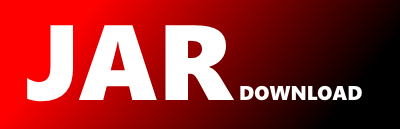
com.amazonaws.services.simpleemail.AmazonSimpleEmailServiceClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ses Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simpleemail;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.services.simpleemail.AmazonSimpleEmailServiceClientBuilder;
import com.amazonaws.services.simpleemail.waiters.AmazonSimpleEmailServiceWaiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.simpleemail.model.*;
import com.amazonaws.services.simpleemail.model.transform.*;
/**
* Client for accessing Amazon SES. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* Amazon Simple Email Service
*
* This is the API Reference for Amazon Simple Email Service (Amazon SES). This documentation is intended to be used in
* conjunction with the Amazon SES Developer
* Guide.
*
*
*
* For a list of Amazon SES endpoints to use in service requests, see Regions and Amazon SES in the Amazon SES
* Developer Guide.
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonSimpleEmailServiceClient extends AmazonWebServiceClient implements AmazonSimpleEmailService {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonSimpleEmailService.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "ses";
private volatile AmazonSimpleEmailServiceWaiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
/**
* List of exception unmarshallers for all modeled exceptions
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
/**
* Constructs a new client to invoke service methods on Amazon SES. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonSimpleEmailServiceClientBuilder#defaultClient()}
*/
@Deprecated
public AmazonSimpleEmailServiceClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon SES. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon SES (ex: proxy settings,
* retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonSimpleEmailServiceClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonSimpleEmailServiceClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on Amazon SES using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AmazonSimpleEmailServiceClientBuilder#withCredentials(AWSCredentialsProvider)} for
* example:
* {@code AmazonSimpleEmailServiceClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AmazonSimpleEmailServiceClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon SES using the specified AWS account credentials and
* client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon SES (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AmazonSimpleEmailServiceClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonSimpleEmailServiceClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonSimpleEmailServiceClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on Amazon SES using the specified AWS account credentials
* provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AmazonSimpleEmailServiceClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AmazonSimpleEmailServiceClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon SES using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon SES (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AmazonSimpleEmailServiceClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonSimpleEmailServiceClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonSimpleEmailServiceClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on Amazon SES using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon SES (ex: proxy settings,
* retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AmazonSimpleEmailServiceClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonSimpleEmailServiceClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AmazonSimpleEmailServiceClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AmazonSimpleEmailServiceClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
public static AmazonSimpleEmailServiceClientBuilder builder() {
return AmazonSimpleEmailServiceClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon SES using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonSimpleEmailServiceClient(AwsSyncClientParams clientParams) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
init();
}
private void init() {
exceptionUnmarshallers.add(new InvalidFirehoseDestinationExceptionUnmarshaller());
exceptionUnmarshallers.add(new ConfigurationSetDoesNotExistExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidS3ConfigurationExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidSNSDestinationExceptionUnmarshaller());
exceptionUnmarshallers.add(new EventDestinationAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new MessageRejectedExceptionUnmarshaller());
exceptionUnmarshallers.add(new RuleDoesNotExistExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidCloudWatchDestinationExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidConfigurationSetExceptionUnmarshaller());
exceptionUnmarshallers.add(new RuleSetDoesNotExistExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidSnsTopicExceptionUnmarshaller());
exceptionUnmarshallers.add(new LimitExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new EventDestinationDoesNotExistExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidLambdaFunctionExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidPolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new AlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new CannotDeleteExceptionUnmarshaller());
exceptionUnmarshallers.add(new ConfigurationSetAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new MailFromDomainNotVerifiedExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller(com.amazonaws.services.simpleemail.model.AmazonSimpleEmailServiceException.class));
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("https://email.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/simpleemail/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/simpleemail/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates a receipt rule set by cloning an existing one. All receipt rules and configurations are copied to the new
* receipt rule set and are completely independent of the source rule set.
*
*
* For information about setting up rule sets, see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param cloneReceiptRuleSetRequest
* Represents a request to create a receipt rule set by cloning an existing one. You use receipt rule sets to
* receive email with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the CloneReceiptRuleSet operation returned by the service.
* @throws RuleSetDoesNotExistException
* Indicates that the provided receipt rule set does not exist.
* @throws AlreadyExistsException
* Indicates that a resource could not be created because of a naming conflict.
* @throws LimitExceededException
* Indicates that a resource could not be created because of service limits. For a list of Amazon SES
* limits, see the Amazon SES
* Developer Guide.
* @sample AmazonSimpleEmailService.CloneReceiptRuleSet
* @see AWS API
* Documentation
*/
@Override
public CloneReceiptRuleSetResult cloneReceiptRuleSet(CloneReceiptRuleSetRequest request) {
request = beforeClientExecution(request);
return executeCloneReceiptRuleSet(request);
}
@SdkInternalApi
final CloneReceiptRuleSetResult executeCloneReceiptRuleSet(CloneReceiptRuleSetRequest cloneReceiptRuleSetRequest) {
ExecutionContext executionContext = createExecutionContext(cloneReceiptRuleSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CloneReceiptRuleSetRequestMarshaller().marshall(super.beforeMarshalling(cloneReceiptRuleSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CloneReceiptRuleSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a configuration set.
*
*
* Configuration sets enable you to publish email sending events. For information about using configuration sets,
* see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createConfigurationSetRequest
* Represents a request to create a configuration set. Configuration sets enable you to publish email sending
* events. For information about using configuration sets, see the Amazon SES
* Developer Guide.
* @return Result of the CreateConfigurationSet operation returned by the service.
* @throws ConfigurationSetAlreadyExistsException
* Indicates that the configuration set could not be created because of a naming conflict.
* @throws InvalidConfigurationSetException
* Indicates that the configuration set is invalid. See the error message for details.
* @throws LimitExceededException
* Indicates that a resource could not be created because of service limits. For a list of Amazon SES
* limits, see the Amazon SES
* Developer Guide.
* @sample AmazonSimpleEmailService.CreateConfigurationSet
* @see AWS
* API Documentation
*/
@Override
public CreateConfigurationSetResult createConfigurationSet(CreateConfigurationSetRequest request) {
request = beforeClientExecution(request);
return executeCreateConfigurationSet(request);
}
@SdkInternalApi
final CreateConfigurationSetResult executeCreateConfigurationSet(CreateConfigurationSetRequest createConfigurationSetRequest) {
ExecutionContext executionContext = createExecutionContext(createConfigurationSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConfigurationSetRequestMarshaller().marshall(super.beforeMarshalling(createConfigurationSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateConfigurationSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a configuration set event destination.
*
*
*
* When you create or update an event destination, you must provide one, and only one, destination. The destination
* can be Amazon CloudWatch, Amazon Kinesis Firehose, or Amazon Simple Notification Service (Amazon SNS).
*
*
*
* An event destination is the AWS service to which Amazon SES publishes the email sending events associated with a
* configuration set. For information about using configuration sets, see the Amazon SES Developer
* Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createConfigurationSetEventDestinationRequest
* Represents a request to create a configuration set event destination. A configuration set event
* destination, which can be either Amazon CloudWatch or Amazon Kinesis Firehose, describes an AWS service in
* which Amazon SES publishes the email sending events associated with a configuration set. For information
* about using configuration sets, see the Amazon SES
* Developer Guide.
* @return Result of the CreateConfigurationSetEventDestination operation returned by the service.
* @throws ConfigurationSetDoesNotExistException
* Indicates that the configuration set does not exist.
* @throws EventDestinationAlreadyExistsException
* Indicates that the event destination could not be created because of a naming conflict.
* @throws InvalidCloudWatchDestinationException
* Indicates that the Amazon CloudWatch destination is invalid. See the error message for details.
* @throws InvalidFirehoseDestinationException
* Indicates that the Amazon Kinesis Firehose destination is invalid. See the error message for details.
* @throws InvalidSNSDestinationException
* Indicates that the Amazon Simple Notification Service (Amazon SNS) destination is invalid. See the error
* message for details.
* @throws LimitExceededException
* Indicates that a resource could not be created because of service limits. For a list of Amazon SES
* limits, see the Amazon SES
* Developer Guide.
* @sample AmazonSimpleEmailService.CreateConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public CreateConfigurationSetEventDestinationResult createConfigurationSetEventDestination(CreateConfigurationSetEventDestinationRequest request) {
request = beforeClientExecution(request);
return executeCreateConfigurationSetEventDestination(request);
}
@SdkInternalApi
final CreateConfigurationSetEventDestinationResult executeCreateConfigurationSetEventDestination(
CreateConfigurationSetEventDestinationRequest createConfigurationSetEventDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(createConfigurationSetEventDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConfigurationSetEventDestinationRequestMarshaller().marshall(super
.beforeMarshalling(createConfigurationSetEventDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateConfigurationSetEventDestinationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new IP address filter.
