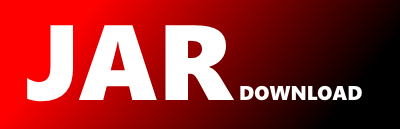
com.amazonaws.services.simplesystemsmanagement.model.AssociationDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the parameters for a document.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AssociationDescription implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the SSM document.
*
*/
private String name;
/**
*
* The managed node ID.
*
*/
private String instanceId;
/**
*
* The association version.
*
*/
private String associationVersion;
/**
*
* The date when the association was made.
*
*/
private java.util.Date date;
/**
*
* The date when the association was last updated.
*
*/
private java.util.Date lastUpdateAssociationDate;
/**
*
* The association status.
*
*/
private AssociationStatus status;
/**
*
* Information about the association.
*
*/
private AssociationOverview overview;
/**
*
* The document version.
*
*/
private String documentVersion;
/**
*
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*
*/
private String automationTargetParameterName;
/**
*
* A description of the parameters for a document.
*
*/
private java.util.Map> parameters;
/**
*
* The association ID.
*
*/
private String associationId;
/**
*
* The managed nodes targeted by the request.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* A cron expression that specifies a schedule when the association runs.
*
*/
private String scheduleExpression;
/**
*
* An S3 bucket where you want to store the output details of the request.
*
*/
private InstanceAssociationOutputLocation outputLocation;
/**
*
* The date on which the association was last run.
*
*/
private java.util.Date lastExecutionDate;
/**
*
* The last date on which the association was successfully run.
*
*/
private java.util.Date lastSuccessfulExecutionDate;
/**
*
* The association name.
*
*/
private String associationName;
/**
*
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops sending requests when the fourth
* error is received. If you specify 0, then the system stops sending requests after the first error is returned. If
* you run an association on 50 managed nodes and set MaxError
to 10%, then the system stops sending
* the request when the sixth error is received.
*
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more than
* max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at a time.
*
*/
private String maxErrors;
/**
*
* The maximum number of targets allowed to run the association at the same time. You can specify a number, for
* example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means all
* targets run the association at the same time.
*
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*
*/
private String maxConcurrency;
/**
*
* The severity level that is assigned to the association.
*
*/
private String complianceSeverity;
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager, a
* capability of Amazon Web Services Systems Manager. It is managed by your direct call to the
* PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*/
private String syncCompliance;
/**
*
* By default, when you create a new associations, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*/
private Boolean applyOnlyAtCronInterval;
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are gated
* under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*/
private com.amazonaws.internal.SdkInternalList calendarNames;
/**
*
* The combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run the
* association.
*
*/
private com.amazonaws.internal.SdkInternalList targetLocations;
/**
*
* Number of days to wait after the scheduled day to run an association.
*
*/
private Integer scheduleOffset;
/**
*
* The number of hours that an association can run on specified targets. After the resulting cutoff time passes,
* associations that are currently running are cancelled, and no pending executions are started on remaining
* targets.
*
*/
private Integer duration;
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*/
private com.amazonaws.internal.SdkInternalList>> targetMaps;
private AlarmConfiguration alarmConfiguration;
/**
*
* The CloudWatch alarm that was invoked during the association.
*
*/
private com.amazonaws.internal.SdkInternalList triggeredAlarms;
/**
*
* The name of the SSM document.
*
*
* @param name
* The name of the SSM document.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the SSM document.
*
*
* @return The name of the SSM document.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the SSM document.
*
*
* @param name
* The name of the SSM document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withName(String name) {
setName(name);
return this;
}
/**
*
* The managed node ID.
*
*
* @param instanceId
* The managed node ID.
*/
public void setInstanceId(String instanceId) {
this.instanceId = instanceId;
}
/**
*
* The managed node ID.
*
*
* @return The managed node ID.
*/
public String getInstanceId() {
return this.instanceId;
}
/**
*
* The managed node ID.
*
*
* @param instanceId
* The managed node ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withInstanceId(String instanceId) {
setInstanceId(instanceId);
return this;
}
/**
*
* The association version.
*
*
* @param associationVersion
* The association version.
*/
public void setAssociationVersion(String associationVersion) {
this.associationVersion = associationVersion;
}
/**
*
* The association version.
*
*
* @return The association version.
*/
public String getAssociationVersion() {
return this.associationVersion;
}
/**
*
* The association version.
