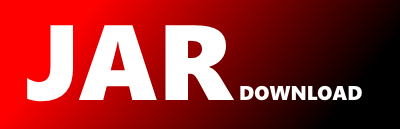
com.amazonaws.services.simplesystemsmanagement.AWSSimpleSystemsManagementAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement;
import javax.annotation.Generated;
import com.amazonaws.services.simplesystemsmanagement.model.*;
/**
* Interface for accessing Amazon SSM asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.simplesystemsmanagement.AbstractAWSSimpleSystemsManagementAsync} instead.
*
*
*
* Amazon Web Services Systems Manager is the operations hub for your Amazon Web Services applications and resources and
* a secure end-to-end management solution for hybrid cloud environments that enables safe and secure operations at
* scale.
*
*
* This reference is intended to be used with the Amazon Web Services Systems Manager User
* Guide. To get started, see Setting up Amazon
* Web Services Systems Manager.
*
*
* Related resources
*
*
* -
*
* For information about each of the capabilities that comprise Systems Manager, see Systems Manager capabilities in the Amazon Web Services Systems Manager User Guide.
*
*
* -
*
* For details about predefined runbooks for Automation, a capability of Amazon Web Services Systems Manager, see the
* Systems Manager Automation runbook reference .
*
*
* -
*
* For information about AppConfig, a capability of Systems Manager, see the AppConfig User Guide and the AppConfig API Reference .
*
*
* -
*
* For information about Incident Manager, a capability of Systems Manager, see the Systems Manager Incident Manager User Guide
* and the Systems Manager Incident
* Manager API Reference .
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSSimpleSystemsManagementAsync extends AWSSimpleSystemsManagement {
/**
*
* Adds or overwrites one or more tags for the specified resource. Tags are metadata that you can assign to
* your automations, documents, managed nodes, maintenance windows, Parameter Store parameters, and patch baselines.
* Tags enable you to categorize your resources in different ways, for example, by purpose, owner, or environment.
* Each tag consists of a key and an optional value, both of which you define. For example, you could define a set
* of tags for your account's managed nodes that helps you track each node's owner and stack level. For example:
*
*
* -
*
* Key=Owner,Value=DbAdmin
*
*
* -
*
* Key=Owner,Value=SysAdmin
*
*
* -
*
* Key=Owner,Value=Dev
*
*
* -
*
* Key=Stack,Value=Production
*
*
* -
*
* Key=Stack,Value=Pre-Production
*
*
* -
*
* Key=Stack,Value=Test
*
*
*
*
* Most resources can have a maximum of 50 tags. Automations can have a maximum of 5 tags.
*
*
* We recommend that you devise a set of tag keys that meets your needs for each resource type. Using a consistent
* set of tag keys makes it easier for you to manage your resources. You can search and filter the resources based
* on the tags you add. Tags don't have any semantic meaning to and are interpreted strictly as a string of
* characters.
*
*
* For more information about using tags with Amazon Elastic Compute Cloud (Amazon EC2) instances, see Tag your Amazon EC2 resources in
* the Amazon EC2 User Guide.
*
*
* @param addTagsToResourceRequest
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Adds or overwrites one or more tags for the specified resource. Tags are metadata that you can assign to
* your automations, documents, managed nodes, maintenance windows, Parameter Store parameters, and patch baselines.
* Tags enable you to categorize your resources in different ways, for example, by purpose, owner, or environment.
* Each tag consists of a key and an optional value, both of which you define. For example, you could define a set
* of tags for your account's managed nodes that helps you track each node's owner and stack level. For example:
*
*
* -
*
* Key=Owner,Value=DbAdmin
*
*
* -
*
* Key=Owner,Value=SysAdmin
*
*
* -
*
* Key=Owner,Value=Dev
*
*
* -
*
* Key=Stack,Value=Production
*
*
* -
*
* Key=Stack,Value=Pre-Production
*
*
* -
*
* Key=Stack,Value=Test
*
*
*
*
* Most resources can have a maximum of 50 tags. Automations can have a maximum of 5 tags.
*
*
* We recommend that you devise a set of tag keys that meets your needs for each resource type. Using a consistent
* set of tag keys makes it easier for you to manage your resources. You can search and filter the resources based
* on the tags you add. Tags don't have any semantic meaning to and are interpreted strictly as a string of
* characters.
*
*
* For more information about using tags with Amazon Elastic Compute Cloud (Amazon EC2) instances, see Tag your Amazon EC2 resources in
* the Amazon EC2 User Guide.
*
*
* @param addTagsToResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a related item to a Systems Manager OpsCenter OpsItem. For example, you can associate an Incident
* Manager incident or analysis with an OpsItem. Incident Manager and OpsCenter are capabilities of Amazon Web
* Services Systems Manager.
*
*
* @param associateOpsItemRelatedItemRequest
* @return A Java Future containing the result of the AssociateOpsItemRelatedItem operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.AssociateOpsItemRelatedItem
* @see AWS API Documentation
*/
java.util.concurrent.Future associateOpsItemRelatedItemAsync(
AssociateOpsItemRelatedItemRequest associateOpsItemRelatedItemRequest);
/**
*
* Associates a related item to a Systems Manager OpsCenter OpsItem. For example, you can associate an Incident
* Manager incident or analysis with an OpsItem. Incident Manager and OpsCenter are capabilities of Amazon Web
* Services Systems Manager.
*
*
* @param associateOpsItemRelatedItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateOpsItemRelatedItem operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.AssociateOpsItemRelatedItem
* @see AWS API Documentation
*/
java.util.concurrent.Future associateOpsItemRelatedItemAsync(
AssociateOpsItemRelatedItemRequest associateOpsItemRelatedItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attempts to cancel the command specified by the Command ID. There is no guarantee that the command will be
* terminated and the underlying process stopped.
*
*
* @param cancelCommandRequest
* @return A Java Future containing the result of the CancelCommand operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CancelCommand
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelCommandAsync(CancelCommandRequest cancelCommandRequest);
/**
*
* Attempts to cancel the command specified by the Command ID. There is no guarantee that the command will be
* terminated and the underlying process stopped.
*
*
* @param cancelCommandRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelCommand operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CancelCommand
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelCommandAsync(CancelCommandRequest cancelCommandRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a maintenance window execution that is already in progress and cancels any tasks in the window that haven't
* already starting running. Tasks already in progress will continue to completion.
*
*
* @param cancelMaintenanceWindowExecutionRequest
* @return A Java Future containing the result of the CancelMaintenanceWindowExecution operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.CancelMaintenanceWindowExecution
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelMaintenanceWindowExecutionAsync(
CancelMaintenanceWindowExecutionRequest cancelMaintenanceWindowExecutionRequest);
/**
*
* Stops a maintenance window execution that is already in progress and cancels any tasks in the window that haven't
* already starting running. Tasks already in progress will continue to completion.
*
*
* @param cancelMaintenanceWindowExecutionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelMaintenanceWindowExecution operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CancelMaintenanceWindowExecution
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelMaintenanceWindowExecutionAsync(
CancelMaintenanceWindowExecutionRequest cancelMaintenanceWindowExecutionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates an activation code and activation ID you can use to register your on-premises servers, edge devices, or
* virtual machine (VM) with Amazon Web Services Systems Manager. Registering these machines with Systems Manager
* makes it possible to manage them using Systems Manager capabilities. You use the activation code and ID when
* installing SSM Agent on machines in your hybrid environment. For more information about requirements for managing
* on-premises machines using Systems Manager, see Setting
* up Amazon Web Services Systems Manager for hybrid and multicloud environments in the Amazon Web Services
* Systems Manager User Guide.
*
*
*
* Amazon Elastic Compute Cloud (Amazon EC2) instances, edge devices, and on-premises servers and VMs that are
* configured for Systems Manager are all called managed nodes.
*
*
*
* @param createActivationRequest
* @return A Java Future containing the result of the CreateActivation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CreateActivation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createActivationAsync(CreateActivationRequest createActivationRequest);
/**
*
* Generates an activation code and activation ID you can use to register your on-premises servers, edge devices, or
* virtual machine (VM) with Amazon Web Services Systems Manager. Registering these machines with Systems Manager
* makes it possible to manage them using Systems Manager capabilities. You use the activation code and ID when
* installing SSM Agent on machines in your hybrid environment. For more information about requirements for managing
* on-premises machines using Systems Manager, see Setting
* up Amazon Web Services Systems Manager for hybrid and multicloud environments in the Amazon Web Services
* Systems Manager User Guide.
*
*
*
* Amazon Elastic Compute Cloud (Amazon EC2) instances, edge devices, and on-premises servers and VMs that are
* configured for Systems Manager are all called managed nodes.
*
*
*
* @param createActivationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateActivation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CreateActivation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createActivationAsync(CreateActivationRequest createActivationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A State Manager association defines the state that you want to maintain on your managed nodes. For example, an
* association can specify that anti-virus software must be installed and running on your managed nodes, or that
* certain ports must be closed. For static targets, the association specifies a schedule for when the configuration
* is reapplied. For dynamic targets, such as an Amazon Web Services resource group or an Amazon Web Services
* autoscaling group, State Manager, a capability of Amazon Web Services Systems Manager applies the configuration
* when new managed nodes are added to the group. The association also specifies actions to take when applying the
* configuration. For example, an association for anti-virus software might run once a day. If the software isn't
* installed, then State Manager installs it. If the software is installed, but the service isn't running, then the
* association might instruct State Manager to start the service.
