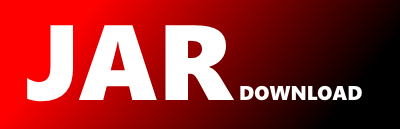
com.amazonaws.services.simpleemail.AmazonSimpleEmailServiceAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ses Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.simpleemail;
import com.amazonaws.services.simpleemail.model.*;
/**
* Interface for accessing Amazon SES asynchronously. Each asynchronous method
* will return a Java Future object representing the asynchronous operation;
* overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Amazon Simple Email Service
*
* This is the API Reference for Amazon Simple Email Service (Amazon SES). This
* documentation is intended to be used in conjunction with the Amazon SES Developer Guide.
*
*
*
* For a list of Amazon SES endpoints to use in service requests, see Regions and Amazon SES in the Amazon SES Developer Guide.
*
*
*/
public interface AmazonSimpleEmailServiceAsync extends AmazonSimpleEmailService {
/**
*
* Creates a receipt rule set by cloning an existing one. All receipt rules
* and configurations are copied to the new receipt rule set and are
* completely independent of the source rule set.
*
*
* For information about setting up rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param cloneReceiptRuleSetRequest
* Represents a request to create a receipt rule set by cloning an
* existing one. You use receipt rule sets to receive email with
* Amazon SES. For more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the CloneReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.CloneReceiptRuleSet
*/
java.util.concurrent.Future cloneReceiptRuleSetAsync(
CloneReceiptRuleSetRequest cloneReceiptRuleSetRequest);
/**
*
* Creates a receipt rule set by cloning an existing one. All receipt rules
* and configurations are copied to the new receipt rule set and are
* completely independent of the source rule set.
*
*
* For information about setting up rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param cloneReceiptRuleSetRequest
* Represents a request to create a receipt rule set by cloning an
* existing one. You use receipt rule sets to receive email with
* Amazon SES. For more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CloneReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.CloneReceiptRuleSet
*/
java.util.concurrent.Future cloneReceiptRuleSetAsync(
CloneReceiptRuleSetRequest cloneReceiptRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new IP address filter.
*
*
* For information about setting up IP address filters, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createReceiptFilterRequest
* Represents a request to create a new IP address filter. You use IP
* address filters when you receive email with Amazon SES. For more
* information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the CreateReceiptFilter
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.CreateReceiptFilter
*/
java.util.concurrent.Future createReceiptFilterAsync(
CreateReceiptFilterRequest createReceiptFilterRequest);
/**
*
* Creates a new IP address filter.
*
*
* For information about setting up IP address filters, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createReceiptFilterRequest
* Represents a request to create a new IP address filter. You use IP
* address filters when you receive email with Amazon SES. For more
* information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReceiptFilter
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.CreateReceiptFilter
*/
java.util.concurrent.Future createReceiptFilterAsync(
CreateReceiptFilterRequest createReceiptFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a receipt rule.
*
*
* For information about setting up receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createReceiptRuleRequest
* Represents a request to create a receipt rule. You use receipt
* rules to receive email with Amazon SES. For more information, see
* the Amazon SES Developer Guide.
* @return A Java Future containing the result of the CreateReceiptRule
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.CreateReceiptRule
*/
java.util.concurrent.Future createReceiptRuleAsync(
CreateReceiptRuleRequest createReceiptRuleRequest);
/**
*
* Creates a receipt rule.
*
*
* For information about setting up receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createReceiptRuleRequest
* Represents a request to create a receipt rule. You use receipt
* rules to receive email with Amazon SES. For more information, see
* the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReceiptRule
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.CreateReceiptRule
*/
java.util.concurrent.Future createReceiptRuleAsync(
CreateReceiptRuleRequest createReceiptRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an empty receipt rule set.
*
*
* For information about setting up receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createReceiptRuleSetRequest
* Represents a request to create an empty receipt rule set. You use
* receipt rule sets to receive email with Amazon SES. For more
* information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the CreateReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.CreateReceiptRuleSet
*/
java.util.concurrent.Future createReceiptRuleSetAsync(
CreateReceiptRuleSetRequest createReceiptRuleSetRequest);
/**
*
* Creates an empty receipt rule set.
*
*
* For information about setting up receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param createReceiptRuleSetRequest
* Represents a request to create an empty receipt rule set. You use
* receipt rule sets to receive email with Amazon SES. For more
* information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.CreateReceiptRuleSet
*/
java.util.concurrent.Future createReceiptRuleSetAsync(
CreateReceiptRuleSetRequest createReceiptRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified identity (an email address or a domain) from the
* list of verified identities.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteIdentityRequest
* Represents a request to delete one of your Amazon SES identities
* (an email address or domain).
* @return A Java Future containing the result of the DeleteIdentity
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.DeleteIdentity
*/
java.util.concurrent.Future deleteIdentityAsync(
DeleteIdentityRequest deleteIdentityRequest);
/**
*
* Deletes the specified identity (an email address or a domain) from the
* list of verified identities.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteIdentityRequest
* Represents a request to delete one of your Amazon SES identities
* (an email address or domain).
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIdentity
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.DeleteIdentity
*/
java.util.concurrent.Future deleteIdentityAsync(
DeleteIdentityRequest deleteIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified sending authorization policy for the given identity
* (an email address or a domain). This API returns successfully even if a
* policy with the specified name does not exist.
*
*
*
* This API is for the identity owner only. If you have not verified the
* identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to
* authorize other senders to use its identities. For information about
* using sending authorization, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteIdentityPolicyRequest
* Represents a request to delete a sending authorization policy for
* an identity. Sending authorization is an Amazon SES feature that
* enables you to authorize other senders to use your identities. For
* information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the DeleteIdentityPolicy
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.DeleteIdentityPolicy
*/
java.util.concurrent.Future deleteIdentityPolicyAsync(
DeleteIdentityPolicyRequest deleteIdentityPolicyRequest);
/**
*
* Deletes the specified sending authorization policy for the given identity
* (an email address or a domain). This API returns successfully even if a
* policy with the specified name does not exist.
*
*
*
* This API is for the identity owner only. If you have not verified the
* identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to
* authorize other senders to use its identities. For information about
* using sending authorization, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteIdentityPolicyRequest
* Represents a request to delete a sending authorization policy for
* an identity. Sending authorization is an Amazon SES feature that
* enables you to authorize other senders to use your identities. For
* information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIdentityPolicy
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.DeleteIdentityPolicy
*/
java.util.concurrent.Future deleteIdentityPolicyAsync(
DeleteIdentityPolicyRequest deleteIdentityPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified IP address filter.
*
*
* For information about managing IP address filters, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteReceiptFilterRequest
* Represents a request to delete an IP address filter. You use IP
* address filters when you receive email with Amazon SES. For more
* information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the DeleteReceiptFilter
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.DeleteReceiptFilter
*/
java.util.concurrent.Future deleteReceiptFilterAsync(
DeleteReceiptFilterRequest deleteReceiptFilterRequest);
/**
*
* Deletes the specified IP address filter.
*
*
* For information about managing IP address filters, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteReceiptFilterRequest
* Represents a request to delete an IP address filter. You use IP
* address filters when you receive email with Amazon SES. For more
* information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReceiptFilter
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.DeleteReceiptFilter
*/
java.util.concurrent.Future deleteReceiptFilterAsync(
DeleteReceiptFilterRequest deleteReceiptFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified receipt rule.
*
*
* For information about managing receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteReceiptRuleRequest
* Represents a request to delete a receipt rule. You use receipt
* rules to receive email with Amazon SES. For more information, see
* the Amazon SES Developer Guide.
