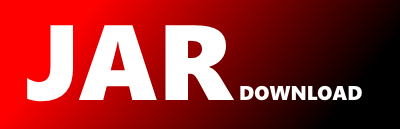
com.amazonaws.services.simpleemailv2.model.PutAccountDetailsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sesv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simpleemailv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* A request to submit new account details.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutAccountDetailsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The type of email your account will send.
*
*/
private String mailType;
/**
*
* The URL of your website. This information helps us better understand the type of content that you plan to send.
*
*/
private String websiteURL;
/**
*
* The language you would prefer to be contacted with.
*
*/
private String contactLanguage;
/**
*
* A description of the types of email that you plan to send.
*
*/
private String useCaseDescription;
/**
*
* Additional email addresses that you would like to be notified regarding Amazon SES matters.
*
*/
private java.util.List additionalContactEmailAddresses;
/**
*
* Indicates whether or not your account should have production access in the current Amazon Web Services Region.
*
*
* If the value is false
, then your account is in the sandbox. When your account is in the
* sandbox, you can only send email to verified identities.
*
*
* If the value is true
, then your account has production access. When your account has production
* access, you can send email to any address. The sending quota and maximum sending rate for your account vary based
* on your specific use case.
*
*/
private Boolean productionAccessEnabled;
/**
*
* The type of email your account will send.
*
*
* @param mailType
* The type of email your account will send.
* @see MailType
*/
public void setMailType(String mailType) {
this.mailType = mailType;
}
/**
*
* The type of email your account will send.
*
*
* @return The type of email your account will send.
* @see MailType
*/
public String getMailType() {
return this.mailType;
}
/**
*
* The type of email your account will send.
*
*
* @param mailType
* The type of email your account will send.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MailType
*/
public PutAccountDetailsRequest withMailType(String mailType) {
setMailType(mailType);
return this;
}
/**
*
* The type of email your account will send.
*
*
* @param mailType
* The type of email your account will send.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MailType
*/
public PutAccountDetailsRequest withMailType(MailType mailType) {
this.mailType = mailType.toString();
return this;
}
/**
*
* The URL of your website. This information helps us better understand the type of content that you plan to send.
*
*
* @param websiteURL
* The URL of your website. This information helps us better understand the type of content that you plan to
* send.
*/
public void setWebsiteURL(String websiteURL) {
this.websiteURL = websiteURL;
}
/**
*
* The URL of your website. This information helps us better understand the type of content that you plan to send.
*
*
* @return The URL of your website. This information helps us better understand the type of content that you plan to
* send.
*/
public String getWebsiteURL() {
return this.websiteURL;
}
/**
*
* The URL of your website. This information helps us better understand the type of content that you plan to send.
*
*
* @param websiteURL
* The URL of your website. This information helps us better understand the type of content that you plan to
* send.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutAccountDetailsRequest withWebsiteURL(String websiteURL) {
setWebsiteURL(websiteURL);
return this;
}
/**
*
* The language you would prefer to be contacted with.
*
*
* @param contactLanguage
* The language you would prefer to be contacted with.
* @see ContactLanguage
*/
public void setContactLanguage(String contactLanguage) {
this.contactLanguage = contactLanguage;
}
/**
*
* The language you would prefer to be contacted with.
*
*
* @return The language you would prefer to be contacted with.
* @see ContactLanguage
*/
public String getContactLanguage() {
return this.contactLanguage;
}
/**
*
* The language you would prefer to be contacted with.
*
*
* @param contactLanguage
* The language you would prefer to be contacted with.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContactLanguage
*/
public PutAccountDetailsRequest withContactLanguage(String contactLanguage) {
setContactLanguage(contactLanguage);
return this;
}
/**
*
* The language you would prefer to be contacted with.
*
*
* @param contactLanguage
* The language you would prefer to be contacted with.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ContactLanguage
*/
public PutAccountDetailsRequest withContactLanguage(ContactLanguage contactLanguage) {
this.contactLanguage = contactLanguage.toString();
return this;
}
/**
*
* A description of the types of email that you plan to send.
*
*
* @param useCaseDescription
* A description of the types of email that you plan to send.
*/
public void setUseCaseDescription(String useCaseDescription) {
this.useCaseDescription = useCaseDescription;
}
/**
*
* A description of the types of email that you plan to send.
*
*
* @return A description of the types of email that you plan to send.
*/
public String getUseCaseDescription() {
return this.useCaseDescription;
}
/**
*
* A description of the types of email that you plan to send.
