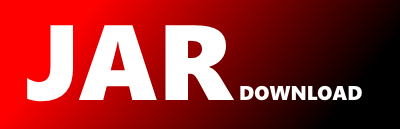
com.amazonaws.services.simpleemailv2.model.MailFromAttributes Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sesv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simpleemailv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A list of attributes that are associated with a MAIL FROM domain.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MailFromAttributes implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of a domain that an email identity uses as a custom MAIL FROM domain.
*
*/
private String mailFromDomain;
/**
*
* The status of the MAIL FROM domain. This status can have the following values:
*
*
* -
*
* PENDING
– Amazon SES hasn't started searching for the MX record yet.
*
*
* -
*
* SUCCESS
– Amazon SES detected the required MX record for the MAIL FROM domain.
*
*
* -
*
* FAILED
– Amazon SES can't find the required MX record, or the record no longer exists.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue occurred, which prevented Amazon SES from determining the
* status of the MAIL FROM domain.
*
*
*
*/
private String mailFromDomainStatus;
/**
*
* The action to take if the required MX record can't be found when you send an email. When you set this value to
* USE_DEFAULT_VALUE
, the mail is sent using amazonses.com as the MAIL FROM domain. When you set
* this value to REJECT_MESSAGE
, the Amazon SES API v2 returns a MailFromDomainNotVerified
* error, and doesn't attempt to deliver the email.
*
*
* These behaviors are taken when the custom MAIL FROM domain configuration is in the Pending
,
* Failed
, and TemporaryFailure
states.
*
*/
private String behaviorOnMxFailure;
/**
*
* The name of a domain that an email identity uses as a custom MAIL FROM domain.
*
*
* @param mailFromDomain
* The name of a domain that an email identity uses as a custom MAIL FROM domain.
*/
public void setMailFromDomain(String mailFromDomain) {
this.mailFromDomain = mailFromDomain;
}
/**
*
* The name of a domain that an email identity uses as a custom MAIL FROM domain.
*
*
* @return The name of a domain that an email identity uses as a custom MAIL FROM domain.
*/
public String getMailFromDomain() {
return this.mailFromDomain;
}
/**
*
* The name of a domain that an email identity uses as a custom MAIL FROM domain.
*
*
* @param mailFromDomain
* The name of a domain that an email identity uses as a custom MAIL FROM domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MailFromAttributes withMailFromDomain(String mailFromDomain) {
setMailFromDomain(mailFromDomain);
return this;
}
/**
*
* The status of the MAIL FROM domain. This status can have the following values:
*
*
* -
*
* PENDING
– Amazon SES hasn't started searching for the MX record yet.
*
*
* -
*
* SUCCESS
– Amazon SES detected the required MX record for the MAIL FROM domain.
*
*
* -
*
* FAILED
– Amazon SES can't find the required MX record, or the record no longer exists.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue occurred, which prevented Amazon SES from determining the
* status of the MAIL FROM domain.
*
*
*
*
* @param mailFromDomainStatus
* The status of the MAIL FROM domain. This status can have the following values:
*
* -
*
* PENDING
– Amazon SES hasn't started searching for the MX record yet.
*
*
* -
*
* SUCCESS
– Amazon SES detected the required MX record for the MAIL FROM domain.
*
*
* -
*
* FAILED
– Amazon SES can't find the required MX record, or the record no longer exists.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue occurred, which prevented Amazon SES from determining
* the status of the MAIL FROM domain.
*
*
* @see MailFromDomainStatus
*/
public void setMailFromDomainStatus(String mailFromDomainStatus) {
this.mailFromDomainStatus = mailFromDomainStatus;
}
/**
*
* The status of the MAIL FROM domain. This status can have the following values:
*
*
* -
*
* PENDING
– Amazon SES hasn't started searching for the MX record yet.
*
*
* -
*
* SUCCESS
– Amazon SES detected the required MX record for the MAIL FROM domain.
*
*
* -
*
* FAILED
– Amazon SES can't find the required MX record, or the record no longer exists.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue occurred, which prevented Amazon SES from determining the
* status of the MAIL FROM domain.
*
*
*
*
* @return The status of the MAIL FROM domain. This status can have the following values:
*
* -
*
* PENDING
– Amazon SES hasn't started searching for the MX record yet.
*
*
* -
*
* SUCCESS
– Amazon SES detected the required MX record for the MAIL FROM domain.
*
*
* -
*
* FAILED
– Amazon SES can't find the required MX record, or the record no longer exists.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue occurred, which prevented Amazon SES from determining
* the status of the MAIL FROM domain.
