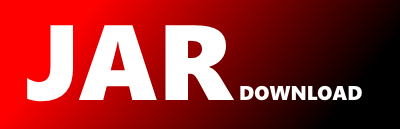
com.amazonaws.services.simpleemailv2.AmazonSimpleEmailServiceV2Async Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sesv2 Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simpleemailv2;
import javax.annotation.Generated;
import com.amazonaws.services.simpleemailv2.model.*;
/**
* Interface for accessing Amazon SES V2 asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.simpleemailv2.AbstractAmazonSimpleEmailServiceV2Async} instead.
*
*
* Amazon SES API v2
*
* Amazon SES is an Amazon Web Services service that you can use to send email
* messages to your customers.
*
*
* If you're new to Amazon SES API v2, you might find it helpful to review the Amazon Simple Email Service Developer Guide. The
* Amazon SES Developer Guide provides information and code samples that demonstrate how to use Amazon SES API v2
* features programmatically.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonSimpleEmailServiceV2Async extends AmazonSimpleEmailServiceV2 {
/**
*
* Create a configuration set. Configuration sets are groups of rules that you can apply to the emails that
* you send. You apply a configuration set to an email by specifying the name of the configuration set when you call
* the Amazon SES API v2. When you apply a configuration set to an email, all of the rules in that configuration set
* are applied to the email.
*
*
* @param createConfigurationSetRequest
* A request to create a configuration set.
* @return A Java Future containing the result of the CreateConfigurationSet operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateConfigurationSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createConfigurationSetAsync(CreateConfigurationSetRequest createConfigurationSetRequest);
/**
*
* Create a configuration set. Configuration sets are groups of rules that you can apply to the emails that
* you send. You apply a configuration set to an email by specifying the name of the configuration set when you call
* the Amazon SES API v2. When you apply a configuration set to an email, all of the rules in that configuration set
* are applied to the email.
*
*
* @param createConfigurationSetRequest
* A request to create a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConfigurationSet operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateConfigurationSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createConfigurationSetAsync(CreateConfigurationSetRequest createConfigurationSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an event destination. Events include message sends, deliveries, opens, clicks, bounces, and
* complaints. Event destinations are places that you can send information about these events to. For
* example, you can send event data to Amazon SNS to receive notifications when you receive bounces or complaints,
* or you can use Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* A single configuration set can include more than one event destination.
*
*
* @param createConfigurationSetEventDestinationRequest
* A request to add an event destination to a configuration set.
* @return A Java Future containing the result of the CreateConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future createConfigurationSetEventDestinationAsync(
CreateConfigurationSetEventDestinationRequest createConfigurationSetEventDestinationRequest);
/**
*
* Create an event destination. Events include message sends, deliveries, opens, clicks, bounces, and
* complaints. Event destinations are places that you can send information about these events to. For
* example, you can send event data to Amazon SNS to receive notifications when you receive bounces or complaints,
* or you can use Amazon Kinesis Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* A single configuration set can include more than one event destination.
*
*
* @param createConfigurationSetEventDestinationRequest
* A request to add an event destination to a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future createConfigurationSetEventDestinationAsync(
CreateConfigurationSetEventDestinationRequest createConfigurationSetEventDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a contact, which is an end-user who is receiving the email, and adds them to a contact list.
*
*
* @param createContactRequest
* @return A Java Future containing the result of the CreateContact operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateContact
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createContactAsync(CreateContactRequest createContactRequest);
/**
*
* Creates a contact, which is an end-user who is receiving the email, and adds them to a contact list.
*
*
* @param createContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateContact operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateContact
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createContactAsync(CreateContactRequest createContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a contact list.
*
*
* @param createContactListRequest
* @return A Java Future containing the result of the CreateContactList operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateContactList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createContactListAsync(CreateContactListRequest createContactListRequest);
/**
*
* Creates a contact list.
*
*
* @param createContactListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateContactList operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateContactList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createContactListAsync(CreateContactListRequest createContactListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new custom verification email template.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createCustomVerificationEmailTemplateRequest
* Represents a request to create a custom verification email template.
* @return A Java Future containing the result of the CreateCustomVerificationEmailTemplate operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomVerificationEmailTemplateAsync(
CreateCustomVerificationEmailTemplateRequest createCustomVerificationEmailTemplateRequest);
/**
*
* Creates a new custom verification email template.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createCustomVerificationEmailTemplateRequest
* Represents a request to create a custom verification email template.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomVerificationEmailTemplate operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomVerificationEmailTemplateAsync(
CreateCustomVerificationEmailTemplateRequest createCustomVerificationEmailTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new pool of dedicated IP addresses. A pool can include one or more dedicated IP addresses that are
* associated with your Amazon Web Services account. You can associate a pool with a configuration set. When you
* send an email that uses that configuration set, the message is sent from one of the addresses in the associated
* pool.
*
*
* @param createDedicatedIpPoolRequest
* A request to create a new dedicated IP pool.
* @return A Java Future containing the result of the CreateDedicatedIpPool operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateDedicatedIpPool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDedicatedIpPoolAsync(CreateDedicatedIpPoolRequest createDedicatedIpPoolRequest);
/**
*
* Create a new pool of dedicated IP addresses. A pool can include one or more dedicated IP addresses that are
* associated with your Amazon Web Services account. You can associate a pool with a configuration set. When you
* send an email that uses that configuration set, the message is sent from one of the addresses in the associated
* pool.
*
*
* @param createDedicatedIpPoolRequest
* A request to create a new dedicated IP pool.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDedicatedIpPool operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateDedicatedIpPool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDedicatedIpPoolAsync(CreateDedicatedIpPoolRequest createDedicatedIpPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new predictive inbox placement test. Predictive inbox placement tests can help you predict how your
* messages will be handled by various email providers around the world. When you perform a predictive inbox
* placement test, you provide a sample message that contains the content that you plan to send to your customers.
* Amazon SES then sends that message to special email addresses spread across several major email providers. After
* about 24 hours, the test is complete, and you can use the GetDeliverabilityTestReport
operation to
* view the results of the test.
*
*
* @param createDeliverabilityTestReportRequest
* A request to perform a predictive inbox placement test. Predictive inbox placement tests can help you
* predict how your messages will be handled by various email providers around the world. When you perform a
* predictive inbox placement test, you provide a sample message that contains the content that you plan to
* send to your customers. We send that message to special email addresses spread across several major email
* providers around the world. The test takes about 24 hours to complete. When the test is complete, you can
* use the GetDeliverabilityTestReport
operation to view the results of the test.
* @return A Java Future containing the result of the CreateDeliverabilityTestReport operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.CreateDeliverabilityTestReport
* @see AWS API Documentation
*/
java.util.concurrent.Future createDeliverabilityTestReportAsync(
CreateDeliverabilityTestReportRequest createDeliverabilityTestReportRequest);
/**
*
* Create a new predictive inbox placement test. Predictive inbox placement tests can help you predict how your
* messages will be handled by various email providers around the world. When you perform a predictive inbox
* placement test, you provide a sample message that contains the content that you plan to send to your customers.
* Amazon SES then sends that message to special email addresses spread across several major email providers. After
* about 24 hours, the test is complete, and you can use the GetDeliverabilityTestReport
operation to
* view the results of the test.
*
*
* @param createDeliverabilityTestReportRequest
* A request to perform a predictive inbox placement test. Predictive inbox placement tests can help you
* predict how your messages will be handled by various email providers around the world. When you perform a
* predictive inbox placement test, you provide a sample message that contains the content that you plan to
* send to your customers. We send that message to special email addresses spread across several major email
* providers around the world. The test takes about 24 hours to complete. When the test is complete, you can
* use the GetDeliverabilityTestReport
operation to view the results of the test.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDeliverabilityTestReport operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateDeliverabilityTestReport
* @see AWS API Documentation
*/
java.util.concurrent.Future createDeliverabilityTestReportAsync(
CreateDeliverabilityTestReportRequest createDeliverabilityTestReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the process of verifying an email identity. An identity is an email address or domain that you use
* when you send email. Before you can use an identity to send email, you first have to verify it. By verifying an
* identity, you demonstrate that you're the owner of the identity, and that you've given Amazon SES API v2
* permission to send email from the identity.
*
*
* When you verify an email address, Amazon SES sends an email to the address. Your email address is verified as
* soon as you follow the link in the verification email.
*
*
* When you verify a domain without specifying the DkimSigningAttributes
object, this operation
* provides a set of DKIM tokens. You can convert these tokens into CNAME records, which you then add to the DNS
* configuration for your domain. Your domain is verified when Amazon SES detects these records in the DNS
* configuration for your domain. This verification method is known as Easy DKIM.
*
*
* Alternatively, you can perform the verification process by providing your own public-private key pair. This
* verification method is known as Bring Your Own DKIM (BYODKIM). To use BYODKIM, your call to the
* CreateEmailIdentity
operation has to include the DkimSigningAttributes
object. When you
* specify this object, you provide a selector (a component of the DNS record name that identifies the public key to
* use for DKIM authentication) and a private key.
*
*
* When you verify a domain, this operation provides a set of DKIM tokens, which you can convert into CNAME tokens.
* You add these CNAME tokens to the DNS configuration for your domain. Your domain is verified when Amazon SES
* detects these records in the DNS configuration for your domain. For some DNS providers, it can take 72 hours or
* more to complete the domain verification process.
