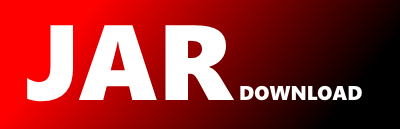
com.amazonaws.services.simpleworkflow.model.HistoryEvent Maven / Gradle / Ivy
Show all versions of aws-java-sdk-simpleworkflow Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simpleworkflow.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Event within a workflow execution. A history event can be one of these types:
*
*
* -
*
* ActivityTaskCancelRequested
– A RequestCancelActivityTask
decision was received by the
* system.
*
*
* -
*
* ActivityTaskCanceled
– The activity task was successfully canceled.
*
*
* -
*
* ActivityTaskCompleted
– An activity worker successfully completed an activity task by calling
* RespondActivityTaskCompleted.
*
*
* -
*
* ActivityTaskFailed
– An activity worker failed an activity task by calling
* RespondActivityTaskFailed.
*
*
* -
*
* ActivityTaskScheduled
– An activity task was scheduled for execution.
*
*
* -
*
* ActivityTaskStarted
– The scheduled activity task was dispatched to a worker.
*
*
* -
*
* ActivityTaskTimedOut
– The activity task timed out.
*
*
* -
*
* CancelTimerFailed
– Failed to process CancelTimer decision. This happens when the decision isn't
* configured properly, for example no timer exists with the specified timer Id.
*
*
* -
*
* CancelWorkflowExecutionFailed
– A request to cancel a workflow execution failed.
*
*
* -
*
* ChildWorkflowExecutionCanceled
– A child workflow execution, started by this workflow execution, was
* canceled and closed.
*
*
* -
*
* ChildWorkflowExecutionCompleted
– A child workflow execution, started by this workflow execution,
* completed successfully and was closed.
*
*
* -
*
* ChildWorkflowExecutionFailed
– A child workflow execution, started by this workflow execution, failed to
* complete successfully and was closed.
*
*
* -
*
* ChildWorkflowExecutionStarted
– A child workflow execution was successfully started.
*
*
* -
*
* ChildWorkflowExecutionTerminated
– A child workflow execution, started by this workflow execution, was
* terminated.
*
*
* -
*
* ChildWorkflowExecutionTimedOut
– A child workflow execution, started by this workflow execution, timed
* out and was closed.
*
*
* -
*
* CompleteWorkflowExecutionFailed
– The workflow execution failed to complete.
*
*
* -
*
* ContinueAsNewWorkflowExecutionFailed
– The workflow execution failed to complete after being continued
* as a new workflow execution.
*
*
* -
*
* DecisionTaskCompleted
– The decider successfully completed a decision task by calling
* RespondDecisionTaskCompleted.
*
*
* -
*
* DecisionTaskScheduled
– A decision task was scheduled for the workflow execution.
*
*
* -
*
* DecisionTaskStarted
– The decision task was dispatched to a decider.
*
*
* -
*
* DecisionTaskTimedOut
– The decision task timed out.
*
*
* -
*
* ExternalWorkflowExecutionCancelRequested
– Request to cancel an external workflow execution was
* successfully delivered to the target execution.
*
*
* -
*
* ExternalWorkflowExecutionSignaled
– A signal, requested by this workflow execution, was successfully
* delivered to the target external workflow execution.
*
*
* -
*
* FailWorkflowExecutionFailed
– A request to mark a workflow execution as failed, itself failed.
*
*
* -
*
* MarkerRecorded
– A marker was recorded in the workflow history as the result of a
* RecordMarker
decision.
*
*
* -
*
* RecordMarkerFailed
– A RecordMarker
decision was returned as failed.
*
*
* -
*
* RequestCancelActivityTaskFailed
– Failed to process RequestCancelActivityTask decision. This happens
* when the decision isn't configured properly.
*
*
* -
*
* RequestCancelExternalWorkflowExecutionFailed
– Request to cancel an external workflow execution failed.
*
*
* -
*
* RequestCancelExternalWorkflowExecutionInitiated
– A request was made to request the cancellation of an
* external workflow execution.
*
*
* -
*
* ScheduleActivityTaskFailed
– Failed to process ScheduleActivityTask decision. This happens when the
* decision isn't configured properly, for example the activity type specified isn't registered.
*
*
* -
*
* SignalExternalWorkflowExecutionFailed
– The request to signal an external workflow execution failed.
*
*
* -
*
* SignalExternalWorkflowExecutionInitiated
– A request to signal an external workflow was made.
*
*
* -
*
* StartActivityTaskFailed
– A scheduled activity task failed to start.
*
*
* -
*
* StartChildWorkflowExecutionFailed
– Failed to process StartChildWorkflowExecution decision. This happens
* when the decision isn't configured properly, for example the workflow type specified isn't registered.
*
*
* -
*
* StartChildWorkflowExecutionInitiated
– A request was made to start a child workflow execution.
*
*
* -
*
* StartTimerFailed
– Failed to process StartTimer decision. This happens when the decision isn't
* configured properly, for example a timer already exists with the specified timer Id.
*
*
* -
*
* TimerCanceled
– A timer, previously started for this workflow execution, was successfully canceled.
*
*
* -
*
* TimerFired
– A timer, previously started for this workflow execution, fired.
*
*
* -
*
* TimerStarted
– A timer was started for the workflow execution due to a StartTimer
decision.
*
*
* -
*
* WorkflowExecutionCancelRequested
– A request to cancel this workflow execution was made.
*
*
* -
*
* WorkflowExecutionCanceled
– The workflow execution was successfully canceled and closed.
*
*
* -
*
* WorkflowExecutionCompleted
– The workflow execution was closed due to successful completion.
*
*
* -
*
* WorkflowExecutionContinuedAsNew
– The workflow execution was closed and a new execution of the same type
* was created with the same workflowId.
*
*
* -
*
* WorkflowExecutionFailed
– The workflow execution closed due to a failure.
*
*
* -
*
* WorkflowExecutionSignaled
– An external signal was received for the workflow execution.
*
*
* -
*
* WorkflowExecutionStarted
– The workflow execution was started.
*
*
* -
*
* WorkflowExecutionTerminated
– The workflow execution was terminated.
*
*
* -
*
* WorkflowExecutionTimedOut
– The workflow execution was closed because a time out was exceeded.
*
*
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class HistoryEvent implements Serializable, Cloneable, StructuredPojo {
/**
*
* The date and time when the event occurred.
*
*/
private java.util.Date eventTimestamp;
/**
*
* The type of the history event.
*
*/
private String eventType;
/**
*
* The system generated ID of the event. This ID uniquely identifies the event with in the workflow execution
* history.
*
*/
private Long eventId;
/**
*
* If the event is of type WorkflowExecutionStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private WorkflowExecutionStartedEventAttributes workflowExecutionStartedEventAttributes;
/**
*
* If the event is of type WorkflowExecutionCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private WorkflowExecutionCompletedEventAttributes workflowExecutionCompletedEventAttributes;
/**
*
* If the event is of type CompleteWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private CompleteWorkflowExecutionFailedEventAttributes completeWorkflowExecutionFailedEventAttributes;
/**
*
* If the event is of type WorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private WorkflowExecutionFailedEventAttributes workflowExecutionFailedEventAttributes;
/**
*
* If the event is of type FailWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private FailWorkflowExecutionFailedEventAttributes failWorkflowExecutionFailedEventAttributes;
/**
*
* If the event is of type WorkflowExecutionTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private WorkflowExecutionTimedOutEventAttributes workflowExecutionTimedOutEventAttributes;
/**
*
* If the event is of type WorkflowExecutionCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private WorkflowExecutionCanceledEventAttributes workflowExecutionCanceledEventAttributes;
/**
*
* If the event is of type CancelWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private CancelWorkflowExecutionFailedEventAttributes cancelWorkflowExecutionFailedEventAttributes;
/**
*
* If the event is of type WorkflowExecutionContinuedAsNew
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private WorkflowExecutionContinuedAsNewEventAttributes workflowExecutionContinuedAsNewEventAttributes;
/**
*
* If the event is of type ContinueAsNewWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private ContinueAsNewWorkflowExecutionFailedEventAttributes continueAsNewWorkflowExecutionFailedEventAttributes;
/**
*
* If the event is of type WorkflowExecutionTerminated
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private WorkflowExecutionTerminatedEventAttributes workflowExecutionTerminatedEventAttributes;
/**
*
* If the event is of type WorkflowExecutionCancelRequested
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private WorkflowExecutionCancelRequestedEventAttributes workflowExecutionCancelRequestedEventAttributes;
/**
*
* If the event is of type DecisionTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private DecisionTaskScheduledEventAttributes decisionTaskScheduledEventAttributes;
/**
*
* If the event is of type DecisionTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private DecisionTaskStartedEventAttributes decisionTaskStartedEventAttributes;
/**
*
* If the event is of type DecisionTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private DecisionTaskCompletedEventAttributes decisionTaskCompletedEventAttributes;
/**
*
* If the event is of type DecisionTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private DecisionTaskTimedOutEventAttributes decisionTaskTimedOutEventAttributes;
/**
*
* If the event is of type ActivityTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ActivityTaskScheduledEventAttributes activityTaskScheduledEventAttributes;
/**
*
* If the event is of type ActivityTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ActivityTaskStartedEventAttributes activityTaskStartedEventAttributes;
/**
*
* If the event is of type ActivityTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ActivityTaskCompletedEventAttributes activityTaskCompletedEventAttributes;
/**
*
* If the event is of type ActivityTaskFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*/
private ActivityTaskFailedEventAttributes activityTaskFailedEventAttributes;
/**
*
* If the event is of type ActivityTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ActivityTaskTimedOutEventAttributes activityTaskTimedOutEventAttributes;
/**
*
* If the event is of type ActivityTaskCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ActivityTaskCanceledEventAttributes activityTaskCanceledEventAttributes;
/**
*
* If the event is of type ActivityTaskcancelRequested
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ActivityTaskCancelRequestedEventAttributes activityTaskCancelRequestedEventAttributes;
/**
*
* If the event is of type WorkflowExecutionSignaled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private WorkflowExecutionSignaledEventAttributes workflowExecutionSignaledEventAttributes;
/**
*
* If the event is of type MarkerRecorded
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*/
private MarkerRecordedEventAttributes markerRecordedEventAttributes;
/**
*
* If the event is of type DecisionTaskFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*/
private RecordMarkerFailedEventAttributes recordMarkerFailedEventAttributes;
/**
*
* If the event is of type TimerStarted
then this member is set and provides detailed information about
* the event. It isn't set for other event types.
*
*/
private TimerStartedEventAttributes timerStartedEventAttributes;
/**
*
* If the event is of type TimerFired
then this member is set and provides detailed information about
* the event. It isn't set for other event types.
*
*/
private TimerFiredEventAttributes timerFiredEventAttributes;
/**
*
* If the event is of type TimerCanceled
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*/
private TimerCanceledEventAttributes timerCanceledEventAttributes;
/**
*
* If the event is of type StartChildWorkflowExecutionInitiated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private StartChildWorkflowExecutionInitiatedEventAttributes startChildWorkflowExecutionInitiatedEventAttributes;
/**
*
* If the event is of type ChildWorkflowExecutionStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ChildWorkflowExecutionStartedEventAttributes childWorkflowExecutionStartedEventAttributes;
/**
*
* If the event is of type ChildWorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private ChildWorkflowExecutionCompletedEventAttributes childWorkflowExecutionCompletedEventAttributes;
/**
*
* If the event is of type ChildWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ChildWorkflowExecutionFailedEventAttributes childWorkflowExecutionFailedEventAttributes;
/**
*
* If the event is of type ChildWorkflowExecutionTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ChildWorkflowExecutionTimedOutEventAttributes childWorkflowExecutionTimedOutEventAttributes;
/**
*
* If the event is of type ChildWorkflowExecutionCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ChildWorkflowExecutionCanceledEventAttributes childWorkflowExecutionCanceledEventAttributes;
/**
*
* If the event is of type ChildWorkflowExecutionTerminated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private ChildWorkflowExecutionTerminatedEventAttributes childWorkflowExecutionTerminatedEventAttributes;
/**
*
* If the event is of type SignalExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*/
private SignalExternalWorkflowExecutionInitiatedEventAttributes signalExternalWorkflowExecutionInitiatedEventAttributes;
/**
*
* If the event is of type ExternalWorkflowExecutionSignaled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private ExternalWorkflowExecutionSignaledEventAttributes externalWorkflowExecutionSignaledEventAttributes;
/**
*
* If the event is of type SignalExternalWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private SignalExternalWorkflowExecutionFailedEventAttributes signalExternalWorkflowExecutionFailedEventAttributes;
/**
*
* If the event is of type ExternalWorkflowExecutionCancelRequested
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*/
private ExternalWorkflowExecutionCancelRequestedEventAttributes externalWorkflowExecutionCancelRequestedEventAttributes;
/**
*
* If the event is of type RequestCancelExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*/
private RequestCancelExternalWorkflowExecutionInitiatedEventAttributes requestCancelExternalWorkflowExecutionInitiatedEventAttributes;
/**
*
* If the event is of type RequestCancelExternalWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*/
private RequestCancelExternalWorkflowExecutionFailedEventAttributes requestCancelExternalWorkflowExecutionFailedEventAttributes;
/**
*
* If the event is of type ScheduleActivityTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*/
private ScheduleActivityTaskFailedEventAttributes scheduleActivityTaskFailedEventAttributes;
/**
*
* If the event is of type RequestCancelActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private RequestCancelActivityTaskFailedEventAttributes requestCancelActivityTaskFailedEventAttributes;
/**
*
* If the event is of type StartTimerFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*/
private StartTimerFailedEventAttributes startTimerFailedEventAttributes;
/**
*
* If the event is of type CancelTimerFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*/
private CancelTimerFailedEventAttributes cancelTimerFailedEventAttributes;
/**
*
* If the event is of type StartChildWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*/
private StartChildWorkflowExecutionFailedEventAttributes startChildWorkflowExecutionFailedEventAttributes;
/**
*
* Provides the details of the LambdaFunctionScheduled
event. It isn't set for other event types.
*
*/
private LambdaFunctionScheduledEventAttributes lambdaFunctionScheduledEventAttributes;
/**
*
* Provides the details of the LambdaFunctionStarted
event. It isn't set for other event types.