* @return A Java Future containing the result of the DeleteReceiptRule
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.DeleteReceiptRule
*/
java.util.concurrent.Future deleteReceiptRuleAsync(
DeleteReceiptRuleRequest deleteReceiptRuleRequest);
/**
*
* Deletes the specified receipt rule.
*
*
* For information about managing receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteReceiptRuleRequest
* Represents a request to delete a receipt rule. You use receipt
* rules to receive email with Amazon SES. For more information, see
* the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReceiptRule
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.DeleteReceiptRule
*/
java.util.concurrent.Future deleteReceiptRuleAsync(
DeleteReceiptRuleRequest deleteReceiptRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified receipt rule set and all of the receipt rules it
* contains.
*
*
*
* The currently active rule set cannot be deleted.
*
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteReceiptRuleSetRequest
* Represents a request to delete a receipt rule set and all of the
* receipt rules it contains. You use receipt rule sets to receive
* email with Amazon SES. For more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the DeleteReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.DeleteReceiptRuleSet
*/
java.util.concurrent.Future deleteReceiptRuleSetAsync(
DeleteReceiptRuleSetRequest deleteReceiptRuleSetRequest);
/**
*
* Deletes the specified receipt rule set and all of the receipt rules it
* contains.
*
*
*
* The currently active rule set cannot be deleted.
*
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteReceiptRuleSetRequest
* Represents a request to delete a receipt rule set and all of the
* receipt rules it contains. You use receipt rule sets to receive
* email with Amazon SES. For more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.DeleteReceiptRuleSet
*/
java.util.concurrent.Future deleteReceiptRuleSetAsync(
DeleteReceiptRuleSetRequest deleteReceiptRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified email address from the list of verified addresses.
*
*
*
* The DeleteVerifiedEmailAddress action is deprecated as of the May 15,
* 2012 release of Domain Verification. The DeleteIdentity action is now
* preferred.
*
*
*
* This action is throttled at one request per second.
*
*
* @param deleteVerifiedEmailAddressRequest
* Represents a request to delete an email address from the list of
* email addresses you have attempted to verify under your AWS
* account.
* @return A Java Future containing the result of the
* DeleteVerifiedEmailAddress operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.DeleteVerifiedEmailAddress
*/
java.util.concurrent.Future deleteVerifiedEmailAddressAsync(
DeleteVerifiedEmailAddressRequest deleteVerifiedEmailAddressRequest);
/**
*
* Deletes the specified email address from the list of verified addresses.
*
*
*
* The DeleteVerifiedEmailAddress action is deprecated as of the May 15,
* 2012 release of Domain Verification. The DeleteIdentity action is now
* preferred.
*
*
*
* This action is throttled at one request per second.
*
*
* @param deleteVerifiedEmailAddressRequest
* Represents a request to delete an email address from the list of
* email addresses you have attempted to verify under your AWS
* account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteVerifiedEmailAddress operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.DeleteVerifiedEmailAddress
*/
java.util.concurrent.Future deleteVerifiedEmailAddressAsync(
DeleteVerifiedEmailAddressRequest deleteVerifiedEmailAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the metadata and receipt rules for the receipt rule set that is
* currently active.
*
*
* For information about setting up receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeActiveReceiptRuleSetRequest
* Represents a request to return the metadata and receipt rules for
* the receipt rule set that is currently active. You use receipt
* rule sets to receive email with Amazon SES. For more information,
* see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* DescribeActiveReceiptRuleSet operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.DescribeActiveReceiptRuleSet
*/
java.util.concurrent.Future describeActiveReceiptRuleSetAsync(
DescribeActiveReceiptRuleSetRequest describeActiveReceiptRuleSetRequest);
/**
*
* Returns the metadata and receipt rules for the receipt rule set that is
* currently active.
*
*
* For information about setting up receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeActiveReceiptRuleSetRequest
* Represents a request to return the metadata and receipt rules for
* the receipt rule set that is currently active. You use receipt
* rule sets to receive email with Amazon SES. For more information,
* see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeActiveReceiptRuleSet operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.DescribeActiveReceiptRuleSet
*/
java.util.concurrent.Future describeActiveReceiptRuleSetAsync(
DescribeActiveReceiptRuleSetRequest describeActiveReceiptRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the details of the specified receipt rule.
*
*
* For information about setting up receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeReceiptRuleRequest
* Represents a request to return the details of a receipt rule. You
* use receipt rules to receive email with Amazon SES. For more
* information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the DescribeReceiptRule
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.DescribeReceiptRule
*/
java.util.concurrent.Future describeReceiptRuleAsync(
DescribeReceiptRuleRequest describeReceiptRuleRequest);
/**
*
* Returns the details of the specified receipt rule.
*
*
* For information about setting up receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeReceiptRuleRequest
* Represents a request to return the details of a receipt rule. You
* use receipt rules to receive email with Amazon SES. For more
* information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReceiptRule
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.DescribeReceiptRule
*/
java.util.concurrent.Future describeReceiptRuleAsync(
DescribeReceiptRuleRequest describeReceiptRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the details of the specified receipt rule set.
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeReceiptRuleSetRequest
* Represents a request to return the details of a receipt rule set.
* You use receipt rule sets to receive email with Amazon SES. For
* more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the DescribeReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.DescribeReceiptRuleSet
*/
java.util.concurrent.Future describeReceiptRuleSetAsync(
DescribeReceiptRuleSetRequest describeReceiptRuleSetRequest);
/**
*
* Returns the details of the specified receipt rule set.
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param describeReceiptRuleSetRequest
* Represents a request to return the details of a receipt rule set.
* You use receipt rule sets to receive email with Amazon SES. For
* more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.DescribeReceiptRuleSet
*/
java.util.concurrent.Future describeReceiptRuleSetAsync(
DescribeReceiptRuleSetRequest describeReceiptRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the current status of Easy DKIM signing for an entity. For domain
* name identities, this action also returns the DKIM tokens that are
* required for Easy DKIM signing, and whether Amazon SES has successfully
* verified that these tokens have been published.
*
*
* This action takes a list of identities as input and returns the following
* information for each:
*
*
* -
*
* Whether Easy DKIM signing is enabled or disabled.
*
*
* -
*
* A set of DKIM tokens that represent the identity. If the identity is an
* email address, the tokens represent the domain of that address.
*
*
* -
*
* Whether Amazon SES has successfully verified the DKIM tokens published in
* the domain's DNS. This information is only returned for domain name
* identities, not for email addresses.
*
*
*
*
* This action is throttled at one request per second and can only get DKIM
* attributes for up to 100 identities at a time.
*
*
* For more information about creating DNS records using DKIM tokens, go to
* the Amazon SES Developer Guide.
*
*
* @param getIdentityDkimAttributesRequest
* Represents a request for the status of Amazon SES Easy DKIM
* signing for an identity. For domain identities, this request also
* returns the DKIM tokens that are required for Easy DKIM signing,
* and whether Amazon SES successfully verified that these tokens
* were published. For more information about Easy DKIM, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* GetIdentityDkimAttributes operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.GetIdentityDkimAttributes
*/
java.util.concurrent.Future getIdentityDkimAttributesAsync(
GetIdentityDkimAttributesRequest getIdentityDkimAttributesRequest);
/**
*
* Returns the current status of Easy DKIM signing for an entity. For domain
* name identities, this action also returns the DKIM tokens that are
* required for Easy DKIM signing, and whether Amazon SES has successfully
* verified that these tokens have been published.
*
*
* This action takes a list of identities as input and returns the following
* information for each:
*
*
* -
*
* Whether Easy DKIM signing is enabled or disabled.
*
*
* -
*
* A set of DKIM tokens that represent the identity. If the identity is an
* email address, the tokens represent the domain of that address.