*
*
* @param createAssociationRequest
* @return A Java Future containing the result of the CreateAssociation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CreateAssociation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssociationAsync(CreateAssociationRequest createAssociationRequest);
/**
*
* A State Manager association defines the state that you want to maintain on your managed nodes. For example, an
* association can specify that anti-virus software must be installed and running on your managed nodes, or that
* certain ports must be closed. For static targets, the association specifies a schedule for when the configuration
* is reapplied. For dynamic targets, such as an Amazon Web Services resource group or an Amazon Web Services
* autoscaling group, State Manager, a capability of Amazon Web Services Systems Manager applies the configuration
* when new managed nodes are added to the group. The association also specifies actions to take when applying the
* configuration. For example, an association for anti-virus software might run once a day. If the software isn't
* installed, then State Manager installs it. If the software is installed, but the service isn't running, then the
* association might instruct State Manager to start the service.
*
*
* @param createAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssociation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CreateAssociation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssociationAsync(CreateAssociationRequest createAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified Amazon Web Services Systems Manager document (SSM document) with the specified managed
* nodes or targets.
*
*
* When you associate a document with one or more managed nodes using IDs or tags, Amazon Web Services Systems
* Manager Agent (SSM Agent) running on the managed node processes the document and configures the node as
* specified.
*
*
* If you associate a document with a managed node that already has an associated document, the system returns the
* AssociationAlreadyExists exception.
*
*
* @param createAssociationBatchRequest
* @return A Java Future containing the result of the CreateAssociationBatch operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CreateAssociationBatch
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssociationBatchAsync(CreateAssociationBatchRequest createAssociationBatchRequest);
/**
*
* Associates the specified Amazon Web Services Systems Manager document (SSM document) with the specified managed
* nodes or targets.
*
*
* When you associate a document with one or more managed nodes using IDs or tags, Amazon Web Services Systems
* Manager Agent (SSM Agent) running on the managed node processes the document and configures the node as
* specified.
*
*
* If you associate a document with a managed node that already has an associated document, the system returns the
* AssociationAlreadyExists exception.
*
*
* @param createAssociationBatchRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssociationBatch operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CreateAssociationBatch
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssociationBatchAsync(CreateAssociationBatchRequest createAssociationBatchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Amazon Web Services Systems Manager (SSM document). An SSM document defines the actions that Systems
* Manager performs on your managed nodes. For more information about SSM documents, including information about
* supported schemas, features, and syntax, see Amazon Web Services
* Systems Manager Documents in the Amazon Web Services Systems Manager User Guide.
*
*
* @param createDocumentRequest
* @return A Java Future containing the result of the CreateDocument operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CreateDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDocumentAsync(CreateDocumentRequest createDocumentRequest);
/**
*
* Creates a Amazon Web Services Systems Manager (SSM document). An SSM document defines the actions that Systems
* Manager performs on your managed nodes. For more information about SSM documents, including information about
* supported schemas, features, and syntax, see Amazon Web Services
* Systems Manager Documents in the Amazon Web Services Systems Manager User Guide.
*
*
* @param createDocumentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDocument operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CreateDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDocumentAsync(CreateDocumentRequest createDocumentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new maintenance window.
*
*
*
* The value you specify for Duration
determines the specific end time for the maintenance window based
* on the time it begins. No maintenance window tasks are permitted to start after the resulting endtime minus the
* number of hours you specify for Cutoff
. For example, if the maintenance window starts at 3 PM, the
* duration is three hours, and the value you specify for Cutoff
is one hour, no maintenance window
* tasks can start after 5 PM.
*
*
*
* @param createMaintenanceWindowRequest
* @return A Java Future containing the result of the CreateMaintenanceWindow operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CreateMaintenanceWindow
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createMaintenanceWindowAsync(CreateMaintenanceWindowRequest createMaintenanceWindowRequest);
/**
*
* Creates a new maintenance window.
*
*
*
* The value you specify for Duration
determines the specific end time for the maintenance window based
* on the time it begins. No maintenance window tasks are permitted to start after the resulting endtime minus the
* number of hours you specify for Cutoff
. For example, if the maintenance window starts at 3 PM, the
* duration is three hours, and the value you specify for Cutoff
is one hour, no maintenance window
* tasks can start after 5 PM.
*
*
*
* @param createMaintenanceWindowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMaintenanceWindow operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CreateMaintenanceWindow
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createMaintenanceWindowAsync(CreateMaintenanceWindowRequest createMaintenanceWindowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new OpsItem. You must have permission in Identity and Access Management (IAM) to create a new OpsItem.
* For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param createOpsItemRequest
* @return A Java Future containing the result of the CreateOpsItem operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CreateOpsItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOpsItemAsync(CreateOpsItemRequest createOpsItemRequest);
/**
*
* Creates a new OpsItem. You must have permission in Identity and Access Management (IAM) to create a new OpsItem.
* For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param createOpsItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateOpsItem operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CreateOpsItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOpsItemAsync(CreateOpsItemRequest createOpsItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* If you create a new application in Application Manager, Amazon Web Services Systems Manager calls this API
* operation to specify information about the new application, including the application type.
*
*
* @param createOpsMetadataRequest
* @return A Java Future containing the result of the CreateOpsMetadata operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CreateOpsMetadata
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOpsMetadataAsync(CreateOpsMetadataRequest createOpsMetadataRequest);
/**
*
* If you create a new application in Application Manager, Amazon Web Services Systems Manager calls this API
* operation to specify information about the new application, including the application type.
*
*
* @param createOpsMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateOpsMetadata operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CreateOpsMetadata
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOpsMetadataAsync(CreateOpsMetadataRequest createOpsMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a patch baseline.
*
*
*
* For information about valid key-value pairs in PatchFilters
for each supported operating system
* type, see PatchFilter.
*
*
*
* @param createPatchBaselineRequest
* @return A Java Future containing the result of the CreatePatchBaseline operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CreatePatchBaseline
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPatchBaselineAsync(CreatePatchBaselineRequest createPatchBaselineRequest);
/**
*
* Creates a patch baseline.
*
*
*
* For information about valid key-value pairs in PatchFilters
for each supported operating system
* type, see PatchFilter.
*
*
*
* @param createPatchBaselineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePatchBaseline operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CreatePatchBaseline
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPatchBaselineAsync(CreatePatchBaselineRequest createPatchBaselineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A resource data sync helps you view data from multiple sources in a single location. Amazon Web Services Systems
* Manager offers two types of resource data sync: SyncToDestination
and SyncFromSource
.
*
*
* You can configure Systems Manager Inventory to use the SyncToDestination
type to synchronize
* Inventory data from multiple Amazon Web Services Regions to a single Amazon Simple Storage Service (Amazon S3)
* bucket. For more information, see Configuring
* resource data sync for Inventory in the Amazon Web Services Systems Manager User Guide.
*
*
* You can configure Systems Manager Explorer to use the SyncFromSource
type to synchronize operational
* work items (OpsItems) and operational data (OpsData) from multiple Amazon Web Services Regions to a single Amazon
* S3 bucket. This type can synchronize OpsItems and OpsData from multiple Amazon Web Services accounts and Amazon
* Web Services Regions or EntireOrganization
by using Organizations. For more information, see Setting up
* Systems Manager Explorer to display data from multiple accounts and Regions in the Amazon Web Services
* Systems Manager User Guide.
*
*
* A resource data sync is an asynchronous operation that returns immediately. After a successful initial sync is
* completed, the system continuously syncs data. To check the status of a sync, use the
* ListResourceDataSync.
*
*
*
* By default, data isn't encrypted in Amazon S3. We strongly recommend that you enable encryption in Amazon S3 to
* ensure secure data storage. We also recommend that you secure access to the Amazon S3 bucket by creating a
* restrictive bucket policy.
*
*
*
* @param createResourceDataSyncRequest
* @return A Java Future containing the result of the CreateResourceDataSync operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.CreateResourceDataSync
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createResourceDataSyncAsync(CreateResourceDataSyncRequest createResourceDataSyncRequest);
/**
*
* A resource data sync helps you view data from multiple sources in a single location. Amazon Web Services Systems
* Manager offers two types of resource data sync: SyncToDestination
and SyncFromSource
.
*
*
* You can configure Systems Manager Inventory to use the SyncToDestination
type to synchronize
* Inventory data from multiple Amazon Web Services Regions to a single Amazon Simple Storage Service (Amazon S3)
* bucket. For more information, see Configuring
* resource data sync for Inventory in the Amazon Web Services Systems Manager User Guide.
*
*
* You can configure Systems Manager Explorer to use the SyncFromSource
type to synchronize operational
* work items (OpsItems) and operational data (OpsData) from multiple Amazon Web Services Regions to a single Amazon
* S3 bucket. This type can synchronize OpsItems and OpsData from multiple Amazon Web Services accounts and Amazon
* Web Services Regions or EntireOrganization
by using Organizations. For more information, see Setting up
* Systems Manager Explorer to display data from multiple accounts and Regions in the Amazon Web Services
* Systems Manager User Guide.
*
*
* A resource data sync is an asynchronous operation that returns immediately. After a successful initial sync is
* completed, the system continuously syncs data. To check the status of a sync, use the
* ListResourceDataSync.
*
*
*
* By default, data isn't encrypted in Amazon S3. We strongly recommend that you enable encryption in Amazon S3 to
* ensure secure data storage. We also recommend that you secure access to the Amazon S3 bucket by creating a
* restrictive bucket policy.
*
*
*
* @param createResourceDataSyncRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateResourceDataSync operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.CreateResourceDataSync
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createResourceDataSyncAsync(CreateResourceDataSyncRequest createResourceDataSyncRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an activation. You aren't required to delete an activation. If you delete an activation, you can no
* longer use it to register additional managed nodes. Deleting an activation doesn't de-register managed nodes. You
* must manually de-register managed nodes.
