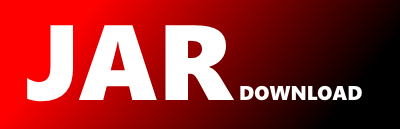
com.amazonaws.services.simplesystemsmanagement.AWSSimpleSystemsManagement Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.simplesystemsmanagement.model.*;
import com.amazonaws.services.simplesystemsmanagement.waiters.AWSSimpleSystemsManagementWaiters;
/**
* Interface for accessing Amazon SSM.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.simplesystemsmanagement.AbstractAWSSimpleSystemsManagement} instead.
*
*
*
* Amazon Web Services Systems Manager is the operations hub for your Amazon Web Services applications and resources and
* a secure end-to-end management solution for hybrid cloud environments that enables safe and secure operations at
* scale.
*
*
* This reference is intended to be used with the Amazon Web Services Systems Manager User
* Guide. To get started, see Setting up Amazon
* Web Services Systems Manager.
*
*
* Related resources
*
*
* -
*
* For information about each of the capabilities that comprise Systems Manager, see Systems Manager capabilities in the Amazon Web Services Systems Manager User Guide.
*
*
* -
*
* For details about predefined runbooks for Automation, a capability of Amazon Web Services Systems Manager, see the
* Systems Manager Automation runbook reference .
*
*
* -
*
* For information about AppConfig, a capability of Systems Manager, see the AppConfig User Guide and the AppConfig API Reference .
*
*
* -
*
* For information about Incident Manager, a capability of Systems Manager, see the Systems Manager Incident Manager User Guide
* and the Systems Manager Incident
* Manager API Reference .
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSSimpleSystemsManagement {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "ssm";
/**
* Overrides the default endpoint for this client ("https://ssm.us-east-1.amazonaws.com"). Callers can use this
* method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "ssm.us-east-1.amazonaws.com") or a full URL, including the protocol
* (ex: "https://ssm.us-east-1.amazonaws.com"). If the protocol is not specified here, the default protocol from
* this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "ssm.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "https://ssm.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will communicate
* with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSSimpleSystemsManagement#setEndpoint(String)}, sets the regional endpoint for this
* client's service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Adds or overwrites one or more tags for the specified resource. Tags are metadata that you can assign to
* your automations, documents, managed nodes, maintenance windows, Parameter Store parameters, and patch baselines.
* Tags enable you to categorize your resources in different ways, for example, by purpose, owner, or environment.
* Each tag consists of a key and an optional value, both of which you define. For example, you could define a set
* of tags for your account's managed nodes that helps you track each node's owner and stack level. For example:
*
*
* -
*
* Key=Owner,Value=DbAdmin
*
*
* -
*
* Key=Owner,Value=SysAdmin
*
*
* -
*
* Key=Owner,Value=Dev
*
*
* -
*
* Key=Stack,Value=Production
*
*
* -
*
* Key=Stack,Value=Pre-Production
*
*
* -
*
* Key=Stack,Value=Test
*
*
*
*
* Most resources can have a maximum of 50 tags. Automations can have a maximum of 5 tags.
*
*
* We recommend that you devise a set of tag keys that meets your needs for each resource type. Using a consistent
* set of tag keys makes it easier for you to manage your resources. You can search and filter the resources based
* on the tags you add. Tags don't have any semantic meaning to and are interpreted strictly as a string of
* characters.
*
*
* For more information about using tags with Amazon Elastic Compute Cloud (Amazon EC2) instances, see Tag your Amazon EC2 resources in
* the Amazon EC2 User Guide.
*
*
* @param addTagsToResourceRequest
* @return Result of the AddTagsToResource operation returned by the service.
* @throws InvalidResourceTypeException
* The resource type isn't valid. For example, if you are attempting to tag an EC2 instance, the instance
* must be a registered managed node.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws TooManyTagsErrorException
* The Targets
parameter includes too many tags. Remove one or more tags and try the command
* again.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @sample AWSSimpleSystemsManagement.AddTagsToResource
* @see AWS API
* Documentation
*/
AddTagsToResourceResult addTagsToResource(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Associates a related item to a Systems Manager OpsCenter OpsItem. For example, you can associate an Incident
* Manager incident or analysis with an OpsItem. Incident Manager and OpsCenter are capabilities of Amazon Web
* Services Systems Manager.
*
*
* @param associateOpsItemRelatedItemRequest
* @return Result of the AssociateOpsItemRelatedItem operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws OpsItemNotFoundException
* The specified OpsItem ID doesn't exist. Verify the ID and try again.
* @throws OpsItemLimitExceededException
* The request caused OpsItems to exceed one or more quotas.
* @throws OpsItemInvalidParameterException
* A specified parameter argument isn't valid. Verify the available arguments and try again.
* @throws OpsItemRelatedItemAlreadyExistsException
* The Amazon Resource Name (ARN) is already associated with the OpsItem.
* @throws OpsItemConflictException
* The specified OpsItem is in the process of being deleted.
* @sample AWSSimpleSystemsManagement.AssociateOpsItemRelatedItem
* @see AWS API Documentation
*/
AssociateOpsItemRelatedItemResult associateOpsItemRelatedItem(AssociateOpsItemRelatedItemRequest associateOpsItemRelatedItemRequest);
/**
*
* Attempts to cancel the command specified by the Command ID. There is no guarantee that the command will be
* terminated and the underlying process stopped.
*
*
* @param cancelCommandRequest
* @return Result of the CancelCommand operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidCommandIdException
* The specified command ID isn't valid. Verify the ID and try again.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws DuplicateInstanceIdException
* You can't specify a managed node ID in more than one association.
* @sample AWSSimpleSystemsManagement.CancelCommand
* @see AWS API
* Documentation
*/
CancelCommandResult cancelCommand(CancelCommandRequest cancelCommandRequest);
/**
*
* Stops a maintenance window execution that is already in progress and cancels any tasks in the window that haven't
* already starting running. Tasks already in progress will continue to completion.
*
*
* @param cancelMaintenanceWindowExecutionRequest
* @return Result of the CancelMaintenanceWindowExecution operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @sample AWSSimpleSystemsManagement.CancelMaintenanceWindowExecution
* @see AWS API Documentation
*/
CancelMaintenanceWindowExecutionResult cancelMaintenanceWindowExecution(CancelMaintenanceWindowExecutionRequest cancelMaintenanceWindowExecutionRequest);
/**
*
* Generates an activation code and activation ID you can use to register your on-premises servers, edge devices, or
* virtual machine (VM) with Amazon Web Services Systems Manager. Registering these machines with Systems Manager
* makes it possible to manage them using Systems Manager capabilities. You use the activation code and ID when
* installing SSM Agent on machines in your hybrid environment. For more information about requirements for managing
* on-premises machines using Systems Manager, see Setting
* up Amazon Web Services Systems Manager for hybrid and multicloud environments in the Amazon Web Services
* Systems Manager User Guide.
*
*
*
* Amazon Elastic Compute Cloud (Amazon EC2) instances, edge devices, and on-premises servers and VMs that are
* configured for Systems Manager are all called managed nodes.
*
*
*
* @param createActivationRequest
* @return Result of the CreateActivation operation returned by the service.
* @throws InvalidParametersException
* You must specify values for all required parameters in the Amazon Web Services Systems Manager document
* (SSM document). You can only supply values to parameters defined in the SSM document.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.CreateActivation
* @see AWS API
* Documentation
*/
CreateActivationResult createActivation(CreateActivationRequest createActivationRequest);
/**
*
* A State Manager association defines the state that you want to maintain on your managed nodes. For example, an
* association can specify that anti-virus software must be installed and running on your managed nodes, or that
* certain ports must be closed. For static targets, the association specifies a schedule for when the configuration
* is reapplied. For dynamic targets, such as an Amazon Web Services resource group or an Amazon Web Services
* autoscaling group, State Manager, a capability of Amazon Web Services Systems Manager applies the configuration
* when new managed nodes are added to the group. The association also specifies actions to take when applying the
* configuration. For example, an association for anti-virus software might run once a day. If the software isn't
* installed, then State Manager installs it. If the software is installed, but the service isn't running, then the
* association might instruct State Manager to start the service.
*
*
* @param createAssociationRequest
* @return Result of the CreateAssociation operation returned by the service.
* @throws AssociationAlreadyExistsException
* The specified association already exists.
* @throws AssociationLimitExceededException
* You can have at most 2,000 active associations.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws UnsupportedPlatformTypeException
* The document doesn't support the platform type of the given managed node IDs. For example, you sent an
* document for a Windows managed node to a Linux node.
* @throws InvalidOutputLocationException
* The output location isn't valid or doesn't exist.
* @throws InvalidParametersException
* You must specify values for all required parameters in the Amazon Web Services Systems Manager document
* (SSM document). You can only supply values to parameters defined in the SSM document.
* @throws InvalidTargetException
* The target isn't valid or doesn't exist. It might not be configured for Systems Manager or you might not
* have permission to perform the operation.
* @throws InvalidScheduleException
* The schedule is invalid. Verify your cron or rate expression and try again.
* @throws InvalidTargetMapsException
* TargetMap parameter isn't valid.
* @throws InvalidTagException
* The specified tag key or value isn't valid.
* @sample AWSSimpleSystemsManagement.CreateAssociation
* @see AWS API
* Documentation
*/
CreateAssociationResult createAssociation(CreateAssociationRequest createAssociationRequest);
/**
*
* Associates the specified Amazon Web Services Systems Manager document (SSM document) with the specified managed
* nodes or targets.
*
*
* When you associate a document with one or more managed nodes using IDs or tags, Amazon Web Services Systems
* Manager Agent (SSM Agent) running on the managed node processes the document and configures the node as
* specified.
*
*
* If you associate a document with a managed node that already has an associated document, the system returns the
* AssociationAlreadyExists exception.
*
*
* @param createAssociationBatchRequest
* @return Result of the CreateAssociationBatch operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidParametersException
* You must specify values for all required parameters in the Amazon Web Services Systems Manager document
* (SSM document). You can only supply values to parameters defined in the SSM document.
* @throws DuplicateInstanceIdException
* You can't specify a managed node ID in more than one association.
* @throws AssociationLimitExceededException
* You can have at most 2,000 active associations.
* @throws UnsupportedPlatformTypeException
* The document doesn't support the platform type of the given managed node IDs. For example, you sent an
* document for a Windows managed node to a Linux node.
* @throws InvalidOutputLocationException
* The output location isn't valid or doesn't exist.
* @throws InvalidTargetException
* The target isn't valid or doesn't exist. It might not be configured for Systems Manager or you might not
* have permission to perform the operation.
* @throws InvalidScheduleException
* The schedule is invalid. Verify your cron or rate expression and try again.
* @throws InvalidTargetMapsException
* TargetMap parameter isn't valid.
* @sample AWSSimpleSystemsManagement.CreateAssociationBatch
* @see AWS API
* Documentation
*/
CreateAssociationBatchResult createAssociationBatch(CreateAssociationBatchRequest createAssociationBatchRequest);
/**
*
* Creates a Amazon Web Services Systems Manager (SSM document). An SSM document defines the actions that Systems
* Manager performs on your managed nodes. For more information about SSM documents, including information about
* supported schemas, features, and syntax, see Amazon Web Services
* Systems Manager Documents in the Amazon Web Services Systems Manager User Guide.
*
*
* @param createDocumentRequest
* @return Result of the CreateDocument operation returned by the service.
* @throws DocumentAlreadyExistsException
* The specified document already exists.
* @throws MaxDocumentSizeExceededException
* The size limit of a document is 64 KB.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentContentException
* The content for the document isn't valid.
* @throws DocumentLimitExceededException
* You can have at most 500 active SSM documents.
* @throws InvalidDocumentSchemaVersionException
* The version of the document schema isn't supported.
* @sample AWSSimpleSystemsManagement.CreateDocument
* @see AWS API
* Documentation
*/
CreateDocumentResult createDocument(CreateDocumentRequest createDocumentRequest);
/**
*
* Creates a new maintenance window.
*
*
*
* The value you specify for Duration
determines the specific end time for the maintenance window based
* on the time it begins. No maintenance window tasks are permitted to start after the resulting endtime minus the
* number of hours you specify for Cutoff
. For example, if the maintenance window starts at 3 PM, the
* duration is three hours, and the value you specify for Cutoff
is one hour, no maintenance window
* tasks can start after 5 PM.
*
*
*
* @param createMaintenanceWindowRequest
* @return Result of the CreateMaintenanceWindow operation returned by the service.
* @throws IdempotentParameterMismatchException
* Error returned when an idempotent operation is retried and the parameters don't match the original call
* to the API with the same idempotency token.
* @throws ResourceLimitExceededException
* Error returned when the caller has exceeded the default resource quotas. For example, too many
* maintenance windows or patch baselines have been created.
*
* For information about resource quotas in Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.CreateMaintenanceWindow
* @see AWS
* API Documentation
*/
CreateMaintenanceWindowResult createMaintenanceWindow(CreateMaintenanceWindowRequest createMaintenanceWindowRequest);
/**
*
* Creates a new OpsItem. You must have permission in Identity and Access Management (IAM) to create a new OpsItem.
* For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param createOpsItemRequest
* @return Result of the CreateOpsItem operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws OpsItemAlreadyExistsException
* The OpsItem already exists.
* @throws OpsItemLimitExceededException
* The request caused OpsItems to exceed one or more quotas.
* @throws OpsItemInvalidParameterException
* A specified parameter argument isn't valid. Verify the available arguments and try again.
* @throws OpsItemAccessDeniedException
* You don't have permission to view OpsItems in the specified account. Verify that your account is
* configured either as a Systems Manager delegated administrator or that you are logged into the
* Organizations management account.
* @sample AWSSimpleSystemsManagement.CreateOpsItem
* @see AWS API
* Documentation
*/
CreateOpsItemResult createOpsItem(CreateOpsItemRequest createOpsItemRequest);
/**
*
* If you create a new application in Application Manager, Amazon Web Services Systems Manager calls this API
* operation to specify information about the new application, including the application type.
*
*
* @param createOpsMetadataRequest
* @return Result of the CreateOpsMetadata operation returned by the service.
* @throws OpsMetadataAlreadyExistsException
* An OpsMetadata object already exists for the selected resource.
* @throws OpsMetadataTooManyUpdatesException
* The system is processing too many concurrent updates. Wait a few moments and try again.
* @throws OpsMetadataInvalidArgumentException
* One of the arguments passed is invalid.