*
*
* @param associationVersion
* The association version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withAssociationVersion(String associationVersion) {
setAssociationVersion(associationVersion);
return this;
}
/**
*
* The date when the association was made.
*
*
* @param date
* The date when the association was made.
*/
public void setDate(java.util.Date date) {
this.date = date;
}
/**
*
* The date when the association was made.
*
*
* @return The date when the association was made.
*/
public java.util.Date getDate() {
return this.date;
}
/**
*
* The date when the association was made.
*
*
* @param date
* The date when the association was made.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withDate(java.util.Date date) {
setDate(date);
return this;
}
/**
*
* The date when the association was last updated.
*
*
* @param lastUpdateAssociationDate
* The date when the association was last updated.
*/
public void setLastUpdateAssociationDate(java.util.Date lastUpdateAssociationDate) {
this.lastUpdateAssociationDate = lastUpdateAssociationDate;
}
/**
*
* The date when the association was last updated.
*
*
* @return The date when the association was last updated.
*/
public java.util.Date getLastUpdateAssociationDate() {
return this.lastUpdateAssociationDate;
}
/**
*
* The date when the association was last updated.
*
*
* @param lastUpdateAssociationDate
* The date when the association was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withLastUpdateAssociationDate(java.util.Date lastUpdateAssociationDate) {
setLastUpdateAssociationDate(lastUpdateAssociationDate);
return this;
}
/**
*
* The association status.
*
*
* @param status
* The association status.
*/
public void setStatus(AssociationStatus status) {
this.status = status;
}
/**
*
* The association status.
*
*
* @return The association status.
*/
public AssociationStatus getStatus() {
return this.status;
}
/**
*
* The association status.
*
*
* @param status
* The association status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withStatus(AssociationStatus status) {
setStatus(status);
return this;
}
/**
*
* Information about the association.
*
*
* @param overview
* Information about the association.
*/
public void setOverview(AssociationOverview overview) {
this.overview = overview;
}
/**
*
* Information about the association.
*
*
* @return Information about the association.
*/
public AssociationOverview getOverview() {
return this.overview;
}
/**
*
* Information about the association.
*
*
* @param overview
* Information about the association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withOverview(AssociationOverview overview) {
setOverview(overview);
return this;
}
/**
*
* The document version.
*
*
* @param documentVersion
* The document version.
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The document version.
*
*
* @return The document version.
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The document version.
*
*
* @param documentVersion
* The document version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*
*
* @param automationTargetParameterName
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*/
public void setAutomationTargetParameterName(String automationTargetParameterName) {
this.automationTargetParameterName = automationTargetParameterName;
}
/**
*
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*
*
* @return Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*/
public String getAutomationTargetParameterName() {
return this.automationTargetParameterName;
}
/**
*
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*
*
* @param automationTargetParameterName
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withAutomationTargetParameterName(String automationTargetParameterName) {
setAutomationTargetParameterName(automationTargetParameterName);
return this;
}
/**
*
* A description of the parameters for a document.
*
*
* @return A description of the parameters for a document.
*/
public java.util.Map> getParameters() {
return parameters;
}
/**
*
* A description of the parameters for a document.
*
*
* @param parameters
* A description of the parameters for a document.
*/
public void setParameters(java.util.Map> parameters) {
this.parameters = parameters;
}
/**
*
* A description of the parameters for a document.
*
*
* @param parameters
* A description of the parameters for a document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withParameters(java.util.Map> parameters) {
setParameters(parameters);
return this;
}
/**
* Add a single Parameters entry
*
* @see AssociationDescription#withParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription addParametersEntry(String key, java.util.List value) {
if (null == this.parameters) {
this.parameters = new java.util.HashMap>();
}
if (this.parameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.parameters.put(key, value);
return this;
}
/**
* Removes all the entries added into Parameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription clearParametersEntries() {
this.parameters = null;
return this;
}
/**
*
* The association ID.
*
*
* @param associationId
* The association ID.
*/
public void setAssociationId(String associationId) {
this.associationId = associationId;
}
/**
*
* The association ID.
*
*
* @return The association ID.
*/
public String getAssociationId() {
return this.associationId;
}
/**
*
* The association ID.
*
*
* @param associationId
* The association ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withAssociationId(String associationId) {
setAssociationId(associationId);
return this;
}
/**
*
* The managed nodes targeted by the request.