*
*/
private LambdaFunctionStartedEventAttributes lambdaFunctionStartedEventAttributes;
/**
*
* Provides the details of the LambdaFunctionCompleted
event. It isn't set for other event types.
*
*/
private LambdaFunctionCompletedEventAttributes lambdaFunctionCompletedEventAttributes;
/**
*
* Provides the details of the LambdaFunctionFailed
event. It isn't set for other event types.
*
*/
private LambdaFunctionFailedEventAttributes lambdaFunctionFailedEventAttributes;
/**
*
* Provides the details of the LambdaFunctionTimedOut
event. It isn't set for other event types.
*
*/
private LambdaFunctionTimedOutEventAttributes lambdaFunctionTimedOutEventAttributes;
/**
*
* Provides the details of the ScheduleLambdaFunctionFailed
event. It isn't set for other event types.
*
*/
private ScheduleLambdaFunctionFailedEventAttributes scheduleLambdaFunctionFailedEventAttributes;
/**
*
* Provides the details of the StartLambdaFunctionFailed
event. It isn't set for other event types.
*
*/
private StartLambdaFunctionFailedEventAttributes startLambdaFunctionFailedEventAttributes;
/**
*
* The date and time when the event occurred.
*
*
* @param eventTimestamp
* The date and time when the event occurred.
*/
public void setEventTimestamp(java.util.Date eventTimestamp) {
this.eventTimestamp = eventTimestamp;
}
/**
*
* The date and time when the event occurred.
*
*
* @return The date and time when the event occurred.
*/
public java.util.Date getEventTimestamp() {
return this.eventTimestamp;
}
/**
*
* The date and time when the event occurred.
*
*
* @param eventTimestamp
* The date and time when the event occurred.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withEventTimestamp(java.util.Date eventTimestamp) {
setEventTimestamp(eventTimestamp);
return this;
}
/**
*
* The type of the history event.
*
*
* @param eventType
* The type of the history event.
* @see EventType
*/
public void setEventType(String eventType) {
this.eventType = eventType;
}
/**
*
* The type of the history event.
*
*
* @return The type of the history event.
* @see EventType
*/
public String getEventType() {
return this.eventType;
}
/**
*
* The type of the history event.
*
*
* @param eventType
* The type of the history event.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventType
*/
public HistoryEvent withEventType(String eventType) {
setEventType(eventType);
return this;
}
/**
*
* The type of the history event.
*
*
* @param eventType
* The type of the history event.
* @see EventType
*/
public void setEventType(EventType eventType) {
withEventType(eventType);
}
/**
*
* The type of the history event.
*
*
* @param eventType
* The type of the history event.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EventType
*/
public HistoryEvent withEventType(EventType eventType) {
this.eventType = eventType.toString();
return this;
}
/**
*
* The system generated ID of the event. This ID uniquely identifies the event with in the workflow execution
* history.
*
*
* @param eventId
* The system generated ID of the event. This ID uniquely identifies the event with in the workflow execution
* history.
*/
public void setEventId(Long eventId) {
this.eventId = eventId;
}
/**
*
* The system generated ID of the event. This ID uniquely identifies the event with in the workflow execution
* history.
*
*
* @return The system generated ID of the event. This ID uniquely identifies the event with in the workflow
* execution history.
*/
public Long getEventId() {
return this.eventId;
}
/**
*
* The system generated ID of the event. This ID uniquely identifies the event with in the workflow execution
* history.
*
*
* @param eventId
* The system generated ID of the event. This ID uniquely identifies the event with in the workflow execution
* history.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withEventId(Long eventId) {
setEventId(eventId);
return this;
}
/**
*
* If the event is of type WorkflowExecutionStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionStartedEventAttributes
* If the event is of type WorkflowExecutionStarted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setWorkflowExecutionStartedEventAttributes(WorkflowExecutionStartedEventAttributes workflowExecutionStartedEventAttributes) {
this.workflowExecutionStartedEventAttributes = workflowExecutionStartedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type WorkflowExecutionStarted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public WorkflowExecutionStartedEventAttributes getWorkflowExecutionStartedEventAttributes() {
return this.workflowExecutionStartedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionStartedEventAttributes
* If the event is of type WorkflowExecutionStarted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withWorkflowExecutionStartedEventAttributes(WorkflowExecutionStartedEventAttributes workflowExecutionStartedEventAttributes) {
setWorkflowExecutionStartedEventAttributes(workflowExecutionStartedEventAttributes);
return this;
}
/**
*
* If the event is of type WorkflowExecutionCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionCompletedEventAttributes
* If the event is of type WorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setWorkflowExecutionCompletedEventAttributes(WorkflowExecutionCompletedEventAttributes workflowExecutionCompletedEventAttributes) {
this.workflowExecutionCompletedEventAttributes = workflowExecutionCompletedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type WorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public WorkflowExecutionCompletedEventAttributes getWorkflowExecutionCompletedEventAttributes() {
return this.workflowExecutionCompletedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionCompletedEventAttributes
* If the event is of type WorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withWorkflowExecutionCompletedEventAttributes(WorkflowExecutionCompletedEventAttributes workflowExecutionCompletedEventAttributes) {
setWorkflowExecutionCompletedEventAttributes(workflowExecutionCompletedEventAttributes);
return this;
}
/**
*
* If the event is of type CompleteWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param completeWorkflowExecutionFailedEventAttributes
* If the event is of type CompleteWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setCompleteWorkflowExecutionFailedEventAttributes(CompleteWorkflowExecutionFailedEventAttributes completeWorkflowExecutionFailedEventAttributes) {
this.completeWorkflowExecutionFailedEventAttributes = completeWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type CompleteWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type CompleteWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public CompleteWorkflowExecutionFailedEventAttributes getCompleteWorkflowExecutionFailedEventAttributes() {
return this.completeWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type CompleteWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param completeWorkflowExecutionFailedEventAttributes
* If the event is of type CompleteWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withCompleteWorkflowExecutionFailedEventAttributes(
CompleteWorkflowExecutionFailedEventAttributes completeWorkflowExecutionFailedEventAttributes) {
setCompleteWorkflowExecutionFailedEventAttributes(completeWorkflowExecutionFailedEventAttributes);
return this;
}
/**
*
* If the event is of type WorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionFailedEventAttributes
* If the event is of type WorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setWorkflowExecutionFailedEventAttributes(WorkflowExecutionFailedEventAttributes workflowExecutionFailedEventAttributes) {
this.workflowExecutionFailedEventAttributes = workflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type WorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public WorkflowExecutionFailedEventAttributes getWorkflowExecutionFailedEventAttributes() {
return this.workflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionFailedEventAttributes
* If the event is of type WorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withWorkflowExecutionFailedEventAttributes(WorkflowExecutionFailedEventAttributes workflowExecutionFailedEventAttributes) {
setWorkflowExecutionFailedEventAttributes(workflowExecutionFailedEventAttributes);
return this;
}
/**
*
* If the event is of type FailWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param failWorkflowExecutionFailedEventAttributes
* If the event is of type FailWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setFailWorkflowExecutionFailedEventAttributes(FailWorkflowExecutionFailedEventAttributes failWorkflowExecutionFailedEventAttributes) {
this.failWorkflowExecutionFailedEventAttributes = failWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type FailWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type FailWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public FailWorkflowExecutionFailedEventAttributes getFailWorkflowExecutionFailedEventAttributes() {
return this.failWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type FailWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param failWorkflowExecutionFailedEventAttributes
* If the event is of type FailWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withFailWorkflowExecutionFailedEventAttributes(FailWorkflowExecutionFailedEventAttributes failWorkflowExecutionFailedEventAttributes) {
setFailWorkflowExecutionFailedEventAttributes(failWorkflowExecutionFailedEventAttributes);
return this;
}
/**
*
* If the event is of type WorkflowExecutionTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionTimedOutEventAttributes
* If the event is of type WorkflowExecutionTimedOut
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setWorkflowExecutionTimedOutEventAttributes(WorkflowExecutionTimedOutEventAttributes workflowExecutionTimedOutEventAttributes) {
this.workflowExecutionTimedOutEventAttributes = workflowExecutionTimedOutEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type WorkflowExecutionTimedOut
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public WorkflowExecutionTimedOutEventAttributes getWorkflowExecutionTimedOutEventAttributes() {
return this.workflowExecutionTimedOutEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionTimedOutEventAttributes
* If the event is of type WorkflowExecutionTimedOut
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withWorkflowExecutionTimedOutEventAttributes(WorkflowExecutionTimedOutEventAttributes workflowExecutionTimedOutEventAttributes) {
setWorkflowExecutionTimedOutEventAttributes(workflowExecutionTimedOutEventAttributes);
return this;
}
/**
*
* If the event is of type WorkflowExecutionCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionCanceledEventAttributes
* If the event is of type WorkflowExecutionCanceled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setWorkflowExecutionCanceledEventAttributes(WorkflowExecutionCanceledEventAttributes workflowExecutionCanceledEventAttributes) {
this.workflowExecutionCanceledEventAttributes = workflowExecutionCanceledEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type WorkflowExecutionCanceled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public WorkflowExecutionCanceledEventAttributes getWorkflowExecutionCanceledEventAttributes() {
return this.workflowExecutionCanceledEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionCanceledEventAttributes
* If the event is of type WorkflowExecutionCanceled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withWorkflowExecutionCanceledEventAttributes(WorkflowExecutionCanceledEventAttributes workflowExecutionCanceledEventAttributes) {
setWorkflowExecutionCanceledEventAttributes(workflowExecutionCanceledEventAttributes);
return this;
}
/**
*
* If the event is of type CancelWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param cancelWorkflowExecutionFailedEventAttributes
* If the event is of type CancelWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setCancelWorkflowExecutionFailedEventAttributes(CancelWorkflowExecutionFailedEventAttributes cancelWorkflowExecutionFailedEventAttributes) {
this.cancelWorkflowExecutionFailedEventAttributes = cancelWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type CancelWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type CancelWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public CancelWorkflowExecutionFailedEventAttributes getCancelWorkflowExecutionFailedEventAttributes() {
return this.cancelWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type CancelWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param cancelWorkflowExecutionFailedEventAttributes
* If the event is of type CancelWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withCancelWorkflowExecutionFailedEventAttributes(
CancelWorkflowExecutionFailedEventAttributes cancelWorkflowExecutionFailedEventAttributes) {
setCancelWorkflowExecutionFailedEventAttributes(cancelWorkflowExecutionFailedEventAttributes);
return this;
}
/**
*
* If the event is of type WorkflowExecutionContinuedAsNew
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionContinuedAsNewEventAttributes
* If the event is of type WorkflowExecutionContinuedAsNew
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setWorkflowExecutionContinuedAsNewEventAttributes(WorkflowExecutionContinuedAsNewEventAttributes workflowExecutionContinuedAsNewEventAttributes) {
this.workflowExecutionContinuedAsNewEventAttributes = workflowExecutionContinuedAsNewEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionContinuedAsNew
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type WorkflowExecutionContinuedAsNew
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public WorkflowExecutionContinuedAsNewEventAttributes getWorkflowExecutionContinuedAsNewEventAttributes() {
return this.workflowExecutionContinuedAsNewEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionContinuedAsNew
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionContinuedAsNewEventAttributes
* If the event is of type WorkflowExecutionContinuedAsNew
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withWorkflowExecutionContinuedAsNewEventAttributes(
WorkflowExecutionContinuedAsNewEventAttributes workflowExecutionContinuedAsNewEventAttributes) {
setWorkflowExecutionContinuedAsNewEventAttributes(workflowExecutionContinuedAsNewEventAttributes);
return this;
}
/**
*
* If the event is of type ContinueAsNewWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param continueAsNewWorkflowExecutionFailedEventAttributes
* If the event is of type ContinueAsNewWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public void setContinueAsNewWorkflowExecutionFailedEventAttributes(
ContinueAsNewWorkflowExecutionFailedEventAttributes continueAsNewWorkflowExecutionFailedEventAttributes) {
this.continueAsNewWorkflowExecutionFailedEventAttributes = continueAsNewWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type ContinueAsNewWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ContinueAsNewWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public ContinueAsNewWorkflowExecutionFailedEventAttributes getContinueAsNewWorkflowExecutionFailedEventAttributes() {
return this.continueAsNewWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type ContinueAsNewWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param continueAsNewWorkflowExecutionFailedEventAttributes
* If the event is of type ContinueAsNewWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withContinueAsNewWorkflowExecutionFailedEventAttributes(
ContinueAsNewWorkflowExecutionFailedEventAttributes continueAsNewWorkflowExecutionFailedEventAttributes) {
setContinueAsNewWorkflowExecutionFailedEventAttributes(continueAsNewWorkflowExecutionFailedEventAttributes);
return this;
}
/**
*
* If the event is of type WorkflowExecutionTerminated
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionTerminatedEventAttributes
* If the event is of type WorkflowExecutionTerminated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setWorkflowExecutionTerminatedEventAttributes(WorkflowExecutionTerminatedEventAttributes workflowExecutionTerminatedEventAttributes) {
this.workflowExecutionTerminatedEventAttributes = workflowExecutionTerminatedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionTerminated
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type WorkflowExecutionTerminated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public WorkflowExecutionTerminatedEventAttributes getWorkflowExecutionTerminatedEventAttributes() {
return this.workflowExecutionTerminatedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionTerminated
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionTerminatedEventAttributes
* If the event is of type WorkflowExecutionTerminated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withWorkflowExecutionTerminatedEventAttributes(WorkflowExecutionTerminatedEventAttributes workflowExecutionTerminatedEventAttributes) {
setWorkflowExecutionTerminatedEventAttributes(workflowExecutionTerminatedEventAttributes);
return this;
}
/**
*
* If the event is of type WorkflowExecutionCancelRequested
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionCancelRequestedEventAttributes
* If the event is of type WorkflowExecutionCancelRequested
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setWorkflowExecutionCancelRequestedEventAttributes(
WorkflowExecutionCancelRequestedEventAttributes workflowExecutionCancelRequestedEventAttributes) {
this.workflowExecutionCancelRequestedEventAttributes = workflowExecutionCancelRequestedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionCancelRequested
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type WorkflowExecutionCancelRequested
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public WorkflowExecutionCancelRequestedEventAttributes getWorkflowExecutionCancelRequestedEventAttributes() {
return this.workflowExecutionCancelRequestedEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionCancelRequested
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionCancelRequestedEventAttributes
* If the event is of type WorkflowExecutionCancelRequested
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withWorkflowExecutionCancelRequestedEventAttributes(
WorkflowExecutionCancelRequestedEventAttributes workflowExecutionCancelRequestedEventAttributes) {
setWorkflowExecutionCancelRequestedEventAttributes(workflowExecutionCancelRequestedEventAttributes);
return this;
}
/**
*
* If the event is of type DecisionTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param decisionTaskScheduledEventAttributes
* If the event is of type DecisionTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setDecisionTaskScheduledEventAttributes(DecisionTaskScheduledEventAttributes decisionTaskScheduledEventAttributes) {
this.decisionTaskScheduledEventAttributes = decisionTaskScheduledEventAttributes;
}
/**
*
* If the event is of type DecisionTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type DecisionTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public DecisionTaskScheduledEventAttributes getDecisionTaskScheduledEventAttributes() {
return this.decisionTaskScheduledEventAttributes;
}
/**
*
* If the event is of type DecisionTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param decisionTaskScheduledEventAttributes
* If the event is of type DecisionTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withDecisionTaskScheduledEventAttributes(DecisionTaskScheduledEventAttributes decisionTaskScheduledEventAttributes) {
setDecisionTaskScheduledEventAttributes(decisionTaskScheduledEventAttributes);
return this;
}
/**
*
* If the event is of type DecisionTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param decisionTaskStartedEventAttributes
* If the event is of type DecisionTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setDecisionTaskStartedEventAttributes(DecisionTaskStartedEventAttributes decisionTaskStartedEventAttributes) {
this.decisionTaskStartedEventAttributes = decisionTaskStartedEventAttributes;
}
/**
*
* If the event is of type DecisionTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type DecisionTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public DecisionTaskStartedEventAttributes getDecisionTaskStartedEventAttributes() {
return this.decisionTaskStartedEventAttributes;
}
/**
*
* If the event is of type DecisionTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param decisionTaskStartedEventAttributes
* If the event is of type DecisionTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withDecisionTaskStartedEventAttributes(DecisionTaskStartedEventAttributes decisionTaskStartedEventAttributes) {
setDecisionTaskStartedEventAttributes(decisionTaskStartedEventAttributes);
return this;
}
/**
*
* If the event is of type DecisionTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param decisionTaskCompletedEventAttributes
* If the event is of type DecisionTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setDecisionTaskCompletedEventAttributes(DecisionTaskCompletedEventAttributes decisionTaskCompletedEventAttributes) {
this.decisionTaskCompletedEventAttributes = decisionTaskCompletedEventAttributes;
}
/**
*
* If the event is of type DecisionTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type DecisionTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public DecisionTaskCompletedEventAttributes getDecisionTaskCompletedEventAttributes() {
return this.decisionTaskCompletedEventAttributes;
}
/**
*
* If the event is of type DecisionTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param decisionTaskCompletedEventAttributes
* If the event is of type DecisionTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withDecisionTaskCompletedEventAttributes(DecisionTaskCompletedEventAttributes decisionTaskCompletedEventAttributes) {
setDecisionTaskCompletedEventAttributes(decisionTaskCompletedEventAttributes);
return this;
}
/**
*
* If the event is of type DecisionTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param decisionTaskTimedOutEventAttributes
* If the event is of type DecisionTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setDecisionTaskTimedOutEventAttributes(DecisionTaskTimedOutEventAttributes decisionTaskTimedOutEventAttributes) {
this.decisionTaskTimedOutEventAttributes = decisionTaskTimedOutEventAttributes;
}
/**
*
* If the event is of type DecisionTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type DecisionTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public DecisionTaskTimedOutEventAttributes getDecisionTaskTimedOutEventAttributes() {
return this.decisionTaskTimedOutEventAttributes;
}
/**
*
* If the event is of type DecisionTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param decisionTaskTimedOutEventAttributes
* If the event is of type DecisionTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withDecisionTaskTimedOutEventAttributes(DecisionTaskTimedOutEventAttributes decisionTaskTimedOutEventAttributes) {
setDecisionTaskTimedOutEventAttributes(decisionTaskTimedOutEventAttributes);
return this;
}
/**
*
* If the event is of type ActivityTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskScheduledEventAttributes
* If the event is of type ActivityTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setActivityTaskScheduledEventAttributes(ActivityTaskScheduledEventAttributes activityTaskScheduledEventAttributes) {
this.activityTaskScheduledEventAttributes = activityTaskScheduledEventAttributes;
}
/**
*
* If the event is of type ActivityTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ActivityTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public ActivityTaskScheduledEventAttributes getActivityTaskScheduledEventAttributes() {
return this.activityTaskScheduledEventAttributes;
}
/**
*
* If the event is of type ActivityTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskScheduledEventAttributes
* If the event is of type ActivityTaskScheduled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withActivityTaskScheduledEventAttributes(ActivityTaskScheduledEventAttributes activityTaskScheduledEventAttributes) {
setActivityTaskScheduledEventAttributes(activityTaskScheduledEventAttributes);
return this;
}
/**
*
* If the event is of type ActivityTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskStartedEventAttributes
* If the event is of type ActivityTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setActivityTaskStartedEventAttributes(ActivityTaskStartedEventAttributes activityTaskStartedEventAttributes) {
this.activityTaskStartedEventAttributes = activityTaskStartedEventAttributes;
}
/**
*
* If the event is of type ActivityTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ActivityTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public ActivityTaskStartedEventAttributes getActivityTaskStartedEventAttributes() {
return this.activityTaskStartedEventAttributes;
}
/**
*
* If the event is of type ActivityTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskStartedEventAttributes
* If the event is of type ActivityTaskStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withActivityTaskStartedEventAttributes(ActivityTaskStartedEventAttributes activityTaskStartedEventAttributes) {
setActivityTaskStartedEventAttributes(activityTaskStartedEventAttributes);
return this;
}
/**
*
* If the event is of type ActivityTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskCompletedEventAttributes
* If the event is of type ActivityTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setActivityTaskCompletedEventAttributes(ActivityTaskCompletedEventAttributes activityTaskCompletedEventAttributes) {
this.activityTaskCompletedEventAttributes = activityTaskCompletedEventAttributes;
}
/**
*
* If the event is of type ActivityTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ActivityTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public ActivityTaskCompletedEventAttributes getActivityTaskCompletedEventAttributes() {
return this.activityTaskCompletedEventAttributes;
}
/**
*
* If the event is of type ActivityTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskCompletedEventAttributes
* If the event is of type ActivityTaskCompleted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withActivityTaskCompletedEventAttributes(ActivityTaskCompletedEventAttributes activityTaskCompletedEventAttributes) {
setActivityTaskCompletedEventAttributes(activityTaskCompletedEventAttributes);
return this;
}
/**
*
* If the event is of type ActivityTaskFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param activityTaskFailedEventAttributes
* If the event is of type ActivityTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setActivityTaskFailedEventAttributes(ActivityTaskFailedEventAttributes activityTaskFailedEventAttributes) {
this.activityTaskFailedEventAttributes = activityTaskFailedEventAttributes;
}
/**
*
* If the event is of type ActivityTaskFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @return If the event is of type ActivityTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public ActivityTaskFailedEventAttributes getActivityTaskFailedEventAttributes() {
return this.activityTaskFailedEventAttributes;
}
/**
*
* If the event is of type ActivityTaskFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param activityTaskFailedEventAttributes
* If the event is of type ActivityTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withActivityTaskFailedEventAttributes(ActivityTaskFailedEventAttributes activityTaskFailedEventAttributes) {
setActivityTaskFailedEventAttributes(activityTaskFailedEventAttributes);
return this;
}
/**
*
* If the event is of type ActivityTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskTimedOutEventAttributes
* If the event is of type ActivityTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setActivityTaskTimedOutEventAttributes(ActivityTaskTimedOutEventAttributes activityTaskTimedOutEventAttributes) {
this.activityTaskTimedOutEventAttributes = activityTaskTimedOutEventAttributes;
}
/**
*
* If the event is of type ActivityTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ActivityTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public ActivityTaskTimedOutEventAttributes getActivityTaskTimedOutEventAttributes() {
return this.activityTaskTimedOutEventAttributes;
}
/**
*
* If the event is of type ActivityTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskTimedOutEventAttributes
* If the event is of type ActivityTaskTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withActivityTaskTimedOutEventAttributes(ActivityTaskTimedOutEventAttributes activityTaskTimedOutEventAttributes) {
setActivityTaskTimedOutEventAttributes(activityTaskTimedOutEventAttributes);
return this;
}
/**
*
* If the event is of type ActivityTaskCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskCanceledEventAttributes
* If the event is of type ActivityTaskCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setActivityTaskCanceledEventAttributes(ActivityTaskCanceledEventAttributes activityTaskCanceledEventAttributes) {
this.activityTaskCanceledEventAttributes = activityTaskCanceledEventAttributes;
}
/**
*
* If the event is of type ActivityTaskCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ActivityTaskCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public ActivityTaskCanceledEventAttributes getActivityTaskCanceledEventAttributes() {
return this.activityTaskCanceledEventAttributes;
}
/**
*
* If the event is of type ActivityTaskCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskCanceledEventAttributes
* If the event is of type ActivityTaskCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withActivityTaskCanceledEventAttributes(ActivityTaskCanceledEventAttributes activityTaskCanceledEventAttributes) {
setActivityTaskCanceledEventAttributes(activityTaskCanceledEventAttributes);
return this;
}
/**
*
* If the event is of type ActivityTaskcancelRequested
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskCancelRequestedEventAttributes
* If the event is of type ActivityTaskcancelRequested
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setActivityTaskCancelRequestedEventAttributes(ActivityTaskCancelRequestedEventAttributes activityTaskCancelRequestedEventAttributes) {
this.activityTaskCancelRequestedEventAttributes = activityTaskCancelRequestedEventAttributes;
}
/**
*
* If the event is of type ActivityTaskcancelRequested
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ActivityTaskcancelRequested
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public ActivityTaskCancelRequestedEventAttributes getActivityTaskCancelRequestedEventAttributes() {
return this.activityTaskCancelRequestedEventAttributes;
}
/**
*
* If the event is of type ActivityTaskcancelRequested
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param activityTaskCancelRequestedEventAttributes
* If the event is of type ActivityTaskcancelRequested
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withActivityTaskCancelRequestedEventAttributes(ActivityTaskCancelRequestedEventAttributes activityTaskCancelRequestedEventAttributes) {
setActivityTaskCancelRequestedEventAttributes(activityTaskCancelRequestedEventAttributes);
return this;
}
/**
*
* If the event is of type WorkflowExecutionSignaled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionSignaledEventAttributes
* If the event is of type WorkflowExecutionSignaled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setWorkflowExecutionSignaledEventAttributes(WorkflowExecutionSignaledEventAttributes workflowExecutionSignaledEventAttributes) {
this.workflowExecutionSignaledEventAttributes = workflowExecutionSignaledEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionSignaled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type WorkflowExecutionSignaled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public WorkflowExecutionSignaledEventAttributes getWorkflowExecutionSignaledEventAttributes() {
return this.workflowExecutionSignaledEventAttributes;
}
/**
*
* If the event is of type WorkflowExecutionSignaled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param workflowExecutionSignaledEventAttributes
* If the event is of type WorkflowExecutionSignaled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withWorkflowExecutionSignaledEventAttributes(WorkflowExecutionSignaledEventAttributes workflowExecutionSignaledEventAttributes) {
setWorkflowExecutionSignaledEventAttributes(workflowExecutionSignaledEventAttributes);
return this;
}
/**
*
* If the event is of type MarkerRecorded
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param markerRecordedEventAttributes
* If the event is of type MarkerRecorded
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setMarkerRecordedEventAttributes(MarkerRecordedEventAttributes markerRecordedEventAttributes) {
this.markerRecordedEventAttributes = markerRecordedEventAttributes;
}
/**
*
* If the event is of type MarkerRecorded
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @return If the event is of type MarkerRecorded
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public MarkerRecordedEventAttributes getMarkerRecordedEventAttributes() {
return this.markerRecordedEventAttributes;
}
/**
*
* If the event is of type MarkerRecorded
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param markerRecordedEventAttributes
* If the event is of type MarkerRecorded
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withMarkerRecordedEventAttributes(MarkerRecordedEventAttributes markerRecordedEventAttributes) {
setMarkerRecordedEventAttributes(markerRecordedEventAttributes);
return this;
}
/**
*
* If the event is of type DecisionTaskFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param recordMarkerFailedEventAttributes
* If the event is of type DecisionTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setRecordMarkerFailedEventAttributes(RecordMarkerFailedEventAttributes recordMarkerFailedEventAttributes) {
this.recordMarkerFailedEventAttributes = recordMarkerFailedEventAttributes;
}
/**
*
* If the event is of type DecisionTaskFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @return If the event is of type DecisionTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public RecordMarkerFailedEventAttributes getRecordMarkerFailedEventAttributes() {
return this.recordMarkerFailedEventAttributes;
}
/**
*
* If the event is of type DecisionTaskFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param recordMarkerFailedEventAttributes
* If the event is of type DecisionTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withRecordMarkerFailedEventAttributes(RecordMarkerFailedEventAttributes recordMarkerFailedEventAttributes) {
setRecordMarkerFailedEventAttributes(recordMarkerFailedEventAttributes);
return this;
}
/**
*
* If the event is of type TimerStarted
then this member is set and provides detailed information about
* the event. It isn't set for other event types.
*
*
* @param timerStartedEventAttributes
* If the event is of type TimerStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setTimerStartedEventAttributes(TimerStartedEventAttributes timerStartedEventAttributes) {
this.timerStartedEventAttributes = timerStartedEventAttributes;
}
/**
*
* If the event is of type TimerStarted
then this member is set and provides detailed information about
* the event. It isn't set for other event types.
*
*
* @return If the event is of type TimerStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public TimerStartedEventAttributes getTimerStartedEventAttributes() {
return this.timerStartedEventAttributes;
}
/**
*
* If the event is of type TimerStarted
then this member is set and provides detailed information about
* the event. It isn't set for other event types.
