.concurrent.Future describeConstraintAsync(DescribeConstraintRequest describeConstraintRequest);
/**
*
* Gets information about the specified constraint.
*
*
* @param describeConstraintRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConstraint operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeConstraint
* @see AWS API Documentation
*/
java.util.concurrent.Future describeConstraintAsync(DescribeConstraintRequest describeConstraintRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the status of the specified copy product operation.
*
*
* @param describeCopyProductStatusRequest
* @return A Java Future containing the result of the DescribeCopyProductStatus operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribeCopyProductStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCopyProductStatusAsync(
DescribeCopyProductStatusRequest describeCopyProductStatusRequest);
/**
*
* Gets the status of the specified copy product operation.
*
*
* @param describeCopyProductStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCopyProductStatus operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeCopyProductStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCopyProductStatusAsync(
DescribeCopyProductStatusRequest describeCopyProductStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param describePortfolioRequest
* @return A Java Future containing the result of the DescribePortfolio operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribePortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future describePortfolioAsync(DescribePortfolioRequest describePortfolioRequest);
/**
*
* Gets information about the specified portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param describePortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePortfolio operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribePortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future describePortfolioAsync(DescribePortfolioRequest describePortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the status of the specified portfolio share operation. This API can only be called by the management account
* in the organization or by a delegated admin.
*
*
* @param describePortfolioShareStatusRequest
* @return A Java Future containing the result of the DescribePortfolioShareStatus operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DescribePortfolioShareStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describePortfolioShareStatusAsync(
DescribePortfolioShareStatusRequest describePortfolioShareStatusRequest);
/**
*
* Gets the status of the specified portfolio share operation. This API can only be called by the management account
* in the organization or by a delegated admin.
*
*
* @param describePortfolioShareStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePortfolioShareStatus operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DescribePortfolioShareStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describePortfolioShareStatusAsync(
DescribePortfolioShareStatusRequest describePortfolioShareStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a summary of each of the portfolio shares that were created for the specified portfolio.
*
*
* You can use this API to determine which accounts or organizational nodes this portfolio have been shared, whether
* the recipient entity has imported the share, and whether TagOptions are included with the share.
*
*
* The PortfolioId
and Type
parameters are both required.
*
*
* @param describePortfolioSharesRequest
* @return A Java Future containing the result of the DescribePortfolioShares operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribePortfolioShares
* @see AWS API Documentation
*/
java.util.concurrent.Future describePortfolioSharesAsync(DescribePortfolioSharesRequest describePortfolioSharesRequest);
/**
*
* Returns a summary of each of the portfolio shares that were created for the specified portfolio.
*
*
* You can use this API to determine which accounts or organizational nodes this portfolio have been shared, whether
* the recipient entity has imported the share, and whether TagOptions are included with the share.
*
*
* The PortfolioId
and Type
parameters are both required.
*
*
* @param describePortfolioSharesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePortfolioShares operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribePortfolioShares
* @see AWS API Documentation
*/
java.util.concurrent.Future describePortfolioSharesAsync(DescribePortfolioSharesRequest describePortfolioSharesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified product.
*
*
*
* Running this operation with administrator access results in a failure. DescribeProductAsAdmin should be
* used instead.
*
*
*
* @param describeProductRequest
* @return A Java Future containing the result of the DescribeProduct operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribeProduct
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeProductAsync(DescribeProductRequest describeProductRequest);
/**
*
* Gets information about the specified product.
*
*
*
* Running this operation with administrator access results in a failure. DescribeProductAsAdmin should be
* used instead.
*
*
*
* @param describeProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProduct operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeProduct
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeProductAsync(DescribeProductRequest describeProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified product. This operation is run with administrator access.
*
*
* @param describeProductAsAdminRequest
* @return A Java Future containing the result of the DescribeProductAsAdmin operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribeProductAsAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProductAsAdminAsync(DescribeProductAsAdminRequest describeProductAsAdminRequest);
/**
*
* Gets information about the specified product. This operation is run with administrator access.
*
*
* @param describeProductAsAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProductAsAdmin operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeProductAsAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProductAsAdminAsync(DescribeProductAsAdminRequest describeProductAsAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified product.
*
*
* @param describeProductViewRequest
* @return A Java Future containing the result of the DescribeProductView operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribeProductView
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProductViewAsync(DescribeProductViewRequest describeProductViewRequest);
/**
*
* Gets information about the specified product.
*
*
* @param describeProductViewRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProductView operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeProductView
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProductViewAsync(DescribeProductViewRequest describeProductViewRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified provisioned product.
*
*
* @param describeProvisionedProductRequest
* DescribeProvisionedProductAPI input structure. AcceptLanguage - [Optional] The language code for
* localization. Id - [Optional] The provisioned product identifier. Name - [Optional] Another provisioned
* product identifier. Customers must provide either Id or Name.
* @return A Java Future containing the result of the DescribeProvisionedProduct operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribeProvisionedProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProvisionedProductAsync(
DescribeProvisionedProductRequest describeProvisionedProductRequest);
/**
*
* Gets information about the specified provisioned product.
*
*
* @param describeProvisionedProductRequest
* DescribeProvisionedProductAPI input structure. AcceptLanguage - [Optional] The language code for
* localization. Id - [Optional] The provisioned product identifier. Name - [Optional] Another provisioned
* product identifier. Customers must provide either Id or Name.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProvisionedProduct operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeProvisionedProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProvisionedProductAsync(
DescribeProvisionedProductRequest describeProvisionedProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the resource changes for the specified plan.
*
*
* @param describeProvisionedProductPlanRequest
* @return A Java Future containing the result of the DescribeProvisionedProductPlan operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DescribeProvisionedProductPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProvisionedProductPlanAsync(
DescribeProvisionedProductPlanRequest describeProvisionedProductPlanRequest);
/**
*
* Gets information about the resource changes for the specified plan.
*
*
* @param describeProvisionedProductPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProvisionedProductPlan operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DescribeProvisionedProductPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProvisionedProductPlanAsync(
DescribeProvisionedProductPlanRequest describeProvisionedProductPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified provisioning artifact (also known as a version) for the specified product.
*
*
* @param describeProvisioningArtifactRequest
* @return A Java Future containing the result of the DescribeProvisioningArtifact operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DescribeProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProvisioningArtifactAsync(
DescribeProvisioningArtifactRequest describeProvisioningArtifactRequest);
/**
*
* Gets information about the specified provisioning artifact (also known as a version) for the specified product.