*
*
* Additionally, you can associate an existing configuration set with the email identity that you're verifying.
*
*
* @param createEmailIdentityRequest
* A request to begin the verification process for an email identity (an email address or domain).
* @return A Java Future containing the result of the CreateEmailIdentity operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateEmailIdentity
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEmailIdentityAsync(CreateEmailIdentityRequest createEmailIdentityRequest);
/**
*
* Starts the process of verifying an email identity. An identity is an email address or domain that you use
* when you send email. Before you can use an identity to send email, you first have to verify it. By verifying an
* identity, you demonstrate that you're the owner of the identity, and that you've given Amazon SES API v2
* permission to send email from the identity.
*
*
* When you verify an email address, Amazon SES sends an email to the address. Your email address is verified as
* soon as you follow the link in the verification email.
*
*
* When you verify a domain without specifying the DkimSigningAttributes
object, this operation
* provides a set of DKIM tokens. You can convert these tokens into CNAME records, which you then add to the DNS
* configuration for your domain. Your domain is verified when Amazon SES detects these records in the DNS
* configuration for your domain. This verification method is known as Easy DKIM.
*
*
* Alternatively, you can perform the verification process by providing your own public-private key pair. This
* verification method is known as Bring Your Own DKIM (BYODKIM). To use BYODKIM, your call to the
* CreateEmailIdentity
operation has to include the DkimSigningAttributes
object. When you
* specify this object, you provide a selector (a component of the DNS record name that identifies the public key to
* use for DKIM authentication) and a private key.
*
*
* When you verify a domain, this operation provides a set of DKIM tokens, which you can convert into CNAME tokens.
* You add these CNAME tokens to the DNS configuration for your domain. Your domain is verified when Amazon SES
* detects these records in the DNS configuration for your domain. For some DNS providers, it can take 72 hours or
* more to complete the domain verification process.
*
*
* Additionally, you can associate an existing configuration set with the email identity that you're verifying.
*
*
* @param createEmailIdentityRequest
* A request to begin the verification process for an email identity (an email address or domain).
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEmailIdentity operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateEmailIdentity
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEmailIdentityAsync(CreateEmailIdentityRequest createEmailIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the specified sending authorization policy for the given identity (an email address or a domain).
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createEmailIdentityPolicyRequest
* Represents a request to create a sending authorization policy for an identity. Sending authorization is an
* Amazon SES feature that enables you to authorize other senders to use your identities. For information,
* see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the CreateEmailIdentityPolicy operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateEmailIdentityPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createEmailIdentityPolicyAsync(
CreateEmailIdentityPolicyRequest createEmailIdentityPolicyRequest);
/**
*
* Creates the specified sending authorization policy for the given identity (an email address or a domain).
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createEmailIdentityPolicyRequest
* Represents a request to create a sending authorization policy for an identity. Sending authorization is an
* Amazon SES feature that enables you to authorize other senders to use your identities. For information,
* see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEmailIdentityPolicy operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateEmailIdentityPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createEmailIdentityPolicyAsync(
CreateEmailIdentityPolicyRequest createEmailIdentityPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an email template. Email templates enable you to send personalized email to one or more destinations in a
* single API operation. For more information, see the Amazon SES
* Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createEmailTemplateRequest
* Represents a request to create an email template. For more information, see the Amazon SES
* Developer Guide.
* @return A Java Future containing the result of the CreateEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateEmailTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEmailTemplateAsync(CreateEmailTemplateRequest createEmailTemplateRequest);
/**
*
* Creates an email template. Email templates enable you to send personalized email to one or more destinations in a
* single API operation. For more information, see the Amazon SES
* Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createEmailTemplateRequest
* Represents a request to create an email template. For more information, see the Amazon SES
* Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateEmailTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEmailTemplateAsync(CreateEmailTemplateRequest createEmailTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an import job for a data destination.
*
*
* @param createImportJobRequest
* Represents a request to create an import job from a data source for a data destination.
* @return A Java Future containing the result of the CreateImportJob operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.CreateImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createImportJobAsync(CreateImportJobRequest createImportJobRequest);
/**
*
* Creates an import job for a data destination.
*
*
* @param createImportJobRequest
* Represents a request to create an import job from a data source for a data destination.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateImportJob operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.CreateImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createImportJobAsync(CreateImportJobRequest createImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an existing configuration set.
*
*
* Configuration sets are groups of rules that you can apply to the emails you send. You apply a
* configuration set to an email by including a reference to the configuration set in the headers of the email. When
* you apply a configuration set to an email, all of the rules in that configuration set are applied to the email.
*
*
* @param deleteConfigurationSetRequest
* A request to delete a configuration set.
* @return A Java Future containing the result of the DeleteConfigurationSet operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteConfigurationSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetAsync(DeleteConfigurationSetRequest deleteConfigurationSetRequest);
/**
*
* Delete an existing configuration set.
*
*
* Configuration sets are groups of rules that you can apply to the emails you send. You apply a
* configuration set to an email by including a reference to the configuration set in the headers of the email. When
* you apply a configuration set to an email, all of the rules in that configuration set are applied to the email.
*
*
* @param deleteConfigurationSetRequest
* A request to delete a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConfigurationSet operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteConfigurationSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetAsync(DeleteConfigurationSetRequest deleteConfigurationSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an event destination.
*
*
* Events include message sends, deliveries, opens, clicks, bounces, and complaints. Event
* destinations are places that you can send information about these events to. For example, you can send event
* data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use Amazon Kinesis
* Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param deleteConfigurationSetEventDestinationRequest
* A request to delete an event destination from a configuration set.
* @return A Java Future containing the result of the DeleteConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetEventDestinationAsync(
DeleteConfigurationSetEventDestinationRequest deleteConfigurationSetEventDestinationRequest);
/**
*
* Delete an event destination.
*
*
* Events include message sends, deliveries, opens, clicks, bounces, and complaints. Event
* destinations are places that you can send information about these events to. For example, you can send event
* data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use Amazon Kinesis
* Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param deleteConfigurationSetEventDestinationRequest
* A request to delete an event destination from a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetEventDestinationAsync(
DeleteConfigurationSetEventDestinationRequest deleteConfigurationSetEventDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a contact from a contact list.
*
*
* @param deleteContactRequest
* @return A Java Future containing the result of the DeleteContact operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteContact
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteContactAsync(DeleteContactRequest deleteContactRequest);
/**
*
* Removes a contact from a contact list.
*
*
* @param deleteContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteContact operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteContact
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteContactAsync(DeleteContactRequest deleteContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a contact list and all of the contacts on that list.
*
*
* @param deleteContactListRequest
* @return A Java Future containing the result of the DeleteContactList operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteContactList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteContactListAsync(DeleteContactListRequest deleteContactListRequest);
/**
*
* Deletes a contact list and all of the contacts on that list.
*
*
* @param deleteContactListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteContactList operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteContactList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteContactListAsync(DeleteContactListRequest deleteContactListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing custom verification email template.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteCustomVerificationEmailTemplateRequest
* Represents a request to delete an existing custom verification email template.
* @return A Java Future containing the result of the DeleteCustomVerificationEmailTemplate operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomVerificationEmailTemplateAsync(
DeleteCustomVerificationEmailTemplateRequest deleteCustomVerificationEmailTemplateRequest);
/**
*
* Deletes an existing custom verification email template.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteCustomVerificationEmailTemplateRequest
* Represents a request to delete an existing custom verification email template.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCustomVerificationEmailTemplate operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomVerificationEmailTemplateAsync(
DeleteCustomVerificationEmailTemplateRequest deleteCustomVerificationEmailTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a dedicated IP pool.
*
*
* @param deleteDedicatedIpPoolRequest
* A request to delete a dedicated IP pool.
* @return A Java Future containing the result of the DeleteDedicatedIpPool operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteDedicatedIpPool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDedicatedIpPoolAsync(DeleteDedicatedIpPoolRequest deleteDedicatedIpPoolRequest);
/**
*
* Delete a dedicated IP pool.
*
*
* @param deleteDedicatedIpPoolRequest
* A request to delete a dedicated IP pool.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDedicatedIpPool operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteDedicatedIpPool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDedicatedIpPoolAsync(DeleteDedicatedIpPoolRequest deleteDedicatedIpPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an email identity. An identity can be either an email address or a domain name.
*
*
* @param deleteEmailIdentityRequest
* A request to delete an existing email identity. When you delete an identity, you lose the ability to send
* email from that identity. You can restore your ability to send email by completing the verification
* process for the identity again.
* @return A Java Future containing the result of the DeleteEmailIdentity operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteEmailIdentity
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEmailIdentityAsync(DeleteEmailIdentityRequest deleteEmailIdentityRequest);
/**
*
* Deletes an email identity. An identity can be either an email address or a domain name.
*
*
* @param deleteEmailIdentityRequest
* A request to delete an existing email identity. When you delete an identity, you lose the ability to send
* email from that identity. You can restore your ability to send email by completing the verification
* process for the identity again.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEmailIdentity operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteEmailIdentity
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEmailIdentityAsync(DeleteEmailIdentityRequest deleteEmailIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified sending authorization policy for the given identity (an email address or a domain). This
* API returns successfully even if a policy with the specified name does not exist.
