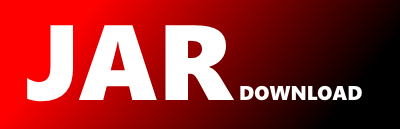
com.amazonaws.services.simpleemailv2.model.EmailContent Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sesv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simpleemailv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An object that defines the entire content of the email, including the message headers and the body content. You can
* create a simple email message, in which you specify the subject and the text and HTML versions of the message body.
* You can also create raw messages, in which you specify a complete MIME-formatted message. Raw messages can include
* attachments and custom headers.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EmailContent implements Serializable, Cloneable, StructuredPojo {
/**
*
* The simple email message. The message consists of a subject and a message body.
*
*/
private Message simple;
/**
*
* The raw email message. The message has to meet the following criteria:
*
*
* -
*
* The message has to contain a header and a body, separated by one blank line.
*
*
* -
*
* All of the required header fields must be present in the message.
*
*
* -
*
* Each part of a multipart MIME message must be formatted properly.
*
*
* -
*
* If you include attachments, they must be in a file format that the Amazon SES API v2 supports.
*
*
* -
*
* The raw data of the message needs to base64-encoded if you are accessing Amazon SES directly through the HTTPS
* interface. If you are accessing Amazon SES using an Amazon Web Services SDK, the SDK takes care of the base
* 64-encoding for you.
*
*
* -
*
* If any of the MIME parts in your message contain content that is outside of the 7-bit ASCII character range, you
* should encode that content to ensure that recipients' email clients render the message properly.
*
*
* -
*
* The length of any single line of text in the message can't exceed 1,000 characters. This restriction is defined
* in RFC 5321.
*
*
*
*/
private RawMessage raw;
/**
*
* The template to use for the email message.
*
*/
private Template template;
/**
*
* The simple email message. The message consists of a subject and a message body.
*
*
* @param simple
* The simple email message. The message consists of a subject and a message body.
*/
public void setSimple(Message simple) {
this.simple = simple;
}
/**
*
* The simple email message. The message consists of a subject and a message body.
*
*
* @return The simple email message. The message consists of a subject and a message body.
*/
public Message getSimple() {
return this.simple;
}
/**
*
* The simple email message. The message consists of a subject and a message body.
*
*
* @param simple
* The simple email message. The message consists of a subject and a message body.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EmailContent withSimple(Message simple) {
setSimple(simple);
return this;
}
/**
*
* The raw email message. The message has to meet the following criteria:
*
*
* -
*
* The message has to contain a header and a body, separated by one blank line.
*
*
* -
*
* All of the required header fields must be present in the message.
*
*
* -
*
* Each part of a multipart MIME message must be formatted properly.
*
*
* -
*
* If you include attachments, they must be in a file format that the Amazon SES API v2 supports.
*
*
* -
*
* The raw data of the message needs to base64-encoded if you are accessing Amazon SES directly through the HTTPS
* interface. If you are accessing Amazon SES using an Amazon Web Services SDK, the SDK takes care of the base
* 64-encoding for you.
*
*
* -
*
* If any of the MIME parts in your message contain content that is outside of the 7-bit ASCII character range, you
* should encode that content to ensure that recipients' email clients render the message properly.
*
*
* -
*
* The length of any single line of text in the message can't exceed 1,000 characters. This restriction is defined
* in RFC 5321.
*
*
*
*
* @param raw
* The raw email message. The message has to meet the following criteria:
*
* -
*
* The message has to contain a header and a body, separated by one blank line.
*
*
* -
*
* All of the required header fields must be present in the message.
*
*
* -
*
* Each part of a multipart MIME message must be formatted properly.
*
*
* -
*
* If you include attachments, they must be in a file format that the Amazon SES API v2 supports.
*
*
* -
*
* The raw data of the message needs to base64-encoded if you are accessing Amazon SES directly through the
* HTTPS interface. If you are accessing Amazon SES using an Amazon Web Services SDK, the SDK takes care of
* the base 64-encoding for you.
*
*
* -
*
* If any of the MIME parts in your message contain content that is outside of the 7-bit ASCII character
* range, you should encode that content to ensure that recipients' email clients render the message
* properly.
*
*
* -
*
* The length of any single line of text in the message can't exceed 1,000 characters. This restriction is
* defined in RFC 5321.
*
*
*/
public void setRaw(RawMessage raw) {
this.raw = raw;
}
/**
*
* The raw email message. The message has to meet the following criteria:
*
*
* -
*
* The message has to contain a header and a body, separated by one blank line.
*
*
* -
*
* All of the required header fields must be present in the message.
*
*
* -
*
* Each part of a multipart MIME message must be formatted properly.
*
*
* -
*
* If you include attachments, they must be in a file format that the Amazon SES API v2 supports.
*
*
* -
*
* The raw data of the message needs to base64-encoded if you are accessing Amazon SES directly through the HTTPS
* interface. If you are accessing Amazon SES using an Amazon Web Services SDK, the SDK takes care of the base
* 64-encoding for you.
