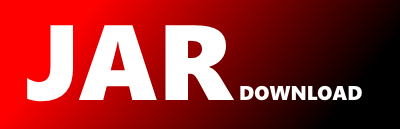
com.amazonaws.services.simpleemailv2.model.GetEmailIdentityResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sesv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simpleemailv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Details about an email identity.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetEmailIdentityResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The email identity type. Note: the MANAGED_DOMAIN
identity type is not supported.
*
*/
private String identityType;
/**
*
* The feedback forwarding configuration for the identity.
*
*
* If the value is true
, you receive email notifications when bounce or complaint events occur. These
* notifications are sent to the address that you specified in the Return-Path
header of the original
* email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another mechanism for
* receiving bounce or complaint notifications (for example, by setting up an event destination), you receive an
* email notification when these events occur (even if this setting is disabled).
*
*/
private Boolean feedbackForwardingStatus;
/**
*
* Specifies whether or not the identity is verified. You can only send email from verified email addresses or
* domains. For more information about verifying identities, see the Amazon Pinpoint
* User Guide.
*
*/
private Boolean verifiedForSendingStatus;
/**
*
* An object that contains information about the DKIM attributes for the identity.
*
*/
private DkimAttributes dkimAttributes;
/**
*
* An object that contains information about the Mail-From attributes for the email identity.
*
*/
private MailFromAttributes mailFromAttributes;
/**
*
* A map of policy names to policies.
*
*/
private java.util.Map policies;
/**
*
* An array of objects that define the tags (keys and values) that are associated with the email identity.
*
*/
private java.util.List tags;
/**
*
* The configuration set used by default when sending from this identity.
*
*/
private String configurationSetName;
/**
*
* The verification status of the identity. The status can be one of the following:
*
*
* -
*
* PENDING
– The verification process was initiated, but Amazon SES hasn't yet been able to verify the
* identity.
*
*
* -
*
* SUCCESS
– The verification process completed successfully.
*
*
* -
*
* FAILED
– The verification process failed.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue is preventing Amazon SES from determining the verification
* status of the identity.
*
*
* -
*
* NOT_STARTED
– The verification process hasn't been initiated for the identity.
*
*
*
*/
private String verificationStatus;
/**
*
* An object that contains additional information about the verification status for the identity.
*
*/
private VerificationInfo verificationInfo;
/**
*
* The email identity type. Note: the MANAGED_DOMAIN
identity type is not supported.
*
*
* @param identityType
* The email identity type. Note: the MANAGED_DOMAIN
identity type is not supported.
* @see IdentityType
*/
public void setIdentityType(String identityType) {
this.identityType = identityType;
}
/**
*
* The email identity type. Note: the MANAGED_DOMAIN
identity type is not supported.
*
*
* @return The email identity type. Note: the MANAGED_DOMAIN
identity type is not supported.
* @see IdentityType
*/
public String getIdentityType() {
return this.identityType;
}
/**
*
* The email identity type. Note: the MANAGED_DOMAIN
identity type is not supported.
*
*
* @param identityType
* The email identity type. Note: the MANAGED_DOMAIN
identity type is not supported.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IdentityType
*/
public GetEmailIdentityResult withIdentityType(String identityType) {
setIdentityType(identityType);
return this;
}
/**
*
* The email identity type. Note: the MANAGED_DOMAIN
identity type is not supported.
*
*
* @param identityType
* The email identity type. Note: the MANAGED_DOMAIN
identity type is not supported.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IdentityType
*/
public GetEmailIdentityResult withIdentityType(IdentityType identityType) {
this.identityType = identityType.toString();
return this;
}
/**
*
* The feedback forwarding configuration for the identity.
*
*
* If the value is true
, you receive email notifications when bounce or complaint events occur. These
* notifications are sent to the address that you specified in the Return-Path
header of the original
* email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another mechanism for
* receiving bounce or complaint notifications (for example, by setting up an event destination), you receive an
* email notification when these events occur (even if this setting is disabled).
*
*
* @param feedbackForwardingStatus
* The feedback forwarding configuration for the identity.
*
* If the value is true
, you receive email notifications when bounce or complaint events occur.
* These notifications are sent to the address that you specified in the Return-Path
header of
* the original email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another
* mechanism for receiving bounce or complaint notifications (for example, by setting up an event
* destination), you receive an email notification when these events occur (even if this setting is
* disabled).
*/
public void setFeedbackForwardingStatus(Boolean feedbackForwardingStatus) {
this.feedbackForwardingStatus = feedbackForwardingStatus;
}
/**
*
* The feedback forwarding configuration for the identity.
