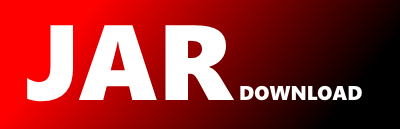
com.amazonaws.services.servicecatalog.model.UpdateConstraintRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.servicecatalog.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateConstraintRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The language code.
*
*
* -
*
* jp
- Japanese
*
*
* -
*
* zh
- Chinese
*
*
*
*/
private String acceptLanguage;
/**
*
* The identifier of the constraint.
*
*/
private String id;
/**
*
* The updated description of the constraint.
*
*/
private String description;
/**
*
* The constraint parameters, in JSON format. The syntax depends on the constraint type as follows:
*
*
* - LAUNCH
* -
*
* You are required to specify either the RoleArn
or the LocalRoleName
but can't use both.
*
*
* Specify the RoleArn
property as follows:
*
*
* {"RoleArn" : "arn:aws:iam::123456789012:role/LaunchRole"}
*
*
* Specify the LocalRoleName
property as follows:
*
*
* {"LocalRoleName": "SCBasicLaunchRole"}
*
*
* If you specify the LocalRoleName
property, when an account uses the launch constraint, the IAM role
* with that name in the account will be used. This allows launch-role constraints to be account-agnostic so the
* administrator can create fewer resources per shared account.
*
*
*
* The given role name must exist in the account used to create the launch constraint and the account of the user
* who launches a product with this launch constraint.
*
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one LAUNCH
constraint on a product and portfolio.
*
*
* - NOTIFICATION
* -
*
* Specify the NotificationArns
property as follows:
*
*
* {"NotificationArns" : ["arn:aws:sns:us-east-1:123456789012:Topic"]}
*
*
* - RESOURCE_UPDATE
* -
*
* Specify the TagUpdatesOnProvisionedProduct
property as follows:
*
*
* {"Version":"2.0","Properties":{"TagUpdateOnProvisionedProduct":"String"}}
*
*
* The TagUpdatesOnProvisionedProduct
property accepts a string value of ALLOWED
or
* NOT_ALLOWED
.
*
*
* - STACKSET
* -
*
* Specify the Parameters
property as follows:
*
*
* {"Version": "String", "Properties": {"AccountList": [ "String" ], "RegionList": [ "String" ], "AdminRole": "String", "ExecutionRole": "String"}}
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one STACKSET
constraint on a product and portfolio.
*
*
* Products with a STACKSET
constraint will launch an CloudFormation stack set.
*
*
* - TEMPLATE
* -
*
* Specify the Rules
property. For more information, see Template Constraint Rules.
*
*
*
*/
private String parameters;
/**
*
* The language code.
*
*
* -
*
* jp
- Japanese
*
*
* -
*
* zh
- Chinese
*
*
*
*
* @param acceptLanguage
* The language code.
*
* -
*
* jp
- Japanese
*
*
* -
*
* zh
- Chinese
*
*
*/
public void setAcceptLanguage(String acceptLanguage) {
this.acceptLanguage = acceptLanguage;
}
/**
*
* The language code.
*
*
* -
*
* jp
- Japanese
*
*
* -
*
* zh
- Chinese
*
*
*
*
* @return The language code.
*
* -
*
* jp
- Japanese
*
*
* -
*
* zh
- Chinese
*
*
*/
public String getAcceptLanguage() {
return this.acceptLanguage;
}
/**
*
* The language code.
*
*
* -
*
* jp
- Japanese
*
*
* -
*
* zh
- Chinese
*
*
*
*
* @param acceptLanguage
* The language code.
*
* -
*
* jp
- Japanese
*
*
* -
*
* zh
- Chinese
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateConstraintRequest withAcceptLanguage(String acceptLanguage) {
setAcceptLanguage(acceptLanguage);
return this;
}
/**
*
* The identifier of the constraint.
*
*
* @param id
* The identifier of the constraint.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The identifier of the constraint.
*
*
* @return The identifier of the constraint.
*/
public String getId() {
return this.id;
}
/**
*
* The identifier of the constraint.
*
*
* @param id
* The identifier of the constraint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateConstraintRequest withId(String id) {
setId(id);
return this;
}
/**
*
* The updated description of the constraint.
*
*
* @param description
* The updated description of the constraint.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The updated description of the constraint.
*
*
* @return The updated description of the constraint.