*
*
* @param describeProvisioningArtifactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProvisioningArtifact operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DescribeProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProvisioningArtifactAsync(
DescribeProvisioningArtifactRequest describeProvisioningArtifactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the configuration required to provision the specified product using the specified
* provisioning artifact.
*
*
* If the output contains a TagOption key with an empty list of values, there is a TagOption conflict for that key.
* The end user cannot take action to fix the conflict, and launch is not blocked. In subsequent calls to
* ProvisionProduct, do not include conflicted TagOption keys as tags, or this causes the error
* "Parameter validation failed: Missing required parameter in Tags[N]:Value". Tag the provisioned
* product with the value sc-tagoption-conflict-portfolioId-productId
.
*
*
* @param describeProvisioningParametersRequest
* @return A Java Future containing the result of the DescribeProvisioningParameters operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DescribeProvisioningParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProvisioningParametersAsync(
DescribeProvisioningParametersRequest describeProvisioningParametersRequest);
/**
*
* Gets information about the configuration required to provision the specified product using the specified
* provisioning artifact.
*
*
* If the output contains a TagOption key with an empty list of values, there is a TagOption conflict for that key.
* The end user cannot take action to fix the conflict, and launch is not blocked. In subsequent calls to
* ProvisionProduct, do not include conflicted TagOption keys as tags, or this causes the error
* "Parameter validation failed: Missing required parameter in Tags[N]:Value". Tag the provisioned
* product with the value sc-tagoption-conflict-portfolioId-productId
.
*
*
* @param describeProvisioningParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProvisioningParameters operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DescribeProvisioningParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProvisioningParametersAsync(
DescribeProvisioningParametersRequest describeProvisioningParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified request operation.
*
*
* Use this operation after calling a request operation (for example, ProvisionProduct,
* TerminateProvisionedProduct, or UpdateProvisionedProduct).
*
*
*
* If a provisioned product was transferred to a new owner using UpdateProvisionedProductProperties, the new
* owner will be able to describe all past records for that product. The previous owner will no longer be able to
* describe the records, but will be able to use ListRecordHistory to see the product's history from when he
* was the owner.
*
*
*
* @param describeRecordRequest
* @return A Java Future containing the result of the DescribeRecord operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribeRecord
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeRecordAsync(DescribeRecordRequest describeRecordRequest);
/**
*
* Gets information about the specified request operation.
*
*
* Use this operation after calling a request operation (for example, ProvisionProduct,
* TerminateProvisionedProduct, or UpdateProvisionedProduct).
*
*
*
* If a provisioned product was transferred to a new owner using UpdateProvisionedProductProperties, the new
* owner will be able to describe all past records for that product. The previous owner will no longer be able to
* describe the records, but will be able to use ListRecordHistory to see the product's history from when he
* was the owner.
*
*
*
* @param describeRecordRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRecord operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeRecord
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeRecordAsync(DescribeRecordRequest describeRecordRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a self-service action.
*
*
* @param describeServiceActionRequest
* @return A Java Future containing the result of the DescribeServiceAction operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribeServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future describeServiceActionAsync(DescribeServiceActionRequest describeServiceActionRequest);
/**
*
* Describes a self-service action.
*
*
* @param describeServiceActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeServiceAction operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future describeServiceActionAsync(DescribeServiceActionRequest describeServiceActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Finds the default parameters for a specific self-service action on a specific provisioned product and returns a
* map of the results to the user.
*
*
* @param describeServiceActionExecutionParametersRequest
* @return A Java Future containing the result of the DescribeServiceActionExecutionParameters operation returned by
* the service.
* @sample AWSServiceCatalogAsync.DescribeServiceActionExecutionParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeServiceActionExecutionParametersAsync(
DescribeServiceActionExecutionParametersRequest describeServiceActionExecutionParametersRequest);
/**
*
* Finds the default parameters for a specific self-service action on a specific provisioned product and returns a
* map of the results to the user.
*
*
* @param describeServiceActionExecutionParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeServiceActionExecutionParameters operation returned by
* the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeServiceActionExecutionParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeServiceActionExecutionParametersAsync(
DescribeServiceActionExecutionParametersRequest describeServiceActionExecutionParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified TagOption.
*
*
* @param describeTagOptionRequest
* @return A Java Future containing the result of the DescribeTagOption operation returned by the service.
* @sample AWSServiceCatalogAsync.DescribeTagOption
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTagOptionAsync(DescribeTagOptionRequest describeTagOptionRequest);
/**
*
* Gets information about the specified TagOption.
*
*
* @param describeTagOptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTagOption operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DescribeTagOption
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTagOptionAsync(DescribeTagOptionRequest describeTagOptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disable portfolio sharing through the Organizations service. This command will not delete your current shares,
* but prevents you from creating new shares throughout your organization. Current shares are not kept in sync with
* your organization structure if the structure changes after calling this API. Only the management account in the
* organization can call this API.
*
*
* You cannot call this API if there are active delegated administrators in the organization.
*
*
* Note that a delegated administrator is not authorized to invoke DisableAWSOrganizationsAccess
.
*
*
*
* If you share an Service Catalog portfolio in an organization within Organizations, and then disable Organizations
* access for Service Catalog, the portfolio access permissions will not sync with the latest changes to the
* organization structure. Specifically, accounts that you removed from the organization after disabling Service
* Catalog access will retain access to the previously shared portfolio.
*
*
*
* @param disableAWSOrganizationsAccessRequest
* @return A Java Future containing the result of the DisableAWSOrganizationsAccess operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DisableAWSOrganizationsAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future disableAWSOrganizationsAccessAsync(
DisableAWSOrganizationsAccessRequest disableAWSOrganizationsAccessRequest);
/**
*
* Disable portfolio sharing through the Organizations service. This command will not delete your current shares,
* but prevents you from creating new shares throughout your organization. Current shares are not kept in sync with
* your organization structure if the structure changes after calling this API. Only the management account in the
* organization can call this API.
*
*
* You cannot call this API if there are active delegated administrators in the organization.
*
*
* Note that a delegated administrator is not authorized to invoke DisableAWSOrganizationsAccess
.
*
*
*
* If you share an Service Catalog portfolio in an organization within Organizations, and then disable Organizations
* access for Service Catalog, the portfolio access permissions will not sync with the latest changes to the
* organization structure. Specifically, accounts that you removed from the organization after disabling Service
* Catalog access will retain access to the previously shared portfolio.
*
*
*
* @param disableAWSOrganizationsAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableAWSOrganizationsAccess operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DisableAWSOrganizationsAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future disableAWSOrganizationsAccessAsync(
DisableAWSOrganizationsAccessRequest disableAWSOrganizationsAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified budget from the specified resource.