*
*
* @param timerStartedEventAttributes
* If the event is of type TimerStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withTimerStartedEventAttributes(TimerStartedEventAttributes timerStartedEventAttributes) {
setTimerStartedEventAttributes(timerStartedEventAttributes);
return this;
}
/**
*
* If the event is of type TimerFired
then this member is set and provides detailed information about
* the event. It isn't set for other event types.
*
*
* @param timerFiredEventAttributes
* If the event is of type TimerFired
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*/
public void setTimerFiredEventAttributes(TimerFiredEventAttributes timerFiredEventAttributes) {
this.timerFiredEventAttributes = timerFiredEventAttributes;
}
/**
*
* If the event is of type TimerFired
then this member is set and provides detailed information about
* the event. It isn't set for other event types.
*
*
* @return If the event is of type TimerFired
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*/
public TimerFiredEventAttributes getTimerFiredEventAttributes() {
return this.timerFiredEventAttributes;
}
/**
*
* If the event is of type TimerFired
then this member is set and provides detailed information about
* the event. It isn't set for other event types.
*
*
* @param timerFiredEventAttributes
* If the event is of type TimerFired
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withTimerFiredEventAttributes(TimerFiredEventAttributes timerFiredEventAttributes) {
setTimerFiredEventAttributes(timerFiredEventAttributes);
return this;
}
/**
*
* If the event is of type TimerCanceled
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param timerCanceledEventAttributes
* If the event is of type TimerCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setTimerCanceledEventAttributes(TimerCanceledEventAttributes timerCanceledEventAttributes) {
this.timerCanceledEventAttributes = timerCanceledEventAttributes;
}
/**
*
* If the event is of type TimerCanceled
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @return If the event is of type TimerCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public TimerCanceledEventAttributes getTimerCanceledEventAttributes() {
return this.timerCanceledEventAttributes;
}
/**
*
* If the event is of type TimerCanceled
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param timerCanceledEventAttributes
* If the event is of type TimerCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withTimerCanceledEventAttributes(TimerCanceledEventAttributes timerCanceledEventAttributes) {
setTimerCanceledEventAttributes(timerCanceledEventAttributes);
return this;
}
/**
*
* If the event is of type StartChildWorkflowExecutionInitiated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param startChildWorkflowExecutionInitiatedEventAttributes
* If the event is of type StartChildWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public void setStartChildWorkflowExecutionInitiatedEventAttributes(
StartChildWorkflowExecutionInitiatedEventAttributes startChildWorkflowExecutionInitiatedEventAttributes) {
this.startChildWorkflowExecutionInitiatedEventAttributes = startChildWorkflowExecutionInitiatedEventAttributes;
}
/**
*
* If the event is of type StartChildWorkflowExecutionInitiated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type StartChildWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public StartChildWorkflowExecutionInitiatedEventAttributes getStartChildWorkflowExecutionInitiatedEventAttributes() {
return this.startChildWorkflowExecutionInitiatedEventAttributes;
}
/**
*
* If the event is of type StartChildWorkflowExecutionInitiated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param startChildWorkflowExecutionInitiatedEventAttributes
* If the event is of type StartChildWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withStartChildWorkflowExecutionInitiatedEventAttributes(
StartChildWorkflowExecutionInitiatedEventAttributes startChildWorkflowExecutionInitiatedEventAttributes) {
setStartChildWorkflowExecutionInitiatedEventAttributes(startChildWorkflowExecutionInitiatedEventAttributes);
return this;
}
/**
*
* If the event is of type ChildWorkflowExecutionStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionStartedEventAttributes
* If the event is of type ChildWorkflowExecutionStarted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setChildWorkflowExecutionStartedEventAttributes(ChildWorkflowExecutionStartedEventAttributes childWorkflowExecutionStartedEventAttributes) {
this.childWorkflowExecutionStartedEventAttributes = childWorkflowExecutionStartedEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ChildWorkflowExecutionStarted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public ChildWorkflowExecutionStartedEventAttributes getChildWorkflowExecutionStartedEventAttributes() {
return this.childWorkflowExecutionStartedEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionStarted
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionStartedEventAttributes
* If the event is of type ChildWorkflowExecutionStarted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withChildWorkflowExecutionStartedEventAttributes(
ChildWorkflowExecutionStartedEventAttributes childWorkflowExecutionStartedEventAttributes) {
setChildWorkflowExecutionStartedEventAttributes(childWorkflowExecutionStartedEventAttributes);
return this;
}
/**
*
* If the event is of type ChildWorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionCompletedEventAttributes
* If the event is of type ChildWorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setChildWorkflowExecutionCompletedEventAttributes(ChildWorkflowExecutionCompletedEventAttributes childWorkflowExecutionCompletedEventAttributes) {
this.childWorkflowExecutionCompletedEventAttributes = childWorkflowExecutionCompletedEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ChildWorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public ChildWorkflowExecutionCompletedEventAttributes getChildWorkflowExecutionCompletedEventAttributes() {
return this.childWorkflowExecutionCompletedEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionCompletedEventAttributes
* If the event is of type ChildWorkflowExecutionCompleted
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withChildWorkflowExecutionCompletedEventAttributes(
ChildWorkflowExecutionCompletedEventAttributes childWorkflowExecutionCompletedEventAttributes) {
setChildWorkflowExecutionCompletedEventAttributes(childWorkflowExecutionCompletedEventAttributes);
return this;
}
/**
*
* If the event is of type ChildWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionFailedEventAttributes
* If the event is of type ChildWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setChildWorkflowExecutionFailedEventAttributes(ChildWorkflowExecutionFailedEventAttributes childWorkflowExecutionFailedEventAttributes) {
this.childWorkflowExecutionFailedEventAttributes = childWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ChildWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public ChildWorkflowExecutionFailedEventAttributes getChildWorkflowExecutionFailedEventAttributes() {
return this.childWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionFailedEventAttributes
* If the event is of type ChildWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withChildWorkflowExecutionFailedEventAttributes(ChildWorkflowExecutionFailedEventAttributes childWorkflowExecutionFailedEventAttributes) {
setChildWorkflowExecutionFailedEventAttributes(childWorkflowExecutionFailedEventAttributes);
return this;
}
/**
*
* If the event is of type ChildWorkflowExecutionTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionTimedOutEventAttributes
* If the event is of type ChildWorkflowExecutionTimedOut
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setChildWorkflowExecutionTimedOutEventAttributes(ChildWorkflowExecutionTimedOutEventAttributes childWorkflowExecutionTimedOutEventAttributes) {
this.childWorkflowExecutionTimedOutEventAttributes = childWorkflowExecutionTimedOutEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ChildWorkflowExecutionTimedOut
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public ChildWorkflowExecutionTimedOutEventAttributes getChildWorkflowExecutionTimedOutEventAttributes() {
return this.childWorkflowExecutionTimedOutEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionTimedOut
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionTimedOutEventAttributes
* If the event is of type ChildWorkflowExecutionTimedOut
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withChildWorkflowExecutionTimedOutEventAttributes(
ChildWorkflowExecutionTimedOutEventAttributes childWorkflowExecutionTimedOutEventAttributes) {
setChildWorkflowExecutionTimedOutEventAttributes(childWorkflowExecutionTimedOutEventAttributes);
return this;
}
/**
*
* If the event is of type ChildWorkflowExecutionCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionCanceledEventAttributes
* If the event is of type ChildWorkflowExecutionCanceled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setChildWorkflowExecutionCanceledEventAttributes(ChildWorkflowExecutionCanceledEventAttributes childWorkflowExecutionCanceledEventAttributes) {
this.childWorkflowExecutionCanceledEventAttributes = childWorkflowExecutionCanceledEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ChildWorkflowExecutionCanceled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public ChildWorkflowExecutionCanceledEventAttributes getChildWorkflowExecutionCanceledEventAttributes() {
return this.childWorkflowExecutionCanceledEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionCanceled
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionCanceledEventAttributes
* If the event is of type ChildWorkflowExecutionCanceled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withChildWorkflowExecutionCanceledEventAttributes(
ChildWorkflowExecutionCanceledEventAttributes childWorkflowExecutionCanceledEventAttributes) {
setChildWorkflowExecutionCanceledEventAttributes(childWorkflowExecutionCanceledEventAttributes);
return this;
}
/**
*
* If the event is of type ChildWorkflowExecutionTerminated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionTerminatedEventAttributes
* If the event is of type ChildWorkflowExecutionTerminated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setChildWorkflowExecutionTerminatedEventAttributes(
ChildWorkflowExecutionTerminatedEventAttributes childWorkflowExecutionTerminatedEventAttributes) {
this.childWorkflowExecutionTerminatedEventAttributes = childWorkflowExecutionTerminatedEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionTerminated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ChildWorkflowExecutionTerminated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public ChildWorkflowExecutionTerminatedEventAttributes getChildWorkflowExecutionTerminatedEventAttributes() {
return this.childWorkflowExecutionTerminatedEventAttributes;
}
/**
*
* If the event is of type ChildWorkflowExecutionTerminated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param childWorkflowExecutionTerminatedEventAttributes
* If the event is of type ChildWorkflowExecutionTerminated
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withChildWorkflowExecutionTerminatedEventAttributes(
ChildWorkflowExecutionTerminatedEventAttributes childWorkflowExecutionTerminatedEventAttributes) {
setChildWorkflowExecutionTerminatedEventAttributes(childWorkflowExecutionTerminatedEventAttributes);
return this;
}
/**
*
* If the event is of type SignalExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @param signalExternalWorkflowExecutionInitiatedEventAttributes
* If the event is of type SignalExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public void setSignalExternalWorkflowExecutionInitiatedEventAttributes(
SignalExternalWorkflowExecutionInitiatedEventAttributes signalExternalWorkflowExecutionInitiatedEventAttributes) {
this.signalExternalWorkflowExecutionInitiatedEventAttributes = signalExternalWorkflowExecutionInitiatedEventAttributes;
}
/**
*
* If the event is of type SignalExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type SignalExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public SignalExternalWorkflowExecutionInitiatedEventAttributes getSignalExternalWorkflowExecutionInitiatedEventAttributes() {
return this.signalExternalWorkflowExecutionInitiatedEventAttributes;
}
/**
*
* If the event is of type SignalExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @param signalExternalWorkflowExecutionInitiatedEventAttributes
* If the event is of type SignalExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withSignalExternalWorkflowExecutionInitiatedEventAttributes(
SignalExternalWorkflowExecutionInitiatedEventAttributes signalExternalWorkflowExecutionInitiatedEventAttributes) {
setSignalExternalWorkflowExecutionInitiatedEventAttributes(signalExternalWorkflowExecutionInitiatedEventAttributes);
return this;
}
/**
*
* If the event is of type ExternalWorkflowExecutionSignaled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param externalWorkflowExecutionSignaledEventAttributes
* If the event is of type ExternalWorkflowExecutionSignaled
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public void setExternalWorkflowExecutionSignaledEventAttributes(
ExternalWorkflowExecutionSignaledEventAttributes externalWorkflowExecutionSignaledEventAttributes) {
this.externalWorkflowExecutionSignaledEventAttributes = externalWorkflowExecutionSignaledEventAttributes;
}
/**
*
* If the event is of type ExternalWorkflowExecutionSignaled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ExternalWorkflowExecutionSignaled
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public ExternalWorkflowExecutionSignaledEventAttributes getExternalWorkflowExecutionSignaledEventAttributes() {
return this.externalWorkflowExecutionSignaledEventAttributes;
}
/**
*
* If the event is of type ExternalWorkflowExecutionSignaled
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param externalWorkflowExecutionSignaledEventAttributes
* If the event is of type ExternalWorkflowExecutionSignaled
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withExternalWorkflowExecutionSignaledEventAttributes(
ExternalWorkflowExecutionSignaledEventAttributes externalWorkflowExecutionSignaledEventAttributes) {
setExternalWorkflowExecutionSignaledEventAttributes(externalWorkflowExecutionSignaledEventAttributes);
return this;
}
/**
*
* If the event is of type SignalExternalWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param signalExternalWorkflowExecutionFailedEventAttributes
* If the event is of type SignalExternalWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public void setSignalExternalWorkflowExecutionFailedEventAttributes(
SignalExternalWorkflowExecutionFailedEventAttributes signalExternalWorkflowExecutionFailedEventAttributes) {
this.signalExternalWorkflowExecutionFailedEventAttributes = signalExternalWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type SignalExternalWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type SignalExternalWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public SignalExternalWorkflowExecutionFailedEventAttributes getSignalExternalWorkflowExecutionFailedEventAttributes() {
return this.signalExternalWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type SignalExternalWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param signalExternalWorkflowExecutionFailedEventAttributes
* If the event is of type SignalExternalWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withSignalExternalWorkflowExecutionFailedEventAttributes(
SignalExternalWorkflowExecutionFailedEventAttributes signalExternalWorkflowExecutionFailedEventAttributes) {
setSignalExternalWorkflowExecutionFailedEventAttributes(signalExternalWorkflowExecutionFailedEventAttributes);
return this;
}
/**
*
* If the event is of type ExternalWorkflowExecutionCancelRequested
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @param externalWorkflowExecutionCancelRequestedEventAttributes
* If the event is of type ExternalWorkflowExecutionCancelRequested
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public void setExternalWorkflowExecutionCancelRequestedEventAttributes(
ExternalWorkflowExecutionCancelRequestedEventAttributes externalWorkflowExecutionCancelRequestedEventAttributes) {
this.externalWorkflowExecutionCancelRequestedEventAttributes = externalWorkflowExecutionCancelRequestedEventAttributes;
}
/**
*
* If the event is of type ExternalWorkflowExecutionCancelRequested
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ExternalWorkflowExecutionCancelRequested
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public ExternalWorkflowExecutionCancelRequestedEventAttributes getExternalWorkflowExecutionCancelRequestedEventAttributes() {
return this.externalWorkflowExecutionCancelRequestedEventAttributes;
}
/**
*
* If the event is of type ExternalWorkflowExecutionCancelRequested
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @param externalWorkflowExecutionCancelRequestedEventAttributes
* If the event is of type ExternalWorkflowExecutionCancelRequested
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withExternalWorkflowExecutionCancelRequestedEventAttributes(
ExternalWorkflowExecutionCancelRequestedEventAttributes externalWorkflowExecutionCancelRequestedEventAttributes) {
setExternalWorkflowExecutionCancelRequestedEventAttributes(externalWorkflowExecutionCancelRequestedEventAttributes);
return this;
}
/**
*
* If the event is of type RequestCancelExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @param requestCancelExternalWorkflowExecutionInitiatedEventAttributes
* If the event is of type RequestCancelExternalWorkflowExecutionInitiated
then this member is
* set and provides detailed information about the event. It isn't set for other event types.