*/
public String getDescription() {
return this.description;
}
/**
*
* The updated description of the constraint.
*
*
* @param description
* The updated description of the constraint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateConstraintRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The constraint parameters, in JSON format. The syntax depends on the constraint type as follows:
*
*
* - LAUNCH
* -
*
* You are required to specify either the RoleArn
or the LocalRoleName
but can't use both.
*
*
* Specify the RoleArn
property as follows:
*
*
* {"RoleArn" : "arn:aws:iam::123456789012:role/LaunchRole"}
*
*
* Specify the LocalRoleName
property as follows:
*
*
* {"LocalRoleName": "SCBasicLaunchRole"}
*
*
* If you specify the LocalRoleName
property, when an account uses the launch constraint, the IAM role
* with that name in the account will be used. This allows launch-role constraints to be account-agnostic so the
* administrator can create fewer resources per shared account.
*
*
*
* The given role name must exist in the account used to create the launch constraint and the account of the user
* who launches a product with this launch constraint.
*
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one LAUNCH
constraint on a product and portfolio.
*
*
* - NOTIFICATION
* -
*
* Specify the NotificationArns
property as follows:
*
*
* {"NotificationArns" : ["arn:aws:sns:us-east-1:123456789012:Topic"]}
*
*
* - RESOURCE_UPDATE
* -
*
* Specify the TagUpdatesOnProvisionedProduct
property as follows:
*
*
* {"Version":"2.0","Properties":{"TagUpdateOnProvisionedProduct":"String"}}
*
*
* The TagUpdatesOnProvisionedProduct
property accepts a string value of ALLOWED
or
* NOT_ALLOWED
.
*
*
* - STACKSET
* -
*
* Specify the Parameters
property as follows:
*
*
* {"Version": "String", "Properties": {"AccountList": [ "String" ], "RegionList": [ "String" ], "AdminRole": "String", "ExecutionRole": "String"}}
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one STACKSET
constraint on a product and portfolio.
*
*
* Products with a STACKSET
constraint will launch an CloudFormation stack set.
*
*
* - TEMPLATE
* -
*
* Specify the Rules
property. For more information, see Template Constraint Rules.
*
*
*
*
* @param parameters
* The constraint parameters, in JSON format. The syntax depends on the constraint type as follows:
*
* - LAUNCH
* -
*
* You are required to specify either the RoleArn
or the LocalRoleName
but can't
* use both.
*
*
* Specify the RoleArn
property as follows:
*
*
* {"RoleArn" : "arn:aws:iam::123456789012:role/LaunchRole"}
*
*
* Specify the LocalRoleName
property as follows:
*
*
* {"LocalRoleName": "SCBasicLaunchRole"}
*
*
* If you specify the LocalRoleName
property, when an account uses the launch constraint, the
* IAM role with that name in the account will be used. This allows launch-role constraints to be
* account-agnostic so the administrator can create fewer resources per shared account.
*
*
*
* The given role name must exist in the account used to create the launch constraint and the account of the
* user who launches a product with this launch constraint.
*
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one LAUNCH
constraint on a product and portfolio.
*
*
* - NOTIFICATION
* -
*
* Specify the NotificationArns
property as follows:
*
*
* {"NotificationArns" : ["arn:aws:sns:us-east-1:123456789012:Topic"]}
*
*
* - RESOURCE_UPDATE
* -
*
* Specify the TagUpdatesOnProvisionedProduct
property as follows:
*
*
* {"Version":"2.0","Properties":{"TagUpdateOnProvisionedProduct":"String"}}
*
*
* The TagUpdatesOnProvisionedProduct
property accepts a string value of ALLOWED
or
* NOT_ALLOWED
.
*
*
* - STACKSET
* -
*
* Specify the Parameters
property as follows:
*
*
* {"Version": "String", "Properties": {"AccountList": [ "String" ], "RegionList": [ "String" ], "AdminRole": "String", "ExecutionRole": "String"}}
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one STACKSET
constraint on a product and portfolio.
*
*
* Products with a STACKSET
constraint will launch an CloudFormation stack set.
*
*
* - TEMPLATE
* -
*
* Specify the Rules
property. For more information, see Template Constraint Rules.
*
*
*/
public void setParameters(String parameters) {
this.parameters = parameters;
}
/**
*
* The constraint parameters, in JSON format. The syntax depends on the constraint type as follows:
*
*
* - LAUNCH
* -
*
* You are required to specify either the RoleArn
or the LocalRoleName
but can't use both.