*
*
* For information about setting up IP address filters, see the Amazon SES Developer
* Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createReceiptFilterRequest
* Represents a request to create a new IP address filter. You use IP address filters when you receive email
* with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the CreateReceiptFilter operation returned by the service.
* @throws LimitExceededException
* Indicates that a resource could not be created because of service limits. For a list of Amazon SES
* limits, see the Amazon SES
* Developer Guide.
* @throws AlreadyExistsException
* Indicates that a resource could not be created because of a naming conflict.
* @sample AmazonSimpleEmailService.CreateReceiptFilter
* @see AWS API
* Documentation
*/
@Override
public CreateReceiptFilterResult createReceiptFilter(CreateReceiptFilterRequest request) {
request = beforeClientExecution(request);
return executeCreateReceiptFilter(request);
}
@SdkInternalApi
final CreateReceiptFilterResult executeCreateReceiptFilter(CreateReceiptFilterRequest createReceiptFilterRequest) {
ExecutionContext executionContext = createExecutionContext(createReceiptFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateReceiptFilterRequestMarshaller().marshall(super.beforeMarshalling(createReceiptFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateReceiptFilterResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a receipt rule.
*
*
* For information about setting up receipt rules, see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createReceiptRuleRequest
* Represents a request to create a receipt rule. You use receipt rules to receive email with Amazon SES. For
* more information, see the Amazon SES
* Developer Guide.
* @return Result of the CreateReceiptRule operation returned by the service.
* @throws InvalidSnsTopicException
* Indicates that the provided Amazon SNS topic is invalid, or that Amazon SES could not publish to the
* topic, possibly due to permissions issues. For information about giving permissions, see the Amazon SES
* Developer Guide.
* @throws InvalidS3ConfigurationException
* Indicates that the provided Amazon S3 bucket or AWS KMS encryption key is invalid, or that Amazon SES
* could not publish to the bucket, possibly due to permissions issues. For information about giving
* permissions, see the Amazon SES
* Developer Guide.
* @throws InvalidLambdaFunctionException
* Indicates that the provided AWS Lambda function is invalid, or that Amazon SES could not execute the
* provided function, possibly due to permissions issues. For information about giving permissions, see the
* Amazon
* SES Developer Guide.
* @throws AlreadyExistsException
* Indicates that a resource could not be created because of a naming conflict.
* @throws RuleDoesNotExistException
* Indicates that the provided receipt rule does not exist.
* @throws RuleSetDoesNotExistException
* Indicates that the provided receipt rule set does not exist.
* @throws LimitExceededException
* Indicates that a resource could not be created because of service limits. For a list of Amazon SES
* limits, see the Amazon SES
* Developer Guide.
* @sample AmazonSimpleEmailService.CreateReceiptRule
* @see AWS API
* Documentation
*/
@Override
public CreateReceiptRuleResult createReceiptRule(CreateReceiptRuleRequest request) {
request = beforeClientExecution(request);
return executeCreateReceiptRule(request);
}
@SdkInternalApi
final CreateReceiptRuleResult executeCreateReceiptRule(CreateReceiptRuleRequest createReceiptRuleRequest) {
ExecutionContext executionContext = createExecutionContext(createReceiptRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateReceiptRuleRequestMarshaller().marshall(super.beforeMarshalling(createReceiptRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateReceiptRuleResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an empty receipt rule set.
*
*
* For information about setting up receipt rule sets, see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createReceiptRuleSetRequest
* Represents a request to create an empty receipt rule set. You use receipt rule sets to receive email with
* Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the CreateReceiptRuleSet operation returned by the service.
* @throws AlreadyExistsException
* Indicates that a resource could not be created because of a naming conflict.
* @throws LimitExceededException
* Indicates that a resource could not be created because of service limits. For a list of Amazon SES
* limits, see the Amazon SES
* Developer Guide.
* @sample AmazonSimpleEmailService.CreateReceiptRuleSet
* @see AWS API
* Documentation
*/
@Override
public CreateReceiptRuleSetResult createReceiptRuleSet(CreateReceiptRuleSetRequest request) {
request = beforeClientExecution(request);
return executeCreateReceiptRuleSet(request);
}
@SdkInternalApi
final CreateReceiptRuleSetResult executeCreateReceiptRuleSet(CreateReceiptRuleSetRequest createReceiptRuleSetRequest) {
ExecutionContext executionContext = createExecutionContext(createReceiptRuleSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateReceiptRuleSetRequestMarshaller().marshall(super.beforeMarshalling(createReceiptRuleSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateReceiptRuleSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a configuration set.
*
*
* Configuration sets enable you to publish email sending events. For information about using configuration sets,
* see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteConfigurationSetRequest
* Represents a request to delete a configuration set. Configuration sets enable you to publish email sending
* events. For information about using configuration sets, see the Amazon SES
* Developer Guide.
* @return Result of the DeleteConfigurationSet operation returned by the service.
* @throws ConfigurationSetDoesNotExistException
* Indicates that the configuration set does not exist.
* @sample AmazonSimpleEmailService.DeleteConfigurationSet
* @see AWS
* API Documentation
*/
@Override
public DeleteConfigurationSetResult deleteConfigurationSet(DeleteConfigurationSetRequest request) {
request = beforeClientExecution(request);
return executeDeleteConfigurationSet(request);
}
@SdkInternalApi
final DeleteConfigurationSetResult executeDeleteConfigurationSet(DeleteConfigurationSetRequest deleteConfigurationSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConfigurationSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConfigurationSetRequestMarshaller().marshall(super.beforeMarshalling(deleteConfigurationSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteConfigurationSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a configuration set event destination.
*
*
* Configuration set event destinations are associated with configuration sets, which enable you to publish email
* sending events. For information about using configuration sets, see the Amazon SES Developer
* Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteConfigurationSetEventDestinationRequest
* Represents a request to delete a configuration set event destination. Configuration set event destinations
* are associated with configuration sets, which enable you to publish email sending events. For information
* about using configuration sets, see the Amazon SES
* Developer Guide.
* @return Result of the DeleteConfigurationSetEventDestination operation returned by the service.
* @throws ConfigurationSetDoesNotExistException
* Indicates that the configuration set does not exist.
* @throws EventDestinationDoesNotExistException
* Indicates that the event destination does not exist.
* @sample AmazonSimpleEmailService.DeleteConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public DeleteConfigurationSetEventDestinationResult deleteConfigurationSetEventDestination(DeleteConfigurationSetEventDestinationRequest request) {
request = beforeClientExecution(request);
return executeDeleteConfigurationSetEventDestination(request);
}
@SdkInternalApi
final DeleteConfigurationSetEventDestinationResult executeDeleteConfigurationSetEventDestination(
DeleteConfigurationSetEventDestinationRequest deleteConfigurationSetEventDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConfigurationSetEventDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConfigurationSetEventDestinationRequestMarshaller().marshall(super
.beforeMarshalling(deleteConfigurationSetEventDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteConfigurationSetEventDestinationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified identity (an email address or a domain) from the list of verified identities.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteIdentityRequest
* Represents a request to delete one of your Amazon SES identities (an email address or domain).
* @return Result of the DeleteIdentity operation returned by the service.
* @sample AmazonSimpleEmailService.DeleteIdentity
* @see AWS API
* Documentation
*/
@Override
public DeleteIdentityResult deleteIdentity(DeleteIdentityRequest request) {
request = beforeClientExecution(request);
return executeDeleteIdentity(request);
}
@SdkInternalApi
final DeleteIdentityResult executeDeleteIdentity(DeleteIdentityRequest deleteIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(deleteIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteIdentityRequestMarshaller().marshall(super.beforeMarshalling(deleteIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteIdentityResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified sending authorization policy for the given identity (an email address or a domain). This
* API returns successfully even if a policy with the specified name does not exist.
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteIdentityPolicyRequest
* Represents a request to delete a sending authorization policy for an identity. Sending authorization is an
* Amazon SES feature that enables you to authorize other senders to use your identities. For information,
* see the Amazon
* SES Developer Guide.
* @return Result of the DeleteIdentityPolicy operation returned by the service.
* @sample AmazonSimpleEmailService.DeleteIdentityPolicy
* @see AWS API
* Documentation
*/
@Override
public DeleteIdentityPolicyResult deleteIdentityPolicy(DeleteIdentityPolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteIdentityPolicy(request);
}
@SdkInternalApi
final DeleteIdentityPolicyResult executeDeleteIdentityPolicy(DeleteIdentityPolicyRequest deleteIdentityPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteIdentityPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteIdentityPolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteIdentityPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteIdentityPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified IP address filter.
*
*
* For information about managing IP address filters, see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteReceiptFilterRequest
* Represents a request to delete an IP address filter. You use IP address filters when you receive email
* with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the DeleteReceiptFilter operation returned by the service.
* @sample AmazonSimpleEmailService.DeleteReceiptFilter
* @see AWS API
* Documentation
*/
@Override
public DeleteReceiptFilterResult deleteReceiptFilter(DeleteReceiptFilterRequest request) {
request = beforeClientExecution(request);
return executeDeleteReceiptFilter(request);
}
@SdkInternalApi
final DeleteReceiptFilterResult executeDeleteReceiptFilter(DeleteReceiptFilterRequest deleteReceiptFilterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteReceiptFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteReceiptFilterRequestMarshaller().marshall(super.beforeMarshalling(deleteReceiptFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteReceiptFilterResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified receipt rule.