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteEmailIdentityPolicyRequest
* Represents a request to delete a sending authorization policy for an identity. Sending authorization is an
* Amazon SES feature that enables you to authorize other senders to use your identities. For information,
* see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the DeleteEmailIdentityPolicy operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteEmailIdentityPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEmailIdentityPolicyAsync(
DeleteEmailIdentityPolicyRequest deleteEmailIdentityPolicyRequest);
/**
*
* Deletes the specified sending authorization policy for the given identity (an email address or a domain). This
* API returns successfully even if a policy with the specified name does not exist.
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteEmailIdentityPolicyRequest
* Represents a request to delete a sending authorization policy for an identity. Sending authorization is an
* Amazon SES feature that enables you to authorize other senders to use your identities. For information,
* see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEmailIdentityPolicy operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteEmailIdentityPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEmailIdentityPolicyAsync(
DeleteEmailIdentityPolicyRequest deleteEmailIdentityPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an email template.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteEmailTemplateRequest
* Represents a request to delete an email template. For more information, see the Amazon SES
* Developer Guide.
* @return A Java Future containing the result of the DeleteEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteEmailTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEmailTemplateAsync(DeleteEmailTemplateRequest deleteEmailTemplateRequest);
/**
*
* Deletes an email template.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteEmailTemplateRequest
* Represents a request to delete an email template. For more information, see the Amazon SES
* Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteEmailTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEmailTemplateAsync(DeleteEmailTemplateRequest deleteEmailTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes an email address from the suppression list for your account.
*
*
* @param deleteSuppressedDestinationRequest
* A request to remove an email address from the suppression list for your account.
* @return A Java Future containing the result of the DeleteSuppressedDestination operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.DeleteSuppressedDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSuppressedDestinationAsync(
DeleteSuppressedDestinationRequest deleteSuppressedDestinationRequest);
/**
*
* Removes an email address from the suppression list for your account.
*
*
* @param deleteSuppressedDestinationRequest
* A request to remove an email address from the suppression list for your account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSuppressedDestination operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.DeleteSuppressedDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSuppressedDestinationAsync(
DeleteSuppressedDestinationRequest deleteSuppressedDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtain information about the email-sending status and capabilities of your Amazon SES account in the current
* Amazon Web Services Region.
*
*
* @param getAccountRequest
* A request to obtain information about the email-sending capabilities of your Amazon SES account.
* @return A Java Future containing the result of the GetAccount operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountAsync(GetAccountRequest getAccountRequest);
/**
*
* Obtain information about the email-sending status and capabilities of your Amazon SES account in the current
* Amazon Web Services Region.
*
*
* @param getAccountRequest
* A request to obtain information about the email-sending capabilities of your Amazon SES account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccount operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountAsync(GetAccountRequest getAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve a list of the blacklists that your dedicated IP addresses appear on.
*
*
* @param getBlacklistReportsRequest
* A request to retrieve a list of the blacklists that your dedicated IP addresses appear on.
* @return A Java Future containing the result of the GetBlacklistReports operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetBlacklistReports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBlacklistReportsAsync(GetBlacklistReportsRequest getBlacklistReportsRequest);
/**
*
* Retrieve a list of the blacklists that your dedicated IP addresses appear on.
*
*
* @param getBlacklistReportsRequest
* A request to retrieve a list of the blacklists that your dedicated IP addresses appear on.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBlacklistReports operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetBlacklistReports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBlacklistReportsAsync(GetBlacklistReportsRequest getBlacklistReportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get information about an existing configuration set, including the dedicated IP pool that it's associated with,
* whether or not it's enabled for sending email, and more.
*
*
* Configuration sets are groups of rules that you can apply to the emails you send. You apply a
* configuration set to an email by including a reference to the configuration set in the headers of the email. When
* you apply a configuration set to an email, all of the rules in that configuration set are applied to the email.
*
*
* @param getConfigurationSetRequest
* A request to obtain information about a configuration set.
* @return A Java Future containing the result of the GetConfigurationSet operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetConfigurationSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getConfigurationSetAsync(GetConfigurationSetRequest getConfigurationSetRequest);
/**
*
* Get information about an existing configuration set, including the dedicated IP pool that it's associated with,
* whether or not it's enabled for sending email, and more.
*
*
* Configuration sets are groups of rules that you can apply to the emails you send. You apply a
* configuration set to an email by including a reference to the configuration set in the headers of the email. When
* you apply a configuration set to an email, all of the rules in that configuration set are applied to the email.
*
*
* @param getConfigurationSetRequest
* A request to obtain information about a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetConfigurationSet operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetConfigurationSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getConfigurationSetAsync(GetConfigurationSetRequest getConfigurationSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve a list of event destinations that are associated with a configuration set.
*
*
* Events include message sends, deliveries, opens, clicks, bounces, and complaints. Event
* destinations are places that you can send information about these events to. For example, you can send event
* data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use Amazon Kinesis
* Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param getConfigurationSetEventDestinationsRequest
* A request to obtain information about the event destinations for a configuration set.
* @return A Java Future containing the result of the GetConfigurationSetEventDestinations operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.GetConfigurationSetEventDestinations
* @see AWS API Documentation
*/
java.util.concurrent.Future getConfigurationSetEventDestinationsAsync(
GetConfigurationSetEventDestinationsRequest getConfigurationSetEventDestinationsRequest);
/**
*
* Retrieve a list of event destinations that are associated with a configuration set.
*
*
* Events include message sends, deliveries, opens, clicks, bounces, and complaints. Event
* destinations are places that you can send information about these events to. For example, you can send event
* data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use Amazon Kinesis
* Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param getConfigurationSetEventDestinationsRequest
* A request to obtain information about the event destinations for a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetConfigurationSetEventDestinations operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetConfigurationSetEventDestinations
* @see AWS API Documentation
*/
java.util.concurrent.Future getConfigurationSetEventDestinationsAsync(
GetConfigurationSetEventDestinationsRequest getConfigurationSetEventDestinationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a contact from a contact list.
*
*
* @param getContactRequest
* @return A Java Future containing the result of the GetContact operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetContact
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getContactAsync(GetContactRequest getContactRequest);
/**
*
* Returns a contact from a contact list.
*
*
* @param getContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetContact operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetContact
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getContactAsync(GetContactRequest getContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns contact list metadata. It does not return any information about the contacts present in the list.
*
*
* @param getContactListRequest
* @return A Java Future containing the result of the GetContactList operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetContactList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getContactListAsync(GetContactListRequest getContactListRequest);
/**
*
* Returns contact list metadata. It does not return any information about the contacts present in the list.
*
*
* @param getContactListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetContactList operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetContactList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getContactListAsync(GetContactListRequest getContactListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the custom email verification template for the template name you specify.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getCustomVerificationEmailTemplateRequest
* Represents a request to retrieve an existing custom verification email template.
* @return A Java Future containing the result of the GetCustomVerificationEmailTemplate operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.GetCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getCustomVerificationEmailTemplateAsync(
GetCustomVerificationEmailTemplateRequest getCustomVerificationEmailTemplateRequest);
/**
*
* Returns the custom email verification template for the template name you specify.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getCustomVerificationEmailTemplateRequest
* Represents a request to retrieve an existing custom verification email template.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCustomVerificationEmailTemplate operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getCustomVerificationEmailTemplateAsync(
GetCustomVerificationEmailTemplateRequest getCustomVerificationEmailTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get information about a dedicated IP address, including the name of the dedicated IP pool that it's associated
* with, as well information about the automatic warm-up process for the address.
*
*
* @param getDedicatedIpRequest
* A request to obtain more information about a dedicated IP address.
* @return A Java Future containing the result of the GetDedicatedIp operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetDedicatedIp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDedicatedIpAsync(GetDedicatedIpRequest getDedicatedIpRequest);
/**
*
* Get information about a dedicated IP address, including the name of the dedicated IP pool that it's associated
* with, as well information about the automatic warm-up process for the address.
*
*
* @param getDedicatedIpRequest
* A request to obtain more information about a dedicated IP address.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDedicatedIp operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetDedicatedIp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDedicatedIpAsync(GetDedicatedIpRequest getDedicatedIpRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the dedicated IP addresses that are associated with your Amazon Web Services account.
*
*
* @param getDedicatedIpsRequest
* A request to obtain more information about dedicated IP pools.
* @return A Java Future containing the result of the GetDedicatedIps operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetDedicatedIps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDedicatedIpsAsync(GetDedicatedIpsRequest getDedicatedIpsRequest);
/**
*
* List the dedicated IP addresses that are associated with your Amazon Web Services account.
*
*
* @param getDedicatedIpsRequest
* A request to obtain more information about dedicated IP pools.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDedicatedIps operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetDedicatedIps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDedicatedIpsAsync(GetDedicatedIpsRequest getDedicatedIpsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve information about the status of the Deliverability dashboard for your account. When the Deliverability
* dashboard is enabled, you gain access to reputation, deliverability, and other metrics for the domains that you
* use to send email. You also gain the ability to perform predictive inbox placement tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon SES and other Amazon Web Services services. For more information about the
* features and cost of a Deliverability dashboard subscription, see Amazon SES Pricing.
*
*
* @param getDeliverabilityDashboardOptionsRequest
* Retrieve information about the status of the Deliverability dashboard for your Amazon Web Services
* account. When the Deliverability dashboard is enabled, you gain access to reputation, deliverability, and
* other metrics for your domains. You also gain the ability to perform predictive inbox placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon SES and other Amazon Web Services services. For more information
* about the features and cost of a Deliverability dashboard subscription, see Amazon Pinpoint Pricing.