*
*
* @param disassociateBudgetFromResourceRequest
* @return A Java Future containing the result of the DisassociateBudgetFromResource operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DisassociateBudgetFromResource
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateBudgetFromResourceAsync(
DisassociateBudgetFromResourceRequest disassociateBudgetFromResourceRequest);
/**
*
* Disassociates the specified budget from the specified resource.
*
*
* @param disassociateBudgetFromResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateBudgetFromResource operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DisassociateBudgetFromResource
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateBudgetFromResourceAsync(
DisassociateBudgetFromResourceRequest disassociateBudgetFromResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a previously associated principal ARN from a specified portfolio.
*
*
* The PrincipalType
and PrincipalARN
must match the
* AssociatePrincipalWithPortfolio
call request details. For example, to disassociate an association
* created with a PrincipalARN
of PrincipalType
IAM you must use the
* PrincipalType
IAM when calling DisassociatePrincipalFromPortfolio
.
*
*
* For portfolios that have been shared with principal name sharing enabled: after disassociating a principal, share
* recipient accounts will no longer be able to provision products in this portfolio using a role matching the name
* of the associated principal.
*
*
* For more information, review associate-principal-with-portfolio in the Amazon Web Services CLI Command Reference.
*
*
*
* If you disassociate a principal from a portfolio, with PrincipalType as IAM
, the same principal will
* still have access to the portfolio if it matches one of the associated principals of type
* IAM_PATTERN
. To fully remove access for a principal, verify all the associated Principals of type
* IAM_PATTERN
, and then ensure you disassociate any IAM_PATTERN
principals that match the
* principal whose access you are removing.
*
*
*
* @param disassociatePrincipalFromPortfolioRequest
* @return A Java Future containing the result of the DisassociatePrincipalFromPortfolio operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DisassociatePrincipalFromPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociatePrincipalFromPortfolioAsync(
DisassociatePrincipalFromPortfolioRequest disassociatePrincipalFromPortfolioRequest);
/**
*
* Disassociates a previously associated principal ARN from a specified portfolio.
*
*
* The PrincipalType
and PrincipalARN
must match the
* AssociatePrincipalWithPortfolio
call request details. For example, to disassociate an association
* created with a PrincipalARN
of PrincipalType
IAM you must use the
* PrincipalType
IAM when calling DisassociatePrincipalFromPortfolio
.
*
*
* For portfolios that have been shared with principal name sharing enabled: after disassociating a principal, share
* recipient accounts will no longer be able to provision products in this portfolio using a role matching the name
* of the associated principal.
*
*
* For more information, review associate-principal-with-portfolio in the Amazon Web Services CLI Command Reference.
*
*
*
* If you disassociate a principal from a portfolio, with PrincipalType as IAM
, the same principal will
* still have access to the portfolio if it matches one of the associated principals of type
* IAM_PATTERN
. To fully remove access for a principal, verify all the associated Principals of type
* IAM_PATTERN
, and then ensure you disassociate any IAM_PATTERN
principals that match the
* principal whose access you are removing.
*
*
*
* @param disassociatePrincipalFromPortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociatePrincipalFromPortfolio operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DisassociatePrincipalFromPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociatePrincipalFromPortfolioAsync(
DisassociatePrincipalFromPortfolioRequest disassociatePrincipalFromPortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified product from the specified portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param disassociateProductFromPortfolioRequest
* @return A Java Future containing the result of the DisassociateProductFromPortfolio operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DisassociateProductFromPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateProductFromPortfolioAsync(
DisassociateProductFromPortfolioRequest disassociateProductFromPortfolioRequest);
/**
*
* Disassociates the specified product from the specified portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param disassociateProductFromPortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateProductFromPortfolio operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DisassociateProductFromPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateProductFromPortfolioAsync(
DisassociateProductFromPortfolioRequest disassociateProductFromPortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified self-service action association from the specified provisioning artifact.
*
*
* @param disassociateServiceActionFromProvisioningArtifactRequest
* @return A Java Future containing the result of the DisassociateServiceActionFromProvisioningArtifact operation
* returned by the service.
* @sample AWSServiceCatalogAsync.DisassociateServiceActionFromProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateServiceActionFromProvisioningArtifactAsync(
DisassociateServiceActionFromProvisioningArtifactRequest disassociateServiceActionFromProvisioningArtifactRequest);
/**
*
* Disassociates the specified self-service action association from the specified provisioning artifact.
*
*
* @param disassociateServiceActionFromProvisioningArtifactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateServiceActionFromProvisioningArtifact operation
* returned by the service.
* @sample AWSServiceCatalogAsyncHandler.DisassociateServiceActionFromProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateServiceActionFromProvisioningArtifactAsync(
DisassociateServiceActionFromProvisioningArtifactRequest disassociateServiceActionFromProvisioningArtifactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified TagOption from the specified resource.
*
*
* @param disassociateTagOptionFromResourceRequest
* @return A Java Future containing the result of the DisassociateTagOptionFromResource operation returned by the
* service.
* @sample AWSServiceCatalogAsync.DisassociateTagOptionFromResource
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateTagOptionFromResourceAsync(
DisassociateTagOptionFromResourceRequest disassociateTagOptionFromResourceRequest);
/**
*
* Disassociates the specified TagOption from the specified resource.
*
*
* @param disassociateTagOptionFromResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateTagOptionFromResource operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.DisassociateTagOptionFromResource
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateTagOptionFromResourceAsync(
DisassociateTagOptionFromResourceRequest disassociateTagOptionFromResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable portfolio sharing feature through Organizations. This API will allow Service Catalog to receive updates on
* your organization in order to sync your shares with the current structure. This API can only be called by the
* management account in the organization.
*
*
* When you call this API, Service Catalog calls organizations:EnableAWSServiceAccess
on your behalf so
* that your shares stay in sync with any changes in your Organizations structure.
*
*
* Note that a delegated administrator is not authorized to invoke EnableAWSOrganizationsAccess
.
*
*
*
* If you have previously disabled Organizations access for Service Catalog, and then enable access again, the
* portfolio access permissions might not sync with the latest changes to the organization structure. Specifically,
* accounts that you removed from the organization after disabling Service Catalog access, and before you enabled
* access again, can retain access to the previously shared portfolio. As a result, an account that has been removed
* from the organization might still be able to create or manage Amazon Web Services resources when it is no longer
* authorized to do so. Amazon Web Services is working to resolve this issue.
*
*
*
* @param enableAWSOrganizationsAccessRequest
* @return A Java Future containing the result of the EnableAWSOrganizationsAccess operation returned by the
* service.