*
*
* For information about managing receipt rules, see the Amazon
* SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteReceiptRuleRequest
* Represents a request to delete a receipt rule. You use receipt rules to receive email with Amazon SES. For
* more information, see the Amazon SES
* Developer Guide.
* @return Result of the DeleteReceiptRule operation returned by the service.
* @throws RuleSetDoesNotExistException
* Indicates that the provided receipt rule set does not exist.
* @sample AmazonSimpleEmailService.DeleteReceiptRule
* @see AWS API
* Documentation
*/
@Override
public DeleteReceiptRuleResult deleteReceiptRule(DeleteReceiptRuleRequest request) {
request = beforeClientExecution(request);
return executeDeleteReceiptRule(request);
}
@SdkInternalApi
final DeleteReceiptRuleResult executeDeleteReceiptRule(DeleteReceiptRuleRequest deleteReceiptRuleRequest) {
ExecutionContext executionContext = createExecutionContext(deleteReceiptRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteReceiptRuleRequestMarshaller().marshall(super.beforeMarshalling(deleteReceiptRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteReceiptRuleResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified receipt rule set and all of the receipt rules it contains.
*
*
*
* The currently active rule set cannot be deleted.
*
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteReceiptRuleSetRequest
* Represents a request to delete a receipt rule set and all of the receipt rules it contains. You use
* receipt rule sets to receive email with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the DeleteReceiptRuleSet operation returned by the service.
* @throws CannotDeleteException
* Indicates that the delete operation could not be completed.
* @sample AmazonSimpleEmailService.DeleteReceiptRuleSet
* @see AWS API
* Documentation
*/
@Override
public DeleteReceiptRuleSetResult deleteReceiptRuleSet(DeleteReceiptRuleSetRequest request) {
request = beforeClientExecution(request);
return executeDeleteReceiptRuleSet(request);
}
@SdkInternalApi
final DeleteReceiptRuleSetResult executeDeleteReceiptRuleSet(DeleteReceiptRuleSetRequest deleteReceiptRuleSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteReceiptRuleSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteReceiptRuleSetRequestMarshaller().marshall(super.beforeMarshalling(deleteReceiptRuleSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteReceiptRuleSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified email address from the list of verified addresses.
*
*
*
* The DeleteVerifiedEmailAddress action is deprecated as of the May 15, 2012 release of Domain Verification. The
* DeleteIdentity action is now preferred.
*
*
*
* This action is throttled at one request per second.
*
*
* @param deleteVerifiedEmailAddressRequest
* Represents a request to delete an email address from the list of email addresses you have attempted to
* verify under your AWS account.
* @return Result of the DeleteVerifiedEmailAddress operation returned by the service.
* @sample AmazonSimpleEmailService.DeleteVerifiedEmailAddress
* @see AWS API Documentation
*/
@Override
public DeleteVerifiedEmailAddressResult deleteVerifiedEmailAddress(DeleteVerifiedEmailAddressRequest request) {
request = beforeClientExecution(request);
return executeDeleteVerifiedEmailAddress(request);
}
@SdkInternalApi
final DeleteVerifiedEmailAddressResult executeDeleteVerifiedEmailAddress(DeleteVerifiedEmailAddressRequest deleteVerifiedEmailAddressRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVerifiedEmailAddressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVerifiedEmailAddressRequestMarshaller().marshall(super.beforeMarshalling(deleteVerifiedEmailAddressRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteVerifiedEmailAddressResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the metadata and receipt rules for the receipt rule set that is currently active.
*
*
* For information about setting up receipt rule sets, see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeActiveReceiptRuleSetRequest
* Represents a request to return the metadata and receipt rules for the receipt rule set that is currently
* active. You use receipt rule sets to receive email with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the DescribeActiveReceiptRuleSet operation returned by the service.
* @sample AmazonSimpleEmailService.DescribeActiveReceiptRuleSet
* @see AWS API Documentation
*/
@Override
public DescribeActiveReceiptRuleSetResult describeActiveReceiptRuleSet(DescribeActiveReceiptRuleSetRequest request) {
request = beforeClientExecution(request);
return executeDescribeActiveReceiptRuleSet(request);
}
@SdkInternalApi
final DescribeActiveReceiptRuleSetResult executeDescribeActiveReceiptRuleSet(DescribeActiveReceiptRuleSetRequest describeActiveReceiptRuleSetRequest) {
ExecutionContext executionContext = createExecutionContext(describeActiveReceiptRuleSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeActiveReceiptRuleSetRequestMarshaller().marshall(super.beforeMarshalling(describeActiveReceiptRuleSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeActiveReceiptRuleSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the details of the specified configuration set.
*
*
* Configuration sets enable you to publish email sending events. For information about using configuration sets,
* see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeConfigurationSetRequest
* Represents a request to return the details of a configuration set. Configuration sets enable you to
* publish email sending events. For information about using configuration sets, see the Amazon SES
* Developer Guide.
* @return Result of the DescribeConfigurationSet operation returned by the service.
* @throws ConfigurationSetDoesNotExistException
* Indicates that the configuration set does not exist.
* @sample AmazonSimpleEmailService.DescribeConfigurationSet
* @see AWS
* API Documentation
*/
@Override
public DescribeConfigurationSetResult describeConfigurationSet(DescribeConfigurationSetRequest request) {
request = beforeClientExecution(request);
return executeDescribeConfigurationSet(request);
}
@SdkInternalApi
final DescribeConfigurationSetResult executeDescribeConfigurationSet(DescribeConfigurationSetRequest describeConfigurationSetRequest) {
ExecutionContext executionContext = createExecutionContext(describeConfigurationSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeConfigurationSetRequestMarshaller().marshall(super.beforeMarshalling(describeConfigurationSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeConfigurationSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the details of the specified receipt rule.
*
*
* For information about setting up receipt rules, see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeReceiptRuleRequest
* Represents a request to return the details of a receipt rule. You use receipt rules to receive email with
* Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the DescribeReceiptRule operation returned by the service.
* @throws RuleDoesNotExistException
* Indicates that the provided receipt rule does not exist.
* @throws RuleSetDoesNotExistException
* Indicates that the provided receipt rule set does not exist.
* @sample AmazonSimpleEmailService.DescribeReceiptRule
* @see AWS API
* Documentation
*/
@Override
public DescribeReceiptRuleResult describeReceiptRule(DescribeReceiptRuleRequest request) {
request = beforeClientExecution(request);
return executeDescribeReceiptRule(request);
}
@SdkInternalApi
final DescribeReceiptRuleResult executeDescribeReceiptRule(DescribeReceiptRuleRequest describeReceiptRuleRequest) {
ExecutionContext executionContext = createExecutionContext(describeReceiptRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReceiptRuleRequestMarshaller().marshall(super.beforeMarshalling(describeReceiptRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeReceiptRuleResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the details of the specified receipt rule set.
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeReceiptRuleSetRequest
* Represents a request to return the details of a receipt rule set. You use receipt rule sets to receive
* email with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the DescribeReceiptRuleSet operation returned by the service.
* @throws RuleSetDoesNotExistException
* Indicates that the provided receipt rule set does not exist.
* @sample AmazonSimpleEmailService.DescribeReceiptRuleSet
* @see AWS
* API Documentation
*/
@Override
public DescribeReceiptRuleSetResult describeReceiptRuleSet(DescribeReceiptRuleSetRequest request) {
request = beforeClientExecution(request);
return executeDescribeReceiptRuleSet(request);
}
@SdkInternalApi
final DescribeReceiptRuleSetResult executeDescribeReceiptRuleSet(DescribeReceiptRuleSetRequest describeReceiptRuleSetRequest) {
ExecutionContext executionContext = createExecutionContext(describeReceiptRuleSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReceiptRuleSetRequestMarshaller().marshall(super.beforeMarshalling(describeReceiptRuleSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeReceiptRuleSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the current status of Easy DKIM signing for an entity. For domain name identities, this action also
* returns the DKIM tokens that are required for Easy DKIM signing, and whether Amazon SES has successfully verified
* that these tokens have been published.
*
*
* This action takes a list of identities as input and returns the following information for each:
*
*
* -
*
* Whether Easy DKIM signing is enabled or disabled.
*
*
* -
*
* A set of DKIM tokens that represent the identity. If the identity is an email address, the tokens represent the
* domain of that address.
*
*
* -
*
* Whether Amazon SES has successfully verified the DKIM tokens published in the domain's DNS. This information is
* only returned for domain name identities, not for email addresses.
*
*
*
*
* This action is throttled at one request per second and can only get DKIM attributes for up to 100 identities at a
* time.
*
*
* For more information about creating DNS records using DKIM tokens, go to the Amazon SES Developer
* Guide.