*
*
* @see MailFromDomainStatus
*/
public String getMailFromDomainStatus() {
return this.mailFromDomainStatus;
}
/**
*
* The status of the MAIL FROM domain. This status can have the following values:
*
*
* -
*
* PENDING
– Amazon SES hasn't started searching for the MX record yet.
*
*
* -
*
* SUCCESS
– Amazon SES detected the required MX record for the MAIL FROM domain.
*
*
* -
*
* FAILED
– Amazon SES can't find the required MX record, or the record no longer exists.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue occurred, which prevented Amazon SES from determining the
* status of the MAIL FROM domain.
*
*
*
*
* @param mailFromDomainStatus
* The status of the MAIL FROM domain. This status can have the following values:
*
* -
*
* PENDING
– Amazon SES hasn't started searching for the MX record yet.
*
*
* -
*
* SUCCESS
– Amazon SES detected the required MX record for the MAIL FROM domain.
*
*
* -
*
* FAILED
– Amazon SES can't find the required MX record, or the record no longer exists.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue occurred, which prevented Amazon SES from determining
* the status of the MAIL FROM domain.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MailFromDomainStatus
*/
public MailFromAttributes withMailFromDomainStatus(String mailFromDomainStatus) {
setMailFromDomainStatus(mailFromDomainStatus);
return this;
}
/**
*
* The status of the MAIL FROM domain. This status can have the following values:
*
*
* -
*
* PENDING
– Amazon SES hasn't started searching for the MX record yet.
*
*
* -
*
* SUCCESS
– Amazon SES detected the required MX record for the MAIL FROM domain.
*
*
* -
*
* FAILED
– Amazon SES can't find the required MX record, or the record no longer exists.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue occurred, which prevented Amazon SES from determining the
* status of the MAIL FROM domain.
*
*
*
*
* @param mailFromDomainStatus
* The status of the MAIL FROM domain. This status can have the following values:
*
* -
*
* PENDING
– Amazon SES hasn't started searching for the MX record yet.
*
*
* -
*
* SUCCESS
– Amazon SES detected the required MX record for the MAIL FROM domain.
*
*
* -
*
* FAILED
– Amazon SES can't find the required MX record, or the record no longer exists.
*
*
* -
*
* TEMPORARY_FAILURE
– A temporary issue occurred, which prevented Amazon SES from determining
* the status of the MAIL FROM domain.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MailFromDomainStatus
*/
public MailFromAttributes withMailFromDomainStatus(MailFromDomainStatus mailFromDomainStatus) {
this.mailFromDomainStatus = mailFromDomainStatus.toString();
return this;
}
/**
*
* The action to take if the required MX record can't be found when you send an email. When you set this value to
* USE_DEFAULT_VALUE
, the mail is sent using amazonses.com as the MAIL FROM domain. When you set
* this value to REJECT_MESSAGE
, the Amazon SES API v2 returns a MailFromDomainNotVerified
* error, and doesn't attempt to deliver the email.
*
*
* These behaviors are taken when the custom MAIL FROM domain configuration is in the Pending
,
* Failed
, and TemporaryFailure
states.
*
*
* @param behaviorOnMxFailure
* The action to take if the required MX record can't be found when you send an email. When you set this
* value to USE_DEFAULT_VALUE
, the mail is sent using amazonses.com as the MAIL FROM
* domain. When you set this value to REJECT_MESSAGE
, the Amazon SES API v2 returns a
* MailFromDomainNotVerified
error, and doesn't attempt to deliver the email.
*
* These behaviors are taken when the custom MAIL FROM domain configuration is in the Pending
,
* Failed
, and TemporaryFailure
states.
* @see BehaviorOnMxFailure
*/
public void setBehaviorOnMxFailure(String behaviorOnMxFailure) {
this.behaviorOnMxFailure = behaviorOnMxFailure;
}
/**
*
* The action to take if the required MX record can't be found when you send an email. When you set this value to
* USE_DEFAULT_VALUE
, the mail is sent using amazonses.com as the MAIL FROM domain. When you set
* this value to REJECT_MESSAGE
, the Amazon SES API v2 returns a MailFromDomainNotVerified
* error, and doesn't attempt to deliver the email.
*
*
* These behaviors are taken when the custom MAIL FROM domain configuration is in the Pending
,
* Failed
, and TemporaryFailure
states.
*
*
* @return The action to take if the required MX record can't be found when you send an email. When you set this
* value to USE_DEFAULT_VALUE
, the mail is sent using amazonses.com as the MAIL FROM
* domain. When you set this value to REJECT_MESSAGE
, the Amazon SES API v2 returns a
* MailFromDomainNotVerified
error, and doesn't attempt to deliver the email.
