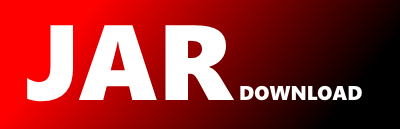
com.amazonaws.services.servicequotas.AWSServiceQuotasAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-servicequotas Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.servicequotas;
import javax.annotation.Generated;
import com.amazonaws.services.servicequotas.model.*;
/**
* Interface for accessing Service Quotas asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.servicequotas.AbstractAWSServiceQuotasAsync} instead.
*
*
*
* With Service Quotas, you can view and manage your quotas easily as your Amazon Web Services workloads grow. Quotas,
* also referred to as limits, are the maximum number of resources that you can create in your Amazon Web Services
* account. For more information, see the Service
* Quotas User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSServiceQuotasAsync extends AWSServiceQuotas {
/**
*
* Associates your quota request template with your organization. When a new Amazon Web Services account is created
* in your organization, the quota increase requests in the template are automatically applied to the account. You
* can add a quota increase request for any adjustable quota to your template.
*
*
* @param associateServiceQuotaTemplateRequest
* @return A Java Future containing the result of the AssociateServiceQuotaTemplate operation returned by the
* service.
* @sample AWSServiceQuotasAsync.AssociateServiceQuotaTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future associateServiceQuotaTemplateAsync(
AssociateServiceQuotaTemplateRequest associateServiceQuotaTemplateRequest);
/**
*
* Associates your quota request template with your organization. When a new Amazon Web Services account is created
* in your organization, the quota increase requests in the template are automatically applied to the account. You
* can add a quota increase request for any adjustable quota to your template.
*
*
* @param associateServiceQuotaTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateServiceQuotaTemplate operation returned by the
* service.
* @sample AWSServiceQuotasAsyncHandler.AssociateServiceQuotaTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future associateServiceQuotaTemplateAsync(
AssociateServiceQuotaTemplateRequest associateServiceQuotaTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the quota increase request for the specified quota from your quota request template.
*
*
* @param deleteServiceQuotaIncreaseRequestFromTemplateRequest
* @return A Java Future containing the result of the DeleteServiceQuotaIncreaseRequestFromTemplate operation
* returned by the service.
* @sample AWSServiceQuotasAsync.DeleteServiceQuotaIncreaseRequestFromTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteServiceQuotaIncreaseRequestFromTemplateAsync(
DeleteServiceQuotaIncreaseRequestFromTemplateRequest deleteServiceQuotaIncreaseRequestFromTemplateRequest);
/**
*
* Deletes the quota increase request for the specified quota from your quota request template.
*
*
* @param deleteServiceQuotaIncreaseRequestFromTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteServiceQuotaIncreaseRequestFromTemplate operation
* returned by the service.
* @sample AWSServiceQuotasAsyncHandler.DeleteServiceQuotaIncreaseRequestFromTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteServiceQuotaIncreaseRequestFromTemplateAsync(
DeleteServiceQuotaIncreaseRequestFromTemplateRequest deleteServiceQuotaIncreaseRequestFromTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables your quota request template. After a template is disabled, the quota increase requests in the template
* are not applied to new Amazon Web Services accounts in your organization. Disabling a quota request template does
* not apply its quota increase requests.
*
*
* @param disassociateServiceQuotaTemplateRequest
* @return A Java Future containing the result of the DisassociateServiceQuotaTemplate operation returned by the
* service.
* @sample AWSServiceQuotasAsync.DisassociateServiceQuotaTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateServiceQuotaTemplateAsync(
DisassociateServiceQuotaTemplateRequest disassociateServiceQuotaTemplateRequest);
/**
*
* Disables your quota request template. After a template is disabled, the quota increase requests in the template
* are not applied to new Amazon Web Services accounts in your organization. Disabling a quota request template does
* not apply its quota increase requests.
*
*
* @param disassociateServiceQuotaTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateServiceQuotaTemplate operation returned by the
* service.
* @sample AWSServiceQuotasAsyncHandler.DisassociateServiceQuotaTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateServiceQuotaTemplateAsync(
DisassociateServiceQuotaTemplateRequest disassociateServiceQuotaTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the default value for the specified quota. The default value does not reflect any quota increases.
*
*
* @param getAWSDefaultServiceQuotaRequest
* @return A Java Future containing the result of the GetAWSDefaultServiceQuota operation returned by the service.
