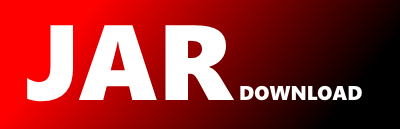
com.amazonaws.services.servicecatalog.AWSServiceCatalogClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-servicecatalog Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.servicecatalog;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.servicecatalog.AWSServiceCatalogClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.servicecatalog.model.*;
import com.amazonaws.services.servicecatalog.model.transform.*;
/**
* Client for accessing AWS Service Catalog. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
* Service Catalog
*
* Service Catalog enables organizations to create and manage
* catalogs of IT services that are approved for Amazon Web Services. To get the most out of this documentation, you
* should be familiar with the terminology discussed in Service Catalog
* Concepts.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSServiceCatalogClient extends AmazonWebServiceClient implements AWSServiceCatalog {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSServiceCatalog.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "servicecatalog";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParametersException").withExceptionUnmarshaller(
com.amazonaws.services.servicecatalog.model.transform.InvalidParametersExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("OperationNotSupportedException").withExceptionUnmarshaller(
com.amazonaws.services.servicecatalog.model.transform.OperationNotSupportedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidStateException").withExceptionUnmarshaller(
com.amazonaws.services.servicecatalog.model.transform.InvalidStateExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DuplicateResourceException").withExceptionUnmarshaller(
com.amazonaws.services.servicecatalog.model.transform.DuplicateResourceExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.servicecatalog.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.servicecatalog.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TagOptionNotMigratedException").withExceptionUnmarshaller(
com.amazonaws.services.servicecatalog.model.transform.TagOptionNotMigratedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceInUseException").withExceptionUnmarshaller(
com.amazonaws.services.servicecatalog.model.transform.ResourceInUseExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.servicecatalog.model.AWSServiceCatalogException.class));
/**
* Constructs a new client to invoke service methods on AWS Service Catalog. A credentials provider chain will be
* used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSServiceCatalogClientBuilder#defaultClient()}
*/
@Deprecated
public AWSServiceCatalogClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS Service Catalog. A credentials provider chain will be
* used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWS Service Catalog (ex: proxy
* settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSServiceCatalogClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSServiceCatalogClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on AWS Service Catalog using the specified AWS account
* credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AWSServiceCatalogClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AWSServiceCatalogClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AWSServiceCatalogClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS Service Catalog using the specified AWS account
* credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWS Service Catalog (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AWSServiceCatalogClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSServiceCatalogClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSServiceCatalogClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on AWS Service Catalog using the specified AWS account
* credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AWSServiceCatalogClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AWSServiceCatalogClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS Service Catalog using the specified AWS account
* credentials provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWS Service Catalog (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AWSServiceCatalogClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSServiceCatalogClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSServiceCatalogClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on AWS Service Catalog using the specified AWS account
* credentials provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWS Service Catalog (ex: proxy
* settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AWSServiceCatalogClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSServiceCatalogClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AWSServiceCatalogClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AWSServiceCatalogClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AWSServiceCatalogClientBuilder builder() {
return AWSServiceCatalogClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS Service Catalog using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSServiceCatalogClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS Service Catalog using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSServiceCatalogClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("servicecatalog.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/servicecatalog/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/servicecatalog/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Accepts an offer to share the specified portfolio.
*
*
* @param acceptPortfolioShareRequest
* @return Result of the AcceptPortfolioShare operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @sample AWSServiceCatalog.AcceptPortfolioShare
* @see AWS API Documentation
*/
@Override
public AcceptPortfolioShareResult acceptPortfolioShare(AcceptPortfolioShareRequest request) {
request = beforeClientExecution(request);
return executeAcceptPortfolioShare(request);
}
@SdkInternalApi
final AcceptPortfolioShareResult executeAcceptPortfolioShare(AcceptPortfolioShareRequest acceptPortfolioShareRequest) {
ExecutionContext executionContext = createExecutionContext(acceptPortfolioShareRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AcceptPortfolioShareRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(acceptPortfolioShareRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AcceptPortfolioShare");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AcceptPortfolioShareResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates the specified budget with the specified resource.
*
*
* @param associateBudgetWithResourceRequest
* @return Result of the AssociateBudgetWithResource operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws DuplicateResourceException
* The specified resource is a duplicate.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.AssociateBudgetWithResource
* @see AWS API Documentation
*/
@Override
public AssociateBudgetWithResourceResult associateBudgetWithResource(AssociateBudgetWithResourceRequest request) {
request = beforeClientExecution(request);
return executeAssociateBudgetWithResource(request);
}
@SdkInternalApi
final AssociateBudgetWithResourceResult executeAssociateBudgetWithResource(AssociateBudgetWithResourceRequest associateBudgetWithResourceRequest) {
ExecutionContext executionContext = createExecutionContext(associateBudgetWithResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateBudgetWithResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateBudgetWithResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateBudgetWithResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateBudgetWithResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates the specified principal ARN with the specified portfolio.
*
*
* If you share the portfolio with principal name sharing enabled, the PrincipalARN
association is
* included in the share.
*
*
* The PortfolioID
, PrincipalARN
, and PrincipalType
parameters are required.
*
*
* You can associate a maximum of 10 Principals with a portfolio using PrincipalType
as
* IAM_PATTERN
.
*
*
*
* When you associate a principal with portfolio, a potential privilege escalation path may occur when that
* portfolio is then shared with other accounts. For a user in a recipient account who is not an Service
* Catalog Admin, but still has the ability to create Principals (Users/Groups/Roles), that user could create a role
* that matches a principal name association for the portfolio. Although this user may not know which principal
* names are associated through Service Catalog, they may be able to guess the user. If this potential escalation
* path is a concern, then Service Catalog recommends using PrincipalType
as IAM
. With
* this configuration, the PrincipalARN
must already exist in the recipient account before it can be
* associated.
*
*
*
* @param associatePrincipalWithPortfolioRequest
* @return Result of the AssociatePrincipalWithPortfolio operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @sample AWSServiceCatalog.AssociatePrincipalWithPortfolio
* @see AWS API Documentation
*/
@Override
public AssociatePrincipalWithPortfolioResult associatePrincipalWithPortfolio(AssociatePrincipalWithPortfolioRequest request) {
request = beforeClientExecution(request);
return executeAssociatePrincipalWithPortfolio(request);
}
@SdkInternalApi
final AssociatePrincipalWithPortfolioResult executeAssociatePrincipalWithPortfolio(
AssociatePrincipalWithPortfolioRequest associatePrincipalWithPortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(associatePrincipalWithPortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociatePrincipalWithPortfolioRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associatePrincipalWithPortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociatePrincipalWithPortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociatePrincipalWithPortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates the specified product with the specified portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param associateProductWithPortfolioRequest
* @return Result of the AssociateProductWithPortfolio operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @sample AWSServiceCatalog.AssociateProductWithPortfolio
* @see AWS API Documentation
*/
@Override
public AssociateProductWithPortfolioResult associateProductWithPortfolio(AssociateProductWithPortfolioRequest request) {
request = beforeClientExecution(request);
return executeAssociateProductWithPortfolio(request);
}
@SdkInternalApi
final AssociateProductWithPortfolioResult executeAssociateProductWithPortfolio(AssociateProductWithPortfolioRequest associateProductWithPortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(associateProductWithPortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateProductWithPortfolioRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateProductWithPortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateProductWithPortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateProductWithPortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a self-service action with a provisioning artifact.
*
*
* @param associateServiceActionWithProvisioningArtifactRequest
* @return Result of the AssociateServiceActionWithProvisioningArtifact operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws DuplicateResourceException
* The specified resource is a duplicate.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.AssociateServiceActionWithProvisioningArtifact
* @see AWS API Documentation
*/
@Override
public AssociateServiceActionWithProvisioningArtifactResult associateServiceActionWithProvisioningArtifact(
AssociateServiceActionWithProvisioningArtifactRequest request) {
request = beforeClientExecution(request);
return executeAssociateServiceActionWithProvisioningArtifact(request);
}
@SdkInternalApi
final AssociateServiceActionWithProvisioningArtifactResult executeAssociateServiceActionWithProvisioningArtifact(
AssociateServiceActionWithProvisioningArtifactRequest associateServiceActionWithProvisioningArtifactRequest) {
ExecutionContext executionContext = createExecutionContext(associateServiceActionWithProvisioningArtifactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateServiceActionWithProvisioningArtifactRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateServiceActionWithProvisioningArtifactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateServiceActionWithProvisioningArtifact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateServiceActionWithProvisioningArtifactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associate the specified TagOption with the specified portfolio or product.
*
*
* @param associateTagOptionWithResourceRequest
* @return Result of the AssociateTagOptionWithResource operation returned by the service.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @throws DuplicateResourceException
* The specified resource is a duplicate.
* @throws InvalidStateException
* An attempt was made to modify a resource that is in a state that is not valid. Check your resources to
* ensure that they are in valid states before retrying the operation.
* @sample AWSServiceCatalog.AssociateTagOptionWithResource
* @see AWS API Documentation
*/
@Override
public AssociateTagOptionWithResourceResult associateTagOptionWithResource(AssociateTagOptionWithResourceRequest request) {
request = beforeClientExecution(request);
return executeAssociateTagOptionWithResource(request);
}
@SdkInternalApi
final AssociateTagOptionWithResourceResult executeAssociateTagOptionWithResource(AssociateTagOptionWithResourceRequest associateTagOptionWithResourceRequest) {
ExecutionContext executionContext = createExecutionContext(associateTagOptionWithResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateTagOptionWithResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateTagOptionWithResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateTagOptionWithResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateTagOptionWithResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates multiple self-service actions with provisioning artifacts.
