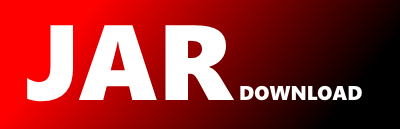
com.amazonaws.services.signer.AWSsignerAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-signer Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.signer;
import javax.annotation.Generated;
import com.amazonaws.services.signer.model.*;
/**
* Interface for accessing signer asynchronously. Each asynchronous method will return a Java Future object representing
* the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive notification when
* an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.signer.AbstractAWSsignerAsync} instead.
*
*
*
* You can use Code Signing for Amazon FreeRTOS (AWS Signer) to sign code that you created for any of the IoT devices
* that Amazon Web Services supports. AWS Signer is integrated with Amazon FreeRTOS, AWS Certificate Manager, and AWS
* CloudTrail. Amazon FreeRTOS customers can use AWS Signer to sign code images before making them available for
* microcontrollers. You can use ACM to import third-party certificates to be used by AWS Signer. For general
* information about using AWS Signer, see the Code Signing for Amazon FreeRTOS
* Developer Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSsignerAsync extends AWSsigner {
/**
*
* Changes the state of an ACTIVE
signing profile to CANCELED
. A canceled profile is still
* viewable with the ListSigningProfiles
operation, but it cannot perform new signing jobs, and is
* deleted two years after cancelation.
*
*
* @param cancelSigningProfileRequest
* @return A Java Future containing the result of the CancelSigningProfile operation returned by the service.
* @sample AWSsignerAsync.CancelSigningProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSigningProfileAsync(CancelSigningProfileRequest cancelSigningProfileRequest);
/**
*
* Changes the state of an ACTIVE
signing profile to CANCELED
. A canceled profile is still
* viewable with the ListSigningProfiles
operation, but it cannot perform new signing jobs, and is
* deleted two years after cancelation.
*
*
* @param cancelSigningProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelSigningProfile operation returned by the service.
* @sample AWSsignerAsyncHandler.CancelSigningProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSigningProfileAsync(CancelSigningProfileRequest cancelSigningProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a specific code signing job. You specify the job by using the jobId
value
* that is returned by the StartSigningJob operation.
*
*
* @param describeSigningJobRequest
* @return A Java Future containing the result of the DescribeSigningJob operation returned by the service.
* @sample AWSsignerAsync.DescribeSigningJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSigningJobAsync(DescribeSigningJobRequest describeSigningJobRequest);
/**
*
* Returns information about a specific code signing job. You specify the job by using the jobId
value
* that is returned by the StartSigningJob operation.
*
*
* @param describeSigningJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSigningJob operation returned by the service.
* @sample AWSsignerAsyncHandler.DescribeSigningJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSigningJobAsync(DescribeSigningJobRequest describeSigningJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information on a specific signing platform.
*
*
* @param getSigningPlatformRequest
* @return A Java Future containing the result of the GetSigningPlatform operation returned by the service.
* @sample AWSsignerAsync.GetSigningPlatform
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSigningPlatformAsync(GetSigningPlatformRequest getSigningPlatformRequest);
/**
*
* Returns information on a specific signing platform.
*
*
* @param getSigningPlatformRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSigningPlatform operation returned by the service.
* @sample AWSsignerAsyncHandler.GetSigningPlatform
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSigningPlatformAsync(GetSigningPlatformRequest getSigningPlatformRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information on a specific signing profile.
*
*
* @param getSigningProfileRequest
* @return A Java Future containing the result of the GetSigningProfile operation returned by the service.
* @sample AWSsignerAsync.GetSigningProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSigningProfileAsync(GetSigningProfileRequest getSigningProfileRequest);
/**
*
* Returns information on a specific signing profile.
*
*
* @param getSigningProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSigningProfile operation returned by the service.
* @sample AWSsignerAsyncHandler.GetSigningProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSigningProfileAsync(GetSigningProfileRequest getSigningProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all your signing jobs. You can use the maxResults
parameter to limit the number of signing
* jobs that are returned in the response. If additional jobs remain to be listed, AWS Signer returns a
* nextToken
value. Use this value in subsequent calls to ListSigningJobs
to fetch the
* remaining values. You can continue calling ListSigningJobs
with your maxResults
* parameter and with new values that AWS Signer returns in the nextToken
parameter until all of your
* signing jobs have been returned.
*
*
* @param listSigningJobsRequest
* @return A Java Future containing the result of the ListSigningJobs operation returned by the service.
* @sample AWSsignerAsync.ListSigningJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSigningJobsAsync(ListSigningJobsRequest listSigningJobsRequest);
/**
*
* Lists all your signing jobs. You can use the maxResults
parameter to limit the number of signing
* jobs that are returned in the response. If additional jobs remain to be listed, AWS Signer returns a
* nextToken
value. Use this value in subsequent calls to ListSigningJobs
to fetch the
* remaining values. You can continue calling ListSigningJobs
with your maxResults
* parameter and with new values that AWS Signer returns in the nextToken
parameter until all of your
* signing jobs have been returned.
*
*
* @param listSigningJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSigningJobs operation returned by the service.