*
*
* If the value is true
, you receive email notifications when bounce or complaint events occur. These
* notifications are sent to the address that you specified in the Return-Path
header of the original
* email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another mechanism for
* receiving bounce or complaint notifications (for example, by setting up an event destination), you receive an
* email notification when these events occur (even if this setting is disabled).
*
*
* @return The feedback forwarding configuration for the identity.
*
* If the value is true
, you receive email notifications when bounce or complaint events occur.
* These notifications are sent to the address that you specified in the Return-Path
header of
* the original email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another
* mechanism for receiving bounce or complaint notifications (for example, by setting up an event
* destination), you receive an email notification when these events occur (even if this setting is
* disabled).
*/
public Boolean getFeedbackForwardingStatus() {
return this.feedbackForwardingStatus;
}
/**
*
* The feedback forwarding configuration for the identity.
*
*
* If the value is true
, you receive email notifications when bounce or complaint events occur. These
* notifications are sent to the address that you specified in the Return-Path
header of the original
* email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another mechanism for
* receiving bounce or complaint notifications (for example, by setting up an event destination), you receive an
* email notification when these events occur (even if this setting is disabled).
*
*
* @param feedbackForwardingStatus
* The feedback forwarding configuration for the identity.
*
* If the value is true
, you receive email notifications when bounce or complaint events occur.
* These notifications are sent to the address that you specified in the Return-Path
header of
* the original email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another
* mechanism for receiving bounce or complaint notifications (for example, by setting up an event
* destination), you receive an email notification when these events occur (even if this setting is
* disabled).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult withFeedbackForwardingStatus(Boolean feedbackForwardingStatus) {
setFeedbackForwardingStatus(feedbackForwardingStatus);
return this;
}
/**
*
* The feedback forwarding configuration for the identity.
*
*
* If the value is true
, you receive email notifications when bounce or complaint events occur. These
* notifications are sent to the address that you specified in the Return-Path
header of the original
* email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another mechanism for
* receiving bounce or complaint notifications (for example, by setting up an event destination), you receive an
* email notification when these events occur (even if this setting is disabled).
*
*
* @return The feedback forwarding configuration for the identity.
*
* If the value is true
, you receive email notifications when bounce or complaint events occur.
* These notifications are sent to the address that you specified in the Return-Path
header of
* the original email.
*
*
* You're required to have a method of tracking bounces and complaints. If you haven't set up another
* mechanism for receiving bounce or complaint notifications (for example, by setting up an event
* destination), you receive an email notification when these events occur (even if this setting is
* disabled).
*/
public Boolean isFeedbackForwardingStatus() {
return this.feedbackForwardingStatus;
}
/**
*
* Specifies whether or not the identity is verified. You can only send email from verified email addresses or
* domains. For more information about verifying identities, see the Amazon Pinpoint
* User Guide.
*
*
* @param verifiedForSendingStatus
* Specifies whether or not the identity is verified. You can only send email from verified email addresses
* or domains. For more information about verifying identities, see the Amazon
* Pinpoint User Guide.
*/
public void setVerifiedForSendingStatus(Boolean verifiedForSendingStatus) {
this.verifiedForSendingStatus = verifiedForSendingStatus;
}
/**
*
* Specifies whether or not the identity is verified. You can only send email from verified email addresses or
* domains. For more information about verifying identities, see the Amazon Pinpoint
* User Guide.
*
*
* @return Specifies whether or not the identity is verified. You can only send email from verified email addresses
* or domains. For more information about verifying identities, see the Amazon
* Pinpoint User Guide.
*/
public Boolean getVerifiedForSendingStatus() {
return this.verifiedForSendingStatus;
}
/**
*
* Specifies whether or not the identity is verified. You can only send email from verified email addresses or
* domains. For more information about verifying identities, see the Amazon Pinpoint
* User Guide.
*
*
* @param verifiedForSendingStatus
* Specifies whether or not the identity is verified. You can only send email from verified email addresses
* or domains. For more information about verifying identities, see the Amazon
* Pinpoint User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult withVerifiedForSendingStatus(Boolean verifiedForSendingStatus) {
setVerifiedForSendingStatus(verifiedForSendingStatus);
return this;
}
/**
*
* Specifies whether or not the identity is verified. You can only send email from verified email addresses or
* domains. For more information about verifying identities, see the Amazon Pinpoint
* User Guide.
*
*
* @return Specifies whether or not the identity is verified. You can only send email from verified email addresses
* or domains. For more information about verifying identities, see the Amazon
* Pinpoint User Guide.