*
*
* @param batchAssociateServiceActionWithProvisioningArtifactRequest
* @return Result of the BatchAssociateServiceActionWithProvisioningArtifact operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.BatchAssociateServiceActionWithProvisioningArtifact
* @see AWS API Documentation
*/
@Override
public BatchAssociateServiceActionWithProvisioningArtifactResult batchAssociateServiceActionWithProvisioningArtifact(
BatchAssociateServiceActionWithProvisioningArtifactRequest request) {
request = beforeClientExecution(request);
return executeBatchAssociateServiceActionWithProvisioningArtifact(request);
}
@SdkInternalApi
final BatchAssociateServiceActionWithProvisioningArtifactResult executeBatchAssociateServiceActionWithProvisioningArtifact(
BatchAssociateServiceActionWithProvisioningArtifactRequest batchAssociateServiceActionWithProvisioningArtifactRequest) {
ExecutionContext executionContext = createExecutionContext(batchAssociateServiceActionWithProvisioningArtifactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchAssociateServiceActionWithProvisioningArtifactRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchAssociateServiceActionWithProvisioningArtifactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchAssociateServiceActionWithProvisioningArtifact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchAssociateServiceActionWithProvisioningArtifactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a batch of self-service actions from the specified provisioning artifact.
*
*
* @param batchDisassociateServiceActionFromProvisioningArtifactRequest
* @return Result of the BatchDisassociateServiceActionFromProvisioningArtifact operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.BatchDisassociateServiceActionFromProvisioningArtifact
* @see AWS API Documentation
*/
@Override
public BatchDisassociateServiceActionFromProvisioningArtifactResult batchDisassociateServiceActionFromProvisioningArtifact(
BatchDisassociateServiceActionFromProvisioningArtifactRequest request) {
request = beforeClientExecution(request);
return executeBatchDisassociateServiceActionFromProvisioningArtifact(request);
}
@SdkInternalApi
final BatchDisassociateServiceActionFromProvisioningArtifactResult executeBatchDisassociateServiceActionFromProvisioningArtifact(
BatchDisassociateServiceActionFromProvisioningArtifactRequest batchDisassociateServiceActionFromProvisioningArtifactRequest) {
ExecutionContext executionContext = createExecutionContext(batchDisassociateServiceActionFromProvisioningArtifactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchDisassociateServiceActionFromProvisioningArtifactRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchDisassociateServiceActionFromProvisioningArtifactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchDisassociateServiceActionFromProvisioningArtifact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchDisassociateServiceActionFromProvisioningArtifactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Copies the specified source product to the specified target product or a new product.
*
*
* You can copy a product to the same account or another account. You can copy a product to the same Region or
* another Region. If you copy a product to another account, you must first share the product in a portfolio using
* CreatePortfolioShare.
*
*
* This operation is performed asynchronously. To track the progress of the operation, use
* DescribeCopyProductStatus.
*
*
* @param copyProductRequest
* @return Result of the CopyProduct operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.CopyProduct
* @see AWS API
* Documentation
*/
@Override
public CopyProductResult copyProduct(CopyProductRequest request) {
request = beforeClientExecution(request);
return executeCopyProduct(request);
}
@SdkInternalApi
final CopyProductResult executeCopyProduct(CopyProductRequest copyProductRequest) {
ExecutionContext executionContext = createExecutionContext(copyProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CopyProductRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(copyProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CopyProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CopyProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a constraint.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param createConstraintRequest
* @return Result of the CreateConstraint operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @throws DuplicateResourceException
* The specified resource is a duplicate.
* @sample AWSServiceCatalog.CreateConstraint
* @see AWS API Documentation
*/
@Override
public CreateConstraintResult createConstraint(CreateConstraintRequest request) {
request = beforeClientExecution(request);
return executeCreateConstraint(request);
}
@SdkInternalApi
final CreateConstraintResult executeCreateConstraint(CreateConstraintRequest createConstraintRequest) {
ExecutionContext executionContext = createExecutionContext(createConstraintRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConstraintRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createConstraintRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateConstraint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateConstraintResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param createPortfolioRequest
* @return Result of the CreatePortfolio operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @sample AWSServiceCatalog.CreatePortfolio
* @see AWS
* API Documentation
*/
@Override
public CreatePortfolioResult createPortfolio(CreatePortfolioRequest request) {
request = beforeClientExecution(request);
return executeCreatePortfolio(request);
}
@SdkInternalApi
final CreatePortfolioResult executeCreatePortfolio(CreatePortfolioRequest createPortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(createPortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePortfolioRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createPortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreatePortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreatePortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Shares the specified portfolio with the specified account or organization node. Shares to an organization node
* can only be created by the management account of an organization or by a delegated administrator. You can share
* portfolios to an organization, an organizational unit, or a specific account.
*
*
* Note that if a delegated admin is de-registered, they can no longer create portfolio shares.
*
*
* AWSOrganizationsAccess
must be enabled in order to create a portfolio share to an organization node.
*
*
* You can't share a shared resource, including portfolios that contain a shared product.
*
*
* If the portfolio share with the specified account or organization node already exists, this action will have no
* effect and will not return an error. To update an existing share, you must use the
* UpdatePortfolioShare
API instead.
*
*
*
* When you associate a principal with portfolio, a potential privilege escalation path may occur when that
* portfolio is then shared with other accounts. For a user in a recipient account who is not an Service
* Catalog Admin, but still has the ability to create Principals (Users/Groups/Roles), that user could create a role
* that matches a principal name association for the portfolio. Although this user may not know which principal
* names are associated through Service Catalog, they may be able to guess the user. If this potential escalation
* path is a concern, then Service Catalog recommends using PrincipalType
as IAM
. With
* this configuration, the PrincipalARN
must already exist in the recipient account before it can be
* associated.
*
*
*
* @param createPortfolioShareRequest
* @return Result of the CreatePortfolioShare operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws OperationNotSupportedException
* The operation is not supported.
* @throws InvalidStateException
* An attempt was made to modify a resource that is in a state that is not valid. Check your resources to
* ensure that they are in valid states before retrying the operation.
* @sample AWSServiceCatalog.CreatePortfolioShare
* @see AWS API Documentation
*/
@Override
public CreatePortfolioShareResult createPortfolioShare(CreatePortfolioShareRequest request) {
request = beforeClientExecution(request);
return executeCreatePortfolioShare(request);
}
@SdkInternalApi
final CreatePortfolioShareResult executeCreatePortfolioShare(CreatePortfolioShareRequest createPortfolioShareRequest) {
ExecutionContext executionContext = createExecutionContext(createPortfolioShareRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePortfolioShareRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createPortfolioShareRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreatePortfolioShare");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreatePortfolioShareResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a product.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* The user or role that performs this operation must have the cloudformation:GetTemplate
IAM policy
* permission. This policy permission is required when using the ImportFromPhysicalId
template source
* in the information data section.
*
*
* @param createProductRequest
* @return Result of the CreateProduct operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @sample AWSServiceCatalog.CreateProduct
* @see AWS
* API Documentation
*/
@Override
public CreateProductResult createProduct(CreateProductRequest request) {
request = beforeClientExecution(request);
return executeCreateProduct(request);
}
@SdkInternalApi
final CreateProductResult executeCreateProduct(CreateProductRequest createProductRequest) {
ExecutionContext executionContext = createExecutionContext(createProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateProductRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a plan.
*
*
* A plan includes the list of resources to be created (when provisioning a new product) or modified (when updating
* a provisioned product) when the plan is executed.
*
*
* You can create one plan for each provisioned product. To create a plan for an existing provisioned product, the
* product status must be AVAILABLE or TAINTED.
*
*
* To view the resource changes in the change set, use DescribeProvisionedProductPlan. To create or modify
* the provisioned product, use ExecuteProvisionedProductPlan.
*
*
* @param createProvisionedProductPlanRequest
* @return Result of the CreateProvisionedProductPlan operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidStateException
* An attempt was made to modify a resource that is in a state that is not valid. Check your resources to
* ensure that they are in valid states before retrying the operation.
* @sample AWSServiceCatalog.CreateProvisionedProductPlan
* @see AWS API Documentation
*/
@Override
public CreateProvisionedProductPlanResult createProvisionedProductPlan(CreateProvisionedProductPlanRequest request) {
request = beforeClientExecution(request);
return executeCreateProvisionedProductPlan(request);
}
@SdkInternalApi
final CreateProvisionedProductPlanResult executeCreateProvisionedProductPlan(CreateProvisionedProductPlanRequest createProvisionedProductPlanRequest) {
ExecutionContext executionContext = createExecutionContext(createProvisionedProductPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateProvisionedProductPlanRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createProvisionedProductPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateProvisionedProductPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateProvisionedProductPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a provisioning artifact (also known as a version) for the specified product.
*
*
* You cannot create a provisioning artifact for a product that was shared with you.
*
*
* The user or role that performs this operation must have the cloudformation:GetTemplate
IAM policy
* permission. This policy permission is required when using the ImportFromPhysicalId
template source
* in the information data section.
*
*
* @param createProvisioningArtifactRequest
* @return Result of the CreateProvisioningArtifact operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @sample AWSServiceCatalog.CreateProvisioningArtifact
* @see AWS API Documentation
*/
@Override
public CreateProvisioningArtifactResult createProvisioningArtifact(CreateProvisioningArtifactRequest request) {
request = beforeClientExecution(request);
return executeCreateProvisioningArtifact(request);
}
@SdkInternalApi
final CreateProvisioningArtifactResult executeCreateProvisioningArtifact(CreateProvisioningArtifactRequest createProvisioningArtifactRequest) {
ExecutionContext executionContext = createExecutionContext(createProvisioningArtifactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateProvisioningArtifactRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createProvisioningArtifactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateProvisioningArtifact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateProvisioningArtifactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a self-service action.
*
*
* @param createServiceActionRequest
* @return Result of the CreateServiceAction operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @sample AWSServiceCatalog.CreateServiceAction
* @see AWS API Documentation
*/
@Override
public CreateServiceActionResult createServiceAction(CreateServiceActionRequest request) {
request = beforeClientExecution(request);
return executeCreateServiceAction(request);
}
@SdkInternalApi
final CreateServiceActionResult executeCreateServiceAction(CreateServiceActionRequest createServiceActionRequest) {
ExecutionContext executionContext = createExecutionContext(createServiceActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateServiceActionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createServiceActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateServiceAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateServiceActionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a TagOption.