* @sample AWSServiceQuotasAsync.GetAWSDefaultServiceQuota
* @see AWS API Documentation
*/
java.util.concurrent.Future getAWSDefaultServiceQuotaAsync(
GetAWSDefaultServiceQuotaRequest getAWSDefaultServiceQuotaRequest);
/**
*
* Retrieves the default value for the specified quota. The default value does not reflect any quota increases.
*
*
* @param getAWSDefaultServiceQuotaRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAWSDefaultServiceQuota operation returned by the service.
* @sample AWSServiceQuotasAsyncHandler.GetAWSDefaultServiceQuota
* @see AWS API Documentation
*/
java.util.concurrent.Future getAWSDefaultServiceQuotaAsync(
GetAWSDefaultServiceQuotaRequest getAWSDefaultServiceQuotaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the status of the association for the quota request template.
*
*
* @param getAssociationForServiceQuotaTemplateRequest
* @return A Java Future containing the result of the GetAssociationForServiceQuotaTemplate operation returned by
* the service.
* @sample AWSServiceQuotasAsync.GetAssociationForServiceQuotaTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssociationForServiceQuotaTemplateAsync(
GetAssociationForServiceQuotaTemplateRequest getAssociationForServiceQuotaTemplateRequest);
/**
*
* Retrieves the status of the association for the quota request template.
*
*
* @param getAssociationForServiceQuotaTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAssociationForServiceQuotaTemplate operation returned by
* the service.
* @sample AWSServiceQuotasAsyncHandler.GetAssociationForServiceQuotaTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssociationForServiceQuotaTemplateAsync(
GetAssociationForServiceQuotaTemplateRequest getAssociationForServiceQuotaTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the specified quota increase request.
*
*
* @param getRequestedServiceQuotaChangeRequest
* @return A Java Future containing the result of the GetRequestedServiceQuotaChange operation returned by the
* service.
* @sample AWSServiceQuotasAsync.GetRequestedServiceQuotaChange
* @see AWS API Documentation
*/
java.util.concurrent.Future getRequestedServiceQuotaChangeAsync(
GetRequestedServiceQuotaChangeRequest getRequestedServiceQuotaChangeRequest);
/**
*
* Retrieves information about the specified quota increase request.
*
*
* @param getRequestedServiceQuotaChangeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRequestedServiceQuotaChange operation returned by the
* service.
* @sample AWSServiceQuotasAsyncHandler.GetRequestedServiceQuotaChange
* @see AWS API Documentation
*/
java.util.concurrent.Future getRequestedServiceQuotaChangeAsync(
GetRequestedServiceQuotaChangeRequest getRequestedServiceQuotaChangeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the applied quota value for the specified quota. For some quotas, only the default values are
* available. If the applied quota value is not available for a quota, the quota is not retrieved.
*
*
* @param getServiceQuotaRequest
* @return A Java Future containing the result of the GetServiceQuota operation returned by the service.
* @sample AWSServiceQuotasAsync.GetServiceQuota
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getServiceQuotaAsync(GetServiceQuotaRequest getServiceQuotaRequest);
/**
*
* Retrieves the applied quota value for the specified quota. For some quotas, only the default values are
* available. If the applied quota value is not available for a quota, the quota is not retrieved.
*
*
* @param getServiceQuotaRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetServiceQuota operation returned by the service.
* @sample AWSServiceQuotasAsyncHandler.GetServiceQuota
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getServiceQuotaAsync(GetServiceQuotaRequest getServiceQuotaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the specified quota increase request in your quota request template.
*
*
* @param getServiceQuotaIncreaseRequestFromTemplateRequest
* @return A Java Future containing the result of the GetServiceQuotaIncreaseRequestFromTemplate operation returned
* by the service.
* @sample AWSServiceQuotasAsync.GetServiceQuotaIncreaseRequestFromTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getServiceQuotaIncreaseRequestFromTemplateAsync(
GetServiceQuotaIncreaseRequestFromTemplateRequest getServiceQuotaIncreaseRequestFromTemplateRequest);
/**
*
* Retrieves information about the specified quota increase request in your quota request template.
*
*
* @param getServiceQuotaIncreaseRequestFromTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetServiceQuotaIncreaseRequestFromTemplate operation returned
* by the service.