* @sample AWSServiceCatalogAsync.EnableAWSOrganizationsAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future enableAWSOrganizationsAccessAsync(
EnableAWSOrganizationsAccessRequest enableAWSOrganizationsAccessRequest);
/**
*
* Enable portfolio sharing feature through Organizations. This API will allow Service Catalog to receive updates on
* your organization in order to sync your shares with the current structure. This API can only be called by the
* management account in the organization.
*
*
* When you call this API, Service Catalog calls organizations:EnableAWSServiceAccess
on your behalf so
* that your shares stay in sync with any changes in your Organizations structure.
*
*
* Note that a delegated administrator is not authorized to invoke EnableAWSOrganizationsAccess
.
*
*
*
* If you have previously disabled Organizations access for Service Catalog, and then enable access again, the
* portfolio access permissions might not sync with the latest changes to the organization structure. Specifically,
* accounts that you removed from the organization after disabling Service Catalog access, and before you enabled
* access again, can retain access to the previously shared portfolio. As a result, an account that has been removed
* from the organization might still be able to create or manage Amazon Web Services resources when it is no longer
* authorized to do so. Amazon Web Services is working to resolve this issue.
*
*
*
* @param enableAWSOrganizationsAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableAWSOrganizationsAccess operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.EnableAWSOrganizationsAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future enableAWSOrganizationsAccessAsync(
EnableAWSOrganizationsAccessRequest enableAWSOrganizationsAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provisions or modifies a product based on the resource changes for the specified plan.
*
*
* @param executeProvisionedProductPlanRequest
* @return A Java Future containing the result of the ExecuteProvisionedProductPlan operation returned by the
* service.
* @sample AWSServiceCatalogAsync.ExecuteProvisionedProductPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future executeProvisionedProductPlanAsync(
ExecuteProvisionedProductPlanRequest executeProvisionedProductPlanRequest);
/**
*
* Provisions or modifies a product based on the resource changes for the specified plan.
*
*
* @param executeProvisionedProductPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ExecuteProvisionedProductPlan operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.ExecuteProvisionedProductPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future executeProvisionedProductPlanAsync(
ExecuteProvisionedProductPlanRequest executeProvisionedProductPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Executes a self-service action against a provisioned product.
*
*
* @param executeProvisionedProductServiceActionRequest
* @return A Java Future containing the result of the ExecuteProvisionedProductServiceAction operation returned by
* the service.
* @sample AWSServiceCatalogAsync.ExecuteProvisionedProductServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future executeProvisionedProductServiceActionAsync(
ExecuteProvisionedProductServiceActionRequest executeProvisionedProductServiceActionRequest);
/**
*
* Executes a self-service action against a provisioned product.
*
*
* @param executeProvisionedProductServiceActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ExecuteProvisionedProductServiceAction operation returned by
* the service.
* @sample AWSServiceCatalogAsyncHandler.ExecuteProvisionedProductServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future executeProvisionedProductServiceActionAsync(
ExecuteProvisionedProductServiceActionRequest executeProvisionedProductServiceActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the Access Status for Organizations portfolio share feature. This API can only be called by the management
* account in the organization or by a delegated admin.
*
*
* @param getAWSOrganizationsAccessStatusRequest
* @return A Java Future containing the result of the GetAWSOrganizationsAccessStatus operation returned by the
* service.
* @sample AWSServiceCatalogAsync.GetAWSOrganizationsAccessStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getAWSOrganizationsAccessStatusAsync(
GetAWSOrganizationsAccessStatusRequest getAWSOrganizationsAccessStatusRequest);
/**
*
* Get the Access Status for Organizations portfolio share feature. This API can only be called by the management
* account in the organization or by a delegated admin.
*
*
* @param getAWSOrganizationsAccessStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAWSOrganizationsAccessStatus operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.GetAWSOrganizationsAccessStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getAWSOrganizationsAccessStatusAsync(
GetAWSOrganizationsAccessStatusRequest getAWSOrganizationsAccessStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This API takes either a ProvisonedProductId
or a ProvisionedProductName
, along with a
* list of one or more output keys, and responds with the key/value pairs of those outputs.
*
*
* @param getProvisionedProductOutputsRequest
* @return A Java Future containing the result of the GetProvisionedProductOutputs operation returned by the
* service.
* @sample AWSServiceCatalogAsync.GetProvisionedProductOutputs
* @see AWS API Documentation
*/
java.util.concurrent.Future getProvisionedProductOutputsAsync(
GetProvisionedProductOutputsRequest getProvisionedProductOutputsRequest);
/**
*
* This API takes either a ProvisonedProductId
or a ProvisionedProductName
, along with a
* list of one or more output keys, and responds with the key/value pairs of those outputs.
*
*
* @param getProvisionedProductOutputsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetProvisionedProductOutputs operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.GetProvisionedProductOutputs
* @see AWS API Documentation
*/
java.util.concurrent.Future getProvisionedProductOutputsAsync(
GetProvisionedProductOutputsRequest getProvisionedProductOutputsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Requests the import of a resource as an Service Catalog provisioned product that is associated to an Service
* Catalog product and provisioning artifact. Once imported, all supported governance actions are supported on the
* provisioned product.
*
*
* Resource import only supports CloudFormation stack ARNs. CloudFormation StackSets, and non-root nested stacks,
* are not supported.
*
*
* The CloudFormation stack must have one of the following statuses to be imported: CREATE_COMPLETE
,
* UPDATE_COMPLETE
, UPDATE_ROLLBACK_COMPLETE
, IMPORT_COMPLETE
, and
* IMPORT_ROLLBACK_COMPLETE
.
*
*
* Import of the resource requires that the CloudFormation stack template matches the associated Service Catalog
* product provisioning artifact.
*
*
*
* When you import an existing CloudFormation stack into a portfolio, Service Catalog does not apply the product's
* associated constraints during the import process. Service Catalog applies the constraints after you call
* UpdateProvisionedProduct
for the provisioned product.
*
*
*
* The user or role that performs this operation must have the cloudformation:GetTemplate
and
* cloudformation:DescribeStacks
IAM policy permissions.
*
*
* You can only import one provisioned product at a time. The product's CloudFormation stack must have the
* IMPORT_COMPLETE
status before you import another.
*
*
* @param importAsProvisionedProductRequest
* @return A Java Future containing the result of the ImportAsProvisionedProduct operation returned by the service.
* @sample AWSServiceCatalogAsync.ImportAsProvisionedProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future importAsProvisionedProductAsync(
ImportAsProvisionedProductRequest importAsProvisionedProductRequest);
/**
*
* Requests the import of a resource as an Service Catalog provisioned product that is associated to an Service
* Catalog product and provisioning artifact. Once imported, all supported governance actions are supported on the
* provisioned product.