*
*
* @param createTagOptionRequest
* @return Result of the CreateTagOption operation returned by the service.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @throws DuplicateResourceException
* The specified resource is a duplicate.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @sample AWSServiceCatalog.CreateTagOption
* @see AWS
* API Documentation
*/
@Override
public CreateTagOptionResult createTagOption(CreateTagOptionRequest request) {
request = beforeClientExecution(request);
return executeCreateTagOption(request);
}
@SdkInternalApi
final CreateTagOptionResult executeCreateTagOption(CreateTagOptionRequest createTagOptionRequest) {
ExecutionContext executionContext = createExecutionContext(createTagOptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTagOptionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createTagOptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateTagOption");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateTagOptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified constraint.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param deleteConstraintRequest
* @return Result of the DeleteConstraint operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DeleteConstraint
* @see AWS API Documentation
*/
@Override
public DeleteConstraintResult deleteConstraint(DeleteConstraintRequest request) {
request = beforeClientExecution(request);
return executeDeleteConstraint(request);
}
@SdkInternalApi
final DeleteConstraintResult executeDeleteConstraint(DeleteConstraintRequest deleteConstraintRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConstraintRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConstraintRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteConstraintRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteConstraint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteConstraintResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified portfolio.
*
*
* You cannot delete a portfolio if it was shared with you or if it has associated products, users, constraints, or
* shared accounts.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param deletePortfolioRequest
* @return Result of the DeletePortfolio operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceInUseException
* A resource that is currently in use. Ensure that the resource is not in use and retry the operation.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @sample AWSServiceCatalog.DeletePortfolio
* @see AWS
* API Documentation
*/
@Override
public DeletePortfolioResult deletePortfolio(DeletePortfolioRequest request) {
request = beforeClientExecution(request);
return executeDeletePortfolio(request);
}
@SdkInternalApi
final DeletePortfolioResult executeDeletePortfolio(DeletePortfolioRequest deletePortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(deletePortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePortfolioRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deletePortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeletePortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops sharing the specified portfolio with the specified account or organization node. Shares to an organization
* node can only be deleted by the management account of an organization or by a delegated administrator.
*
*
* Note that if a delegated admin is de-registered, portfolio shares created from that account are removed.
*
*
* @param deletePortfolioShareRequest
* @return Result of the DeletePortfolioShare operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws OperationNotSupportedException
* The operation is not supported.
* @throws InvalidStateException
* An attempt was made to modify a resource that is in a state that is not valid. Check your resources to
* ensure that they are in valid states before retrying the operation.
* @sample AWSServiceCatalog.DeletePortfolioShare
* @see AWS API Documentation
*/
@Override
public DeletePortfolioShareResult deletePortfolioShare(DeletePortfolioShareRequest request) {
request = beforeClientExecution(request);
return executeDeletePortfolioShare(request);
}
@SdkInternalApi
final DeletePortfolioShareResult executeDeletePortfolioShare(DeletePortfolioShareRequest deletePortfolioShareRequest) {
ExecutionContext executionContext = createExecutionContext(deletePortfolioShareRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePortfolioShareRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deletePortfolioShareRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePortfolioShare");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeletePortfolioShareResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified product.
*
*
* You cannot delete a product if it was shared with you or is associated with a portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param deleteProductRequest
* @return Result of the DeleteProduct operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceInUseException
* A resource that is currently in use. Ensure that the resource is not in use and retry the operation.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @sample AWSServiceCatalog.DeleteProduct
* @see AWS
* API Documentation
*/
@Override
public DeleteProductResult deleteProduct(DeleteProductRequest request) {
request = beforeClientExecution(request);
return executeDeleteProduct(request);
}
@SdkInternalApi
final DeleteProductResult executeDeleteProduct(DeleteProductRequest deleteProductRequest) {
ExecutionContext executionContext = createExecutionContext(deleteProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteProductRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified plan.
*
*
* @param deleteProvisionedProductPlanRequest
* @return Result of the DeleteProvisionedProductPlan operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DeleteProvisionedProductPlan
* @see AWS API Documentation
*/
@Override
public DeleteProvisionedProductPlanResult deleteProvisionedProductPlan(DeleteProvisionedProductPlanRequest request) {
request = beforeClientExecution(request);
return executeDeleteProvisionedProductPlan(request);
}
@SdkInternalApi
final DeleteProvisionedProductPlanResult executeDeleteProvisionedProductPlan(DeleteProvisionedProductPlanRequest deleteProvisionedProductPlanRequest) {
ExecutionContext executionContext = createExecutionContext(deleteProvisionedProductPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteProvisionedProductPlanRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteProvisionedProductPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteProvisionedProductPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteProvisionedProductPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified provisioning artifact (also known as a version) for the specified product.
*
*
* You cannot delete a provisioning artifact associated with a product that was shared with you. You cannot delete
* the last provisioning artifact for a product, because a product must have at least one provisioning artifact.
*
*
* @param deleteProvisioningArtifactRequest
* @return Result of the DeleteProvisioningArtifact operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceInUseException
* A resource that is currently in use. Ensure that the resource is not in use and retry the operation.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DeleteProvisioningArtifact
* @see AWS API Documentation
*/
@Override
public DeleteProvisioningArtifactResult deleteProvisioningArtifact(DeleteProvisioningArtifactRequest request) {
request = beforeClientExecution(request);
return executeDeleteProvisioningArtifact(request);
}
@SdkInternalApi
final DeleteProvisioningArtifactResult executeDeleteProvisioningArtifact(DeleteProvisioningArtifactRequest deleteProvisioningArtifactRequest) {
ExecutionContext executionContext = createExecutionContext(deleteProvisioningArtifactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteProvisioningArtifactRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteProvisioningArtifactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteProvisioningArtifact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteProvisioningArtifactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a self-service action.
*
*
* @param deleteServiceActionRequest
* @return Result of the DeleteServiceAction operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceInUseException
* A resource that is currently in use. Ensure that the resource is not in use and retry the operation.
* @sample AWSServiceCatalog.DeleteServiceAction
* @see AWS API Documentation
*/
@Override
public DeleteServiceActionResult deleteServiceAction(DeleteServiceActionRequest request) {
request = beforeClientExecution(request);
return executeDeleteServiceAction(request);
}
@SdkInternalApi
final DeleteServiceActionResult executeDeleteServiceAction(DeleteServiceActionRequest deleteServiceActionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteServiceActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteServiceActionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteServiceActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteServiceAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteServiceActionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified TagOption.
*
*
* You cannot delete a TagOption if it is associated with a product or portfolio.
*
*
* @param deleteTagOptionRequest
* @return Result of the DeleteTagOption operation returned by the service.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @throws ResourceInUseException
* A resource that is currently in use. Ensure that the resource is not in use and retry the operation.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DeleteTagOption
* @see AWS
* API Documentation
*/
@Override
public DeleteTagOptionResult deleteTagOption(DeleteTagOptionRequest request) {
request = beforeClientExecution(request);
return executeDeleteTagOption(request);
}
@SdkInternalApi
final DeleteTagOptionResult executeDeleteTagOption(DeleteTagOptionRequest deleteTagOptionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTagOptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteTagOptionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteTagOptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteTagOption");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteTagOptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified constraint.
*
*
* @param describeConstraintRequest
* @return Result of the DescribeConstraint operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DescribeConstraint
* @see AWS API Documentation
*/
@Override
public DescribeConstraintResult describeConstraint(DescribeConstraintRequest request) {
request = beforeClientExecution(request);
return executeDescribeConstraint(request);
}
@SdkInternalApi
final DescribeConstraintResult executeDescribeConstraint(DescribeConstraintRequest describeConstraintRequest) {
ExecutionContext executionContext = createExecutionContext(describeConstraintRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeConstraintRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeConstraintRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeConstraint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeConstraintResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the status of the specified copy product operation.
*
*
* @param describeCopyProductStatusRequest
* @return Result of the DescribeCopyProductStatus operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DescribeCopyProductStatus
* @see AWS API Documentation
*/
@Override
public DescribeCopyProductStatusResult describeCopyProductStatus(DescribeCopyProductStatusRequest request) {
request = beforeClientExecution(request);
return executeDescribeCopyProductStatus(request);
}
@SdkInternalApi
final DescribeCopyProductStatusResult executeDescribeCopyProductStatus(DescribeCopyProductStatusRequest describeCopyProductStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describeCopyProductStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCopyProductStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeCopyProductStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeCopyProductStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeCopyProductStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param describePortfolioRequest
* @return Result of the DescribePortfolio operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DescribePortfolio
* @see AWS API Documentation
*/
@Override
public DescribePortfolioResult describePortfolio(DescribePortfolioRequest request) {
request = beforeClientExecution(request);
return executeDescribePortfolio(request);
}
@SdkInternalApi
final DescribePortfolioResult executeDescribePortfolio(DescribePortfolioRequest describePortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(describePortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribePortfolioRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describePortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribePortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribePortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the status of the specified portfolio share operation. This API can only be called by the management account
* in the organization or by a delegated admin.
*
*
* @param describePortfolioShareStatusRequest
* @return Result of the DescribePortfolioShareStatus operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws OperationNotSupportedException
* The operation is not supported.
* @sample AWSServiceCatalog.DescribePortfolioShareStatus
* @see AWS API Documentation
*/
@Override
public DescribePortfolioShareStatusResult describePortfolioShareStatus(DescribePortfolioShareStatusRequest request) {
request = beforeClientExecution(request);
return executeDescribePortfolioShareStatus(request);
}
@SdkInternalApi
final DescribePortfolioShareStatusResult executeDescribePortfolioShareStatus(DescribePortfolioShareStatusRequest describePortfolioShareStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describePortfolioShareStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribePortfolioShareStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describePortfolioShareStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribePortfolioShareStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribePortfolioShareStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a summary of each of the portfolio shares that were created for the specified portfolio.
*
*
* You can use this API to determine which accounts or organizational nodes this portfolio have been shared, whether
* the recipient entity has imported the share, and whether TagOptions are included with the share.
*
*
* The PortfolioId
and Type
parameters are both required.
*
*
* @param describePortfolioSharesRequest
* @return Result of the DescribePortfolioShares operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DescribePortfolioShares
* @see AWS API Documentation
*/
@Override
public DescribePortfolioSharesResult describePortfolioShares(DescribePortfolioSharesRequest request) {
request = beforeClientExecution(request);
return executeDescribePortfolioShares(request);
}
@SdkInternalApi
final DescribePortfolioSharesResult executeDescribePortfolioShares(DescribePortfolioSharesRequest describePortfolioSharesRequest) {
ExecutionContext executionContext = createExecutionContext(describePortfolioSharesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribePortfolioSharesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describePortfolioSharesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribePortfolioShares");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribePortfolioSharesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified product.