* @return A Java Future containing the result of the GetDeliverabilityDashboardOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.GetDeliverabilityDashboardOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeliverabilityDashboardOptionsAsync(
GetDeliverabilityDashboardOptionsRequest getDeliverabilityDashboardOptionsRequest);
/**
*
* Retrieve information about the status of the Deliverability dashboard for your account. When the Deliverability
* dashboard is enabled, you gain access to reputation, deliverability, and other metrics for the domains that you
* use to send email. You also gain the ability to perform predictive inbox placement tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon SES and other Amazon Web Services services. For more information about the
* features and cost of a Deliverability dashboard subscription, see Amazon SES Pricing.
*
*
* @param getDeliverabilityDashboardOptionsRequest
* Retrieve information about the status of the Deliverability dashboard for your Amazon Web Services
* account. When the Deliverability dashboard is enabled, you gain access to reputation, deliverability, and
* other metrics for your domains. You also gain the ability to perform predictive inbox placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon SES and other Amazon Web Services services. For more information
* about the features and cost of a Deliverability dashboard subscription, see Amazon Pinpoint Pricing.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeliverabilityDashboardOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetDeliverabilityDashboardOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeliverabilityDashboardOptionsAsync(
GetDeliverabilityDashboardOptionsRequest getDeliverabilityDashboardOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve the results of a predictive inbox placement test.
*
*
* @param getDeliverabilityTestReportRequest
* A request to retrieve the results of a predictive inbox placement test.
* @return A Java Future containing the result of the GetDeliverabilityTestReport operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetDeliverabilityTestReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeliverabilityTestReportAsync(
GetDeliverabilityTestReportRequest getDeliverabilityTestReportRequest);
/**
*
* Retrieve the results of a predictive inbox placement test.
*
*
* @param getDeliverabilityTestReportRequest
* A request to retrieve the results of a predictive inbox placement test.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeliverabilityTestReport operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetDeliverabilityTestReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeliverabilityTestReportAsync(
GetDeliverabilityTestReportRequest getDeliverabilityTestReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only if the
* campaign sent email by using a domain that the Deliverability dashboard is enabled for.
*
*
* @param getDomainDeliverabilityCampaignRequest
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only
* if the campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
* @return A Java Future containing the result of the GetDomainDeliverabilityCampaign operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.GetDomainDeliverabilityCampaign
* @see AWS API Documentation
*/
java.util.concurrent.Future getDomainDeliverabilityCampaignAsync(
GetDomainDeliverabilityCampaignRequest getDomainDeliverabilityCampaignRequest);
/**
*
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only if the
* campaign sent email by using a domain that the Deliverability dashboard is enabled for.
*
*
* @param getDomainDeliverabilityCampaignRequest
* Retrieve all the deliverability data for a specific campaign. This data is available for a campaign only
* if the campaign sent email by using a domain that the Deliverability dashboard is enabled for (
* PutDeliverabilityDashboardOption
operation).
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDomainDeliverabilityCampaign operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetDomainDeliverabilityCampaign
* @see AWS API Documentation
*/
java.util.concurrent.Future getDomainDeliverabilityCampaignAsync(
GetDomainDeliverabilityCampaignRequest getDomainDeliverabilityCampaignRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve inbox placement and engagement rates for the domains that you use to send email.
*
*
* @param getDomainStatisticsReportRequest
* A request to obtain deliverability metrics for a domain.
* @return A Java Future containing the result of the GetDomainStatisticsReport operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetDomainStatisticsReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getDomainStatisticsReportAsync(
GetDomainStatisticsReportRequest getDomainStatisticsReportRequest);
/**
*
* Retrieve inbox placement and engagement rates for the domains that you use to send email.
*
*
* @param getDomainStatisticsReportRequest
* A request to obtain deliverability metrics for a domain.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDomainStatisticsReport operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetDomainStatisticsReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getDomainStatisticsReportAsync(
GetDomainStatisticsReportRequest getDomainStatisticsReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about a specific identity, including the identity's verification status, sending
* authorization policies, its DKIM authentication status, and its custom Mail-From settings.
*
*
* @param getEmailIdentityRequest
* A request to return details about an email identity.
* @return A Java Future containing the result of the GetEmailIdentity operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetEmailIdentity
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEmailIdentityAsync(GetEmailIdentityRequest getEmailIdentityRequest);
/**
*
* Provides information about a specific identity, including the identity's verification status, sending
* authorization policies, its DKIM authentication status, and its custom Mail-From settings.
*
*
* @param getEmailIdentityRequest
* A request to return details about an email identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEmailIdentity operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetEmailIdentity
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEmailIdentityAsync(GetEmailIdentityRequest getEmailIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the requested sending authorization policies for the given identity (an email address or a domain). The
* policies are returned as a map of policy names to policy contents. You can retrieve a maximum of 20 policies at a
* time.
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getEmailIdentityPoliciesRequest
* A request to return the policies of an email identity.
* @return A Java Future containing the result of the GetEmailIdentityPolicies operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetEmailIdentityPolicies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEmailIdentityPoliciesAsync(GetEmailIdentityPoliciesRequest getEmailIdentityPoliciesRequest);
/**
*
* Returns the requested sending authorization policies for the given identity (an email address or a domain). The
* policies are returned as a map of policy names to policy contents. You can retrieve a maximum of 20 policies at a
* time.
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getEmailIdentityPoliciesRequest
* A request to return the policies of an email identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEmailIdentityPolicies operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetEmailIdentityPolicies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEmailIdentityPoliciesAsync(GetEmailIdentityPoliciesRequest getEmailIdentityPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays the template object (which includes the subject line, HTML part and text part) for the template you
* specify.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getEmailTemplateRequest
* Represents a request to display the template object (which includes the subject line, HTML part and text
* part) for the template you specify.
* @return A Java Future containing the result of the GetEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetEmailTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEmailTemplateAsync(GetEmailTemplateRequest getEmailTemplateRequest);
/**
*
* Displays the template object (which includes the subject line, HTML part and text part) for the template you
* specify.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getEmailTemplateRequest
* Represents a request to display the template object (which includes the subject line, HTML part and text
* part) for the template you specify.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetEmailTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEmailTemplateAsync(GetEmailTemplateRequest getEmailTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about an import job.
*
*
* @param getImportJobRequest
* Represents a request for information about an import job using the import job ID.
* @return A Java Future containing the result of the GetImportJob operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getImportJobAsync(GetImportJobRequest getImportJobRequest);
/**
*
* Provides information about an import job.
*
*
* @param getImportJobRequest
* Represents a request for information about an import job using the import job ID.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetImportJob operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getImportJobAsync(GetImportJobRequest getImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about a specific email address that's on the suppression list for your account.
*
*
* @param getSuppressedDestinationRequest
* A request to retrieve information about an email address that's on the suppression list for your account.
* @return A Java Future containing the result of the GetSuppressedDestination operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.GetSuppressedDestination
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSuppressedDestinationAsync(GetSuppressedDestinationRequest getSuppressedDestinationRequest);
/**
*
* Retrieves information about a specific email address that's on the suppression list for your account.
*
*
* @param getSuppressedDestinationRequest
* A request to retrieve information about an email address that's on the suppression list for your account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSuppressedDestination operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.GetSuppressedDestination
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSuppressedDestinationAsync(GetSuppressedDestinationRequest getSuppressedDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all of the configuration sets associated with your account in the current region.
*
*
* Configuration sets are groups of rules that you can apply to the emails you send. You apply a
* configuration set to an email by including a reference to the configuration set in the headers of the email. When
* you apply a configuration set to an email, all of the rules in that configuration set are applied to the email.
*
*
* @param listConfigurationSetsRequest
* A request to obtain a list of configuration sets for your Amazon SES account in the current Amazon Web
* Services Region.
* @return A Java Future containing the result of the ListConfigurationSets operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.ListConfigurationSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listConfigurationSetsAsync(ListConfigurationSetsRequest listConfigurationSetsRequest);
/**
*
* List all of the configuration sets associated with your account in the current region.
*
*
* Configuration sets are groups of rules that you can apply to the emails you send. You apply a
* configuration set to an email by including a reference to the configuration set in the headers of the email. When
* you apply a configuration set to an email, all of the rules in that configuration set are applied to the email.
*
*
* @param listConfigurationSetsRequest
* A request to obtain a list of configuration sets for your Amazon SES account in the current Amazon Web
* Services Region.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListConfigurationSets operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListConfigurationSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listConfigurationSetsAsync(ListConfigurationSetsRequest listConfigurationSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the contact lists available.
*
*
* @param listContactListsRequest
* @return A Java Future containing the result of the ListContactLists operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.ListContactLists
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContactListsAsync(ListContactListsRequest listContactListsRequest);
/**
*
* Lists all of the contact lists available.
*
*
* @param listContactListsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListContactLists operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListContactLists
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContactListsAsync(ListContactListsRequest listContactListsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the contacts present in a specific contact list.
*
*
* @param listContactsRequest
* @return A Java Future containing the result of the ListContacts operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.ListContacts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContactsAsync(ListContactsRequest listContactsRequest);
/**
*
* Lists the contacts present in a specific contact list.