*
*
* @return The managed nodes targeted by the request.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The managed nodes targeted by the request.
*
*
* @param targets
* The managed nodes targeted by the request.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The managed nodes targeted by the request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The managed nodes targeted by the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The managed nodes targeted by the request.
*
*
* @param targets
* The managed nodes targeted by the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* A cron expression that specifies a schedule when the association runs.
*
*
* @param scheduleExpression
* A cron expression that specifies a schedule when the association runs.
*/
public void setScheduleExpression(String scheduleExpression) {
this.scheduleExpression = scheduleExpression;
}
/**
*
* A cron expression that specifies a schedule when the association runs.
*
*
* @return A cron expression that specifies a schedule when the association runs.
*/
public String getScheduleExpression() {
return this.scheduleExpression;
}
/**
*
* A cron expression that specifies a schedule when the association runs.
*
*
* @param scheduleExpression
* A cron expression that specifies a schedule when the association runs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withScheduleExpression(String scheduleExpression) {
setScheduleExpression(scheduleExpression);
return this;
}
/**
*
* An S3 bucket where you want to store the output details of the request.
*
*
* @param outputLocation
* An S3 bucket where you want to store the output details of the request.
*/
public void setOutputLocation(InstanceAssociationOutputLocation outputLocation) {
this.outputLocation = outputLocation;
}
/**
*
* An S3 bucket where you want to store the output details of the request.
*
*
* @return An S3 bucket where you want to store the output details of the request.
*/
public InstanceAssociationOutputLocation getOutputLocation() {
return this.outputLocation;
}
/**
*
* An S3 bucket where you want to store the output details of the request.
*
*
* @param outputLocation
* An S3 bucket where you want to store the output details of the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withOutputLocation(InstanceAssociationOutputLocation outputLocation) {
setOutputLocation(outputLocation);
return this;
}
/**
*
* The date on which the association was last run.
*
*
* @param lastExecutionDate
* The date on which the association was last run.
*/
public void setLastExecutionDate(java.util.Date lastExecutionDate) {
this.lastExecutionDate = lastExecutionDate;
}
/**
*
* The date on which the association was last run.
*
*
* @return The date on which the association was last run.
*/
public java.util.Date getLastExecutionDate() {
return this.lastExecutionDate;
}
/**
*
* The date on which the association was last run.
*
*
* @param lastExecutionDate
* The date on which the association was last run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withLastExecutionDate(java.util.Date lastExecutionDate) {
setLastExecutionDate(lastExecutionDate);
return this;
}
/**
*
* The last date on which the association was successfully run.
*
*
* @param lastSuccessfulExecutionDate
* The last date on which the association was successfully run.
*/
public void setLastSuccessfulExecutionDate(java.util.Date lastSuccessfulExecutionDate) {
this.lastSuccessfulExecutionDate = lastSuccessfulExecutionDate;
}
/**
*
* The last date on which the association was successfully run.
*
*
* @return The last date on which the association was successfully run.
*/
public java.util.Date getLastSuccessfulExecutionDate() {
return this.lastSuccessfulExecutionDate;
}
/**
*
* The last date on which the association was successfully run.
*
*
* @param lastSuccessfulExecutionDate
* The last date on which the association was successfully run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withLastSuccessfulExecutionDate(java.util.Date lastSuccessfulExecutionDate) {
setLastSuccessfulExecutionDate(lastSuccessfulExecutionDate);
return this;
}
/**
*
* The association name.
*
*
* @param associationName
* The association name.
*/
public void setAssociationName(String associationName) {
this.associationName = associationName;
}
/**
*
* The association name.
*
*
* @return The association name.
*/
public String getAssociationName() {
return this.associationName;
}
/**
*
* The association name.
*
*
* @param associationName
* The association name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withAssociationName(String associationName) {
setAssociationName(associationName);
return this;
}
/**
*
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops sending requests when the fourth
* error is received. If you specify 0, then the system stops sending requests after the first error is returned. If
* you run an association on 50 managed nodes and set MaxError
to 10%, then the system stops sending
* the request when the sixth error is received.
*
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more than
* max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at a time.
*
*
* @param maxErrors
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage
* of the target set, for example 10%. If you specify 3, for example, the system stops sending requests when
* the fourth error is received. If you specify 0, then the system stops sending requests after the first
* error is returned. If you run an association on 50 managed nodes and set MaxError
to 10%,
* then the system stops sending the request when the sixth error is received.
