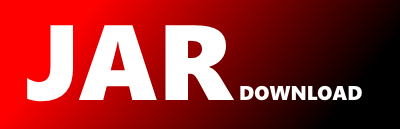
com.amazonaws.services.simpleworkflow.model.PollForDecisionTaskRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-simpleworkflow Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simpleworkflow.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PollForDecisionTaskRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the domain containing the task lists to poll.
*
*/
private String domain;
/**
*
* Specifies the task list to poll for decision tasks.
*
*
* The specified string must not contain a :
(colon), /
(slash), |
(vertical
* bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also, it must
* not be the literal string arn
.
*
*/
private TaskList taskList;
/**
*
* Identity of the decider making the request, which is recorded in the DecisionTaskStarted event in the workflow
* history. This enables diagnostic tracing when problems arise. The form of this identity is user defined.
*
*/
private String identity;
/**
*
* If NextPageToken
is returned there are more results available. The value of
* NextPageToken
is a unique pagination token for each page. Make the call again using the returned
* token to retrieve the next page. Keep all other arguments unchanged. Each pagination token expires after 24
* hours. Using an expired pagination token will return a 400
error: "
* Specified token has exceeded its maximum lifetime
".
*
*
* The configured maximumPageSize
determines how many results can be returned in a single call.
*
*
*
* The nextPageToken
returned by this action cannot be used with GetWorkflowExecutionHistory to
* get the next page. You must call PollForDecisionTask again (with the nextPageToken
) to
* retrieve the next page of history records. Calling PollForDecisionTask with a nextPageToken
* doesn't return a new decision task.
*
*
*/
private String nextPageToken;
/**
*
* The maximum number of results that are returned per call. Use nextPageToken
to obtain further pages
* of results.
*
*
* This is an upper limit only; the actual number of results returned per call may be fewer than the specified
* maximum.
*
*/
private Integer maximumPageSize;
/**
*
* When set to true
, returns the events in reverse order. By default the results are returned in
* ascending order of the eventTimestamp
of the events.
*
*/
private Boolean reverseOrder;
/**
*
* When set to true
, returns the events with eventTimestamp
greater than or equal to
* eventTimestamp
of the most recent DecisionTaskStarted
event. By default, this parameter
* is set to false
.
*
*/
private Boolean startAtPreviousStartedEvent;
/**
*
* The name of the domain containing the task lists to poll.
*
*
* @param domain
* The name of the domain containing the task lists to poll.
*/
public void setDomain(String domain) {
this.domain = domain;
}
/**
*
* The name of the domain containing the task lists to poll.
*
*
* @return The name of the domain containing the task lists to poll.
*/
public String getDomain() {
return this.domain;
}
/**
*
* The name of the domain containing the task lists to poll.
*
*
* @param domain
* The name of the domain containing the task lists to poll.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForDecisionTaskRequest withDomain(String domain) {
setDomain(domain);
return this;
}
/**
*
* Specifies the task list to poll for decision tasks.
*
*
* The specified string must not contain a :
(colon), /
(slash), |
(vertical
* bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also, it must
* not be the literal string arn
.
*
*
* @param taskList
* Specifies the task list to poll for decision tasks.
*
* The specified string must not contain a :
(colon), /
(slash), |
* (vertical bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also,
* it must not be the literal string arn
.
*/
public void setTaskList(TaskList taskList) {
this.taskList = taskList;
}
/**
*
* Specifies the task list to poll for decision tasks.
*
*
* The specified string must not contain a :
(colon), /
(slash), |
(vertical
* bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also, it must
* not be the literal string arn
.
*
*
* @return Specifies the task list to poll for decision tasks.
*
* The specified string must not contain a :
(colon), /
(slash), |
* (vertical bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
).
* Also, it must not be the literal string arn
.
*/
public TaskList getTaskList() {
return this.taskList;
}
/**
*
* Specifies the task list to poll for decision tasks.
*
*
* The specified string must not contain a :
(colon), /
(slash), |
(vertical
* bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also, it must
* not be the literal string arn
.
*
*
* @param taskList
* Specifies the task list to poll for decision tasks.
*
* The specified string must not contain a :
(colon), /
(slash), |
* (vertical bar), or any control characters (\u0000-\u001f
| \u007f-\u009f
). Also,
* it must not be the literal string arn
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForDecisionTaskRequest withTaskList(TaskList taskList) {
setTaskList(taskList);
return this;
}
/**
*
* Identity of the decider making the request, which is recorded in the DecisionTaskStarted event in the workflow
* history. This enables diagnostic tracing when problems arise. The form of this identity is user defined.
*
*
* @param identity
* Identity of the decider making the request, which is recorded in the DecisionTaskStarted event in the
* workflow history. This enables diagnostic tracing when problems arise. The form of this identity is user
* defined.