*
*
* Resource import only supports CloudFormation stack ARNs. CloudFormation StackSets, and non-root nested stacks,
* are not supported.
*
*
* The CloudFormation stack must have one of the following statuses to be imported: CREATE_COMPLETE
,
* UPDATE_COMPLETE
, UPDATE_ROLLBACK_COMPLETE
, IMPORT_COMPLETE
, and
* IMPORT_ROLLBACK_COMPLETE
.
*
*
* Import of the resource requires that the CloudFormation stack template matches the associated Service Catalog
* product provisioning artifact.
*
*
*
* When you import an existing CloudFormation stack into a portfolio, Service Catalog does not apply the product's
* associated constraints during the import process. Service Catalog applies the constraints after you call
* UpdateProvisionedProduct
for the provisioned product.
*
*
*
* The user or role that performs this operation must have the cloudformation:GetTemplate
and
* cloudformation:DescribeStacks
IAM policy permissions.
*
*
* You can only import one provisioned product at a time. The product's CloudFormation stack must have the
* IMPORT_COMPLETE
status before you import another.
*
*
* @param importAsProvisionedProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ImportAsProvisionedProduct operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ImportAsProvisionedProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future importAsProvisionedProductAsync(
ImportAsProvisionedProductRequest importAsProvisionedProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all imported portfolios for which account-to-account shares were accepted by this account. By specifying
* the PortfolioShareType
, you can list portfolios for which organizational shares were accepted by
* this account.
*
*
* @param listAcceptedPortfolioSharesRequest
* @return A Java Future containing the result of the ListAcceptedPortfolioShares operation returned by the service.
* @sample AWSServiceCatalogAsync.ListAcceptedPortfolioShares
* @see AWS API Documentation
*/
java.util.concurrent.Future listAcceptedPortfolioSharesAsync(
ListAcceptedPortfolioSharesRequest listAcceptedPortfolioSharesRequest);
/**
*
* Lists all imported portfolios for which account-to-account shares were accepted by this account. By specifying
* the PortfolioShareType
, you can list portfolios for which organizational shares were accepted by
* this account.
*
*
* @param listAcceptedPortfolioSharesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAcceptedPortfolioShares operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListAcceptedPortfolioShares
* @see AWS API Documentation
*/
java.util.concurrent.Future listAcceptedPortfolioSharesAsync(
ListAcceptedPortfolioSharesRequest listAcceptedPortfolioSharesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the budgets associated to the specified resource.
*
*
* @param listBudgetsForResourceRequest
* @return A Java Future containing the result of the ListBudgetsForResource operation returned by the service.
* @sample AWSServiceCatalogAsync.ListBudgetsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listBudgetsForResourceAsync(ListBudgetsForResourceRequest listBudgetsForResourceRequest);
/**
*
* Lists all the budgets associated to the specified resource.
*
*
* @param listBudgetsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBudgetsForResource operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListBudgetsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listBudgetsForResourceAsync(ListBudgetsForResourceRequest listBudgetsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the constraints for the specified portfolio and product.
*
*
* @param listConstraintsForPortfolioRequest
* @return A Java Future containing the result of the ListConstraintsForPortfolio operation returned by the service.
* @sample AWSServiceCatalogAsync.ListConstraintsForPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future listConstraintsForPortfolioAsync(
ListConstraintsForPortfolioRequest listConstraintsForPortfolioRequest);
/**
*
* Lists the constraints for the specified portfolio and product.
*
*
* @param listConstraintsForPortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListConstraintsForPortfolio operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListConstraintsForPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future listConstraintsForPortfolioAsync(
ListConstraintsForPortfolioRequest listConstraintsForPortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the paths to the specified product. A path describes how the user gets access to a specified product and is
* necessary when provisioning a product. A path also determines the constraints that are put on a product. A path
* is dependent on a specific product, porfolio, and principal.
*
*
*
* When provisioning a product that's been added to a portfolio, you must grant your user, group, or role access to
* the portfolio. For more information, see Granting users
* access in the Service Catalog User Guide.
*
*
*
* @param listLaunchPathsRequest
* @return A Java Future containing the result of the ListLaunchPaths operation returned by the service.
* @sample AWSServiceCatalogAsync.ListLaunchPaths
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLaunchPathsAsync(ListLaunchPathsRequest listLaunchPathsRequest);
/**
*
* Lists the paths to the specified product. A path describes how the user gets access to a specified product and is
* necessary when provisioning a product. A path also determines the constraints that are put on a product. A path
* is dependent on a specific product, porfolio, and principal.
*
*
*
* When provisioning a product that's been added to a portfolio, you must grant your user, group, or role access to
* the portfolio. For more information, see Granting users
* access in the Service Catalog User Guide.
*
*
*
* @param listLaunchPathsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLaunchPaths operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListLaunchPaths
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLaunchPathsAsync(ListLaunchPathsRequest listLaunchPathsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the organization nodes that have access to the specified portfolio. This API can only be called by the
* management account in the organization or by a delegated admin.
*
*
* If a delegated admin is de-registered, they can no longer perform this operation.
*
*
* @param listOrganizationPortfolioAccessRequest
* @return A Java Future containing the result of the ListOrganizationPortfolioAccess operation returned by the
* service.
* @sample AWSServiceCatalogAsync.ListOrganizationPortfolioAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future listOrganizationPortfolioAccessAsync(
ListOrganizationPortfolioAccessRequest listOrganizationPortfolioAccessRequest);
/**
*
* Lists the organization nodes that have access to the specified portfolio. This API can only be called by the
* management account in the organization or by a delegated admin.
*
*
* If a delegated admin is de-registered, they can no longer perform this operation.
*
*
* @param listOrganizationPortfolioAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOrganizationPortfolioAccess operation returned by the
* service.
* @sample AWSServiceCatalogAsyncHandler.ListOrganizationPortfolioAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future listOrganizationPortfolioAccessAsync(
ListOrganizationPortfolioAccessRequest listOrganizationPortfolioAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the account IDs that have access to the specified portfolio.
*
*
* A delegated admin can list the accounts that have access to the shared portfolio. Note that if a delegated admin
* is de-registered, they can no longer perform this operation.
*
*
* @param listPortfolioAccessRequest
* @return A Java Future containing the result of the ListPortfolioAccess operation returned by the service.
* @sample AWSServiceCatalogAsync.ListPortfolioAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future listPortfolioAccessAsync(ListPortfolioAccessRequest listPortfolioAccessRequest);
/**
*
* Lists the account IDs that have access to the specified portfolio.