*
*
*
* Running this operation with administrator access results in a failure. DescribeProductAsAdmin should be
* used instead.
*
*
*
* @param describeProductRequest
* @return Result of the DescribeProduct operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DescribeProduct
* @see AWS
* API Documentation
*/
@Override
public DescribeProductResult describeProduct(DescribeProductRequest request) {
request = beforeClientExecution(request);
return executeDescribeProduct(request);
}
@SdkInternalApi
final DescribeProductResult executeDescribeProduct(DescribeProductRequest describeProductRequest) {
ExecutionContext executionContext = createExecutionContext(describeProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeProductRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified product. This operation is run with administrator access.
*
*
* @param describeProductAsAdminRequest
* @return Result of the DescribeProductAsAdmin operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DescribeProductAsAdmin
* @see AWS API Documentation
*/
@Override
public DescribeProductAsAdminResult describeProductAsAdmin(DescribeProductAsAdminRequest request) {
request = beforeClientExecution(request);
return executeDescribeProductAsAdmin(request);
}
@SdkInternalApi
final DescribeProductAsAdminResult executeDescribeProductAsAdmin(DescribeProductAsAdminRequest describeProductAsAdminRequest) {
ExecutionContext executionContext = createExecutionContext(describeProductAsAdminRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeProductAsAdminRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeProductAsAdminRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeProductAsAdmin");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeProductAsAdminResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified product.
*
*
* @param describeProductViewRequest
* @return Result of the DescribeProductView operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DescribeProductView
* @see AWS API Documentation
*/
@Override
public DescribeProductViewResult describeProductView(DescribeProductViewRequest request) {
request = beforeClientExecution(request);
return executeDescribeProductView(request);
}
@SdkInternalApi
final DescribeProductViewResult executeDescribeProductView(DescribeProductViewRequest describeProductViewRequest) {
ExecutionContext executionContext = createExecutionContext(describeProductViewRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeProductViewRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeProductViewRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeProductView");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeProductViewResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified provisioned product.
*
*
* @param describeProvisionedProductRequest
* DescribeProvisionedProductAPI input structure. AcceptLanguage - [Optional] The language code for
* localization. Id - [Optional] The provisioned product identifier. Name - [Optional] Another provisioned
* product identifier. Customers must provide either Id or Name.
* @return Result of the DescribeProvisionedProduct operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DescribeProvisionedProduct
* @see AWS API Documentation
*/
@Override
public DescribeProvisionedProductResult describeProvisionedProduct(DescribeProvisionedProductRequest request) {
request = beforeClientExecution(request);
return executeDescribeProvisionedProduct(request);
}
@SdkInternalApi
final DescribeProvisionedProductResult executeDescribeProvisionedProduct(DescribeProvisionedProductRequest describeProvisionedProductRequest) {
ExecutionContext executionContext = createExecutionContext(describeProvisionedProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeProvisionedProductRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeProvisionedProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeProvisionedProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeProvisionedProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the resource changes for the specified plan.
*
*
* @param describeProvisionedProductPlanRequest
* @return Result of the DescribeProvisionedProductPlan operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DescribeProvisionedProductPlan
* @see AWS API Documentation
*/
@Override
public DescribeProvisionedProductPlanResult describeProvisionedProductPlan(DescribeProvisionedProductPlanRequest request) {
request = beforeClientExecution(request);
return executeDescribeProvisionedProductPlan(request);
}
@SdkInternalApi
final DescribeProvisionedProductPlanResult executeDescribeProvisionedProductPlan(DescribeProvisionedProductPlanRequest describeProvisionedProductPlanRequest) {
ExecutionContext executionContext = createExecutionContext(describeProvisionedProductPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeProvisionedProductPlanRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeProvisionedProductPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeProvisionedProductPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeProvisionedProductPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified provisioning artifact (also known as a version) for the specified product.
*
*
* @param describeProvisioningArtifactRequest
* @return Result of the DescribeProvisioningArtifact operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DescribeProvisioningArtifact
* @see AWS API Documentation
*/
@Override
public DescribeProvisioningArtifactResult describeProvisioningArtifact(DescribeProvisioningArtifactRequest request) {
request = beforeClientExecution(request);
return executeDescribeProvisioningArtifact(request);
}
@SdkInternalApi
final DescribeProvisioningArtifactResult executeDescribeProvisioningArtifact(DescribeProvisioningArtifactRequest describeProvisioningArtifactRequest) {
ExecutionContext executionContext = createExecutionContext(describeProvisioningArtifactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeProvisioningArtifactRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeProvisioningArtifactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeProvisioningArtifact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeProvisioningArtifactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the configuration required to provision the specified product using the specified
* provisioning artifact.
*
*
* If the output contains a TagOption key with an empty list of values, there is a TagOption conflict for that key.
* The end user cannot take action to fix the conflict, and launch is not blocked. In subsequent calls to
* ProvisionProduct, do not include conflicted TagOption keys as tags, or this causes the error
* "Parameter validation failed: Missing required parameter in Tags[N]:Value". Tag the provisioned
* product with the value sc-tagoption-conflict-portfolioId-productId
.
*
*
* @param describeProvisioningParametersRequest
* @return Result of the DescribeProvisioningParameters operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DescribeProvisioningParameters
* @see AWS API Documentation
*/
@Override
public DescribeProvisioningParametersResult describeProvisioningParameters(DescribeProvisioningParametersRequest request) {
request = beforeClientExecution(request);
return executeDescribeProvisioningParameters(request);
}
@SdkInternalApi
final DescribeProvisioningParametersResult executeDescribeProvisioningParameters(DescribeProvisioningParametersRequest describeProvisioningParametersRequest) {
ExecutionContext executionContext = createExecutionContext(describeProvisioningParametersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeProvisioningParametersRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeProvisioningParametersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeProvisioningParameters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeProvisioningParametersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified request operation.
*
*
* Use this operation after calling a request operation (for example, ProvisionProduct,
* TerminateProvisionedProduct, or UpdateProvisionedProduct).
*
*
*
* If a provisioned product was transferred to a new owner using UpdateProvisionedProductProperties, the new
* owner will be able to describe all past records for that product. The previous owner will no longer be able to
* describe the records, but will be able to use ListRecordHistory to see the product's history from when he
* was the owner.
*
*
*
* @param describeRecordRequest
* @return Result of the DescribeRecord operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DescribeRecord
* @see AWS
* API Documentation
*/
@Override
public DescribeRecordResult describeRecord(DescribeRecordRequest request) {
request = beforeClientExecution(request);
return executeDescribeRecord(request);
}
@SdkInternalApi
final DescribeRecordResult executeDescribeRecord(DescribeRecordRequest describeRecordRequest) {
ExecutionContext executionContext = createExecutionContext(describeRecordRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRecordRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRecordRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRecord");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeRecordResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a self-service action.
*
*
* @param describeServiceActionRequest
* @return Result of the DescribeServiceAction operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DescribeServiceAction
* @see AWS API Documentation
*/
@Override
public DescribeServiceActionResult describeServiceAction(DescribeServiceActionRequest request) {
request = beforeClientExecution(request);
return executeDescribeServiceAction(request);
}
@SdkInternalApi
final DescribeServiceActionResult executeDescribeServiceAction(DescribeServiceActionRequest describeServiceActionRequest) {
ExecutionContext executionContext = createExecutionContext(describeServiceActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeServiceActionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeServiceActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeServiceAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeServiceActionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Finds the default parameters for a specific self-service action on a specific provisioned product and returns a
* map of the results to the user.
*
*
* @param describeServiceActionExecutionParametersRequest
* @return Result of the DescribeServiceActionExecutionParameters operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DescribeServiceActionExecutionParameters
* @see AWS API Documentation
*/
@Override
public DescribeServiceActionExecutionParametersResult describeServiceActionExecutionParameters(DescribeServiceActionExecutionParametersRequest request) {
request = beforeClientExecution(request);
return executeDescribeServiceActionExecutionParameters(request);
}
@SdkInternalApi
final DescribeServiceActionExecutionParametersResult executeDescribeServiceActionExecutionParameters(
DescribeServiceActionExecutionParametersRequest describeServiceActionExecutionParametersRequest) {
ExecutionContext executionContext = createExecutionContext(describeServiceActionExecutionParametersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeServiceActionExecutionParametersRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeServiceActionExecutionParametersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeServiceActionExecutionParameters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeServiceActionExecutionParametersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the specified TagOption.
*
*
* @param describeTagOptionRequest
* @return Result of the DescribeTagOption operation returned by the service.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DescribeTagOption
* @see AWS API Documentation
*/
@Override
public DescribeTagOptionResult describeTagOption(DescribeTagOptionRequest request) {
request = beforeClientExecution(request);
return executeDescribeTagOption(request);
}
@SdkInternalApi
final DescribeTagOptionResult executeDescribeTagOption(DescribeTagOptionRequest describeTagOptionRequest) {
ExecutionContext executionContext = createExecutionContext(describeTagOptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTagOptionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeTagOptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeTagOption");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeTagOptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disable portfolio sharing through the Organizations service. This command will not delete your current shares,
* but prevents you from creating new shares throughout your organization. Current shares are not kept in sync with
* your organization structure if the structure changes after calling this API. Only the management account in the
* organization can call this API.
*
*
* You cannot call this API if there are active delegated administrators in the organization.
*
*
* Note that a delegated administrator is not authorized to invoke DisableAWSOrganizationsAccess
.
*
*
*
* If you share an Service Catalog portfolio in an organization within Organizations, and then disable Organizations
* access for Service Catalog, the portfolio access permissions will not sync with the latest changes to the
* organization structure. Specifically, accounts that you removed from the organization after disabling Service
* Catalog access will retain access to the previously shared portfolio.
*
*
*
* @param disableAWSOrganizationsAccessRequest
* @return Result of the DisableAWSOrganizationsAccess operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidStateException
* An attempt was made to modify a resource that is in a state that is not valid. Check your resources to
* ensure that they are in valid states before retrying the operation.
* @throws OperationNotSupportedException
* The operation is not supported.