*/
public void setIdentity(String identity) {
this.identity = identity;
}
/**
*
* Identity of the decider making the request, which is recorded in the DecisionTaskStarted event in the workflow
* history. This enables diagnostic tracing when problems arise. The form of this identity is user defined.
*
*
* @return Identity of the decider making the request, which is recorded in the DecisionTaskStarted event in the
* workflow history. This enables diagnostic tracing when problems arise. The form of this identity is user
* defined.
*/
public String getIdentity() {
return this.identity;
}
/**
*
* Identity of the decider making the request, which is recorded in the DecisionTaskStarted event in the workflow
* history. This enables diagnostic tracing when problems arise. The form of this identity is user defined.
*
*
* @param identity
* Identity of the decider making the request, which is recorded in the DecisionTaskStarted event in the
* workflow history. This enables diagnostic tracing when problems arise. The form of this identity is user
* defined.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForDecisionTaskRequest withIdentity(String identity) {
setIdentity(identity);
return this;
}
/**
*
* If NextPageToken
is returned there are more results available. The value of
* NextPageToken
is a unique pagination token for each page. Make the call again using the returned
* token to retrieve the next page. Keep all other arguments unchanged. Each pagination token expires after 24
* hours. Using an expired pagination token will return a 400
error: "
* Specified token has exceeded its maximum lifetime
".
*
*
* The configured maximumPageSize
determines how many results can be returned in a single call.
*
*
*
* The nextPageToken
returned by this action cannot be used with GetWorkflowExecutionHistory to
* get the next page. You must call PollForDecisionTask again (with the nextPageToken
) to
* retrieve the next page of history records. Calling PollForDecisionTask with a nextPageToken
* doesn't return a new decision task.
*
*
*
* @param nextPageToken
* If NextPageToken
is returned there are more results available. The value of
* NextPageToken
is a unique pagination token for each page. Make the call again using the
* returned token to retrieve the next page. Keep all other arguments unchanged. Each pagination token
* expires after 24 hours. Using an expired pagination token will return a 400
error: "
* Specified token has exceeded its maximum lifetime
".
*
* The configured maximumPageSize
determines how many results can be returned in a single call.
*
*
*
* The nextPageToken
returned by this action cannot be used with
* GetWorkflowExecutionHistory to get the next page. You must call PollForDecisionTask again
* (with the nextPageToken
) to retrieve the next page of history records. Calling
* PollForDecisionTask with a nextPageToken
doesn't return a new decision task.
*
*/
public void setNextPageToken(String nextPageToken) {
this.nextPageToken = nextPageToken;
}
/**
*
* If NextPageToken
is returned there are more results available. The value of
* NextPageToken
is a unique pagination token for each page. Make the call again using the returned
* token to retrieve the next page. Keep all other arguments unchanged. Each pagination token expires after 24
* hours. Using an expired pagination token will return a 400
error: "
* Specified token has exceeded its maximum lifetime
".
*
*
* The configured maximumPageSize
determines how many results can be returned in a single call.
*
*
*
* The nextPageToken
returned by this action cannot be used with GetWorkflowExecutionHistory to
* get the next page. You must call PollForDecisionTask again (with the nextPageToken
) to
* retrieve the next page of history records. Calling PollForDecisionTask with a nextPageToken
* doesn't return a new decision task.
*
*
*
* @return If NextPageToken
is returned there are more results available. The value of
* NextPageToken
is a unique pagination token for each page. Make the call again using the
* returned token to retrieve the next page. Keep all other arguments unchanged. Each pagination token
* expires after 24 hours. Using an expired pagination token will return a 400
error: "
* Specified token has exceeded its maximum lifetime
".
*
* The configured maximumPageSize
determines how many results can be returned in a single call.
*
*
*
* The nextPageToken
returned by this action cannot be used with
* GetWorkflowExecutionHistory to get the next page. You must call PollForDecisionTask again
* (with the nextPageToken
) to retrieve the next page of history records. Calling
* PollForDecisionTask with a nextPageToken
doesn't return a new decision task.
*
*/
public String getNextPageToken() {
return this.nextPageToken;
}
/**
*
* If NextPageToken
is returned there are more results available. The value of
* NextPageToken
is a unique pagination token for each page. Make the call again using the returned
* token to retrieve the next page. Keep all other arguments unchanged. Each pagination token expires after 24
* hours. Using an expired pagination token will return a 400
error: "
* Specified token has exceeded its maximum lifetime
".
*
*
* The configured maximumPageSize
determines how many results can be returned in a single call.
*
*
*
* The nextPageToken
returned by this action cannot be used with GetWorkflowExecutionHistory to
* get the next page. You must call PollForDecisionTask again (with the nextPageToken
) to
* retrieve the next page of history records. Calling PollForDecisionTask with a nextPageToken
* doesn't return a new decision task.