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more
* than max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at
* a time.
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops sending requests when the fourth
* error is received. If you specify 0, then the system stops sending requests after the first error is returned. If
* you run an association on 50 managed nodes and set MaxError
to 10%, then the system stops sending
* the request when the sixth error is received.
*
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more than
* max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at a time.
*
*
* @return The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage
* of the target set, for example 10%. If you specify 3, for example, the system stops sending requests when
* the fourth error is received. If you specify 0, then the system stops sending requests after the first
* error is returned. If you run an association on 50 managed nodes and set MaxError
to 10%,
* then the system stops sending the request when the sixth error is received.
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more
* than max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at
* a time.
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops sending requests when the fourth
* error is received. If you specify 0, then the system stops sending requests after the first error is returned. If
* you run an association on 50 managed nodes and set MaxError
to 10%, then the system stops sending
* the request when the sixth error is received.
*
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more than
* max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at a time.
*
*
* @param maxErrors
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage
* of the target set, for example 10%. If you specify 3, for example, the system stops sending requests when
* the fourth error is received. If you specify 0, then the system stops sending requests after the first
* error is returned. If you run an association on 50 managed nodes and set MaxError
to 10%,
* then the system stops sending the request when the sixth error is received.
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more
* than max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at
* a time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The maximum number of targets allowed to run the association at the same time. You can specify a number, for
* example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means all
* targets run the association at the same time.
*
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*
*
* @param maxConcurrency
* The maximum number of targets allowed to run the association at the same time. You can specify a number,
* for example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means
* all targets run the association at the same time.
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The maximum number of targets allowed to run the association at the same time. You can specify a number, for
* example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means all
* targets run the association at the same time.
*
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*
*
* @return The maximum number of targets allowed to run the association at the same time. You can specify a number,
* for example 10, or a percentage of the target set, for example 10%. The default value is 100%, which
* means all targets run the association at the same time.
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The maximum number of targets allowed to run the association at the same time. You can specify a number, for
* example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means all
* targets run the association at the same time.
*
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*
*
* @param maxConcurrency
* The maximum number of targets allowed to run the association at the same time. You can specify a number,
* for example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means
* all targets run the association at the same time.
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The severity level that is assigned to the association.
*
*
* @param complianceSeverity
* The severity level that is assigned to the association.
* @see AssociationComplianceSeverity
*/
public void setComplianceSeverity(String complianceSeverity) {
this.complianceSeverity = complianceSeverity;
}
/**
*
* The severity level that is assigned to the association.
*
*
* @return The severity level that is assigned to the association.
* @see AssociationComplianceSeverity
*/
public String getComplianceSeverity() {
return this.complianceSeverity;
}
/**
*
* The severity level that is assigned to the association.
*
*
* @param complianceSeverity
* The severity level that is assigned to the association.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationComplianceSeverity
*/
public AssociationDescription withComplianceSeverity(String complianceSeverity) {
setComplianceSeverity(complianceSeverity);
return this;
}
/**
*
* The severity level that is assigned to the association.
*
*
* @param complianceSeverity
* The severity level that is assigned to the association.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationComplianceSeverity
*/
public AssociationDescription withComplianceSeverity(AssociationComplianceSeverity complianceSeverity) {
this.complianceSeverity = complianceSeverity.toString();
return this;
}
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager, a
* capability of Amazon Web Services Systems Manager. It is managed by your direct call to the
* PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*
* @param syncCompliance
* The mode for generating association compliance. You can specify AUTO
or MANUAL
.
* In AUTO
mode, the system uses the status of the association execution to determine the
* compliance status. If the association execution runs successfully, then the association is
* COMPLIANT
. If the association execution doesn't run successfully, the association is
* NON-COMPLIANT
.
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager, a
* capability of Amazon Web Services Systems Manager. It is managed by your direct call to the
* PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
* @see AssociationSyncCompliance
*/
public void setSyncCompliance(String syncCompliance) {
this.syncCompliance = syncCompliance;
}
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager, a
* capability of Amazon Web Services Systems Manager. It is managed by your direct call to the
* PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*
* @return The mode for generating association compliance. You can specify AUTO
or MANUAL
.
* In AUTO
mode, the system uses the status of the association execution to determine the
* compliance status. If the association execution runs successfully, then the association is
* COMPLIANT
. If the association execution doesn't run successfully, the association is
* NON-COMPLIANT
.