*
*
* @param deleteActivationRequest
* @return A Java Future containing the result of the DeleteActivation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteActivation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteActivationAsync(DeleteActivationRequest deleteActivationRequest);
/**
*
* Deletes an activation. You aren't required to delete an activation. If you delete an activation, you can no
* longer use it to register additional managed nodes. Deleting an activation doesn't de-register managed nodes. You
* must manually de-register managed nodes.
*
*
* @param deleteActivationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteActivation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteActivation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteActivationAsync(DeleteActivationRequest deleteActivationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified Amazon Web Services Systems Manager document (SSM document) from the specified
* managed node. If you created the association by using the Targets
parameter, then you must delete
* the association by using the association ID.
*
*
* When you disassociate a document from a managed node, it doesn't change the configuration of the node. To change
* the configuration state of a managed node after you disassociate a document, you must create a new document with
* the desired configuration and associate it with the node.
*
*
* @param deleteAssociationRequest
* @return A Java Future containing the result of the DeleteAssociation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteAssociation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssociationAsync(DeleteAssociationRequest deleteAssociationRequest);
/**
*
* Disassociates the specified Amazon Web Services Systems Manager document (SSM document) from the specified
* managed node. If you created the association by using the Targets
parameter, then you must delete
* the association by using the association ID.
*
*
* When you disassociate a document from a managed node, it doesn't change the configuration of the node. To change
* the configuration state of a managed node after you disassociate a document, you must create a new document with
* the desired configuration and associate it with the node.
*
*
* @param deleteAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAssociation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteAssociation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssociationAsync(DeleteAssociationRequest deleteAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the Amazon Web Services Systems Manager document (SSM document) and all managed node associations to the
* document.
*
*
* Before you delete the document, we recommend that you use DeleteAssociation to disassociate all managed
* nodes that are associated with the document.
*
*
* @param deleteDocumentRequest
* @return A Java Future containing the result of the DeleteDocument operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDocumentAsync(DeleteDocumentRequest deleteDocumentRequest);
/**
*
* Deletes the Amazon Web Services Systems Manager document (SSM document) and all managed node associations to the
* document.
*
*
* Before you delete the document, we recommend that you use DeleteAssociation to disassociate all managed
* nodes that are associated with the document.
*
*
* @param deleteDocumentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDocument operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDocumentAsync(DeleteDocumentRequest deleteDocumentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a custom inventory type or the data associated with a custom Inventory type. Deleting a custom inventory
* type is also referred to as deleting a custom inventory schema.
*
*
* @param deleteInventoryRequest
* @return A Java Future containing the result of the DeleteInventory operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteInventory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteInventoryAsync(DeleteInventoryRequest deleteInventoryRequest);
/**
*
* Delete a custom inventory type or the data associated with a custom Inventory type. Deleting a custom inventory
* type is also referred to as deleting a custom inventory schema.
*
*
* @param deleteInventoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteInventory operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteInventory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteInventoryAsync(DeleteInventoryRequest deleteInventoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a maintenance window.
*
*
* @param deleteMaintenanceWindowRequest
* @return A Java Future containing the result of the DeleteMaintenanceWindow operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteMaintenanceWindow
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteMaintenanceWindowAsync(DeleteMaintenanceWindowRequest deleteMaintenanceWindowRequest);
/**
*
* Deletes a maintenance window.
*
*
* @param deleteMaintenanceWindowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMaintenanceWindow operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteMaintenanceWindow
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteMaintenanceWindowAsync(DeleteMaintenanceWindowRequest deleteMaintenanceWindowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an OpsItem. You must have permission in Identity and Access Management (IAM) to delete an OpsItem.
*
*
*
* Note the following important information about this operation.
*
*
* -
*
* Deleting an OpsItem is irreversible. You can't restore a deleted OpsItem.
*
*
* -
*
* This operation uses an eventual consistency model, which means the system can take a few minutes to
* complete this operation. If you delete an OpsItem and immediately call, for example, GetOpsItem, the
* deleted OpsItem might still appear in the response.
*
*
* -
*
* This operation is idempotent. The system doesn't throw an exception if you repeatedly call this operation for the
* same OpsItem. If the first call is successful, all additional calls return the same successful response as the
* first call.
*
*
* -
*
* This operation doesn't support cross-account calls. A delegated administrator or management account can't delete
* OpsItems in other accounts, even if OpsCenter has been set up for cross-account administration. For more
* information about cross-account administration, see Setting up OpsCenter to centrally manage OpsItems across accounts in the Systems Manager User Guide.
*
*
*
*
*
* @param deleteOpsItemRequest
* @return A Java Future containing the result of the DeleteOpsItem operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteOpsItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteOpsItemAsync(DeleteOpsItemRequest deleteOpsItemRequest);
/**
*
* Delete an OpsItem. You must have permission in Identity and Access Management (IAM) to delete an OpsItem.
*
*
*
* Note the following important information about this operation.
*
*
* -
*
* Deleting an OpsItem is irreversible. You can't restore a deleted OpsItem.
*
*
* -
*
* This operation uses an eventual consistency model, which means the system can take a few minutes to
* complete this operation. If you delete an OpsItem and immediately call, for example, GetOpsItem, the
* deleted OpsItem might still appear in the response.
*
*
* -
*
* This operation is idempotent. The system doesn't throw an exception if you repeatedly call this operation for the
* same OpsItem. If the first call is successful, all additional calls return the same successful response as the
* first call.
*
*
* -
*
* This operation doesn't support cross-account calls. A delegated administrator or management account can't delete
* OpsItems in other accounts, even if OpsCenter has been set up for cross-account administration. For more
* information about cross-account administration, see Setting up OpsCenter to centrally manage OpsItems across accounts in the Systems Manager User Guide.
*
*
*
*
*
* @param deleteOpsItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOpsItem operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteOpsItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteOpsItemAsync(DeleteOpsItemRequest deleteOpsItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete OpsMetadata related to an application.
*
*
* @param deleteOpsMetadataRequest
* @return A Java Future containing the result of the DeleteOpsMetadata operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteOpsMetadata
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteOpsMetadataAsync(DeleteOpsMetadataRequest deleteOpsMetadataRequest);
/**
*
* Delete OpsMetadata related to an application.
*
*
* @param deleteOpsMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOpsMetadata operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteOpsMetadata
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteOpsMetadataAsync(DeleteOpsMetadataRequest deleteOpsMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a parameter from the system. After deleting a parameter, wait for at least 30 seconds to create a
* parameter with the same name.
*
*
* @param deleteParameterRequest
* @return A Java Future containing the result of the DeleteParameter operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteParameter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteParameterAsync(DeleteParameterRequest deleteParameterRequest);
/**
*
* Delete a parameter from the system. After deleting a parameter, wait for at least 30 seconds to create a
* parameter with the same name.
*
*
* @param deleteParameterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteParameter operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteParameter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteParameterAsync(DeleteParameterRequest deleteParameterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a list of parameters. After deleting a parameter, wait for at least 30 seconds to create a parameter with
* the same name.
*
*
* @param deleteParametersRequest
* @return A Java Future containing the result of the DeleteParameters operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteParameters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteParametersAsync(DeleteParametersRequest deleteParametersRequest);
/**
*
* Delete a list of parameters. After deleting a parameter, wait for at least 30 seconds to create a parameter with
* the same name.
*
*
* @param deleteParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteParameters operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteParameters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteParametersAsync(DeleteParametersRequest deleteParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a patch baseline.
*
*
* @param deletePatchBaselineRequest
* @return A Java Future containing the result of the DeletePatchBaseline operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeletePatchBaseline
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePatchBaselineAsync(DeletePatchBaselineRequest deletePatchBaselineRequest);
/**
*
* Deletes a patch baseline.
*
*
* @param deletePatchBaselineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePatchBaseline operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeletePatchBaseline
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePatchBaselineAsync(DeletePatchBaselineRequest deletePatchBaselineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a resource data sync configuration. After the configuration is deleted, changes to data on managed nodes
* are no longer synced to or from the target. Deleting a sync configuration doesn't delete data.
*
*
* @param deleteResourceDataSyncRequest
* @return A Java Future containing the result of the DeleteResourceDataSync operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteResourceDataSync
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteResourceDataSyncAsync(DeleteResourceDataSyncRequest deleteResourceDataSyncRequest);
/**
*
* Deletes a resource data sync configuration. After the configuration is deleted, changes to data on managed nodes
* are no longer synced to or from the target. Deleting a sync configuration doesn't delete data.
*
*
* @param deleteResourceDataSyncRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteResourceDataSync operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteResourceDataSync
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteResourceDataSyncAsync(DeleteResourceDataSyncRequest deleteResourceDataSyncRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Systems Manager resource policy. A resource policy helps you to define the IAM entity (for example, an
* Amazon Web Services account) that can manage your Systems Manager resources. The following resources support
* Systems Manager resource policies.
*
*
* -
*
* OpsItemGroup
- The resource policy for OpsItemGroup
enables Amazon Web Services
* accounts to view and interact with OpsCenter operational work items (OpsItems).
*
*
* -
*
* Parameter
- The resource policy is used to share a parameter with other accounts using Resource
* Access Manager (RAM). For more information about cross-account sharing of parameters, see Working with shared parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
*
* @param deleteResourcePolicyRequest
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeleteResourcePolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteResourcePolicyAsync(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Deletes a Systems Manager resource policy. A resource policy helps you to define the IAM entity (for example, an
* Amazon Web Services account) that can manage your Systems Manager resources. The following resources support
* Systems Manager resource policies.