*
*
*
* @param nextPageToken
* If NextPageToken
is returned there are more results available. The value of
* NextPageToken
is a unique pagination token for each page. Make the call again using the
* returned token to retrieve the next page. Keep all other arguments unchanged. Each pagination token
* expires after 24 hours. Using an expired pagination token will return a 400
error: "
* Specified token has exceeded its maximum lifetime
".
*
* The configured maximumPageSize
determines how many results can be returned in a single call.
*
*
*
* The nextPageToken
returned by this action cannot be used with
* GetWorkflowExecutionHistory to get the next page. You must call PollForDecisionTask again
* (with the nextPageToken
) to retrieve the next page of history records. Calling
* PollForDecisionTask with a nextPageToken
doesn't return a new decision task.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForDecisionTaskRequest withNextPageToken(String nextPageToken) {
setNextPageToken(nextPageToken);
return this;
}
/**
*
* The maximum number of results that are returned per call. Use nextPageToken
to obtain further pages
* of results.
*
*
* This is an upper limit only; the actual number of results returned per call may be fewer than the specified
* maximum.
*
*
* @param maximumPageSize
* The maximum number of results that are returned per call. Use nextPageToken
to obtain further
* pages of results.
*
* This is an upper limit only; the actual number of results returned per call may be fewer than the
* specified maximum.
*/
public void setMaximumPageSize(Integer maximumPageSize) {
this.maximumPageSize = maximumPageSize;
}
/**
*
* The maximum number of results that are returned per call. Use nextPageToken
to obtain further pages
* of results.
*
*
* This is an upper limit only; the actual number of results returned per call may be fewer than the specified
* maximum.
*
*
* @return The maximum number of results that are returned per call. Use nextPageToken
to obtain
* further pages of results.
*
* This is an upper limit only; the actual number of results returned per call may be fewer than the
* specified maximum.
*/
public Integer getMaximumPageSize() {
return this.maximumPageSize;
}
/**
*
* The maximum number of results that are returned per call. Use nextPageToken
to obtain further pages
* of results.
*
*
* This is an upper limit only; the actual number of results returned per call may be fewer than the specified
* maximum.
*
*
* @param maximumPageSize
* The maximum number of results that are returned per call. Use nextPageToken
to obtain further
* pages of results.
*
* This is an upper limit only; the actual number of results returned per call may be fewer than the
* specified maximum.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForDecisionTaskRequest withMaximumPageSize(Integer maximumPageSize) {
setMaximumPageSize(maximumPageSize);
return this;
}
/**
*
* When set to true
, returns the events in reverse order. By default the results are returned in
* ascending order of the eventTimestamp
of the events.
*
*
* @param reverseOrder
* When set to true
, returns the events in reverse order. By default the results are returned in
* ascending order of the eventTimestamp
of the events.
*/
public void setReverseOrder(Boolean reverseOrder) {
this.reverseOrder = reverseOrder;
}
/**
*
* When set to true
, returns the events in reverse order. By default the results are returned in
* ascending order of the eventTimestamp
of the events.
*
*
* @return When set to true
, returns the events in reverse order. By default the results are returned
* in ascending order of the eventTimestamp
of the events.
*/
public Boolean getReverseOrder() {
return this.reverseOrder;
}
/**
*
* When set to true
, returns the events in reverse order. By default the results are returned in
* ascending order of the eventTimestamp
of the events.
*
*
* @param reverseOrder
* When set to true
, returns the events in reverse order. By default the results are returned in
* ascending order of the eventTimestamp
of the events.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForDecisionTaskRequest withReverseOrder(Boolean reverseOrder) {
setReverseOrder(reverseOrder);
return this;
}
/**
*
* When set to true
, returns the events in reverse order. By default the results are returned in
* ascending order of the eventTimestamp
of the events.
*
*
* @return When set to true
, returns the events in reverse order. By default the results are returned
* in ascending order of the eventTimestamp
of the events.
*/
public Boolean isReverseOrder() {
return this.reverseOrder;
}
/**
*
* When set to true
, returns the events with eventTimestamp
greater than or equal to
* eventTimestamp
of the most recent DecisionTaskStarted
event. By default, this parameter
* is set to false
.
*
*
* @param startAtPreviousStartedEvent
* When set to true
, returns the events with eventTimestamp
greater than or equal
* to eventTimestamp
of the most recent DecisionTaskStarted
event. By default, this
* parameter is set to false
.
*/
public void setStartAtPreviousStartedEvent(Boolean startAtPreviousStartedEvent) {
this.startAtPreviousStartedEvent = startAtPreviousStartedEvent;
}
/**
*
* When set to true
, returns the events with eventTimestamp
greater than or equal to
* eventTimestamp
of the most recent DecisionTaskStarted
event. By default, this parameter
* is set to false
.