*
*
* A delegated admin can list the accounts that have access to the shared portfolio. Note that if a delegated admin
* is de-registered, they can no longer perform this operation.
*
*
* @param listPortfolioAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPortfolioAccess operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListPortfolioAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future listPortfolioAccessAsync(ListPortfolioAccessRequest listPortfolioAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all portfolios in the catalog.
*
*
* @param listPortfoliosRequest
* @return A Java Future containing the result of the ListPortfolios operation returned by the service.
* @sample AWSServiceCatalogAsync.ListPortfolios
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listPortfoliosAsync(ListPortfoliosRequest listPortfoliosRequest);
/**
*
* Lists all portfolios in the catalog.
*
*
* @param listPortfoliosRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPortfolios operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListPortfolios
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listPortfoliosAsync(ListPortfoliosRequest listPortfoliosRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all portfolios that the specified product is associated with.
*
*
* @param listPortfoliosForProductRequest
* @return A Java Future containing the result of the ListPortfoliosForProduct operation returned by the service.
* @sample AWSServiceCatalogAsync.ListPortfoliosForProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future listPortfoliosForProductAsync(ListPortfoliosForProductRequest listPortfoliosForProductRequest);
/**
*
* Lists all portfolios that the specified product is associated with.
*
*
* @param listPortfoliosForProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPortfoliosForProduct operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListPortfoliosForProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future listPortfoliosForProductAsync(ListPortfoliosForProductRequest listPortfoliosForProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all PrincipalARN
s and corresponding PrincipalType
s associated with the specified
* portfolio.
*
*
* @param listPrincipalsForPortfolioRequest
* @return A Java Future containing the result of the ListPrincipalsForPortfolio operation returned by the service.
* @sample AWSServiceCatalogAsync.ListPrincipalsForPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future listPrincipalsForPortfolioAsync(
ListPrincipalsForPortfolioRequest listPrincipalsForPortfolioRequest);
/**
*
* Lists all PrincipalARN
s and corresponding PrincipalType
s associated with the specified
* portfolio.
*
*
* @param listPrincipalsForPortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPrincipalsForPortfolio operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListPrincipalsForPortfolio
* @see AWS API Documentation
*/
java.util.concurrent.Future listPrincipalsForPortfolioAsync(
ListPrincipalsForPortfolioRequest listPrincipalsForPortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the plans for the specified provisioned product or all plans to which the user has access.
*
*
* @param listProvisionedProductPlansRequest
* @return A Java Future containing the result of the ListProvisionedProductPlans operation returned by the service.
* @sample AWSServiceCatalogAsync.ListProvisionedProductPlans
* @see AWS API Documentation
*/
java.util.concurrent.Future listProvisionedProductPlansAsync(
ListProvisionedProductPlansRequest listProvisionedProductPlansRequest);
/**
*
* Lists the plans for the specified provisioned product or all plans to which the user has access.
*
*
* @param listProvisionedProductPlansRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProvisionedProductPlans operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListProvisionedProductPlans
* @see AWS API Documentation
*/
java.util.concurrent.Future listProvisionedProductPlansAsync(
ListProvisionedProductPlansRequest listProvisionedProductPlansRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all provisioning artifacts (also known as versions) for the specified product.
*
*
* @param listProvisioningArtifactsRequest
* @return A Java Future containing the result of the ListProvisioningArtifacts operation returned by the service.
* @sample AWSServiceCatalogAsync.ListProvisioningArtifacts
* @see AWS API Documentation
*/
java.util.concurrent.Future listProvisioningArtifactsAsync(
ListProvisioningArtifactsRequest listProvisioningArtifactsRequest);
/**
*
* Lists all provisioning artifacts (also known as versions) for the specified product.
*
*
* @param listProvisioningArtifactsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProvisioningArtifacts operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListProvisioningArtifacts
* @see AWS API Documentation
*/
java.util.concurrent.Future listProvisioningArtifactsAsync(
ListProvisioningArtifactsRequest listProvisioningArtifactsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all provisioning artifacts (also known as versions) for the specified self-service action.
*
*
* @param listProvisioningArtifactsForServiceActionRequest
* @return A Java Future containing the result of the ListProvisioningArtifactsForServiceAction operation returned
* by the service.
* @sample AWSServiceCatalogAsync.ListProvisioningArtifactsForServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future listProvisioningArtifactsForServiceActionAsync(
ListProvisioningArtifactsForServiceActionRequest listProvisioningArtifactsForServiceActionRequest);
/**
*
* Lists all provisioning artifacts (also known as versions) for the specified self-service action.
*
*
* @param listProvisioningArtifactsForServiceActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProvisioningArtifactsForServiceAction operation returned
* by the service.
* @sample AWSServiceCatalogAsyncHandler.ListProvisioningArtifactsForServiceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future listProvisioningArtifactsForServiceActionAsync(
ListProvisioningArtifactsForServiceActionRequest listProvisioningArtifactsForServiceActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the specified requests or all performed requests.
*
*
* @param listRecordHistoryRequest
* @return A Java Future containing the result of the ListRecordHistory operation returned by the service.
* @sample AWSServiceCatalogAsync.ListRecordHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecordHistoryAsync(ListRecordHistoryRequest listRecordHistoryRequest);
/**
*
* Lists the specified requests or all performed requests.
*
*
* @param listRecordHistoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRecordHistory operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListRecordHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecordHistoryAsync(ListRecordHistoryRequest listRecordHistoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the resources associated with the specified TagOption.
*
*
* @param listResourcesForTagOptionRequest
* @return A Java Future containing the result of the ListResourcesForTagOption operation returned by the service.
* @sample AWSServiceCatalogAsync.ListResourcesForTagOption
* @see AWS API Documentation
*/
java.util.concurrent.Future listResourcesForTagOptionAsync(
ListResourcesForTagOptionRequest listResourcesForTagOptionRequest);
/**
*
* Lists the resources associated with the specified TagOption.
*
*
* @param listResourcesForTagOptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListResourcesForTagOption operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListResourcesForTagOption
* @see AWS API Documentation
*/
java.util.concurrent.Future listResourcesForTagOptionAsync(
ListResourcesForTagOptionRequest listResourcesForTagOptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all self-service actions.
*
*
* @param listServiceActionsRequest
* @return A Java Future containing the result of the ListServiceActions operation returned by the service.
* @sample AWSServiceCatalogAsync.ListServiceActions
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceActionsAsync(ListServiceActionsRequest listServiceActionsRequest);
/**
*
* Lists all self-service actions.
*
*
* @param listServiceActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServiceActions operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListServiceActions
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceActionsAsync(ListServiceActionsRequest listServiceActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of self-service actions associated with the specified Product ID and Provisioning
* Artifact ID.