*/
public void setRequestCancelExternalWorkflowExecutionInitiatedEventAttributes(
RequestCancelExternalWorkflowExecutionInitiatedEventAttributes requestCancelExternalWorkflowExecutionInitiatedEventAttributes) {
this.requestCancelExternalWorkflowExecutionInitiatedEventAttributes = requestCancelExternalWorkflowExecutionInitiatedEventAttributes;
}
/**
*
* If the event is of type RequestCancelExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type RequestCancelExternalWorkflowExecutionInitiated
then this member is
* set and provides detailed information about the event. It isn't set for other event types.
*/
public RequestCancelExternalWorkflowExecutionInitiatedEventAttributes getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes() {
return this.requestCancelExternalWorkflowExecutionInitiatedEventAttributes;
}
/**
*
* If the event is of type RequestCancelExternalWorkflowExecutionInitiated
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @param requestCancelExternalWorkflowExecutionInitiatedEventAttributes
* If the event is of type RequestCancelExternalWorkflowExecutionInitiated
then this member is
* set and provides detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withRequestCancelExternalWorkflowExecutionInitiatedEventAttributes(
RequestCancelExternalWorkflowExecutionInitiatedEventAttributes requestCancelExternalWorkflowExecutionInitiatedEventAttributes) {
setRequestCancelExternalWorkflowExecutionInitiatedEventAttributes(requestCancelExternalWorkflowExecutionInitiatedEventAttributes);
return this;
}
/**
*
* If the event is of type RequestCancelExternalWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @param requestCancelExternalWorkflowExecutionFailedEventAttributes
* If the event is of type RequestCancelExternalWorkflowExecutionFailed
then this member is set
* and provides detailed information about the event. It isn't set for other event types.
*/
public void setRequestCancelExternalWorkflowExecutionFailedEventAttributes(
RequestCancelExternalWorkflowExecutionFailedEventAttributes requestCancelExternalWorkflowExecutionFailedEventAttributes) {
this.requestCancelExternalWorkflowExecutionFailedEventAttributes = requestCancelExternalWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type RequestCancelExternalWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type RequestCancelExternalWorkflowExecutionFailed
then this member is set
* and provides detailed information about the event. It isn't set for other event types.
*/
public RequestCancelExternalWorkflowExecutionFailedEventAttributes getRequestCancelExternalWorkflowExecutionFailedEventAttributes() {
return this.requestCancelExternalWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type RequestCancelExternalWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*
*
* @param requestCancelExternalWorkflowExecutionFailedEventAttributes
* If the event is of type RequestCancelExternalWorkflowExecutionFailed
then this member is set
* and provides detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withRequestCancelExternalWorkflowExecutionFailedEventAttributes(
RequestCancelExternalWorkflowExecutionFailedEventAttributes requestCancelExternalWorkflowExecutionFailedEventAttributes) {
setRequestCancelExternalWorkflowExecutionFailedEventAttributes(requestCancelExternalWorkflowExecutionFailedEventAttributes);
return this;
}
/**
*
* If the event is of type ScheduleActivityTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param scheduleActivityTaskFailedEventAttributes
* If the event is of type ScheduleActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setScheduleActivityTaskFailedEventAttributes(ScheduleActivityTaskFailedEventAttributes scheduleActivityTaskFailedEventAttributes) {
this.scheduleActivityTaskFailedEventAttributes = scheduleActivityTaskFailedEventAttributes;
}
/**
*
* If the event is of type ScheduleActivityTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @return If the event is of type ScheduleActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public ScheduleActivityTaskFailedEventAttributes getScheduleActivityTaskFailedEventAttributes() {
return this.scheduleActivityTaskFailedEventAttributes;
}
/**
*
* If the event is of type ScheduleActivityTaskFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*
*
* @param scheduleActivityTaskFailedEventAttributes
* If the event is of type ScheduleActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withScheduleActivityTaskFailedEventAttributes(ScheduleActivityTaskFailedEventAttributes scheduleActivityTaskFailedEventAttributes) {
setScheduleActivityTaskFailedEventAttributes(scheduleActivityTaskFailedEventAttributes);
return this;
}
/**
*
* If the event is of type RequestCancelActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param requestCancelActivityTaskFailedEventAttributes
* If the event is of type RequestCancelActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public void setRequestCancelActivityTaskFailedEventAttributes(RequestCancelActivityTaskFailedEventAttributes requestCancelActivityTaskFailedEventAttributes) {
this.requestCancelActivityTaskFailedEventAttributes = requestCancelActivityTaskFailedEventAttributes;
}
/**
*
* If the event is of type RequestCancelActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type RequestCancelActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*/
public RequestCancelActivityTaskFailedEventAttributes getRequestCancelActivityTaskFailedEventAttributes() {
return this.requestCancelActivityTaskFailedEventAttributes;
}
/**
*
* If the event is of type RequestCancelActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param requestCancelActivityTaskFailedEventAttributes
* If the event is of type RequestCancelActivityTaskFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withRequestCancelActivityTaskFailedEventAttributes(
RequestCancelActivityTaskFailedEventAttributes requestCancelActivityTaskFailedEventAttributes) {
setRequestCancelActivityTaskFailedEventAttributes(requestCancelActivityTaskFailedEventAttributes);
return this;
}
/**
*
* If the event is of type StartTimerFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param startTimerFailedEventAttributes
* If the event is of type StartTimerFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setStartTimerFailedEventAttributes(StartTimerFailedEventAttributes startTimerFailedEventAttributes) {
this.startTimerFailedEventAttributes = startTimerFailedEventAttributes;
}
/**
*
* If the event is of type StartTimerFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @return If the event is of type StartTimerFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public StartTimerFailedEventAttributes getStartTimerFailedEventAttributes() {
return this.startTimerFailedEventAttributes;
}
/**
*
* If the event is of type StartTimerFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param startTimerFailedEventAttributes
* If the event is of type StartTimerFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withStartTimerFailedEventAttributes(StartTimerFailedEventAttributes startTimerFailedEventAttributes) {
setStartTimerFailedEventAttributes(startTimerFailedEventAttributes);
return this;
}
/**
*
* If the event is of type CancelTimerFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param cancelTimerFailedEventAttributes
* If the event is of type CancelTimerFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public void setCancelTimerFailedEventAttributes(CancelTimerFailedEventAttributes cancelTimerFailedEventAttributes) {
this.cancelTimerFailedEventAttributes = cancelTimerFailedEventAttributes;
}
/**
*
* If the event is of type CancelTimerFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @return If the event is of type CancelTimerFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
*/
public CancelTimerFailedEventAttributes getCancelTimerFailedEventAttributes() {
return this.cancelTimerFailedEventAttributes;
}
/**
*
* If the event is of type CancelTimerFailed
then this member is set and provides detailed information
* about the event. It isn't set for other event types.
*
*
* @param cancelTimerFailedEventAttributes
* If the event is of type CancelTimerFailed
then this member is set and provides detailed
* information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withCancelTimerFailedEventAttributes(CancelTimerFailedEventAttributes cancelTimerFailedEventAttributes) {
setCancelTimerFailedEventAttributes(cancelTimerFailedEventAttributes);
return this;
}
/**
*
* If the event is of type StartChildWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param startChildWorkflowExecutionFailedEventAttributes
* If the event is of type StartChildWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public void setStartChildWorkflowExecutionFailedEventAttributes(
StartChildWorkflowExecutionFailedEventAttributes startChildWorkflowExecutionFailedEventAttributes) {
this.startChildWorkflowExecutionFailedEventAttributes = startChildWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type StartChildWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @return If the event is of type StartChildWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
*/
public StartChildWorkflowExecutionFailedEventAttributes getStartChildWorkflowExecutionFailedEventAttributes() {
return this.startChildWorkflowExecutionFailedEventAttributes;
}
/**
*
* If the event is of type StartChildWorkflowExecutionFailed
then this member is set and provides
* detailed information about the event. It isn't set for other event types.
*
*
* @param startChildWorkflowExecutionFailedEventAttributes
* If the event is of type StartChildWorkflowExecutionFailed
then this member is set and
* provides detailed information about the event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withStartChildWorkflowExecutionFailedEventAttributes(
StartChildWorkflowExecutionFailedEventAttributes startChildWorkflowExecutionFailedEventAttributes) {
setStartChildWorkflowExecutionFailedEventAttributes(startChildWorkflowExecutionFailedEventAttributes);
return this;
}
/**
*
* Provides the details of the LambdaFunctionScheduled
event. It isn't set for other event types.
*
*
* @param lambdaFunctionScheduledEventAttributes
* Provides the details of the LambdaFunctionScheduled
event. It isn't set for other event
* types.
*/
public void setLambdaFunctionScheduledEventAttributes(LambdaFunctionScheduledEventAttributes lambdaFunctionScheduledEventAttributes) {
this.lambdaFunctionScheduledEventAttributes = lambdaFunctionScheduledEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionScheduled
event. It isn't set for other event types.
*
*
* @return Provides the details of the LambdaFunctionScheduled
event. It isn't set for other event
* types.
*/
public LambdaFunctionScheduledEventAttributes getLambdaFunctionScheduledEventAttributes() {
return this.lambdaFunctionScheduledEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionScheduled
event. It isn't set for other event types.
*
*
* @param lambdaFunctionScheduledEventAttributes
* Provides the details of the LambdaFunctionScheduled
event. It isn't set for other event
* types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withLambdaFunctionScheduledEventAttributes(LambdaFunctionScheduledEventAttributes lambdaFunctionScheduledEventAttributes) {
setLambdaFunctionScheduledEventAttributes(lambdaFunctionScheduledEventAttributes);
return this;
}
/**
*
* Provides the details of the LambdaFunctionStarted
event. It isn't set for other event types.
*
*
* @param lambdaFunctionStartedEventAttributes
* Provides the details of the LambdaFunctionStarted
event. It isn't set for other event types.
*/
public void setLambdaFunctionStartedEventAttributes(LambdaFunctionStartedEventAttributes lambdaFunctionStartedEventAttributes) {
this.lambdaFunctionStartedEventAttributes = lambdaFunctionStartedEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionStarted
event. It isn't set for other event types.
*
*
* @return Provides the details of the LambdaFunctionStarted
event. It isn't set for other event types.
*/
public LambdaFunctionStartedEventAttributes getLambdaFunctionStartedEventAttributes() {
return this.lambdaFunctionStartedEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionStarted
event. It isn't set for other event types.
*
*
* @param lambdaFunctionStartedEventAttributes
* Provides the details of the LambdaFunctionStarted
event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withLambdaFunctionStartedEventAttributes(LambdaFunctionStartedEventAttributes lambdaFunctionStartedEventAttributes) {
setLambdaFunctionStartedEventAttributes(lambdaFunctionStartedEventAttributes);
return this;
}
/**
*
* Provides the details of the LambdaFunctionCompleted
event. It isn't set for other event types.
*
*
* @param lambdaFunctionCompletedEventAttributes
* Provides the details of the LambdaFunctionCompleted
event. It isn't set for other event
* types.
*/
public void setLambdaFunctionCompletedEventAttributes(LambdaFunctionCompletedEventAttributes lambdaFunctionCompletedEventAttributes) {
this.lambdaFunctionCompletedEventAttributes = lambdaFunctionCompletedEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionCompleted
event. It isn't set for other event types.
*
*
* @return Provides the details of the LambdaFunctionCompleted
event. It isn't set for other event
* types.
*/
public LambdaFunctionCompletedEventAttributes getLambdaFunctionCompletedEventAttributes() {
return this.lambdaFunctionCompletedEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionCompleted
event. It isn't set for other event types.
*
*
* @param lambdaFunctionCompletedEventAttributes
* Provides the details of the LambdaFunctionCompleted
event. It isn't set for other event
* types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withLambdaFunctionCompletedEventAttributes(LambdaFunctionCompletedEventAttributes lambdaFunctionCompletedEventAttributes) {
setLambdaFunctionCompletedEventAttributes(lambdaFunctionCompletedEventAttributes);
return this;
}
/**
*
* Provides the details of the LambdaFunctionFailed
event. It isn't set for other event types.
*
*
* @param lambdaFunctionFailedEventAttributes
* Provides the details of the LambdaFunctionFailed
event. It isn't set for other event types.
*/
public void setLambdaFunctionFailedEventAttributes(LambdaFunctionFailedEventAttributes lambdaFunctionFailedEventAttributes) {
this.lambdaFunctionFailedEventAttributes = lambdaFunctionFailedEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionFailed
event. It isn't set for other event types.
*
*
* @return Provides the details of the LambdaFunctionFailed
event. It isn't set for other event types.
*/
public LambdaFunctionFailedEventAttributes getLambdaFunctionFailedEventAttributes() {
return this.lambdaFunctionFailedEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionFailed
event. It isn't set for other event types.
*
*
* @param lambdaFunctionFailedEventAttributes
* Provides the details of the LambdaFunctionFailed
event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withLambdaFunctionFailedEventAttributes(LambdaFunctionFailedEventAttributes lambdaFunctionFailedEventAttributes) {
setLambdaFunctionFailedEventAttributes(lambdaFunctionFailedEventAttributes);
return this;
}
/**
*
* Provides the details of the LambdaFunctionTimedOut
event. It isn't set for other event types.
*
*
* @param lambdaFunctionTimedOutEventAttributes
* Provides the details of the LambdaFunctionTimedOut
event. It isn't set for other event types.
*/
public void setLambdaFunctionTimedOutEventAttributes(LambdaFunctionTimedOutEventAttributes lambdaFunctionTimedOutEventAttributes) {
this.lambdaFunctionTimedOutEventAttributes = lambdaFunctionTimedOutEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionTimedOut
event. It isn't set for other event types.
*
*
* @return Provides the details of the LambdaFunctionTimedOut
event. It isn't set for other event
* types.
