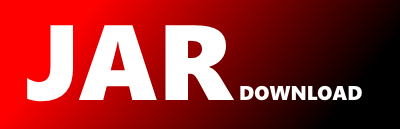
com.amazonaws.services.simpleemailv2.model.VerificationInfo Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sesv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simpleemailv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An object that contains additional information about the verification status for the identity.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class VerificationInfo implements Serializable, Cloneable, StructuredPojo {
/**
*
* The last time a verification attempt was made for this identity.
*
*/
private java.util.Date lastCheckedTimestamp;
/**
*
* The last time a successful verification was made for this identity.
*
*/
private java.util.Date lastSuccessTimestamp;
/**
*
* Provides the reason for the failure describing why Amazon SES was not able to successfully verify the identity.
* Below are the possible values:
*
*
* -
*
* INVALID_VALUE
– Amazon SES was able to find the record, but the value contained within the record
* was invalid. Ensure you have published the correct values for the record.
*
*
* -
*
* TYPE_NOT_FOUND
– The queried hostname exists but does not have the requested type of DNS record.
* Ensure that you have published the correct type of DNS record.
*
*
* -
*
* HOST_NOT_FOUND
– The queried hostname does not exist or was not reachable at the time of the
* request. Ensure that you have published the required DNS record(s).
*
*
* -
*
* SERVICE_ERROR
– A temporary issue is preventing Amazon SES from determining the verification status
* of the domain.
*
*
* -
*
* DNS_SERVER_ERROR
– The DNS server encountered an issue and was unable to complete the request.
*
*
*
*/
private String errorType;
/**
*
* An object that contains information about the start of authority (SOA) record associated with the identity.
*
*/
private SOARecord sOARecord;
/**
*
* The last time a verification attempt was made for this identity.
*
*
* @param lastCheckedTimestamp
* The last time a verification attempt was made for this identity.
*/
public void setLastCheckedTimestamp(java.util.Date lastCheckedTimestamp) {
this.lastCheckedTimestamp = lastCheckedTimestamp;
}
/**
*
* The last time a verification attempt was made for this identity.
*
*
* @return The last time a verification attempt was made for this identity.
*/
public java.util.Date getLastCheckedTimestamp() {
return this.lastCheckedTimestamp;
}
/**
*
* The last time a verification attempt was made for this identity.
*
*
* @param lastCheckedTimestamp
* The last time a verification attempt was made for this identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public VerificationInfo withLastCheckedTimestamp(java.util.Date lastCheckedTimestamp) {
setLastCheckedTimestamp(lastCheckedTimestamp);
return this;
}
/**
*
* The last time a successful verification was made for this identity.
*
*
* @param lastSuccessTimestamp
* The last time a successful verification was made for this identity.
*/
public void setLastSuccessTimestamp(java.util.Date lastSuccessTimestamp) {
this.lastSuccessTimestamp = lastSuccessTimestamp;
}
/**
*
* The last time a successful verification was made for this identity.
*
*
* @return The last time a successful verification was made for this identity.
*/
public java.util.Date getLastSuccessTimestamp() {
return this.lastSuccessTimestamp;
}
/**
*
* The last time a successful verification was made for this identity.
*
*
* @param lastSuccessTimestamp
* The last time a successful verification was made for this identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public VerificationInfo withLastSuccessTimestamp(java.util.Date lastSuccessTimestamp) {
setLastSuccessTimestamp(lastSuccessTimestamp);
return this;
}
/**
*
* Provides the reason for the failure describing why Amazon SES was not able to successfully verify the identity.
* Below are the possible values:
*
*
* -
*
* INVALID_VALUE
– Amazon SES was able to find the record, but the value contained within the record
* was invalid. Ensure you have published the correct values for the record.
*
*
* -
*
* TYPE_NOT_FOUND
– The queried hostname exists but does not have the requested type of DNS record.
* Ensure that you have published the correct type of DNS record.
*
*
* -
*
* HOST_NOT_FOUND
– The queried hostname does not exist or was not reachable at the time of the
* request. Ensure that you have published the required DNS record(s).
*
*
* -
*
* SERVICE_ERROR
– A temporary issue is preventing Amazon SES from determining the verification status
* of the domain.
*
*
* -
*
* DNS_SERVER_ERROR
– The DNS server encountered an issue and was unable to complete the request.
*
*
*
*
* @param errorType
* Provides the reason for the failure describing why Amazon SES was not able to successfully verify the
* identity. Below are the possible values:
*
* -
*
* INVALID_VALUE
– Amazon SES was able to find the record, but the value contained within the
* record was invalid. Ensure you have published the correct values for the record.