*
*
* -
*
* OpsItemGroup
- The resource policy for OpsItemGroup
enables Amazon Web Services
* accounts to view and interact with OpsCenter operational work items (OpsItems).
*
*
* -
*
* Parameter
- The resource policy is used to share a parameter with other accounts using Resource
* Access Manager (RAM). For more information about cross-account sharing of parameters, see Working with shared parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
*
* @param deleteResourcePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeleteResourcePolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteResourcePolicyAsync(DeleteResourcePolicyRequest deleteResourcePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the server or virtual machine from the list of registered servers. You can reregister the node again at
* any time. If you don't plan to use Run Command on the server, we suggest uninstalling SSM Agent first.
*
*
* @param deregisterManagedInstanceRequest
* @return A Java Future containing the result of the DeregisterManagedInstance operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DeregisterManagedInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deregisterManagedInstanceAsync(
DeregisterManagedInstanceRequest deregisterManagedInstanceRequest);
/**
*
* Removes the server or virtual machine from the list of registered servers. You can reregister the node again at
* any time. If you don't plan to use Run Command on the server, we suggest uninstalling SSM Agent first.
*
*
* @param deregisterManagedInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterManagedInstance operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeregisterManagedInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deregisterManagedInstanceAsync(
DeregisterManagedInstanceRequest deregisterManagedInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a patch group from a patch baseline.
*
*
* @param deregisterPatchBaselineForPatchGroupRequest
* @return A Java Future containing the result of the DeregisterPatchBaselineForPatchGroup operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DeregisterPatchBaselineForPatchGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterPatchBaselineForPatchGroupAsync(
DeregisterPatchBaselineForPatchGroupRequest deregisterPatchBaselineForPatchGroupRequest);
/**
*
* Removes a patch group from a patch baseline.
*
*
* @param deregisterPatchBaselineForPatchGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterPatchBaselineForPatchGroup operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeregisterPatchBaselineForPatchGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterPatchBaselineForPatchGroupAsync(
DeregisterPatchBaselineForPatchGroupRequest deregisterPatchBaselineForPatchGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a target from a maintenance window.
*
*
* @param deregisterTargetFromMaintenanceWindowRequest
* @return A Java Future containing the result of the DeregisterTargetFromMaintenanceWindow operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsync.DeregisterTargetFromMaintenanceWindow
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterTargetFromMaintenanceWindowAsync(
DeregisterTargetFromMaintenanceWindowRequest deregisterTargetFromMaintenanceWindowRequest);
/**
*
* Removes a target from a maintenance window.
*
*
* @param deregisterTargetFromMaintenanceWindowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterTargetFromMaintenanceWindow operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeregisterTargetFromMaintenanceWindow
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterTargetFromMaintenanceWindowAsync(
DeregisterTargetFromMaintenanceWindowRequest deregisterTargetFromMaintenanceWindowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a task from a maintenance window.
*
*
* @param deregisterTaskFromMaintenanceWindowRequest
* @return A Java Future containing the result of the DeregisterTaskFromMaintenanceWindow operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DeregisterTaskFromMaintenanceWindow
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterTaskFromMaintenanceWindowAsync(
DeregisterTaskFromMaintenanceWindowRequest deregisterTaskFromMaintenanceWindowRequest);
/**
*
* Removes a task from a maintenance window.
*
*
* @param deregisterTaskFromMaintenanceWindowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterTaskFromMaintenanceWindow operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DeregisterTaskFromMaintenanceWindow
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterTaskFromMaintenanceWindowAsync(
DeregisterTaskFromMaintenanceWindowRequest deregisterTaskFromMaintenanceWindowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes details about the activation, such as the date and time the activation was created, its expiration
* date, the Identity and Access Management (IAM) role assigned to the managed nodes in the activation, and the
* number of nodes registered by using this activation.
*
*
* @param describeActivationsRequest
* @return A Java Future containing the result of the DescribeActivations operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeActivations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeActivationsAsync(DescribeActivationsRequest describeActivationsRequest);
/**
*
* Describes details about the activation, such as the date and time the activation was created, its expiration
* date, the Identity and Access Management (IAM) role assigned to the managed nodes in the activation, and the
* number of nodes registered by using this activation.
*
*
* @param describeActivationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeActivations operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeActivations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeActivationsAsync(DescribeActivationsRequest describeActivationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the association for the specified target or managed node. If you created the association by using the
* Targets
parameter, then you must retrieve the association by using the association ID.
*
*
* @param describeAssociationRequest
* @return A Java Future containing the result of the DescribeAssociation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeAssociation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAssociationAsync(DescribeAssociationRequest describeAssociationRequest);
/**
*
* Describes the association for the specified target or managed node. If you created the association by using the
* Targets
parameter, then you must retrieve the association by using the association ID.
*
*
* @param describeAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAssociation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeAssociation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAssociationAsync(DescribeAssociationRequest describeAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Views information about a specific execution of a specific association.
*
*
* @param describeAssociationExecutionTargetsRequest
* @return A Java Future containing the result of the DescribeAssociationExecutionTargets operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeAssociationExecutionTargets
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAssociationExecutionTargetsAsync(
DescribeAssociationExecutionTargetsRequest describeAssociationExecutionTargetsRequest);
/**
*
* Views information about a specific execution of a specific association.
*
*
* @param describeAssociationExecutionTargetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAssociationExecutionTargets operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeAssociationExecutionTargets
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAssociationExecutionTargetsAsync(
DescribeAssociationExecutionTargetsRequest describeAssociationExecutionTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Views all executions for a specific association ID.
*
*
* @param describeAssociationExecutionsRequest
* @return A Java Future containing the result of the DescribeAssociationExecutions operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeAssociationExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAssociationExecutionsAsync(
DescribeAssociationExecutionsRequest describeAssociationExecutionsRequest);
/**
*
* Views all executions for a specific association ID.
*
*
* @param describeAssociationExecutionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAssociationExecutions operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeAssociationExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAssociationExecutionsAsync(
DescribeAssociationExecutionsRequest describeAssociationExecutionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides details about all active and terminated Automation executions.
*
*
* @param describeAutomationExecutionsRequest
* @return A Java Future containing the result of the DescribeAutomationExecutions operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeAutomationExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAutomationExecutionsAsync(
DescribeAutomationExecutionsRequest describeAutomationExecutionsRequest);
/**
*
* Provides details about all active and terminated Automation executions.
*
*
* @param describeAutomationExecutionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAutomationExecutions operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeAutomationExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAutomationExecutionsAsync(
DescribeAutomationExecutionsRequest describeAutomationExecutionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Information about all active and terminated step executions in an Automation workflow.
*
*
* @param describeAutomationStepExecutionsRequest
* @return A Java Future containing the result of the DescribeAutomationStepExecutions operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeAutomationStepExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAutomationStepExecutionsAsync(
DescribeAutomationStepExecutionsRequest describeAutomationStepExecutionsRequest);
/**
*
* Information about all active and terminated step executions in an Automation workflow.
*
*
* @param describeAutomationStepExecutionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAutomationStepExecutions operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeAutomationStepExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAutomationStepExecutionsAsync(
DescribeAutomationStepExecutionsRequest describeAutomationStepExecutionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all patches eligible to be included in a patch baseline.
*
*
*
* Currently, DescribeAvailablePatches
supports only the Amazon Linux 1, Amazon Linux 2, and Windows
* Server operating systems.
*
*
*
* @param describeAvailablePatchesRequest
* @return A Java Future containing the result of the DescribeAvailablePatches operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeAvailablePatches
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAvailablePatchesAsync(DescribeAvailablePatchesRequest describeAvailablePatchesRequest);
/**
*
* Lists all patches eligible to be included in a patch baseline.
*
*
*
* Currently, DescribeAvailablePatches
supports only the Amazon Linux 1, Amazon Linux 2, and Windows
* Server operating systems.
*
*
*
* @param describeAvailablePatchesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAvailablePatches operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeAvailablePatches
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAvailablePatchesAsync(DescribeAvailablePatchesRequest describeAvailablePatchesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified Amazon Web Services Systems Manager document (SSM document).
*
*
* @param describeDocumentRequest
* @return A Java Future containing the result of the DescribeDocument operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDocumentAsync(DescribeDocumentRequest describeDocumentRequest);
/**
*
* Describes the specified Amazon Web Services Systems Manager document (SSM document).
*
*
* @param describeDocumentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDocument operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDocumentAsync(DescribeDocumentRequest describeDocumentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the permissions for a Amazon Web Services Systems Manager document (SSM document). If you created the
* document, you are the owner. If a document is shared, it can either be shared privately (by specifying a user's
* Amazon Web Services account ID) or publicly (All).
*
*
* @param describeDocumentPermissionRequest
* @return A Java Future containing the result of the DescribeDocumentPermission operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeDocumentPermission
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDocumentPermissionAsync(
DescribeDocumentPermissionRequest describeDocumentPermissionRequest);
/**
*
* Describes the permissions for a Amazon Web Services Systems Manager document (SSM document). If you created the
* document, you are the owner. If a document is shared, it can either be shared privately (by specifying a user's
* Amazon Web Services account ID) or publicly (All).
*
*
* @param describeDocumentPermissionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDocumentPermission operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeDocumentPermission
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDocumentPermissionAsync(
DescribeDocumentPermissionRequest describeDocumentPermissionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* All associations for the managed nodes.