* @sample AWSServiceQuotasAsyncHandler.GetServiceQuotaIncreaseRequestFromTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getServiceQuotaIncreaseRequestFromTemplateAsync(
GetServiceQuotaIncreaseRequestFromTemplateRequest getServiceQuotaIncreaseRequestFromTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the default values for the quotas for the specified Amazon Web Service. A default value does not reflect
* any quota increases.
*
*
* @param listAWSDefaultServiceQuotasRequest
* @return A Java Future containing the result of the ListAWSDefaultServiceQuotas operation returned by the service.
* @sample AWSServiceQuotasAsync.ListAWSDefaultServiceQuotas
* @see AWS API Documentation
*/
java.util.concurrent.Future listAWSDefaultServiceQuotasAsync(
ListAWSDefaultServiceQuotasRequest listAWSDefaultServiceQuotasRequest);
/**
*
* Lists the default values for the quotas for the specified Amazon Web Service. A default value does not reflect
* any quota increases.
*
*
* @param listAWSDefaultServiceQuotasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAWSDefaultServiceQuotas operation returned by the service.
* @sample AWSServiceQuotasAsyncHandler.ListAWSDefaultServiceQuotas
* @see AWS API Documentation
*/
java.util.concurrent.Future listAWSDefaultServiceQuotasAsync(
ListAWSDefaultServiceQuotasRequest listAWSDefaultServiceQuotasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the quota increase requests for the specified Amazon Web Service.
*
*
* @param listRequestedServiceQuotaChangeHistoryRequest
* @return A Java Future containing the result of the ListRequestedServiceQuotaChangeHistory operation returned by
* the service.
* @sample AWSServiceQuotasAsync.ListRequestedServiceQuotaChangeHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future listRequestedServiceQuotaChangeHistoryAsync(
ListRequestedServiceQuotaChangeHistoryRequest listRequestedServiceQuotaChangeHistoryRequest);
/**
*
* Retrieves the quota increase requests for the specified Amazon Web Service.
*
*
* @param listRequestedServiceQuotaChangeHistoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRequestedServiceQuotaChangeHistory operation returned by
* the service.
* @sample AWSServiceQuotasAsyncHandler.ListRequestedServiceQuotaChangeHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future listRequestedServiceQuotaChangeHistoryAsync(
ListRequestedServiceQuotaChangeHistoryRequest listRequestedServiceQuotaChangeHistoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the quota increase requests for the specified quota.
*
*
* @param listRequestedServiceQuotaChangeHistoryByQuotaRequest
* @return A Java Future containing the result of the ListRequestedServiceQuotaChangeHistoryByQuota operation
* returned by the service.
* @sample AWSServiceQuotasAsync.ListRequestedServiceQuotaChangeHistoryByQuota
* @see AWS API Documentation
*/
java.util.concurrent.Future listRequestedServiceQuotaChangeHistoryByQuotaAsync(
ListRequestedServiceQuotaChangeHistoryByQuotaRequest listRequestedServiceQuotaChangeHistoryByQuotaRequest);
/**
*
* Retrieves the quota increase requests for the specified quota.
*
*
* @param listRequestedServiceQuotaChangeHistoryByQuotaRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRequestedServiceQuotaChangeHistoryByQuota operation
* returned by the service.
* @sample AWSServiceQuotasAsyncHandler.ListRequestedServiceQuotaChangeHistoryByQuota
* @see AWS API Documentation
*/
java.util.concurrent.Future listRequestedServiceQuotaChangeHistoryByQuotaAsync(
ListRequestedServiceQuotaChangeHistoryByQuotaRequest listRequestedServiceQuotaChangeHistoryByQuotaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the quota increase requests in the specified quota request template.
*
*
* @param listServiceQuotaIncreaseRequestsInTemplateRequest
* @return A Java Future containing the result of the ListServiceQuotaIncreaseRequestsInTemplate operation returned
* by the service.
* @sample AWSServiceQuotasAsync.ListServiceQuotaIncreaseRequestsInTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceQuotaIncreaseRequestsInTemplateAsync(
ListServiceQuotaIncreaseRequestsInTemplateRequest listServiceQuotaIncreaseRequestsInTemplateRequest);
/**
*
* Lists the quota increase requests in the specified quota request template.