*
*
* @param listServiceActionsForProvisioningArtifactRequest
* @return A Java Future containing the result of the ListServiceActionsForProvisioningArtifact operation returned
* by the service.
* @sample AWSServiceCatalogAsync.ListServiceActionsForProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceActionsForProvisioningArtifactAsync(
ListServiceActionsForProvisioningArtifactRequest listServiceActionsForProvisioningArtifactRequest);
/**
*
* Returns a paginated list of self-service actions associated with the specified Product ID and Provisioning
* Artifact ID.
*
*
* @param listServiceActionsForProvisioningArtifactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServiceActionsForProvisioningArtifact operation returned
* by the service.
* @sample AWSServiceCatalogAsyncHandler.ListServiceActionsForProvisioningArtifact
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceActionsForProvisioningArtifactAsync(
ListServiceActionsForProvisioningArtifactRequest listServiceActionsForProvisioningArtifactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns summary information about stack instances that are associated with the specified
* CFN_STACKSET
type provisioned product. You can filter for stack instances that are associated with a
* specific Amazon Web Services account name or Region.
*
*
* @param listStackInstancesForProvisionedProductRequest
* @return A Java Future containing the result of the ListStackInstancesForProvisionedProduct operation returned by
* the service.
* @sample AWSServiceCatalogAsync.ListStackInstancesForProvisionedProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackInstancesForProvisionedProductAsync(
ListStackInstancesForProvisionedProductRequest listStackInstancesForProvisionedProductRequest);
/**
*
* Returns summary information about stack instances that are associated with the specified
* CFN_STACKSET
type provisioned product. You can filter for stack instances that are associated with a
* specific Amazon Web Services account name or Region.
*
*
* @param listStackInstancesForProvisionedProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStackInstancesForProvisionedProduct operation returned by
* the service.
* @sample AWSServiceCatalogAsyncHandler.ListStackInstancesForProvisionedProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackInstancesForProvisionedProductAsync(
ListStackInstancesForProvisionedProductRequest listStackInstancesForProvisionedProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the specified TagOptions or all TagOptions.
*
*
* @param listTagOptionsRequest
* @return A Java Future containing the result of the ListTagOptions operation returned by the service.
* @sample AWSServiceCatalogAsync.ListTagOptions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagOptionsAsync(ListTagOptionsRequest listTagOptionsRequest);
/**
*
* Lists the specified TagOptions or all TagOptions.
*
*
* @param listTagOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagOptions operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ListTagOptions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagOptionsAsync(ListTagOptionsRequest listTagOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Notifies the result of the provisioning engine execution.
*
*
* @param notifyProvisionProductEngineWorkflowResultRequest
* @return A Java Future containing the result of the NotifyProvisionProductEngineWorkflowResult operation returned
* by the service.
* @sample AWSServiceCatalogAsync.NotifyProvisionProductEngineWorkflowResult
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyProvisionProductEngineWorkflowResultAsync(
NotifyProvisionProductEngineWorkflowResultRequest notifyProvisionProductEngineWorkflowResultRequest);
/**
*
* Notifies the result of the provisioning engine execution.
*
*
* @param notifyProvisionProductEngineWorkflowResultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the NotifyProvisionProductEngineWorkflowResult operation returned
* by the service.
* @sample AWSServiceCatalogAsyncHandler.NotifyProvisionProductEngineWorkflowResult
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyProvisionProductEngineWorkflowResultAsync(
NotifyProvisionProductEngineWorkflowResultRequest notifyProvisionProductEngineWorkflowResultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Notifies the result of the terminate engine execution.
*
*
* @param notifyTerminateProvisionedProductEngineWorkflowResultRequest
* @return A Java Future containing the result of the NotifyTerminateProvisionedProductEngineWorkflowResult
* operation returned by the service.
* @sample AWSServiceCatalogAsync.NotifyTerminateProvisionedProductEngineWorkflowResult
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyTerminateProvisionedProductEngineWorkflowResultAsync(
NotifyTerminateProvisionedProductEngineWorkflowResultRequest notifyTerminateProvisionedProductEngineWorkflowResultRequest);
/**
*
* Notifies the result of the terminate engine execution.
*
*
* @param notifyTerminateProvisionedProductEngineWorkflowResultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the NotifyTerminateProvisionedProductEngineWorkflowResult
* operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.NotifyTerminateProvisionedProductEngineWorkflowResult
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyTerminateProvisionedProductEngineWorkflowResultAsync(
NotifyTerminateProvisionedProductEngineWorkflowResultRequest notifyTerminateProvisionedProductEngineWorkflowResultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Notifies the result of the update engine execution.
*
*
* @param notifyUpdateProvisionedProductEngineWorkflowResultRequest
* @return A Java Future containing the result of the NotifyUpdateProvisionedProductEngineWorkflowResult operation
* returned by the service.
* @sample AWSServiceCatalogAsync.NotifyUpdateProvisionedProductEngineWorkflowResult
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyUpdateProvisionedProductEngineWorkflowResultAsync(
NotifyUpdateProvisionedProductEngineWorkflowResultRequest notifyUpdateProvisionedProductEngineWorkflowResultRequest);
/**
*
* Notifies the result of the update engine execution.
*
*
* @param notifyUpdateProvisionedProductEngineWorkflowResultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the NotifyUpdateProvisionedProductEngineWorkflowResult operation
* returned by the service.
* @sample AWSServiceCatalogAsyncHandler.NotifyUpdateProvisionedProductEngineWorkflowResult
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyUpdateProvisionedProductEngineWorkflowResultAsync(
NotifyUpdateProvisionedProductEngineWorkflowResultRequest notifyUpdateProvisionedProductEngineWorkflowResultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provisions the specified product.
*
*
* A provisioned product is a resourced instance of a product. For example, provisioning a product that's based on
* an CloudFormation template launches an CloudFormation stack and its underlying resources. You can check the
* status of this request using DescribeRecord.
*
*
* If the request contains a tag key with an empty list of values, there's a tag conflict for that key. Don't
* include conflicted keys as tags, or this will cause the error
* "Parameter validation failed: Missing required parameter in Tags[N]:Value".
*
*
*
* When provisioning a product that's been added to a portfolio, you must grant your user, group, or role access to
* the portfolio. For more information, see Granting users
* access in the Service Catalog User Guide.
*
*
*
* @param provisionProductRequest
* @return A Java Future containing the result of the ProvisionProduct operation returned by the service.
* @sample AWSServiceCatalogAsync.ProvisionProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future provisionProductAsync(ProvisionProductRequest provisionProductRequest);
/**
*
* Provisions the specified product.