*
*
* @param listContactsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListContacts operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListContacts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContactsAsync(ListContactsRequest listContactsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the existing custom verification email templates for your account in the current Amazon Web Services
* Region.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param listCustomVerificationEmailTemplatesRequest
* Represents a request to list the existing custom verification email templates for your account.
* @return A Java Future containing the result of the ListCustomVerificationEmailTemplates operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.ListCustomVerificationEmailTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future listCustomVerificationEmailTemplatesAsync(
ListCustomVerificationEmailTemplatesRequest listCustomVerificationEmailTemplatesRequest);
/**
*
* Lists the existing custom verification email templates for your account in the current Amazon Web Services
* Region.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param listCustomVerificationEmailTemplatesRequest
* Represents a request to list the existing custom verification email templates for your account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCustomVerificationEmailTemplates operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListCustomVerificationEmailTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future listCustomVerificationEmailTemplatesAsync(
ListCustomVerificationEmailTemplatesRequest listCustomVerificationEmailTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all of the dedicated IP pools that exist in your Amazon Web Services account in the current Region.
*
*
* @param listDedicatedIpPoolsRequest
* A request to obtain a list of dedicated IP pools.
* @return A Java Future containing the result of the ListDedicatedIpPools operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.ListDedicatedIpPools
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDedicatedIpPoolsAsync(ListDedicatedIpPoolsRequest listDedicatedIpPoolsRequest);
/**
*
* List all of the dedicated IP pools that exist in your Amazon Web Services account in the current Region.
*
*
* @param listDedicatedIpPoolsRequest
* A request to obtain a list of dedicated IP pools.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDedicatedIpPools operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListDedicatedIpPools
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDedicatedIpPoolsAsync(ListDedicatedIpPoolsRequest listDedicatedIpPoolsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Show a list of the predictive inbox placement tests that you've performed, regardless of their statuses. For
* predictive inbox placement tests that are complete, you can use the GetDeliverabilityTestReport
* operation to view the results.
*
*
* @param listDeliverabilityTestReportsRequest
* A request to list all of the predictive inbox placement tests that you've performed.
* @return A Java Future containing the result of the ListDeliverabilityTestReports operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.ListDeliverabilityTestReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeliverabilityTestReportsAsync(
ListDeliverabilityTestReportsRequest listDeliverabilityTestReportsRequest);
/**
*
* Show a list of the predictive inbox placement tests that you've performed, regardless of their statuses. For
* predictive inbox placement tests that are complete, you can use the GetDeliverabilityTestReport
* operation to view the results.
*
*
* @param listDeliverabilityTestReportsRequest
* A request to list all of the predictive inbox placement tests that you've performed.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDeliverabilityTestReports operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListDeliverabilityTestReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listDeliverabilityTestReportsAsync(
ListDeliverabilityTestReportsRequest listDeliverabilityTestReportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a specified
* time range. This data is available for a domain only if you enabled the Deliverability dashboard for the domain.
*
*
* @param listDomainDeliverabilityCampaignsRequest
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a
* specified time range. This data is available for a domain only if you enabled the Deliverability
* dashboard.
* @return A Java Future containing the result of the ListDomainDeliverabilityCampaigns operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.ListDomainDeliverabilityCampaigns
* @see AWS API Documentation
*/
java.util.concurrent.Future listDomainDeliverabilityCampaignsAsync(
ListDomainDeliverabilityCampaignsRequest listDomainDeliverabilityCampaignsRequest);
/**
*
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a specified
* time range. This data is available for a domain only if you enabled the Deliverability dashboard for the domain.
*
*
* @param listDomainDeliverabilityCampaignsRequest
* Retrieve deliverability data for all the campaigns that used a specific domain to send email during a
* specified time range. This data is available for a domain only if you enabled the Deliverability
* dashboard.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDomainDeliverabilityCampaigns operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListDomainDeliverabilityCampaigns
* @see AWS API Documentation
*/
java.util.concurrent.Future listDomainDeliverabilityCampaignsAsync(
ListDomainDeliverabilityCampaignsRequest listDomainDeliverabilityCampaignsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all of the email identities that are associated with your Amazon Web Services account. An
* identity can be either an email address or a domain. This operation returns identities that are verified as well
* as those that aren't. This operation returns identities that are associated with Amazon SES and Amazon Pinpoint.
*
*
* @param listEmailIdentitiesRequest
* A request to list all of the email identities associated with your Amazon Web Services account. This list
* includes identities that you've already verified, identities that are unverified, and identities that were
* verified in the past, but are no longer verified.
* @return A Java Future containing the result of the ListEmailIdentities operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.ListEmailIdentities
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEmailIdentitiesAsync(ListEmailIdentitiesRequest listEmailIdentitiesRequest);
/**
*
* Returns a list of all of the email identities that are associated with your Amazon Web Services account. An
* identity can be either an email address or a domain. This operation returns identities that are verified as well
* as those that aren't. This operation returns identities that are associated with Amazon SES and Amazon Pinpoint.
*
*
* @param listEmailIdentitiesRequest
* A request to list all of the email identities associated with your Amazon Web Services account. This list
* includes identities that you've already verified, identities that are unverified, and identities that were
* verified in the past, but are no longer verified.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEmailIdentities operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListEmailIdentities
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEmailIdentitiesAsync(ListEmailIdentitiesRequest listEmailIdentitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the email templates present in your Amazon SES account in the current Amazon Web Services Region.
*
*
* You can execute this operation no more than once per second.
*
*
* @param listEmailTemplatesRequest
* Represents a request to list the email templates present in your Amazon SES account in the current Amazon
* Web Services Region. For more information, see the Amazon SES
* Developer Guide.
* @return A Java Future containing the result of the ListEmailTemplates operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.ListEmailTemplates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEmailTemplatesAsync(ListEmailTemplatesRequest listEmailTemplatesRequest);
/**
*
* Lists the email templates present in your Amazon SES account in the current Amazon Web Services Region.
*
*
* You can execute this operation no more than once per second.
*
*
* @param listEmailTemplatesRequest
* Represents a request to list the email templates present in your Amazon SES account in the current Amazon
* Web Services Region. For more information, see the Amazon SES
* Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEmailTemplates operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListEmailTemplates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEmailTemplatesAsync(ListEmailTemplatesRequest listEmailTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the import jobs.
*
*
* @param listImportJobsRequest
* Represents a request to list all of the import jobs for a data destination within the specified maximum
* number of import jobs.
* @return A Java Future containing the result of the ListImportJobs operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.ListImportJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportJobsAsync(ListImportJobsRequest listImportJobsRequest);
/**
*
* Lists all of the import jobs.
*
*
* @param listImportJobsRequest
* Represents a request to list all of the import jobs for a data destination within the specified maximum
* number of import jobs.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListImportJobs operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListImportJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportJobsAsync(ListImportJobsRequest listImportJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of email addresses that are on the suppression list for your account.
*
*
* @param listSuppressedDestinationsRequest
* A request to obtain a list of email destinations that are on the suppression list for your account.
* @return A Java Future containing the result of the ListSuppressedDestinations operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.ListSuppressedDestinations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSuppressedDestinationsAsync(
ListSuppressedDestinationsRequest listSuppressedDestinationsRequest);
/**
*
* Retrieves a list of email addresses that are on the suppression list for your account.
*
*
* @param listSuppressedDestinationsRequest
* A request to obtain a list of email destinations that are on the suppression list for your account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSuppressedDestinations operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListSuppressedDestinations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSuppressedDestinationsAsync(
ListSuppressedDestinationsRequest listSuppressedDestinationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve a list of the tags (keys and values) that are associated with a specified resource. A tag is a
* label that you optionally define and associate with a resource. Each tag consists of a required tag
* key and an optional associated tag value. A tag key is a general label that acts as a category for
* more specific tag values. A tag value acts as a descriptor within a tag key.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieve a list of the tags (keys and values) that are associated with a specified resource. A tag is a
* label that you optionally define and associate with a resource. Each tag consists of a required tag
* key and an optional associated tag value. A tag key is a general label that acts as a category for
* more specific tag values. A tag value acts as a descriptor within a tag key.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable the automatic warm-up feature for dedicated IP addresses.
*
*
* @param putAccountDedicatedIpWarmupAttributesRequest
* A request to enable or disable the automatic IP address warm-up feature.
* @return A Java Future containing the result of the PutAccountDedicatedIpWarmupAttributes operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2Async.PutAccountDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountDedicatedIpWarmupAttributesAsync(
PutAccountDedicatedIpWarmupAttributesRequest putAccountDedicatedIpWarmupAttributesRequest);
/**
*
* Enable or disable the automatic warm-up feature for dedicated IP addresses.
*
*
* @param putAccountDedicatedIpWarmupAttributesRequest
* A request to enable or disable the automatic IP address warm-up feature.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAccountDedicatedIpWarmupAttributes operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutAccountDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountDedicatedIpWarmupAttributesAsync(
PutAccountDedicatedIpWarmupAttributesRequest putAccountDedicatedIpWarmupAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update your Amazon SES account details.
*
*
* @param putAccountDetailsRequest
* A request to submit new account details.
* @return A Java Future containing the result of the PutAccountDetails operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.PutAccountDetails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putAccountDetailsAsync(PutAccountDetailsRequest putAccountDetailsRequest);
/**
*
* Update your Amazon SES account details.