*/
public Boolean isVerifiedForSendingStatus() {
return this.verifiedForSendingStatus;
}
/**
*
* An object that contains information about the DKIM attributes for the identity.
*
*
* @param dkimAttributes
* An object that contains information about the DKIM attributes for the identity.
*/
public void setDkimAttributes(DkimAttributes dkimAttributes) {
this.dkimAttributes = dkimAttributes;
}
/**
*
* An object that contains information about the DKIM attributes for the identity.
*
*
* @return An object that contains information about the DKIM attributes for the identity.
*/
public DkimAttributes getDkimAttributes() {
return this.dkimAttributes;
}
/**
*
* An object that contains information about the DKIM attributes for the identity.
*
*
* @param dkimAttributes
* An object that contains information about the DKIM attributes for the identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult withDkimAttributes(DkimAttributes dkimAttributes) {
setDkimAttributes(dkimAttributes);
return this;
}
/**
*
* An object that contains information about the Mail-From attributes for the email identity.
*
*
* @param mailFromAttributes
* An object that contains information about the Mail-From attributes for the email identity.
*/
public void setMailFromAttributes(MailFromAttributes mailFromAttributes) {
this.mailFromAttributes = mailFromAttributes;
}
/**
*
* An object that contains information about the Mail-From attributes for the email identity.
*
*
* @return An object that contains information about the Mail-From attributes for the email identity.
*/
public MailFromAttributes getMailFromAttributes() {
return this.mailFromAttributes;
}
/**
*
* An object that contains information about the Mail-From attributes for the email identity.
*
*
* @param mailFromAttributes
* An object that contains information about the Mail-From attributes for the email identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult withMailFromAttributes(MailFromAttributes mailFromAttributes) {
setMailFromAttributes(mailFromAttributes);
return this;
}
/**
*
* A map of policy names to policies.
*
*
* @return A map of policy names to policies.
*/
public java.util.Map getPolicies() {
return policies;
}
/**
*
* A map of policy names to policies.
*
*
* @param policies
* A map of policy names to policies.
*/
public void setPolicies(java.util.Map policies) {
this.policies = policies;
}
/**
*
* A map of policy names to policies.
*
*
* @param policies
* A map of policy names to policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult withPolicies(java.util.Map policies) {
setPolicies(policies);
return this;
}
/**
* Add a single Policies entry
*
* @see GetEmailIdentityResult#withPolicies
* @returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult addPoliciesEntry(String key, String value) {
if (null == this.policies) {
this.policies = new java.util.HashMap();
}
if (this.policies.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.policies.put(key, value);
return this;
}
/**
* Removes all the entries added into Policies.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult clearPoliciesEntries() {
this.policies = null;
return this;
}
/**
*
* An array of objects that define the tags (keys and values) that are associated with the email identity.
*
*
* @return An array of objects that define the tags (keys and values) that are associated with the email identity.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of objects that define the tags (keys and values) that are associated with the email identity.
*
*
* @param tags
* An array of objects that define the tags (keys and values) that are associated with the email identity.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of objects that define the tags (keys and values) that are associated with the email identity.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of objects that define the tags (keys and values) that are associated with the email identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of objects that define the tags (keys and values) that are associated with the email identity.
*
*
* @param tags
* An array of objects that define the tags (keys and values) that are associated with the email identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The configuration set used by default when sending from this identity.
*
*
* @param configurationSetName
* The configuration set used by default when sending from this identity.
*/
public void setConfigurationSetName(String configurationSetName) {
this.configurationSetName = configurationSetName;
}
/**
*
* The configuration set used by default when sending from this identity.
*
*
* @return The configuration set used by default when sending from this identity.
*/
public String getConfigurationSetName() {
return this.configurationSetName;
}
/**
*
* The configuration set used by default when sending from this identity.
*
*
* @param configurationSetName
* The configuration set used by default when sending from this identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult withConfigurationSetName(String configurationSetName) {
setConfigurationSetName(configurationSetName);
return this;
}
/**
*
* The verification status of the identity. The status can be one of the following:
*
*
* -
*
* PENDING
– The verification process was initiated, but Amazon SES hasn't yet been able to verify the
* identity.
*
*
* -
*
* SUCCESS
– The verification process completed successfully.
*
*
* -
*
* FAILED
– The verification process failed.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue is preventing Amazon SES from determining the verification
* status of the identity.
*
*
* -
*
* NOT_STARTED
– The verification process hasn't been initiated for the identity.