* @throws OpsMetadataLimitExceededException
* Your account reached the maximum number of OpsMetadata objects allowed by Application Manager. The
* maximum is 200 OpsMetadata objects. Delete one or more OpsMetadata object and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.CreateOpsMetadata
* @see AWS API
* Documentation
*/
CreateOpsMetadataResult createOpsMetadata(CreateOpsMetadataRequest createOpsMetadataRequest);
/**
*
* Creates a patch baseline.
*
*
*
* For information about valid key-value pairs in PatchFilters
for each supported operating system
* type, see PatchFilter.
*
*
*
* @param createPatchBaselineRequest
* @return Result of the CreatePatchBaseline operation returned by the service.
* @throws IdempotentParameterMismatchException
* Error returned when an idempotent operation is retried and the parameters don't match the original call
* to the API with the same idempotency token.
* @throws ResourceLimitExceededException
* Error returned when the caller has exceeded the default resource quotas. For example, too many
* maintenance windows or patch baselines have been created.
*
* For information about resource quotas in Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.CreatePatchBaseline
* @see AWS API
* Documentation
*/
CreatePatchBaselineResult createPatchBaseline(CreatePatchBaselineRequest createPatchBaselineRequest);
/**
*
* A resource data sync helps you view data from multiple sources in a single location. Amazon Web Services Systems
* Manager offers two types of resource data sync: SyncToDestination
and SyncFromSource
.
*
*
* You can configure Systems Manager Inventory to use the SyncToDestination
type to synchronize
* Inventory data from multiple Amazon Web Services Regions to a single Amazon Simple Storage Service (Amazon S3)
* bucket. For more information, see Configuring
* resource data sync for Inventory in the Amazon Web Services Systems Manager User Guide.
*
*
* You can configure Systems Manager Explorer to use the SyncFromSource
type to synchronize operational
* work items (OpsItems) and operational data (OpsData) from multiple Amazon Web Services Regions to a single Amazon
* S3 bucket. This type can synchronize OpsItems and OpsData from multiple Amazon Web Services accounts and Amazon
* Web Services Regions or EntireOrganization
by using Organizations. For more information, see Setting up
* Systems Manager Explorer to display data from multiple accounts and Regions in the Amazon Web Services
* Systems Manager User Guide.
*
*
* A resource data sync is an asynchronous operation that returns immediately. After a successful initial sync is
* completed, the system continuously syncs data. To check the status of a sync, use the
* ListResourceDataSync.
*
*
*
* By default, data isn't encrypted in Amazon S3. We strongly recommend that you enable encryption in Amazon S3 to
* ensure secure data storage. We also recommend that you secure access to the Amazon S3 bucket by creating a
* restrictive bucket policy.
*
*
*
* @param createResourceDataSyncRequest
* @return Result of the CreateResourceDataSync operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ResourceDataSyncCountExceededException
* You have exceeded the allowed maximum sync configurations.
* @throws ResourceDataSyncAlreadyExistsException
* A sync configuration with the same name already exists.
* @throws ResourceDataSyncInvalidConfigurationException
* The specified sync configuration is invalid.
* @sample AWSSimpleSystemsManagement.CreateResourceDataSync
* @see AWS API
* Documentation
*/
CreateResourceDataSyncResult createResourceDataSync(CreateResourceDataSyncRequest createResourceDataSyncRequest);
/**
*
* Deletes an activation. You aren't required to delete an activation. If you delete an activation, you can no
* longer use it to register additional managed nodes. Deleting an activation doesn't de-register managed nodes. You
* must manually de-register managed nodes.
*
*
* @param deleteActivationRequest
* @return Result of the DeleteActivation operation returned by the service.
* @throws InvalidActivationIdException
* The activation ID isn't valid. Verify the you entered the correct ActivationId or ActivationCode and try
* again.
* @throws InvalidActivationException
* The activation isn't valid. The activation might have been deleted, or the ActivationId and the
* ActivationCode don't match.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @sample AWSSimpleSystemsManagement.DeleteActivation
* @see AWS API
* Documentation
*/
DeleteActivationResult deleteActivation(DeleteActivationRequest deleteActivationRequest);
/**
*
* Disassociates the specified Amazon Web Services Systems Manager document (SSM document) from the specified
* managed node. If you created the association by using the Targets
parameter, then you must delete
* the association by using the association ID.
*
*
* When you disassociate a document from a managed node, it doesn't change the configuration of the node. To change
* the configuration state of a managed node after you disassociate a document, you must create a new document with
* the desired configuration and associate it with the node.
*
*
* @param deleteAssociationRequest
* @return Result of the DeleteAssociation operation returned by the service.
* @throws AssociationDoesNotExistException
* The specified association doesn't exist.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @sample AWSSimpleSystemsManagement.DeleteAssociation
* @see AWS API
* Documentation
*/
DeleteAssociationResult deleteAssociation(DeleteAssociationRequest deleteAssociationRequest);
/**
*
* Deletes the Amazon Web Services Systems Manager document (SSM document) and all managed node associations to the
* document.
*
*
* Before you delete the document, we recommend that you use DeleteAssociation to disassociate all managed
* nodes that are associated with the document.
*
*
* @param deleteDocumentRequest
* @return Result of the DeleteDocument operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentOperationException
* You attempted to delete a document while it is still shared. You must stop sharing the document before
* you can delete it.
* @throws AssociatedInstancesException
* You must disassociate a document from all managed nodes before you can delete it.
* @sample AWSSimpleSystemsManagement.DeleteDocument
* @see AWS API
* Documentation
*/
DeleteDocumentResult deleteDocument(DeleteDocumentRequest deleteDocumentRequest);
/**
*
* Delete a custom inventory type or the data associated with a custom Inventory type. Deleting a custom inventory
* type is also referred to as deleting a custom inventory schema.
*
*
* @param deleteInventoryRequest
* @return Result of the DeleteInventory operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidTypeNameException
* The parameter type name isn't valid.
* @throws InvalidOptionException
* The delete inventory option specified isn't valid. Verify the option and try again.
* @throws InvalidDeleteInventoryParametersException
* One or more of the parameters specified for the delete operation isn't valid. Verify all parameters and
* try again.
* @throws InvalidInventoryRequestException
* The request isn't valid.
* @sample AWSSimpleSystemsManagement.DeleteInventory
* @see AWS API
* Documentation
*/
DeleteInventoryResult deleteInventory(DeleteInventoryRequest deleteInventoryRequest);
/**
*
* Deletes a maintenance window.
*
*
* @param deleteMaintenanceWindowRequest
* @return Result of the DeleteMaintenanceWindow operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DeleteMaintenanceWindow
* @see AWS
* API Documentation
*/
DeleteMaintenanceWindowResult deleteMaintenanceWindow(DeleteMaintenanceWindowRequest deleteMaintenanceWindowRequest);
/**
*
* Delete an OpsItem. You must have permission in Identity and Access Management (IAM) to delete an OpsItem.
*
*
*
* Note the following important information about this operation.
*
*
* -
*
* Deleting an OpsItem is irreversible. You can't restore a deleted OpsItem.
*
*
* -
*
* This operation uses an eventual consistency model, which means the system can take a few minutes to
* complete this operation. If you delete an OpsItem and immediately call, for example, GetOpsItem, the
* deleted OpsItem might still appear in the response.
*
*
* -
*
* This operation is idempotent. The system doesn't throw an exception if you repeatedly call this operation for the
* same OpsItem. If the first call is successful, all additional calls return the same successful response as the
* first call.
*
*
* -
*
* This operation doesn't support cross-account calls. A delegated administrator or management account can't delete
* OpsItems in other accounts, even if OpsCenter has been set up for cross-account administration. For more
* information about cross-account administration, see Setting up OpsCenter to centrally manage OpsItems across accounts in the Systems Manager User Guide.
*
*
*
*
*
* @param deleteOpsItemRequest
* @return Result of the DeleteOpsItem operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws OpsItemInvalidParameterException
* A specified parameter argument isn't valid. Verify the available arguments and try again.
* @sample AWSSimpleSystemsManagement.DeleteOpsItem
* @see AWS API
* Documentation
*/
DeleteOpsItemResult deleteOpsItem(DeleteOpsItemRequest deleteOpsItemRequest);
/**
*
* Delete OpsMetadata related to an application.
*
*
* @param deleteOpsMetadataRequest
* @return Result of the DeleteOpsMetadata operation returned by the service.
* @throws OpsMetadataNotFoundException
* The OpsMetadata object doesn't exist.
* @throws OpsMetadataInvalidArgumentException
* One of the arguments passed is invalid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DeleteOpsMetadata
* @see AWS API
* Documentation
*/
DeleteOpsMetadataResult deleteOpsMetadata(DeleteOpsMetadataRequest deleteOpsMetadataRequest);
/**
*
* Delete a parameter from the system. After deleting a parameter, wait for at least 30 seconds to create a
* parameter with the same name.
*
*
* @param deleteParameterRequest
* @return Result of the DeleteParameter operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ParameterNotFoundException
* The parameter couldn't be found. Verify the name and try again.
* @sample AWSSimpleSystemsManagement.DeleteParameter
* @see AWS API
* Documentation
*/
DeleteParameterResult deleteParameter(DeleteParameterRequest deleteParameterRequest);
/**
*
* Delete a list of parameters. After deleting a parameter, wait for at least 30 seconds to create a parameter with
* the same name.
*
*
* @param deleteParametersRequest
* @return Result of the DeleteParameters operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DeleteParameters
* @see AWS API
* Documentation
*/
DeleteParametersResult deleteParameters(DeleteParametersRequest deleteParametersRequest);
/**
*
* Deletes a patch baseline.
*
*
* @param deletePatchBaselineRequest
* @return Result of the DeletePatchBaseline operation returned by the service.
* @throws ResourceInUseException
* Error returned if an attempt is made to delete a patch baseline that is registered for a patch group.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DeletePatchBaseline
* @see AWS API
* Documentation
*/
DeletePatchBaselineResult deletePatchBaseline(DeletePatchBaselineRequest deletePatchBaselineRequest);
/**
*
* Deletes a resource data sync configuration. After the configuration is deleted, changes to data on managed nodes
* are no longer synced to or from the target. Deleting a sync configuration doesn't delete data.
*
*
* @param deleteResourceDataSyncRequest
* @return Result of the DeleteResourceDataSync operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ResourceDataSyncNotFoundException
* The specified sync name wasn't found.
* @throws ResourceDataSyncInvalidConfigurationException
* The specified sync configuration is invalid.
* @sample AWSSimpleSystemsManagement.DeleteResourceDataSync
* @see AWS API
* Documentation
*/
DeleteResourceDataSyncResult deleteResourceDataSync(DeleteResourceDataSyncRequest deleteResourceDataSyncRequest);
/**
*
* Deletes a Systems Manager resource policy. A resource policy helps you to define the IAM entity (for example, an
* Amazon Web Services account) that can manage your Systems Manager resources. The following resources support
* Systems Manager resource policies.
*
*
* -
*
* OpsItemGroup
- The resource policy for OpsItemGroup
enables Amazon Web Services
* accounts to view and interact with OpsCenter operational work items (OpsItems).
*
*
* -
*
* Parameter
- The resource policy is used to share a parameter with other accounts using Resource
* Access Manager (RAM). For more information about cross-account sharing of parameters, see Working with shared parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ResourcePolicyInvalidParameterException
* One or more parameters specified for the call aren't valid. Verify the parameters and their values and
* try again.
* @throws ResourcePolicyConflictException
* The hash provided in the call doesn't match the stored hash. This exception is thrown when trying to
* update an obsolete policy version or when multiple requests to update a policy are sent.
* @throws ResourceNotFoundException
* The specified parameter to be shared could not be found.
* @throws MalformedResourcePolicyDocumentException
* The specified policy document is malformed or invalid, or excessive PutResourcePolicy
or
* DeleteResourcePolicy
calls have been made.
* @throws ResourcePolicyNotFoundException
* No policies with the specified policy ID and hash could be found.
* @sample AWSSimpleSystemsManagement.DeleteResourcePolicy
* @see AWS API
* Documentation
*/
DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Removes the server or virtual machine from the list of registered servers. You can reregister the node again at
* any time. If you don't plan to use Run Command on the server, we suggest uninstalling SSM Agent first.
*
*
* @param deregisterManagedInstanceRequest
* @return Result of the DeregisterManagedInstance operation returned by the service.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DeregisterManagedInstance
* @see AWS
* API Documentation
*/
DeregisterManagedInstanceResult deregisterManagedInstance(DeregisterManagedInstanceRequest deregisterManagedInstanceRequest);
/**
*
* Removes a patch group from a patch baseline.
*
*
* @param deregisterPatchBaselineForPatchGroupRequest
* @return Result of the DeregisterPatchBaselineForPatchGroup operation returned by the service.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DeregisterPatchBaselineForPatchGroup
* @see AWS API Documentation
*/
DeregisterPatchBaselineForPatchGroupResult deregisterPatchBaselineForPatchGroup(
DeregisterPatchBaselineForPatchGroupRequest deregisterPatchBaselineForPatchGroupRequest);
/**
*
* Removes a target from a maintenance window.
*
*
* @param deregisterTargetFromMaintenanceWindowRequest
* @return Result of the DeregisterTargetFromMaintenanceWindow operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws TargetInUseException
* You specified the Safe
option for the DeregisterTargetFromMaintenanceWindow operation, but
* the target is still referenced in a task.
* @sample AWSSimpleSystemsManagement.DeregisterTargetFromMaintenanceWindow
* @see AWS API Documentation
*/
DeregisterTargetFromMaintenanceWindowResult deregisterTargetFromMaintenanceWindow(
DeregisterTargetFromMaintenanceWindowRequest deregisterTargetFromMaintenanceWindowRequest);
/**
*
* Removes a task from a maintenance window.
*
*
* @param deregisterTaskFromMaintenanceWindowRequest
* @return Result of the DeregisterTaskFromMaintenanceWindow operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DeregisterTaskFromMaintenanceWindow
* @see AWS API Documentation
*/
DeregisterTaskFromMaintenanceWindowResult deregisterTaskFromMaintenanceWindow(
DeregisterTaskFromMaintenanceWindowRequest deregisterTaskFromMaintenanceWindowRequest);
/**
*
* Describes details about the activation, such as the date and time the activation was created, its expiration
* date, the Identity and Access Management (IAM) role assigned to the managed nodes in the activation, and the
* number of nodes registered by using this activation.
*
*
* @param describeActivationsRequest
* @return Result of the DescribeActivations operation returned by the service.