*/
public LambdaFunctionTimedOutEventAttributes getLambdaFunctionTimedOutEventAttributes() {
return this.lambdaFunctionTimedOutEventAttributes;
}
/**
*
* Provides the details of the LambdaFunctionTimedOut
event. It isn't set for other event types.
*
*
* @param lambdaFunctionTimedOutEventAttributes
* Provides the details of the LambdaFunctionTimedOut
event. It isn't set for other event types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withLambdaFunctionTimedOutEventAttributes(LambdaFunctionTimedOutEventAttributes lambdaFunctionTimedOutEventAttributes) {
setLambdaFunctionTimedOutEventAttributes(lambdaFunctionTimedOutEventAttributes);
return this;
}
/**
*
* Provides the details of the ScheduleLambdaFunctionFailed
event. It isn't set for other event types.
*
*
* @param scheduleLambdaFunctionFailedEventAttributes
* Provides the details of the ScheduleLambdaFunctionFailed
event. It isn't set for other event
* types.
*/
public void setScheduleLambdaFunctionFailedEventAttributes(ScheduleLambdaFunctionFailedEventAttributes scheduleLambdaFunctionFailedEventAttributes) {
this.scheduleLambdaFunctionFailedEventAttributes = scheduleLambdaFunctionFailedEventAttributes;
}
/**
*
* Provides the details of the ScheduleLambdaFunctionFailed
event. It isn't set for other event types.
*
*
* @return Provides the details of the ScheduleLambdaFunctionFailed
event. It isn't set for other event
* types.
*/
public ScheduleLambdaFunctionFailedEventAttributes getScheduleLambdaFunctionFailedEventAttributes() {
return this.scheduleLambdaFunctionFailedEventAttributes;
}
/**
*
* Provides the details of the ScheduleLambdaFunctionFailed
event. It isn't set for other event types.
*
*
* @param scheduleLambdaFunctionFailedEventAttributes
* Provides the details of the ScheduleLambdaFunctionFailed
event. It isn't set for other event
* types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withScheduleLambdaFunctionFailedEventAttributes(ScheduleLambdaFunctionFailedEventAttributes scheduleLambdaFunctionFailedEventAttributes) {
setScheduleLambdaFunctionFailedEventAttributes(scheduleLambdaFunctionFailedEventAttributes);
return this;
}
/**
*
* Provides the details of the StartLambdaFunctionFailed
event. It isn't set for other event types.
*
*
* @param startLambdaFunctionFailedEventAttributes
* Provides the details of the StartLambdaFunctionFailed
event. It isn't set for other event
* types.
*/
public void setStartLambdaFunctionFailedEventAttributes(StartLambdaFunctionFailedEventAttributes startLambdaFunctionFailedEventAttributes) {
this.startLambdaFunctionFailedEventAttributes = startLambdaFunctionFailedEventAttributes;
}
/**
*
* Provides the details of the StartLambdaFunctionFailed
event. It isn't set for other event types.
*
*
* @return Provides the details of the StartLambdaFunctionFailed
event. It isn't set for other event
* types.
*/
public StartLambdaFunctionFailedEventAttributes getStartLambdaFunctionFailedEventAttributes() {
return this.startLambdaFunctionFailedEventAttributes;
}
/**
*
* Provides the details of the StartLambdaFunctionFailed
event. It isn't set for other event types.
*
*
* @param startLambdaFunctionFailedEventAttributes
* Provides the details of the StartLambdaFunctionFailed
event. It isn't set for other event
* types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HistoryEvent withStartLambdaFunctionFailedEventAttributes(StartLambdaFunctionFailedEventAttributes startLambdaFunctionFailedEventAttributes) {
setStartLambdaFunctionFailedEventAttributes(startLambdaFunctionFailedEventAttributes);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEventTimestamp() != null)
sb.append("EventTimestamp: ").append(getEventTimestamp()).append(",");
if (getEventType() != null)
sb.append("EventType: ").append(getEventType()).append(",");
if (getEventId() != null)
sb.append("EventId: ").append(getEventId()).append(",");
if (getWorkflowExecutionStartedEventAttributes() != null)
sb.append("WorkflowExecutionStartedEventAttributes: ").append(getWorkflowExecutionStartedEventAttributes()).append(",");
if (getWorkflowExecutionCompletedEventAttributes() != null)
sb.append("WorkflowExecutionCompletedEventAttributes: ").append(getWorkflowExecutionCompletedEventAttributes()).append(",");
if (getCompleteWorkflowExecutionFailedEventAttributes() != null)
sb.append("CompleteWorkflowExecutionFailedEventAttributes: ").append(getCompleteWorkflowExecutionFailedEventAttributes()).append(",");
if (getWorkflowExecutionFailedEventAttributes() != null)
sb.append("WorkflowExecutionFailedEventAttributes: ").append(getWorkflowExecutionFailedEventAttributes()).append(",");
if (getFailWorkflowExecutionFailedEventAttributes() != null)
sb.append("FailWorkflowExecutionFailedEventAttributes: ").append(getFailWorkflowExecutionFailedEventAttributes()).append(",");
if (getWorkflowExecutionTimedOutEventAttributes() != null)
sb.append("WorkflowExecutionTimedOutEventAttributes: ").append(getWorkflowExecutionTimedOutEventAttributes()).append(",");
if (getWorkflowExecutionCanceledEventAttributes() != null)
sb.append("WorkflowExecutionCanceledEventAttributes: ").append(getWorkflowExecutionCanceledEventAttributes()).append(",");
if (getCancelWorkflowExecutionFailedEventAttributes() != null)
sb.append("CancelWorkflowExecutionFailedEventAttributes: ").append(getCancelWorkflowExecutionFailedEventAttributes()).append(",");
if (getWorkflowExecutionContinuedAsNewEventAttributes() != null)
sb.append("WorkflowExecutionContinuedAsNewEventAttributes: ").append(getWorkflowExecutionContinuedAsNewEventAttributes()).append(",");
if (getContinueAsNewWorkflowExecutionFailedEventAttributes() != null)
sb.append("ContinueAsNewWorkflowExecutionFailedEventAttributes: ").append(getContinueAsNewWorkflowExecutionFailedEventAttributes()).append(",");
if (getWorkflowExecutionTerminatedEventAttributes() != null)
sb.append("WorkflowExecutionTerminatedEventAttributes: ").append(getWorkflowExecutionTerminatedEventAttributes()).append(",");
if (getWorkflowExecutionCancelRequestedEventAttributes() != null)
sb.append("WorkflowExecutionCancelRequestedEventAttributes: ").append(getWorkflowExecutionCancelRequestedEventAttributes()).append(",");
if (getDecisionTaskScheduledEventAttributes() != null)
sb.append("DecisionTaskScheduledEventAttributes: ").append(getDecisionTaskScheduledEventAttributes()).append(",");
if (getDecisionTaskStartedEventAttributes() != null)
sb.append("DecisionTaskStartedEventAttributes: ").append(getDecisionTaskStartedEventAttributes()).append(",");
if (getDecisionTaskCompletedEventAttributes() != null)
sb.append("DecisionTaskCompletedEventAttributes: ").append(getDecisionTaskCompletedEventAttributes()).append(",");
if (getDecisionTaskTimedOutEventAttributes() != null)
sb.append("DecisionTaskTimedOutEventAttributes: ").append(getDecisionTaskTimedOutEventAttributes()).append(",");
if (getActivityTaskScheduledEventAttributes() != null)
sb.append("ActivityTaskScheduledEventAttributes: ").append(getActivityTaskScheduledEventAttributes()).append(",");
if (getActivityTaskStartedEventAttributes() != null)
sb.append("ActivityTaskStartedEventAttributes: ").append(getActivityTaskStartedEventAttributes()).append(",");
if (getActivityTaskCompletedEventAttributes() != null)
sb.append("ActivityTaskCompletedEventAttributes: ").append(getActivityTaskCompletedEventAttributes()).append(",");
if (getActivityTaskFailedEventAttributes() != null)
sb.append("ActivityTaskFailedEventAttributes: ").append(getActivityTaskFailedEventAttributes()).append(",");
if (getActivityTaskTimedOutEventAttributes() != null)
sb.append("ActivityTaskTimedOutEventAttributes: ").append(getActivityTaskTimedOutEventAttributes()).append(",");
if (getActivityTaskCanceledEventAttributes() != null)
sb.append("ActivityTaskCanceledEventAttributes: ").append(getActivityTaskCanceledEventAttributes()).append(",");
if (getActivityTaskCancelRequestedEventAttributes() != null)
sb.append("ActivityTaskCancelRequestedEventAttributes: ").append(getActivityTaskCancelRequestedEventAttributes()).append(",");
if (getWorkflowExecutionSignaledEventAttributes() != null)
sb.append("WorkflowExecutionSignaledEventAttributes: ").append(getWorkflowExecutionSignaledEventAttributes()).append(",");
if (getMarkerRecordedEventAttributes() != null)
sb.append("MarkerRecordedEventAttributes: ").append(getMarkerRecordedEventAttributes()).append(",");
if (getRecordMarkerFailedEventAttributes() != null)
sb.append("RecordMarkerFailedEventAttributes: ").append(getRecordMarkerFailedEventAttributes()).append(",");
if (getTimerStartedEventAttributes() != null)
sb.append("TimerStartedEventAttributes: ").append(getTimerStartedEventAttributes()).append(",");
if (getTimerFiredEventAttributes() != null)
sb.append("TimerFiredEventAttributes: ").append(getTimerFiredEventAttributes()).append(",");
if (getTimerCanceledEventAttributes() != null)
sb.append("TimerCanceledEventAttributes: ").append(getTimerCanceledEventAttributes()).append(",");
if (getStartChildWorkflowExecutionInitiatedEventAttributes() != null)
sb.append("StartChildWorkflowExecutionInitiatedEventAttributes: ").append(getStartChildWorkflowExecutionInitiatedEventAttributes()).append(",");
if (getChildWorkflowExecutionStartedEventAttributes() != null)
sb.append("ChildWorkflowExecutionStartedEventAttributes: ").append(getChildWorkflowExecutionStartedEventAttributes()).append(",");
if (getChildWorkflowExecutionCompletedEventAttributes() != null)
sb.append("ChildWorkflowExecutionCompletedEventAttributes: ").append(getChildWorkflowExecutionCompletedEventAttributes()).append(",");
if (getChildWorkflowExecutionFailedEventAttributes() != null)
sb.append("ChildWorkflowExecutionFailedEventAttributes: ").append(getChildWorkflowExecutionFailedEventAttributes()).append(",");
if (getChildWorkflowExecutionTimedOutEventAttributes() != null)
sb.append("ChildWorkflowExecutionTimedOutEventAttributes: ").append(getChildWorkflowExecutionTimedOutEventAttributes()).append(",");
if (getChildWorkflowExecutionCanceledEventAttributes() != null)
sb.append("ChildWorkflowExecutionCanceledEventAttributes: ").append(getChildWorkflowExecutionCanceledEventAttributes()).append(",");
if (getChildWorkflowExecutionTerminatedEventAttributes() != null)
sb.append("ChildWorkflowExecutionTerminatedEventAttributes: ").append(getChildWorkflowExecutionTerminatedEventAttributes()).append(",");
if (getSignalExternalWorkflowExecutionInitiatedEventAttributes() != null)
sb.append("SignalExternalWorkflowExecutionInitiatedEventAttributes: ").append(getSignalExternalWorkflowExecutionInitiatedEventAttributes())
.append(",");
if (getExternalWorkflowExecutionSignaledEventAttributes() != null)
sb.append("ExternalWorkflowExecutionSignaledEventAttributes: ").append(getExternalWorkflowExecutionSignaledEventAttributes()).append(",");
if (getSignalExternalWorkflowExecutionFailedEventAttributes() != null)
sb.append("SignalExternalWorkflowExecutionFailedEventAttributes: ").append(getSignalExternalWorkflowExecutionFailedEventAttributes()).append(",");
if (getExternalWorkflowExecutionCancelRequestedEventAttributes() != null)
sb.append("ExternalWorkflowExecutionCancelRequestedEventAttributes: ").append(getExternalWorkflowExecutionCancelRequestedEventAttributes())
.append(",");
if (getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes() != null)
sb.append("RequestCancelExternalWorkflowExecutionInitiatedEventAttributes: ")
.append(getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes()).append(",");
if (getRequestCancelExternalWorkflowExecutionFailedEventAttributes() != null)
sb.append("RequestCancelExternalWorkflowExecutionFailedEventAttributes: ").append(getRequestCancelExternalWorkflowExecutionFailedEventAttributes())
.append(",");
if (getScheduleActivityTaskFailedEventAttributes() != null)
sb.append("ScheduleActivityTaskFailedEventAttributes: ").append(getScheduleActivityTaskFailedEventAttributes()).append(",");
if (getRequestCancelActivityTaskFailedEventAttributes() != null)
sb.append("RequestCancelActivityTaskFailedEventAttributes: ").append(getRequestCancelActivityTaskFailedEventAttributes()).append(",");
if (getStartTimerFailedEventAttributes() != null)
sb.append("StartTimerFailedEventAttributes: ").append(getStartTimerFailedEventAttributes()).append(",");
if (getCancelTimerFailedEventAttributes() != null)
sb.append("CancelTimerFailedEventAttributes: ").append(getCancelTimerFailedEventAttributes()).append(",");
if (getStartChildWorkflowExecutionFailedEventAttributes() != null)
sb.append("StartChildWorkflowExecutionFailedEventAttributes: ").append(getStartChildWorkflowExecutionFailedEventAttributes()).append(",");
if (getLambdaFunctionScheduledEventAttributes() != null)
sb.append("LambdaFunctionScheduledEventAttributes: ").append(getLambdaFunctionScheduledEventAttributes()).append(",");
if (getLambdaFunctionStartedEventAttributes() != null)
sb.append("LambdaFunctionStartedEventAttributes: ").append(getLambdaFunctionStartedEventAttributes()).append(",");
if (getLambdaFunctionCompletedEventAttributes() != null)
sb.append("LambdaFunctionCompletedEventAttributes: ").append(getLambdaFunctionCompletedEventAttributes()).append(",");
if (getLambdaFunctionFailedEventAttributes() != null)
sb.append("LambdaFunctionFailedEventAttributes: ").append(getLambdaFunctionFailedEventAttributes()).append(",");
if (getLambdaFunctionTimedOutEventAttributes() != null)
sb.append("LambdaFunctionTimedOutEventAttributes: ").append(getLambdaFunctionTimedOutEventAttributes()).append(",");
if (getScheduleLambdaFunctionFailedEventAttributes() != null)
sb.append("ScheduleLambdaFunctionFailedEventAttributes: ").append(getScheduleLambdaFunctionFailedEventAttributes()).append(",");
if (getStartLambdaFunctionFailedEventAttributes() != null)
sb.append("StartLambdaFunctionFailedEventAttributes: ").append(getStartLambdaFunctionFailedEventAttributes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof HistoryEvent == false)