*
*
* -
*
* Whether Amazon SES has successfully verified the DKIM tokens published in
* the domain's DNS. This information is only returned for domain name
* identities, not for email addresses.
*
*
*
*
* This action is throttled at one request per second and can only get DKIM
* attributes for up to 100 identities at a time.
*
*
* For more information about creating DNS records using DKIM tokens, go to
* the Amazon SES Developer Guide.
*
*
* @param getIdentityDkimAttributesRequest
* Represents a request for the status of Amazon SES Easy DKIM
* signing for an identity. For domain identities, this request also
* returns the DKIM tokens that are required for Easy DKIM signing,
* and whether Amazon SES successfully verified that these tokens
* were published. For more information about Easy DKIM, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GetIdentityDkimAttributes operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.GetIdentityDkimAttributes
*/
java.util.concurrent.Future getIdentityDkimAttributesAsync(
GetIdentityDkimAttributesRequest getIdentityDkimAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the custom MAIL FROM attributes for a list of identities (email
* addresses and/or domains).
*
*
* This action is throttled at one request per second and can only get
* custom MAIL FROM attributes for up to 100 identities at a time.
*
*
* @param getIdentityMailFromDomainAttributesRequest
* Represents a request to return the Amazon SES custom MAIL FROM
* attributes for a list of identities. For information about using a
* custom MAIL FROM domain, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* GetIdentityMailFromDomainAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceAsync.GetIdentityMailFromDomainAttributes
*/
java.util.concurrent.Future getIdentityMailFromDomainAttributesAsync(
GetIdentityMailFromDomainAttributesRequest getIdentityMailFromDomainAttributesRequest);
/**
*
* Returns the custom MAIL FROM attributes for a list of identities (email
* addresses and/or domains).
*
*
* This action is throttled at one request per second and can only get
* custom MAIL FROM attributes for up to 100 identities at a time.
*
*
* @param getIdentityMailFromDomainAttributesRequest
* Represents a request to return the Amazon SES custom MAIL FROM
* attributes for a list of identities. For information about using a
* custom MAIL FROM domain, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GetIdentityMailFromDomainAttributes operation returned by the
* service.
* @sample
* AmazonSimpleEmailServiceAsyncHandler.GetIdentityMailFromDomainAttributes
*/
java.util.concurrent.Future getIdentityMailFromDomainAttributesAsync(
GetIdentityMailFromDomainAttributesRequest getIdentityMailFromDomainAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Given a list of verified identities (email addresses and/or domains),
* returns a structure describing identity notification attributes.
*
*
* This action is throttled at one request per second and can only get
* notification attributes for up to 100 identities at a time.
*
*
* For more information about using notifications with Amazon SES, see the
* Amazon SES Developer Guide.
*
*
* @param getIdentityNotificationAttributesRequest
* Represents a request to return the notification attributes for a
* list of identities you verified with Amazon SES. For information
* about Amazon SES notifications, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* GetIdentityNotificationAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceAsync.GetIdentityNotificationAttributes
*/
java.util.concurrent.Future getIdentityNotificationAttributesAsync(
GetIdentityNotificationAttributesRequest getIdentityNotificationAttributesRequest);
/**
*
* Given a list of verified identities (email addresses and/or domains),
* returns a structure describing identity notification attributes.
*
*
* This action is throttled at one request per second and can only get
* notification attributes for up to 100 identities at a time.
*
*
* For more information about using notifications with Amazon SES, see the
* Amazon SES Developer Guide.
*
*
* @param getIdentityNotificationAttributesRequest
* Represents a request to return the notification attributes for a
* list of identities you verified with Amazon SES. For information
* about Amazon SES notifications, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GetIdentityNotificationAttributes operation returned by the
* service.
* @sample
* AmazonSimpleEmailServiceAsyncHandler.GetIdentityNotificationAttributes
*/
java.util.concurrent.Future getIdentityNotificationAttributesAsync(
GetIdentityNotificationAttributesRequest getIdentityNotificationAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the requested sending authorization policies for the given
* identity (an email address or a domain). The policies are returned as a
* map of policy names to policy contents. You can retrieve a maximum of 20
* policies at a time.
*
*
*
* This API is for the identity owner only. If you have not verified the
* identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to
* authorize other senders to use its identities. For information about
* using sending authorization, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param getIdentityPoliciesRequest
* Represents a request to return the requested sending authorization
* policies for an identity. Sending authorization is an Amazon SES
* feature that enables you to authorize other senders to use your
* identities. For information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the GetIdentityPolicies
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.GetIdentityPolicies
*/
java.util.concurrent.Future getIdentityPoliciesAsync(
GetIdentityPoliciesRequest getIdentityPoliciesRequest);
/**
*
* Returns the requested sending authorization policies for the given
* identity (an email address or a domain). The policies are returned as a
* map of policy names to policy contents. You can retrieve a maximum of 20
* policies at a time.
*
*
*
* This API is for the identity owner only. If you have not verified the
* identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to
* authorize other senders to use its identities. For information about
* using sending authorization, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param getIdentityPoliciesRequest
* Represents a request to return the requested sending authorization
* policies for an identity. Sending authorization is an Amazon SES
* feature that enables you to authorize other senders to use your
* identities. For information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIdentityPolicies
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.GetIdentityPolicies
*/
java.util.concurrent.Future getIdentityPoliciesAsync(
GetIdentityPoliciesRequest getIdentityPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Given a list of identities (email addresses and/or domains), returns the
* verification status and (for domain identities) the verification token
* for each identity.
*
*
* This action is throttled at one request per second and can only get
* verification attributes for up to 100 identities at a time.
*
*
* @param getIdentityVerificationAttributesRequest
* Represents a request to return the Amazon SES verification status
* of a list of identities. For domain identities, this request also
* returns the verification token. For information about verifying
* identities with Amazon SES, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* GetIdentityVerificationAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceAsync.GetIdentityVerificationAttributes
*/
java.util.concurrent.Future getIdentityVerificationAttributesAsync(
GetIdentityVerificationAttributesRequest getIdentityVerificationAttributesRequest);
/**
*
* Given a list of identities (email addresses and/or domains), returns the
* verification status and (for domain identities) the verification token
* for each identity.
*
*
* This action is throttled at one request per second and can only get
* verification attributes for up to 100 identities at a time.
*
*
* @param getIdentityVerificationAttributesRequest
* Represents a request to return the Amazon SES verification status
* of a list of identities. For domain identities, this request also
* returns the verification token. For information about verifying
* identities with Amazon SES, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* GetIdentityVerificationAttributes operation returned by the
* service.
* @sample
* AmazonSimpleEmailServiceAsyncHandler.GetIdentityVerificationAttributes
*/
java.util.concurrent.Future getIdentityVerificationAttributesAsync(
GetIdentityVerificationAttributesRequest getIdentityVerificationAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the user's current sending limits.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendQuotaRequest
* @return A Java Future containing the result of the GetSendQuota operation
* returned by the service.
* @sample AmazonSimpleEmailServiceAsync.GetSendQuota
*/
java.util.concurrent.Future getSendQuotaAsync(
GetSendQuotaRequest getSendQuotaRequest);
/**
*
* Returns the user's current sending limits.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendQuotaRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSendQuota operation
* returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.GetSendQuota
*/
java.util.concurrent.Future getSendQuotaAsync(
GetSendQuotaRequest getSendQuotaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetSendQuota operation.
*
* @see #getSendQuotaAsync(GetSendQuotaRequest)
*/
java.util.concurrent.Future getSendQuotaAsync();
/**
* Simplified method form for invoking the GetSendQuota operation with an
* AsyncHandler.