* @throws InvalidFilterException
* The filter name isn't valid. Verify the you entered the correct name and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeActivations
* @see AWS API
* Documentation
*/
DescribeActivationsResult describeActivations(DescribeActivationsRequest describeActivationsRequest);
/**
*
* Describes the association for the specified target or managed node. If you created the association by using the
* Targets
parameter, then you must retrieve the association by using the association ID.
*
*
* @param describeAssociationRequest
* @return Result of the DescribeAssociation operation returned by the service.
* @throws AssociationDoesNotExistException
* The specified association doesn't exist.
* @throws InvalidAssociationVersionException
* The version you specified isn't valid. Use ListAssociationVersions to view all versions of an association
* according to the association ID. Or, use the $LATEST
parameter to view the latest version of
* the association.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @sample AWSSimpleSystemsManagement.DescribeAssociation
* @see AWS API
* Documentation
*/
DescribeAssociationResult describeAssociation(DescribeAssociationRequest describeAssociationRequest);
/**
*
* Views information about a specific execution of a specific association.
*
*
* @param describeAssociationExecutionTargetsRequest
* @return Result of the DescribeAssociationExecutionTargets operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws AssociationDoesNotExistException
* The specified association doesn't exist.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws AssociationExecutionDoesNotExistException
* The specified execution ID doesn't exist. Verify the ID number and try again.
* @sample AWSSimpleSystemsManagement.DescribeAssociationExecutionTargets
* @see AWS API Documentation
*/
DescribeAssociationExecutionTargetsResult describeAssociationExecutionTargets(
DescribeAssociationExecutionTargetsRequest describeAssociationExecutionTargetsRequest);
/**
*
* Views all executions for a specific association ID.
*
*
* @param describeAssociationExecutionsRequest
* @return Result of the DescribeAssociationExecutions operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws AssociationDoesNotExistException
* The specified association doesn't exist.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeAssociationExecutions
* @see AWS API Documentation
*/
DescribeAssociationExecutionsResult describeAssociationExecutions(DescribeAssociationExecutionsRequest describeAssociationExecutionsRequest);
/**
*
* Provides details about all active and terminated Automation executions.
*
*
* @param describeAutomationExecutionsRequest
* @return Result of the DescribeAutomationExecutions operation returned by the service.
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @throws InvalidFilterValueException
* The filter value isn't valid. Verify the value and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeAutomationExecutions
* @see AWS API Documentation
*/
DescribeAutomationExecutionsResult describeAutomationExecutions(DescribeAutomationExecutionsRequest describeAutomationExecutionsRequest);
/**
*
* Information about all active and terminated step executions in an Automation workflow.
*
*
* @param describeAutomationStepExecutionsRequest
* @return Result of the DescribeAutomationStepExecutions operation returned by the service.
* @throws AutomationExecutionNotFoundException
* There is no automation execution information for the requested automation execution ID.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @throws InvalidFilterValueException
* The filter value isn't valid. Verify the value and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeAutomationStepExecutions
* @see AWS API Documentation
*/
DescribeAutomationStepExecutionsResult describeAutomationStepExecutions(DescribeAutomationStepExecutionsRequest describeAutomationStepExecutionsRequest);
/**
*
* Lists all patches eligible to be included in a patch baseline.
*
*
*
* Currently, DescribeAvailablePatches
supports only the Amazon Linux 1, Amazon Linux 2, and Windows
* Server operating systems.
*
*
*
* @param describeAvailablePatchesRequest
* @return Result of the DescribeAvailablePatches operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeAvailablePatches
* @see AWS
* API Documentation
*/
DescribeAvailablePatchesResult describeAvailablePatches(DescribeAvailablePatchesRequest describeAvailablePatchesRequest);
/**
*
* Describes the specified Amazon Web Services Systems Manager document (SSM document).
*
*
* @param describeDocumentRequest
* @return Result of the DescribeDocument operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @sample AWSSimpleSystemsManagement.DescribeDocument
* @see AWS API
* Documentation
*/
DescribeDocumentResult describeDocument(DescribeDocumentRequest describeDocumentRequest);
/**
*
* Describes the permissions for a Amazon Web Services Systems Manager document (SSM document). If you created the
* document, you are the owner. If a document is shared, it can either be shared privately (by specifying a user's
* Amazon Web Services account ID) or publicly (All).
*
*
* @param describeDocumentPermissionRequest
* @return Result of the DescribeDocumentPermission operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InvalidPermissionTypeException
* The permission type isn't supported. Share is the only supported permission type.
* @throws InvalidDocumentOperationException
* You attempted to delete a document while it is still shared. You must stop sharing the document before
* you can delete it.
* @sample AWSSimpleSystemsManagement.DescribeDocumentPermission
* @see AWS
* API Documentation
*/
DescribeDocumentPermissionResult describeDocumentPermission(DescribeDocumentPermissionRequest describeDocumentPermissionRequest);
/**
*
* All associations for the managed nodes.
*
*
* @param describeEffectiveInstanceAssociationsRequest
* @return Result of the DescribeEffectiveInstanceAssociations operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeEffectiveInstanceAssociations
* @see AWS API Documentation
*/
DescribeEffectiveInstanceAssociationsResult describeEffectiveInstanceAssociations(
DescribeEffectiveInstanceAssociationsRequest describeEffectiveInstanceAssociationsRequest);
/**
*
* Retrieves the current effective patches (the patch and the approval state) for the specified patch baseline.
* Applies to patch baselines for Windows only.
*
*
* @param describeEffectivePatchesForPatchBaselineRequest
* @return Result of the DescribeEffectivePatchesForPatchBaseline operation returned by the service.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws UnsupportedOperatingSystemException
* The operating systems you specified isn't supported, or the operation isn't supported for the operating
* system.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeEffectivePatchesForPatchBaseline
* @see AWS API Documentation
*/
DescribeEffectivePatchesForPatchBaselineResult describeEffectivePatchesForPatchBaseline(
DescribeEffectivePatchesForPatchBaselineRequest describeEffectivePatchesForPatchBaselineRequest);
/**
*
* The status of the associations for the managed nodes.
*
*
* @param describeInstanceAssociationsStatusRequest
* @return Result of the DescribeInstanceAssociationsStatus operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeInstanceAssociationsStatus
* @see AWS API Documentation
*/
DescribeInstanceAssociationsStatusResult describeInstanceAssociationsStatus(
DescribeInstanceAssociationsStatusRequest describeInstanceAssociationsStatusRequest);
/**
*
* Provides information about one or more of your managed nodes, including the operating system platform, SSM Agent
* version, association status, and IP address. This operation does not return information for nodes that are either
* Stopped or Terminated.
*
*
* If you specify one or more node IDs, the operation returns information for those managed nodes. If you don't
* specify node IDs, it returns information for all your managed nodes. If you specify a node ID that isn't valid or
* a node that you don't own, you receive an error.
*
*
*
* The IamRole
field returned for this API operation is the Identity and Access Management (IAM) role
* assigned to on-premises managed nodes. This operation does not return the IAM role for EC2 instances.
*
*
*
* @param describeInstanceInformationRequest
* @return Result of the DescribeInstanceInformation operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InvalidInstanceInformationFilterValueException
* The specified filter value isn't valid.
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeInstanceInformation
* @see AWS API Documentation
*/
DescribeInstanceInformationResult describeInstanceInformation(DescribeInstanceInformationRequest describeInstanceInformationRequest);
/**
*
* Retrieves the high-level patch state of one or more managed nodes.
*
*
* @param describeInstancePatchStatesRequest
* @return Result of the DescribeInstancePatchStates operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeInstancePatchStates
* @see AWS API Documentation
*/
DescribeInstancePatchStatesResult describeInstancePatchStates(DescribeInstancePatchStatesRequest describeInstancePatchStatesRequest);
/**
*
* Retrieves the high-level patch state for the managed nodes in the specified patch group.
*
*
* @param describeInstancePatchStatesForPatchGroupRequest
* @return Result of the DescribeInstancePatchStatesForPatchGroup operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidFilterException
* The filter name isn't valid. Verify the you entered the correct name and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeInstancePatchStatesForPatchGroup
* @see AWS API Documentation
*/
DescribeInstancePatchStatesForPatchGroupResult describeInstancePatchStatesForPatchGroup(
DescribeInstancePatchStatesForPatchGroupRequest describeInstancePatchStatesForPatchGroupRequest);
/**
*
* Retrieves information about the patches on the specified managed node and their state relative to the patch
* baseline being used for the node.
*
*
* @param describeInstancePatchesRequest
* @return Result of the DescribeInstancePatches operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidFilterException
* The filter name isn't valid. Verify the you entered the correct name and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeInstancePatches
* @see AWS
* API Documentation
*/
DescribeInstancePatchesResult describeInstancePatches(DescribeInstancePatchesRequest describeInstancePatchesRequest);
/**
*
* An API operation used by the Systems Manager console to display information about Systems Manager managed nodes.
*
*
* @param describeInstancePropertiesRequest
* @return Result of the DescribeInstanceProperties operation returned by the service.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidActivationIdException
* The activation ID isn't valid. Verify the you entered the correct ActivationId or ActivationCode and try
* again.
* @throws InvalidInstancePropertyFilterValueException
* The specified filter value isn't valid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @sample AWSSimpleSystemsManagement.DescribeInstanceProperties
* @see AWS
* API Documentation
*/
DescribeInstancePropertiesResult describeInstanceProperties(DescribeInstancePropertiesRequest describeInstancePropertiesRequest);
/**
*
* Describes a specific delete inventory operation.
*
*
* @param describeInventoryDeletionsRequest
* @return Result of the DescribeInventoryDeletions operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDeletionIdException
* The ID specified for the delete operation doesn't exist or isn't valid. Verify the ID and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeInventoryDeletions
* @see AWS
* API Documentation
*/
DescribeInventoryDeletionsResult describeInventoryDeletions(DescribeInventoryDeletionsRequest describeInventoryDeletionsRequest);
/**
*
* Retrieves the individual task executions (one per target) for a particular task run as part of a maintenance
* window execution.
*
*
* @param describeMaintenanceWindowExecutionTaskInvocationsRequest
* @return Result of the DescribeMaintenanceWindowExecutionTaskInvocations operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeMaintenanceWindowExecutionTaskInvocations
* @see AWS API Documentation
*/
DescribeMaintenanceWindowExecutionTaskInvocationsResult describeMaintenanceWindowExecutionTaskInvocations(
DescribeMaintenanceWindowExecutionTaskInvocationsRequest describeMaintenanceWindowExecutionTaskInvocationsRequest);
/**
*
* For a given maintenance window execution, lists the tasks that were run.
*
*
* @param describeMaintenanceWindowExecutionTasksRequest
* @return Result of the DescribeMaintenanceWindowExecutionTasks operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeMaintenanceWindowExecutionTasks
* @see AWS API Documentation
*/
DescribeMaintenanceWindowExecutionTasksResult describeMaintenanceWindowExecutionTasks(
DescribeMaintenanceWindowExecutionTasksRequest describeMaintenanceWindowExecutionTasksRequest);
/**
*
* Lists the executions of a maintenance window. This includes information about when the maintenance window was
* scheduled to be active, and information about tasks registered and run with the maintenance window.
*
*
* @param describeMaintenanceWindowExecutionsRequest
* @return Result of the DescribeMaintenanceWindowExecutions operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeMaintenanceWindowExecutions
* @see AWS API Documentation
*/
DescribeMaintenanceWindowExecutionsResult describeMaintenanceWindowExecutions(
DescribeMaintenanceWindowExecutionsRequest describeMaintenanceWindowExecutionsRequest);
/**
*
* Retrieves information about upcoming executions of a maintenance window.
*
*
* @param describeMaintenanceWindowScheduleRequest
* @return Result of the DescribeMaintenanceWindowSchedule operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @sample AWSSimpleSystemsManagement.DescribeMaintenanceWindowSchedule
* @see AWS API Documentation
*/
DescribeMaintenanceWindowScheduleResult describeMaintenanceWindowSchedule(DescribeMaintenanceWindowScheduleRequest describeMaintenanceWindowScheduleRequest);
/**
*
* Lists the targets registered with the maintenance window.
*
*
* @param describeMaintenanceWindowTargetsRequest
* @return Result of the DescribeMaintenanceWindowTargets operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeMaintenanceWindowTargets
* @see AWS API Documentation
*/
DescribeMaintenanceWindowTargetsResult describeMaintenanceWindowTargets(DescribeMaintenanceWindowTargetsRequest describeMaintenanceWindowTargetsRequest);
/**
*
* Lists the tasks in a maintenance window.
*
*
*
* For maintenance window tasks without a specified target, you can't supply values for --max-errors
* and --max-concurrency
. Instead, the system inserts a placeholder value of 1
, which may
* be reported in the response to this command. These values don't affect the running of your task and can be
* ignored.
*
*
*
* @param describeMaintenanceWindowTasksRequest
* @return Result of the DescribeMaintenanceWindowTasks operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeMaintenanceWindowTasks
* @see AWS API Documentation
*/
DescribeMaintenanceWindowTasksResult describeMaintenanceWindowTasks(DescribeMaintenanceWindowTasksRequest describeMaintenanceWindowTasksRequest);
/**
*
* Retrieves the maintenance windows in an Amazon Web Services account.
*
*
* @param describeMaintenanceWindowsRequest
* @return Result of the DescribeMaintenanceWindows operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeMaintenanceWindows
* @see AWS
* API Documentation
*/
DescribeMaintenanceWindowsResult describeMaintenanceWindows(DescribeMaintenanceWindowsRequest describeMaintenanceWindowsRequest);
/**
*
* Retrieves information about the maintenance window targets or tasks that a managed node is associated with.
*
*
* @param describeMaintenanceWindowsForTargetRequest
* @return Result of the DescribeMaintenanceWindowsForTarget operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeMaintenanceWindowsForTarget
* @see AWS API Documentation
*/
DescribeMaintenanceWindowsForTargetResult describeMaintenanceWindowsForTarget(
DescribeMaintenanceWindowsForTargetRequest describeMaintenanceWindowsForTargetRequest);
/**
*
* Query a set of OpsItems. You must have permission in Identity and Access Management (IAM) to query a list of
* OpsItems. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param describeOpsItemsRequest
* @return Result of the DescribeOpsItems operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribeOpsItems
* @see AWS API
* Documentation
*/
DescribeOpsItemsResult describeOpsItems(DescribeOpsItemsRequest describeOpsItemsRequest);
/**
*
* Lists the parameters in your Amazon Web Services account or the parameters shared with you when you enable the Shared option.
