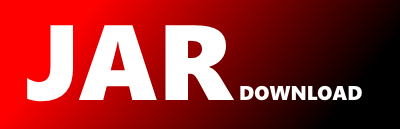
com.amazonaws.services.servicequotas.AWSServiceQuotas Maven / Gradle / Ivy
Show all versions of aws-java-sdk-servicequotas Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.servicequotas;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.servicequotas.model.*;
/**
* Interface for accessing Service Quotas.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.servicequotas.AbstractAWSServiceQuotas} instead.
*
*
*
* With Service Quotas, you can view and manage your quotas easily as your Amazon Web Services workloads grow. Quotas,
* also referred to as limits, are the maximum number of resources that you can create in your Amazon Web Services
* account. For more information, see the Service
* Quotas User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSServiceQuotas {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "servicequotas";
/**
*
* Associates your quota request template with your organization. When a new Amazon Web Services account is created
* in your organization, the quota increase requests in the template are automatically applied to the account. You
* can add a quota increase request for any adjustable quota to your template.
*
*
* @param associateServiceQuotaTemplateRequest
* @return Result of the AssociateServiceQuotaTemplate operation returned by the service.
* @throws DependencyAccessDeniedException
* You can't perform this action because a dependency does not have access.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws AWSServiceAccessNotEnabledException
* The action you attempted is not allowed unless Service Access with Service Quotas is enabled in your
* organization.
* @throws OrganizationNotInAllFeaturesModeException
* The organization that your Amazon Web Services account belongs to is not in All Features mode.
* @throws TemplatesNotAvailableInRegionException
* The Service Quotas template is not available in this Amazon Web Services Region.
* @throws NoAvailableOrganizationException
* The Amazon Web Services account making this call is not a member of an organization.
* @sample AWSServiceQuotas.AssociateServiceQuotaTemplate
* @see AWS API Documentation
*/
AssociateServiceQuotaTemplateResult associateServiceQuotaTemplate(AssociateServiceQuotaTemplateRequest associateServiceQuotaTemplateRequest);
/**
*
* Deletes the quota increase request for the specified quota from your quota request template.
*
*
* @param deleteServiceQuotaIncreaseRequestFromTemplateRequest
* @return Result of the DeleteServiceQuotaIncreaseRequestFromTemplate operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws ServiceException
* Something went wrong.
* @throws DependencyAccessDeniedException
* You can't perform this action because a dependency does not have access.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws AWSServiceAccessNotEnabledException
* The action you attempted is not allowed unless Service Access with Service Quotas is enabled in your
* organization.
* @throws TemplatesNotAvailableInRegionException
* The Service Quotas template is not available in this Amazon Web Services Region.
* @throws NoAvailableOrganizationException
* The Amazon Web Services account making this call is not a member of an organization.
* @sample AWSServiceQuotas.DeleteServiceQuotaIncreaseRequestFromTemplate
* @see AWS API Documentation
*/
DeleteServiceQuotaIncreaseRequestFromTemplateResult deleteServiceQuotaIncreaseRequestFromTemplate(
DeleteServiceQuotaIncreaseRequestFromTemplateRequest deleteServiceQuotaIncreaseRequestFromTemplateRequest);
/**
*
* Disables your quota request template. After a template is disabled, the quota increase requests in the template
* are not applied to new Amazon Web Services accounts in your organization. Disabling a quota request template does
* not apply its quota increase requests.
*
*
* @param disassociateServiceQuotaTemplateRequest
* @return Result of the DisassociateServiceQuotaTemplate operation returned by the service.
* @throws DependencyAccessDeniedException
* You can't perform this action because a dependency does not have access.
* @throws ServiceQuotaTemplateNotInUseException
* The quota request template is not associated with your organization.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws AWSServiceAccessNotEnabledException
* The action you attempted is not allowed unless Service Access with Service Quotas is enabled in your
* organization.
* @throws TemplatesNotAvailableInRegionException
* The Service Quotas template is not available in this Amazon Web Services Region.
* @throws NoAvailableOrganizationException
* The Amazon Web Services account making this call is not a member of an organization.
* @sample AWSServiceQuotas.DisassociateServiceQuotaTemplate
* @see AWS API Documentation
*/
DisassociateServiceQuotaTemplateResult disassociateServiceQuotaTemplate(DisassociateServiceQuotaTemplateRequest disassociateServiceQuotaTemplateRequest);
/**
*
* Retrieves the default value for the specified quota. The default value does not reflect any quota increases.
*
*
* @param getAWSDefaultServiceQuotaRequest
* @return Result of the GetAWSDefaultServiceQuota operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @sample AWSServiceQuotas.GetAWSDefaultServiceQuota
* @see AWS API Documentation
*/
GetAWSDefaultServiceQuotaResult getAWSDefaultServiceQuota(GetAWSDefaultServiceQuotaRequest getAWSDefaultServiceQuotaRequest);
/**
*
* Retrieves the status of the association for the quota request template.