*
*
* @param describeEffectiveInstanceAssociationsRequest
* @return A Java Future containing the result of the DescribeEffectiveInstanceAssociations operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeEffectiveInstanceAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEffectiveInstanceAssociationsAsync(
DescribeEffectiveInstanceAssociationsRequest describeEffectiveInstanceAssociationsRequest);
/**
*
* All associations for the managed nodes.
*
*
* @param describeEffectiveInstanceAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEffectiveInstanceAssociations operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeEffectiveInstanceAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEffectiveInstanceAssociationsAsync(
DescribeEffectiveInstanceAssociationsRequest describeEffectiveInstanceAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the current effective patches (the patch and the approval state) for the specified patch baseline.
* Applies to patch baselines for Windows only.
*
*
* @param describeEffectivePatchesForPatchBaselineRequest
* @return A Java Future containing the result of the DescribeEffectivePatchesForPatchBaseline operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeEffectivePatchesForPatchBaseline
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEffectivePatchesForPatchBaselineAsync(
DescribeEffectivePatchesForPatchBaselineRequest describeEffectivePatchesForPatchBaselineRequest);
/**
*
* Retrieves the current effective patches (the patch and the approval state) for the specified patch baseline.
* Applies to patch baselines for Windows only.
*
*
* @param describeEffectivePatchesForPatchBaselineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEffectivePatchesForPatchBaseline operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeEffectivePatchesForPatchBaseline
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEffectivePatchesForPatchBaselineAsync(
DescribeEffectivePatchesForPatchBaselineRequest describeEffectivePatchesForPatchBaselineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The status of the associations for the managed nodes.
*
*
* @param describeInstanceAssociationsStatusRequest
* @return A Java Future containing the result of the DescribeInstanceAssociationsStatus operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeInstanceAssociationsStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInstanceAssociationsStatusAsync(
DescribeInstanceAssociationsStatusRequest describeInstanceAssociationsStatusRequest);
/**
*
* The status of the associations for the managed nodes.
*
*
* @param describeInstanceAssociationsStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInstanceAssociationsStatus operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeInstanceAssociationsStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInstanceAssociationsStatusAsync(
DescribeInstanceAssociationsStatusRequest describeInstanceAssociationsStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about one or more of your managed nodes, including the operating system platform, SSM Agent
* version, association status, and IP address. This operation does not return information for nodes that are either
* Stopped or Terminated.
*
*
* If you specify one or more node IDs, the operation returns information for those managed nodes. If you don't
* specify node IDs, it returns information for all your managed nodes. If you specify a node ID that isn't valid or
* a node that you don't own, you receive an error.
*
*
*
* The IamRole
field returned for this API operation is the Identity and Access Management (IAM) role
* assigned to on-premises managed nodes. This operation does not return the IAM role for EC2 instances.
*
*
*
* @param describeInstanceInformationRequest
* @return A Java Future containing the result of the DescribeInstanceInformation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeInstanceInformation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInstanceInformationAsync(
DescribeInstanceInformationRequest describeInstanceInformationRequest);
/**
*
* Provides information about one or more of your managed nodes, including the operating system platform, SSM Agent
* version, association status, and IP address. This operation does not return information for nodes that are either
* Stopped or Terminated.
*
*
* If you specify one or more node IDs, the operation returns information for those managed nodes. If you don't
* specify node IDs, it returns information for all your managed nodes. If you specify a node ID that isn't valid or
* a node that you don't own, you receive an error.
*
*
*
* The IamRole
field returned for this API operation is the Identity and Access Management (IAM) role
* assigned to on-premises managed nodes. This operation does not return the IAM role for EC2 instances.
*
*
*
* @param describeInstanceInformationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInstanceInformation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeInstanceInformation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInstanceInformationAsync(
DescribeInstanceInformationRequest describeInstanceInformationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the high-level patch state of one or more managed nodes.
*
*
* @param describeInstancePatchStatesRequest
* @return A Java Future containing the result of the DescribeInstancePatchStates operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeInstancePatchStates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInstancePatchStatesAsync(
DescribeInstancePatchStatesRequest describeInstancePatchStatesRequest);
/**
*
* Retrieves the high-level patch state of one or more managed nodes.
*
*
* @param describeInstancePatchStatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInstancePatchStates operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeInstancePatchStates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInstancePatchStatesAsync(
DescribeInstancePatchStatesRequest describeInstancePatchStatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the high-level patch state for the managed nodes in the specified patch group.
*
*
* @param describeInstancePatchStatesForPatchGroupRequest
* @return A Java Future containing the result of the DescribeInstancePatchStatesForPatchGroup operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeInstancePatchStatesForPatchGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInstancePatchStatesForPatchGroupAsync(
DescribeInstancePatchStatesForPatchGroupRequest describeInstancePatchStatesForPatchGroupRequest);
/**
*
* Retrieves the high-level patch state for the managed nodes in the specified patch group.
*
*
* @param describeInstancePatchStatesForPatchGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInstancePatchStatesForPatchGroup operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeInstancePatchStatesForPatchGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInstancePatchStatesForPatchGroupAsync(
DescribeInstancePatchStatesForPatchGroupRequest describeInstancePatchStatesForPatchGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the patches on the specified managed node and their state relative to the patch
* baseline being used for the node.
*
*
* @param describeInstancePatchesRequest
* @return A Java Future containing the result of the DescribeInstancePatches operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeInstancePatches
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeInstancePatchesAsync(DescribeInstancePatchesRequest describeInstancePatchesRequest);
/**
*
* Retrieves information about the patches on the specified managed node and their state relative to the patch
* baseline being used for the node.
*
*
* @param describeInstancePatchesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInstancePatches operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeInstancePatches
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeInstancePatchesAsync(DescribeInstancePatchesRequest describeInstancePatchesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* An API operation used by the Systems Manager console to display information about Systems Manager managed nodes.
*
*
* @param describeInstancePropertiesRequest
* @return A Java Future containing the result of the DescribeInstanceProperties operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeInstanceProperties
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeInstancePropertiesAsync(
DescribeInstancePropertiesRequest describeInstancePropertiesRequest);
/**
*
* An API operation used by the Systems Manager console to display information about Systems Manager managed nodes.
*
*
* @param describeInstancePropertiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInstanceProperties operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeInstanceProperties
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeInstancePropertiesAsync(
DescribeInstancePropertiesRequest describeInstancePropertiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a specific delete inventory operation.
*
*
* @param describeInventoryDeletionsRequest
* @return A Java Future containing the result of the DescribeInventoryDeletions operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeInventoryDeletions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeInventoryDeletionsAsync(
DescribeInventoryDeletionsRequest describeInventoryDeletionsRequest);
/**
*
* Describes a specific delete inventory operation.
*
*
* @param describeInventoryDeletionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInventoryDeletions operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeInventoryDeletions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeInventoryDeletionsAsync(
DescribeInventoryDeletionsRequest describeInventoryDeletionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the individual task executions (one per target) for a particular task run as part of a maintenance
* window execution.
*
*
* @param describeMaintenanceWindowExecutionTaskInvocationsRequest
* @return A Java Future containing the result of the DescribeMaintenanceWindowExecutionTaskInvocations operation
* returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeMaintenanceWindowExecutionTaskInvocations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowExecutionTaskInvocationsAsync(
DescribeMaintenanceWindowExecutionTaskInvocationsRequest describeMaintenanceWindowExecutionTaskInvocationsRequest);
/**
*
* Retrieves the individual task executions (one per target) for a particular task run as part of a maintenance
* window execution.
*
*
* @param describeMaintenanceWindowExecutionTaskInvocationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMaintenanceWindowExecutionTaskInvocations operation
* returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeMaintenanceWindowExecutionTaskInvocations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowExecutionTaskInvocationsAsync(
DescribeMaintenanceWindowExecutionTaskInvocationsRequest describeMaintenanceWindowExecutionTaskInvocationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* For a given maintenance window execution, lists the tasks that were run.
*
*
* @param describeMaintenanceWindowExecutionTasksRequest
* @return A Java Future containing the result of the DescribeMaintenanceWindowExecutionTasks operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeMaintenanceWindowExecutionTasks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowExecutionTasksAsync(
DescribeMaintenanceWindowExecutionTasksRequest describeMaintenanceWindowExecutionTasksRequest);
/**
*
* For a given maintenance window execution, lists the tasks that were run.
*
*
* @param describeMaintenanceWindowExecutionTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMaintenanceWindowExecutionTasks operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeMaintenanceWindowExecutionTasks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowExecutionTasksAsync(
DescribeMaintenanceWindowExecutionTasksRequest describeMaintenanceWindowExecutionTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the executions of a maintenance window. This includes information about when the maintenance window was
* scheduled to be active, and information about tasks registered and run with the maintenance window.
*
*
* @param describeMaintenanceWindowExecutionsRequest
* @return A Java Future containing the result of the DescribeMaintenanceWindowExecutions operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeMaintenanceWindowExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowExecutionsAsync(
DescribeMaintenanceWindowExecutionsRequest describeMaintenanceWindowExecutionsRequest);
/**
*
* Lists the executions of a maintenance window. This includes information about when the maintenance window was
* scheduled to be active, and information about tasks registered and run with the maintenance window.
*
*
* @param describeMaintenanceWindowExecutionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMaintenanceWindowExecutions operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeMaintenanceWindowExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowExecutionsAsync(
DescribeMaintenanceWindowExecutionsRequest describeMaintenanceWindowExecutionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about upcoming executions of a maintenance window.