return false;
HistoryEvent other = (HistoryEvent) obj;
if (other.getEventTimestamp() == null ^ this.getEventTimestamp() == null)
return false;
if (other.getEventTimestamp() != null && other.getEventTimestamp().equals(this.getEventTimestamp()) == false)
return false;
if (other.getEventType() == null ^ this.getEventType() == null)
return false;
if (other.getEventType() != null && other.getEventType().equals(this.getEventType()) == false)
return false;
if (other.getEventId() == null ^ this.getEventId() == null)
return false;
if (other.getEventId() != null && other.getEventId().equals(this.getEventId()) == false)
return false;
if (other.getWorkflowExecutionStartedEventAttributes() == null ^ this.getWorkflowExecutionStartedEventAttributes() == null)
return false;
if (other.getWorkflowExecutionStartedEventAttributes() != null
&& other.getWorkflowExecutionStartedEventAttributes().equals(this.getWorkflowExecutionStartedEventAttributes()) == false)
return false;
if (other.getWorkflowExecutionCompletedEventAttributes() == null ^ this.getWorkflowExecutionCompletedEventAttributes() == null)
return false;
if (other.getWorkflowExecutionCompletedEventAttributes() != null
&& other.getWorkflowExecutionCompletedEventAttributes().equals(this.getWorkflowExecutionCompletedEventAttributes()) == false)
return false;
if (other.getCompleteWorkflowExecutionFailedEventAttributes() == null ^ this.getCompleteWorkflowExecutionFailedEventAttributes() == null)
return false;
if (other.getCompleteWorkflowExecutionFailedEventAttributes() != null
&& other.getCompleteWorkflowExecutionFailedEventAttributes().equals(this.getCompleteWorkflowExecutionFailedEventAttributes()) == false)
return false;
if (other.getWorkflowExecutionFailedEventAttributes() == null ^ this.getWorkflowExecutionFailedEventAttributes() == null)
return false;
if (other.getWorkflowExecutionFailedEventAttributes() != null
&& other.getWorkflowExecutionFailedEventAttributes().equals(this.getWorkflowExecutionFailedEventAttributes()) == false)
return false;
if (other.getFailWorkflowExecutionFailedEventAttributes() == null ^ this.getFailWorkflowExecutionFailedEventAttributes() == null)
return false;
if (other.getFailWorkflowExecutionFailedEventAttributes() != null
&& other.getFailWorkflowExecutionFailedEventAttributes().equals(this.getFailWorkflowExecutionFailedEventAttributes()) == false)
return false;
if (other.getWorkflowExecutionTimedOutEventAttributes() == null ^ this.getWorkflowExecutionTimedOutEventAttributes() == null)
return false;
if (other.getWorkflowExecutionTimedOutEventAttributes() != null
&& other.getWorkflowExecutionTimedOutEventAttributes().equals(this.getWorkflowExecutionTimedOutEventAttributes()) == false)
return false;
if (other.getWorkflowExecutionCanceledEventAttributes() == null ^ this.getWorkflowExecutionCanceledEventAttributes() == null)
return false;
if (other.getWorkflowExecutionCanceledEventAttributes() != null
&& other.getWorkflowExecutionCanceledEventAttributes().equals(this.getWorkflowExecutionCanceledEventAttributes()) == false)
return false;
if (other.getCancelWorkflowExecutionFailedEventAttributes() == null ^ this.getCancelWorkflowExecutionFailedEventAttributes() == null)
return false;
if (other.getCancelWorkflowExecutionFailedEventAttributes() != null
&& other.getCancelWorkflowExecutionFailedEventAttributes().equals(this.getCancelWorkflowExecutionFailedEventAttributes()) == false)
return false;
if (other.getWorkflowExecutionContinuedAsNewEventAttributes() == null ^ this.getWorkflowExecutionContinuedAsNewEventAttributes() == null)
return false;
if (other.getWorkflowExecutionContinuedAsNewEventAttributes() != null
&& other.getWorkflowExecutionContinuedAsNewEventAttributes().equals(this.getWorkflowExecutionContinuedAsNewEventAttributes()) == false)
return false;
if (other.getContinueAsNewWorkflowExecutionFailedEventAttributes() == null ^ this.getContinueAsNewWorkflowExecutionFailedEventAttributes() == null)
return false;
if (other.getContinueAsNewWorkflowExecutionFailedEventAttributes() != null
&& other.getContinueAsNewWorkflowExecutionFailedEventAttributes().equals(this.getContinueAsNewWorkflowExecutionFailedEventAttributes()) == false)
return false;
if (other.getWorkflowExecutionTerminatedEventAttributes() == null ^ this.getWorkflowExecutionTerminatedEventAttributes() == null)
return false;
if (other.getWorkflowExecutionTerminatedEventAttributes() != null
&& other.getWorkflowExecutionTerminatedEventAttributes().equals(this.getWorkflowExecutionTerminatedEventAttributes()) == false)
return false;
if (other.getWorkflowExecutionCancelRequestedEventAttributes() == null ^ this.getWorkflowExecutionCancelRequestedEventAttributes() == null)
return false;
if (other.getWorkflowExecutionCancelRequestedEventAttributes() != null
&& other.getWorkflowExecutionCancelRequestedEventAttributes().equals(this.getWorkflowExecutionCancelRequestedEventAttributes()) == false)
return false;
if (other.getDecisionTaskScheduledEventAttributes() == null ^ this.getDecisionTaskScheduledEventAttributes() == null)
return false;
if (other.getDecisionTaskScheduledEventAttributes() != null
&& other.getDecisionTaskScheduledEventAttributes().equals(this.getDecisionTaskScheduledEventAttributes()) == false)
return false;
if (other.getDecisionTaskStartedEventAttributes() == null ^ this.getDecisionTaskStartedEventAttributes() == null)
return false;
if (other.getDecisionTaskStartedEventAttributes() != null
&& other.getDecisionTaskStartedEventAttributes().equals(this.getDecisionTaskStartedEventAttributes()) == false)
return false;
if (other.getDecisionTaskCompletedEventAttributes() == null ^ this.getDecisionTaskCompletedEventAttributes() == null)
return false;
if (other.getDecisionTaskCompletedEventAttributes() != null
&& other.getDecisionTaskCompletedEventAttributes().equals(this.getDecisionTaskCompletedEventAttributes()) == false)
return false;
if (other.getDecisionTaskTimedOutEventAttributes() == null ^ this.getDecisionTaskTimedOutEventAttributes() == null)
return false;
if (other.getDecisionTaskTimedOutEventAttributes() != null
&& other.getDecisionTaskTimedOutEventAttributes().equals(this.getDecisionTaskTimedOutEventAttributes()) == false)
return false;
if (other.getActivityTaskScheduledEventAttributes() == null ^ this.getActivityTaskScheduledEventAttributes() == null)
return false;
if (other.getActivityTaskScheduledEventAttributes() != null
&& other.getActivityTaskScheduledEventAttributes().equals(this.getActivityTaskScheduledEventAttributes()) == false)
return false;
if (other.getActivityTaskStartedEventAttributes() == null ^ this.getActivityTaskStartedEventAttributes() == null)
return false;
if (other.getActivityTaskStartedEventAttributes() != null
&& other.getActivityTaskStartedEventAttributes().equals(this.getActivityTaskStartedEventAttributes()) == false)
return false;
if (other.getActivityTaskCompletedEventAttributes() == null ^ this.getActivityTaskCompletedEventAttributes() == null)
return false;
if (other.getActivityTaskCompletedEventAttributes() != null
&& other.getActivityTaskCompletedEventAttributes().equals(this.getActivityTaskCompletedEventAttributes()) == false)
return false;
if (other.getActivityTaskFailedEventAttributes() == null ^ this.getActivityTaskFailedEventAttributes() == null)
return false;
if (other.getActivityTaskFailedEventAttributes() != null
&& other.getActivityTaskFailedEventAttributes().equals(this.getActivityTaskFailedEventAttributes()) == false)
return false;
if (other.getActivityTaskTimedOutEventAttributes() == null ^ this.getActivityTaskTimedOutEventAttributes() == null)
return false;
if (other.getActivityTaskTimedOutEventAttributes() != null
&& other.getActivityTaskTimedOutEventAttributes().equals(this.getActivityTaskTimedOutEventAttributes()) == false)
return false;
if (other.getActivityTaskCanceledEventAttributes() == null ^ this.getActivityTaskCanceledEventAttributes() == null)
return false;
if (other.getActivityTaskCanceledEventAttributes() != null
&& other.getActivityTaskCanceledEventAttributes().equals(this.getActivityTaskCanceledEventAttributes()) == false)
return false;
if (other.getActivityTaskCancelRequestedEventAttributes() == null ^ this.getActivityTaskCancelRequestedEventAttributes() == null)
return false;
if (other.getActivityTaskCancelRequestedEventAttributes() != null
&& other.getActivityTaskCancelRequestedEventAttributes().equals(this.getActivityTaskCancelRequestedEventAttributes()) == false)
return false;
if (other.getWorkflowExecutionSignaledEventAttributes() == null ^ this.getWorkflowExecutionSignaledEventAttributes() == null)
return false;
if (other.getWorkflowExecutionSignaledEventAttributes() != null
&& other.getWorkflowExecutionSignaledEventAttributes().equals(this.getWorkflowExecutionSignaledEventAttributes()) == false)
return false;
if (other.getMarkerRecordedEventAttributes() == null ^ this.getMarkerRecordedEventAttributes() == null)
return false;
if (other.getMarkerRecordedEventAttributes() != null
&& other.getMarkerRecordedEventAttributes().equals(this.getMarkerRecordedEventAttributes()) == false)
return false;
if (other.getRecordMarkerFailedEventAttributes() == null ^ this.getRecordMarkerFailedEventAttributes() == null)
return false;
if (other.getRecordMarkerFailedEventAttributes() != null
&& other.getRecordMarkerFailedEventAttributes().equals(this.getRecordMarkerFailedEventAttributes()) == false)
return false;
if (other.getTimerStartedEventAttributes() == null ^ this.getTimerStartedEventAttributes() == null)
return false;
if (other.getTimerStartedEventAttributes() != null && other.getTimerStartedEventAttributes().equals(this.getTimerStartedEventAttributes()) == false)
return false;
if (other.getTimerFiredEventAttributes() == null ^ this.getTimerFiredEventAttributes() == null)
return false;
if (other.getTimerFiredEventAttributes() != null && other.getTimerFiredEventAttributes().equals(this.getTimerFiredEventAttributes()) == false)
return false;
if (other.getTimerCanceledEventAttributes() == null ^ this.getTimerCanceledEventAttributes() == null)
return false;
if (other.getTimerCanceledEventAttributes() != null && other.getTimerCanceledEventAttributes().equals(this.getTimerCanceledEventAttributes()) == false)
return false;
if (other.getStartChildWorkflowExecutionInitiatedEventAttributes() == null ^ this.getStartChildWorkflowExecutionInitiatedEventAttributes() == null)
return false;
if (other.getStartChildWorkflowExecutionInitiatedEventAttributes() != null
&& other.getStartChildWorkflowExecutionInitiatedEventAttributes().equals(this.getStartChildWorkflowExecutionInitiatedEventAttributes()) == false)
return false;
if (other.getChildWorkflowExecutionStartedEventAttributes() == null ^ this.getChildWorkflowExecutionStartedEventAttributes() == null)
return false;
if (other.getChildWorkflowExecutionStartedEventAttributes() != null
&& other.getChildWorkflowExecutionStartedEventAttributes().equals(this.getChildWorkflowExecutionStartedEventAttributes()) == false)
return false;
if (other.getChildWorkflowExecutionCompletedEventAttributes() == null ^ this.getChildWorkflowExecutionCompletedEventAttributes() == null)
return false;
if (other.getChildWorkflowExecutionCompletedEventAttributes() != null
&& other.getChildWorkflowExecutionCompletedEventAttributes().equals(this.getChildWorkflowExecutionCompletedEventAttributes()) == false)
return false;
if (other.getChildWorkflowExecutionFailedEventAttributes() == null ^ this.getChildWorkflowExecutionFailedEventAttributes() == null)
return false;
if (other.getChildWorkflowExecutionFailedEventAttributes() != null
&& other.getChildWorkflowExecutionFailedEventAttributes().equals(this.getChildWorkflowExecutionFailedEventAttributes()) == false)
return false;
if (other.getChildWorkflowExecutionTimedOutEventAttributes() == null ^ this.getChildWorkflowExecutionTimedOutEventAttributes() == null)
return false;
if (other.getChildWorkflowExecutionTimedOutEventAttributes() != null
&& other.getChildWorkflowExecutionTimedOutEventAttributes().equals(this.getChildWorkflowExecutionTimedOutEventAttributes()) == false)
return false;
if (other.getChildWorkflowExecutionCanceledEventAttributes() == null ^ this.getChildWorkflowExecutionCanceledEventAttributes() == null)
return false;
if (other.getChildWorkflowExecutionCanceledEventAttributes() != null
&& other.getChildWorkflowExecutionCanceledEventAttributes().equals(this.getChildWorkflowExecutionCanceledEventAttributes()) == false)
return false;
if (other.getChildWorkflowExecutionTerminatedEventAttributes() == null ^ this.getChildWorkflowExecutionTerminatedEventAttributes() == null)
return false;
if (other.getChildWorkflowExecutionTerminatedEventAttributes() != null
&& other.getChildWorkflowExecutionTerminatedEventAttributes().equals(this.getChildWorkflowExecutionTerminatedEventAttributes()) == false)
return false;
if (other.getSignalExternalWorkflowExecutionInitiatedEventAttributes() == null
^ this.getSignalExternalWorkflowExecutionInitiatedEventAttributes() == null)
return false;
if (other.getSignalExternalWorkflowExecutionInitiatedEventAttributes() != null
&& other.getSignalExternalWorkflowExecutionInitiatedEventAttributes().equals(this.getSignalExternalWorkflowExecutionInitiatedEventAttributes()) == false)
return false;
if (other.getExternalWorkflowExecutionSignaledEventAttributes() == null ^ this.getExternalWorkflowExecutionSignaledEventAttributes() == null)
return false;
if (other.getExternalWorkflowExecutionSignaledEventAttributes() != null
&& other.getExternalWorkflowExecutionSignaledEventAttributes().equals(this.getExternalWorkflowExecutionSignaledEventAttributes()) == false)
return false;
if (other.getSignalExternalWorkflowExecutionFailedEventAttributes() == null ^ this.getSignalExternalWorkflowExecutionFailedEventAttributes() == null)
return false;
if (other.getSignalExternalWorkflowExecutionFailedEventAttributes() != null