*
* @see #getSendQuotaAsync(GetSendQuotaRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getSendQuotaAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the user's sending statistics. The result is a list of data
* points, representing the last two weeks of sending activity.
*
*
* Each data point in the list contains statistics for a 15-minute interval.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendStatisticsRequest
* @return A Java Future containing the result of the GetSendStatistics
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.GetSendStatistics
*/
java.util.concurrent.Future getSendStatisticsAsync(
GetSendStatisticsRequest getSendStatisticsRequest);
/**
*
* Returns the user's sending statistics. The result is a list of data
* points, representing the last two weeks of sending activity.
*
*
* Each data point in the list contains statistics for a 15-minute interval.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSendStatistics
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.GetSendStatistics
*/
java.util.concurrent.Future getSendStatisticsAsync(
GetSendStatisticsRequest getSendStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetSendStatistics operation.
*
* @see #getSendStatisticsAsync(GetSendStatisticsRequest)
*/
java.util.concurrent.Future getSendStatisticsAsync();
/**
* Simplified method form for invoking the GetSendStatistics operation with
* an AsyncHandler.
*
* @see #getSendStatisticsAsync(GetSendStatisticsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getSendStatisticsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list containing all of the identities (email addresses and
* domains) for your AWS account, regardless of verification status.
*
*
* This action is throttled at one request per second.
*
*
* @param listIdentitiesRequest
* Represents a request to return a list of all identities (email
* addresses and domains) that you have attempted to verify under
* your AWS account, regardless of verification status.
* @return A Java Future containing the result of the ListIdentities
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.ListIdentities
*/
java.util.concurrent.Future listIdentitiesAsync(
ListIdentitiesRequest listIdentitiesRequest);
/**
*
* Returns a list containing all of the identities (email addresses and
* domains) for your AWS account, regardless of verification status.
*
*
* This action is throttled at one request per second.
*
*
* @param listIdentitiesRequest
* Represents a request to return a list of all identities (email
* addresses and domains) that you have attempted to verify under
* your AWS account, regardless of verification status.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIdentities
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.ListIdentities
*/
java.util.concurrent.Future listIdentitiesAsync(
ListIdentitiesRequest listIdentitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListIdentities operation.
*
* @see #listIdentitiesAsync(ListIdentitiesRequest)
*/
java.util.concurrent.Future listIdentitiesAsync();
/**
* Simplified method form for invoking the ListIdentities operation with an
* AsyncHandler.
*
* @see #listIdentitiesAsync(ListIdentitiesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listIdentitiesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of sending authorization policies that are attached to the
* given identity (an email address or a domain). This API returns only a
* list. If you want the actual policy content, you can use
* GetIdentityPolicies
.
*
*
*
* This API is for the identity owner only. If you have not verified the
* identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to
* authorize other senders to use its identities. For information about
* using sending authorization, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param listIdentityPoliciesRequest
* Represents a request to return a list of sending authorization
* policies that are attached to an identity. Sending authorization
* is an Amazon SES feature that enables you to authorize other
* senders to use your identities. For information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the ListIdentityPolicies
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.ListIdentityPolicies
*/
java.util.concurrent.Future listIdentityPoliciesAsync(
ListIdentityPoliciesRequest listIdentityPoliciesRequest);
/**
*
* Returns a list of sending authorization policies that are attached to the
* given identity (an email address or a domain). This API returns only a
* list. If you want the actual policy content, you can use
* GetIdentityPolicies
.
*
*
*
* This API is for the identity owner only. If you have not verified the
* identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to
* authorize other senders to use its identities. For information about
* using sending authorization, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param listIdentityPoliciesRequest
* Represents a request to return a list of sending authorization
* policies that are attached to an identity. Sending authorization
* is an Amazon SES feature that enables you to authorize other
* senders to use your identities. For information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIdentityPolicies
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.ListIdentityPolicies
*/
java.util.concurrent.Future listIdentityPoliciesAsync(
ListIdentityPoliciesRequest listIdentityPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the IP address filters associated with your AWS account.
*
*
* For information about managing IP address filters, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param listReceiptFiltersRequest
* : Represents a request to list the IP address filters that exist
* under your AWS account. You use IP address filters when you
* receive email with Amazon SES. For more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the ListReceiptFilters
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.ListReceiptFilters
*/
java.util.concurrent.Future listReceiptFiltersAsync(
ListReceiptFiltersRequest listReceiptFiltersRequest);
/**
*
* Lists the IP address filters associated with your AWS account.
*
*
* For information about managing IP address filters, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param listReceiptFiltersRequest
* : Represents a request to list the IP address filters that exist
* under your AWS account. You use IP address filters when you
* receive email with Amazon SES. For more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListReceiptFilters
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.ListReceiptFilters
*/
java.util.concurrent.Future listReceiptFiltersAsync(
ListReceiptFiltersRequest listReceiptFiltersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the receipt rule sets that exist under your AWS account. If there
* are additional receipt rule sets to be retrieved, you will receive a
* NextToken
that you can provide to the next call to
* ListReceiptRuleSets
to retrieve the additional entries.
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param listReceiptRuleSetsRequest
* Represents a request to list the receipt rule sets that exist
* under your AWS account. You use receipt rule sets to receive email
* with Amazon SES. For more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the ListReceiptRuleSets
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.ListReceiptRuleSets
*/
java.util.concurrent.Future listReceiptRuleSetsAsync(
ListReceiptRuleSetsRequest listReceiptRuleSetsRequest);
/**
*
* Lists the receipt rule sets that exist under your AWS account. If there
* are additional receipt rule sets to be retrieved, you will receive a
* NextToken
that you can provide to the next call to
* ListReceiptRuleSets
to retrieve the additional entries.
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param listReceiptRuleSetsRequest
* Represents a request to list the receipt rule sets that exist
* under your AWS account. You use receipt rule sets to receive email
* with Amazon SES. For more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListReceiptRuleSets
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.ListReceiptRuleSets
*/
java.util.concurrent.Future listReceiptRuleSetsAsync(
ListReceiptRuleSetsRequest listReceiptRuleSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list containing all of the email addresses that have been
* verified.
*
*
*
* The ListVerifiedEmailAddresses action is deprecated as of the May 15,
* 2012 release of Domain Verification. The ListIdentities action is now
* preferred.
*
*
*
* This action is throttled at one request per second.
*
*
* @param listVerifiedEmailAddressesRequest
* @return A Java Future containing the result of the
* ListVerifiedEmailAddresses operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.ListVerifiedEmailAddresses
*/
java.util.concurrent.Future listVerifiedEmailAddressesAsync(
ListVerifiedEmailAddressesRequest listVerifiedEmailAddressesRequest);
/**
*
* Returns a list containing all of the email addresses that have been
* verified.
*
*
*
* The ListVerifiedEmailAddresses action is deprecated as of the May 15,
* 2012 release of Domain Verification. The ListIdentities action is now
* preferred.
*
*
*
* This action is throttled at one request per second.
*
*
* @param listVerifiedEmailAddressesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ListVerifiedEmailAddresses operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.ListVerifiedEmailAddresses
*/
java.util.concurrent.Future listVerifiedEmailAddressesAsync(
ListVerifiedEmailAddressesRequest listVerifiedEmailAddressesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListVerifiedEmailAddresses
* operation.
*
* @see #listVerifiedEmailAddressesAsync(ListVerifiedEmailAddressesRequest)
*/
java.util.concurrent.Future listVerifiedEmailAddressesAsync();
/**
* Simplified method form for invoking the ListVerifiedEmailAddresses
* operation with an AsyncHandler.
*
* @see #listVerifiedEmailAddressesAsync(ListVerifiedEmailAddressesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listVerifiedEmailAddressesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or updates a sending authorization policy for the specified identity
* (an email address or a domain).