* @sample AWSServiceCatalog.DisableAWSOrganizationsAccess
* @see AWS API Documentation
*/
@Override
public DisableAWSOrganizationsAccessResult disableAWSOrganizationsAccess(DisableAWSOrganizationsAccessRequest request) {
request = beforeClientExecution(request);
return executeDisableAWSOrganizationsAccess(request);
}
@SdkInternalApi
final DisableAWSOrganizationsAccessResult executeDisableAWSOrganizationsAccess(DisableAWSOrganizationsAccessRequest disableAWSOrganizationsAccessRequest) {
ExecutionContext executionContext = createExecutionContext(disableAWSOrganizationsAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableAWSOrganizationsAccessRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disableAWSOrganizationsAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableAWSOrganizationsAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisableAWSOrganizationsAccessResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified budget from the specified resource.
*
*
* @param disassociateBudgetFromResourceRequest
* @return Result of the DisassociateBudgetFromResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DisassociateBudgetFromResource
* @see AWS API Documentation
*/
@Override
public DisassociateBudgetFromResourceResult disassociateBudgetFromResource(DisassociateBudgetFromResourceRequest request) {
request = beforeClientExecution(request);
return executeDisassociateBudgetFromResource(request);
}
@SdkInternalApi
final DisassociateBudgetFromResourceResult executeDisassociateBudgetFromResource(DisassociateBudgetFromResourceRequest disassociateBudgetFromResourceRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateBudgetFromResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateBudgetFromResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateBudgetFromResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateBudgetFromResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateBudgetFromResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a previously associated principal ARN from a specified portfolio.
*
*
* The PrincipalType
and PrincipalARN
must match the
* AssociatePrincipalWithPortfolio
call request details. For example, to disassociate an association
* created with a PrincipalARN
of PrincipalType
IAM you must use the
* PrincipalType
IAM when calling DisassociatePrincipalFromPortfolio
.
*
*
* For portfolios that have been shared with principal name sharing enabled: after disassociating a principal, share
* recipient accounts will no longer be able to provision products in this portfolio using a role matching the name
* of the associated principal.
*
*
* For more information, review associate-principal-with-portfolio in the Amazon Web Services CLI Command Reference.
*
*
*
* If you disassociate a principal from a portfolio, with PrincipalType as IAM
, the same principal will
* still have access to the portfolio if it matches one of the associated principals of type
* IAM_PATTERN
. To fully remove access for a principal, verify all the associated Principals of type
* IAM_PATTERN
, and then ensure you disassociate any IAM_PATTERN
principals that match the
* principal whose access you are removing.
*
*
*
* @param disassociatePrincipalFromPortfolioRequest
* @return Result of the DisassociatePrincipalFromPortfolio operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DisassociatePrincipalFromPortfolio
* @see AWS API Documentation
*/
@Override
public DisassociatePrincipalFromPortfolioResult disassociatePrincipalFromPortfolio(DisassociatePrincipalFromPortfolioRequest request) {
request = beforeClientExecution(request);
return executeDisassociatePrincipalFromPortfolio(request);
}
@SdkInternalApi
final DisassociatePrincipalFromPortfolioResult executeDisassociatePrincipalFromPortfolio(
DisassociatePrincipalFromPortfolioRequest disassociatePrincipalFromPortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(disassociatePrincipalFromPortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociatePrincipalFromPortfolioRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociatePrincipalFromPortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociatePrincipalFromPortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociatePrincipalFromPortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified product from the specified portfolio.
*
*
* A delegated admin is authorized to invoke this command.
*
*
* @param disassociateProductFromPortfolioRequest
* @return Result of the DisassociateProductFromPortfolio operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceInUseException
* A resource that is currently in use. Ensure that the resource is not in use and retry the operation.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.DisassociateProductFromPortfolio
* @see AWS API Documentation
*/
@Override
public DisassociateProductFromPortfolioResult disassociateProductFromPortfolio(DisassociateProductFromPortfolioRequest request) {
request = beforeClientExecution(request);
return executeDisassociateProductFromPortfolio(request);
}
@SdkInternalApi
final DisassociateProductFromPortfolioResult executeDisassociateProductFromPortfolio(
DisassociateProductFromPortfolioRequest disassociateProductFromPortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateProductFromPortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateProductFromPortfolioRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateProductFromPortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateProductFromPortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateProductFromPortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified self-service action association from the specified provisioning artifact.
*
*
* @param disassociateServiceActionFromProvisioningArtifactRequest
* @return Result of the DisassociateServiceActionFromProvisioningArtifact operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DisassociateServiceActionFromProvisioningArtifact
* @see AWS API Documentation
*/
@Override
public DisassociateServiceActionFromProvisioningArtifactResult disassociateServiceActionFromProvisioningArtifact(
DisassociateServiceActionFromProvisioningArtifactRequest request) {
request = beforeClientExecution(request);
return executeDisassociateServiceActionFromProvisioningArtifact(request);
}
@SdkInternalApi
final DisassociateServiceActionFromProvisioningArtifactResult executeDisassociateServiceActionFromProvisioningArtifact(
DisassociateServiceActionFromProvisioningArtifactRequest disassociateServiceActionFromProvisioningArtifactRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateServiceActionFromProvisioningArtifactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateServiceActionFromProvisioningArtifactRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateServiceActionFromProvisioningArtifactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateServiceActionFromProvisioningArtifact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateServiceActionFromProvisioningArtifactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified TagOption from the specified resource.
*
*
* @param disassociateTagOptionFromResourceRequest
* @return Result of the DisassociateTagOptionFromResource operation returned by the service.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.DisassociateTagOptionFromResource
* @see AWS API Documentation
*/
@Override
public DisassociateTagOptionFromResourceResult disassociateTagOptionFromResource(DisassociateTagOptionFromResourceRequest request) {
request = beforeClientExecution(request);
return executeDisassociateTagOptionFromResource(request);
}
@SdkInternalApi
final DisassociateTagOptionFromResourceResult executeDisassociateTagOptionFromResource(
DisassociateTagOptionFromResourceRequest disassociateTagOptionFromResourceRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateTagOptionFromResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateTagOptionFromResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateTagOptionFromResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateTagOptionFromResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateTagOptionFromResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enable portfolio sharing feature through Organizations. This API will allow Service Catalog to receive updates on
* your organization in order to sync your shares with the current structure. This API can only be called by the
* management account in the organization.
*
*
* When you call this API, Service Catalog calls organizations:EnableAWSServiceAccess
on your behalf so
* that your shares stay in sync with any changes in your Organizations structure.
*
*
* Note that a delegated administrator is not authorized to invoke EnableAWSOrganizationsAccess
.
*
*
*
* If you have previously disabled Organizations access for Service Catalog, and then enable access again, the
* portfolio access permissions might not sync with the latest changes to the organization structure. Specifically,
* accounts that you removed from the organization after disabling Service Catalog access, and before you enabled
* access again, can retain access to the previously shared portfolio. As a result, an account that has been removed
* from the organization might still be able to create or manage Amazon Web Services resources when it is no longer
* authorized to do so. Amazon Web Services is working to resolve this issue.
*
*
*
* @param enableAWSOrganizationsAccessRequest
* @return Result of the EnableAWSOrganizationsAccess operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidStateException
* An attempt was made to modify a resource that is in a state that is not valid. Check your resources to
* ensure that they are in valid states before retrying the operation.
* @throws OperationNotSupportedException
* The operation is not supported.
* @sample AWSServiceCatalog.EnableAWSOrganizationsAccess
* @see AWS API Documentation
*/
@Override
public EnableAWSOrganizationsAccessResult enableAWSOrganizationsAccess(EnableAWSOrganizationsAccessRequest request) {
request = beforeClientExecution(request);
return executeEnableAWSOrganizationsAccess(request);
}
@SdkInternalApi
final EnableAWSOrganizationsAccessResult executeEnableAWSOrganizationsAccess(EnableAWSOrganizationsAccessRequest enableAWSOrganizationsAccessRequest) {
ExecutionContext executionContext = createExecutionContext(enableAWSOrganizationsAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableAWSOrganizationsAccessRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(enableAWSOrganizationsAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableAWSOrganizationsAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new EnableAWSOrganizationsAccessResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provisions or modifies a product based on the resource changes for the specified plan.
*
*
* @param executeProvisionedProductPlanRequest
* @return Result of the ExecuteProvisionedProductPlan operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidStateException
* An attempt was made to modify a resource that is in a state that is not valid. Check your resources to
* ensure that they are in valid states before retrying the operation.
* @sample AWSServiceCatalog.ExecuteProvisionedProductPlan
* @see AWS API Documentation
*/
@Override
public ExecuteProvisionedProductPlanResult executeProvisionedProductPlan(ExecuteProvisionedProductPlanRequest request) {
request = beforeClientExecution(request);
return executeExecuteProvisionedProductPlan(request);
}
@SdkInternalApi
final ExecuteProvisionedProductPlanResult executeExecuteProvisionedProductPlan(ExecuteProvisionedProductPlanRequest executeProvisionedProductPlanRequest) {
ExecutionContext executionContext = createExecutionContext(executeProvisionedProductPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ExecuteProvisionedProductPlanRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(executeProvisionedProductPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ExecuteProvisionedProductPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ExecuteProvisionedProductPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Executes a self-service action against a provisioned product.
*
*
* @param executeProvisionedProductServiceActionRequest
* @return Result of the ExecuteProvisionedProductServiceAction operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidStateException
* An attempt was made to modify a resource that is in a state that is not valid. Check your resources to
* ensure that they are in valid states before retrying the operation.
* @sample AWSServiceCatalog.ExecuteProvisionedProductServiceAction
* @see AWS API Documentation
*/
@Override
public ExecuteProvisionedProductServiceActionResult executeProvisionedProductServiceAction(ExecuteProvisionedProductServiceActionRequest request) {
request = beforeClientExecution(request);
return executeExecuteProvisionedProductServiceAction(request);
}
@SdkInternalApi
final ExecuteProvisionedProductServiceActionResult executeExecuteProvisionedProductServiceAction(
ExecuteProvisionedProductServiceActionRequest executeProvisionedProductServiceActionRequest) {
ExecutionContext executionContext = createExecutionContext(executeProvisionedProductServiceActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ExecuteProvisionedProductServiceActionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(executeProvisionedProductServiceActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ExecuteProvisionedProductServiceAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ExecuteProvisionedProductServiceActionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the Access Status for Organizations portfolio share feature. This API can only be called by the management
* account in the organization or by a delegated admin.