*
*
* -
*
* TYPE_NOT_FOUND
– The queried hostname exists but does not have the requested type of DNS
* record. Ensure that you have published the correct type of DNS record.
*
*
* -
*
* HOST_NOT_FOUND
– The queried hostname does not exist or was not reachable at the time of the
* request. Ensure that you have published the required DNS record(s).
*
*
* -
*
* SERVICE_ERROR
– A temporary issue is preventing Amazon SES from determining the verification
* status of the domain.
*
*
* -
*
* DNS_SERVER_ERROR
– The DNS server encountered an issue and was unable to complete the
* request.
*
*
* @see VerificationError
*/
public void setErrorType(String errorType) {
this.errorType = errorType;
}
/**
*
* Provides the reason for the failure describing why Amazon SES was not able to successfully verify the identity.
* Below are the possible values:
*
*
* -
*
* INVALID_VALUE
– Amazon SES was able to find the record, but the value contained within the record
* was invalid. Ensure you have published the correct values for the record.
*
*
* -
*
* TYPE_NOT_FOUND
– The queried hostname exists but does not have the requested type of DNS record.
* Ensure that you have published the correct type of DNS record.
*
*
* -
*
* HOST_NOT_FOUND
– The queried hostname does not exist or was not reachable at the time of the
* request. Ensure that you have published the required DNS record(s).
*
*
* -
*
* SERVICE_ERROR
– A temporary issue is preventing Amazon SES from determining the verification status
* of the domain.
*
*
* -
*
* DNS_SERVER_ERROR
– The DNS server encountered an issue and was unable to complete the request.
*
*
*
*
* @return Provides the reason for the failure describing why Amazon SES was not able to successfully verify the
* identity. Below are the possible values:
*
* -
*
* INVALID_VALUE
– Amazon SES was able to find the record, but the value contained within the
* record was invalid. Ensure you have published the correct values for the record.
*
*
* -
*
* TYPE_NOT_FOUND
– The queried hostname exists but does not have the requested type of DNS
* record. Ensure that you have published the correct type of DNS record.
*
*
* -
*
* HOST_NOT_FOUND
– The queried hostname does not exist or was not reachable at the time of the
* request. Ensure that you have published the required DNS record(s).
*
*
* -
*
* SERVICE_ERROR
– A temporary issue is preventing Amazon SES from determining the verification
* status of the domain.
*
*
* -
*
* DNS_SERVER_ERROR
– The DNS server encountered an issue and was unable to complete the
* request.
*
*
* @see VerificationError
*/
public String getErrorType() {
return this.errorType;
}
/**
*
* Provides the reason for the failure describing why Amazon SES was not able to successfully verify the identity.
* Below are the possible values:
*
*
* -
*
* INVALID_VALUE
– Amazon SES was able to find the record, but the value contained within the record
* was invalid. Ensure you have published the correct values for the record.
*
*
* -
*
* TYPE_NOT_FOUND
– The queried hostname exists but does not have the requested type of DNS record.
* Ensure that you have published the correct type of DNS record.
*
*
* -
*
* HOST_NOT_FOUND
– The queried hostname does not exist or was not reachable at the time of the
* request. Ensure that you have published the required DNS record(s).
*
*
* -
*
* SERVICE_ERROR
– A temporary issue is preventing Amazon SES from determining the verification status
* of the domain.
*
*
* -
*
* DNS_SERVER_ERROR
– The DNS server encountered an issue and was unable to complete the request.
*
*
*
*
* @param errorType
* Provides the reason for the failure describing why Amazon SES was not able to successfully verify the
* identity. Below are the possible values:
*
* -
*
* INVALID_VALUE
– Amazon SES was able to find the record, but the value contained within the
* record was invalid. Ensure you have published the correct values for the record.
*
*
* -
*
* TYPE_NOT_FOUND
– The queried hostname exists but does not have the requested type of DNS
* record. Ensure that you have published the correct type of DNS record.
*
*
* -
*
* HOST_NOT_FOUND
– The queried hostname does not exist or was not reachable at the time of the
* request. Ensure that you have published the required DNS record(s).
*
*
* -
*
* SERVICE_ERROR
– A temporary issue is preventing Amazon SES from determining the verification
* status of the domain.
*
*
* -
*
* DNS_SERVER_ERROR
– The DNS server encountered an issue and was unable to complete the
* request.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see VerificationError
*/
public VerificationInfo withErrorType(String errorType) {
setErrorType(errorType);
return this;
}
/**
*
* Provides the reason for the failure describing why Amazon SES was not able to successfully verify the identity.