*
*
* A provisioned product is a resourced instance of a product. For example, provisioning a product that's based on
* an CloudFormation template launches an CloudFormation stack and its underlying resources. You can check the
* status of this request using DescribeRecord.
*
*
* If the request contains a tag key with an empty list of values, there's a tag conflict for that key. Don't
* include conflicted keys as tags, or this will cause the error
* "Parameter validation failed: Missing required parameter in Tags[N]:Value".
*
*
*
* When provisioning a product that's been added to a portfolio, you must grant your user, group, or role access to
* the portfolio. For more information, see Granting users
* access in the Service Catalog User Guide.
*
*
*
* @param provisionProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ProvisionProduct operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ProvisionProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future provisionProductAsync(ProvisionProductRequest provisionProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Rejects an offer to share the specified portfolio.
*
*
* @param rejectPortfolioShareRequest
* @return A Java Future containing the result of the RejectPortfolioShare operation returned by the service.
* @sample AWSServiceCatalogAsync.RejectPortfolioShare
* @see AWS API Documentation
*/
java.util.concurrent.Future rejectPortfolioShareAsync(RejectPortfolioShareRequest rejectPortfolioShareRequest);
/**
*
* Rejects an offer to share the specified portfolio.
*
*
* @param rejectPortfolioShareRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RejectPortfolioShare operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.RejectPortfolioShare
* @see AWS API Documentation
*/
java.util.concurrent.Future rejectPortfolioShareAsync(RejectPortfolioShareRequest rejectPortfolioShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the provisioned products that are available (not terminated).
*
*
* To use additional filtering, see SearchProvisionedProducts.
*
*
* @param scanProvisionedProductsRequest
* @return A Java Future containing the result of the ScanProvisionedProducts operation returned by the service.
* @sample AWSServiceCatalogAsync.ScanProvisionedProducts
* @see AWS API Documentation
*/
java.util.concurrent.Future scanProvisionedProductsAsync(ScanProvisionedProductsRequest scanProvisionedProductsRequest);
/**
*
* Lists the provisioned products that are available (not terminated).
*
*
* To use additional filtering, see SearchProvisionedProducts.
*
*
* @param scanProvisionedProductsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ScanProvisionedProducts operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.ScanProvisionedProducts
* @see AWS API Documentation
*/
java.util.concurrent.Future scanProvisionedProductsAsync(ScanProvisionedProductsRequest scanProvisionedProductsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the products to which the caller has access.
*
*
* @param searchProductsRequest
* @return A Java Future containing the result of the SearchProducts operation returned by the service.
* @sample AWSServiceCatalogAsync.SearchProducts
* @see AWS
* API Documentation
*/
java.util.concurrent.Future searchProductsAsync(SearchProductsRequest searchProductsRequest);
/**
*
* Gets information about the products to which the caller has access.
*
*
* @param searchProductsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchProducts operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.SearchProducts
* @see AWS
* API Documentation
*/
java.util.concurrent.Future searchProductsAsync(SearchProductsRequest searchProductsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the products for the specified portfolio or all products.
*
*
* @param searchProductsAsAdminRequest
* @return A Java Future containing the result of the SearchProductsAsAdmin operation returned by the service.
* @sample AWSServiceCatalogAsync.SearchProductsAsAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future searchProductsAsAdminAsync(SearchProductsAsAdminRequest searchProductsAsAdminRequest);
/**
*
* Gets information about the products for the specified portfolio or all products.
*
*
* @param searchProductsAsAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchProductsAsAdmin operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.SearchProductsAsAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future searchProductsAsAdminAsync(SearchProductsAsAdminRequest searchProductsAsAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the provisioned products that meet the specified criteria.
*
*
* @param searchProvisionedProductsRequest
* @return A Java Future containing the result of the SearchProvisionedProducts operation returned by the service.
* @sample AWSServiceCatalogAsync.SearchProvisionedProducts
* @see AWS API Documentation
*/
java.util.concurrent.Future searchProvisionedProductsAsync(
SearchProvisionedProductsRequest searchProvisionedProductsRequest);
/**
*
* Gets information about the provisioned products that meet the specified criteria.
*
*
* @param searchProvisionedProductsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchProvisionedProducts operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.SearchProvisionedProducts
* @see AWS API Documentation
*/
java.util.concurrent.Future searchProvisionedProductsAsync(
SearchProvisionedProductsRequest searchProvisionedProductsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Terminates the specified provisioned product.
*
*
* This operation does not delete any records associated with the provisioned product.
*
*
* You can check the status of this request using DescribeRecord.
*
*
* @param terminateProvisionedProductRequest
* @return A Java Future containing the result of the TerminateProvisionedProduct operation returned by the service.
* @sample AWSServiceCatalogAsync.TerminateProvisionedProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future terminateProvisionedProductAsync(
TerminateProvisionedProductRequest terminateProvisionedProductRequest);
/**
*
* Terminates the specified provisioned product.
*
*
* This operation does not delete any records associated with the provisioned product.
*
*
* You can check the status of this request using DescribeRecord.
*
*
* @param terminateProvisionedProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TerminateProvisionedProduct operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.TerminateProvisionedProduct
* @see AWS API Documentation
*/
java.util.concurrent.Future terminateProvisionedProductAsync(
TerminateProvisionedProductRequest terminateProvisionedProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified constraint.
*
*
* @param updateConstraintRequest
* @return A Java Future containing the result of the UpdateConstraint operation returned by the service.
* @sample AWSServiceCatalogAsync.UpdateConstraint
* @see AWS API Documentation
*/
java.util.concurrent.Future updateConstraintAsync(UpdateConstraintRequest updateConstraintRequest);
/**
*
* Updates the specified constraint.
*
*
* @param updateConstraintRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateConstraint operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.UpdateConstraint
* @see AWS API Documentation
*/
java.util.concurrent.Future updateConstraintAsync(UpdateConstraintRequest updateConstraintRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified portfolio.
*
*
* You cannot update a product that was shared with you.
*
*
* @param updatePortfolioRequest
* @return A Java Future containing the result of the UpdatePortfolio operation returned by the service.
* @sample AWSServiceCatalogAsync.UpdatePortfolio
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updatePortfolioAsync(UpdatePortfolioRequest updatePortfolioRequest);
/**
*
* Updates the specified portfolio.
*
*
* You cannot update a product that was shared with you.
*
*
* @param updatePortfolioRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePortfolio operation returned by the service.
* @sample AWSServiceCatalogAsyncHandler.UpdatePortfolio
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updatePortfolioAsync(UpdatePortfolioRequest updatePortfolioRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified portfolio share. You can use this API to enable or disable