*
*
* Request results are returned on a best-effort basis. If you specify MaxResults
in the request, the
* response includes information up to the limit specified. The number of items returned, however, can be between
* zero and the value of MaxResults
. If the service reaches an internal limit while processing the
* results, it stops the operation and returns the matching values up to that point and a NextToken
.
* You can specify the NextToken
in a subsequent call to get the next set of results.
*
*
*
* If you change the KMS key alias for the KMS key used to encrypt a parameter, then you must also update the key
* alias the parameter uses to reference KMS. Otherwise, DescribeParameters
retrieves whatever the
* original key alias was referencing.
*
*
*
* @param describeParametersRequest
* @return Result of the DescribeParameters operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @throws InvalidFilterOptionException
* The specified filter option isn't valid. Valid options are Equals and BeginsWith. For Path filter, valid
* options are Recursive and OneLevel.
* @throws InvalidFilterValueException
* The filter value isn't valid. Verify the value and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeParameters
* @see AWS API
* Documentation
*/
DescribeParametersResult describeParameters(DescribeParametersRequest describeParametersRequest);
/**
*
* Lists the patch baselines in your Amazon Web Services account.
*
*
* @param describePatchBaselinesRequest
* @return Result of the DescribePatchBaselines operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribePatchBaselines
* @see AWS API
* Documentation
*/
DescribePatchBaselinesResult describePatchBaselines(DescribePatchBaselinesRequest describePatchBaselinesRequest);
/**
*
* Returns high-level aggregated patch compliance state information for a patch group.
*
*
* @param describePatchGroupStateRequest
* @return Result of the DescribePatchGroupState operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribePatchGroupState
* @see AWS
* API Documentation
*/
DescribePatchGroupStateResult describePatchGroupState(DescribePatchGroupStateRequest describePatchGroupStateRequest);
/**
*
* Lists all patch groups that have been registered with patch baselines.
*
*
* @param describePatchGroupsRequest
* @return Result of the DescribePatchGroups operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribePatchGroups
* @see AWS API
* Documentation
*/
DescribePatchGroupsResult describePatchGroups(DescribePatchGroupsRequest describePatchGroupsRequest);
/**
*
* Lists the properties of available patches organized by product, product family, classification, severity, and
* other properties of available patches. You can use the reported properties in the filters you specify in requests
* for operations such as CreatePatchBaseline, UpdatePatchBaseline, DescribeAvailablePatches,
* and DescribePatchBaselines.
*
*
* The following section lists the properties that can be used in filters for each major operating system type:
*
*
* - AMAZON_LINUX
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - AMAZON_LINUX_2
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - CENTOS
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - DEBIAN
* -
*
* Valid properties: PRODUCT
| PRIORITY
*
*
* - MACOS
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
*
*
* - ORACLE_LINUX
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - REDHAT_ENTERPRISE_LINUX
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - SUSE
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - UBUNTU
* -
*
* Valid properties: PRODUCT
| PRIORITY
*
*
* - WINDOWS
* -
*
* Valid properties: PRODUCT
| PRODUCT_FAMILY
| CLASSIFICATION
|
* MSRC_SEVERITY
*
*
*
*
* @param describePatchPropertiesRequest
* @return Result of the DescribePatchProperties operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.DescribePatchProperties
* @see AWS
* API Documentation
*/
DescribePatchPropertiesResult describePatchProperties(DescribePatchPropertiesRequest describePatchPropertiesRequest);
/**
*
* Retrieves a list of all active sessions (both connected and disconnected) or terminated sessions from the past 30
* days.
*
*
* @param describeSessionsRequest
* @return Result of the DescribeSessions operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.DescribeSessions
* @see AWS API
* Documentation
*/
DescribeSessionsResult describeSessions(DescribeSessionsRequest describeSessionsRequest);
/**
*
* Deletes the association between an OpsItem and a related item. For example, this API operation can delete an
* Incident Manager incident from an OpsItem. Incident Manager is a capability of Amazon Web Services Systems
* Manager.
*
*
* @param disassociateOpsItemRelatedItemRequest
* @return Result of the DisassociateOpsItemRelatedItem operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws OpsItemRelatedItemAssociationNotFoundException
* The association wasn't found using the parameters you specified in the call. Verify the information and
* try again.
* @throws OpsItemNotFoundException
* The specified OpsItem ID doesn't exist. Verify the ID and try again.
* @throws OpsItemInvalidParameterException
* A specified parameter argument isn't valid. Verify the available arguments and try again.
* @throws OpsItemConflictException
* The specified OpsItem is in the process of being deleted.
* @sample AWSSimpleSystemsManagement.DisassociateOpsItemRelatedItem
* @see AWS API Documentation
*/
DisassociateOpsItemRelatedItemResult disassociateOpsItemRelatedItem(DisassociateOpsItemRelatedItemRequest disassociateOpsItemRelatedItemRequest);
/**
*
* Get detailed information about a particular Automation execution.
*
*
* @param getAutomationExecutionRequest
* @return Result of the GetAutomationExecution operation returned by the service.
* @throws AutomationExecutionNotFoundException
* There is no automation execution information for the requested automation execution ID.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetAutomationExecution
* @see AWS API
* Documentation
*/
GetAutomationExecutionResult getAutomationExecution(GetAutomationExecutionRequest getAutomationExecutionRequest);
/**
*
* Gets the state of a Amazon Web Services Systems Manager change calendar at the current time or a specified time.
* If you specify a time, GetCalendarState
returns the state of the calendar at that specific time, and
* returns the next time that the change calendar state will transition. If you don't specify a time,
* GetCalendarState
uses the current time. Change Calendar entries have two possible states:
* OPEN
or CLOSED
.
*
*
* If you specify more than one calendar in a request, the command returns the status of OPEN
only if
* all calendars in the request are open. If one or more calendars in the request are closed, the status returned is
* CLOSED
.
*
*
* For more information about Change Calendar, a capability of Amazon Web Services Systems Manager, see Amazon
* Web Services Systems Manager Change Calendar in the Amazon Web Services Systems Manager User Guide.
*
*
* @param getCalendarStateRequest
* @return Result of the GetCalendarState operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentTypeException
* The SSM document type isn't valid. Valid document types are described in the DocumentType
* property.
* @throws UnsupportedCalendarException
* The calendar entry contained in the specified SSM document isn't supported.
* @sample AWSSimpleSystemsManagement.GetCalendarState
* @see AWS API
* Documentation
*/
GetCalendarStateResult getCalendarState(GetCalendarStateRequest getCalendarStateRequest);
/**
*
* Returns detailed information about command execution for an invocation or plugin.
*
*
* GetCommandInvocation
only gives the execution status of a plugin in a document. To get the command
* execution status on a specific managed node, use ListCommandInvocations. To get the command execution
* status across managed nodes, use ListCommands.
*
*
* @param getCommandInvocationRequest
* @return Result of the GetCommandInvocation operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidCommandIdException
* The specified command ID isn't valid. Verify the ID and try again.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidPluginNameException
* The plugin name isn't valid.
* @throws InvocationDoesNotExistException
* The command ID and managed node ID you specified didn't match any invocations. Verify the command ID and
* the managed node ID and try again.
* @sample AWSSimpleSystemsManagement.GetCommandInvocation
* @see AWS API
* Documentation
*/
GetCommandInvocationResult getCommandInvocation(GetCommandInvocationRequest getCommandInvocationRequest);
/**
*
* Retrieves the Session Manager connection status for a managed node to determine whether it is running and ready
* to receive Session Manager connections.
*
*
* @param getConnectionStatusRequest
* @return Result of the GetConnectionStatus operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetConnectionStatus
* @see AWS API
* Documentation
*/
GetConnectionStatusResult getConnectionStatus(GetConnectionStatusRequest getConnectionStatusRequest);
/**
*
* Retrieves the default patch baseline. Amazon Web Services Systems Manager supports creating multiple default
* patch baselines. For example, you can create a default patch baseline for each operating system.
*
*
* If you don't specify an operating system value, the default patch baseline for Windows is returned.
*
*
* @param getDefaultPatchBaselineRequest
* @return Result of the GetDefaultPatchBaseline operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetDefaultPatchBaseline
* @see AWS
* API Documentation
*/
GetDefaultPatchBaselineResult getDefaultPatchBaseline(GetDefaultPatchBaselineRequest getDefaultPatchBaselineRequest);
/**
*
* Retrieves the current snapshot for the patch baseline the managed node uses. This API is primarily used by the
* AWS-RunPatchBaseline
Systems Manager document (SSM document).
*
*
*
* If you run the command locally, such as with the Command Line Interface (CLI), the system attempts to use your
* local Amazon Web Services credentials and the operation fails. To avoid this, you can run the command in the
* Amazon Web Services Systems Manager console. Use Run Command, a capability of Amazon Web Services Systems
* Manager, with an SSM document that enables you to target a managed node with a script or command. For example,
* run the command using the AWS-RunShellScript
document or the AWS-RunPowerShellScript
* document.
*
*
*
* @param getDeployablePatchSnapshotForInstanceRequest
* @return Result of the GetDeployablePatchSnapshotForInstance operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws UnsupportedOperatingSystemException
* The operating systems you specified isn't supported, or the operation isn't supported for the operating
* system.
* @throws UnsupportedFeatureRequiredException
* Patching for applications released by Microsoft is only available on EC2 instances and advanced
* instances. To patch applications released by Microsoft on on-premises servers and VMs, you must enable
* advanced instances. For more information, see Turning on the advanced-instances tier in the Amazon Web Services Systems Manager User Guide.
* @sample AWSSimpleSystemsManagement.GetDeployablePatchSnapshotForInstance
* @see AWS API Documentation
*/
GetDeployablePatchSnapshotForInstanceResult getDeployablePatchSnapshotForInstance(
GetDeployablePatchSnapshotForInstanceRequest getDeployablePatchSnapshotForInstanceRequest);
/**
*
* Gets the contents of the specified Amazon Web Services Systems Manager document (SSM document).
*
*
* @param getDocumentRequest
* @return Result of the GetDocument operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @sample AWSSimpleSystemsManagement.GetDocument
* @see AWS API
* Documentation
*/
GetDocumentResult getDocument(GetDocumentRequest getDocumentRequest);
/**
*
* Query inventory information. This includes managed node status, such as Stopped
or
* Terminated
.
*
*
* @param getInventoryRequest
* @return Result of the GetInventory operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidFilterException
* The filter name isn't valid. Verify the you entered the correct name and try again.
* @throws InvalidInventoryGroupException
* The specified inventory group isn't valid.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InvalidTypeNameException
* The parameter type name isn't valid.
* @throws InvalidAggregatorException
* The specified aggregator isn't valid for inventory groups. Verify that the aggregator uses a valid
* inventory type such as AWS:Application
or AWS:InstanceInformation
.
* @throws InvalidResultAttributeException
* The specified inventory item result attribute isn't valid.
* @sample AWSSimpleSystemsManagement.GetInventory
* @see AWS API
* Documentation
*/
GetInventoryResult getInventory(GetInventoryRequest getInventoryRequest);
/**
*
* Return a list of inventory type names for the account, or return a list of attribute names for a specific
* Inventory item type.
*
*
* @param getInventorySchemaRequest
* @return Result of the GetInventorySchema operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidTypeNameException
* The parameter type name isn't valid.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.GetInventorySchema
* @see AWS API
* Documentation
*/
GetInventorySchemaResult getInventorySchema(GetInventorySchemaRequest getInventorySchemaRequest);
/**
*
* Retrieves a maintenance window.
*
*
* @param getMaintenanceWindowRequest
* @return Result of the GetMaintenanceWindow operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetMaintenanceWindow
* @see AWS API
* Documentation
*/
GetMaintenanceWindowResult getMaintenanceWindow(GetMaintenanceWindowRequest getMaintenanceWindowRequest);
/**
*
* Retrieves details about a specific a maintenance window execution.
*
*
* @param getMaintenanceWindowExecutionRequest
* @return Result of the GetMaintenanceWindowExecution operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetMaintenanceWindowExecution
* @see AWS API Documentation
*/
GetMaintenanceWindowExecutionResult getMaintenanceWindowExecution(GetMaintenanceWindowExecutionRequest getMaintenanceWindowExecutionRequest);
/**
*
* Retrieves the details about a specific task run as part of a maintenance window execution.
*
*
* @param getMaintenanceWindowExecutionTaskRequest
* @return Result of the GetMaintenanceWindowExecutionTask operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetMaintenanceWindowExecutionTask
* @see AWS API Documentation
*/
GetMaintenanceWindowExecutionTaskResult getMaintenanceWindowExecutionTask(GetMaintenanceWindowExecutionTaskRequest getMaintenanceWindowExecutionTaskRequest);
/**
*
* Retrieves information about a specific task running on a specific target.
*
*
* @param getMaintenanceWindowExecutionTaskInvocationRequest
* @return Result of the GetMaintenanceWindowExecutionTaskInvocation operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetMaintenanceWindowExecutionTaskInvocation
* @see AWS API Documentation
*/
GetMaintenanceWindowExecutionTaskInvocationResult getMaintenanceWindowExecutionTaskInvocation(
GetMaintenanceWindowExecutionTaskInvocationRequest getMaintenanceWindowExecutionTaskInvocationRequest);
/**
*
* Retrieves the details of a maintenance window task.
*
*
*
* For maintenance window tasks without a specified target, you can't supply values for --max-errors
* and --max-concurrency
. Instead, the system inserts a placeholder value of 1
, which may
* be reported in the response to this command. These values don't affect the running of your task and can be
* ignored.
*
*
*
* To retrieve a list of tasks in a maintenance window, instead use the DescribeMaintenanceWindowTasks
* command.
*
*
* @param getMaintenanceWindowTaskRequest
* @return Result of the GetMaintenanceWindowTask operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetMaintenanceWindowTask
* @see AWS
* API Documentation
*/
GetMaintenanceWindowTaskResult getMaintenanceWindowTask(GetMaintenanceWindowTaskRequest getMaintenanceWindowTaskRequest);
/**
*
* Get information about an OpsItem by using the ID. You must have permission in Identity and Access Management
* (IAM) to view information about an OpsItem. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param getOpsItemRequest
* @return Result of the GetOpsItem operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws OpsItemNotFoundException
* The specified OpsItem ID doesn't exist. Verify the ID and try again.