*
*
* @param describeMaintenanceWindowScheduleRequest
* @return A Java Future containing the result of the DescribeMaintenanceWindowSchedule operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeMaintenanceWindowSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowScheduleAsync(
DescribeMaintenanceWindowScheduleRequest describeMaintenanceWindowScheduleRequest);
/**
*
* Retrieves information about upcoming executions of a maintenance window.
*
*
* @param describeMaintenanceWindowScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMaintenanceWindowSchedule operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeMaintenanceWindowSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowScheduleAsync(
DescribeMaintenanceWindowScheduleRequest describeMaintenanceWindowScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the targets registered with the maintenance window.
*
*
* @param describeMaintenanceWindowTargetsRequest
* @return A Java Future containing the result of the DescribeMaintenanceWindowTargets operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeMaintenanceWindowTargets
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowTargetsAsync(
DescribeMaintenanceWindowTargetsRequest describeMaintenanceWindowTargetsRequest);
/**
*
* Lists the targets registered with the maintenance window.
*
*
* @param describeMaintenanceWindowTargetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMaintenanceWindowTargets operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeMaintenanceWindowTargets
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowTargetsAsync(
DescribeMaintenanceWindowTargetsRequest describeMaintenanceWindowTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tasks in a maintenance window.
*
*
*
* For maintenance window tasks without a specified target, you can't supply values for --max-errors
* and --max-concurrency
. Instead, the system inserts a placeholder value of 1
, which may
* be reported in the response to this command. These values don't affect the running of your task and can be
* ignored.
*
*
*
* @param describeMaintenanceWindowTasksRequest
* @return A Java Future containing the result of the DescribeMaintenanceWindowTasks operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeMaintenanceWindowTasks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowTasksAsync(
DescribeMaintenanceWindowTasksRequest describeMaintenanceWindowTasksRequest);
/**
*
* Lists the tasks in a maintenance window.
*
*
*
* For maintenance window tasks without a specified target, you can't supply values for --max-errors
* and --max-concurrency
. Instead, the system inserts a placeholder value of 1
, which may
* be reported in the response to this command. These values don't affect the running of your task and can be
* ignored.
*
*
*
* @param describeMaintenanceWindowTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMaintenanceWindowTasks operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeMaintenanceWindowTasks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowTasksAsync(
DescribeMaintenanceWindowTasksRequest describeMaintenanceWindowTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the maintenance windows in an Amazon Web Services account.
*
*
* @param describeMaintenanceWindowsRequest
* @return A Java Future containing the result of the DescribeMaintenanceWindows operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeMaintenanceWindows
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowsAsync(
DescribeMaintenanceWindowsRequest describeMaintenanceWindowsRequest);
/**
*
* Retrieves the maintenance windows in an Amazon Web Services account.
*
*
* @param describeMaintenanceWindowsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMaintenanceWindows operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeMaintenanceWindows
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowsAsync(
DescribeMaintenanceWindowsRequest describeMaintenanceWindowsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the maintenance window targets or tasks that a managed node is associated with.
*
*
* @param describeMaintenanceWindowsForTargetRequest
* @return A Java Future containing the result of the DescribeMaintenanceWindowsForTarget operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DescribeMaintenanceWindowsForTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowsForTargetAsync(
DescribeMaintenanceWindowsForTargetRequest describeMaintenanceWindowsForTargetRequest);
/**
*
* Retrieves information about the maintenance window targets or tasks that a managed node is associated with.
*
*
* @param describeMaintenanceWindowsForTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMaintenanceWindowsForTarget operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeMaintenanceWindowsForTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceWindowsForTargetAsync(
DescribeMaintenanceWindowsForTargetRequest describeMaintenanceWindowsForTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Query a set of OpsItems. You must have permission in Identity and Access Management (IAM) to query a list of
* OpsItems. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param describeOpsItemsRequest
* @return A Java Future containing the result of the DescribeOpsItems operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeOpsItems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeOpsItemsAsync(DescribeOpsItemsRequest describeOpsItemsRequest);
/**
*
* Query a set of OpsItems. You must have permission in Identity and Access Management (IAM) to query a list of
* OpsItems. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param describeOpsItemsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOpsItems operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeOpsItems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeOpsItemsAsync(DescribeOpsItemsRequest describeOpsItemsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the parameters in your Amazon Web Services account or the parameters shared with you when you enable the Shared option.
*
*
* Request results are returned on a best-effort basis. If you specify MaxResults
in the request, the
* response includes information up to the limit specified. The number of items returned, however, can be between
* zero and the value of MaxResults
. If the service reaches an internal limit while processing the
* results, it stops the operation and returns the matching values up to that point and a NextToken
.
* You can specify the NextToken
in a subsequent call to get the next set of results.
*
*
*
* If you change the KMS key alias for the KMS key used to encrypt a parameter, then you must also update the key
* alias the parameter uses to reference KMS. Otherwise, DescribeParameters
retrieves whatever the
* original key alias was referencing.
*
*
*
* @param describeParametersRequest
* @return A Java Future containing the result of the DescribeParameters operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeParameters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeParametersAsync(DescribeParametersRequest describeParametersRequest);
/**
*
* Lists the parameters in your Amazon Web Services account or the parameters shared with you when you enable the Shared option.
*
*
* Request results are returned on a best-effort basis. If you specify MaxResults
in the request, the
* response includes information up to the limit specified. The number of items returned, however, can be between
* zero and the value of MaxResults
. If the service reaches an internal limit while processing the
* results, it stops the operation and returns the matching values up to that point and a NextToken
.
* You can specify the NextToken
in a subsequent call to get the next set of results.
*
*
*
* If you change the KMS key alias for the KMS key used to encrypt a parameter, then you must also update the key
* alias the parameter uses to reference KMS. Otherwise, DescribeParameters
retrieves whatever the
* original key alias was referencing.
*
*
*
* @param describeParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeParameters operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeParameters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeParametersAsync(DescribeParametersRequest describeParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the patch baselines in your Amazon Web Services account.
*
*
* @param describePatchBaselinesRequest
* @return A Java Future containing the result of the DescribePatchBaselines operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribePatchBaselines
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describePatchBaselinesAsync(DescribePatchBaselinesRequest describePatchBaselinesRequest);
/**
*
* Lists the patch baselines in your Amazon Web Services account.
*
*
* @param describePatchBaselinesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePatchBaselines operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribePatchBaselines
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describePatchBaselinesAsync(DescribePatchBaselinesRequest describePatchBaselinesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns high-level aggregated patch compliance state information for a patch group.
*
*
* @param describePatchGroupStateRequest
* @return A Java Future containing the result of the DescribePatchGroupState operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribePatchGroupState
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describePatchGroupStateAsync(DescribePatchGroupStateRequest describePatchGroupStateRequest);
/**
*
* Returns high-level aggregated patch compliance state information for a patch group.
*
*
* @param describePatchGroupStateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePatchGroupState operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribePatchGroupState
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describePatchGroupStateAsync(DescribePatchGroupStateRequest describePatchGroupStateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all patch groups that have been registered with patch baselines.
*
*
* @param describePatchGroupsRequest
* @return A Java Future containing the result of the DescribePatchGroups operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribePatchGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describePatchGroupsAsync(DescribePatchGroupsRequest describePatchGroupsRequest);
/**
*
* Lists all patch groups that have been registered with patch baselines.
*
*
* @param describePatchGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePatchGroups operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribePatchGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describePatchGroupsAsync(DescribePatchGroupsRequest describePatchGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the properties of available patches organized by product, product family, classification, severity, and
* other properties of available patches. You can use the reported properties in the filters you specify in requests
* for operations such as CreatePatchBaseline, UpdatePatchBaseline, DescribeAvailablePatches,
* and DescribePatchBaselines.
*
*
* The following section lists the properties that can be used in filters for each major operating system type:
*
*
* - AMAZON_LINUX
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - AMAZON_LINUX_2
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - CENTOS
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - DEBIAN
* -
*
* Valid properties: PRODUCT
| PRIORITY
*
*
* - MACOS
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
*
*
* - ORACLE_LINUX
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - REDHAT_ENTERPRISE_LINUX
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - SUSE
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - UBUNTU
* -
*
* Valid properties: PRODUCT
| PRIORITY
*
*
* - WINDOWS
* -
*
* Valid properties: PRODUCT
| PRODUCT_FAMILY
| CLASSIFICATION
|
* MSRC_SEVERITY
*
*
*
*
* @param describePatchPropertiesRequest
* @return A Java Future containing the result of the DescribePatchProperties operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribePatchProperties
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describePatchPropertiesAsync(DescribePatchPropertiesRequest describePatchPropertiesRequest);
/**
*
* Lists the properties of available patches organized by product, product family, classification, severity, and
* other properties of available patches. You can use the reported properties in the filters you specify in requests
* for operations such as CreatePatchBaseline, UpdatePatchBaseline, DescribeAvailablePatches,
* and DescribePatchBaselines.
*
*
* The following section lists the properties that can be used in filters for each major operating system type:
*
*
* - AMAZON_LINUX
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - AMAZON_LINUX_2
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - CENTOS
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - DEBIAN
* -
*
* Valid properties: PRODUCT
| PRIORITY
*
*
* - MACOS
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
*
*
* - ORACLE_LINUX
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - REDHAT_ENTERPRISE_LINUX
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - SUSE
* -
*
* Valid properties: PRODUCT
| CLASSIFICATION
| SEVERITY
*
*
* - UBUNTU
* -
*
* Valid properties: PRODUCT
| PRIORITY
*
*
* - WINDOWS
* -
*
* Valid properties: PRODUCT
| PRODUCT_FAMILY
| CLASSIFICATION
|
* MSRC_SEVERITY
*
*
*
*
* @param describePatchPropertiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePatchProperties operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribePatchProperties
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describePatchPropertiesAsync(DescribePatchPropertiesRequest describePatchPropertiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of all active sessions (both connected and disconnected) or terminated sessions from the past 30
* days.