*
*
*
* This API is for the identity owner only. If you have not verified the
* identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to
* authorize other senders to use its identities. For information about
* using sending authorization, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param putIdentityPolicyRequest
* Represents a request to add or update a sending authorization
* policy for an identity. Sending authorization is an Amazon SES
* feature that enables you to authorize other senders to use your
* identities. For information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the PutIdentityPolicy
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.PutIdentityPolicy
*/
java.util.concurrent.Future putIdentityPolicyAsync(
PutIdentityPolicyRequest putIdentityPolicyRequest);
/**
*
* Adds or updates a sending authorization policy for the specified identity
* (an email address or a domain).
*
*
*
* This API is for the identity owner only. If you have not verified the
* identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to
* authorize other senders to use its identities. For information about
* using sending authorization, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param putIdentityPolicyRequest
* Represents a request to add or update a sending authorization
* policy for an identity. Sending authorization is an Amazon SES
* feature that enables you to authorize other senders to use your
* identities. For information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutIdentityPolicy
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.PutIdentityPolicy
*/
java.util.concurrent.Future putIdentityPolicyAsync(
PutIdentityPolicyRequest putIdentityPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Reorders the receipt rules within a receipt rule set.
*
*
*
* All of the rules in the rule set must be represented in this request.
* That is, this API will return an error if the reorder request doesn't
* explicitly position all of the rules.
*
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param reorderReceiptRuleSetRequest
* Represents a request to reorder the receipt rules within a receipt
* rule set. You use receipt rule sets to receive email with Amazon
* SES. For more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the ReorderReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.ReorderReceiptRuleSet
*/
java.util.concurrent.Future reorderReceiptRuleSetAsync(
ReorderReceiptRuleSetRequest reorderReceiptRuleSetRequest);
/**
*
* Reorders the receipt rules within a receipt rule set.
*
*
*
* All of the rules in the rule set must be represented in this request.
* That is, this API will return an error if the reorder request doesn't
* explicitly position all of the rules.
*
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param reorderReceiptRuleSetRequest
* Represents a request to reorder the receipt rules within a receipt
* rule set. You use receipt rule sets to receive email with Amazon
* SES. For more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ReorderReceiptRuleSet
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.ReorderReceiptRuleSet
*/
java.util.concurrent.Future reorderReceiptRuleSetAsync(
ReorderReceiptRuleSetRequest reorderReceiptRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates and sends a bounce message to the sender of an email you
* received through Amazon SES. You can only use this API on an email up to
* 24 hours after you receive it.
*
*
*
* You cannot use this API to send generic bounces for mail that was not
* received by Amazon SES.
*
*
*
* For information about receiving email through Amazon SES, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param sendBounceRequest
* Represents a request to send a bounce message to the sender of an
* email you received through Amazon SES.
* @return A Java Future containing the result of the SendBounce operation
* returned by the service.
* @sample AmazonSimpleEmailServiceAsync.SendBounce
*/
java.util.concurrent.Future sendBounceAsync(
SendBounceRequest sendBounceRequest);
/**
*
* Generates and sends a bounce message to the sender of an email you
* received through Amazon SES. You can only use this API on an email up to
* 24 hours after you receive it.
*
*
*
* You cannot use this API to send generic bounces for mail that was not
* received by Amazon SES.
*
*
*
* For information about receiving email through Amazon SES, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param sendBounceRequest
* Represents a request to send a bounce message to the sender of an
* email you received through Amazon SES.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendBounce operation
* returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.SendBounce
*/
java.util.concurrent.Future sendBounceAsync(
SendBounceRequest sendBounceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Composes an email message based on input data, and then immediately
* queues the message for sending.
*
*
* There are several important points to know about SendEmail
:
*
*
* -
*
* You can only send email from verified email addresses and domains;
* otherwise, you will get an "Email address not verified" error. If your
* account is still in the Amazon SES sandbox, you must also verify every
* recipient email address except for the recipients provided by the Amazon
* SES mailbox simulator. For more information, go to the Amazon SES Developer Guide.
*
*
* -
*
* The total size of the message cannot exceed 10 MB. This includes any
* attachments that are part of the message.
*
*
* -
*
* Amazon SES has a limit on the total number of recipients per message. The
* combined number of To:, CC: and BCC: email addresses cannot exceed 50. If
* you need to send an email message to a larger audience, you can divide
* your recipient list into groups of 50 or fewer, and then call Amazon SES
* repeatedly to send the message to each group.
*
*
* -
*
* For every message that you send, the total number of recipients (To:, CC:
* and BCC:) is counted against your sending quota - the maximum number of
* emails you can send in a 24-hour period. For information about your
* sending quota, go to the Amazon SES Developer Guide.
*
*
*
*
* @param sendEmailRequest
* Represents a request to send a single formatted email using Amazon
* SES. For more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the SendEmail operation
* returned by the service.
* @sample AmazonSimpleEmailServiceAsync.SendEmail
*/
java.util.concurrent.Future sendEmailAsync(
SendEmailRequest sendEmailRequest);
/**
*
* Composes an email message based on input data, and then immediately
* queues the message for sending.
*
*
* There are several important points to know about SendEmail
:
*
*
* -
*
* You can only send email from verified email addresses and domains;
* otherwise, you will get an "Email address not verified" error. If your
* account is still in the Amazon SES sandbox, you must also verify every
* recipient email address except for the recipients provided by the Amazon
* SES mailbox simulator. For more information, go to the Amazon SES Developer Guide.
*
*
* -
*
* The total size of the message cannot exceed 10 MB. This includes any
* attachments that are part of the message.
*
*
* -
*
* Amazon SES has a limit on the total number of recipients per message. The
* combined number of To:, CC: and BCC: email addresses cannot exceed 50. If
* you need to send an email message to a larger audience, you can divide
* your recipient list into groups of 50 or fewer, and then call Amazon SES
* repeatedly to send the message to each group.
*
*
* -
*
* For every message that you send, the total number of recipients (To:, CC:
* and BCC:) is counted against your sending quota - the maximum number of
* emails you can send in a 24-hour period. For information about your
* sending quota, go to the Amazon SES Developer Guide.
*
*
*
*
* @param sendEmailRequest
* Represents a request to send a single formatted email using Amazon
* SES. For more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendEmail operation
* returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.SendEmail
*/
java.util.concurrent.Future sendEmailAsync(
SendEmailRequest sendEmailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends an email message, with header and content specified by the client.
* The SendRawEmail
action is useful for sending multipart MIME
* emails. The raw text of the message must comply with Internet email
* standards; otherwise, the message cannot be sent.
*
*
* There are several important points to know about
* SendRawEmail
:
*
*
* -
*
* You can only send email from verified email addresses and domains;
* otherwise, you will get an "Email address not verified" error. If your
* account is still in the Amazon SES sandbox, you must also verify every
* recipient email address except for the recipients provided by the Amazon
* SES mailbox simulator. For more information, go to the Amazon SES Developer Guide.
*
*
* -
*
* The total size of the message cannot exceed 10 MB. This includes any
* attachments that are part of the message.
*
*
* -
*
* Amazon SES has a limit on the total number of recipients per message. The
* combined number of To:, CC: and BCC: email addresses cannot exceed 50. If
* you need to send an email message to a larger audience, you can divide
* your recipient list into groups of 50 or fewer, and then call Amazon SES
* repeatedly to send the message to each group.
*
*
* -
*
* The To:, CC:, and BCC: headers in the raw message can contain a group
* list. Note that each recipient in a group list counts towards the
* 50-recipient limit.
*
*
* -
*
* For every message that you send, the total number of recipients (To:, CC:
* and BCC:) is counted against your sending quota - the maximum number of
* emails you can send in a 24-hour period. For information about your
* sending quota, go to the Amazon SES Developer Guide.