*
*
* @param useCaseDescription
* A description of the types of email that you plan to send.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutAccountDetailsRequest withUseCaseDescription(String useCaseDescription) {
setUseCaseDescription(useCaseDescription);
return this;
}
/**
*
* Additional email addresses that you would like to be notified regarding Amazon SES matters.
*
*
* @return Additional email addresses that you would like to be notified regarding Amazon SES matters.
*/
public java.util.List getAdditionalContactEmailAddresses() {
return additionalContactEmailAddresses;
}
/**
*
* Additional email addresses that you would like to be notified regarding Amazon SES matters.
*
*
* @param additionalContactEmailAddresses
* Additional email addresses that you would like to be notified regarding Amazon SES matters.
*/
public void setAdditionalContactEmailAddresses(java.util.Collection additionalContactEmailAddresses) {
if (additionalContactEmailAddresses == null) {
this.additionalContactEmailAddresses = null;
return;
}
this.additionalContactEmailAddresses = new java.util.ArrayList(additionalContactEmailAddresses);
}
/**
*
* Additional email addresses that you would like to be notified regarding Amazon SES matters.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdditionalContactEmailAddresses(java.util.Collection)} or
* {@link #withAdditionalContactEmailAddresses(java.util.Collection)} if you want to override the existing values.
*
*
* @param additionalContactEmailAddresses
* Additional email addresses that you would like to be notified regarding Amazon SES matters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutAccountDetailsRequest withAdditionalContactEmailAddresses(String... additionalContactEmailAddresses) {
if (this.additionalContactEmailAddresses == null) {
setAdditionalContactEmailAddresses(new java.util.ArrayList(additionalContactEmailAddresses.length));
}
for (String ele : additionalContactEmailAddresses) {
this.additionalContactEmailAddresses.add(ele);
}
return this;
}
/**
*
* Additional email addresses that you would like to be notified regarding Amazon SES matters.
*
*
* @param additionalContactEmailAddresses
* Additional email addresses that you would like to be notified regarding Amazon SES matters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutAccountDetailsRequest withAdditionalContactEmailAddresses(java.util.Collection additionalContactEmailAddresses) {
setAdditionalContactEmailAddresses(additionalContactEmailAddresses);
return this;
}
/**
*
* Indicates whether or not your account should have production access in the current Amazon Web Services Region.
*
*
* If the value is false
, then your account is in the sandbox. When your account is in the
* sandbox, you can only send email to verified identities.
*
*
* If the value is true
, then your account has production access. When your account has production
* access, you can send email to any address. The sending quota and maximum sending rate for your account vary based
* on your specific use case.
*
*
* @param productionAccessEnabled
* Indicates whether or not your account should have production access in the current Amazon Web Services
* Region.
*
* If the value is false
, then your account is in the sandbox. When your account is in
* the sandbox, you can only send email to verified identities.
*
*
* If the value is true
, then your account has production access. When your account has
* production access, you can send email to any address. The sending quota and maximum sending rate for your
* account vary based on your specific use case.
*/
public void setProductionAccessEnabled(Boolean productionAccessEnabled) {
this.productionAccessEnabled = productionAccessEnabled;
}
/**
*
* Indicates whether or not your account should have production access in the current Amazon Web Services Region.
*
*
* If the value is false
, then your account is in the sandbox. When your account is in the
* sandbox, you can only send email to verified identities.
*
*
* If the value is true
, then your account has production access. When your account has production
* access, you can send email to any address. The sending quota and maximum sending rate for your account vary based
* on your specific use case.
*
*
* @return Indicates whether or not your account should have production access in the current Amazon Web Services
* Region.
*
* If the value is false
, then your account is in the sandbox. When your account is in
* the sandbox, you can only send email to verified identities.
*
*
* If the value is true
, then your account has production access. When your account has
* production access, you can send email to any address. The sending quota and maximum sending rate for your
* account vary based on your specific use case.
*/
public Boolean getProductionAccessEnabled() {
return this.productionAccessEnabled;
}
/**
*
* Indicates whether or not your account should have production access in the current Amazon Web Services Region.
*
*
* If the value is false
, then your account is in the sandbox. When your account is in the
* sandbox, you can only send email to verified identities.
*
*
* If the value is true
, then your account has production access. When your account has production
* access, you can send email to any address. The sending quota and maximum sending rate for your account vary based
* on your specific use case.