*
*
* @param getAWSOrganizationsAccessStatusRequest
* @return Result of the GetAWSOrganizationsAccessStatus operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotSupportedException
* The operation is not supported.
* @sample AWSServiceCatalog.GetAWSOrganizationsAccessStatus
* @see AWS API Documentation
*/
@Override
public GetAWSOrganizationsAccessStatusResult getAWSOrganizationsAccessStatus(GetAWSOrganizationsAccessStatusRequest request) {
request = beforeClientExecution(request);
return executeGetAWSOrganizationsAccessStatus(request);
}
@SdkInternalApi
final GetAWSOrganizationsAccessStatusResult executeGetAWSOrganizationsAccessStatus(
GetAWSOrganizationsAccessStatusRequest getAWSOrganizationsAccessStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getAWSOrganizationsAccessStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAWSOrganizationsAccessStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getAWSOrganizationsAccessStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAWSOrganizationsAccessStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetAWSOrganizationsAccessStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This API takes either a ProvisonedProductId
or a ProvisionedProductName
, along with a
* list of one or more output keys, and responds with the key/value pairs of those outputs.
*
*
* @param getProvisionedProductOutputsRequest
* @return Result of the GetProvisionedProductOutputs operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.GetProvisionedProductOutputs
* @see AWS API Documentation
*/
@Override
public GetProvisionedProductOutputsResult getProvisionedProductOutputs(GetProvisionedProductOutputsRequest request) {
request = beforeClientExecution(request);
return executeGetProvisionedProductOutputs(request);
}
@SdkInternalApi
final GetProvisionedProductOutputsResult executeGetProvisionedProductOutputs(GetProvisionedProductOutputsRequest getProvisionedProductOutputsRequest) {
ExecutionContext executionContext = createExecutionContext(getProvisionedProductOutputsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetProvisionedProductOutputsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getProvisionedProductOutputsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetProvisionedProductOutputs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetProvisionedProductOutputsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Requests the import of a resource as an Service Catalog provisioned product that is associated to an Service
* Catalog product and provisioning artifact. Once imported, all supported governance actions are supported on the
* provisioned product.
*
*
* Resource import only supports CloudFormation stack ARNs. CloudFormation StackSets, and non-root nested stacks,
* are not supported.
*
*
* The CloudFormation stack must have one of the following statuses to be imported: CREATE_COMPLETE
,
* UPDATE_COMPLETE
, UPDATE_ROLLBACK_COMPLETE
, IMPORT_COMPLETE
, and
* IMPORT_ROLLBACK_COMPLETE
.
*
*
* Import of the resource requires that the CloudFormation stack template matches the associated Service Catalog
* product provisioning artifact.
*
*
*
* When you import an existing CloudFormation stack into a portfolio, Service Catalog does not apply the product's
* associated constraints during the import process. Service Catalog applies the constraints after you call
* UpdateProvisionedProduct
for the provisioned product.
*
*
*
* The user or role that performs this operation must have the cloudformation:GetTemplate
and
* cloudformation:DescribeStacks
IAM policy permissions.
*
*
* You can only import one provisioned product at a time. The product's CloudFormation stack must have the
* IMPORT_COMPLETE
status before you import another.
*
*
* @param importAsProvisionedProductRequest
* @return Result of the ImportAsProvisionedProduct operation returned by the service.
* @throws DuplicateResourceException
* The specified resource is a duplicate.
* @throws InvalidStateException
* An attempt was made to modify a resource that is in a state that is not valid. Check your resources to
* ensure that they are in valid states before retrying the operation.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ImportAsProvisionedProduct
* @see AWS API Documentation
*/
@Override
public ImportAsProvisionedProductResult importAsProvisionedProduct(ImportAsProvisionedProductRequest request) {
request = beforeClientExecution(request);
return executeImportAsProvisionedProduct(request);
}
@SdkInternalApi
final ImportAsProvisionedProductResult executeImportAsProvisionedProduct(ImportAsProvisionedProductRequest importAsProvisionedProductRequest) {
ExecutionContext executionContext = createExecutionContext(importAsProvisionedProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ImportAsProvisionedProductRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(importAsProvisionedProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ImportAsProvisionedProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ImportAsProvisionedProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all imported portfolios for which account-to-account shares were accepted by this account. By specifying
* the PortfolioShareType
, you can list portfolios for which organizational shares were accepted by
* this account.
*
*
* @param listAcceptedPortfolioSharesRequest
* @return Result of the ListAcceptedPortfolioShares operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws OperationNotSupportedException
* The operation is not supported.
* @sample AWSServiceCatalog.ListAcceptedPortfolioShares
* @see AWS API Documentation
*/
@Override
public ListAcceptedPortfolioSharesResult listAcceptedPortfolioShares(ListAcceptedPortfolioSharesRequest request) {
request = beforeClientExecution(request);
return executeListAcceptedPortfolioShares(request);
}
@SdkInternalApi
final ListAcceptedPortfolioSharesResult executeListAcceptedPortfolioShares(ListAcceptedPortfolioSharesRequest listAcceptedPortfolioSharesRequest) {
ExecutionContext executionContext = createExecutionContext(listAcceptedPortfolioSharesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAcceptedPortfolioSharesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAcceptedPortfolioSharesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAcceptedPortfolioShares");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAcceptedPortfolioSharesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the budgets associated to the specified resource.
*
*
* @param listBudgetsForResourceRequest
* @return Result of the ListBudgetsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListBudgetsForResource
* @see AWS API Documentation
*/
@Override
public ListBudgetsForResourceResult listBudgetsForResource(ListBudgetsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListBudgetsForResource(request);
}
@SdkInternalApi
final ListBudgetsForResourceResult executeListBudgetsForResource(ListBudgetsForResourceRequest listBudgetsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listBudgetsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBudgetsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listBudgetsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBudgetsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListBudgetsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the constraints for the specified portfolio and product.
*
*
* @param listConstraintsForPortfolioRequest
* @return Result of the ListConstraintsForPortfolio operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListConstraintsForPortfolio
* @see AWS API Documentation
*/
@Override
public ListConstraintsForPortfolioResult listConstraintsForPortfolio(ListConstraintsForPortfolioRequest request) {
request = beforeClientExecution(request);
return executeListConstraintsForPortfolio(request);
}
@SdkInternalApi
final ListConstraintsForPortfolioResult executeListConstraintsForPortfolio(ListConstraintsForPortfolioRequest listConstraintsForPortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(listConstraintsForPortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListConstraintsForPortfolioRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listConstraintsForPortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListConstraintsForPortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListConstraintsForPortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the paths to the specified product. A path describes how the user gets access to a specified product and is
* necessary when provisioning a product. A path also determines the constraints that are put on a product. A path
* is dependent on a specific product, porfolio, and principal.
*
*
*
* When provisioning a product that's been added to a portfolio, you must grant your user, group, or role access to
* the portfolio. For more information, see Granting users
* access in the Service Catalog User Guide.
*
*
*
* @param listLaunchPathsRequest
* @return Result of the ListLaunchPaths operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.ListLaunchPaths
* @see AWS
* API Documentation
*/
@Override
public ListLaunchPathsResult listLaunchPaths(ListLaunchPathsRequest request) {
request = beforeClientExecution(request);
return executeListLaunchPaths(request);
}
@SdkInternalApi
final ListLaunchPathsResult executeListLaunchPaths(ListLaunchPathsRequest listLaunchPathsRequest) {
ExecutionContext executionContext = createExecutionContext(listLaunchPathsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListLaunchPathsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listLaunchPathsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListLaunchPaths");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListLaunchPathsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the organization nodes that have access to the specified portfolio. This API can only be called by the
* management account in the organization or by a delegated admin.
*
*
* If a delegated admin is de-registered, they can no longer perform this operation.
*
*
* @param listOrganizationPortfolioAccessRequest
* @return Result of the ListOrganizationPortfolioAccess operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws OperationNotSupportedException
* The operation is not supported.
* @sample AWSServiceCatalog.ListOrganizationPortfolioAccess
* @see AWS API Documentation
*/
@Override
public ListOrganizationPortfolioAccessResult listOrganizationPortfolioAccess(ListOrganizationPortfolioAccessRequest request) {
request = beforeClientExecution(request);
return executeListOrganizationPortfolioAccess(request);
}
@SdkInternalApi
final ListOrganizationPortfolioAccessResult executeListOrganizationPortfolioAccess(
ListOrganizationPortfolioAccessRequest listOrganizationPortfolioAccessRequest) {
ExecutionContext executionContext = createExecutionContext(listOrganizationPortfolioAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListOrganizationPortfolioAccessRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listOrganizationPortfolioAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListOrganizationPortfolioAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListOrganizationPortfolioAccessResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the account IDs that have access to the specified portfolio.
*
*
* A delegated admin can list the accounts that have access to the shared portfolio. Note that if a delegated admin
* is de-registered, they can no longer perform this operation.
*
*
* @param listPortfolioAccessRequest
* @return Result of the ListPortfolioAccess operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListPortfolioAccess
* @see AWS API Documentation
*/
@Override
public ListPortfolioAccessResult listPortfolioAccess(ListPortfolioAccessRequest request) {
request = beforeClientExecution(request);
return executeListPortfolioAccess(request);
}
@SdkInternalApi
final ListPortfolioAccessResult executeListPortfolioAccess(ListPortfolioAccessRequest listPortfolioAccessRequest) {
ExecutionContext executionContext = createExecutionContext(listPortfolioAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPortfolioAccessRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPortfolioAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPortfolioAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPortfolioAccessResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all portfolios in the catalog.
*
*
* @param listPortfoliosRequest
* @return Result of the ListPortfolios operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListPortfolios
* @see AWS
* API Documentation
*/
@Override
public ListPortfoliosResult listPortfolios(ListPortfoliosRequest request) {
request = beforeClientExecution(request);
return executeListPortfolios(request);
}
@SdkInternalApi
final ListPortfoliosResult executeListPortfolios(ListPortfoliosRequest listPortfoliosRequest) {
ExecutionContext executionContext = createExecutionContext(listPortfoliosRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPortfoliosRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPortfoliosRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPortfolios");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPortfoliosResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all portfolios that the specified product is associated with.