*
*
* -
*
* If you are using sending authorization to send on behalf of another user,
* SendRawEmail
enables you to specify the cross-account
* identity for the email's "Source," "From," and "Return-Path" parameters
* in one of two ways: you can pass optional parameters
* SourceArn
, FromArn
, and/or
* ReturnPathArn
to the API, or you can include the following
* X-headers in the header of your raw email:
*
*
* -
*
* X-SES-SOURCE-ARN
*
*
* -
*
* X-SES-FROM-ARN
*
*
* -
*
* X-SES-RETURN-PATH-ARN
*
*
*
*
*
* Do not include these X-headers in the DKIM signature, because they are
* removed by Amazon SES before sending the email.
*
*
*
* For the most common sending authorization use case, we recommend that you
* specify the SourceIdentityArn
and do not specify either the
* FromIdentityArn
or ReturnPathIdentityArn
. (The
* same note applies to the corresponding X-headers.) If you only specify
* the SourceIdentityArn
, Amazon SES will simply set the "From"
* address and the "Return Path" address to the identity specified in
* SourceIdentityArn
. For more information about sending
* authorization, see the Amazon SES Developer Guide.
*
*
*
*
* @param sendRawEmailRequest
* Represents a request to send a single raw email using Amazon SES.
* For more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the SendRawEmail operation
* returned by the service.
* @sample AmazonSimpleEmailServiceAsync.SendRawEmail
*/
java.util.concurrent.Future sendRawEmailAsync(
SendRawEmailRequest sendRawEmailRequest);
/**
*
* Sends an email message, with header and content specified by the client.
* The SendRawEmail
action is useful for sending multipart MIME
* emails. The raw text of the message must comply with Internet email
* standards; otherwise, the message cannot be sent.
*
*
* There are several important points to know about
* SendRawEmail
:
*
*
* -
*
* You can only send email from verified email addresses and domains;
* otherwise, you will get an "Email address not verified" error. If your
* account is still in the Amazon SES sandbox, you must also verify every
* recipient email address except for the recipients provided by the Amazon
* SES mailbox simulator. For more information, go to the Amazon SES Developer Guide.
*
*
* -
*
* The total size of the message cannot exceed 10 MB. This includes any
* attachments that are part of the message.
*
*
* -
*
* Amazon SES has a limit on the total number of recipients per message. The
* combined number of To:, CC: and BCC: email addresses cannot exceed 50. If
* you need to send an email message to a larger audience, you can divide
* your recipient list into groups of 50 or fewer, and then call Amazon SES
* repeatedly to send the message to each group.
*
*
* -
*
* The To:, CC:, and BCC: headers in the raw message can contain a group
* list. Note that each recipient in a group list counts towards the
* 50-recipient limit.
*
*
* -
*
* For every message that you send, the total number of recipients (To:, CC:
* and BCC:) is counted against your sending quota - the maximum number of
* emails you can send in a 24-hour period. For information about your
* sending quota, go to the Amazon SES Developer Guide.
*
*
* -
*
* If you are using sending authorization to send on behalf of another user,
* SendRawEmail
enables you to specify the cross-account
* identity for the email's "Source," "From," and "Return-Path" parameters
* in one of two ways: you can pass optional parameters
* SourceArn
, FromArn
, and/or
* ReturnPathArn
to the API, or you can include the following
* X-headers in the header of your raw email:
*
*
* -
*
* X-SES-SOURCE-ARN
*
*
* -
*
* X-SES-FROM-ARN
*
*
* -
*
* X-SES-RETURN-PATH-ARN
*
*
*
*
*
* Do not include these X-headers in the DKIM signature, because they are
* removed by Amazon SES before sending the email.
*
*
*
* For the most common sending authorization use case, we recommend that you
* specify the SourceIdentityArn
and do not specify either the
* FromIdentityArn
or ReturnPathIdentityArn
. (The
* same note applies to the corresponding X-headers.) If you only specify
* the SourceIdentityArn
, Amazon SES will simply set the "From"
* address and the "Return Path" address to the identity specified in
* SourceIdentityArn
. For more information about sending
* authorization, see the Amazon SES Developer Guide.
*
*
*
*
* @param sendRawEmailRequest
* Represents a request to send a single raw email using Amazon SES.
* For more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendRawEmail operation
* returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.SendRawEmail
*/
java.util.concurrent.Future sendRawEmailAsync(
SendRawEmailRequest sendRawEmailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the specified receipt rule set as the active receipt rule set.
*
*
*
* To disable your email-receiving through Amazon SES completely, you can
* call this API with RuleSetName set to null.
*
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param setActiveReceiptRuleSetRequest
* Represents a request to set a receipt rule set as the active
* receipt rule set. You use receipt rule sets to receive email with
* Amazon SES. For more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* SetActiveReceiptRuleSet operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.SetActiveReceiptRuleSet
*/
java.util.concurrent.Future setActiveReceiptRuleSetAsync(
SetActiveReceiptRuleSetRequest setActiveReceiptRuleSetRequest);
/**
*
* Sets the specified receipt rule set as the active receipt rule set.
*
*
*
* To disable your email-receiving through Amazon SES completely, you can
* call this API with RuleSetName set to null.
*
*
*
* For information about managing receipt rule sets, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param setActiveReceiptRuleSetRequest
* Represents a request to set a receipt rule set as the active
* receipt rule set. You use receipt rule sets to receive email with
* Amazon SES. For more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* SetActiveReceiptRuleSet operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.SetActiveReceiptRuleSet
*/
java.util.concurrent.Future setActiveReceiptRuleSetAsync(
SetActiveReceiptRuleSetRequest setActiveReceiptRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables or disables Easy DKIM signing of email sent from an identity:
*
*
* -
*
* If Easy DKIM signing is enabled for a domain name identity (e.g.,
* example.com
), then Amazon SES will DKIM-sign all email sent
* by addresses under that domain name (e.g., [email protected]
* ).
*
*
* -
*
* If Easy DKIM signing is enabled for an email address, then Amazon SES
* will DKIM-sign all email sent by that email address.
*
*
*
*
* For email addresses (e.g., [email protected]
), you can only
* enable Easy DKIM signing if the corresponding domain (e.g.,
* example.com
) has been set up for Easy DKIM using the AWS
* Console or the VerifyDomainDkim
action.
*
*
* This action is throttled at one request per second.
*
*
* For more information about Easy DKIM signing, go to the Amazon SES Developer Guide.
*
*
* @param setIdentityDkimEnabledRequest
* Represents a request to enable or disable Amazon SES Easy DKIM
* signing for an identity. For more information about setting up
* Easy DKIM, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the SetIdentityDkimEnabled
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.SetIdentityDkimEnabled
*/
java.util.concurrent.Future setIdentityDkimEnabledAsync(
SetIdentityDkimEnabledRequest setIdentityDkimEnabledRequest);
/**
*
* Enables or disables Easy DKIM signing of email sent from an identity:
*
*
* -
*
* If Easy DKIM signing is enabled for a domain name identity (e.g.,
* example.com
), then Amazon SES will DKIM-sign all email sent
* by addresses under that domain name (e.g., [email protected]
* ).
*
*
* -
*
* If Easy DKIM signing is enabled for an email address, then Amazon SES
* will DKIM-sign all email sent by that email address.
*
*
*
*
* For email addresses (e.g., [email protected]
), you can only
* enable Easy DKIM signing if the corresponding domain (e.g.,
* example.com
) has been set up for Easy DKIM using the AWS
* Console or the VerifyDomainDkim
action.
*
*
* This action is throttled at one request per second.