*
*
* @param getIdentityDkimAttributesRequest
* Represents a request for the status of Amazon SES Easy DKIM signing for an identity. For domain
* identities, this request also returns the DKIM tokens that are required for Easy DKIM signing, and whether
* Amazon SES successfully verified that these tokens were published. For more information about Easy DKIM,
* see the Amazon SES Developer
* Guide.
* @return Result of the GetIdentityDkimAttributes operation returned by the service.
* @sample AmazonSimpleEmailService.GetIdentityDkimAttributes
* @see AWS API Documentation
*/
@Override
public GetIdentityDkimAttributesResult getIdentityDkimAttributes(GetIdentityDkimAttributesRequest request) {
request = beforeClientExecution(request);
return executeGetIdentityDkimAttributes(request);
}
@SdkInternalApi
final GetIdentityDkimAttributesResult executeGetIdentityDkimAttributes(GetIdentityDkimAttributesRequest getIdentityDkimAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(getIdentityDkimAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetIdentityDkimAttributesRequestMarshaller().marshall(super.beforeMarshalling(getIdentityDkimAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetIdentityDkimAttributesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the custom MAIL FROM attributes for a list of identities (email addresses and/or domains).
*
*
* This action is throttled at one request per second and can only get custom MAIL FROM attributes for up to 100
* identities at a time.
*
*
* @param getIdentityMailFromDomainAttributesRequest
* Represents a request to return the Amazon SES custom MAIL FROM attributes for a list of identities. For
* information about using a custom MAIL FROM domain, see the Amazon SES Developer Guide.
* @return Result of the GetIdentityMailFromDomainAttributes operation returned by the service.
* @sample AmazonSimpleEmailService.GetIdentityMailFromDomainAttributes
* @see AWS API Documentation
*/
@Override
public GetIdentityMailFromDomainAttributesResult getIdentityMailFromDomainAttributes(GetIdentityMailFromDomainAttributesRequest request) {
request = beforeClientExecution(request);
return executeGetIdentityMailFromDomainAttributes(request);
}
@SdkInternalApi
final GetIdentityMailFromDomainAttributesResult executeGetIdentityMailFromDomainAttributes(
GetIdentityMailFromDomainAttributesRequest getIdentityMailFromDomainAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(getIdentityMailFromDomainAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetIdentityMailFromDomainAttributesRequestMarshaller().marshall(super
.beforeMarshalling(getIdentityMailFromDomainAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetIdentityMailFromDomainAttributesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Given a list of verified identities (email addresses and/or domains), returns a structure describing identity
* notification attributes.
*
*
* This action is throttled at one request per second and can only get notification attributes for up to 100
* identities at a time.
*
*
* For more information about using notifications with Amazon SES, see the Amazon SES Developer Guide.
*
*
* @param getIdentityNotificationAttributesRequest
* Represents a request to return the notification attributes for a list of identities you verified with
* Amazon SES. For information about Amazon SES notifications, see the Amazon SES Developer
* Guide.
* @return Result of the GetIdentityNotificationAttributes operation returned by the service.
* @sample AmazonSimpleEmailService.GetIdentityNotificationAttributes
* @see AWS API Documentation
*/
@Override
public GetIdentityNotificationAttributesResult getIdentityNotificationAttributes(GetIdentityNotificationAttributesRequest request) {
request = beforeClientExecution(request);
return executeGetIdentityNotificationAttributes(request);
}
@SdkInternalApi
final GetIdentityNotificationAttributesResult executeGetIdentityNotificationAttributes(
GetIdentityNotificationAttributesRequest getIdentityNotificationAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(getIdentityNotificationAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetIdentityNotificationAttributesRequestMarshaller().marshall(super.beforeMarshalling(getIdentityNotificationAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetIdentityNotificationAttributesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the requested sending authorization policies for the given identity (an email address or a domain). The
* policies are returned as a map of policy names to policy contents. You can retrieve a maximum of 20 policies at a
* time.
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param getIdentityPoliciesRequest
* Represents a request to return the requested sending authorization policies for an identity. Sending
* authorization is an Amazon SES feature that enables you to authorize other senders to use your identities.
* For information, see the Amazon SES
* Developer Guide.
* @return Result of the GetIdentityPolicies operation returned by the service.
* @sample AmazonSimpleEmailService.GetIdentityPolicies
* @see AWS API
* Documentation
*/
@Override
public GetIdentityPoliciesResult getIdentityPolicies(GetIdentityPoliciesRequest request) {
request = beforeClientExecution(request);
return executeGetIdentityPolicies(request);
}
@SdkInternalApi
final GetIdentityPoliciesResult executeGetIdentityPolicies(GetIdentityPoliciesRequest getIdentityPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(getIdentityPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetIdentityPoliciesRequestMarshaller().marshall(super.beforeMarshalling(getIdentityPoliciesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetIdentityPoliciesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Given a list of identities (email addresses and/or domains), returns the verification status and (for domain
* identities) the verification token for each identity.
*
*
* The verification status of an email address is "Pending" until the email address owner clicks the link within the
* verification email that Amazon SES sent to that address. If the email address owner clicks the link within 24
* hours, the verification status of the email address changes to "Success". If the link is not clicked within 24
* hours, the verification status changes to "Failed." In that case, if you still want to verify the email address,
* you must restart the verification process from the beginning.
*
*
* For domain identities, the domain's verification status is "Pending" as Amazon SES searches for the required TXT
* record in the DNS settings of the domain. When Amazon SES detects the record, the domain's verification status
* changes to "Success". If Amazon SES is unable to detect the record within 72 hours, the domain's verification
* status changes to "Failed." In that case, if you still want to verify the domain, you must restart the
* verification process from the beginning.
*
*
* This action is throttled at one request per second and can only get verification attributes for up to 100
* identities at a time.
*
*
* @param getIdentityVerificationAttributesRequest
* Represents a request to return the Amazon SES verification status of a list of identities. For domain
* identities, this request also returns the verification token. For information about verifying identities
* with Amazon SES, see the Amazon SES
* Developer Guide.
* @return Result of the GetIdentityVerificationAttributes operation returned by the service.
* @sample AmazonSimpleEmailService.GetIdentityVerificationAttributes
* @see AWS API Documentation
*/
@Override
public GetIdentityVerificationAttributesResult getIdentityVerificationAttributes(GetIdentityVerificationAttributesRequest request) {
request = beforeClientExecution(request);
return executeGetIdentityVerificationAttributes(request);
}
@SdkInternalApi
final GetIdentityVerificationAttributesResult executeGetIdentityVerificationAttributes(
GetIdentityVerificationAttributesRequest getIdentityVerificationAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(getIdentityVerificationAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetIdentityVerificationAttributesRequestMarshaller().marshall(super.beforeMarshalling(getIdentityVerificationAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetIdentityVerificationAttributesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the user's current sending limits.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendQuotaRequest
* @return Result of the GetSendQuota operation returned by the service.
* @sample AmazonSimpleEmailService.GetSendQuota
* @see AWS API
* Documentation
*/
@Override
public GetSendQuotaResult getSendQuota(GetSendQuotaRequest request) {
request = beforeClientExecution(request);
return executeGetSendQuota(request);
}
@SdkInternalApi
final GetSendQuotaResult executeGetSendQuota(GetSendQuotaRequest getSendQuotaRequest) {
ExecutionContext executionContext = createExecutionContext(getSendQuotaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSendQuotaRequestMarshaller().marshall(super.beforeMarshalling(getSendQuotaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new GetSendQuotaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GetSendQuotaResult getSendQuota() {
return getSendQuota(new GetSendQuotaRequest());
}
/**
*
* Returns the user's sending statistics. The result is a list of data points, representing the last two weeks of
* sending activity.
*
*
* Each data point in the list contains statistics for a 15-minute interval.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendStatisticsRequest
* @return Result of the GetSendStatistics operation returned by the service.
* @sample AmazonSimpleEmailService.GetSendStatistics
* @see AWS API
* Documentation
*/
@Override
public GetSendStatisticsResult getSendStatistics(GetSendStatisticsRequest request) {
request = beforeClientExecution(request);
return executeGetSendStatistics(request);
}
@SdkInternalApi
final GetSendStatisticsResult executeGetSendStatistics(GetSendStatisticsRequest getSendStatisticsRequest) {
ExecutionContext executionContext = createExecutionContext(getSendStatisticsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSendStatisticsRequestMarshaller().marshall(super.beforeMarshalling(getSendStatisticsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetSendStatisticsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GetSendStatisticsResult getSendStatistics() {
return getSendStatistics(new GetSendStatisticsRequest());
}
/**
*
* Lists the configuration sets associated with your AWS account.
*
*
* Configuration sets enable you to publish email sending events. For information about using configuration sets,
* see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second and can return up to 50 configuration sets at a time.
*
*
* @param listConfigurationSetsRequest
* Represents a request to list the configuration sets associated with your AWS account. Configuration sets
* enable you to publish email sending events. For information about using configuration sets, see the Amazon SES
* Developer Guide.
* @return Result of the ListConfigurationSets operation returned by the service.