&& other.getSignalExternalWorkflowExecutionFailedEventAttributes().equals(this.getSignalExternalWorkflowExecutionFailedEventAttributes()) == false)
return false;
if (other.getExternalWorkflowExecutionCancelRequestedEventAttributes() == null
^ this.getExternalWorkflowExecutionCancelRequestedEventAttributes() == null)
return false;
if (other.getExternalWorkflowExecutionCancelRequestedEventAttributes() != null
&& other.getExternalWorkflowExecutionCancelRequestedEventAttributes().equals(this.getExternalWorkflowExecutionCancelRequestedEventAttributes()) == false)
return false;
if (other.getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes() == null
^ this.getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes() == null)
return false;
if (other.getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes() != null
&& other.getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes().equals(
this.getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes()) == false)
return false;
if (other.getRequestCancelExternalWorkflowExecutionFailedEventAttributes() == null
^ this.getRequestCancelExternalWorkflowExecutionFailedEventAttributes() == null)
return false;
if (other.getRequestCancelExternalWorkflowExecutionFailedEventAttributes() != null
&& other.getRequestCancelExternalWorkflowExecutionFailedEventAttributes().equals(
this.getRequestCancelExternalWorkflowExecutionFailedEventAttributes()) == false)
return false;
if (other.getScheduleActivityTaskFailedEventAttributes() == null ^ this.getScheduleActivityTaskFailedEventAttributes() == null)
return false;
if (other.getScheduleActivityTaskFailedEventAttributes() != null
&& other.getScheduleActivityTaskFailedEventAttributes().equals(this.getScheduleActivityTaskFailedEventAttributes()) == false)
return false;
if (other.getRequestCancelActivityTaskFailedEventAttributes() == null ^ this.getRequestCancelActivityTaskFailedEventAttributes() == null)
return false;
if (other.getRequestCancelActivityTaskFailedEventAttributes() != null
&& other.getRequestCancelActivityTaskFailedEventAttributes().equals(this.getRequestCancelActivityTaskFailedEventAttributes()) == false)
return false;
if (other.getStartTimerFailedEventAttributes() == null ^ this.getStartTimerFailedEventAttributes() == null)
return false;
if (other.getStartTimerFailedEventAttributes() != null
&& other.getStartTimerFailedEventAttributes().equals(this.getStartTimerFailedEventAttributes()) == false)
return false;
if (other.getCancelTimerFailedEventAttributes() == null ^ this.getCancelTimerFailedEventAttributes() == null)
return false;
if (other.getCancelTimerFailedEventAttributes() != null
&& other.getCancelTimerFailedEventAttributes().equals(this.getCancelTimerFailedEventAttributes()) == false)
return false;
if (other.getStartChildWorkflowExecutionFailedEventAttributes() == null ^ this.getStartChildWorkflowExecutionFailedEventAttributes() == null)
return false;
if (other.getStartChildWorkflowExecutionFailedEventAttributes() != null
&& other.getStartChildWorkflowExecutionFailedEventAttributes().equals(this.getStartChildWorkflowExecutionFailedEventAttributes()) == false)
return false;
if (other.getLambdaFunctionScheduledEventAttributes() == null ^ this.getLambdaFunctionScheduledEventAttributes() == null)
return false;
if (other.getLambdaFunctionScheduledEventAttributes() != null
&& other.getLambdaFunctionScheduledEventAttributes().equals(this.getLambdaFunctionScheduledEventAttributes()) == false)
return false;
if (other.getLambdaFunctionStartedEventAttributes() == null ^ this.getLambdaFunctionStartedEventAttributes() == null)
return false;
if (other.getLambdaFunctionStartedEventAttributes() != null
&& other.getLambdaFunctionStartedEventAttributes().equals(this.getLambdaFunctionStartedEventAttributes()) == false)
return false;
if (other.getLambdaFunctionCompletedEventAttributes() == null ^ this.getLambdaFunctionCompletedEventAttributes() == null)
return false;
if (other.getLambdaFunctionCompletedEventAttributes() != null
&& other.getLambdaFunctionCompletedEventAttributes().equals(this.getLambdaFunctionCompletedEventAttributes()) == false)
return false;
if (other.getLambdaFunctionFailedEventAttributes() == null ^ this.getLambdaFunctionFailedEventAttributes() == null)
return false;
if (other.getLambdaFunctionFailedEventAttributes() != null
&& other.getLambdaFunctionFailedEventAttributes().equals(this.getLambdaFunctionFailedEventAttributes()) == false)
return false;
if (other.getLambdaFunctionTimedOutEventAttributes() == null ^ this.getLambdaFunctionTimedOutEventAttributes() == null)
return false;
if (other.getLambdaFunctionTimedOutEventAttributes() != null
&& other.getLambdaFunctionTimedOutEventAttributes().equals(this.getLambdaFunctionTimedOutEventAttributes()) == false)
return false;
if (other.getScheduleLambdaFunctionFailedEventAttributes() == null ^ this.getScheduleLambdaFunctionFailedEventAttributes() == null)
return false;
if (other.getScheduleLambdaFunctionFailedEventAttributes() != null
&& other.getScheduleLambdaFunctionFailedEventAttributes().equals(this.getScheduleLambdaFunctionFailedEventAttributes()) == false)
return false;
if (other.getStartLambdaFunctionFailedEventAttributes() == null ^ this.getStartLambdaFunctionFailedEventAttributes() == null)
return false;
if (other.getStartLambdaFunctionFailedEventAttributes() != null
&& other.getStartLambdaFunctionFailedEventAttributes().equals(this.getStartLambdaFunctionFailedEventAttributes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEventTimestamp() == null) ? 0 : getEventTimestamp().hashCode());
hashCode = prime * hashCode + ((getEventType() == null) ? 0 : getEventType().hashCode());
hashCode = prime * hashCode + ((getEventId() == null) ? 0 : getEventId().hashCode());
hashCode = prime * hashCode + ((getWorkflowExecutionStartedEventAttributes() == null) ? 0 : getWorkflowExecutionStartedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getWorkflowExecutionCompletedEventAttributes() == null) ? 0 : getWorkflowExecutionCompletedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getCompleteWorkflowExecutionFailedEventAttributes() == null) ? 0 : getCompleteWorkflowExecutionFailedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getWorkflowExecutionFailedEventAttributes() == null) ? 0 : getWorkflowExecutionFailedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getFailWorkflowExecutionFailedEventAttributes() == null) ? 0 : getFailWorkflowExecutionFailedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getWorkflowExecutionTimedOutEventAttributes() == null) ? 0 : getWorkflowExecutionTimedOutEventAttributes().hashCode());
hashCode = prime * hashCode + ((getWorkflowExecutionCanceledEventAttributes() == null) ? 0 : getWorkflowExecutionCanceledEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getCancelWorkflowExecutionFailedEventAttributes() == null) ? 0 : getCancelWorkflowExecutionFailedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getWorkflowExecutionContinuedAsNewEventAttributes() == null) ? 0 : getWorkflowExecutionContinuedAsNewEventAttributes().hashCode());
hashCode = prime
* hashCode
+ ((getContinueAsNewWorkflowExecutionFailedEventAttributes() == null) ? 0 : getContinueAsNewWorkflowExecutionFailedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getWorkflowExecutionTerminatedEventAttributes() == null) ? 0 : getWorkflowExecutionTerminatedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getWorkflowExecutionCancelRequestedEventAttributes() == null) ? 0 : getWorkflowExecutionCancelRequestedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getDecisionTaskScheduledEventAttributes() == null) ? 0 : getDecisionTaskScheduledEventAttributes().hashCode());
hashCode = prime * hashCode + ((getDecisionTaskStartedEventAttributes() == null) ? 0 : getDecisionTaskStartedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getDecisionTaskCompletedEventAttributes() == null) ? 0 : getDecisionTaskCompletedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getDecisionTaskTimedOutEventAttributes() == null) ? 0 : getDecisionTaskTimedOutEventAttributes().hashCode());
hashCode = prime * hashCode + ((getActivityTaskScheduledEventAttributes() == null) ? 0 : getActivityTaskScheduledEventAttributes().hashCode());
hashCode = prime * hashCode + ((getActivityTaskStartedEventAttributes() == null) ? 0 : getActivityTaskStartedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getActivityTaskCompletedEventAttributes() == null) ? 0 : getActivityTaskCompletedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getActivityTaskFailedEventAttributes() == null) ? 0 : getActivityTaskFailedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getActivityTaskTimedOutEventAttributes() == null) ? 0 : getActivityTaskTimedOutEventAttributes().hashCode());
hashCode = prime * hashCode + ((getActivityTaskCanceledEventAttributes() == null) ? 0 : getActivityTaskCanceledEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getActivityTaskCancelRequestedEventAttributes() == null) ? 0 : getActivityTaskCancelRequestedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getWorkflowExecutionSignaledEventAttributes() == null) ? 0 : getWorkflowExecutionSignaledEventAttributes().hashCode());
hashCode = prime * hashCode + ((getMarkerRecordedEventAttributes() == null) ? 0 : getMarkerRecordedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getRecordMarkerFailedEventAttributes() == null) ? 0 : getRecordMarkerFailedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getTimerStartedEventAttributes() == null) ? 0 : getTimerStartedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getTimerFiredEventAttributes() == null) ? 0 : getTimerFiredEventAttributes().hashCode());
hashCode = prime * hashCode + ((getTimerCanceledEventAttributes() == null) ? 0 : getTimerCanceledEventAttributes().hashCode());
hashCode = prime
* hashCode
+ ((getStartChildWorkflowExecutionInitiatedEventAttributes() == null) ? 0 : getStartChildWorkflowExecutionInitiatedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getChildWorkflowExecutionStartedEventAttributes() == null) ? 0 : getChildWorkflowExecutionStartedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getChildWorkflowExecutionCompletedEventAttributes() == null) ? 0 : getChildWorkflowExecutionCompletedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getChildWorkflowExecutionFailedEventAttributes() == null) ? 0 : getChildWorkflowExecutionFailedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getChildWorkflowExecutionTimedOutEventAttributes() == null) ? 0 : getChildWorkflowExecutionTimedOutEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getChildWorkflowExecutionCanceledEventAttributes() == null) ? 0 : getChildWorkflowExecutionCanceledEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getChildWorkflowExecutionTerminatedEventAttributes() == null) ? 0 : getChildWorkflowExecutionTerminatedEventAttributes().hashCode());
hashCode = prime
* hashCode
+ ((getSignalExternalWorkflowExecutionInitiatedEventAttributes() == null) ? 0 : getSignalExternalWorkflowExecutionInitiatedEventAttributes()
.hashCode());
hashCode = prime * hashCode
+ ((getExternalWorkflowExecutionSignaledEventAttributes() == null) ? 0 : getExternalWorkflowExecutionSignaledEventAttributes().hashCode());
hashCode = prime
* hashCode
+ ((getSignalExternalWorkflowExecutionFailedEventAttributes() == null) ? 0 : getSignalExternalWorkflowExecutionFailedEventAttributes()
.hashCode());
hashCode = prime
* hashCode
+ ((getExternalWorkflowExecutionCancelRequestedEventAttributes() == null) ? 0 : getExternalWorkflowExecutionCancelRequestedEventAttributes()
.hashCode());
hashCode = prime
* hashCode
+ ((getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes() == null) ? 0
: getRequestCancelExternalWorkflowExecutionInitiatedEventAttributes().hashCode());
hashCode = prime
* hashCode
+ ((getRequestCancelExternalWorkflowExecutionFailedEventAttributes() == null) ? 0
: getRequestCancelExternalWorkflowExecutionFailedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getScheduleActivityTaskFailedEventAttributes() == null) ? 0 : getScheduleActivityTaskFailedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getRequestCancelActivityTaskFailedEventAttributes() == null) ? 0 : getRequestCancelActivityTaskFailedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getStartTimerFailedEventAttributes() == null) ? 0 : getStartTimerFailedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getCancelTimerFailedEventAttributes() == null) ? 0 : getCancelTimerFailedEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getStartChildWorkflowExecutionFailedEventAttributes() == null) ? 0 : getStartChildWorkflowExecutionFailedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getLambdaFunctionScheduledEventAttributes() == null) ? 0 : getLambdaFunctionScheduledEventAttributes().hashCode());
hashCode = prime * hashCode + ((getLambdaFunctionStartedEventAttributes() == null) ? 0 : getLambdaFunctionStartedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getLambdaFunctionCompletedEventAttributes() == null) ? 0 : getLambdaFunctionCompletedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getLambdaFunctionFailedEventAttributes() == null) ? 0 : getLambdaFunctionFailedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getLambdaFunctionTimedOutEventAttributes() == null) ? 0 : getLambdaFunctionTimedOutEventAttributes().hashCode());
hashCode = prime * hashCode
+ ((getScheduleLambdaFunctionFailedEventAttributes() == null) ? 0 : getScheduleLambdaFunctionFailedEventAttributes().hashCode());
hashCode = prime * hashCode + ((getStartLambdaFunctionFailedEventAttributes() == null) ? 0 : getStartLambdaFunctionFailedEventAttributes().hashCode());
return hashCode;
}
@Override
public HistoryEvent clone() {
try {
return (HistoryEvent) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simpleworkflow.model.transform.HistoryEventMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}