*
*
* @param putAccountDetailsRequest
* A request to submit new account details.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAccountDetails operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutAccountDetails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putAccountDetailsAsync(PutAccountDetailsRequest putAccountDetailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable the ability of your account to send email.
*
*
* @param putAccountSendingAttributesRequest
* A request to change the ability of your account to send email.
* @return A Java Future containing the result of the PutAccountSendingAttributes operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.PutAccountSendingAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountSendingAttributesAsync(
PutAccountSendingAttributesRequest putAccountSendingAttributesRequest);
/**
*
* Enable or disable the ability of your account to send email.
*
*
* @param putAccountSendingAttributesRequest
* A request to change the ability of your account to send email.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAccountSendingAttributes operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutAccountSendingAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountSendingAttributesAsync(
PutAccountSendingAttributesRequest putAccountSendingAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Change the settings for the account-level suppression list.
*
*
* @param putAccountSuppressionAttributesRequest
* A request to change your account's suppression preferences.
* @return A Java Future containing the result of the PutAccountSuppressionAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutAccountSuppressionAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountSuppressionAttributesAsync(
PutAccountSuppressionAttributesRequest putAccountSuppressionAttributesRequest);
/**
*
* Change the settings for the account-level suppression list.
*
*
* @param putAccountSuppressionAttributesRequest
* A request to change your account's suppression preferences.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAccountSuppressionAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutAccountSuppressionAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putAccountSuppressionAttributesAsync(
PutAccountSuppressionAttributesRequest putAccountSuppressionAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate a configuration set with a dedicated IP pool. You can use dedicated IP pools to create groups of
* dedicated IP addresses for sending specific types of email.
*
*
* @param putConfigurationSetDeliveryOptionsRequest
* A request to associate a configuration set with a dedicated IP pool.
* @return A Java Future containing the result of the PutConfigurationSetDeliveryOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutConfigurationSetDeliveryOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetDeliveryOptionsAsync(
PutConfigurationSetDeliveryOptionsRequest putConfigurationSetDeliveryOptionsRequest);
/**
*
* Associate a configuration set with a dedicated IP pool. You can use dedicated IP pools to create groups of
* dedicated IP addresses for sending specific types of email.
*
*
* @param putConfigurationSetDeliveryOptionsRequest
* A request to associate a configuration set with a dedicated IP pool.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfigurationSetDeliveryOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutConfigurationSetDeliveryOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetDeliveryOptionsAsync(
PutConfigurationSetDeliveryOptionsRequest putConfigurationSetDeliveryOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable collection of reputation metrics for emails that you send using a particular configuration set
* in a specific Amazon Web Services Region.
*
*
* @param putConfigurationSetReputationOptionsRequest
* A request to enable or disable tracking of reputation metrics for a configuration set.
* @return A Java Future containing the result of the PutConfigurationSetReputationOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutConfigurationSetReputationOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetReputationOptionsAsync(
PutConfigurationSetReputationOptionsRequest putConfigurationSetReputationOptionsRequest);
/**
*
* Enable or disable collection of reputation metrics for emails that you send using a particular configuration set
* in a specific Amazon Web Services Region.
*
*
* @param putConfigurationSetReputationOptionsRequest
* A request to enable or disable tracking of reputation metrics for a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfigurationSetReputationOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutConfigurationSetReputationOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetReputationOptionsAsync(
PutConfigurationSetReputationOptionsRequest putConfigurationSetReputationOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable email sending for messages that use a particular configuration set in a specific Amazon Web
* Services Region.
*
*
* @param putConfigurationSetSendingOptionsRequest
* A request to enable or disable the ability of Amazon SES to send emails that use a specific configuration
* set.
* @return A Java Future containing the result of the PutConfigurationSetSendingOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutConfigurationSetSendingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetSendingOptionsAsync(
PutConfigurationSetSendingOptionsRequest putConfigurationSetSendingOptionsRequest);
/**
*
* Enable or disable email sending for messages that use a particular configuration set in a specific Amazon Web
* Services Region.
*
*
* @param putConfigurationSetSendingOptionsRequest
* A request to enable or disable the ability of Amazon SES to send emails that use a specific configuration
* set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfigurationSetSendingOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutConfigurationSetSendingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetSendingOptionsAsync(
PutConfigurationSetSendingOptionsRequest putConfigurationSetSendingOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specify the account suppression list preferences for a configuration set.
*
*
* @param putConfigurationSetSuppressionOptionsRequest
* A request to change the account suppression list preferences for a specific configuration set.
* @return A Java Future containing the result of the PutConfigurationSetSuppressionOptions operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2Async.PutConfigurationSetSuppressionOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetSuppressionOptionsAsync(
PutConfigurationSetSuppressionOptionsRequest putConfigurationSetSuppressionOptionsRequest);
/**
*
* Specify the account suppression list preferences for a configuration set.
*
*
* @param putConfigurationSetSuppressionOptionsRequest
* A request to change the account suppression list preferences for a specific configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfigurationSetSuppressionOptions operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutConfigurationSetSuppressionOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetSuppressionOptionsAsync(
PutConfigurationSetSuppressionOptionsRequest putConfigurationSetSuppressionOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specify a custom domain to use for open and click tracking elements in email that you send.
*
*
* @param putConfigurationSetTrackingOptionsRequest
* A request to add a custom domain for tracking open and click events to a configuration set.
* @return A Java Future containing the result of the PutConfigurationSetTrackingOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutConfigurationSetTrackingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetTrackingOptionsAsync(
PutConfigurationSetTrackingOptionsRequest putConfigurationSetTrackingOptionsRequest);
/**
*
* Specify a custom domain to use for open and click tracking elements in email that you send.
*
*
* @param putConfigurationSetTrackingOptionsRequest
* A request to add a custom domain for tracking open and click events to a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfigurationSetTrackingOptions operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutConfigurationSetTrackingOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationSetTrackingOptionsAsync(
PutConfigurationSetTrackingOptionsRequest putConfigurationSetTrackingOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Move a dedicated IP address to an existing dedicated IP pool.
*
*
*
* The dedicated IP address that you specify must already exist, and must be associated with your Amazon Web
* Services account.
*
*
* The dedicated IP pool you specify must already exist. You can create a new pool by using the
* CreateDedicatedIpPool
operation.
*
*
*
* @param putDedicatedIpInPoolRequest
* A request to move a dedicated IP address to a dedicated IP pool.
* @return A Java Future containing the result of the PutDedicatedIpInPool operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.PutDedicatedIpInPool
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putDedicatedIpInPoolAsync(PutDedicatedIpInPoolRequest putDedicatedIpInPoolRequest);
/**
*
* Move a dedicated IP address to an existing dedicated IP pool.
*
*
*
* The dedicated IP address that you specify must already exist, and must be associated with your Amazon Web
* Services account.
*
*
* The dedicated IP pool you specify must already exist. You can create a new pool by using the
* CreateDedicatedIpPool
operation.
*
*
*
* @param putDedicatedIpInPoolRequest
* A request to move a dedicated IP address to a dedicated IP pool.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutDedicatedIpInPool operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutDedicatedIpInPool
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putDedicatedIpInPoolAsync(PutDedicatedIpInPoolRequest putDedicatedIpInPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* @param putDedicatedIpWarmupAttributesRequest
* A request to change the warm-up attributes for a dedicated IP address. This operation is useful when you
* want to resume the warm-up process for an existing IP address.
* @return A Java Future containing the result of the PutDedicatedIpWarmupAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putDedicatedIpWarmupAttributesAsync(
PutDedicatedIpWarmupAttributesRequest putDedicatedIpWarmupAttributesRequest);
/**
*
*
* @param putDedicatedIpWarmupAttributesRequest
* A request to change the warm-up attributes for a dedicated IP address. This operation is useful when you
* want to resume the warm-up process for an existing IP address.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutDedicatedIpWarmupAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutDedicatedIpWarmupAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putDedicatedIpWarmupAttributesAsync(
PutDedicatedIpWarmupAttributesRequest putDedicatedIpWarmupAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enable or disable the Deliverability dashboard. When you enable the Deliverability dashboard, you gain access to
* reputation, deliverability, and other metrics for the domains that you use to send email. You also gain the
* ability to perform predictive inbox placement tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon SES and other Amazon Web Services services. For more information about the
* features and cost of a Deliverability dashboard subscription, see Amazon SES Pricing.
*
*
* @param putDeliverabilityDashboardOptionRequest
* Enable or disable the Deliverability dashboard. When you enable the Deliverability dashboard, you gain
* access to reputation, deliverability, and other metrics for the domains that you use to send email using
* Amazon SES API v2. You also gain the ability to perform predictive inbox placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon SES and other Amazon Web Services services. For more information
* about the features and cost of a Deliverability dashboard subscription, see Amazon Pinpoint Pricing.
* @return A Java Future containing the result of the PutDeliverabilityDashboardOption operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutDeliverabilityDashboardOption
* @see AWS API Documentation
*/
java.util.concurrent.Future putDeliverabilityDashboardOptionAsync(
PutDeliverabilityDashboardOptionRequest putDeliverabilityDashboardOptionRequest);
/**
*
* Enable or disable the Deliverability dashboard. When you enable the Deliverability dashboard, you gain access to
* reputation, deliverability, and other metrics for the domains that you use to send email. You also gain the
* ability to perform predictive inbox placement tests.