*
* These behaviors are taken when the custom MAIL FROM domain configuration is in the Pending
,
* Failed
, and TemporaryFailure
states.
* @see BehaviorOnMxFailure
*/
public String getBehaviorOnMxFailure() {
return this.behaviorOnMxFailure;
}
/**
*
* The action to take if the required MX record can't be found when you send an email. When you set this value to
* USE_DEFAULT_VALUE
, the mail is sent using amazonses.com as the MAIL FROM domain. When you set
* this value to REJECT_MESSAGE
, the Amazon SES API v2 returns a MailFromDomainNotVerified
* error, and doesn't attempt to deliver the email.
*
*
* These behaviors are taken when the custom MAIL FROM domain configuration is in the Pending
,
* Failed
, and TemporaryFailure
states.
*
*
* @param behaviorOnMxFailure
* The action to take if the required MX record can't be found when you send an email. When you set this
* value to USE_DEFAULT_VALUE
, the mail is sent using amazonses.com as the MAIL FROM
* domain. When you set this value to REJECT_MESSAGE
, the Amazon SES API v2 returns a
* MailFromDomainNotVerified
error, and doesn't attempt to deliver the email.
*
* These behaviors are taken when the custom MAIL FROM domain configuration is in the Pending
,
* Failed
, and TemporaryFailure
states.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BehaviorOnMxFailure
*/
public MailFromAttributes withBehaviorOnMxFailure(String behaviorOnMxFailure) {
setBehaviorOnMxFailure(behaviorOnMxFailure);
return this;
}
/**
*
* The action to take if the required MX record can't be found when you send an email. When you set this value to
* USE_DEFAULT_VALUE
, the mail is sent using amazonses.com as the MAIL FROM domain. When you set
* this value to REJECT_MESSAGE
, the Amazon SES API v2 returns a MailFromDomainNotVerified
* error, and doesn't attempt to deliver the email.
*
*
* These behaviors are taken when the custom MAIL FROM domain configuration is in the Pending
,
* Failed
, and TemporaryFailure
states.
*
*
* @param behaviorOnMxFailure
* The action to take if the required MX record can't be found when you send an email. When you set this
* value to USE_DEFAULT_VALUE
, the mail is sent using amazonses.com as the MAIL FROM
* domain. When you set this value to REJECT_MESSAGE
, the Amazon SES API v2 returns a
* MailFromDomainNotVerified
error, and doesn't attempt to deliver the email.
*
* These behaviors are taken when the custom MAIL FROM domain configuration is in the Pending
,
* Failed
, and TemporaryFailure
states.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BehaviorOnMxFailure
*/
public MailFromAttributes withBehaviorOnMxFailure(BehaviorOnMxFailure behaviorOnMxFailure) {
this.behaviorOnMxFailure = behaviorOnMxFailure.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMailFromDomain() != null)
sb.append("MailFromDomain: ").append(getMailFromDomain()).append(",");
if (getMailFromDomainStatus() != null)
sb.append("MailFromDomainStatus: ").append(getMailFromDomainStatus()).append(",");
if (getBehaviorOnMxFailure() != null)
sb.append("BehaviorOnMxFailure: ").append(getBehaviorOnMxFailure());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MailFromAttributes == false)
return false;
MailFromAttributes other = (MailFromAttributes) obj;
if (other.getMailFromDomain() == null ^ this.getMailFromDomain() == null)
return false;
if (other.getMailFromDomain() != null && other.getMailFromDomain().equals(this.getMailFromDomain()) == false)
return false;
if (other.getMailFromDomainStatus() == null ^ this.getMailFromDomainStatus() == null)
return false;
if (other.getMailFromDomainStatus() != null && other.getMailFromDomainStatus().equals(this.getMailFromDomainStatus()) == false)
return false;
if (other.getBehaviorOnMxFailure() == null ^ this.getBehaviorOnMxFailure() == null)
return false;
if (other.getBehaviorOnMxFailure() != null && other.getBehaviorOnMxFailure().equals(this.getBehaviorOnMxFailure()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMailFromDomain() == null) ? 0 : getMailFromDomain().hashCode());
hashCode = prime * hashCode + ((getMailFromDomainStatus() == null) ? 0 : getMailFromDomainStatus().hashCode());
hashCode = prime * hashCode + ((getBehaviorOnMxFailure() == null) ? 0 : getBehaviorOnMxFailure().hashCode());
return hashCode;
}
@Override
public MailFromAttributes clone() {
try {
return (MailFromAttributes) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simpleemailv2.model.transform.MailFromAttributesMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}