*
*
* @param listServiceQuotaIncreaseRequestsInTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServiceQuotaIncreaseRequestsInTemplate operation returned
* by the service.
* @sample AWSServiceQuotasAsyncHandler.ListServiceQuotaIncreaseRequestsInTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceQuotaIncreaseRequestsInTemplateAsync(
ListServiceQuotaIncreaseRequestsInTemplateRequest listServiceQuotaIncreaseRequestsInTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the applied quota values for the specified Amazon Web Service. For some quotas, only the default values are
* available. If the applied quota value is not available for a quota, the quota is not retrieved.
*
*
* @param listServiceQuotasRequest
* @return A Java Future containing the result of the ListServiceQuotas operation returned by the service.
* @sample AWSServiceQuotasAsync.ListServiceQuotas
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceQuotasAsync(ListServiceQuotasRequest listServiceQuotasRequest);
/**
*
* Lists the applied quota values for the specified Amazon Web Service. For some quotas, only the default values are
* available. If the applied quota value is not available for a quota, the quota is not retrieved.
*
*
* @param listServiceQuotasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServiceQuotas operation returned by the service.
* @sample AWSServiceQuotasAsyncHandler.ListServiceQuotas
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceQuotasAsync(ListServiceQuotasRequest listServiceQuotasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the names and codes for the Amazon Web Services integrated with Service Quotas.
*
*
* @param listServicesRequest
* @return A Java Future containing the result of the ListServices operation returned by the service.
* @sample AWSServiceQuotasAsync.ListServices
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listServicesAsync(ListServicesRequest listServicesRequest);
/**
*
* Lists the names and codes for the Amazon Web Services integrated with Service Quotas.
*
*
* @param listServicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServices operation returned by the service.
* @sample AWSServiceQuotasAsyncHandler.ListServices
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listServicesAsync(ListServicesRequest listServicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the tags assigned to the specified applied quota.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSServiceQuotasAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns a list of the tags assigned to the specified applied quota.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSServiceQuotasAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a quota increase request to your quota request template.
*
*
* @param putServiceQuotaIncreaseRequestIntoTemplateRequest
* @return A Java Future containing the result of the PutServiceQuotaIncreaseRequestIntoTemplate operation returned
* by the service.
* @sample AWSServiceQuotasAsync.PutServiceQuotaIncreaseRequestIntoTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future putServiceQuotaIncreaseRequestIntoTemplateAsync(
PutServiceQuotaIncreaseRequestIntoTemplateRequest putServiceQuotaIncreaseRequestIntoTemplateRequest);
/**
*
* Adds a quota increase request to your quota request template.
*
*
* @param putServiceQuotaIncreaseRequestIntoTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutServiceQuotaIncreaseRequestIntoTemplate operation returned
* by the service.
* @sample AWSServiceQuotasAsyncHandler.PutServiceQuotaIncreaseRequestIntoTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future putServiceQuotaIncreaseRequestIntoTemplateAsync(
PutServiceQuotaIncreaseRequestIntoTemplateRequest putServiceQuotaIncreaseRequestIntoTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Submits a quota increase request for the specified quota.
*
*
* @param requestServiceQuotaIncreaseRequest
* @return A Java Future containing the result of the RequestServiceQuotaIncrease operation returned by the service.
* @sample AWSServiceQuotasAsync.RequestServiceQuotaIncrease
* @see AWS API Documentation
*/
java.util.concurrent.Future requestServiceQuotaIncreaseAsync(
RequestServiceQuotaIncreaseRequest requestServiceQuotaIncreaseRequest);
/**
*
* Submits a quota increase request for the specified quota.
*
*
* @param requestServiceQuotaIncreaseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RequestServiceQuotaIncrease operation returned by the service.
* @sample AWSServiceQuotasAsyncHandler.RequestServiceQuotaIncrease
* @see AWS API Documentation
*/
java.util.concurrent.Future requestServiceQuotaIncreaseAsync(
RequestServiceQuotaIncreaseRequest requestServiceQuotaIncreaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds tags to the specified applied quota. You can include one or more tags to add to the quota.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSServiceQuotasAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds tags to the specified applied quota. You can include one or more tags to add to the quota.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSServiceQuotasAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from the specified applied quota. You can specify one or more tags to remove.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSServiceQuotasAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from the specified applied quota. You can specify one or more tags to remove.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSServiceQuotasAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}