*
*
* Specify the RoleArn
property as follows:
*
*
* {"RoleArn" : "arn:aws:iam::123456789012:role/LaunchRole"}
*
*
* Specify the LocalRoleName
property as follows:
*
*
* {"LocalRoleName": "SCBasicLaunchRole"}
*
*
* If you specify the LocalRoleName
property, when an account uses the launch constraint, the IAM role
* with that name in the account will be used. This allows launch-role constraints to be account-agnostic so the
* administrator can create fewer resources per shared account.
*
*
*
* The given role name must exist in the account used to create the launch constraint and the account of the user
* who launches a product with this launch constraint.
*
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one LAUNCH
constraint on a product and portfolio.
*
*
* - NOTIFICATION
* -
*
* Specify the NotificationArns
property as follows:
*
*
* {"NotificationArns" : ["arn:aws:sns:us-east-1:123456789012:Topic"]}
*
*
* - RESOURCE_UPDATE
* -
*
* Specify the TagUpdatesOnProvisionedProduct
property as follows:
*
*
* {"Version":"2.0","Properties":{"TagUpdateOnProvisionedProduct":"String"}}
*
*
* The TagUpdatesOnProvisionedProduct
property accepts a string value of ALLOWED
or
* NOT_ALLOWED
.
*
*
* - STACKSET
* -
*
* Specify the Parameters
property as follows:
*
*
* {"Version": "String", "Properties": {"AccountList": [ "String" ], "RegionList": [ "String" ], "AdminRole": "String", "ExecutionRole": "String"}}
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one STACKSET
constraint on a product and portfolio.
*
*
* Products with a STACKSET
constraint will launch an CloudFormation stack set.
*
*
* - TEMPLATE
* -
*
* Specify the Rules
property. For more information, see Template Constraint Rules.
*
*
*
*
* @return The constraint parameters, in JSON format. The syntax depends on the constraint type as follows:
*
* - LAUNCH
* -
*
* You are required to specify either the RoleArn
or the LocalRoleName
but can't
* use both.
*
*
* Specify the RoleArn
property as follows:
*
*
* {"RoleArn" : "arn:aws:iam::123456789012:role/LaunchRole"}
*
*
* Specify the LocalRoleName
property as follows:
*
*
* {"LocalRoleName": "SCBasicLaunchRole"}
*
*
* If you specify the LocalRoleName
property, when an account uses the launch constraint, the
* IAM role with that name in the account will be used. This allows launch-role constraints to be
* account-agnostic so the administrator can create fewer resources per shared account.
*
*
*
* The given role name must exist in the account used to create the launch constraint and the account of the
* user who launches a product with this launch constraint.
*
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one LAUNCH
constraint on a product and portfolio.
*
*
* - NOTIFICATION
* -
*
* Specify the NotificationArns
property as follows:
*
*
* {"NotificationArns" : ["arn:aws:sns:us-east-1:123456789012:Topic"]}
*
*
* - RESOURCE_UPDATE
* -
*
* Specify the TagUpdatesOnProvisionedProduct
property as follows:
*
*
* {"Version":"2.0","Properties":{"TagUpdateOnProvisionedProduct":"String"}}
*
*
* The TagUpdatesOnProvisionedProduct
property accepts a string value of ALLOWED
* or NOT_ALLOWED
.
*
*
* - STACKSET
* -
*
* Specify the Parameters
property as follows:
*
*
* {"Version": "String", "Properties": {"AccountList": [ "String" ], "RegionList": [ "String" ], "AdminRole": "String", "ExecutionRole": "String"}}
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one STACKSET
constraint on a product and portfolio.
*
*
* Products with a STACKSET
constraint will launch an CloudFormation stack set.
*
*
* - TEMPLATE
* -
*
* Specify the Rules
property. For more information, see Template Constraint Rules.
*
*
*/
public String getParameters() {
return this.parameters;
}
/**
*
* The constraint parameters, in JSON format. The syntax depends on the constraint type as follows:
*
*
* - LAUNCH
* -
*
* You are required to specify either the RoleArn
or the LocalRoleName
but can't use both.