*
*
* @param listPortfoliosForProductRequest
* @return Result of the ListPortfoliosForProduct operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.ListPortfoliosForProduct
* @see AWS API Documentation
*/
@Override
public ListPortfoliosForProductResult listPortfoliosForProduct(ListPortfoliosForProductRequest request) {
request = beforeClientExecution(request);
return executeListPortfoliosForProduct(request);
}
@SdkInternalApi
final ListPortfoliosForProductResult executeListPortfoliosForProduct(ListPortfoliosForProductRequest listPortfoliosForProductRequest) {
ExecutionContext executionContext = createExecutionContext(listPortfoliosForProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPortfoliosForProductRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listPortfoliosForProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPortfoliosForProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListPortfoliosForProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all PrincipalARN
s and corresponding PrincipalType
s associated with the specified
* portfolio.
*
*
* @param listPrincipalsForPortfolioRequest
* @return Result of the ListPrincipalsForPortfolio operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListPrincipalsForPortfolio
* @see AWS API Documentation
*/
@Override
public ListPrincipalsForPortfolioResult listPrincipalsForPortfolio(ListPrincipalsForPortfolioRequest request) {
request = beforeClientExecution(request);
return executeListPrincipalsForPortfolio(request);
}
@SdkInternalApi
final ListPrincipalsForPortfolioResult executeListPrincipalsForPortfolio(ListPrincipalsForPortfolioRequest listPrincipalsForPortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(listPrincipalsForPortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPrincipalsForPortfolioRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listPrincipalsForPortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPrincipalsForPortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListPrincipalsForPortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the plans for the specified provisioned product or all plans to which the user has access.
*
*
* @param listProvisionedProductPlansRequest
* @return Result of the ListProvisionedProductPlans operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListProvisionedProductPlans
* @see AWS API Documentation
*/
@Override
public ListProvisionedProductPlansResult listProvisionedProductPlans(ListProvisionedProductPlansRequest request) {
request = beforeClientExecution(request);
return executeListProvisionedProductPlans(request);
}
@SdkInternalApi
final ListProvisionedProductPlansResult executeListProvisionedProductPlans(ListProvisionedProductPlansRequest listProvisionedProductPlansRequest) {
ExecutionContext executionContext = createExecutionContext(listProvisionedProductPlansRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListProvisionedProductPlansRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listProvisionedProductPlansRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListProvisionedProductPlans");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListProvisionedProductPlansResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all provisioning artifacts (also known as versions) for the specified product.
*
*
* @param listProvisioningArtifactsRequest
* @return Result of the ListProvisioningArtifacts operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListProvisioningArtifacts
* @see AWS API Documentation
*/
@Override
public ListProvisioningArtifactsResult listProvisioningArtifacts(ListProvisioningArtifactsRequest request) {
request = beforeClientExecution(request);
return executeListProvisioningArtifacts(request);
}
@SdkInternalApi
final ListProvisioningArtifactsResult executeListProvisioningArtifacts(ListProvisioningArtifactsRequest listProvisioningArtifactsRequest) {
ExecutionContext executionContext = createExecutionContext(listProvisioningArtifactsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListProvisioningArtifactsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listProvisioningArtifactsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListProvisioningArtifacts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListProvisioningArtifactsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all provisioning artifacts (also known as versions) for the specified self-service action.
*
*
* @param listProvisioningArtifactsForServiceActionRequest
* @return Result of the ListProvisioningArtifactsForServiceAction operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListProvisioningArtifactsForServiceAction
* @see AWS API Documentation
*/
@Override
public ListProvisioningArtifactsForServiceActionResult listProvisioningArtifactsForServiceAction(ListProvisioningArtifactsForServiceActionRequest request) {
request = beforeClientExecution(request);
return executeListProvisioningArtifactsForServiceAction(request);
}
@SdkInternalApi
final ListProvisioningArtifactsForServiceActionResult executeListProvisioningArtifactsForServiceAction(
ListProvisioningArtifactsForServiceActionRequest listProvisioningArtifactsForServiceActionRequest) {
ExecutionContext executionContext = createExecutionContext(listProvisioningArtifactsForServiceActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListProvisioningArtifactsForServiceActionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listProvisioningArtifactsForServiceActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListProvisioningArtifactsForServiceAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListProvisioningArtifactsForServiceActionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the specified requests or all performed requests.
*
*
* @param listRecordHistoryRequest
* @return Result of the ListRecordHistory operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListRecordHistory
* @see AWS API Documentation
*/
@Override
public ListRecordHistoryResult listRecordHistory(ListRecordHistoryRequest request) {
request = beforeClientExecution(request);
return executeListRecordHistory(request);
}
@SdkInternalApi
final ListRecordHistoryResult executeListRecordHistory(ListRecordHistoryRequest listRecordHistoryRequest) {
ExecutionContext executionContext = createExecutionContext(listRecordHistoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRecordHistoryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRecordHistoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRecordHistory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListRecordHistoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the resources associated with the specified TagOption.
*
*
* @param listResourcesForTagOptionRequest
* @return Result of the ListResourcesForTagOption operation returned by the service.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListResourcesForTagOption
* @see AWS API Documentation
*/
@Override
public ListResourcesForTagOptionResult listResourcesForTagOption(ListResourcesForTagOptionRequest request) {
request = beforeClientExecution(request);
return executeListResourcesForTagOption(request);
}
@SdkInternalApi
final ListResourcesForTagOptionResult executeListResourcesForTagOption(ListResourcesForTagOptionRequest listResourcesForTagOptionRequest) {
ExecutionContext executionContext = createExecutionContext(listResourcesForTagOptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListResourcesForTagOptionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listResourcesForTagOptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListResourcesForTagOption");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListResourcesForTagOptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all self-service actions.
*
*
* @param listServiceActionsRequest
* @return Result of the ListServiceActions operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListServiceActions
* @see AWS API Documentation
*/
@Override
public ListServiceActionsResult listServiceActions(ListServiceActionsRequest request) {
request = beforeClientExecution(request);
return executeListServiceActions(request);
}
@SdkInternalApi
final ListServiceActionsResult executeListServiceActions(ListServiceActionsRequest listServiceActionsRequest) {
ExecutionContext executionContext = createExecutionContext(listServiceActionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListServiceActionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listServiceActionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListServiceActions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListServiceActionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a paginated list of self-service actions associated with the specified Product ID and Provisioning
* Artifact ID.
*
*
* @param listServiceActionsForProvisioningArtifactRequest
* @return Result of the ListServiceActionsForProvisioningArtifact operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListServiceActionsForProvisioningArtifact
* @see AWS API Documentation
*/
@Override
public ListServiceActionsForProvisioningArtifactResult listServiceActionsForProvisioningArtifact(ListServiceActionsForProvisioningArtifactRequest request) {
request = beforeClientExecution(request);
return executeListServiceActionsForProvisioningArtifact(request);
}
@SdkInternalApi
final ListServiceActionsForProvisioningArtifactResult executeListServiceActionsForProvisioningArtifact(
ListServiceActionsForProvisioningArtifactRequest listServiceActionsForProvisioningArtifactRequest) {
ExecutionContext executionContext = createExecutionContext(listServiceActionsForProvisioningArtifactRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListServiceActionsForProvisioningArtifactRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listServiceActionsForProvisioningArtifactRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListServiceActionsForProvisioningArtifact");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListServiceActionsForProvisioningArtifactResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns summary information about stack instances that are associated with the specified
* CFN_STACKSET
type provisioned product. You can filter for stack instances that are associated with a
* specific Amazon Web Services account name or Region.
*
*
* @param listStackInstancesForProvisionedProductRequest
* @return Result of the ListStackInstancesForProvisionedProduct operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.ListStackInstancesForProvisionedProduct
* @see AWS API Documentation
*/
@Override
public ListStackInstancesForProvisionedProductResult listStackInstancesForProvisionedProduct(ListStackInstancesForProvisionedProductRequest request) {
request = beforeClientExecution(request);
return executeListStackInstancesForProvisionedProduct(request);
}
@SdkInternalApi
final ListStackInstancesForProvisionedProductResult executeListStackInstancesForProvisionedProduct(
ListStackInstancesForProvisionedProductRequest listStackInstancesForProvisionedProductRequest) {
ExecutionContext executionContext = createExecutionContext(listStackInstancesForProvisionedProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStackInstancesForProvisionedProductRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listStackInstancesForProvisionedProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStackInstancesForProvisionedProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListStackInstancesForProvisionedProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the specified TagOptions or all TagOptions.
*
*
* @param listTagOptionsRequest
* @return Result of the ListTagOptions operation returned by the service.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ListTagOptions
* @see AWS
* API Documentation
*/
@Override
public ListTagOptionsResult listTagOptions(ListTagOptionsRequest request) {
request = beforeClientExecution(request);
return executeListTagOptions(request);
}
@SdkInternalApi
final ListTagOptionsResult executeListTagOptions(ListTagOptionsRequest listTagOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(listTagOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagOptionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagOptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagOptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagOptionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Notifies the result of the provisioning engine execution.
*
*
* @param notifyProvisionProductEngineWorkflowResultRequest
* @return Result of the NotifyProvisionProductEngineWorkflowResult operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.NotifyProvisionProductEngineWorkflowResult
* @see AWS API Documentation
*/
@Override
public NotifyProvisionProductEngineWorkflowResultResult notifyProvisionProductEngineWorkflowResult(NotifyProvisionProductEngineWorkflowResultRequest request) {
request = beforeClientExecution(request);
return executeNotifyProvisionProductEngineWorkflowResult(request);
}
@SdkInternalApi
final NotifyProvisionProductEngineWorkflowResultResult executeNotifyProvisionProductEngineWorkflowResult(
NotifyProvisionProductEngineWorkflowResultRequest notifyProvisionProductEngineWorkflowResultRequest) {
ExecutionContext executionContext = createExecutionContext(notifyProvisionProductEngineWorkflowResultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new NotifyProvisionProductEngineWorkflowResultRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(notifyProvisionProductEngineWorkflowResultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "NotifyProvisionProductEngineWorkflowResult");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new NotifyProvisionProductEngineWorkflowResultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Notifies the result of the terminate engine execution.