*
*
*
*
* @param verificationStatus
* The verification status of the identity. The status can be one of the following:
*
* -
*
* PENDING
– The verification process was initiated, but Amazon SES hasn't yet been able to
* verify the identity.
*
*
* -
*
* SUCCESS
– The verification process completed successfully.
*
*
* -
*
* FAILED
– The verification process failed.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue is preventing Amazon SES from determining the
* verification status of the identity.
*
*
* -
*
* NOT_STARTED
– The verification process hasn't been initiated for the identity.
*
*
* @see VerificationStatus
*/
public void setVerificationStatus(String verificationStatus) {
this.verificationStatus = verificationStatus;
}
/**
*
* The verification status of the identity. The status can be one of the following:
*
*
* -
*
* PENDING
– The verification process was initiated, but Amazon SES hasn't yet been able to verify the
* identity.
*
*
* -
*
* SUCCESS
– The verification process completed successfully.
*
*
* -
*
* FAILED
– The verification process failed.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue is preventing Amazon SES from determining the verification
* status of the identity.
*
*
* -
*
* NOT_STARTED
– The verification process hasn't been initiated for the identity.
*
*
*
*
* @return The verification status of the identity. The status can be one of the following:
*
* -
*
* PENDING
– The verification process was initiated, but Amazon SES hasn't yet been able to
* verify the identity.
*
*
* -
*
* SUCCESS
– The verification process completed successfully.
*
*
* -
*
* FAILED
– The verification process failed.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue is preventing Amazon SES from determining the
* verification status of the identity.
*
*
* -
*
* NOT_STARTED
– The verification process hasn't been initiated for the identity.
*
*
* @see VerificationStatus
*/
public String getVerificationStatus() {
return this.verificationStatus;
}
/**
*
* The verification status of the identity. The status can be one of the following:
*
*
* -
*
* PENDING
– The verification process was initiated, but Amazon SES hasn't yet been able to verify the
* identity.
*
*
* -
*
* SUCCESS
– The verification process completed successfully.
*
*
* -
*
* FAILED
– The verification process failed.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue is preventing Amazon SES from determining the verification
* status of the identity.
*
*
* -
*
* NOT_STARTED
– The verification process hasn't been initiated for the identity.
*
*
*
*
* @param verificationStatus
* The verification status of the identity. The status can be one of the following:
*
* -
*
* PENDING
– The verification process was initiated, but Amazon SES hasn't yet been able to
* verify the identity.
*
*
* -
*
* SUCCESS
– The verification process completed successfully.
*
*
* -
*
* FAILED
– The verification process failed.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue is preventing Amazon SES from determining the
* verification status of the identity.
*
*
* -
*
* NOT_STARTED
– The verification process hasn't been initiated for the identity.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see VerificationStatus
*/
public GetEmailIdentityResult withVerificationStatus(String verificationStatus) {
setVerificationStatus(verificationStatus);
return this;
}
/**
*
* The verification status of the identity. The status can be one of the following:
*
*
* -
*
* PENDING
– The verification process was initiated, but Amazon SES hasn't yet been able to verify the
* identity.
*
*
* -
*
* SUCCESS
– The verification process completed successfully.
*
*
* -
*
* FAILED
– The verification process failed.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue is preventing Amazon SES from determining the verification
* status of the identity.
*
*
* -
*
* NOT_STARTED
– The verification process hasn't been initiated for the identity.
*
*
*
*
* @param verificationStatus
* The verification status of the identity. The status can be one of the following:
*
* -
*
* PENDING
– The verification process was initiated, but Amazon SES hasn't yet been able to
* verify the identity.
*
*
* -
*
* SUCCESS
– The verification process completed successfully.
*
*
* -
*
* FAILED
– The verification process failed.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue is preventing Amazon SES from determining the
* verification status of the identity.
*
*
* -
*
* NOT_STARTED
– The verification process hasn't been initiated for the identity.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see VerificationStatus
*/
public GetEmailIdentityResult withVerificationStatus(VerificationStatus verificationStatus) {
this.verificationStatus = verificationStatus.toString();
return this;
}
/**
*
* An object that contains additional information about the verification status for the identity.
*
*
* @param verificationInfo
* An object that contains additional information about the verification status for the identity.
*/
public void setVerificationInfo(VerificationInfo verificationInfo) {
this.verificationInfo = verificationInfo;
}
/**
*
* An object that contains additional information about the verification status for the identity.
*
*
* @return An object that contains additional information about the verification status for the identity.
*/
public VerificationInfo getVerificationInfo() {
return this.verificationInfo;
}
/**
*
* An object that contains additional information about the verification status for the identity.