* @sample AmazonSimpleEmailService.ListConfigurationSets
* @see AWS
* API Documentation
*/
@Override
public ListConfigurationSetsResult listConfigurationSets(ListConfigurationSetsRequest request) {
request = beforeClientExecution(request);
return executeListConfigurationSets(request);
}
@SdkInternalApi
final ListConfigurationSetsResult executeListConfigurationSets(ListConfigurationSetsRequest listConfigurationSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listConfigurationSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListConfigurationSetsRequestMarshaller().marshall(super.beforeMarshalling(listConfigurationSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListConfigurationSetsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list containing all of the identities (email addresses and domains) for your AWS account, regardless of
* verification status.
*
*
* This action is throttled at one request per second.
*
*
* @param listIdentitiesRequest
* Represents a request to return a list of all identities (email addresses and domains) that you have
* attempted to verify under your AWS account, regardless of verification status.
* @return Result of the ListIdentities operation returned by the service.
* @sample AmazonSimpleEmailService.ListIdentities
* @see AWS API
* Documentation
*/
@Override
public ListIdentitiesResult listIdentities(ListIdentitiesRequest request) {
request = beforeClientExecution(request);
return executeListIdentities(request);
}
@SdkInternalApi
final ListIdentitiesResult executeListIdentities(ListIdentitiesRequest listIdentitiesRequest) {
ExecutionContext executionContext = createExecutionContext(listIdentitiesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListIdentitiesRequestMarshaller().marshall(super.beforeMarshalling(listIdentitiesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListIdentitiesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListIdentitiesResult listIdentities() {
return listIdentities(new ListIdentitiesRequest());
}
/**
*
* Returns a list of sending authorization policies that are attached to the given identity (an email address or a
* domain). This API returns only a list. If you want the actual policy content, you can use
* GetIdentityPolicies
.
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param listIdentityPoliciesRequest
* Represents a request to return a list of sending authorization policies that are attached to an identity.
* Sending authorization is an Amazon SES feature that enables you to authorize other senders to use your
* identities. For information, see the Amazon SES
* Developer Guide.
* @return Result of the ListIdentityPolicies operation returned by the service.
* @sample AmazonSimpleEmailService.ListIdentityPolicies
* @see AWS API
* Documentation
*/
@Override
public ListIdentityPoliciesResult listIdentityPolicies(ListIdentityPoliciesRequest request) {
request = beforeClientExecution(request);
return executeListIdentityPolicies(request);
}
@SdkInternalApi
final ListIdentityPoliciesResult executeListIdentityPolicies(ListIdentityPoliciesRequest listIdentityPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(listIdentityPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListIdentityPoliciesRequestMarshaller().marshall(super.beforeMarshalling(listIdentityPoliciesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListIdentityPoliciesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the IP address filters associated with your AWS account.
*
*
* For information about managing IP address filters, see the Amazon SES
* Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param listReceiptFiltersRequest
* Represents a request to list the IP address filters that exist under your AWS account. You use IP address
* filters when you receive email with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the ListReceiptFilters operation returned by the service.
* @sample AmazonSimpleEmailService.ListReceiptFilters
* @see AWS API
* Documentation
*/
@Override
public ListReceiptFiltersResult listReceiptFilters(ListReceiptFiltersRequest request) {
request = beforeClientExecution(request);
return executeListReceiptFilters(request);
}
@SdkInternalApi
final ListReceiptFiltersResult executeListReceiptFilters(ListReceiptFiltersRequest listReceiptFiltersRequest) {
ExecutionContext executionContext = createExecutionContext(listReceiptFiltersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListReceiptFiltersRequestMarshaller().marshall(super.beforeMarshalling(listReceiptFiltersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListReceiptFiltersResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the receipt rule sets that exist under your AWS account. If there are additional receipt rule sets to be
* retrieved, you will receive a NextToken
that you can provide to the next call to
* ListReceiptRuleSets
to retrieve the additional entries.
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param listReceiptRuleSetsRequest
* Represents a request to list the receipt rule sets that exist under your AWS account. You use receipt rule
* sets to receive email with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the ListReceiptRuleSets operation returned by the service.
* @sample AmazonSimpleEmailService.ListReceiptRuleSets
* @see AWS API
* Documentation
*/
@Override
public ListReceiptRuleSetsResult listReceiptRuleSets(ListReceiptRuleSetsRequest request) {
request = beforeClientExecution(request);
return executeListReceiptRuleSets(request);
}
@SdkInternalApi
final ListReceiptRuleSetsResult executeListReceiptRuleSets(ListReceiptRuleSetsRequest listReceiptRuleSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listReceiptRuleSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListReceiptRuleSetsRequestMarshaller().marshall(super.beforeMarshalling(listReceiptRuleSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListReceiptRuleSetsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list containing all of the email addresses that have been verified.
*
*
*
* The ListVerifiedEmailAddresses action is deprecated as of the May 15, 2012 release of Domain Verification. The
* ListIdentities action is now preferred.
*
*
*
* This action is throttled at one request per second.
*
*
* @param listVerifiedEmailAddressesRequest
* @return Result of the ListVerifiedEmailAddresses operation returned by the service.
* @sample AmazonSimpleEmailService.ListVerifiedEmailAddresses
* @see AWS API Documentation
*/
@Override
public ListVerifiedEmailAddressesResult listVerifiedEmailAddresses(ListVerifiedEmailAddressesRequest request) {
request = beforeClientExecution(request);
return executeListVerifiedEmailAddresses(request);
}
@SdkInternalApi
final ListVerifiedEmailAddressesResult executeListVerifiedEmailAddresses(ListVerifiedEmailAddressesRequest listVerifiedEmailAddressesRequest) {
ExecutionContext executionContext = createExecutionContext(listVerifiedEmailAddressesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVerifiedEmailAddressesRequestMarshaller().marshall(super.beforeMarshalling(listVerifiedEmailAddressesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListVerifiedEmailAddressesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListVerifiedEmailAddressesResult listVerifiedEmailAddresses() {
return listVerifiedEmailAddresses(new ListVerifiedEmailAddressesRequest());
}
/**
*
* Adds or updates a sending authorization policy for the specified identity (an email address or a domain).
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param putIdentityPolicyRequest
* Represents a request to add or update a sending authorization policy for an identity. Sending
* authorization is an Amazon SES feature that enables you to authorize other senders to use your identities.
* For information, see the Amazon SES
* Developer Guide.
* @return Result of the PutIdentityPolicy operation returned by the service.
* @throws InvalidPolicyException
* Indicates that the provided policy is invalid. Check the error stack for more information about what
* caused the error.
* @sample AmazonSimpleEmailService.PutIdentityPolicy
* @see AWS API
* Documentation
*/
@Override
public PutIdentityPolicyResult putIdentityPolicy(PutIdentityPolicyRequest request) {
request = beforeClientExecution(request);
return executePutIdentityPolicy(request);
}
@SdkInternalApi
final PutIdentityPolicyResult executePutIdentityPolicy(PutIdentityPolicyRequest putIdentityPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putIdentityPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutIdentityPolicyRequestMarshaller().marshall(super.beforeMarshalling(putIdentityPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new PutIdentityPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Reorders the receipt rules within a receipt rule set.
*
*
*
* All of the rules in the rule set must be represented in this request. That is, this API will return an error if
* the reorder request doesn't explicitly position all of the rules.
*
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param reorderReceiptRuleSetRequest
* Represents a request to reorder the receipt rules within a receipt rule set. You use receipt rule sets to
* receive email with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the ReorderReceiptRuleSet operation returned by the service.
* @throws RuleSetDoesNotExistException
* Indicates that the provided receipt rule set does not exist.
* @throws RuleDoesNotExistException
* Indicates that the provided receipt rule does not exist.
* @sample AmazonSimpleEmailService.ReorderReceiptRuleSet
* @see AWS
* API Documentation
*/
@Override
public ReorderReceiptRuleSetResult reorderReceiptRuleSet(ReorderReceiptRuleSetRequest request) {
request = beforeClientExecution(request);
return executeReorderReceiptRuleSet(request);
}
@SdkInternalApi
final ReorderReceiptRuleSetResult executeReorderReceiptRuleSet(ReorderReceiptRuleSetRequest reorderReceiptRuleSetRequest) {
ExecutionContext executionContext = createExecutionContext(reorderReceiptRuleSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ReorderReceiptRuleSetRequestMarshaller().marshall(super.beforeMarshalling(reorderReceiptRuleSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ReorderReceiptRuleSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Generates and sends a bounce message to the sender of an email you received through Amazon SES. You can only use
* this API on an email up to 24 hours after you receive it.
*
*
*
* You cannot use this API to send generic bounces for mail that was not received by Amazon SES.
*
*
*
* For information about receiving email through Amazon SES, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param sendBounceRequest
* Represents a request to send a bounce message to the sender of an email you received through Amazon SES.
* @return Result of the SendBounce operation returned by the service.
* @throws MessageRejectedException
* Indicates that the action failed, and the message could not be sent. Check the error stack for more
* information about what caused the error.