*
*
* -
*
* If any of the MIME parts in your message contain content that is outside of the 7-bit ASCII character range, you
* should encode that content to ensure that recipients' email clients render the message properly.
*
*
* -
*
* The length of any single line of text in the message can't exceed 1,000 characters. This restriction is defined
* in RFC 5321.
*
*
*
*
* @return The raw email message. The message has to meet the following criteria:
*
* -
*
* The message has to contain a header and a body, separated by one blank line.
*
*
* -
*
* All of the required header fields must be present in the message.
*
*
* -
*
* Each part of a multipart MIME message must be formatted properly.
*
*
* -
*
* If you include attachments, they must be in a file format that the Amazon SES API v2 supports.
*
*
* -
*
* The raw data of the message needs to base64-encoded if you are accessing Amazon SES directly through the
* HTTPS interface. If you are accessing Amazon SES using an Amazon Web Services SDK, the SDK takes care of
* the base 64-encoding for you.
*
*
* -
*
* If any of the MIME parts in your message contain content that is outside of the 7-bit ASCII character
* range, you should encode that content to ensure that recipients' email clients render the message
* properly.
*
*
* -
*
* The length of any single line of text in the message can't exceed 1,000 characters. This restriction is
* defined in RFC 5321.
*
*
*/
public RawMessage getRaw() {
return this.raw;
}
/**
*
* The raw email message. The message has to meet the following criteria:
*
*
* -
*
* The message has to contain a header and a body, separated by one blank line.
*
*
* -
*
* All of the required header fields must be present in the message.
*
*
* -
*
* Each part of a multipart MIME message must be formatted properly.
*
*
* -
*
* If you include attachments, they must be in a file format that the Amazon SES API v2 supports.
*
*
* -
*
* The raw data of the message needs to base64-encoded if you are accessing Amazon SES directly through the HTTPS
* interface. If you are accessing Amazon SES using an Amazon Web Services SDK, the SDK takes care of the base
* 64-encoding for you.
*
*
* -
*
* If any of the MIME parts in your message contain content that is outside of the 7-bit ASCII character range, you
* should encode that content to ensure that recipients' email clients render the message properly.
*
*
* -
*
* The length of any single line of text in the message can't exceed 1,000 characters. This restriction is defined
* in RFC 5321.
*
*
*
*
* @param raw
* The raw email message. The message has to meet the following criteria:
*
* -
*
* The message has to contain a header and a body, separated by one blank line.
*
*
* -
*
* All of the required header fields must be present in the message.
*
*
* -
*
* Each part of a multipart MIME message must be formatted properly.
*
*
* -
*
* If you include attachments, they must be in a file format that the Amazon SES API v2 supports.
*
*
* -
*
* The raw data of the message needs to base64-encoded if you are accessing Amazon SES directly through the
* HTTPS interface. If you are accessing Amazon SES using an Amazon Web Services SDK, the SDK takes care of
* the base 64-encoding for you.
*
*
* -
*
* If any of the MIME parts in your message contain content that is outside of the 7-bit ASCII character
* range, you should encode that content to ensure that recipients' email clients render the message
* properly.
*
*
* -
*
* The length of any single line of text in the message can't exceed 1,000 characters. This restriction is
* defined in RFC 5321.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EmailContent withRaw(RawMessage raw) {
setRaw(raw);
return this;
}
/**
*
* The template to use for the email message.
*
*
* @param template
* The template to use for the email message.
*/
public void setTemplate(Template template) {
this.template = template;
}
/**
*
* The template to use for the email message.
*
*
* @return The template to use for the email message.
*/
public Template getTemplate() {
return this.template;
}
/**
*
* The template to use for the email message.
*
*
* @param template
* The template to use for the email message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EmailContent withTemplate(Template template) {
setTemplate(template);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSimple() != null)
sb.append("Simple: ").append(getSimple()).append(",");
if (getRaw() != null)
sb.append("Raw: ").append(getRaw()).append(",");
if (getTemplate() != null)
sb.append("Template: ").append(getTemplate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EmailContent == false)
return false;
EmailContent other = (EmailContent) obj;
if (other.getSimple() == null ^ this.getSimple() == null)
return false;
if (other.getSimple() != null && other.getSimple().equals(this.getSimple()) == false)
return false;
if (other.getRaw() == null ^ this.getRaw() == null)
return false;
if (other.getRaw() != null && other.getRaw().equals(this.getRaw()) == false)
return false;
if (other.getTemplate() == null ^ this.getTemplate() == null)
return false;
if (other.getTemplate() != null && other.getTemplate().equals(this.getTemplate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSimple() == null) ? 0 : getSimple().hashCode());
hashCode = prime * hashCode + ((getRaw() == null) ? 0 : getRaw().hashCode());
hashCode = prime * hashCode + ((getTemplate() == null) ? 0 : getTemplate().hashCode());
return hashCode;
}
@Override
public EmailContent clone() {
try {
return (EmailContent) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simpleemailv2.model.transform.EmailContentMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}