*
*
* @param verificationInfo
* An object that contains additional information about the verification status for the identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEmailIdentityResult withVerificationInfo(VerificationInfo verificationInfo) {
setVerificationInfo(verificationInfo);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getIdentityType() != null)
sb.append("IdentityType: ").append(getIdentityType()).append(",");
if (getFeedbackForwardingStatus() != null)
sb.append("FeedbackForwardingStatus: ").append(getFeedbackForwardingStatus()).append(",");
if (getVerifiedForSendingStatus() != null)
sb.append("VerifiedForSendingStatus: ").append(getVerifiedForSendingStatus()).append(",");
if (getDkimAttributes() != null)
sb.append("DkimAttributes: ").append(getDkimAttributes()).append(",");
if (getMailFromAttributes() != null)
sb.append("MailFromAttributes: ").append(getMailFromAttributes()).append(",");
if (getPolicies() != null)
sb.append("Policies: ").append(getPolicies()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getConfigurationSetName() != null)
sb.append("ConfigurationSetName: ").append(getConfigurationSetName()).append(",");
if (getVerificationStatus() != null)
sb.append("VerificationStatus: ").append(getVerificationStatus()).append(",");
if (getVerificationInfo() != null)
sb.append("VerificationInfo: ").append(getVerificationInfo());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetEmailIdentityResult == false)
return false;
GetEmailIdentityResult other = (GetEmailIdentityResult) obj;
if (other.getIdentityType() == null ^ this.getIdentityType() == null)
return false;
if (other.getIdentityType() != null && other.getIdentityType().equals(this.getIdentityType()) == false)
return false;
if (other.getFeedbackForwardingStatus() == null ^ this.getFeedbackForwardingStatus() == null)
return false;
if (other.getFeedbackForwardingStatus() != null && other.getFeedbackForwardingStatus().equals(this.getFeedbackForwardingStatus()) == false)
return false;
if (other.getVerifiedForSendingStatus() == null ^ this.getVerifiedForSendingStatus() == null)
return false;
if (other.getVerifiedForSendingStatus() != null && other.getVerifiedForSendingStatus().equals(this.getVerifiedForSendingStatus()) == false)
return false;
if (other.getDkimAttributes() == null ^ this.getDkimAttributes() == null)
return false;
if (other.getDkimAttributes() != null && other.getDkimAttributes().equals(this.getDkimAttributes()) == false)
return false;
if (other.getMailFromAttributes() == null ^ this.getMailFromAttributes() == null)
return false;
if (other.getMailFromAttributes() != null && other.getMailFromAttributes().equals(this.getMailFromAttributes()) == false)
return false;
if (other.getPolicies() == null ^ this.getPolicies() == null)
return false;
if (other.getPolicies() != null && other.getPolicies().equals(this.getPolicies()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getConfigurationSetName() == null ^ this.getConfigurationSetName() == null)
return false;
if (other.getConfigurationSetName() != null && other.getConfigurationSetName().equals(this.getConfigurationSetName()) == false)
return false;
if (other.getVerificationStatus() == null ^ this.getVerificationStatus() == null)
return false;
if (other.getVerificationStatus() != null && other.getVerificationStatus().equals(this.getVerificationStatus()) == false)
return false;
if (other.getVerificationInfo() == null ^ this.getVerificationInfo() == null)
return false;
if (other.getVerificationInfo() != null && other.getVerificationInfo().equals(this.getVerificationInfo()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getIdentityType() == null) ? 0 : getIdentityType().hashCode());
hashCode = prime * hashCode + ((getFeedbackForwardingStatus() == null) ? 0 : getFeedbackForwardingStatus().hashCode());
hashCode = prime * hashCode + ((getVerifiedForSendingStatus() == null) ? 0 : getVerifiedForSendingStatus().hashCode());
hashCode = prime * hashCode + ((getDkimAttributes() == null) ? 0 : getDkimAttributes().hashCode());
hashCode = prime * hashCode + ((getMailFromAttributes() == null) ? 0 : getMailFromAttributes().hashCode());
hashCode = prime * hashCode + ((getPolicies() == null) ? 0 : getPolicies().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getConfigurationSetName() == null) ? 0 : getConfigurationSetName().hashCode());
hashCode = prime * hashCode + ((getVerificationStatus() == null) ? 0 : getVerificationStatus().hashCode());
hashCode = prime * hashCode + ((getVerificationInfo() == null) ? 0 : getVerificationInfo().hashCode());
return hashCode;
}
@Override
public GetEmailIdentityResult clone() {
try {
return (GetEmailIdentityResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}