*
*
* @param describeSessionsRequest
* @return A Java Future containing the result of the DescribeSessions operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.DescribeSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSessionsAsync(DescribeSessionsRequest describeSessionsRequest);
/**
*
* Retrieves a list of all active sessions (both connected and disconnected) or terminated sessions from the past 30
* days.
*
*
* @param describeSessionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSessions operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DescribeSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSessionsAsync(DescribeSessionsRequest describeSessionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the association between an OpsItem and a related item. For example, this API operation can delete an
* Incident Manager incident from an OpsItem. Incident Manager is a capability of Amazon Web Services Systems
* Manager.
*
*
* @param disassociateOpsItemRelatedItemRequest
* @return A Java Future containing the result of the DisassociateOpsItemRelatedItem operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.DisassociateOpsItemRelatedItem
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateOpsItemRelatedItemAsync(
DisassociateOpsItemRelatedItemRequest disassociateOpsItemRelatedItemRequest);
/**
*
* Deletes the association between an OpsItem and a related item. For example, this API operation can delete an
* Incident Manager incident from an OpsItem. Incident Manager is a capability of Amazon Web Services Systems
* Manager.
*
*
* @param disassociateOpsItemRelatedItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateOpsItemRelatedItem operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.DisassociateOpsItemRelatedItem
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateOpsItemRelatedItemAsync(
DisassociateOpsItemRelatedItemRequest disassociateOpsItemRelatedItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get detailed information about a particular Automation execution.
*
*
* @param getAutomationExecutionRequest
* @return A Java Future containing the result of the GetAutomationExecution operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetAutomationExecution
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAutomationExecutionAsync(GetAutomationExecutionRequest getAutomationExecutionRequest);
/**
*
* Get detailed information about a particular Automation execution.
*
*
* @param getAutomationExecutionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAutomationExecution operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetAutomationExecution
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAutomationExecutionAsync(GetAutomationExecutionRequest getAutomationExecutionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the state of a Amazon Web Services Systems Manager change calendar at the current time or a specified time.
* If you specify a time, GetCalendarState
returns the state of the calendar at that specific time, and
* returns the next time that the change calendar state will transition. If you don't specify a time,
* GetCalendarState
uses the current time. Change Calendar entries have two possible states:
* OPEN
or CLOSED
.
*
*
* If you specify more than one calendar in a request, the command returns the status of OPEN
only if
* all calendars in the request are open. If one or more calendars in the request are closed, the status returned is
* CLOSED
.
*
*
* For more information about Change Calendar, a capability of Amazon Web Services Systems Manager, see Amazon
* Web Services Systems Manager Change Calendar in the Amazon Web Services Systems Manager User Guide.
*
*
* @param getCalendarStateRequest
* @return A Java Future containing the result of the GetCalendarState operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetCalendarState
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCalendarStateAsync(GetCalendarStateRequest getCalendarStateRequest);
/**
*
* Gets the state of a Amazon Web Services Systems Manager change calendar at the current time or a specified time.
* If you specify a time, GetCalendarState
returns the state of the calendar at that specific time, and
* returns the next time that the change calendar state will transition. If you don't specify a time,
* GetCalendarState
uses the current time. Change Calendar entries have two possible states:
* OPEN
or CLOSED
.
*
*
* If you specify more than one calendar in a request, the command returns the status of OPEN
only if
* all calendars in the request are open. If one or more calendars in the request are closed, the status returned is
* CLOSED
.
*
*
* For more information about Change Calendar, a capability of Amazon Web Services Systems Manager, see Amazon
* Web Services Systems Manager Change Calendar in the Amazon Web Services Systems Manager User Guide.
*
*
* @param getCalendarStateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCalendarState operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetCalendarState
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCalendarStateAsync(GetCalendarStateRequest getCalendarStateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns detailed information about command execution for an invocation or plugin.
*
*
* GetCommandInvocation
only gives the execution status of a plugin in a document. To get the command
* execution status on a specific managed node, use ListCommandInvocations. To get the command execution
* status across managed nodes, use ListCommands.
*
*
* @param getCommandInvocationRequest
* @return A Java Future containing the result of the GetCommandInvocation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetCommandInvocation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCommandInvocationAsync(GetCommandInvocationRequest getCommandInvocationRequest);
/**
*
* Returns detailed information about command execution for an invocation or plugin.
*
*
* GetCommandInvocation
only gives the execution status of a plugin in a document. To get the command
* execution status on a specific managed node, use ListCommandInvocations. To get the command execution
* status across managed nodes, use ListCommands.
*
*
* @param getCommandInvocationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCommandInvocation operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetCommandInvocation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCommandInvocationAsync(GetCommandInvocationRequest getCommandInvocationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the Session Manager connection status for a managed node to determine whether it is running and ready
* to receive Session Manager connections.
*
*
* @param getConnectionStatusRequest
* @return A Java Future containing the result of the GetConnectionStatus operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetConnectionStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getConnectionStatusAsync(GetConnectionStatusRequest getConnectionStatusRequest);
/**
*
* Retrieves the Session Manager connection status for a managed node to determine whether it is running and ready
* to receive Session Manager connections.
*
*
* @param getConnectionStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetConnectionStatus operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetConnectionStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getConnectionStatusAsync(GetConnectionStatusRequest getConnectionStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the default patch baseline. Amazon Web Services Systems Manager supports creating multiple default
* patch baselines. For example, you can create a default patch baseline for each operating system.
*
*
* If you don't specify an operating system value, the default patch baseline for Windows is returned.
*
*
* @param getDefaultPatchBaselineRequest
* @return A Java Future containing the result of the GetDefaultPatchBaseline operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetDefaultPatchBaseline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDefaultPatchBaselineAsync(GetDefaultPatchBaselineRequest getDefaultPatchBaselineRequest);
/**
*
* Retrieves the default patch baseline. Amazon Web Services Systems Manager supports creating multiple default
* patch baselines. For example, you can create a default patch baseline for each operating system.
*
*
* If you don't specify an operating system value, the default patch baseline for Windows is returned.
*
*
* @param getDefaultPatchBaselineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDefaultPatchBaseline operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetDefaultPatchBaseline
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDefaultPatchBaselineAsync(GetDefaultPatchBaselineRequest getDefaultPatchBaselineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the current snapshot for the patch baseline the managed node uses. This API is primarily used by the
* AWS-RunPatchBaseline
Systems Manager document (SSM document).
*
*
*
* If you run the command locally, such as with the Command Line Interface (CLI), the system attempts to use your
* local Amazon Web Services credentials and the operation fails. To avoid this, you can run the command in the
* Amazon Web Services Systems Manager console. Use Run Command, a capability of Amazon Web Services Systems
* Manager, with an SSM document that enables you to target a managed node with a script or command. For example,
* run the command using the AWS-RunShellScript
document or the AWS-RunPowerShellScript
* document.
*
*
*
* @param getDeployablePatchSnapshotForInstanceRequest
* @return A Java Future containing the result of the GetDeployablePatchSnapshotForInstance operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsync.GetDeployablePatchSnapshotForInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeployablePatchSnapshotForInstanceAsync(
GetDeployablePatchSnapshotForInstanceRequest getDeployablePatchSnapshotForInstanceRequest);
/**
*
* Retrieves the current snapshot for the patch baseline the managed node uses. This API is primarily used by the
* AWS-RunPatchBaseline
Systems Manager document (SSM document).
*
*
*
* If you run the command locally, such as with the Command Line Interface (CLI), the system attempts to use your
* local Amazon Web Services credentials and the operation fails. To avoid this, you can run the command in the
* Amazon Web Services Systems Manager console. Use Run Command, a capability of Amazon Web Services Systems
* Manager, with an SSM document that enables you to target a managed node with a script or command. For example,
* run the command using the AWS-RunShellScript
document or the AWS-RunPowerShellScript
* document.
*
*
*
* @param getDeployablePatchSnapshotForInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeployablePatchSnapshotForInstance operation returned by
* the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetDeployablePatchSnapshotForInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeployablePatchSnapshotForInstanceAsync(
GetDeployablePatchSnapshotForInstanceRequest getDeployablePatchSnapshotForInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the contents of the specified Amazon Web Services Systems Manager document (SSM document).
*
*
* @param getDocumentRequest
* @return A Java Future containing the result of the GetDocument operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDocumentAsync(GetDocumentRequest getDocumentRequest);
/**
*
* Gets the contents of the specified Amazon Web Services Systems Manager document (SSM document).
*
*
* @param getDocumentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDocument operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDocumentAsync(GetDocumentRequest getDocumentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Query inventory information. This includes managed node status, such as Stopped
or
* Terminated
.
*
*
* @param getInventoryRequest
* @return A Java Future containing the result of the GetInventory operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetInventory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInventoryAsync(GetInventoryRequest getInventoryRequest);
/**
*
* Query inventory information. This includes managed node status, such as Stopped
or
* Terminated
.
*
*
* @param getInventoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInventory operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetInventory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInventoryAsync(GetInventoryRequest getInventoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a list of inventory type names for the account, or return a list of attribute names for a specific
* Inventory item type.