* @throws OpsItemAccessDeniedException
* You don't have permission to view OpsItems in the specified account. Verify that your account is
* configured either as a Systems Manager delegated administrator or that you are logged into the
* Organizations management account.
* @sample AWSSimpleSystemsManagement.GetOpsItem
* @see AWS API
* Documentation
*/
GetOpsItemResult getOpsItem(GetOpsItemRequest getOpsItemRequest);
/**
*
* View operational metadata related to an application in Application Manager.
*
*
* @param getOpsMetadataRequest
* @return Result of the GetOpsMetadata operation returned by the service.
* @throws OpsMetadataNotFoundException
* The OpsMetadata object doesn't exist.
* @throws OpsMetadataInvalidArgumentException
* One of the arguments passed is invalid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetOpsMetadata
* @see AWS API
* Documentation
*/
GetOpsMetadataResult getOpsMetadata(GetOpsMetadataRequest getOpsMetadataRequest);
/**
*
* View a summary of operations metadata (OpsData) based on specified filters and aggregators. OpsData can include
* information about Amazon Web Services Systems Manager OpsCenter operational workitems (OpsItems) as well as
* information about any Amazon Web Services resource or service configured to report OpsData to Amazon Web Services
* Systems Manager Explorer.
*
*
* @param getOpsSummaryRequest
* @return Result of the GetOpsSummary operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ResourceDataSyncNotFoundException
* The specified sync name wasn't found.
* @throws InvalidFilterException
* The filter name isn't valid. Verify the you entered the correct name and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InvalidTypeNameException
* The parameter type name isn't valid.
* @throws InvalidAggregatorException
* The specified aggregator isn't valid for inventory groups. Verify that the aggregator uses a valid
* inventory type such as AWS:Application
or AWS:InstanceInformation
.
* @sample AWSSimpleSystemsManagement.GetOpsSummary
* @see AWS API
* Documentation
*/
GetOpsSummaryResult getOpsSummary(GetOpsSummaryRequest getOpsSummaryRequest);
/**
*
* Get information about a single parameter by specifying the parameter name.
*
*
*
* To get information about more than one parameter at a time, use the GetParameters operation.
*
*
*
* @param getParameterRequest
* @return Result of the GetParameter operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidKeyIdException
* The query key ID isn't valid.
* @throws ParameterNotFoundException
* The parameter couldn't be found. Verify the name and try again.
* @throws ParameterVersionNotFoundException
* The specified parameter version wasn't found. Verify the parameter name and version, and try again.
* @sample AWSSimpleSystemsManagement.GetParameter
* @see AWS API
* Documentation
*/
GetParameterResult getParameter(GetParameterRequest getParameterRequest);
/**
*
* Retrieves the history of all changes to a parameter.
*
*
*
* If you change the KMS key alias for the KMS key used to encrypt a parameter, then you must also update the key
* alias the parameter uses to reference KMS. Otherwise, GetParameterHistory
retrieves whatever the
* original key alias was referencing.
*
*
*
* @param getParameterHistoryRequest
* @return Result of the GetParameterHistory operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ParameterNotFoundException
* The parameter couldn't be found. Verify the name and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InvalidKeyIdException
* The query key ID isn't valid.
* @sample AWSSimpleSystemsManagement.GetParameterHistory
* @see AWS API
* Documentation
*/
GetParameterHistoryResult getParameterHistory(GetParameterHistoryRequest getParameterHistoryRequest);
/**
*
* Get information about one or more parameters by specifying multiple parameter names.
*
*
*
* To get information about a single parameter, you can use the GetParameter operation instead.
*
*
*
* @param getParametersRequest
* @return Result of the GetParameters operation returned by the service.
* @throws InvalidKeyIdException
* The query key ID isn't valid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetParameters
* @see AWS API
* Documentation
*/
GetParametersResult getParameters(GetParametersRequest getParametersRequest);
/**
*
* Retrieve information about one or more parameters in a specific hierarchy.
*
*
* Request results are returned on a best-effort basis. If you specify MaxResults
in the request, the
* response includes information up to the limit specified. The number of items returned, however, can be between
* zero and the value of MaxResults
. If the service reaches an internal limit while processing the
* results, it stops the operation and returns the matching values up to that point and a NextToken
.
* You can specify the NextToken
in a subsequent call to get the next set of results.
*
*
* @param getParametersByPathRequest
* @return Result of the GetParametersByPath operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @throws InvalidFilterOptionException
* The specified filter option isn't valid. Valid options are Equals and BeginsWith. For Path filter, valid
* options are Recursive and OneLevel.
* @throws InvalidFilterValueException
* The filter value isn't valid. Verify the value and try again.
* @throws InvalidKeyIdException
* The query key ID isn't valid.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.GetParametersByPath
* @see AWS API
* Documentation
*/
GetParametersByPathResult getParametersByPath(GetParametersByPathRequest getParametersByPathRequest);
/**
*
* Retrieves information about a patch baseline.
*
*
* @param getPatchBaselineRequest
* @return Result of the GetPatchBaseline operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetPatchBaseline
* @see AWS API
* Documentation
*/
GetPatchBaselineResult getPatchBaseline(GetPatchBaselineRequest getPatchBaselineRequest);
/**
*
* Retrieves the patch baseline that should be used for the specified patch group.
*
*
* @param getPatchBaselineForPatchGroupRequest
* @return Result of the GetPatchBaselineForPatchGroup operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.GetPatchBaselineForPatchGroup
* @see AWS API Documentation
*/
GetPatchBaselineForPatchGroupResult getPatchBaselineForPatchGroup(GetPatchBaselineForPatchGroupRequest getPatchBaselineForPatchGroupRequest);
/**
*
* Returns an array of the Policy
object.
*
*
* @param getResourcePoliciesRequest
* @return Result of the GetResourcePolicies operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ResourcePolicyInvalidParameterException
* One or more parameters specified for the call aren't valid. Verify the parameters and their values and
* try again.
* @throws ResourceNotFoundException
* The specified parameter to be shared could not be found.
* @sample AWSSimpleSystemsManagement.GetResourcePolicies
* @see AWS API
* Documentation
*/
GetResourcePoliciesResult getResourcePolicies(GetResourcePoliciesRequest getResourcePoliciesRequest);
/**
*
* ServiceSetting
is an account-level setting for an Amazon Web Services service. This setting defines
* how a user interacts with or uses a service or a feature of a service. For example, if an Amazon Web Services
* service charges money to the account based on feature or service usage, then the Amazon Web Services service team
* might create a default setting of false
. This means the user can't use this feature unless they
* change the setting to true
and intentionally opt in for a paid feature.
*
*
* Services map a SettingId
object to a setting value. Amazon Web Services services teams define the
* default value for a SettingId
. You can't create a new SettingId
, but you can overwrite
* the default value if you have the ssm:UpdateServiceSetting
permission for the setting. Use the
* UpdateServiceSetting API operation to change the default setting. Or use the ResetServiceSetting to
* change the value back to the original value defined by the Amazon Web Services service team.
*
*
* Query the current service setting for the Amazon Web Services account.
*
*
* @param getServiceSettingRequest
* The request body of the GetServiceSetting API operation.
* @return Result of the GetServiceSetting operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ServiceSettingNotFoundException
* The specified service setting wasn't found. Either the service name or the setting hasn't been
* provisioned by the Amazon Web Services service team.
* @sample AWSSimpleSystemsManagement.GetServiceSetting
* @see AWS API
* Documentation
*/
GetServiceSettingResult getServiceSetting(GetServiceSettingRequest getServiceSettingRequest);
/**
*
* A parameter label is a user-defined alias to help you manage different versions of a parameter. When you modify a
* parameter, Amazon Web Services Systems Manager automatically saves a new version and increments the version
* number by one. A label can help you remember the purpose of a parameter when there are multiple versions.
*
*
* Parameter labels have the following requirements and restrictions.
*
*
* -
*
* A version of a parameter can have a maximum of 10 labels.
*
*
* -
*
* You can't attach the same label to different versions of the same parameter. For example, if version 1 has the
* label Production, then you can't attach Production to version 2.
*
*
* -
*
* You can move a label from one version of a parameter to another.
*
*
* -
*
* You can't create a label when you create a new parameter. You must attach a label to a specific version of a
* parameter.
*
*
* -
*
* If you no longer want to use a parameter label, then you can either delete it or move it to a different version
* of a parameter.
*
*
* -
*
* A label can have a maximum of 100 characters.
*
*
* -
*
* Labels can contain letters (case sensitive), numbers, periods (.), hyphens (-), or underscores (_).
*
*
* -
*
* Labels can't begin with a number, "aws
" or "ssm
" (not case sensitive). If a label fails
* to meet these requirements, then the label isn't associated with a parameter and the system displays it in the
* list of InvalidLabels.
*
*
*
*
* @param labelParameterVersionRequest
* @return Result of the LabelParameterVersion operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @throws ParameterNotFoundException
* The parameter couldn't be found. Verify the name and try again.
* @throws ParameterVersionNotFoundException
* The specified parameter version wasn't found. Verify the parameter name and version, and try again.
* @throws ParameterVersionLabelLimitExceededException
* A parameter version can have a maximum of ten labels.
* @sample AWSSimpleSystemsManagement.LabelParameterVersion
* @see AWS API
* Documentation
*/
LabelParameterVersionResult labelParameterVersion(LabelParameterVersionRequest labelParameterVersionRequest);
/**
*
* Retrieves all versions of an association for a specific association ID.
*
*
* @param listAssociationVersionsRequest
* @return Result of the ListAssociationVersions operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws AssociationDoesNotExistException
* The specified association doesn't exist.
* @sample AWSSimpleSystemsManagement.ListAssociationVersions
* @see AWS
* API Documentation
*/
ListAssociationVersionsResult listAssociationVersions(ListAssociationVersionsRequest listAssociationVersionsRequest);
/**
*
* Returns all State Manager associations in the current Amazon Web Services account and Amazon Web Services Region.
* You can limit the results to a specific State Manager association document or managed node by specifying a
* filter. State Manager is a capability of Amazon Web Services Systems Manager.
*
*
* @param listAssociationsRequest
* @return Result of the ListAssociations operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.ListAssociations
* @see AWS API
* Documentation
*/
ListAssociationsResult listAssociations(ListAssociationsRequest listAssociationsRequest);
/**
*
* An invocation is copy of a command sent to a specific managed node. A command can apply to one or more managed
* nodes. A command invocation applies to one managed node. For example, if a user runs SendCommand
* against three managed nodes, then a command invocation is created for each requested managed node ID.
* ListCommandInvocations
provide status about command execution.
*
*
* @param listCommandInvocationsRequest
* @return Result of the ListCommandInvocations operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidCommandIdException
* The specified command ID isn't valid. Verify the ID and try again.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.ListCommandInvocations
* @see AWS API
* Documentation
*/
ListCommandInvocationsResult listCommandInvocations(ListCommandInvocationsRequest listCommandInvocationsRequest);
/**
*
* Lists the commands requested by users of the Amazon Web Services account.
*
*
* @param listCommandsRequest
* @return Result of the ListCommands operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidCommandIdException
* The specified command ID isn't valid. Verify the ID and try again.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.ListCommands
* @see AWS API
* Documentation
*/
ListCommandsResult listCommands(ListCommandsRequest listCommandsRequest);
/**
*
* For a specified resource ID, this API operation returns a list of compliance statuses for different resource
* types. Currently, you can only specify one resource ID per call. List results depend on the criteria specified in
* the filter.
*
*
* @param listComplianceItemsRequest
* @return Result of the ListComplianceItems operation returned by the service.
* @throws InvalidResourceTypeException
* The resource type isn't valid. For example, if you are attempting to tag an EC2 instance, the instance
* must be a registered managed node.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidFilterException
* The filter name isn't valid. Verify the you entered the correct name and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.ListComplianceItems
* @see AWS API
* Documentation
*/
ListComplianceItemsResult listComplianceItems(ListComplianceItemsRequest listComplianceItemsRequest);
/**
*
* Returns a summary count of compliant and non-compliant resources for a compliance type. For example, this call
* can return State Manager associations, patches, or custom compliance types according to the filter criteria that
* you specify.
*
*
* @param listComplianceSummariesRequest
* @return Result of the ListComplianceSummaries operation returned by the service.
* @throws InvalidFilterException
* The filter name isn't valid. Verify the you entered the correct name and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.ListComplianceSummaries
* @see AWS
* API Documentation
*/
ListComplianceSummariesResult listComplianceSummaries(ListComplianceSummariesRequest listComplianceSummariesRequest);
/**
*
* Information about approval reviews for a version of a change template in Change Manager.
*
*
* @param listDocumentMetadataHistoryRequest
* @return Result of the ListDocumentMetadataHistory operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.ListDocumentMetadataHistory
* @see AWS API Documentation
*/
ListDocumentMetadataHistoryResult listDocumentMetadataHistory(ListDocumentMetadataHistoryRequest listDocumentMetadataHistoryRequest);
/**
*
* List all versions for a document.
*
*
* @param listDocumentVersionsRequest
* @return Result of the ListDocumentVersions operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @sample AWSSimpleSystemsManagement.ListDocumentVersions
* @see AWS API
* Documentation
*/
ListDocumentVersionsResult listDocumentVersions(ListDocumentVersionsRequest listDocumentVersionsRequest);
/**
*
* Returns all Systems Manager (SSM) documents in the current Amazon Web Services account and Amazon Web Services
* Region. You can limit the results of this request by using a filter.
*
*
* @param listDocumentsRequest
* @return Result of the ListDocuments operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InvalidFilterKeyException
* The specified key isn't valid.
* @sample AWSSimpleSystemsManagement.ListDocuments
* @see AWS API
* Documentation
*/
ListDocumentsResult listDocuments(ListDocumentsRequest listDocumentsRequest);
/**
* Simplified method form for invoking the ListDocuments operation.
*
* @see #listDocuments(ListDocumentsRequest)
*/
ListDocumentsResult listDocuments();
/**
*
* A list of inventory items returned by the request.
*
*
* @param listInventoryEntriesRequest
* @return Result of the ListInventoryEntries operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidTypeNameException
* The parameter type name isn't valid.
* @throws InvalidFilterException
* The filter name isn't valid. Verify the you entered the correct name and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.ListInventoryEntries
* @see AWS API
* Documentation
*/
ListInventoryEntriesResult listInventoryEntries(ListInventoryEntriesRequest listInventoryEntriesRequest);
/**
*
* Returns a list of all OpsItem events in the current Amazon Web Services Region and Amazon Web Services account.