*
*
* Specify the RoleArn
property as follows:
*
*
* {"RoleArn" : "arn:aws:iam::123456789012:role/LaunchRole"}
*
*
* Specify the LocalRoleName
property as follows:
*
*
* {"LocalRoleName": "SCBasicLaunchRole"}
*
*
* If you specify the LocalRoleName
property, when an account uses the launch constraint, the IAM role
* with that name in the account will be used. This allows launch-role constraints to be account-agnostic so the
* administrator can create fewer resources per shared account.
*
*
*
* The given role name must exist in the account used to create the launch constraint and the account of the user
* who launches a product with this launch constraint.
*
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one LAUNCH
constraint on a product and portfolio.
*
*
* - NOTIFICATION
* -
*
* Specify the NotificationArns
property as follows:
*
*
* {"NotificationArns" : ["arn:aws:sns:us-east-1:123456789012:Topic"]}
*
*
* - RESOURCE_UPDATE
* -
*
* Specify the TagUpdatesOnProvisionedProduct
property as follows:
*
*
* {"Version":"2.0","Properties":{"TagUpdateOnProvisionedProduct":"String"}}
*
*
* The TagUpdatesOnProvisionedProduct
property accepts a string value of ALLOWED
or
* NOT_ALLOWED
.
*
*
* - STACKSET
* -
*
* Specify the Parameters
property as follows:
*
*
* {"Version": "String", "Properties": {"AccountList": [ "String" ], "RegionList": [ "String" ], "AdminRole": "String", "ExecutionRole": "String"}}
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one STACKSET
constraint on a product and portfolio.
*
*
* Products with a STACKSET
constraint will launch an CloudFormation stack set.
*
*
* - TEMPLATE
* -
*
* Specify the Rules
property. For more information, see Template Constraint Rules.
*
*
*
*
* @param parameters
* The constraint parameters, in JSON format. The syntax depends on the constraint type as follows:
*
* - LAUNCH
* -
*
* You are required to specify either the RoleArn
or the LocalRoleName
but can't
* use both.
*
*
* Specify the RoleArn
property as follows:
*
*
* {"RoleArn" : "arn:aws:iam::123456789012:role/LaunchRole"}
*
*
* Specify the LocalRoleName
property as follows:
*
*
* {"LocalRoleName": "SCBasicLaunchRole"}
*
*
* If you specify the LocalRoleName
property, when an account uses the launch constraint, the
* IAM role with that name in the account will be used. This allows launch-role constraints to be
* account-agnostic so the administrator can create fewer resources per shared account.
*
*
*
* The given role name must exist in the account used to create the launch constraint and the account of the
* user who launches a product with this launch constraint.
*
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one LAUNCH
constraint on a product and portfolio.
*
*
* - NOTIFICATION
* -
*
* Specify the NotificationArns
property as follows:
*
*
* {"NotificationArns" : ["arn:aws:sns:us-east-1:123456789012:Topic"]}
*
*
* - RESOURCE_UPDATE
* -
*
* Specify the TagUpdatesOnProvisionedProduct
property as follows:
*
*
* {"Version":"2.0","Properties":{"TagUpdateOnProvisionedProduct":"String"}}
*
*
* The TagUpdatesOnProvisionedProduct
property accepts a string value of ALLOWED
or
* NOT_ALLOWED
.
*
*
* - STACKSET
* -
*
* Specify the Parameters
property as follows:
*
*
* {"Version": "String", "Properties": {"AccountList": [ "String" ], "RegionList": [ "String" ], "AdminRole": "String", "ExecutionRole": "String"}}
*
*
* You cannot have both a LAUNCH
and a STACKSET
constraint.
*
*
* You also cannot have more than one STACKSET
constraint on a product and portfolio.
*
*
* Products with a STACKSET
constraint will launch an CloudFormation stack set.
*
*
* - TEMPLATE
* -
*
* Specify the Rules
property. For more information, see Template Constraint Rules.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateConstraintRequest withParameters(String parameters) {
setParameters(parameters);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAcceptLanguage() != null)
sb.append("AcceptLanguage: ").append(getAcceptLanguage()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateConstraintRequest == false)
return false;
UpdateConstraintRequest other = (UpdateConstraintRequest) obj;
if (other.getAcceptLanguage() == null ^ this.getAcceptLanguage() == null)
return false;
if (other.getAcceptLanguage() != null && other.getAcceptLanguage().equals(this.getAcceptLanguage()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAcceptLanguage() == null) ? 0 : getAcceptLanguage().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
return hashCode;
}
@Override
public UpdateConstraintRequest clone() {
return (UpdateConstraintRequest) super.clone();
}
}