*
*
* @param getInventorySchemaRequest
* @return A Java Future containing the result of the GetInventorySchema operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetInventorySchema
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInventorySchemaAsync(GetInventorySchemaRequest getInventorySchemaRequest);
/**
*
* Return a list of inventory type names for the account, or return a list of attribute names for a specific
* Inventory item type.
*
*
* @param getInventorySchemaRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInventorySchema operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetInventorySchema
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInventorySchemaAsync(GetInventorySchemaRequest getInventorySchemaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a maintenance window.
*
*
* @param getMaintenanceWindowRequest
* @return A Java Future containing the result of the GetMaintenanceWindow operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetMaintenanceWindow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMaintenanceWindowAsync(GetMaintenanceWindowRequest getMaintenanceWindowRequest);
/**
*
* Retrieves a maintenance window.
*
*
* @param getMaintenanceWindowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMaintenanceWindow operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetMaintenanceWindow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMaintenanceWindowAsync(GetMaintenanceWindowRequest getMaintenanceWindowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves details about a specific a maintenance window execution.
*
*
* @param getMaintenanceWindowExecutionRequest
* @return A Java Future containing the result of the GetMaintenanceWindowExecution operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.GetMaintenanceWindowExecution
* @see AWS API Documentation
*/
java.util.concurrent.Future getMaintenanceWindowExecutionAsync(
GetMaintenanceWindowExecutionRequest getMaintenanceWindowExecutionRequest);
/**
*
* Retrieves details about a specific a maintenance window execution.
*
*
* @param getMaintenanceWindowExecutionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMaintenanceWindowExecution operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetMaintenanceWindowExecution
* @see AWS API Documentation
*/
java.util.concurrent.Future getMaintenanceWindowExecutionAsync(
GetMaintenanceWindowExecutionRequest getMaintenanceWindowExecutionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the details about a specific task run as part of a maintenance window execution.
*
*
* @param getMaintenanceWindowExecutionTaskRequest
* @return A Java Future containing the result of the GetMaintenanceWindowExecutionTask operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsync.GetMaintenanceWindowExecutionTask
* @see AWS API Documentation
*/
java.util.concurrent.Future getMaintenanceWindowExecutionTaskAsync(
GetMaintenanceWindowExecutionTaskRequest getMaintenanceWindowExecutionTaskRequest);
/**
*
* Retrieves the details about a specific task run as part of a maintenance window execution.
*
*
* @param getMaintenanceWindowExecutionTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMaintenanceWindowExecutionTask operation returned by the
* service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetMaintenanceWindowExecutionTask
* @see AWS API Documentation
*/
java.util.concurrent.Future getMaintenanceWindowExecutionTaskAsync(
GetMaintenanceWindowExecutionTaskRequest getMaintenanceWindowExecutionTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about a specific task running on a specific target.
*
*
* @param getMaintenanceWindowExecutionTaskInvocationRequest
* @return A Java Future containing the result of the GetMaintenanceWindowExecutionTaskInvocation operation returned
* by the service.
* @sample AWSSimpleSystemsManagementAsync.GetMaintenanceWindowExecutionTaskInvocation
* @see AWS API Documentation
*/
java.util.concurrent.Future getMaintenanceWindowExecutionTaskInvocationAsync(
GetMaintenanceWindowExecutionTaskInvocationRequest getMaintenanceWindowExecutionTaskInvocationRequest);
/**
*
* Retrieves information about a specific task running on a specific target.
*
*
* @param getMaintenanceWindowExecutionTaskInvocationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMaintenanceWindowExecutionTaskInvocation operation returned
* by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetMaintenanceWindowExecutionTaskInvocation
* @see AWS API Documentation
*/
java.util.concurrent.Future getMaintenanceWindowExecutionTaskInvocationAsync(
GetMaintenanceWindowExecutionTaskInvocationRequest getMaintenanceWindowExecutionTaskInvocationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the details of a maintenance window task.
*
*
*
* For maintenance window tasks without a specified target, you can't supply values for --max-errors
* and --max-concurrency
. Instead, the system inserts a placeholder value of 1
, which may
* be reported in the response to this command. These values don't affect the running of your task and can be
* ignored.
*
*
*
* To retrieve a list of tasks in a maintenance window, instead use the DescribeMaintenanceWindowTasks
* command.
*
*
* @param getMaintenanceWindowTaskRequest
* @return A Java Future containing the result of the GetMaintenanceWindowTask operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetMaintenanceWindowTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMaintenanceWindowTaskAsync(GetMaintenanceWindowTaskRequest getMaintenanceWindowTaskRequest);
/**
*
* Retrieves the details of a maintenance window task.
*
*
*
* For maintenance window tasks without a specified target, you can't supply values for --max-errors
* and --max-concurrency
. Instead, the system inserts a placeholder value of 1
, which may
* be reported in the response to this command. These values don't affect the running of your task and can be
* ignored.
*
*
*
* To retrieve a list of tasks in a maintenance window, instead use the DescribeMaintenanceWindowTasks
* command.
*
*
* @param getMaintenanceWindowTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMaintenanceWindowTask operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetMaintenanceWindowTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMaintenanceWindowTaskAsync(GetMaintenanceWindowTaskRequest getMaintenanceWindowTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get information about an OpsItem by using the ID. You must have permission in Identity and Access Management
* (IAM) to view information about an OpsItem. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param getOpsItemRequest
* @return A Java Future containing the result of the GetOpsItem operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetOpsItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOpsItemAsync(GetOpsItemRequest getOpsItemRequest);
/**
*
* Get information about an OpsItem by using the ID. You must have permission in Identity and Access Management
* (IAM) to view information about an OpsItem. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate,
* and remediate operational issues impacting the performance and health of their Amazon Web Services resources. For
* more information, see Amazon Web Services Systems
* Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @param getOpsItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOpsItem operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetOpsItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOpsItemAsync(GetOpsItemRequest getOpsItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* View operational metadata related to an application in Application Manager.
*
*
* @param getOpsMetadataRequest
* @return A Java Future containing the result of the GetOpsMetadata operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetOpsMetadata
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOpsMetadataAsync(GetOpsMetadataRequest getOpsMetadataRequest);
/**
*
* View operational metadata related to an application in Application Manager.
*
*
* @param getOpsMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOpsMetadata operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetOpsMetadata
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOpsMetadataAsync(GetOpsMetadataRequest getOpsMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* View a summary of operations metadata (OpsData) based on specified filters and aggregators. OpsData can include
* information about Amazon Web Services Systems Manager OpsCenter operational workitems (OpsItems) as well as
* information about any Amazon Web Services resource or service configured to report OpsData to Amazon Web Services
* Systems Manager Explorer.
*
*
* @param getOpsSummaryRequest
* @return A Java Future containing the result of the GetOpsSummary operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetOpsSummary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOpsSummaryAsync(GetOpsSummaryRequest getOpsSummaryRequest);
/**
*
* View a summary of operations metadata (OpsData) based on specified filters and aggregators. OpsData can include
* information about Amazon Web Services Systems Manager OpsCenter operational workitems (OpsItems) as well as
* information about any Amazon Web Services resource or service configured to report OpsData to Amazon Web Services
* Systems Manager Explorer.
*
*
* @param getOpsSummaryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOpsSummary operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetOpsSummary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOpsSummaryAsync(GetOpsSummaryRequest getOpsSummaryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get information about a single parameter by specifying the parameter name.
*
*
*
* To get information about more than one parameter at a time, use the GetParameters operation.
*
*
*
* @param getParameterRequest
* @return A Java Future containing the result of the GetParameter operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetParameter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getParameterAsync(GetParameterRequest getParameterRequest);
/**
*
* Get information about a single parameter by specifying the parameter name.
*
*
*
* To get information about more than one parameter at a time, use the GetParameters operation.
*
*
*
* @param getParameterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetParameter operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetParameter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getParameterAsync(GetParameterRequest getParameterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the history of all changes to a parameter.
*
*
*
* If you change the KMS key alias for the KMS key used to encrypt a parameter, then you must also update the key
* alias the parameter uses to reference KMS. Otherwise, GetParameterHistory
retrieves whatever the
* original key alias was referencing.
*
*
*
* @param getParameterHistoryRequest
* @return A Java Future containing the result of the GetParameterHistory operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetParameterHistory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getParameterHistoryAsync(GetParameterHistoryRequest getParameterHistoryRequest);
/**
*
* Retrieves the history of all changes to a parameter.
*
*
*
* If you change the KMS key alias for the KMS key used to encrypt a parameter, then you must also update the key
* alias the parameter uses to reference KMS. Otherwise, GetParameterHistory
retrieves whatever the
* original key alias was referencing.
*
*
*
* @param getParameterHistoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetParameterHistory operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetParameterHistory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getParameterHistoryAsync(GetParameterHistoryRequest getParameterHistoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get information about one or more parameters by specifying multiple parameter names.
*
*
*
* To get information about a single parameter, you can use the GetParameter operation instead.
*
*
*
* @param getParametersRequest
* @return A Java Future containing the result of the GetParameters operation returned by the service.
* @sample AWSSimpleSystemsManagementAsync.GetParameters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getParametersAsync(GetParametersRequest getParametersRequest);
/**
*
* Get information about one or more parameters by specifying multiple parameter names.
*
*
*
* To get information about a single parameter, you can use the GetParameter operation instead.
*
*
*
* @param getParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetParameters operation returned by the service.
* @sample AWSSimpleSystemsManagementAsyncHandler.GetParameters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getParametersAsync(GetParametersRequest getParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve information about one or more parameters in a specific hierarchy.
*
*
* Request results are returned on a best-effort basis. If you specify