* You can limit the results to events associated with specific OpsItems by specifying a filter.
*
*
* @param listOpsItemEventsRequest
* @return Result of the ListOpsItemEvents operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws OpsItemNotFoundException
* The specified OpsItem ID doesn't exist. Verify the ID and try again.
* @throws OpsItemLimitExceededException
* The request caused OpsItems to exceed one or more quotas.
* @throws OpsItemInvalidParameterException
* A specified parameter argument isn't valid. Verify the available arguments and try again.
* @sample AWSSimpleSystemsManagement.ListOpsItemEvents
* @see AWS API
* Documentation
*/
ListOpsItemEventsResult listOpsItemEvents(ListOpsItemEventsRequest listOpsItemEventsRequest);
/**
*
* Lists all related-item resources associated with a Systems Manager OpsCenter OpsItem. OpsCenter is a capability
* of Amazon Web Services Systems Manager.
*
*
* @param listOpsItemRelatedItemsRequest
* @return Result of the ListOpsItemRelatedItems operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws OpsItemInvalidParameterException
* A specified parameter argument isn't valid. Verify the available arguments and try again.
* @sample AWSSimpleSystemsManagement.ListOpsItemRelatedItems
* @see AWS
* API Documentation
*/
ListOpsItemRelatedItemsResult listOpsItemRelatedItems(ListOpsItemRelatedItemsRequest listOpsItemRelatedItemsRequest);
/**
*
* Amazon Web Services Systems Manager calls this API operation when displaying all Application Manager OpsMetadata
* objects or blobs.
*
*
* @param listOpsMetadataRequest
* @return Result of the ListOpsMetadata operation returned by the service.
* @throws OpsMetadataInvalidArgumentException
* One of the arguments passed is invalid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.ListOpsMetadata
* @see AWS API
* Documentation
*/
ListOpsMetadataResult listOpsMetadata(ListOpsMetadataRequest listOpsMetadataRequest);
/**
*
* Returns a resource-level summary count. The summary includes information about compliant and non-compliant
* statuses and detailed compliance-item severity counts, according to the filter criteria you specify.
*
*
* @param listResourceComplianceSummariesRequest
* @return Result of the ListResourceComplianceSummaries operation returned by the service.
* @throws InvalidFilterException
* The filter name isn't valid. Verify the you entered the correct name and try again.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.ListResourceComplianceSummaries
* @see AWS API Documentation
*/
ListResourceComplianceSummariesResult listResourceComplianceSummaries(ListResourceComplianceSummariesRequest listResourceComplianceSummariesRequest);
/**
*
* Lists your resource data sync configurations. Includes information about the last time a sync attempted to start,
* the last sync status, and the last time a sync successfully completed.
*
*
* The number of sync configurations might be too large to return using a single call to
* ListResourceDataSync
. You can limit the number of sync configurations returned by using the
* MaxResults
parameter. To determine whether there are more sync configurations to list, check the
* value of NextToken
in the output. If there are more sync configurations to list, you can request
* them by specifying the NextToken
returned in the call to the parameter of a subsequent call.
*
*
* @param listResourceDataSyncRequest
* @return Result of the ListResourceDataSync operation returned by the service.
* @throws ResourceDataSyncInvalidConfigurationException
* The specified sync configuration is invalid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidNextTokenException
* The specified token isn't valid.
* @sample AWSSimpleSystemsManagement.ListResourceDataSync
* @see AWS API
* Documentation
*/
ListResourceDataSyncResult listResourceDataSync(ListResourceDataSyncRequest listResourceDataSyncRequest);
/**
*
* Returns a list of the tags assigned to the specified resource.
*
*
* For information about the ID format for each supported resource type, see AddTagsToResource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidResourceTypeException
* The resource type isn't valid. For example, if you are attempting to tag an EC2 instance, the instance
* must be a registered managed node.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.ListTagsForResource
* @see AWS API
* Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Shares a Amazon Web Services Systems Manager document (SSM document)publicly or privately. If you share a
* document privately, you must specify the Amazon Web Services user IDs for those people who can use the document.
* If you share a document publicly, you must specify All as the account ID.
*
*
* @param modifyDocumentPermissionRequest
* @return Result of the ModifyDocumentPermission operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidPermissionTypeException
* The permission type isn't supported. Share is the only supported permission type.
* @throws DocumentPermissionLimitException
* The document can't be shared with more Amazon Web Services accounts. You can specify a maximum of 20
* accounts per API operation to share a private document.
*
* By default, you can share a private document with a maximum of 1,000 accounts and publicly share up to
* five documents.
*
*
* If you need to increase the quota for privately or publicly shared Systems Manager documents, contact
* Amazon Web Services Support.
* @throws DocumentLimitExceededException
* You can have at most 500 active SSM documents.
* @sample AWSSimpleSystemsManagement.ModifyDocumentPermission
* @see AWS
* API Documentation
*/
ModifyDocumentPermissionResult modifyDocumentPermission(ModifyDocumentPermissionRequest modifyDocumentPermissionRequest);
/**
*
* Registers a compliance type and other compliance details on a designated resource. This operation lets you
* register custom compliance details with a resource. This call overwrites existing compliance information on the
* resource, so you must provide a full list of compliance items each time that you send the request.
*
*
* ComplianceType can be one of the following:
*
*
* -
*
* ExecutionId: The execution ID when the patch, association, or custom compliance item was applied.
*
*
* -
*
* ExecutionType: Specify patch, association, or Custom:string
.
*
*
* -
*
* ExecutionTime. The time the patch, association, or custom compliance item was applied to the managed node.
*
*
* -
*
* Id: The patch, association, or custom compliance ID.
*
*
* -
*
* Title: A title.
*
*
* -
*
* Status: The status of the compliance item. For example, approved
for patches, or Failed
* for associations.
*
*
* -
*
* Severity: A patch severity. For example, Critical
.
*
*
* -
*
* DocumentName: An SSM document name. For example, AWS-RunPatchBaseline
.
*
*
* -
*
* DocumentVersion: An SSM document version number. For example, 4.
*
*
* -
*
* Classification: A patch classification. For example, security updates
.
*
*
* -
*
* PatchBaselineId: A patch baseline ID.
*
*
* -
*
* PatchSeverity: A patch severity. For example, Critical
.
*
*
* -
*
* PatchState: A patch state. For example, InstancesWithFailedPatches
.
*
*
* -
*
* PatchGroup: The name of a patch group.
*
*
* -
*
* InstalledTime: The time the association, patch, or custom compliance item was applied to the resource. Specify
* the time by using the following format: yyyy-MM-dd'T'HH:mm:ss'Z'
*
*
*
*
* @param putComplianceItemsRequest
* @return Result of the PutComplianceItems operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidItemContentException
* One or more content items isn't valid.
* @throws TotalSizeLimitExceededException
* The size of inventory data has exceeded the total size limit for the resource.
* @throws ItemSizeLimitExceededException
* The inventory item size has exceeded the size limit.
* @throws ComplianceTypeCountLimitExceededException
* You specified too many custom compliance types. You can specify a maximum of 10 different types.
* @throws InvalidResourceTypeException
* The resource type isn't valid. For example, if you are attempting to tag an EC2 instance, the instance
* must be a registered managed node.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @sample AWSSimpleSystemsManagement.PutComplianceItems
* @see AWS API
* Documentation
*/
PutComplianceItemsResult putComplianceItems(PutComplianceItemsRequest putComplianceItemsRequest);
/**
*
* Bulk update custom inventory items on one or more managed nodes. The request adds an inventory item, if it
* doesn't already exist, or updates an inventory item, if it does exist.
*
*
* @param putInventoryRequest
* @return Result of the PutInventory operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidTypeNameException
* The parameter type name isn't valid.
* @throws InvalidItemContentException
* One or more content items isn't valid.
* @throws TotalSizeLimitExceededException
* The size of inventory data has exceeded the total size limit for the resource.
* @throws ItemSizeLimitExceededException
* The inventory item size has exceeded the size limit.
* @throws ItemContentMismatchException
* The inventory item has invalid content.
* @throws CustomSchemaCountLimitExceededException
* You have exceeded the limit for custom schemas. Delete one or more custom schemas and try again.
* @throws UnsupportedInventorySchemaVersionException
* Inventory item type schema version has to match supported versions in the service. Check output of
* GetInventorySchema to see the available schema version for each type.
* @throws UnsupportedInventoryItemContextException
* The Context
attribute that you specified for the InventoryItem
isn't allowed
* for this inventory type. You can only use the Context
attribute with inventory types like
* AWS:ComplianceItem
.
* @throws InvalidInventoryItemContextException
* You specified invalid keys or values in the Context
attribute for InventoryItem
* . Verify the keys and values, and try again.
* @throws SubTypeCountLimitExceededException
* The sub-type count exceeded the limit for the inventory type.
* @sample AWSSimpleSystemsManagement.PutInventory
* @see AWS API
* Documentation
*/
PutInventoryResult putInventory(PutInventoryRequest putInventoryRequest);
/**
*
* Add a parameter to the system.
*
*
* @param putParameterRequest
* @return Result of the PutParameter operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidKeyIdException
* The query key ID isn't valid.
* @throws ParameterLimitExceededException
* You have exceeded the number of parameters for this Amazon Web Services account. Delete one or more
* parameters and try again.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @throws ParameterAlreadyExistsException
* The parameter already exists. You can't create duplicate parameters.
* @throws HierarchyLevelLimitExceededException
* A hierarchy can have a maximum of 15 levels. For more information, see Requirements and constraints for parameter names in the Amazon Web Services Systems Manager User
* Guide.
* @throws HierarchyTypeMismatchException
* Parameter Store doesn't support changing a parameter type in a hierarchy. For example, you can't change a
* parameter from a String
type to a SecureString
type. You must create a new,
* unique parameter.
* @throws InvalidAllowedPatternException
* The request doesn't meet the regular expression requirement.
* @throws ParameterMaxVersionLimitExceededException
* Parameter Store retains the 100 most recently created versions of a parameter. After this number of
* versions has been created, Parameter Store deletes the oldest version when a new one is created. However,
* if the oldest version has a label attached to it, Parameter Store won't delete the version and
* instead presents this error message:
*
* An error occurred (ParameterMaxVersionLimitExceeded) when calling the PutParameter operation: You attempted to create a new version of parameter-name by calling the PutParameter API with the overwrite flag. Version version-number, the oldest version, can't be deleted because it has a label associated with it. Move the label to another version of the parameter, and try again.
*
*
* This safeguard is to prevent parameter versions with mission critical labels assigned to them from being
* deleted. To continue creating new parameters, first move the label from the oldest version of the
* parameter to a newer one for use in your operations. For information about moving parameter labels, see
* Move a parameter label (console) or Move a parameter label (CLI) in the Amazon Web Services Systems Manager User Guide.
* @throws ParameterPatternMismatchException
* The parameter name isn't valid.
* @throws UnsupportedParameterTypeException
* The parameter type isn't supported.
* @throws PoliciesLimitExceededException
* You specified more than the maximum number of allowed policies for the parameter. The maximum is 10.
* @throws InvalidPolicyTypeException
* The policy type isn't supported. Parameter Store supports the following policy types: Expiration,
* ExpirationNotification, and NoChangeNotification.
* @throws InvalidPolicyAttributeException
* A policy attribute or its value is invalid.
* @throws IncompatiblePolicyException
* There is a conflict in the policies specified for this parameter. You can't, for example, specify two
* Expiration policies for a parameter. Review your policies, and try again.
* @sample AWSSimpleSystemsManagement.PutParameter
* @see AWS API
* Documentation
*/
PutParameterResult putParameter(PutParameterRequest putParameterRequest);
/**
*
* Creates or updates a Systems Manager resource policy. A resource policy helps you to define the IAM entity (for
* example, an Amazon Web Services account) that can manage your Systems Manager resources. The following resources
* support Systems Manager resource policies.
*
*
* -
*
* OpsItemGroup
- The resource policy for OpsItemGroup
enables Amazon Web Services
* accounts to view and interact with OpsCenter operational work items (OpsItems).
*
*
* -
*
* Parameter
- The resource policy is used to share a parameter with other accounts using Resource
* Access Manager (RAM).
*
*
* To share a parameter, it must be in the advanced parameter tier. For information about parameter tiers, see
* Managing parameter tiers. For information about changing an existing standard parameter to an advanced
* parameter, see Changing a standard parameter to an advanced parameter.
*
*
* To share a SecureString
parameter, it must be encrypted with a customer managed key, and you must
* share the key separately through Key Management Service. Amazon Web Services managed keys cannot be shared.
* Parameters encrypted with the default Amazon Web Services managed key can be updated to use a customer managed
* key instead. For KMS key definitions, see KMS concepts in the Key
* Management Service Developer Guide.
*
*
*
* While you can share a parameter using the Systems Manager PutResourcePolicy
operation, we recommend
* using Resource Access Manager (RAM) instead. This is because using PutResourcePolicy
requires the
* extra step of promoting the parameter to a standard RAM Resource Share using the RAM PromoteResourceShareCreatedFromPolicy API operation. Otherwise, the parameter won't be returned by the
* Systems Manager DescribeParameters API operation using the --shared
option.
*
*
* For more information, see Sharing a parameter in the Amazon Web Services Systems Manager User Guide
*
*
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ResourcePolicyInvalidParameterException
* One or more parameters specified for the call aren't valid. Verify the parameters and their values and
* try again.
* @throws ResourcePolicyLimitExceededException
* The PutResourcePolicy API action enforces two limits. A policy can't be greater than 1024 bytes in
* size. And only one policy can be attached to OpsItemGroup
. Verify these limits and try
* again.
* @throws ResourcePolicyConflictException
* The hash provided in the call doesn't match the stored hash. This exception is thrown when trying to
* update an obsolete policy version or when multiple requests to update a policy are sent.
* @throws ResourceNotFoundException
* The specified parameter to be shared could not be found.
* @throws MalformedResourcePolicyDocumentException
* The specified policy document is malformed or invalid, or excessive PutResourcePolicy
or
* DeleteResourcePolicy
calls have been made.
* @throws ResourcePolicyNotFoundException
* No policies with the specified policy ID and hash could be found.
* @sample AWSSimpleSystemsManagement.PutResourcePolicy
* @see AWS API
* Documentation
*/
PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest);
/**
*
* Defines the default patch baseline for the relevant operating system.