*
*
* @return When set to true
, returns the events with eventTimestamp
greater than or equal
* to eventTimestamp
of the most recent DecisionTaskStarted
event. By default,
* this parameter is set to false
.
*/
public Boolean getStartAtPreviousStartedEvent() {
return this.startAtPreviousStartedEvent;
}
/**
*
* When set to true
, returns the events with eventTimestamp
greater than or equal to
* eventTimestamp
of the most recent DecisionTaskStarted
event. By default, this parameter
* is set to false
.
*
*
* @param startAtPreviousStartedEvent
* When set to true
, returns the events with eventTimestamp
greater than or equal
* to eventTimestamp
of the most recent DecisionTaskStarted
event. By default, this
* parameter is set to false
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForDecisionTaskRequest withStartAtPreviousStartedEvent(Boolean startAtPreviousStartedEvent) {
setStartAtPreviousStartedEvent(startAtPreviousStartedEvent);
return this;
}
/**
*
* When set to true
, returns the events with eventTimestamp
greater than or equal to
* eventTimestamp
of the most recent DecisionTaskStarted
event. By default, this parameter
* is set to false
.
*
*
* @return When set to true
, returns the events with eventTimestamp
greater than or equal
* to eventTimestamp
of the most recent DecisionTaskStarted
event. By default,
* this parameter is set to false
.
*/
public Boolean isStartAtPreviousStartedEvent() {
return this.startAtPreviousStartedEvent;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomain() != null)
sb.append("Domain: ").append(getDomain()).append(",");
if (getTaskList() != null)
sb.append("TaskList: ").append(getTaskList()).append(",");
if (getIdentity() != null)
sb.append("Identity: ").append(getIdentity()).append(",");
if (getNextPageToken() != null)
sb.append("NextPageToken: ").append(getNextPageToken()).append(",");
if (getMaximumPageSize() != null)
sb.append("MaximumPageSize: ").append(getMaximumPageSize()).append(",");
if (getReverseOrder() != null)
sb.append("ReverseOrder: ").append(getReverseOrder()).append(",");
if (getStartAtPreviousStartedEvent() != null)
sb.append("StartAtPreviousStartedEvent: ").append(getStartAtPreviousStartedEvent());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PollForDecisionTaskRequest == false)
return false;
PollForDecisionTaskRequest other = (PollForDecisionTaskRequest) obj;
if (other.getDomain() == null ^ this.getDomain() == null)
return false;
if (other.getDomain() != null && other.getDomain().equals(this.getDomain()) == false)
return false;
if (other.getTaskList() == null ^ this.getTaskList() == null)
return false;
if (other.getTaskList() != null && other.getTaskList().equals(this.getTaskList()) == false)
return false;
if (other.getIdentity() == null ^ this.getIdentity() == null)
return false;
if (other.getIdentity() != null && other.getIdentity().equals(this.getIdentity()) == false)
return false;
if (other.getNextPageToken() == null ^ this.getNextPageToken() == null)
return false;
if (other.getNextPageToken() != null && other.getNextPageToken().equals(this.getNextPageToken()) == false)
return false;
if (other.getMaximumPageSize() == null ^ this.getMaximumPageSize() == null)
return false;
if (other.getMaximumPageSize() != null && other.getMaximumPageSize().equals(this.getMaximumPageSize()) == false)
return false;
if (other.getReverseOrder() == null ^ this.getReverseOrder() == null)
return false;
if (other.getReverseOrder() != null && other.getReverseOrder().equals(this.getReverseOrder()) == false)
return false;
if (other.getStartAtPreviousStartedEvent() == null ^ this.getStartAtPreviousStartedEvent() == null)
return false;
if (other.getStartAtPreviousStartedEvent() != null && other.getStartAtPreviousStartedEvent().equals(this.getStartAtPreviousStartedEvent()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDomain() == null) ? 0 : getDomain().hashCode());
hashCode = prime * hashCode + ((getTaskList() == null) ? 0 : getTaskList().hashCode());
hashCode = prime * hashCode + ((getIdentity() == null) ? 0 : getIdentity().hashCode());
hashCode = prime * hashCode + ((getNextPageToken() == null) ? 0 : getNextPageToken().hashCode());
hashCode = prime * hashCode + ((getMaximumPageSize() == null) ? 0 : getMaximumPageSize().hashCode());
hashCode = prime * hashCode + ((getReverseOrder() == null) ? 0 : getReverseOrder().hashCode());
hashCode = prime * hashCode + ((getStartAtPreviousStartedEvent() == null) ? 0 : getStartAtPreviousStartedEvent().hashCode());
return hashCode;
}
@Override
public PollForDecisionTaskRequest clone() {
return (PollForDecisionTaskRequest) super.clone();
}
}