*
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other fees
* that you accrue by using Amazon SES and other Amazon Web Services services. For more information about the
* features and cost of a Deliverability dashboard subscription, see Amazon SES Pricing.
*
*
* @param putDeliverabilityDashboardOptionRequest
* Enable or disable the Deliverability dashboard. When you enable the Deliverability dashboard, you gain
* access to reputation, deliverability, and other metrics for the domains that you use to send email using
* Amazon SES API v2. You also gain the ability to perform predictive inbox placement tests.
*
* When you use the Deliverability dashboard, you pay a monthly subscription charge, in addition to any other
* fees that you accrue by using Amazon SES and other Amazon Web Services services. For more information
* about the features and cost of a Deliverability dashboard subscription, see Amazon Pinpoint Pricing.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutDeliverabilityDashboardOption operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutDeliverabilityDashboardOption
* @see AWS API Documentation
*/
java.util.concurrent.Future putDeliverabilityDashboardOptionAsync(
PutDeliverabilityDashboardOptionRequest putDeliverabilityDashboardOptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to associate a configuration set with an email identity.
*
*
* @param putEmailIdentityConfigurationSetAttributesRequest
* A request to associate a configuration set with an email identity.
* @return A Java Future containing the result of the PutEmailIdentityConfigurationSetAttributes operation returned
* by the service.
* @sample AmazonSimpleEmailServiceV2Async.PutEmailIdentityConfigurationSetAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityConfigurationSetAttributesAsync(
PutEmailIdentityConfigurationSetAttributesRequest putEmailIdentityConfigurationSetAttributesRequest);
/**
*
* Used to associate a configuration set with an email identity.
*
*
* @param putEmailIdentityConfigurationSetAttributesRequest
* A request to associate a configuration set with an email identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEmailIdentityConfigurationSetAttributes operation returned
* by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutEmailIdentityConfigurationSetAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityConfigurationSetAttributesAsync(
PutEmailIdentityConfigurationSetAttributesRequest putEmailIdentityConfigurationSetAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to enable or disable DKIM authentication for an email identity.
*
*
* @param putEmailIdentityDkimAttributesRequest
* A request to enable or disable DKIM signing of email that you send from an email identity.
* @return A Java Future containing the result of the PutEmailIdentityDkimAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutEmailIdentityDkimAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityDkimAttributesAsync(
PutEmailIdentityDkimAttributesRequest putEmailIdentityDkimAttributesRequest);
/**
*
* Used to enable or disable DKIM authentication for an email identity.
*
*
* @param putEmailIdentityDkimAttributesRequest
* A request to enable or disable DKIM signing of email that you send from an email identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEmailIdentityDkimAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutEmailIdentityDkimAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityDkimAttributesAsync(
PutEmailIdentityDkimAttributesRequest putEmailIdentityDkimAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to configure or change the DKIM authentication settings for an email domain identity. You can use this
* operation to do any of the following:
*
*
* -
*
* Update the signing attributes for an identity that uses Bring Your Own DKIM (BYODKIM).
*
*
* -
*
* Update the key length that should be used for Easy DKIM.
*
*
* -
*
* Change from using no DKIM authentication to using Easy DKIM.
*
*
* -
*
* Change from using no DKIM authentication to using BYODKIM.
*
*
* -
*
* Change from using Easy DKIM to using BYODKIM.
*
*
* -
*
* Change from using BYODKIM to using Easy DKIM.
*
*
*
*
* @param putEmailIdentityDkimSigningAttributesRequest
* A request to change the DKIM attributes for an email identity.
* @return A Java Future containing the result of the PutEmailIdentityDkimSigningAttributes operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2Async.PutEmailIdentityDkimSigningAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityDkimSigningAttributesAsync(
PutEmailIdentityDkimSigningAttributesRequest putEmailIdentityDkimSigningAttributesRequest);
/**
*
* Used to configure or change the DKIM authentication settings for an email domain identity. You can use this
* operation to do any of the following:
*
*
* -
*
* Update the signing attributes for an identity that uses Bring Your Own DKIM (BYODKIM).
*
*
* -
*
* Update the key length that should be used for Easy DKIM.
*
*
* -
*
* Change from using no DKIM authentication to using Easy DKIM.
*
*
* -
*
* Change from using no DKIM authentication to using BYODKIM.
*
*
* -
*
* Change from using Easy DKIM to using BYODKIM.
*
*
* -
*
* Change from using BYODKIM to using Easy DKIM.
*
*
*
*
* @param putEmailIdentityDkimSigningAttributesRequest
* A request to change the DKIM attributes for an email identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEmailIdentityDkimSigningAttributes operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutEmailIdentityDkimSigningAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityDkimSigningAttributesAsync(
PutEmailIdentityDkimSigningAttributesRequest putEmailIdentityDkimSigningAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to enable or disable feedback forwarding for an identity. This setting determines what happens when an
* identity is used to send an email that results in a bounce or complaint event.
*
*
* If the value is true
, you receive email notifications when bounce or complaint events occur. These
* notifications are sent to the address that you specified in the Return-Path
header of the original
* email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another mechanism for
* receiving bounce or complaint notifications (for example, by setting up an event destination), you receive an
* email notification when these events occur (even if this setting is disabled).
*
*
* @param putEmailIdentityFeedbackAttributesRequest
* A request to set the attributes that control how bounce and complaint events are processed.
* @return A Java Future containing the result of the PutEmailIdentityFeedbackAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutEmailIdentityFeedbackAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityFeedbackAttributesAsync(
PutEmailIdentityFeedbackAttributesRequest putEmailIdentityFeedbackAttributesRequest);
/**
*
* Used to enable or disable feedback forwarding for an identity. This setting determines what happens when an
* identity is used to send an email that results in a bounce or complaint event.
*
*
* If the value is true
, you receive email notifications when bounce or complaint events occur. These
* notifications are sent to the address that you specified in the Return-Path
header of the original
* email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another mechanism for
* receiving bounce or complaint notifications (for example, by setting up an event destination), you receive an
* email notification when these events occur (even if this setting is disabled).
*
*
* @param putEmailIdentityFeedbackAttributesRequest
* A request to set the attributes that control how bounce and complaint events are processed.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEmailIdentityFeedbackAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutEmailIdentityFeedbackAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityFeedbackAttributesAsync(
PutEmailIdentityFeedbackAttributesRequest putEmailIdentityFeedbackAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to enable or disable the custom Mail-From domain configuration for an email identity.
*
*
* @param putEmailIdentityMailFromAttributesRequest
* A request to configure the custom MAIL FROM domain for a verified identity.
* @return A Java Future containing the result of the PutEmailIdentityMailFromAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2Async.PutEmailIdentityMailFromAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityMailFromAttributesAsync(
PutEmailIdentityMailFromAttributesRequest putEmailIdentityMailFromAttributesRequest);
/**
*
* Used to enable or disable the custom Mail-From domain configuration for an email identity.
*
*
* @param putEmailIdentityMailFromAttributesRequest
* A request to configure the custom MAIL FROM domain for a verified identity.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEmailIdentityMailFromAttributes operation returned by the
* service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutEmailIdentityMailFromAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future putEmailIdentityMailFromAttributesAsync(
PutEmailIdentityMailFromAttributesRequest putEmailIdentityMailFromAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an email address to the suppression list for your account.
*
*
* @param putSuppressedDestinationRequest
* A request to add an email destination to the suppression list for your account.
* @return A Java Future containing the result of the PutSuppressedDestination operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.PutSuppressedDestination
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putSuppressedDestinationAsync(PutSuppressedDestinationRequest putSuppressedDestinationRequest);
/**
*
* Adds an email address to the suppression list for your account.
*
*
* @param putSuppressedDestinationRequest
* A request to add an email destination to the suppression list for your account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutSuppressedDestination operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.PutSuppressedDestination
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putSuppressedDestinationAsync(PutSuppressedDestinationRequest putSuppressedDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Composes an email message to multiple destinations.
*
*
* @param sendBulkEmailRequest
* Represents a request to send email messages to multiple destinations using Amazon SES. For more
* information, see the Amazon SES
* Developer Guide.
* @return A Java Future containing the result of the SendBulkEmail operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.SendBulkEmail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future sendBulkEmailAsync(SendBulkEmailRequest sendBulkEmailRequest);
/**
*
* Composes an email message to multiple destinations.
*
*
* @param sendBulkEmailRequest
* Represents a request to send email messages to multiple destinations using Amazon SES. For more
* information, see the Amazon SES
* Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendBulkEmail operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.SendBulkEmail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future sendBulkEmailAsync(SendBulkEmailRequest sendBulkEmailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an email address to the list of identities for your Amazon SES account in the current Amazon Web Services
* Region and attempts to verify it. As a result of executing this operation, a customized verification email is
* sent to the specified address.
*
*
* To use this operation, you must first create a custom verification email template. For more information about
* creating and using custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param sendCustomVerificationEmailRequest
* Represents a request to send a custom verification email to a specified recipient.
* @return A Java Future containing the result of the SendCustomVerificationEmail operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.SendCustomVerificationEmail
* @see AWS API Documentation
*/
java.util.concurrent.Future sendCustomVerificationEmailAsync(
SendCustomVerificationEmailRequest sendCustomVerificationEmailRequest);
/**
*
* Adds an email address to the list of identities for your Amazon SES account in the current Amazon Web Services
* Region and attempts to verify it. As a result of executing this operation, a customized verification email is
* sent to the specified address.