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager, a
* capability of Amazon Web Services Systems Manager. It is managed by your direct call to the
* PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
* @see AssociationSyncCompliance
*/
public String getSyncCompliance() {
return this.syncCompliance;
}
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager, a
* capability of Amazon Web Services Systems Manager. It is managed by your direct call to the
* PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*
* @param syncCompliance
* The mode for generating association compliance. You can specify AUTO
or MANUAL
.
* In AUTO
mode, the system uses the status of the association execution to determine the
* compliance status. If the association execution runs successfully, then the association is
* COMPLIANT
. If the association execution doesn't run successfully, the association is
* NON-COMPLIANT
.
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager, a
* capability of Amazon Web Services Systems Manager. It is managed by your direct call to the
* PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationSyncCompliance
*/
public AssociationDescription withSyncCompliance(String syncCompliance) {
setSyncCompliance(syncCompliance);
return this;
}
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager, a
* capability of Amazon Web Services Systems Manager. It is managed by your direct call to the
* PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*
* @param syncCompliance
* The mode for generating association compliance. You can specify AUTO
or MANUAL
.
* In AUTO
mode, the system uses the status of the association execution to determine the
* compliance status. If the association execution runs successfully, then the association is
* COMPLIANT
. If the association execution doesn't run successfully, the association is
* NON-COMPLIANT
.
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager, a
* capability of Amazon Web Services Systems Manager. It is managed by your direct call to the
* PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationSyncCompliance
*/
public AssociationDescription withSyncCompliance(AssociationSyncCompliance syncCompliance) {
this.syncCompliance = syncCompliance.toString();
return this;
}
/**
*
* By default, when you create a new associations, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*
* @param applyOnlyAtCronInterval
* By default, when you create a new associations, the system runs it immediately after it is created and
* then according to the schedule you specified. Specify this option if you don't want an association to run
* immediately after you create it. This parameter isn't supported for rate expressions.
*/
public void setApplyOnlyAtCronInterval(Boolean applyOnlyAtCronInterval) {
this.applyOnlyAtCronInterval = applyOnlyAtCronInterval;
}
/**
*
* By default, when you create a new associations, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*
* @return By default, when you create a new associations, the system runs it immediately after it is created and
* then according to the schedule you specified. Specify this option if you don't want an association to run
* immediately after you create it. This parameter isn't supported for rate expressions.
*/
public Boolean getApplyOnlyAtCronInterval() {
return this.applyOnlyAtCronInterval;
}
/**
*
* By default, when you create a new associations, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*
* @param applyOnlyAtCronInterval
* By default, when you create a new associations, the system runs it immediately after it is created and
* then according to the schedule you specified. Specify this option if you don't want an association to run
* immediately after you create it. This parameter isn't supported for rate expressions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withApplyOnlyAtCronInterval(Boolean applyOnlyAtCronInterval) {
setApplyOnlyAtCronInterval(applyOnlyAtCronInterval);
return this;
}
/**
*
* By default, when you create a new associations, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*
* @return By default, when you create a new associations, the system runs it immediately after it is created and
* then according to the schedule you specified. Specify this option if you don't want an association to run
* immediately after you create it. This parameter isn't supported for rate expressions.
*/
public Boolean isApplyOnlyAtCronInterval() {
return this.applyOnlyAtCronInterval;
}
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are gated
* under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*
* @return The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are
* gated under. The associations only run when that change calendar is open. For more information, see Amazon
* Web Services Systems Manager Change Calendar.
*/
public java.util.List getCalendarNames() {
if (calendarNames == null) {
calendarNames = new com.amazonaws.internal.SdkInternalList();
}
return calendarNames;
}
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are gated
* under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*
* @param calendarNames
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are
* gated under. The associations only run when that change calendar is open. For more information, see Amazon
* Web Services Systems Manager Change Calendar.