*
*
* For more information about Easy DKIM signing, go to the Amazon SES Developer Guide.
*
*
* @param setIdentityDkimEnabledRequest
* Represents a request to enable or disable Amazon SES Easy DKIM
* signing for an identity. For more information about setting up
* Easy DKIM, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetIdentityDkimEnabled
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.SetIdentityDkimEnabled
*/
java.util.concurrent.Future setIdentityDkimEnabledAsync(
SetIdentityDkimEnabledRequest setIdentityDkimEnabledRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Given an identity (an email address or a domain), enables or disables
* whether Amazon SES forwards bounce and complaint notifications as email.
* Feedback forwarding can only be disabled when Amazon Simple Notification
* Service (Amazon SNS) topics are specified for both bounces and
* complaints.
*
*
*
* Feedback forwarding does not apply to delivery notifications. Delivery
* notifications are only available through Amazon SNS.
*
*
*
* This action is throttled at one request per second.
*
*
* For more information about using notifications with Amazon SES, see the
* Amazon SES Developer Guide.
*
*
* @param setIdentityFeedbackForwardingEnabledRequest
* Represents a request to enable or disable whether Amazon SES
* forwards you bounce and complaint notifications through email. For
* information about email feedback forwarding, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* SetIdentityFeedbackForwardingEnabled operation returned by the
* service.
* @sample
* AmazonSimpleEmailServiceAsync.SetIdentityFeedbackForwardingEnabled
*/
java.util.concurrent.Future setIdentityFeedbackForwardingEnabledAsync(
SetIdentityFeedbackForwardingEnabledRequest setIdentityFeedbackForwardingEnabledRequest);
/**
*
* Given an identity (an email address or a domain), enables or disables
* whether Amazon SES forwards bounce and complaint notifications as email.
* Feedback forwarding can only be disabled when Amazon Simple Notification
* Service (Amazon SNS) topics are specified for both bounces and
* complaints.
*
*
*
* Feedback forwarding does not apply to delivery notifications. Delivery
* notifications are only available through Amazon SNS.
*
*
*
* This action is throttled at one request per second.
*
*
* For more information about using notifications with Amazon SES, see the
* Amazon SES Developer Guide.
*
*
* @param setIdentityFeedbackForwardingEnabledRequest
* Represents a request to enable or disable whether Amazon SES
* forwards you bounce and complaint notifications through email. For
* information about email feedback forwarding, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* SetIdentityFeedbackForwardingEnabled operation returned by the
* service.
* @sample AmazonSimpleEmailServiceAsyncHandler.
* SetIdentityFeedbackForwardingEnabled
*/
java.util.concurrent.Future setIdentityFeedbackForwardingEnabledAsync(
SetIdentityFeedbackForwardingEnabledRequest setIdentityFeedbackForwardingEnabledRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Given an identity (an email address or a domain), sets whether Amazon SES
* includes the original email headers in the Amazon Simple Notification
* Service (Amazon SNS) notifications of a specified type.
*
*
* This action is throttled at one request per second.
*
*
* For more information about using notifications with Amazon SES, see the
* Amazon SES Developer Guide.
*
*
* @param setIdentityHeadersInNotificationsEnabledRequest
* Represents a request to set whether Amazon SES includes the
* original email headers in the Amazon SNS notifications of a
* specified type. For information about notifications, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* SetIdentityHeadersInNotificationsEnabled operation returned by
* the service.
* @sample
* AmazonSimpleEmailServiceAsync.SetIdentityHeadersInNotificationsEnabled
*/
java.util.concurrent.Future setIdentityHeadersInNotificationsEnabledAsync(
SetIdentityHeadersInNotificationsEnabledRequest setIdentityHeadersInNotificationsEnabledRequest);
/**
*
* Given an identity (an email address or a domain), sets whether Amazon SES
* includes the original email headers in the Amazon Simple Notification
* Service (Amazon SNS) notifications of a specified type.
*
*
* This action is throttled at one request per second.
*
*
* For more information about using notifications with Amazon SES, see the
* Amazon SES Developer Guide.
*
*
* @param setIdentityHeadersInNotificationsEnabledRequest
* Represents a request to set whether Amazon SES includes the
* original email headers in the Amazon SNS notifications of a
* specified type. For information about notifications, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* SetIdentityHeadersInNotificationsEnabled operation returned by
* the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.
* SetIdentityHeadersInNotificationsEnabled
*/
java.util.concurrent.Future setIdentityHeadersInNotificationsEnabledAsync(
SetIdentityHeadersInNotificationsEnabledRequest setIdentityHeadersInNotificationsEnabledRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables or disables the custom MAIL FROM domain setup for a verified
* identity (an email address or a domain).
*
*
*
* To send emails using the specified MAIL FROM domain, you must add an MX
* record to your MAIL FROM domain's DNS settings. If you want your emails
* to pass Sender Policy Framework (SPF) checks, you must also add or update
* an SPF record. For more information, see the Amazon SES Developer Guide.
*
*
*
* This action is throttled at one request per second.
*
*
* @param setIdentityMailFromDomainRequest
* Represents a request to enable or disable the Amazon SES custom
* MAIL FROM domain setup for a verified identity. For information
* about using a custom MAIL FROM domain, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* SetIdentityMailFromDomain operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.SetIdentityMailFromDomain
*/
java.util.concurrent.Future setIdentityMailFromDomainAsync(
SetIdentityMailFromDomainRequest setIdentityMailFromDomainRequest);
/**
*
* Enables or disables the custom MAIL FROM domain setup for a verified
* identity (an email address or a domain).
*
*
*
* To send emails using the specified MAIL FROM domain, you must add an MX
* record to your MAIL FROM domain's DNS settings. If you want your emails
* to pass Sender Policy Framework (SPF) checks, you must also add or update
* an SPF record. For more information, see the Amazon SES Developer Guide.
*
*
*
* This action is throttled at one request per second.
*
*
* @param setIdentityMailFromDomainRequest
* Represents a request to enable or disable the Amazon SES custom
* MAIL FROM domain setup for a verified identity. For information
* about using a custom MAIL FROM domain, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* SetIdentityMailFromDomain operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.SetIdentityMailFromDomain
*/
java.util.concurrent.Future setIdentityMailFromDomainAsync(
SetIdentityMailFromDomainRequest setIdentityMailFromDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Given an identity (an email address or a domain), sets the Amazon Simple
* Notification Service (Amazon SNS) topic to which Amazon SES will publish
* bounce, complaint, and/or delivery notifications for emails sent with
* that identity as the Source
.
*
*
*
* Unless feedback forwarding is enabled, you must specify Amazon SNS topics
* for bounce and complaint notifications. For more information, see
* SetIdentityFeedbackForwardingEnabled
.
*
*
*
* This action is throttled at one request per second.
*
*
* For more information about feedback notification, see the Amazon SES Developer Guide.
*
*
* @param setIdentityNotificationTopicRequest
* Represents a request to specify the Amazon SNS topic to which
* Amazon SES will publish bounce, complaint, or delivery
* notifications for emails sent with that identity as the Source.
* For information about Amazon SES notifications, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the
* SetIdentityNotificationTopic operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.SetIdentityNotificationTopic
*/
java.util.concurrent.Future setIdentityNotificationTopicAsync(
SetIdentityNotificationTopicRequest setIdentityNotificationTopicRequest);
/**
*
* Given an identity (an email address or a domain), sets the Amazon Simple
* Notification Service (Amazon SNS) topic to which Amazon SES will publish
* bounce, complaint, and/or delivery notifications for emails sent with
* that identity as the Source
.
*
*
*
* Unless feedback forwarding is enabled, you must specify Amazon SNS topics
* for bounce and complaint notifications. For more information, see
* SetIdentityFeedbackForwardingEnabled
.