*
*
* @param notifyTerminateProvisionedProductEngineWorkflowResultRequest
* @return Result of the NotifyTerminateProvisionedProductEngineWorkflowResult operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.NotifyTerminateProvisionedProductEngineWorkflowResult
* @see AWS API Documentation
*/
@Override
public NotifyTerminateProvisionedProductEngineWorkflowResultResult notifyTerminateProvisionedProductEngineWorkflowResult(
NotifyTerminateProvisionedProductEngineWorkflowResultRequest request) {
request = beforeClientExecution(request);
return executeNotifyTerminateProvisionedProductEngineWorkflowResult(request);
}
@SdkInternalApi
final NotifyTerminateProvisionedProductEngineWorkflowResultResult executeNotifyTerminateProvisionedProductEngineWorkflowResult(
NotifyTerminateProvisionedProductEngineWorkflowResultRequest notifyTerminateProvisionedProductEngineWorkflowResultRequest) {
ExecutionContext executionContext = createExecutionContext(notifyTerminateProvisionedProductEngineWorkflowResultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new NotifyTerminateProvisionedProductEngineWorkflowResultRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(notifyTerminateProvisionedProductEngineWorkflowResultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "NotifyTerminateProvisionedProductEngineWorkflowResult");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new NotifyTerminateProvisionedProductEngineWorkflowResultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Notifies the result of the update engine execution.
*
*
* @param notifyUpdateProvisionedProductEngineWorkflowResultRequest
* @return Result of the NotifyUpdateProvisionedProductEngineWorkflowResult operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.NotifyUpdateProvisionedProductEngineWorkflowResult
* @see AWS API Documentation
*/
@Override
public NotifyUpdateProvisionedProductEngineWorkflowResultResult notifyUpdateProvisionedProductEngineWorkflowResult(
NotifyUpdateProvisionedProductEngineWorkflowResultRequest request) {
request = beforeClientExecution(request);
return executeNotifyUpdateProvisionedProductEngineWorkflowResult(request);
}
@SdkInternalApi
final NotifyUpdateProvisionedProductEngineWorkflowResultResult executeNotifyUpdateProvisionedProductEngineWorkflowResult(
NotifyUpdateProvisionedProductEngineWorkflowResultRequest notifyUpdateProvisionedProductEngineWorkflowResultRequest) {
ExecutionContext executionContext = createExecutionContext(notifyUpdateProvisionedProductEngineWorkflowResultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new NotifyUpdateProvisionedProductEngineWorkflowResultRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(notifyUpdateProvisionedProductEngineWorkflowResultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "NotifyUpdateProvisionedProductEngineWorkflowResult");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new NotifyUpdateProvisionedProductEngineWorkflowResultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provisions the specified product.
*
*
* A provisioned product is a resourced instance of a product. For example, provisioning a product that's based on
* an CloudFormation template launches an CloudFormation stack and its underlying resources. You can check the
* status of this request using DescribeRecord.
*
*
* If the request contains a tag key with an empty list of values, there's a tag conflict for that key. Don't
* include conflicted keys as tags, or this will cause the error
* "Parameter validation failed: Missing required parameter in Tags[N]:Value".
*
*
*
* When provisioning a product that's been added to a portfolio, you must grant your user, group, or role access to
* the portfolio. For more information, see Granting users
* access in the Service Catalog User Guide.
*
*
*
* @param provisionProductRequest
* @return Result of the ProvisionProduct operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws DuplicateResourceException
* The specified resource is a duplicate.
* @sample AWSServiceCatalog.ProvisionProduct
* @see AWS API Documentation
*/
@Override
public ProvisionProductResult provisionProduct(ProvisionProductRequest request) {
request = beforeClientExecution(request);
return executeProvisionProduct(request);
}
@SdkInternalApi
final ProvisionProductResult executeProvisionProduct(ProvisionProductRequest provisionProductRequest) {
ExecutionContext executionContext = createExecutionContext(provisionProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ProvisionProductRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(provisionProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ProvisionProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ProvisionProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Rejects an offer to share the specified portfolio.
*
*
* @param rejectPortfolioShareRequest
* @return Result of the RejectPortfolioShare operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.RejectPortfolioShare
* @see AWS API Documentation
*/
@Override
public RejectPortfolioShareResult rejectPortfolioShare(RejectPortfolioShareRequest request) {
request = beforeClientExecution(request);
return executeRejectPortfolioShare(request);
}
@SdkInternalApi
final RejectPortfolioShareResult executeRejectPortfolioShare(RejectPortfolioShareRequest rejectPortfolioShareRequest) {
ExecutionContext executionContext = createExecutionContext(rejectPortfolioShareRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RejectPortfolioShareRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(rejectPortfolioShareRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RejectPortfolioShare");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RejectPortfolioShareResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the provisioned products that are available (not terminated).
*
*
* To use additional filtering, see SearchProvisionedProducts.
*
*
* @param scanProvisionedProductsRequest
* @return Result of the ScanProvisionedProducts operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.ScanProvisionedProducts
* @see AWS API Documentation
*/
@Override
public ScanProvisionedProductsResult scanProvisionedProducts(ScanProvisionedProductsRequest request) {
request = beforeClientExecution(request);
return executeScanProvisionedProducts(request);
}
@SdkInternalApi
final ScanProvisionedProductsResult executeScanProvisionedProducts(ScanProvisionedProductsRequest scanProvisionedProductsRequest) {
ExecutionContext executionContext = createExecutionContext(scanProvisionedProductsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ScanProvisionedProductsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(scanProvisionedProductsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ScanProvisionedProducts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ScanProvisionedProductsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the products to which the caller has access.
*
*
* @param searchProductsRequest
* @return Result of the SearchProducts operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.SearchProducts
* @see AWS
* API Documentation
*/
@Override
public SearchProductsResult searchProducts(SearchProductsRequest request) {
request = beforeClientExecution(request);
return executeSearchProducts(request);
}
@SdkInternalApi
final SearchProductsResult executeSearchProducts(SearchProductsRequest searchProductsRequest) {
ExecutionContext executionContext = createExecutionContext(searchProductsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchProductsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(searchProductsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchProducts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new SearchProductsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the products for the specified portfolio or all products.
*
*
* @param searchProductsAsAdminRequest
* @return Result of the SearchProductsAsAdmin operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.SearchProductsAsAdmin
* @see AWS API Documentation
*/
@Override
public SearchProductsAsAdminResult searchProductsAsAdmin(SearchProductsAsAdminRequest request) {
request = beforeClientExecution(request);
return executeSearchProductsAsAdmin(request);
}
@SdkInternalApi
final SearchProductsAsAdminResult executeSearchProductsAsAdmin(SearchProductsAsAdminRequest searchProductsAsAdminRequest) {
ExecutionContext executionContext = createExecutionContext(searchProductsAsAdminRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchProductsAsAdminRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(searchProductsAsAdminRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchProductsAsAdmin");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new SearchProductsAsAdminResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the provisioned products that meet the specified criteria.
*
*
* @param searchProvisionedProductsRequest
* @return Result of the SearchProvisionedProducts operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.SearchProvisionedProducts
* @see AWS API Documentation
*/
@Override
public SearchProvisionedProductsResult searchProvisionedProducts(SearchProvisionedProductsRequest request) {
request = beforeClientExecution(request);
return executeSearchProvisionedProducts(request);
}
@SdkInternalApi
final SearchProvisionedProductsResult executeSearchProvisionedProducts(SearchProvisionedProductsRequest searchProvisionedProductsRequest) {
ExecutionContext executionContext = createExecutionContext(searchProvisionedProductsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchProvisionedProductsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(searchProvisionedProductsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchProvisionedProducts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new SearchProvisionedProductsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Terminates the specified provisioned product.
*
*
* This operation does not delete any records associated with the provisioned product.
*
*
* You can check the status of this request using DescribeRecord.
*
*
* @param terminateProvisionedProductRequest
* @return Result of the TerminateProvisionedProduct operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AWSServiceCatalog.TerminateProvisionedProduct
* @see AWS API Documentation
*/
@Override
public TerminateProvisionedProductResult terminateProvisionedProduct(TerminateProvisionedProductRequest request) {
request = beforeClientExecution(request);
return executeTerminateProvisionedProduct(request);
}
@SdkInternalApi
final TerminateProvisionedProductResult executeTerminateProvisionedProduct(TerminateProvisionedProductRequest terminateProvisionedProductRequest) {
ExecutionContext executionContext = createExecutionContext(terminateProvisionedProductRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TerminateProvisionedProductRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(terminateProvisionedProductRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TerminateProvisionedProduct");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new TerminateProvisionedProductResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the specified constraint.
*
*
* @param updateConstraintRequest
* @return Result of the UpdateConstraint operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @sample AWSServiceCatalog.UpdateConstraint
* @see AWS API Documentation
*/
@Override
public UpdateConstraintResult updateConstraint(UpdateConstraintRequest request) {
request = beforeClientExecution(request);
return executeUpdateConstraint(request);
}
@SdkInternalApi
final UpdateConstraintResult executeUpdateConstraint(UpdateConstraintRequest updateConstraintRequest) {
ExecutionContext executionContext = createExecutionContext(updateConstraintRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateConstraintRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateConstraintRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateConstraint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateConstraintResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the specified portfolio.
*
*
* You cannot update a product that was shared with you.
*
*
* @param updatePortfolioRequest
* @return Result of the UpdatePortfolio operation returned by the service.
* @throws InvalidParametersException
* One or more parameters provided to the operation are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The current limits of the service would have been exceeded by this operation. Decrease your resource use
* or increase your service limits and retry the operation.
* @throws TagOptionNotMigratedException
* An operation requiring TagOptions failed because the TagOptions migration process has not been performed
* for this account. Use the Amazon Web Services Management Console to perform the migration process before
* retrying the operation.
* @sample AWSServiceCatalog.UpdatePortfolio
* @see AWS
* API Documentation
*/
@Override
public UpdatePortfolioResult updatePortfolio(UpdatePortfolioRequest request) {
request = beforeClientExecution(request);
return executeUpdatePortfolio(request);
}
@SdkInternalApi
final UpdatePortfolioResult executeUpdatePortfolio(UpdatePortfolioRequest updatePortfolioRequest) {
ExecutionContext executionContext = createExecutionContext(updatePortfolioRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdatePortfolioRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updatePortfolioRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Service Catalog");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdatePortfolio");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdatePortfolioResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the specified portfolio share. You can use this API to enable or disable