* @sample AmazonSimpleEmailService.SendBounce
* @see AWS API
* Documentation
*/
@Override
public SendBounceResult sendBounce(SendBounceRequest request) {
request = beforeClientExecution(request);
return executeSendBounce(request);
}
@SdkInternalApi
final SendBounceResult executeSendBounce(SendBounceRequest sendBounceRequest) {
ExecutionContext executionContext = createExecutionContext(sendBounceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SendBounceRequestMarshaller().marshall(super.beforeMarshalling(sendBounceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new SendBounceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Composes an email message based on input data, and then immediately queues the message for sending.
*
*
* There are several important points to know about SendEmail
:
*
*
* -
*
* You can only send email from verified email addresses and domains; otherwise, you will get an
* "Email address not verified" error. If your account is still in the Amazon SES sandbox, you must also verify
* every recipient email address except for the recipients provided by the Amazon SES mailbox simulator. For more
* information, go to the Amazon SES
* Developer Guide.
*
*
* -
*
* The total size of the message cannot exceed 10 MB. This includes any attachments that are part of the message.
*
*
* -
*
* You must provide at least one recipient email address. The recipient address can be a To: address, a CC: address,
* or a BCC: address. If any email address you provide is invalid, Amazon SES rejects the entire email.
*
*
* -
*
* Amazon SES has a limit on the total number of recipients per message. The combined number of To:, CC: and BCC:
* email addresses cannot exceed 50. If you need to send an email message to a larger audience, you can divide your
* recipient list into groups of 50 or fewer, and then call Amazon SES repeatedly to send the message to each group.
*
*
* -
*
* For every message that you send, the total number of recipients (To:, CC: and BCC:) is counted against your
* sending quota - the maximum number of emails you can send in a 24-hour period. For information about your sending
* quota, go to the Amazon
* SES Developer Guide.
*
*
*
*
* @param sendEmailRequest
* Represents a request to send a single formatted email using Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return Result of the SendEmail operation returned by the service.
* @throws MessageRejectedException
* Indicates that the action failed, and the message could not be sent. Check the error stack for more
* information about what caused the error.
* @throws MailFromDomainNotVerifiedException
* Indicates that the message could not be sent because Amazon SES could not read the MX record required to
* use the specified MAIL FROM domain. For information about editing the custom MAIL FROM domain settings
* for an identity, see the Amazon SES Developer
* Guide.
* @throws ConfigurationSetDoesNotExistException
* Indicates that the configuration set does not exist.
* @sample AmazonSimpleEmailService.SendEmail
* @see AWS API
* Documentation
*/
@Override
public SendEmailResult sendEmail(SendEmailRequest request) {
request = beforeClientExecution(request);
return executeSendEmail(request);
}
@SdkInternalApi
final SendEmailResult executeSendEmail(SendEmailRequest sendEmailRequest) {
ExecutionContext executionContext = createExecutionContext(sendEmailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SendEmailRequestMarshaller().marshall(super.beforeMarshalling(sendEmailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new SendEmailResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sends an email message, with header and content specified by the client. The SendRawEmail
action is
* useful for sending multipart MIME emails. The raw text of the message must comply with Internet email standards;
* otherwise, the message cannot be sent.
*
*
* There are several important points to know about SendRawEmail
:
*
*
* -
*
* You can only send email from verified email addresses and domains; otherwise, you will get an
* "Email address not verified" error. If your account is still in the Amazon SES sandbox, you must also verify
* every recipient email address except for the recipients provided by the Amazon SES mailbox simulator. For more
* information, go to the Amazon SES
* Developer Guide.
*
*
* -
*
* The total size of the message cannot exceed 10 MB. This includes any attachments that are part of the message.
*
*
* -
*
* You must provide at least one recipient email address. The recipient address can be a To: address, a CC: address,
* or a BCC: address. If any email address you provide is invalid, Amazon SES rejects the entire email.
*
*
* -
*
* Amazon SES has a limit on the total number of recipients per message. The combined number of To:, CC: and BCC:
* email addresses cannot exceed 50. If you need to send an email message to a larger audience, you can divide your
* recipient list into groups of 50 or fewer, and then call Amazon SES repeatedly to send the message to each group.
*
*
* -
*
* The To:, CC:, and BCC: headers in the raw message can contain a group list. Note that each recipient in a group
* list counts towards the 50-recipient limit.
*
*
* -
*
* Amazon SES overrides any Message-ID and Date headers you provide.
*
*
* -
*
* For every message that you send, the total number of recipients (To:, CC: and BCC:) is counted against your
* sending quota - the maximum number of emails you can send in a 24-hour period. For information about your sending
* quota, go to the Amazon
* SES Developer Guide.
*
*
* -
*
* If you are using sending authorization to send on behalf of another user, SendRawEmail
enables you
* to specify the cross-account identity for the email's "Source," "From," and "Return-Path" parameters in one of
* two ways: you can pass optional parameters SourceArn
, FromArn
, and/or
* ReturnPathArn
to the API, or you can include the following X-headers in the header of your raw
* email:
*
*
* -
*
* X-SES-SOURCE-ARN
*
*
* -
*
* X-SES-FROM-ARN
*
*
* -
*
* X-SES-RETURN-PATH-ARN
*
*
*
*
*
* Do not include these X-headers in the DKIM signature, because they are removed by Amazon SES before sending the
* email.
*
*
*
* For the most common sending authorization use case, we recommend that you specify the
* SourceIdentityArn
and do not specify either the FromIdentityArn
or
* ReturnPathIdentityArn
. (The same note applies to the corresponding X-headers.) If you only specify
* the SourceIdentityArn
, Amazon SES will simply set the "From" address and the "Return Path" address
* to the identity specified in SourceIdentityArn
. For more information about sending authorization,
* see the Amazon SES
* Developer Guide.
*
*
*
*
* @param sendRawEmailRequest
* Represents a request to send a single raw email using Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return Result of the SendRawEmail operation returned by the service.
* @throws MessageRejectedException
* Indicates that the action failed, and the message could not be sent. Check the error stack for more
* information about what caused the error.
* @throws MailFromDomainNotVerifiedException
* Indicates that the message could not be sent because Amazon SES could not read the MX record required to
* use the specified MAIL FROM domain. For information about editing the custom MAIL FROM domain settings
* for an identity, see the Amazon SES Developer
* Guide.
* @throws ConfigurationSetDoesNotExistException
* Indicates that the configuration set does not exist.
* @sample AmazonSimpleEmailService.SendRawEmail
* @see AWS API
* Documentation
*/
@Override
public SendRawEmailResult sendRawEmail(SendRawEmailRequest request) {
request = beforeClientExecution(request);
return executeSendRawEmail(request);
}
@SdkInternalApi
final SendRawEmailResult executeSendRawEmail(SendRawEmailRequest sendRawEmailRequest) {
ExecutionContext executionContext = createExecutionContext(sendRawEmailRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SendRawEmailRequestMarshaller().marshall(super.beforeMarshalling(sendRawEmailRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new SendRawEmailResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sets the specified receipt rule set as the active receipt rule set.
*
*
*
* To disable your email-receiving through Amazon SES completely, you can call this API with RuleSetName set to
* null.
*
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param setActiveReceiptRuleSetRequest
* Represents a request to set a receipt rule set as the active receipt rule set. You use receipt rule sets
* to receive email with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the SetActiveReceiptRuleSet operation returned by the service.
* @throws RuleSetDoesNotExistException
* Indicates that the provided receipt rule set does not exist.
* @sample AmazonSimpleEmailService.SetActiveReceiptRuleSet
* @see AWS
* API Documentation
*/
@Override
public SetActiveReceiptRuleSetResult setActiveReceiptRuleSet(SetActiveReceiptRuleSetRequest request) {
request = beforeClientExecution(request);
return executeSetActiveReceiptRuleSet(request);
}
@SdkInternalApi
final SetActiveReceiptRuleSetResult executeSetActiveReceiptRuleSet(SetActiveReceiptRuleSetRequest setActiveReceiptRuleSetRequest) {
ExecutionContext executionContext = createExecutionContext(setActiveReceiptRuleSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetActiveReceiptRuleSetRequestMarshaller().marshall(super.beforeMarshalling(setActiveReceiptRuleSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetActiveReceiptRuleSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables or disables Easy DKIM signing of email sent from an identity:
*
*
* -
*
* If Easy DKIM signing is enabled for a domain name identity (e.g., example.com
), then Amazon SES will
* DKIM-sign all email sent by addresses under that domain name (e.g., [email protected]
).
*
*
* -
*
* If Easy DKIM signing is enabled for an email address, then Amazon SES will DKIM-sign all email sent by that email
* address.
*
*
*
*
* For email addresses (e.g., [email protected]
), you can only enable Easy DKIM signing if the
* corresponding domain (e.g., example.com
) has been set up for Easy DKIM using the AWS Console or the
* VerifyDomainDkim
action.
*
*
* This action is throttled at one request per second.
*
*
* For more information about Easy DKIM signing, go to the Amazon SES Developer Guide.