*
*
* @param getAssociationForServiceQuotaTemplateRequest
* @return Result of the GetAssociationForServiceQuotaTemplate operation returned by the service.
* @throws DependencyAccessDeniedException
* You can't perform this action because a dependency does not have access.
* @throws ServiceQuotaTemplateNotInUseException
* The quota request template is not associated with your organization.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws AWSServiceAccessNotEnabledException
* The action you attempted is not allowed unless Service Access with Service Quotas is enabled in your
* organization.
* @throws TemplatesNotAvailableInRegionException
* The Service Quotas template is not available in this Amazon Web Services Region.
* @throws NoAvailableOrganizationException
* The Amazon Web Services account making this call is not a member of an organization.
* @sample AWSServiceQuotas.GetAssociationForServiceQuotaTemplate
* @see AWS API Documentation
*/
GetAssociationForServiceQuotaTemplateResult getAssociationForServiceQuotaTemplate(
GetAssociationForServiceQuotaTemplateRequest getAssociationForServiceQuotaTemplateRequest);
/**
*
* Retrieves information about the specified quota increase request.
*
*
* @param getRequestedServiceQuotaChangeRequest
* @return Result of the GetRequestedServiceQuotaChange operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @sample AWSServiceQuotas.GetRequestedServiceQuotaChange
* @see AWS API Documentation
*/
GetRequestedServiceQuotaChangeResult getRequestedServiceQuotaChange(GetRequestedServiceQuotaChangeRequest getRequestedServiceQuotaChangeRequest);
/**
*
* Retrieves the applied quota value for the specified quota. For some quotas, only the default values are
* available. If the applied quota value is not available for a quota, the quota is not retrieved.
*
*
* @param getServiceQuotaRequest
* @return Result of the GetServiceQuota operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @sample AWSServiceQuotas.GetServiceQuota
* @see AWS
* API Documentation
*/
GetServiceQuotaResult getServiceQuota(GetServiceQuotaRequest getServiceQuotaRequest);
/**
*
* Retrieves information about the specified quota increase request in your quota request template.
*
*
* @param getServiceQuotaIncreaseRequestFromTemplateRequest
* @return Result of the GetServiceQuotaIncreaseRequestFromTemplate operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws DependencyAccessDeniedException
* You can't perform this action because a dependency does not have access.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws AWSServiceAccessNotEnabledException
* The action you attempted is not allowed unless Service Access with Service Quotas is enabled in your
* organization.
* @throws TemplatesNotAvailableInRegionException
* The Service Quotas template is not available in this Amazon Web Services Region.
* @throws NoAvailableOrganizationException
* The Amazon Web Services account making this call is not a member of an organization.
* @sample AWSServiceQuotas.GetServiceQuotaIncreaseRequestFromTemplate
* @see AWS API Documentation
*/
GetServiceQuotaIncreaseRequestFromTemplateResult getServiceQuotaIncreaseRequestFromTemplate(
GetServiceQuotaIncreaseRequestFromTemplateRequest getServiceQuotaIncreaseRequestFromTemplateRequest);
/**
*
* Lists the default values for the quotas for the specified Amazon Web Service. A default value does not reflect
* any quota increases.
*
*
* @param listAWSDefaultServiceQuotasRequest
* @return Result of the ListAWSDefaultServiceQuotas operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws InvalidPaginationTokenException
* Invalid input was provided.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @sample AWSServiceQuotas.ListAWSDefaultServiceQuotas
* @see AWS API Documentation
*/
ListAWSDefaultServiceQuotasResult listAWSDefaultServiceQuotas(ListAWSDefaultServiceQuotasRequest listAWSDefaultServiceQuotasRequest);
/**
*
* Retrieves the quota increase requests for the specified Amazon Web Service.
*
*
* @param listRequestedServiceQuotaChangeHistoryRequest
* @return Result of the ListRequestedServiceQuotaChangeHistory operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws InvalidPaginationTokenException
* Invalid input was provided.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @sample AWSServiceQuotas.ListRequestedServiceQuotaChangeHistory
* @see AWS API Documentation
*/
ListRequestedServiceQuotaChangeHistoryResult listRequestedServiceQuotaChangeHistory(
ListRequestedServiceQuotaChangeHistoryRequest listRequestedServiceQuotaChangeHistoryRequest);
/**
*
* Retrieves the quota increase requests for the specified quota.
*
*
* @param listRequestedServiceQuotaChangeHistoryByQuotaRequest
* @return Result of the ListRequestedServiceQuotaChangeHistoryByQuota operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws InvalidPaginationTokenException
* Invalid input was provided.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @sample AWSServiceQuotas.ListRequestedServiceQuotaChangeHistoryByQuota
* @see AWS API Documentation
*/
ListRequestedServiceQuotaChangeHistoryByQuotaResult listRequestedServiceQuotaChangeHistoryByQuota(
ListRequestedServiceQuotaChangeHistoryByQuotaRequest listRequestedServiceQuotaChangeHistoryByQuotaRequest);
/**
*
* Lists the quota increase requests in the specified quota request template.