* Below are the possible values:
*
*
* -
*
* INVALID_VALUE
– Amazon SES was able to find the record, but the value contained within the record
* was invalid. Ensure you have published the correct values for the record.
*
*
* -
*
* TYPE_NOT_FOUND
– The queried hostname exists but does not have the requested type of DNS record.
* Ensure that you have published the correct type of DNS record.
*
*
* -
*
* HOST_NOT_FOUND
– The queried hostname does not exist or was not reachable at the time of the
* request. Ensure that you have published the required DNS record(s).
*
*
* -
*
* SERVICE_ERROR
– A temporary issue is preventing Amazon SES from determining the verification status
* of the domain.
*
*
* -
*
* DNS_SERVER_ERROR
– The DNS server encountered an issue and was unable to complete the request.
*
*
*
*
* @param errorType
* Provides the reason for the failure describing why Amazon SES was not able to successfully verify the
* identity. Below are the possible values:
*
* -
*
* INVALID_VALUE
– Amazon SES was able to find the record, but the value contained within the
* record was invalid. Ensure you have published the correct values for the record.
*
*
* -
*
* TYPE_NOT_FOUND
– The queried hostname exists but does not have the requested type of DNS
* record. Ensure that you have published the correct type of DNS record.
*
*
* -
*
* HOST_NOT_FOUND
– The queried hostname does not exist or was not reachable at the time of the
* request. Ensure that you have published the required DNS record(s).
*
*
* -
*
* SERVICE_ERROR
– A temporary issue is preventing Amazon SES from determining the verification
* status of the domain.
*
*
* -
*
* DNS_SERVER_ERROR
– The DNS server encountered an issue and was unable to complete the
* request.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see VerificationError
*/
public VerificationInfo withErrorType(VerificationError errorType) {
this.errorType = errorType.toString();
return this;
}
/**
*
* An object that contains information about the start of authority (SOA) record associated with the identity.
*
*
* @param sOARecord
* An object that contains information about the start of authority (SOA) record associated with the
* identity.
*/
public void setSOARecord(SOARecord sOARecord) {
this.sOARecord = sOARecord;
}
/**
*
* An object that contains information about the start of authority (SOA) record associated with the identity.
*
*
* @return An object that contains information about the start of authority (SOA) record associated with the
* identity.
*/
public SOARecord getSOARecord() {
return this.sOARecord;
}
/**
*
* An object that contains information about the start of authority (SOA) record associated with the identity.
*
*
* @param sOARecord
* An object that contains information about the start of authority (SOA) record associated with the
* identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public VerificationInfo withSOARecord(SOARecord sOARecord) {
setSOARecord(sOARecord);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getLastCheckedTimestamp() != null)
sb.append("LastCheckedTimestamp: ").append(getLastCheckedTimestamp()).append(",");
if (getLastSuccessTimestamp() != null)
sb.append("LastSuccessTimestamp: ").append(getLastSuccessTimestamp()).append(",");
if (getErrorType() != null)
sb.append("ErrorType: ").append(getErrorType()).append(",");
if (getSOARecord() != null)
sb.append("SOARecord: ").append(getSOARecord());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof VerificationInfo == false)
return false;
VerificationInfo other = (VerificationInfo) obj;
if (other.getLastCheckedTimestamp() == null ^ this.getLastCheckedTimestamp() == null)
return false;
if (other.getLastCheckedTimestamp() != null && other.getLastCheckedTimestamp().equals(this.getLastCheckedTimestamp()) == false)
return false;
if (other.getLastSuccessTimestamp() == null ^ this.getLastSuccessTimestamp() == null)
return false;
if (other.getLastSuccessTimestamp() != null && other.getLastSuccessTimestamp().equals(this.getLastSuccessTimestamp()) == false)
return false;
if (other.getErrorType() == null ^ this.getErrorType() == null)
return false;
if (other.getErrorType() != null && other.getErrorType().equals(this.getErrorType()) == false)
return false;
if (other.getSOARecord() == null ^ this.getSOARecord() == null)
return false;
if (other.getSOARecord() != null && other.getSOARecord().equals(this.getSOARecord()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getLastCheckedTimestamp() == null) ? 0 : getLastCheckedTimestamp().hashCode());
hashCode = prime * hashCode + ((getLastSuccessTimestamp() == null) ? 0 : getLastSuccessTimestamp().hashCode());
hashCode = prime * hashCode + ((getErrorType() == null) ? 0 : getErrorType().hashCode());
hashCode = prime * hashCode + ((getSOARecord() == null) ? 0 : getSOARecord().hashCode());
return hashCode;
}
@Override
public VerificationInfo clone() {
try {
return (VerificationInfo) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simpleemailv2.model.transform.VerificationInfoMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}