*
*
* To use this operation, you must first create a custom verification email template. For more information about
* creating and using custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param sendCustomVerificationEmailRequest
* Represents a request to send a custom verification email to a specified recipient.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendCustomVerificationEmail operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.SendCustomVerificationEmail
* @see AWS API Documentation
*/
java.util.concurrent.Future sendCustomVerificationEmailAsync(
SendCustomVerificationEmailRequest sendCustomVerificationEmailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends an email message. You can use the Amazon SES API v2 to send the following types of messages:
*
*
* -
*
* Simple – A standard email message. When you create this type of message, you specify the sender, the
* recipient, and the message body, and Amazon SES assembles the message for you.
*
*
* -
*
* Raw – A raw, MIME-formatted email message. When you send this type of email, you have to specify all of
* the message headers, as well as the message body. You can use this message type to send messages that contain
* attachments. The message that you specify has to be a valid MIME message.
*
*
* -
*
* Templated – A message that contains personalization tags. When you send this type of email, Amazon SES API
* v2 automatically replaces the tags with values that you specify.
*
*
*
*
* @param sendEmailRequest
* Represents a request to send a single formatted email using Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @return A Java Future containing the result of the SendEmail operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.SendEmail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future sendEmailAsync(SendEmailRequest sendEmailRequest);
/**
*
* Sends an email message. You can use the Amazon SES API v2 to send the following types of messages:
*
*
* -
*
* Simple – A standard email message. When you create this type of message, you specify the sender, the
* recipient, and the message body, and Amazon SES assembles the message for you.
*
*
* -
*
* Raw – A raw, MIME-formatted email message. When you send this type of email, you have to specify all of
* the message headers, as well as the message body. You can use this message type to send messages that contain
* attachments. The message that you specify has to be a valid MIME message.
*
*
* -
*
* Templated – A message that contains personalization tags. When you send this type of email, Amazon SES API
* v2 automatically replaces the tags with values that you specify.
*
*
*
*
* @param sendEmailRequest
* Represents a request to send a single formatted email using Amazon SES. For more information, see the Amazon SES
* Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendEmail operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.SendEmail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future sendEmailAsync(SendEmailRequest sendEmailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Add one or more tags (keys and values) to a specified resource. A tag is a label that you optionally
* define and associate with a resource. Tags can help you categorize and manage resources in different ways, such
* as by purpose, owner, environment, or other criteria. A resource can have as many as 50 tags.
*
*
* Each tag consists of a required tag key and an associated tag value, both of which you define. A
* tag key is a general label that acts as a category for more specific tag values. A tag value acts as a descriptor
* within a tag key.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Add one or more tags (keys and values) to a specified resource. A tag is a label that you optionally
* define and associate with a resource. Tags can help you categorize and manage resources in different ways, such
* as by purpose, owner, environment, or other criteria. A resource can have as many as 50 tags.
*
*
* Each tag consists of a required tag key and an associated tag value, both of which you define. A
* tag key is a general label that acts as a category for more specific tag values. A tag value acts as a descriptor
* within a tag key.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a preview of the MIME content of an email when provided with a template and a set of replacement data.
*
*
* You can execute this operation no more than once per second.
*
*
* @param testRenderEmailTemplateRequest
* >Represents a request to create a preview of the MIME content of an email when provided with a template
* and a set of replacement data.
* @return A Java Future containing the result of the TestRenderEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.TestRenderEmailTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future testRenderEmailTemplateAsync(TestRenderEmailTemplateRequest testRenderEmailTemplateRequest);
/**
*
* Creates a preview of the MIME content of an email when provided with a template and a set of replacement data.
*
*
* You can execute this operation no more than once per second.
*
*
* @param testRenderEmailTemplateRequest
* >Represents a request to create a preview of the MIME content of an email when provided with a template
* and a set of replacement data.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TestRenderEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.TestRenderEmailTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future testRenderEmailTemplateAsync(TestRenderEmailTemplateRequest testRenderEmailTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove one or more tags (keys and values) from a specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Remove one or more tags (keys and values) from a specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update the configuration of an event destination for a configuration set.
*
*
* Events include message sends, deliveries, opens, clicks, bounces, and complaints. Event
* destinations are places that you can send information about these events to. For example, you can send event
* data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use Amazon Kinesis
* Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param updateConfigurationSetEventDestinationRequest
* A request to change the settings for an event destination for a configuration set.
* @return A Java Future containing the result of the UpdateConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2Async.UpdateConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future updateConfigurationSetEventDestinationAsync(
UpdateConfigurationSetEventDestinationRequest updateConfigurationSetEventDestinationRequest);
/**
*
* Update the configuration of an event destination for a configuration set.
*
*
* Events include message sends, deliveries, opens, clicks, bounces, and complaints. Event
* destinations are places that you can send information about these events to. For example, you can send event
* data to Amazon SNS to receive notifications when you receive bounces or complaints, or you can use Amazon Kinesis
* Data Firehose to stream data to Amazon S3 for long-term storage.
*
*
* @param updateConfigurationSetEventDestinationRequest
* A request to change the settings for an event destination for a configuration set.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateConfigurationSetEventDestination operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.UpdateConfigurationSetEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future updateConfigurationSetEventDestinationAsync(
UpdateConfigurationSetEventDestinationRequest updateConfigurationSetEventDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a contact's preferences for a list. It is not necessary to specify all existing topic preferences in the
* TopicPreferences object, just the ones that need updating.
*
*
* @param updateContactRequest
* @return A Java Future containing the result of the UpdateContact operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.UpdateContact
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateContactAsync(UpdateContactRequest updateContactRequest);
/**
*
* Updates a contact's preferences for a list. It is not necessary to specify all existing topic preferences in the
* TopicPreferences object, just the ones that need updating.
*
*
* @param updateContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateContact operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.UpdateContact
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateContactAsync(UpdateContactRequest updateContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates contact list metadata. This operation does a complete replacement.
*
*
* @param updateContactListRequest
* @return A Java Future containing the result of the UpdateContactList operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.UpdateContactList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateContactListAsync(UpdateContactListRequest updateContactListRequest);
/**
*
* Updates contact list metadata. This operation does a complete replacement.
*
*
* @param updateContactListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateContactList operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.UpdateContactList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateContactListAsync(UpdateContactListRequest updateContactListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing custom verification email template.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param updateCustomVerificationEmailTemplateRequest
* Represents a request to update an existing custom verification email template.
* @return A Java Future containing the result of the UpdateCustomVerificationEmailTemplate operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2Async.UpdateCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCustomVerificationEmailTemplateAsync(
UpdateCustomVerificationEmailTemplateRequest updateCustomVerificationEmailTemplateRequest);
/**
*
* Updates an existing custom verification email template.
*
*
* For more information about custom verification email templates, see Using Custom
* Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param updateCustomVerificationEmailTemplateRequest
* Represents a request to update an existing custom verification email template.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateCustomVerificationEmailTemplate operation returned by
* the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.UpdateCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCustomVerificationEmailTemplateAsync(
UpdateCustomVerificationEmailTemplateRequest updateCustomVerificationEmailTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified sending authorization policy for the given identity (an email address or a domain). This
* API returns successfully even if a policy with the specified name does not exist.
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param updateEmailIdentityPolicyRequest
* Represents a request to update a sending authorization policy for an identity. Sending authorization is an
* Amazon SES feature that enables you to authorize other senders to use your identities. For information,
* see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the UpdateEmailIdentityPolicy operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.UpdateEmailIdentityPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future updateEmailIdentityPolicyAsync(
UpdateEmailIdentityPolicyRequest updateEmailIdentityPolicyRequest);
/**
*
* Updates the specified sending authorization policy for the given identity (an email address or a domain). This
* API returns successfully even if a policy with the specified name does not exist.
*
*
*
* This API is for the identity owner only. If you have not verified the identity, this API will return an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer
* Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param updateEmailIdentityPolicyRequest
* Represents a request to update a sending authorization policy for an identity. Sending authorization is an
* Amazon SES feature that enables you to authorize other senders to use your identities. For information,
* see the Amazon SES Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEmailIdentityPolicy operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.UpdateEmailIdentityPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future updateEmailIdentityPolicyAsync(
UpdateEmailIdentityPolicyRequest updateEmailIdentityPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an email template. Email templates enable you to send personalized email to one or more destinations in a
* single API operation. For more information, see the Amazon SES
* Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param updateEmailTemplateRequest
* Represents a request to update an email template. For more information, see the Amazon SES
* Developer Guide.
* @return A Java Future containing the result of the UpdateEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2Async.UpdateEmailTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateEmailTemplateAsync(UpdateEmailTemplateRequest updateEmailTemplateRequest);
/**
*
* Updates an email template. Email templates enable you to send personalized email to one or more destinations in a
* single API operation. For more information, see the Amazon SES
* Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param updateEmailTemplateRequest
* Represents a request to update an email template. For more information, see the Amazon SES
* Developer Guide.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEmailTemplate operation returned by the service.
* @sample AmazonSimpleEmailServiceV2AsyncHandler.UpdateEmailTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateEmailTemplateAsync(UpdateEmailTemplateRequest updateEmailTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}