* @sample AWSsignerAsyncHandler.ListSigningJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSigningJobsAsync(ListSigningJobsRequest listSigningJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all signing platforms available in AWS Signer that match the request parameters. If additional jobs remain
* to be listed, AWS Signer returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that AWS Signer returns in the
* nextToken
parameter until all of your signing jobs have been returned.
*
*
* @param listSigningPlatformsRequest
* @return A Java Future containing the result of the ListSigningPlatforms operation returned by the service.
* @sample AWSsignerAsync.ListSigningPlatforms
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSigningPlatformsAsync(ListSigningPlatformsRequest listSigningPlatformsRequest);
/**
*
* Lists all signing platforms available in AWS Signer that match the request parameters. If additional jobs remain
* to be listed, AWS Signer returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that AWS Signer returns in the
* nextToken
parameter until all of your signing jobs have been returned.
*
*
* @param listSigningPlatformsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSigningPlatforms operation returned by the service.
* @sample AWSsignerAsyncHandler.ListSigningPlatforms
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSigningPlatformsAsync(ListSigningPlatformsRequest listSigningPlatformsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all available signing profiles in your AWS account. Returns only profiles with an ACTIVE
* status unless the includeCanceled
request field is set to true
. If additional jobs
* remain to be listed, AWS Signer returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that AWS Signer returns in the
* nextToken
parameter until all of your signing jobs have been returned.
*
*
* @param listSigningProfilesRequest
* @return A Java Future containing the result of the ListSigningProfiles operation returned by the service.
* @sample AWSsignerAsync.ListSigningProfiles
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSigningProfilesAsync(ListSigningProfilesRequest listSigningProfilesRequest);
/**
*
* Lists all available signing profiles in your AWS account. Returns only profiles with an ACTIVE
* status unless the includeCanceled
request field is set to true
. If additional jobs
* remain to be listed, AWS Signer returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that AWS Signer returns in the
* nextToken
parameter until all of your signing jobs have been returned.
*
*
* @param listSigningProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSigningProfiles operation returned by the service.
* @sample AWSsignerAsyncHandler.ListSigningProfiles
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSigningProfilesAsync(ListSigningProfilesRequest listSigningProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a signing profile. A signing profile is an AWS Signer template that can be used to carry out a
* pre-defined signing job. For more information, see http://docs.aws.amazon.com/signer/latest/developerguide/gs-profile.html
*
*
* @param putSigningProfileRequest
* @return A Java Future containing the result of the PutSigningProfile operation returned by the service.
* @sample AWSsignerAsync.PutSigningProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putSigningProfileAsync(PutSigningProfileRequest putSigningProfileRequest);
/**
*
* Creates a signing profile. A signing profile is an AWS Signer template that can be used to carry out a
* pre-defined signing job. For more information, see http://docs.aws.amazon.com/signer/latest/developerguide/gs-profile.html
*
*
* @param putSigningProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutSigningProfile operation returned by the service.
* @sample AWSsignerAsyncHandler.PutSigningProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putSigningProfileAsync(PutSigningProfileRequest putSigningProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates a signing job to be performed on the code provided. Signing jobs are viewable by the
* ListSigningJobs
operation for two years after they are performed. Note the following requirements:
*
*
* -
*
* You must create an Amazon S3 source bucket. For more information, see Create a Bucket in the Amazon
* S3 Getting Started Guide.
*
*
* -
*
* Your S3 source bucket must be version enabled.
*
*
* -
*
* You must create an S3 destination bucket. AWS Signer uses your S3 destination bucket to write your signed code.
*
*
* -
*
* You specify the name of the source and destination buckets when calling the StartSigningJob
* operation.
*
*
* -
*
* You must also specify a request token that identifies your request to AWS Signer.
*
*
*
*
* You can call the DescribeSigningJob and the ListSigningJobs actions after you call
* StartSigningJob
.
*
*
* For a Java example that shows how to use this action, see http://docs.aws.amazon.com/acm/latest/userguide/
*
*
* @param startSigningJobRequest
* @return A Java Future containing the result of the StartSigningJob operation returned by the service.
* @sample AWSsignerAsync.StartSigningJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startSigningJobAsync(StartSigningJobRequest startSigningJobRequest);
/**
*
* Initiates a signing job to be performed on the code provided. Signing jobs are viewable by the
* ListSigningJobs
operation for two years after they are performed. Note the following requirements:
*
*
* -
*
* You must create an Amazon S3 source bucket. For more information, see Create a Bucket in the Amazon
* S3 Getting Started Guide.
*
*
* -
*
* Your S3 source bucket must be version enabled.
*
*
* -
*
* You must create an S3 destination bucket. AWS Signer uses your S3 destination bucket to write your signed code.
*
*
* -
*
* You specify the name of the source and destination buckets when calling the StartSigningJob
* operation.
*
*
* -
*
* You must also specify a request token that identifies your request to AWS Signer.
*
*
*
*
* You can call the DescribeSigningJob and the ListSigningJobs actions after you call
* StartSigningJob
.
*
*
* For a Java example that shows how to use this action, see http://docs.aws.amazon.com/acm/latest/userguide/
*
*
* @param startSigningJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartSigningJob operation returned by the service.
* @sample AWSsignerAsyncHandler.StartSigningJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startSigningJobAsync(StartSigningJobRequest startSigningJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}