*
*
* @param listServiceQuotaIncreaseRequestsInTemplateRequest
* @return Result of the ListServiceQuotaIncreaseRequestsInTemplate operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws DependencyAccessDeniedException
* You can't perform this action because a dependency does not have access.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws AWSServiceAccessNotEnabledException
* The action you attempted is not allowed unless Service Access with Service Quotas is enabled in your
* organization.
* @throws TemplatesNotAvailableInRegionException
* The Service Quotas template is not available in this Amazon Web Services Region.
* @throws NoAvailableOrganizationException
* The Amazon Web Services account making this call is not a member of an organization.
* @sample AWSServiceQuotas.ListServiceQuotaIncreaseRequestsInTemplate
* @see AWS API Documentation
*/
ListServiceQuotaIncreaseRequestsInTemplateResult listServiceQuotaIncreaseRequestsInTemplate(
ListServiceQuotaIncreaseRequestsInTemplateRequest listServiceQuotaIncreaseRequestsInTemplateRequest);
/**
*
* Lists the applied quota values for the specified Amazon Web Service. For some quotas, only the default values are
* available. If the applied quota value is not available for a quota, the quota is not retrieved.
*
*
* @param listServiceQuotasRequest
* @return Result of the ListServiceQuotas operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws InvalidPaginationTokenException
* Invalid input was provided.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @sample AWSServiceQuotas.ListServiceQuotas
* @see AWS API Documentation
*/
ListServiceQuotasResult listServiceQuotas(ListServiceQuotasRequest listServiceQuotasRequest);
/**
*
* Lists the names and codes for the Amazon Web Services integrated with Service Quotas.
*
*
* @param listServicesRequest
* @return Result of the ListServices operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws InvalidPaginationTokenException
* Invalid input was provided.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @sample AWSServiceQuotas.ListServices
* @see AWS
* API Documentation
*/
ListServicesResult listServices(ListServicesRequest listServicesRequest);
/**
*
* Returns a list of the tags assigned to the specified applied quota.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws ServiceException
* Something went wrong.
* @sample AWSServiceQuotas.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Adds a quota increase request to your quota request template.
*
*
* @param putServiceQuotaIncreaseRequestIntoTemplateRequest
* @return Result of the PutServiceQuotaIncreaseRequestIntoTemplate operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws DependencyAccessDeniedException
* You can't perform this action because a dependency does not have access.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws QuotaExceededException
* You have exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use Service Quotas to request a service quota increase.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws AWSServiceAccessNotEnabledException
* The action you attempted is not allowed unless Service Access with Service Quotas is enabled in your
* organization.
* @throws TemplatesNotAvailableInRegionException
* The Service Quotas template is not available in this Amazon Web Services Region.
* @throws NoAvailableOrganizationException
* The Amazon Web Services account making this call is not a member of an organization.
* @sample AWSServiceQuotas.PutServiceQuotaIncreaseRequestIntoTemplate
* @see AWS API Documentation
*/
PutServiceQuotaIncreaseRequestIntoTemplateResult putServiceQuotaIncreaseRequestIntoTemplate(
PutServiceQuotaIncreaseRequestIntoTemplateRequest putServiceQuotaIncreaseRequestIntoTemplateRequest);
/**
*
* Submits a quota increase request for the specified quota.
*
*
* @param requestServiceQuotaIncreaseRequest
* @return Result of the RequestServiceQuotaIncrease operation returned by the service.
* @throws DependencyAccessDeniedException
* You can't perform this action because a dependency does not have access.
* @throws QuotaExceededException
* You have exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use Service Quotas to request a service quota increase.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws InvalidResourceStateException
* The resource is in an invalid state.
* @throws ServiceException
* Something went wrong.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @sample AWSServiceQuotas.RequestServiceQuotaIncrease
* @see AWS API Documentation
*/
RequestServiceQuotaIncreaseResult requestServiceQuotaIncrease(RequestServiceQuotaIncreaseRequest requestServiceQuotaIncreaseRequest);
/**
*
* Adds tags to the specified applied quota. You can include one or more tags to add to the quota.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws TooManyTagsException
* You've exceeded the number of tags allowed for a resource. For more information, see Tag
* restrictions in the Service Quotas User Guide.
* @throws TagPolicyViolationException
* The specified tag is a reserved word and cannot be used.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws ServiceException
* Something went wrong.
* @sample AWSServiceQuotas.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from the specified applied quota. You can specify one or more tags to remove.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws TooManyRequestsException
* Due to throttling, the request was denied. Slow down the rate of request calls, or request an increase
* for this quota.
* @throws NoSuchResourceException
* The specified resource does not exist.
* @throws IllegalArgumentException
* Invalid input was provided.
* @throws AccessDeniedException
* You do not have sufficient permission to perform this action.
* @throws ServiceException
* Something went wrong.
* @sample AWSServiceQuotas.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}