*
*
* @param productionAccessEnabled
* Indicates whether or not your account should have production access in the current Amazon Web Services
* Region.
*
* If the value is false
, then your account is in the sandbox. When your account is in
* the sandbox, you can only send email to verified identities.
*
*
* If the value is true
, then your account has production access. When your account has
* production access, you can send email to any address. The sending quota and maximum sending rate for your
* account vary based on your specific use case.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutAccountDetailsRequest withProductionAccessEnabled(Boolean productionAccessEnabled) {
setProductionAccessEnabled(productionAccessEnabled);
return this;
}
/**
*
* Indicates whether or not your account should have production access in the current Amazon Web Services Region.
*
*
* If the value is false
, then your account is in the sandbox. When your account is in the
* sandbox, you can only send email to verified identities.
*
*
* If the value is true
, then your account has production access. When your account has production
* access, you can send email to any address. The sending quota and maximum sending rate for your account vary based
* on your specific use case.
*
*
* @return Indicates whether or not your account should have production access in the current Amazon Web Services
* Region.
*
* If the value is false
, then your account is in the sandbox. When your account is in
* the sandbox, you can only send email to verified identities.
*
*
* If the value is true
, then your account has production access. When your account has
* production access, you can send email to any address. The sending quota and maximum sending rate for your
* account vary based on your specific use case.
*/
public Boolean isProductionAccessEnabled() {
return this.productionAccessEnabled;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMailType() != null)
sb.append("MailType: ").append(getMailType()).append(",");
if (getWebsiteURL() != null)
sb.append("WebsiteURL: ").append("***Sensitive Data Redacted***").append(",");
if (getContactLanguage() != null)
sb.append("ContactLanguage: ").append(getContactLanguage()).append(",");
if (getUseCaseDescription() != null)
sb.append("UseCaseDescription: ").append("***Sensitive Data Redacted***").append(",");
if (getAdditionalContactEmailAddresses() != null)
sb.append("AdditionalContactEmailAddresses: ").append("***Sensitive Data Redacted***").append(",");
if (getProductionAccessEnabled() != null)
sb.append("ProductionAccessEnabled: ").append(getProductionAccessEnabled());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutAccountDetailsRequest == false)
return false;
PutAccountDetailsRequest other = (PutAccountDetailsRequest) obj;
if (other.getMailType() == null ^ this.getMailType() == null)
return false;
if (other.getMailType() != null && other.getMailType().equals(this.getMailType()) == false)
return false;
if (other.getWebsiteURL() == null ^ this.getWebsiteURL() == null)
return false;
if (other.getWebsiteURL() != null && other.getWebsiteURL().equals(this.getWebsiteURL()) == false)
return false;
if (other.getContactLanguage() == null ^ this.getContactLanguage() == null)
return false;
if (other.getContactLanguage() != null && other.getContactLanguage().equals(this.getContactLanguage()) == false)
return false;
if (other.getUseCaseDescription() == null ^ this.getUseCaseDescription() == null)
return false;
if (other.getUseCaseDescription() != null && other.getUseCaseDescription().equals(this.getUseCaseDescription()) == false)
return false;
if (other.getAdditionalContactEmailAddresses() == null ^ this.getAdditionalContactEmailAddresses() == null)
return false;
if (other.getAdditionalContactEmailAddresses() != null
&& other.getAdditionalContactEmailAddresses().equals(this.getAdditionalContactEmailAddresses()) == false)
return false;
if (other.getProductionAccessEnabled() == null ^ this.getProductionAccessEnabled() == null)
return false;
if (other.getProductionAccessEnabled() != null && other.getProductionAccessEnabled().equals(this.getProductionAccessEnabled()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMailType() == null) ? 0 : getMailType().hashCode());
hashCode = prime * hashCode + ((getWebsiteURL() == null) ? 0 : getWebsiteURL().hashCode());
hashCode = prime * hashCode + ((getContactLanguage() == null) ? 0 : getContactLanguage().hashCode());
hashCode = prime * hashCode + ((getUseCaseDescription() == null) ? 0 : getUseCaseDescription().hashCode());
hashCode = prime * hashCode + ((getAdditionalContactEmailAddresses() == null) ? 0 : getAdditionalContactEmailAddresses().hashCode());
hashCode = prime * hashCode + ((getProductionAccessEnabled() == null) ? 0 : getProductionAccessEnabled().hashCode());
return hashCode;
}
@Override
public PutAccountDetailsRequest clone() {
return (PutAccountDetailsRequest) super.clone();
}
}