*
*
* To reset the Amazon Web Services-predefined patch baseline as the default, specify the full patch baseline Amazon
* Resource Name (ARN) as the baseline ID value. For example, for CentOS, specify
* arn:aws:ssm:us-east-2:733109147000:patchbaseline/pb-0574b43a65ea646ed
instead of
* pb-0574b43a65ea646ed
.
*
*
* @param registerDefaultPatchBaselineRequest
* @return Result of the RegisterDefaultPatchBaseline operation returned by the service.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.RegisterDefaultPatchBaseline
* @see AWS API Documentation
*/
RegisterDefaultPatchBaselineResult registerDefaultPatchBaseline(RegisterDefaultPatchBaselineRequest registerDefaultPatchBaselineRequest);
/**
*
* Registers a patch baseline for a patch group.
*
*
* @param registerPatchBaselineForPatchGroupRequest
* @return Result of the RegisterPatchBaselineForPatchGroup operation returned by the service.
* @throws AlreadyExistsException
* Error returned if an attempt is made to register a patch group with a patch baseline that is already
* registered with a different patch baseline.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @throws ResourceLimitExceededException
* Error returned when the caller has exceeded the default resource quotas. For example, too many
* maintenance windows or patch baselines have been created.
*
*
* For information about resource quotas in Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.RegisterPatchBaselineForPatchGroup
* @see AWS API Documentation
*/
RegisterPatchBaselineForPatchGroupResult registerPatchBaselineForPatchGroup(
RegisterPatchBaselineForPatchGroupRequest registerPatchBaselineForPatchGroupRequest);
/**
*
* Registers a target with a maintenance window.
*
*
* @param registerTargetWithMaintenanceWindowRequest
* @return Result of the RegisterTargetWithMaintenanceWindow operation returned by the service.
* @throws IdempotentParameterMismatchException
* Error returned when an idempotent operation is retried and the parameters don't match the original call
* to the API with the same idempotency token.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws ResourceLimitExceededException
* Error returned when the caller has exceeded the default resource quotas. For example, too many
* maintenance windows or patch baselines have been created.
*
*
* For information about resource quotas in Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.RegisterTargetWithMaintenanceWindow
* @see AWS API Documentation
*/
RegisterTargetWithMaintenanceWindowResult registerTargetWithMaintenanceWindow(
RegisterTargetWithMaintenanceWindowRequest registerTargetWithMaintenanceWindowRequest);
/**
*
* Adds a new task to a maintenance window.
*
*
* @param registerTaskWithMaintenanceWindowRequest
* @return Result of the RegisterTaskWithMaintenanceWindow operation returned by the service.
* @throws IdempotentParameterMismatchException
* Error returned when an idempotent operation is retried and the parameters don't match the original call
* to the API with the same idempotency token.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws ResourceLimitExceededException
* Error returned when the caller has exceeded the default resource quotas. For example, too many
* maintenance windows or patch baselines have been created.
*
*
* For information about resource quotas in Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws FeatureNotAvailableException
* You attempted to register a LAMBDA
or STEP_FUNCTIONS
task in a region where the
* corresponding service isn't available.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.RegisterTaskWithMaintenanceWindow
* @see AWS API Documentation
*/
RegisterTaskWithMaintenanceWindowResult registerTaskWithMaintenanceWindow(RegisterTaskWithMaintenanceWindowRequest registerTaskWithMaintenanceWindowRequest);
/**
*
* Removes tag keys from the specified resource.
*
*
* @param removeTagsFromResourceRequest
* @return Result of the RemoveTagsFromResource operation returned by the service.
* @throws InvalidResourceTypeException
* The resource type isn't valid. For example, if you are attempting to tag an EC2 instance, the instance
* must be a registered managed node.
* @throws InvalidResourceIdException
* The resource ID isn't valid. Verify that you entered the correct ID and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @sample AWSSimpleSystemsManagement.RemoveTagsFromResource
* @see AWS API
* Documentation
*/
RemoveTagsFromResourceResult removeTagsFromResource(RemoveTagsFromResourceRequest removeTagsFromResourceRequest);
/**
*
* ServiceSetting
is an account-level setting for an Amazon Web Services service. This setting defines
* how a user interacts with or uses a service or a feature of a service. For example, if an Amazon Web Services
* service charges money to the account based on feature or service usage, then the Amazon Web Services service team
* might create a default setting of "false". This means the user can't use this feature unless they change the
* setting to "true" and intentionally opt in for a paid feature.
*
*
* Services map a SettingId
object to a setting value. Amazon Web Services services teams define the
* default value for a SettingId
. You can't create a new SettingId
, but you can overwrite
* the default value if you have the ssm:UpdateServiceSetting
permission for the setting. Use the
* GetServiceSetting API operation to view the current value. Use the UpdateServiceSetting API
* operation to change the default setting.
*
*
* Reset the service setting for the account to the default value as provisioned by the Amazon Web Services service
* team.
*
*
* @param resetServiceSettingRequest
* The request body of the ResetServiceSetting API operation.
* @return Result of the ResetServiceSetting operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ServiceSettingNotFoundException
* The specified service setting wasn't found. Either the service name or the setting hasn't been
* provisioned by the Amazon Web Services service team.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @sample AWSSimpleSystemsManagement.ResetServiceSetting
* @see AWS API
* Documentation
*/
ResetServiceSettingResult resetServiceSetting(ResetServiceSettingRequest resetServiceSettingRequest);
/**
*
* Reconnects a session to a managed node after it has been disconnected. Connections can be resumed for
* disconnected sessions, but not terminated sessions.
*
*
*
* This command is primarily for use by client machines to automatically reconnect during intermittent network
* issues. It isn't intended for any other use.
*
*
*
* @param resumeSessionRequest
* @return Result of the ResumeSession operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.ResumeSession
* @see AWS API
* Documentation
*/
ResumeSessionResult resumeSession(ResumeSessionRequest resumeSessionRequest);
/**
*
* Sends a signal to an Automation execution to change the current behavior or status of the execution.
*
*
* @param sendAutomationSignalRequest
* @return Result of the SendAutomationSignal operation returned by the service.
* @throws AutomationExecutionNotFoundException
* There is no automation execution information for the requested automation execution ID.
* @throws AutomationStepNotFoundException
* The specified step name and execution ID don't exist. Verify the information and try again.
* @throws InvalidAutomationSignalException
* The signal isn't valid for the current Automation execution.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.SendAutomationSignal
* @see AWS API
* Documentation
*/
SendAutomationSignalResult sendAutomationSignal(SendAutomationSignalRequest sendAutomationSignalRequest);
/**
*
* Runs commands on one or more managed nodes.
*
*
* @param sendCommandRequest
* @return Result of the SendCommand operation returned by the service.
* @throws DuplicateInstanceIdException
* You can't specify a managed node ID in more than one association.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @throws InvalidOutputFolderException
* The S3 bucket doesn't exist.
* @throws InvalidParametersException
* You must specify values for all required parameters in the Amazon Web Services Systems Manager document
* (SSM document). You can only supply values to parameters defined in the SSM document.
* @throws UnsupportedPlatformTypeException
* The document doesn't support the platform type of the given managed node IDs. For example, you sent an
* document for a Windows managed node to a Linux node.
* @throws MaxDocumentSizeExceededException
* The size limit of a document is 64 KB.
* @throws InvalidRoleException
* The role name can't contain invalid characters. Also verify that you specified an IAM role for
* notifications that includes the required trust policy. For information about configuring the IAM role for
* Run Command notifications, see Monitoring Systems Manager status changes using Amazon SNS notifications in the Amazon Web
* Services Systems Manager User Guide.
* @throws InvalidNotificationConfigException
* One or more configuration items isn't valid. Verify that a valid Amazon Resource Name (ARN) was provided
* for an Amazon Simple Notification Service topic.
* @sample AWSSimpleSystemsManagement.SendCommand
* @see AWS API
* Documentation
*/
SendCommandResult sendCommand(SendCommandRequest sendCommandRequest);
/**
*
* Runs an association immediately and only one time. This operation can be helpful when troubleshooting
* associations.
*
*
* @param startAssociationsOnceRequest
* @return Result of the StartAssociationsOnce operation returned by the service.
* @throws InvalidAssociationException
* The association isn't valid or doesn't exist.
* @throws AssociationDoesNotExistException
* The specified association doesn't exist.
* @sample AWSSimpleSystemsManagement.StartAssociationsOnce
* @see AWS API
* Documentation
*/
StartAssociationsOnceResult startAssociationsOnce(StartAssociationsOnceRequest startAssociationsOnceRequest);
/**
*
* Initiates execution of an Automation runbook.
*
*
* @param startAutomationExecutionRequest
* @return Result of the StartAutomationExecution operation returned by the service.
* @throws AutomationDefinitionNotFoundException
* An Automation runbook with the specified name couldn't be found.
* @throws InvalidAutomationExecutionParametersException
* The supplied parameters for invoking the specified Automation runbook are incorrect. For example, they
* may not match the set of parameters permitted for the specified Automation document.
* @throws AutomationExecutionLimitExceededException
* The number of simultaneously running Automation executions exceeded the allowable limit.
* @throws AutomationDefinitionVersionNotFoundException
* An Automation runbook with the specified name and version couldn't be found.
* @throws IdempotentParameterMismatchException
* Error returned when an idempotent operation is retried and the parameters don't match the original call
* to the API with the same idempotency token.
* @throws InvalidTargetException
* The target isn't valid or doesn't exist. It might not be configured for Systems Manager or you might not
* have permission to perform the operation.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.StartAutomationExecution
* @see AWS
* API Documentation
*/
StartAutomationExecutionResult startAutomationExecution(StartAutomationExecutionRequest startAutomationExecutionRequest);
/**
*
* Creates a change request for Change Manager. The Automation runbooks specified in the change request run only
* after all required approvals for the change request have been received.
*
*
* @param startChangeRequestExecutionRequest
* @return Result of the StartChangeRequestExecution operation returned by the service.
* @throws AutomationDefinitionNotFoundException
* An Automation runbook with the specified name couldn't be found.
* @throws InvalidAutomationExecutionParametersException
* The supplied parameters for invoking the specified Automation runbook are incorrect. For example, they
* may not match the set of parameters permitted for the specified Automation document.
* @throws AutomationExecutionLimitExceededException
* The number of simultaneously running Automation executions exceeded the allowable limit.
* @throws AutomationDefinitionVersionNotFoundException
* An Automation runbook with the specified name and version couldn't be found.
* @throws IdempotentParameterMismatchException
* Error returned when an idempotent operation is retried and the parameters don't match the original call
* to the API with the same idempotency token.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws AutomationDefinitionNotApprovedException
* Indicates that the Change Manager change template used in the change request was rejected or is still in
* a pending state.
* @sample AWSSimpleSystemsManagement.StartChangeRequestExecution
* @see AWS API Documentation
*/
StartChangeRequestExecutionResult startChangeRequestExecution(StartChangeRequestExecutionRequest startChangeRequestExecutionRequest);
/**
*
* Initiates a connection to a target (for example, a managed node) for a Session Manager session. Returns a URL and
* token that can be used to open a WebSocket connection for sending input and receiving outputs.
*
*
*
* Amazon Web Services CLI usage: start-session
is an interactive command that requires the Session
* Manager plugin to be installed on the client machine making the call. For information, see Install the Session Manager plugin for the Amazon Web Services CLI in the Amazon Web Services Systems
* Manager User Guide.
*
*
* Amazon Web Services Tools for PowerShell usage: Start-SSMSession isn't currently supported by Amazon Web Services
* Tools for PowerShell on Windows local machines.
*
*
*
* @param startSessionRequest
* @return Result of the StartSession operation returned by the service.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws TargetNotConnectedException
* The specified target managed node for the session isn't fully configured for use with Session Manager.
* For more information, see Getting started with Session Manager in the Amazon Web Services Systems Manager User Guide.
* This error is also returned if you attempt to start a session on a managed node that is located in a
* different account or Region
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.StartSession
* @see AWS API
* Documentation
*/
StartSessionResult startSession(StartSessionRequest startSessionRequest);
/**
*
* Stop an Automation that is currently running.
*
*
* @param stopAutomationExecutionRequest
* @return Result of the StopAutomationExecution operation returned by the service.
* @throws AutomationExecutionNotFoundException
* There is no automation execution information for the requested automation execution ID.
* @throws InvalidAutomationStatusUpdateException
* The specified update status operation isn't valid.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.StopAutomationExecution
* @see AWS
* API Documentation
*/
StopAutomationExecutionResult stopAutomationExecution(StopAutomationExecutionRequest stopAutomationExecutionRequest);
/**
*
* Permanently ends a session and closes the data connection between the Session Manager client and SSM Agent on the
* managed node. A terminated session can't be resumed.
*
*
* @param terminateSessionRequest
* @return Result of the TerminateSession operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.TerminateSession
* @see AWS API
* Documentation
*/
TerminateSessionResult terminateSession(TerminateSessionRequest terminateSessionRequest);
/**
*
* Remove a label or labels from a parameter.
*
*
* @param unlabelParameterVersionRequest
* @return Result of the UnlabelParameterVersion operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @throws ParameterNotFoundException
* The parameter couldn't be found. Verify the name and try again.
* @throws ParameterVersionNotFoundException
* The specified parameter version wasn't found. Verify the parameter name and version, and try again.
* @sample AWSSimpleSystemsManagement.UnlabelParameterVersion
* @see AWS
* API Documentation
*/
UnlabelParameterVersionResult unlabelParameterVersion(UnlabelParameterVersionRequest unlabelParameterVersionRequest);
/**
*
* Updates an association. You can update the association name and version, the document version, schedule,
* parameters, and Amazon Simple Storage Service (Amazon S3) output. When you call UpdateAssociation
,
* the system removes all optional parameters from the request and overwrites the association with null values for
* those parameters. This is by design. You must specify all optional parameters in the call, even if you are not
* changing the parameters. This includes the Name
parameter. Before calling this API action, we
* recommend that you call the DescribeAssociation API operation and make a note of all optional parameters
* required for your UpdateAssociation
call.
*
*
* In order to call this API operation, a user, group, or role must be granted permission to call the
* DescribeAssociation API operation. If you don't have permission to call DescribeAssociation
,
* then you receive the following error:
* An error occurred (AccessDeniedException) when calling the UpdateAssociation operation: User: <user_arn> isn't authorized to perform: ssm:DescribeAssociation on resource: <resource_arn>
*
*
*
* When you update an association, the association immediately runs against the specified targets. You can add the
* ApplyOnlyAtCronInterval
parameter to run the association during the next schedule run.