*
*
*
* This action is throttled at one request per second.
*
*
* For more information about feedback notification, see the Amazon SES Developer Guide.
*
*
* @param setIdentityNotificationTopicRequest
* Represents a request to specify the Amazon SNS topic to which
* Amazon SES will publish bounce, complaint, or delivery
* notifications for emails sent with that identity as the Source.
* For information about Amazon SES notifications, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* SetIdentityNotificationTopic operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.SetIdentityNotificationTopic
*/
java.util.concurrent.Future setIdentityNotificationTopicAsync(
SetIdentityNotificationTopicRequest setIdentityNotificationTopicRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the position of the specified receipt rule in the receipt rule set.
*
*
* For information about managing receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param setReceiptRulePositionRequest
* Represents a request to set the position of a receipt rule in a
* receipt rule set. You use receipt rule sets to receive email with
* Amazon SES. For more information, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the SetReceiptRulePosition
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.SetReceiptRulePosition
*/
java.util.concurrent.Future setReceiptRulePositionAsync(
SetReceiptRulePositionRequest setReceiptRulePositionRequest);
/**
*
* Sets the position of the specified receipt rule in the receipt rule set.
*
*
* For information about managing receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param setReceiptRulePositionRequest
* Represents a request to set the position of a receipt rule in a
* receipt rule set. You use receipt rule sets to receive email with
* Amazon SES. For more information, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetReceiptRulePosition
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.SetReceiptRulePosition
*/
java.util.concurrent.Future setReceiptRulePositionAsync(
SetReceiptRulePositionRequest setReceiptRulePositionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a receipt rule.
*
*
* For information about managing receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param updateReceiptRuleRequest
* Represents a request to update a receipt rule. You use receipt
* rules to receive email with Amazon SES. For more information, see
* the Amazon SES Developer Guide.
* @return A Java Future containing the result of the UpdateReceiptRule
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.UpdateReceiptRule
*/
java.util.concurrent.Future updateReceiptRuleAsync(
UpdateReceiptRuleRequest updateReceiptRuleRequest);
/**
*
* Updates a receipt rule.
*
*
* For information about managing receipt rules, see the Amazon SES Developer Guide.
*
*
* This action is throttled at one request per second.
*
*
* @param updateReceiptRuleRequest
* Represents a request to update a receipt rule. You use receipt
* rules to receive email with Amazon SES. For more information, see
* the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateReceiptRule
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.UpdateReceiptRule
*/
java.util.concurrent.Future updateReceiptRuleAsync(
UpdateReceiptRuleRequest updateReceiptRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a set of DKIM tokens for a domain. DKIM tokens are
* character strings that represent your domain's identity. Using these
* tokens, you will need to create DNS CNAME records that point to DKIM
* public keys hosted by Amazon SES. Amazon Web Services will eventually
* detect that you have updated your DNS records; this detection process may
* take up to 72 hours. Upon successful detection, Amazon SES will be able
* to DKIM-sign email originating from that domain.
*
*
* This action is throttled at one request per second.
*
*
* To enable or disable Easy DKIM signing for a domain, use the
* SetIdentityDkimEnabled
action.
*
*
* For more information about creating DNS records using DKIM tokens, go to
* the Amazon SES Developer Guide.
*
*
* @param verifyDomainDkimRequest
* Represents a request to generate the CNAME records needed to set
* up Easy DKIM with Amazon SES. For more information about setting
* up Easy DKIM, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the VerifyDomainDkim
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.VerifyDomainDkim
*/
java.util.concurrent.Future verifyDomainDkimAsync(
VerifyDomainDkimRequest verifyDomainDkimRequest);
/**
*
* Returns a set of DKIM tokens for a domain. DKIM tokens are
* character strings that represent your domain's identity. Using these
* tokens, you will need to create DNS CNAME records that point to DKIM
* public keys hosted by Amazon SES. Amazon Web Services will eventually
* detect that you have updated your DNS records; this detection process may
* take up to 72 hours. Upon successful detection, Amazon SES will be able
* to DKIM-sign email originating from that domain.
*
*
* This action is throttled at one request per second.
*
*
* To enable or disable Easy DKIM signing for a domain, use the
* SetIdentityDkimEnabled
action.
*
*
* For more information about creating DNS records using DKIM tokens, go to
* the Amazon SES Developer Guide.
*
*
* @param verifyDomainDkimRequest
* Represents a request to generate the CNAME records needed to set
* up Easy DKIM with Amazon SES. For more information about setting
* up Easy DKIM, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the VerifyDomainDkim
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.VerifyDomainDkim
*/
java.util.concurrent.Future verifyDomainDkimAsync(
VerifyDomainDkimRequest verifyDomainDkimRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Verifies a domain.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyDomainIdentityRequest
* Represents a request to begin Amazon SES domain verification and
* to generate the TXT records that you must publish to the DNS
* server of your domain to complete the verification. For
* information about domain verification, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the VerifyDomainIdentity
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.VerifyDomainIdentity
*/
java.util.concurrent.Future verifyDomainIdentityAsync(
VerifyDomainIdentityRequest verifyDomainIdentityRequest);
/**
*
* Verifies a domain.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyDomainIdentityRequest
* Represents a request to begin Amazon SES domain verification and
* to generate the TXT records that you must publish to the DNS
* server of your domain to complete the verification. For
* information about domain verification, see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the VerifyDomainIdentity
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.VerifyDomainIdentity
*/
java.util.concurrent.Future verifyDomainIdentityAsync(
VerifyDomainIdentityRequest verifyDomainIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Verifies an email address. This action causes a confirmation email
* message to be sent to the specified address.
*
*
*
* The VerifyEmailAddress action is deprecated as of the May 15, 2012
* release of Domain Verification. The VerifyEmailIdentity action is now
* preferred.
*
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailAddressRequest
* Represents a request to begin email address verification with
* Amazon SES. For information about email address verification, see
* the Amazon SES Developer Guide.
* @return A Java Future containing the result of the VerifyEmailAddress
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.VerifyEmailAddress
*/
java.util.concurrent.Future verifyEmailAddressAsync(
VerifyEmailAddressRequest verifyEmailAddressRequest);
/**
*
* Verifies an email address. This action causes a confirmation email
* message to be sent to the specified address.
*
*
*
* The VerifyEmailAddress action is deprecated as of the May 15, 2012
* release of Domain Verification. The VerifyEmailIdentity action is now
* preferred.
*
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailAddressRequest
* Represents a request to begin email address verification with
* Amazon SES. For information about email address verification, see
* the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the VerifyEmailAddress
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.VerifyEmailAddress
*/
java.util.concurrent.Future verifyEmailAddressAsync(
VerifyEmailAddressRequest verifyEmailAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Verifies an email address. This action causes a confirmation email
* message to be sent to the specified address.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailIdentityRequest
* Represents a request to begin email address verification with
* Amazon SES. For information about email address verification, see
* the Amazon SES Developer Guide.
* @return A Java Future containing the result of the VerifyEmailIdentity
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsync.VerifyEmailIdentity
*/
java.util.concurrent.Future verifyEmailIdentityAsync(
VerifyEmailIdentityRequest verifyEmailIdentityRequest);
/**
*
* Verifies an email address. This action causes a confirmation email
* message to be sent to the specified address.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailIdentityRequest
* Represents a request to begin email address verification with
* Amazon SES. For information about email address verification, see
* the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the VerifyEmailIdentity
* operation returned by the service.
* @sample AmazonSimpleEmailServiceAsyncHandler.VerifyEmailIdentity
*/
java.util.concurrent.Future verifyEmailIdentityAsync(
VerifyEmailIdentityRequest verifyEmailIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}