*
*
* @param setIdentityDkimEnabledRequest
* Represents a request to enable or disable Amazon SES Easy DKIM signing for an identity. For more
* information about setting up Easy DKIM, see the Amazon SES Developer Guide.
* @return Result of the SetIdentityDkimEnabled operation returned by the service.
* @sample AmazonSimpleEmailService.SetIdentityDkimEnabled
* @see AWS
* API Documentation
*/
@Override
public SetIdentityDkimEnabledResult setIdentityDkimEnabled(SetIdentityDkimEnabledRequest request) {
request = beforeClientExecution(request);
return executeSetIdentityDkimEnabled(request);
}
@SdkInternalApi
final SetIdentityDkimEnabledResult executeSetIdentityDkimEnabled(SetIdentityDkimEnabledRequest setIdentityDkimEnabledRequest) {
ExecutionContext executionContext = createExecutionContext(setIdentityDkimEnabledRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetIdentityDkimEnabledRequestMarshaller().marshall(super.beforeMarshalling(setIdentityDkimEnabledRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetIdentityDkimEnabledResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Given an identity (an email address or a domain), enables or disables whether Amazon SES forwards bounce and
* complaint notifications as email. Feedback forwarding can only be disabled when Amazon Simple Notification
* Service (Amazon SNS) topics are specified for both bounces and complaints.
*
*
*
* Feedback forwarding does not apply to delivery notifications. Delivery notifications are only available through
* Amazon SNS.
*
*
*
* This action is throttled at one request per second.
*
*
* For more information about using notifications with Amazon SES, see the Amazon SES Developer Guide.
*
*
* @param setIdentityFeedbackForwardingEnabledRequest
* Represents a request to enable or disable whether Amazon SES forwards you bounce and complaint
* notifications through email. For information about email feedback forwarding, see the Amazon SES
* Developer Guide.
* @return Result of the SetIdentityFeedbackForwardingEnabled operation returned by the service.
* @sample AmazonSimpleEmailService.SetIdentityFeedbackForwardingEnabled
* @see AWS API Documentation
*/
@Override
public SetIdentityFeedbackForwardingEnabledResult setIdentityFeedbackForwardingEnabled(SetIdentityFeedbackForwardingEnabledRequest request) {
request = beforeClientExecution(request);
return executeSetIdentityFeedbackForwardingEnabled(request);
}
@SdkInternalApi
final SetIdentityFeedbackForwardingEnabledResult executeSetIdentityFeedbackForwardingEnabled(
SetIdentityFeedbackForwardingEnabledRequest setIdentityFeedbackForwardingEnabledRequest) {
ExecutionContext executionContext = createExecutionContext(setIdentityFeedbackForwardingEnabledRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetIdentityFeedbackForwardingEnabledRequestMarshaller().marshall(super
.beforeMarshalling(setIdentityFeedbackForwardingEnabledRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetIdentityFeedbackForwardingEnabledResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Given an identity (an email address or a domain), sets whether Amazon SES includes the original email headers in
* the Amazon Simple Notification Service (Amazon SNS) notifications of a specified type.
*
*
* This action is throttled at one request per second.
*
*
* For more information about using notifications with Amazon SES, see the Amazon SES Developer Guide.
*
*
* @param setIdentityHeadersInNotificationsEnabledRequest
* Represents a request to set whether Amazon SES includes the original email headers in the Amazon SNS
* notifications of a specified type. For information about notifications, see the Amazon SES
* Developer Guide.
* @return Result of the SetIdentityHeadersInNotificationsEnabled operation returned by the service.
* @sample AmazonSimpleEmailService.SetIdentityHeadersInNotificationsEnabled
* @see AWS API Documentation
*/
@Override
public SetIdentityHeadersInNotificationsEnabledResult setIdentityHeadersInNotificationsEnabled(SetIdentityHeadersInNotificationsEnabledRequest request) {
request = beforeClientExecution(request);
return executeSetIdentityHeadersInNotificationsEnabled(request);
}
@SdkInternalApi
final SetIdentityHeadersInNotificationsEnabledResult executeSetIdentityHeadersInNotificationsEnabled(
SetIdentityHeadersInNotificationsEnabledRequest setIdentityHeadersInNotificationsEnabledRequest) {
ExecutionContext executionContext = createExecutionContext(setIdentityHeadersInNotificationsEnabledRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetIdentityHeadersInNotificationsEnabledRequestMarshaller().marshall(super
.beforeMarshalling(setIdentityHeadersInNotificationsEnabledRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetIdentityHeadersInNotificationsEnabledResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables or disables the custom MAIL FROM domain setup for a verified identity (an email address or a domain).
*
*
*
* To send emails using the specified MAIL FROM domain, you must add an MX record to your MAIL FROM domain's DNS
* settings. If you want your emails to pass Sender Policy Framework (SPF) checks, you must also add or update an
* SPF record. For more information, see the Amazon SES Developer Guide.
*
*
*
* This action is throttled at one request per second.
*
*
* @param setIdentityMailFromDomainRequest
* Represents a request to enable or disable the Amazon SES custom MAIL FROM domain setup for a verified
* identity. For information about using a custom MAIL FROM domain, see the Amazon SES Developer Guide.
* @return Result of the SetIdentityMailFromDomain operation returned by the service.
* @sample AmazonSimpleEmailService.SetIdentityMailFromDomain
* @see AWS API Documentation
*/
@Override
public SetIdentityMailFromDomainResult setIdentityMailFromDomain(SetIdentityMailFromDomainRequest request) {
request = beforeClientExecution(request);
return executeSetIdentityMailFromDomain(request);
}
@SdkInternalApi
final SetIdentityMailFromDomainResult executeSetIdentityMailFromDomain(SetIdentityMailFromDomainRequest setIdentityMailFromDomainRequest) {
ExecutionContext executionContext = createExecutionContext(setIdentityMailFromDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetIdentityMailFromDomainRequestMarshaller().marshall(super.beforeMarshalling(setIdentityMailFromDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetIdentityMailFromDomainResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Given an identity (an email address or a domain), sets the Amazon Simple Notification Service (Amazon SNS) topic
* to which Amazon SES will publish bounce, complaint, and/or delivery notifications for emails sent with that
* identity as the Source
.
*
*
*
* Unless feedback forwarding is enabled, you must specify Amazon SNS topics for bounce and complaint notifications.
* For more information, see SetIdentityFeedbackForwardingEnabled
.
*
*
*
* This action is throttled at one request per second.
*
*
* For more information about feedback notification, see the Amazon SES Developer Guide.
*
*
* @param setIdentityNotificationTopicRequest
* Represents a request to specify the Amazon SNS topic to which Amazon SES will publish bounce, complaint,
* or delivery notifications for emails sent with that identity as the Source. For information about Amazon
* SES notifications, see the Amazon SES
* Developer Guide.
* @return Result of the SetIdentityNotificationTopic operation returned by the service.
* @sample AmazonSimpleEmailService.SetIdentityNotificationTopic
* @see AWS API Documentation
*/
@Override
public SetIdentityNotificationTopicResult setIdentityNotificationTopic(SetIdentityNotificationTopicRequest request) {
request = beforeClientExecution(request);
return executeSetIdentityNotificationTopic(request);
}
@SdkInternalApi
final SetIdentityNotificationTopicResult executeSetIdentityNotificationTopic(SetIdentityNotificationTopicRequest setIdentityNotificationTopicRequest) {
ExecutionContext executionContext = createExecutionContext(setIdentityNotificationTopicRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetIdentityNotificationTopicRequestMarshaller().marshall(super.beforeMarshalling(setIdentityNotificationTopicRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetIdentityNotificationTopicResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sets the position of the specified receipt rule in the receipt rule set.
*
*
* For information about managing receipt rules, see the Amazon
* SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param setReceiptRulePositionRequest
* Represents a request to set the position of a receipt rule in a receipt rule set. You use receipt rule
* sets to receive email with Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return Result of the SetReceiptRulePosition operation returned by the service.
* @throws RuleSetDoesNotExistException
* Indicates that the provided receipt rule set does not exist.
* @throws RuleDoesNotExistException
* Indicates that the provided receipt rule does not exist.
* @sample AmazonSimpleEmailService.SetReceiptRulePosition
* @see AWS
* API Documentation
*/
@Override
public SetReceiptRulePositionResult setReceiptRulePosition(SetReceiptRulePositionRequest request) {
request = beforeClientExecution(request);
return executeSetReceiptRulePosition(request);
}
@SdkInternalApi
final SetReceiptRulePositionResult executeSetReceiptRulePosition(SetReceiptRulePositionRequest setReceiptRulePositionRequest) {
ExecutionContext executionContext = createExecutionContext(setReceiptRulePositionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetReceiptRulePositionRequestMarshaller().marshall(super.beforeMarshalling(setReceiptRulePositionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetReceiptRulePositionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the event destination of a configuration set.
*
*
*
* When you create or update an event destination, you must provide one, and only one, destination. The destination
* can be Amazon CloudWatch, Amazon Kinesis Firehose, or Amazon Simple Notification Service (Amazon SNS).