*
*
*
* @param updateAssociationRequest
* @return Result of the UpdateAssociation operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidScheduleException
* The schedule is invalid. Verify your cron or rate expression and try again.
* @throws InvalidParametersException
* You must specify values for all required parameters in the Amazon Web Services Systems Manager document
* (SSM document). You can only supply values to parameters defined in the SSM document.
* @throws InvalidOutputLocationException
* The output location isn't valid or doesn't exist.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @throws AssociationDoesNotExistException
* The specified association doesn't exist.
* @throws InvalidUpdateException
* The update isn't valid.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidTargetException
* The target isn't valid or doesn't exist. It might not be configured for Systems Manager or you might not
* have permission to perform the operation.
* @throws InvalidAssociationVersionException
* The version you specified isn't valid. Use ListAssociationVersions to view all versions of an association
* according to the association ID. Or, use the $LATEST
parameter to view the latest version of
* the association.
* @throws AssociationVersionLimitExceededException
* You have reached the maximum number versions allowed for an association. Each association has a limit of
* 1,000 versions.
* @throws InvalidTargetMapsException
* TargetMap parameter isn't valid.
* @sample AWSSimpleSystemsManagement.UpdateAssociation
* @see AWS API
* Documentation
*/
UpdateAssociationResult updateAssociation(UpdateAssociationRequest updateAssociationRequest);
/**
*
* Updates the status of the Amazon Web Services Systems Manager document (SSM document) associated with the
* specified managed node.
*
*
* UpdateAssociationStatus
is primarily used by the Amazon Web Services Systems Manager Agent (SSM
* Agent) to report status updates about your associations and is only used for associations created with the
* InstanceId
legacy parameter.
*
*
* @param updateAssociationStatusRequest
* @return Result of the UpdateAssociationStatus operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws AssociationDoesNotExistException
* The specified association doesn't exist.
* @throws StatusUnchangedException
* The updated status is the same as the current status.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @sample AWSSimpleSystemsManagement.UpdateAssociationStatus
* @see AWS
* API Documentation
*/
UpdateAssociationStatusResult updateAssociationStatus(UpdateAssociationStatusRequest updateAssociationStatusRequest);
/**
*
* Updates one or more values for an SSM document.
*
*
* @param updateDocumentRequest
* @return Result of the UpdateDocument operation returned by the service.
* @throws MaxDocumentSizeExceededException
* The size limit of a document is 64 KB.
* @throws DocumentVersionLimitExceededException
* The document has too many versions. Delete one or more document versions and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws DuplicateDocumentContentException
* The content of the association document matches another document. Change the content of the document and
* try again.
* @throws DuplicateDocumentVersionNameException
* The version name has already been used in this document. Specify a different version name, and then try
* again.
* @throws InvalidDocumentContentException
* The content for the document isn't valid.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @throws InvalidDocumentSchemaVersionException
* The version of the document schema isn't supported.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentOperationException
* You attempted to delete a document while it is still shared. You must stop sharing the document before
* you can delete it.
* @sample AWSSimpleSystemsManagement.UpdateDocument
* @see AWS API
* Documentation
*/
UpdateDocumentResult updateDocument(UpdateDocumentRequest updateDocumentRequest);
/**
*
* Set the default version of a document.
*
*
*
* If you change a document version for a State Manager association, Systems Manager immediately runs the
* association unless you previously specifed the apply-only-at-cron-interval
parameter.
*
*
*
* @param updateDocumentDefaultVersionRequest
* @return Result of the UpdateDocumentDefaultVersion operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @throws InvalidDocumentSchemaVersionException
* The version of the document schema isn't supported.
* @sample AWSSimpleSystemsManagement.UpdateDocumentDefaultVersion
* @see AWS API Documentation
*/
UpdateDocumentDefaultVersionResult updateDocumentDefaultVersion(UpdateDocumentDefaultVersionRequest updateDocumentDefaultVersionRequest);
/**
*
* Updates information related to approval reviews for a specific version of a change template in Change Manager.
*
*
* @param updateDocumentMetadataRequest
* @return Result of the UpdateDocumentMetadata operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws InvalidDocumentException
* The specified SSM document doesn't exist.
* @throws InvalidDocumentOperationException
* You attempted to delete a document while it is still shared. You must stop sharing the document before
* you can delete it.
* @throws InvalidDocumentVersionException
* The document version isn't valid or doesn't exist.
* @sample AWSSimpleSystemsManagement.UpdateDocumentMetadata
* @see AWS API
* Documentation
*/
UpdateDocumentMetadataResult updateDocumentMetadata(UpdateDocumentMetadataRequest updateDocumentMetadataRequest);
/**
*
* Updates an existing maintenance window. Only specified parameters are modified.
*
*
*
* The value you specify for Duration
determines the specific end time for the maintenance window based
* on the time it begins. No maintenance window tasks are permitted to start after the resulting endtime minus the
* number of hours you specify for Cutoff
. For example, if the maintenance window starts at 3 PM, the
* duration is three hours, and the value you specify for Cutoff
is one hour, no maintenance window
* tasks can start after 5 PM.
*
*
*
* @param updateMaintenanceWindowRequest
* @return Result of the UpdateMaintenanceWindow operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.UpdateMaintenanceWindow
* @see AWS
* API Documentation
*/
UpdateMaintenanceWindowResult updateMaintenanceWindow(UpdateMaintenanceWindowRequest updateMaintenanceWindowRequest);
/**
*
* Modifies the target of an existing maintenance window. You can change the following:
*
*
* -
*
* Name
*
*
* -
*
* Description
*
*
* -
*
* Owner
*
*
* -
*
* IDs for an ID target
*
*
* -
*
* Tags for a Tag target
*
*
* -
*
* From any supported tag type to another. The three supported tag types are ID target, Tag target, and resource
* group. For more information, see Target.
*
*
*
*
*
* If a parameter is null, then the corresponding field isn't modified.
*
*
*
* @param updateMaintenanceWindowTargetRequest
* @return Result of the UpdateMaintenanceWindowTarget operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.UpdateMaintenanceWindowTarget
* @see AWS API Documentation
*/
UpdateMaintenanceWindowTargetResult updateMaintenanceWindowTarget(UpdateMaintenanceWindowTargetRequest updateMaintenanceWindowTargetRequest);
/**
*
* Modifies a task assigned to a maintenance window. You can't change the task type, but you can change the
* following values:
*
*
* -
*
* TaskARN
. For example, you can change a RUN_COMMAND
task from
* AWS-RunPowerShellScript
to AWS-RunShellScript
.
*
*
* -
*
* ServiceRoleArn
*
*
* -
*
* TaskInvocationParameters
*
*
* -
*
* Priority
*
*
* -
*
* MaxConcurrency
*
*
* -
*
* MaxErrors
*
*
*
*
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the task,
* targets are optional for other maintenance window task types (Automation, Lambda, and Step Functions). For more
* information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* If the value for a parameter in UpdateMaintenanceWindowTask
is null, then the corresponding field
* isn't modified. If you set Replace
to true, then all fields required by the
* RegisterTaskWithMaintenanceWindow operation are required for this request. Optional fields that aren't
* specified are set to null.
*
*
*
* When you update a maintenance window task that has options specified in TaskInvocationParameters
,
* you must provide again all the TaskInvocationParameters
values that you want to retain. The values
* you don't specify again are removed. For example, suppose that when you registered a Run Command task, you
* specified TaskInvocationParameters
values for Comment
, NotificationConfig
,
* and OutputS3BucketName
. If you update the maintenance window task and specify only a different
* OutputS3BucketName
value, the values for Comment
and NotificationConfig
* are removed.
*
*
*
* @param updateMaintenanceWindowTaskRequest
* @return Result of the UpdateMaintenanceWindowTask operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.UpdateMaintenanceWindowTask
* @see AWS API Documentation
*/
UpdateMaintenanceWindowTaskResult updateMaintenanceWindowTask(UpdateMaintenanceWindowTaskRequest updateMaintenanceWindowTaskRequest);
/**
*
* Changes the Identity and Access Management (IAM) role that is assigned to the on-premises server, edge device, or
* virtual machines (VM). IAM roles are first assigned to these hybrid nodes during the activation process. For more
* information, see CreateActivation.
*
*
* @param updateManagedInstanceRoleRequest
* @return Result of the UpdateManagedInstanceRole operation returned by the service.
* @throws InvalidInstanceIdException
* The following problems can cause this exception:
*
* -
*
* You don't have permission to access the managed node.
*
*
* -
*
* Amazon Web Services Systems Manager Agent (SSM Agent) isn't running. Verify that SSM Agent is running.
*
*
* -
*
* SSM Agent isn't registered with the SSM endpoint. Try reinstalling SSM Agent.
*
*
* -
*
* The managed node isn't in a valid state. Valid states are: Running
, Pending
,
* Stopped
, and Stopping
. Invalid states are: Shutting-down
and
* Terminated
.
*
*
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.UpdateManagedInstanceRole
* @see AWS
* API Documentation
*/
UpdateManagedInstanceRoleResult updateManagedInstanceRole(UpdateManagedInstanceRoleRequest updateManagedInstanceRoleRequest);
/**
*
* Edit or change an OpsItem. You must have permission in Identity and Access Management (IAM) to update an OpsItem.
* For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param updateOpsItemRequest
* @return Result of the UpdateOpsItem operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws OpsItemNotFoundException
* The specified OpsItem ID doesn't exist. Verify the ID and try again.
* @throws OpsItemAlreadyExistsException
* The OpsItem already exists.
* @throws OpsItemLimitExceededException
* The request caused OpsItems to exceed one or more quotas.
* @throws OpsItemInvalidParameterException
* A specified parameter argument isn't valid. Verify the available arguments and try again.
* @throws OpsItemAccessDeniedException
* You don't have permission to view OpsItems in the specified account. Verify that your account is
* configured either as a Systems Manager delegated administrator or that you are logged into the
* Organizations management account.
* @throws OpsItemConflictException
* The specified OpsItem is in the process of being deleted.
* @sample AWSSimpleSystemsManagement.UpdateOpsItem
* @see AWS API
* Documentation
*/
UpdateOpsItemResult updateOpsItem(UpdateOpsItemRequest updateOpsItemRequest);
/**
*
* Amazon Web Services Systems Manager calls this API operation when you edit OpsMetadata in Application Manager.
*
*
* @param updateOpsMetadataRequest
* @return Result of the UpdateOpsMetadata operation returned by the service.
* @throws OpsMetadataNotFoundException
* The OpsMetadata object doesn't exist.
* @throws OpsMetadataInvalidArgumentException
* One of the arguments passed is invalid.
* @throws OpsMetadataKeyLimitExceededException
* The OpsMetadata object exceeds the maximum number of OpsMetadata keys that you can assign to an
* application in Application Manager.
* @throws OpsMetadataTooManyUpdatesException
* The system is processing too many concurrent updates. Wait a few moments and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.UpdateOpsMetadata
* @see AWS API
* Documentation
*/
UpdateOpsMetadataResult updateOpsMetadata(UpdateOpsMetadataRequest updateOpsMetadataRequest);
/**
*
* Modifies an existing patch baseline. Fields not specified in the request are left unchanged.
*
*
*
* For information about valid key-value pairs in PatchFilters
for each supported operating system
* type, see PatchFilter.
*
*
*
* @param updatePatchBaselineRequest
* @return Result of the UpdatePatchBaseline operation returned by the service.
* @throws DoesNotExistException
* Error returned when the ID specified for a resource, such as a maintenance window or patch baseline,
* doesn't exist.
*
* For information about resource quotas in Amazon Web Services Systems Manager, see Systems Manager service
* quotas in the Amazon Web Services General Reference.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.UpdatePatchBaseline
* @see AWS API
* Documentation
*/
UpdatePatchBaselineResult updatePatchBaseline(UpdatePatchBaselineRequest updatePatchBaselineRequest);
/**
*
* Update a resource data sync. After you create a resource data sync for a Region, you can't change the account
* options for that sync. For example, if you create a sync in the us-east-2 (Ohio) Region and you choose the
* Include only the current account
option, you can't edit that sync later and choose the
* Include all accounts from my Organizations configuration
option. Instead, you must delete the first
* resource data sync, and create a new one.
*
*
*
* This API operation only supports a resource data sync that was created with a SyncFromSource
* SyncType
.
*
*
*
* @param updateResourceDataSyncRequest
* @return Result of the UpdateResourceDataSync operation returned by the service.
* @throws ResourceDataSyncNotFoundException
* The specified sync name wasn't found.
* @throws ResourceDataSyncInvalidConfigurationException
* The specified sync configuration is invalid.
* @throws ResourceDataSyncConflictException
* Another UpdateResourceDataSync
request is being processed. Wait a few minutes and try again.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @sample AWSSimpleSystemsManagement.UpdateResourceDataSync
* @see AWS API
* Documentation
*/
UpdateResourceDataSyncResult updateResourceDataSync(UpdateResourceDataSyncRequest updateResourceDataSyncRequest);
/**
*
* ServiceSetting
is an account-level setting for an Amazon Web Services service. This setting defines
* how a user interacts with or uses a service or a feature of a service. For example, if an Amazon Web Services
* service charges money to the account based on feature or service usage, then the Amazon Web Services service team
* might create a default setting of "false". This means the user can't use this feature unless they change the
* setting to "true" and intentionally opt in for a paid feature.
*
*
* Services map a SettingId
object to a setting value. Amazon Web Services services teams define the
* default value for a SettingId
. You can't create a new SettingId
, but you can overwrite
* the default value if you have the ssm:UpdateServiceSetting
permission for the setting. Use the
* GetServiceSetting API operation to view the current value. Or, use the ResetServiceSetting to
* change the value back to the original value defined by the Amazon Web Services service team.
*
*
* Update the service setting for the account.
*
*
* @param updateServiceSettingRequest
* The request body of the UpdateServiceSetting API operation.
* @return Result of the UpdateServiceSetting operation returned by the service.
* @throws InternalServerErrorException
* An error occurred on the server side.
* @throws ServiceSettingNotFoundException
* The specified service setting wasn't found. Either the service name or the setting hasn't been
* provisioned by the Amazon Web Services service team.
* @throws TooManyUpdatesException
* There are concurrent updates for a resource that supports one update at a time.
* @sample AWSSimpleSystemsManagement.UpdateServiceSetting
* @see AWS API
* Documentation
*/
UpdateServiceSettingResult updateServiceSetting(UpdateServiceSettingRequest updateServiceSettingRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AWSSimpleSystemsManagementWaiters waiters();
}