*
*
*
* Event destinations are associated with configuration sets, which enable you to publish email sending events to
* Amazon CloudWatch, Amazon Kinesis Firehose, or Amazon Simple Notification Service (Amazon SNS). For information
* about using configuration sets, see the Amazon SES Developer
* Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param updateConfigurationSetEventDestinationRequest
* Represents a request to update the event destination of a configuration set. Configuration sets enable you
* to publish email sending events. For information about using configuration sets, see the Amazon SES
* Developer Guide.
* @return Result of the UpdateConfigurationSetEventDestination operation returned by the service.
* @throws ConfigurationSetDoesNotExistException
* Indicates that the configuration set does not exist.
* @throws EventDestinationDoesNotExistException
* Indicates that the event destination does not exist.
* @throws InvalidCloudWatchDestinationException
* Indicates that the Amazon CloudWatch destination is invalid. See the error message for details.
* @throws InvalidFirehoseDestinationException
* Indicates that the Amazon Kinesis Firehose destination is invalid. See the error message for details.
* @throws InvalidSNSDestinationException
* Indicates that the Amazon Simple Notification Service (Amazon SNS) destination is invalid. See the error
* message for details.
* @sample AmazonSimpleEmailService.UpdateConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public UpdateConfigurationSetEventDestinationResult updateConfigurationSetEventDestination(UpdateConfigurationSetEventDestinationRequest request) {
request = beforeClientExecution(request);
return executeUpdateConfigurationSetEventDestination(request);
}
@SdkInternalApi
final UpdateConfigurationSetEventDestinationResult executeUpdateConfigurationSetEventDestination(
UpdateConfigurationSetEventDestinationRequest updateConfigurationSetEventDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(updateConfigurationSetEventDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateConfigurationSetEventDestinationRequestMarshaller().marshall(super
.beforeMarshalling(updateConfigurationSetEventDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateConfigurationSetEventDestinationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a receipt rule.
*
*
* For information about managing receipt rules, see the Amazon
* SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param updateReceiptRuleRequest
* Represents a request to update a receipt rule. You use receipt rules to receive email with Amazon SES. For
* more information, see the Amazon SES
* Developer Guide.
* @return Result of the UpdateReceiptRule operation returned by the service.
* @throws InvalidSnsTopicException
* Indicates that the provided Amazon SNS topic is invalid, or that Amazon SES could not publish to the
* topic, possibly due to permissions issues. For information about giving permissions, see the Amazon SES
* Developer Guide.
* @throws InvalidS3ConfigurationException
* Indicates that the provided Amazon S3 bucket or AWS KMS encryption key is invalid, or that Amazon SES
* could not publish to the bucket, possibly due to permissions issues. For information about giving
* permissions, see the Amazon SES
* Developer Guide.
* @throws InvalidLambdaFunctionException
* Indicates that the provided AWS Lambda function is invalid, or that Amazon SES could not execute the
* provided function, possibly due to permissions issues. For information about giving permissions, see the
* Amazon
* SES Developer Guide.
* @throws RuleSetDoesNotExistException
* Indicates that the provided receipt rule set does not exist.
* @throws RuleDoesNotExistException
* Indicates that the provided receipt rule does not exist.
* @throws LimitExceededException
* Indicates that a resource could not be created because of service limits. For a list of Amazon SES
* limits, see the Amazon SES
* Developer Guide.
* @sample AmazonSimpleEmailService.UpdateReceiptRule
* @see AWS API
* Documentation
*/
@Override
public UpdateReceiptRuleResult updateReceiptRule(UpdateReceiptRuleRequest request) {
request = beforeClientExecution(request);
return executeUpdateReceiptRule(request);
}
@SdkInternalApi
final UpdateReceiptRuleResult executeUpdateReceiptRule(UpdateReceiptRuleRequest updateReceiptRuleRequest) {
ExecutionContext executionContext = createExecutionContext(updateReceiptRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateReceiptRuleRequestMarshaller().marshall(super.beforeMarshalling(updateReceiptRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateReceiptRuleResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a set of DKIM tokens for a domain. DKIM tokens are character strings that represent your domain's
* identity. Using these tokens, you will need to create DNS CNAME records that point to DKIM public keys hosted by
* Amazon SES. Amazon Web Services will eventually detect that you have updated your DNS records; this detection
* process may take up to 72 hours. Upon successful detection, Amazon SES will be able to DKIM-sign email
* originating from that domain.
*
*
* This action is throttled at one request per second.
*
*
* To enable or disable Easy DKIM signing for a domain, use the SetIdentityDkimEnabled
action.
*
*
* For more information about creating DNS records using DKIM tokens, go to the Amazon SES Developer
* Guide.
*
*
* @param verifyDomainDkimRequest
* Represents a request to generate the CNAME records needed to set up Easy DKIM with Amazon SES. For more
* information about setting up Easy DKIM, see the Amazon SES Developer Guide.
* @return Result of the VerifyDomainDkim operation returned by the service.
* @sample AmazonSimpleEmailService.VerifyDomainDkim
* @see AWS API
* Documentation
*/
@Override
public VerifyDomainDkimResult verifyDomainDkim(VerifyDomainDkimRequest request) {
request = beforeClientExecution(request);
return executeVerifyDomainDkim(request);
}
@SdkInternalApi
final VerifyDomainDkimResult executeVerifyDomainDkim(VerifyDomainDkimRequest verifyDomainDkimRequest) {
ExecutionContext executionContext = createExecutionContext(verifyDomainDkimRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new VerifyDomainDkimRequestMarshaller().marshall(super.beforeMarshalling(verifyDomainDkimRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new VerifyDomainDkimResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Verifies a domain.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyDomainIdentityRequest
* Represents a request to begin Amazon SES domain verification and to generate the TXT records that you must
* publish to the DNS server of your domain to complete the verification. For information about domain
* verification, see the Amazon SES Developer
* Guide.
* @return Result of the VerifyDomainIdentity operation returned by the service.
* @sample AmazonSimpleEmailService.VerifyDomainIdentity
* @see AWS API
* Documentation
*/
@Override
public VerifyDomainIdentityResult verifyDomainIdentity(VerifyDomainIdentityRequest request) {
request = beforeClientExecution(request);
return executeVerifyDomainIdentity(request);
}
@SdkInternalApi
final VerifyDomainIdentityResult executeVerifyDomainIdentity(VerifyDomainIdentityRequest verifyDomainIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(verifyDomainIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new VerifyDomainIdentityRequestMarshaller().marshall(super.beforeMarshalling(verifyDomainIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new VerifyDomainIdentityResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Verifies an email address. This action causes a confirmation email message to be sent to the specified address.
*
*
*
* The VerifyEmailAddress action is deprecated as of the May 15, 2012 release of Domain Verification. The
* VerifyEmailIdentity action is now preferred.
*
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailAddressRequest
* Represents a request to begin email address verification with Amazon SES. For information about email
* address verification, see the Amazon SES
* Developer Guide.
* @return Result of the VerifyEmailAddress operation returned by the service.
* @sample AmazonSimpleEmailService.VerifyEmailAddress
* @see AWS API
* Documentation
*/
@Override
public VerifyEmailAddressResult verifyEmailAddress(VerifyEmailAddressRequest request) {
request = beforeClientExecution(request);
return executeVerifyEmailAddress(request);
}
@SdkInternalApi
final VerifyEmailAddressResult executeVerifyEmailAddress(VerifyEmailAddressRequest verifyEmailAddressRequest) {
ExecutionContext executionContext = createExecutionContext(verifyEmailAddressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new VerifyEmailAddressRequestMarshaller().marshall(super.beforeMarshalling(verifyEmailAddressRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new VerifyEmailAddressResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Verifies an email address. This action causes a confirmation email message to be sent to the specified address.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailIdentityRequest
* Represents a request to begin email address verification with Amazon SES. For information about email
* address verification, see the Amazon SES
* Developer Guide.
* @return Result of the VerifyEmailIdentity operation returned by the service.
* @sample AmazonSimpleEmailService.VerifyEmailIdentity
* @see AWS API
* Documentation
*/
@Override
public VerifyEmailIdentityResult verifyEmailIdentity(VerifyEmailIdentityRequest request) {
request = beforeClientExecution(request);
return executeVerifyEmailIdentity(request);
}
@SdkInternalApi
final VerifyEmailIdentityResult executeVerifyEmailIdentity(VerifyEmailIdentityRequest verifyEmailIdentityRequest) {
ExecutionContext executionContext = createExecutionContext(verifyEmailIdentityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new VerifyEmailIdentityRequestMarshaller().marshall(super.beforeMarshalling(verifyEmailIdentityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new VerifyEmailIdentityResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@Override
public AmazonSimpleEmailServiceWaiters waiters() {
if (waiters == null) {
synchronized (this) {
if (waiters == null) {
waiters = new AmazonSimpleEmailServiceWaiters(this);
}
}
}
return waiters;
}
@Override
public void shutdown() {
super.shutdown();
if (waiters != null) {
waiters.shutdown();
}
}
}