*/
public void setCalendarNames(java.util.Collection calendarNames) {
if (calendarNames == null) {
this.calendarNames = null;
return;
}
this.calendarNames = new com.amazonaws.internal.SdkInternalList(calendarNames);
}
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are gated
* under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCalendarNames(java.util.Collection)} or {@link #withCalendarNames(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param calendarNames
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are
* gated under. The associations only run when that change calendar is open. For more information, see Amazon
* Web Services Systems Manager Change Calendar.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withCalendarNames(String... calendarNames) {
if (this.calendarNames == null) {
setCalendarNames(new com.amazonaws.internal.SdkInternalList(calendarNames.length));
}
for (String ele : calendarNames) {
this.calendarNames.add(ele);
}
return this;
}
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are gated
* under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*
* @param calendarNames
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are
* gated under. The associations only run when that change calendar is open. For more information, see Amazon
* Web Services Systems Manager Change Calendar.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withCalendarNames(java.util.Collection calendarNames) {
setCalendarNames(calendarNames);
return this;
}
/**
*
* The combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run the
* association.
*
*
* @return The combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run the
* association.
*/
public java.util.List getTargetLocations() {
if (targetLocations == null) {
targetLocations = new com.amazonaws.internal.SdkInternalList();
}
return targetLocations;
}
/**
*
* The combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run the
* association.
*
*
* @param targetLocations
* The combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run the
* association.
*/
public void setTargetLocations(java.util.Collection targetLocations) {
if (targetLocations == null) {
this.targetLocations = null;
return;
}
this.targetLocations = new com.amazonaws.internal.SdkInternalList(targetLocations);
}
/**
*
* The combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run the
* association.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetLocations(java.util.Collection)} or {@link #withTargetLocations(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param targetLocations
* The combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run the
* association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withTargetLocations(TargetLocation... targetLocations) {
if (this.targetLocations == null) {
setTargetLocations(new com.amazonaws.internal.SdkInternalList(targetLocations.length));
}
for (TargetLocation ele : targetLocations) {
this.targetLocations.add(ele);
}
return this;
}
/**
*
* The combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run the
* association.
*
*
* @param targetLocations
* The combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run the
* association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withTargetLocations(java.util.Collection targetLocations) {
setTargetLocations(targetLocations);
return this;
}
/**
*
* Number of days to wait after the scheduled day to run an association.
*
*
* @param scheduleOffset
* Number of days to wait after the scheduled day to run an association.
*/
public void setScheduleOffset(Integer scheduleOffset) {
this.scheduleOffset = scheduleOffset;
}
/**
*
* Number of days to wait after the scheduled day to run an association.
*
*
* @return Number of days to wait after the scheduled day to run an association.
*/
public Integer getScheduleOffset() {
return this.scheduleOffset;
}
/**
*
* Number of days to wait after the scheduled day to run an association.
*
*
* @param scheduleOffset
* Number of days to wait after the scheduled day to run an association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withScheduleOffset(Integer scheduleOffset) {
setScheduleOffset(scheduleOffset);
return this;
}
/**
*
* The number of hours that an association can run on specified targets. After the resulting cutoff time passes,
* associations that are currently running are cancelled, and no pending executions are started on remaining
* targets.
*
*
* @param duration
* The number of hours that an association can run on specified targets. After the resulting cutoff time
* passes, associations that are currently running are cancelled, and no pending executions are started on
* remaining targets.
*/
public void setDuration(Integer duration) {
this.duration = duration;
}
/**
*
* The number of hours that an association can run on specified targets. After the resulting cutoff time passes,
* associations that are currently running are cancelled, and no pending executions are started on remaining
* targets.
*
*
* @return The number of hours that an association can run on specified targets. After the resulting cutoff time
* passes, associations that are currently running are cancelled, and no pending executions are started on
* remaining targets.
*/
public Integer getDuration() {
return this.duration;
}
/**
*
* The number of hours that an association can run on specified targets. After the resulting cutoff time passes,
* associations that are currently running are cancelled, and no pending executions are started on remaining
* targets.
*
*
* @param duration
* The number of hours that an association can run on specified targets. After the resulting cutoff time
* passes, associations that are currently running are cancelled, and no pending executions are started on
* remaining targets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withDuration(Integer duration) {
setDuration(duration);
return this;
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* @return A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
*/
public java.util.List>> getTargetMaps() {
if (targetMaps == null) {
targetMaps = new com.amazonaws.internal.SdkInternalList>>();
}
return targetMaps;
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* @param targetMaps
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
*/
public void setTargetMaps(java.util.Collection>> targetMaps) {
if (targetMaps == null) {
this.targetMaps = null;
return;
}
this.targetMaps = new com.amazonaws.internal.SdkInternalList>>(targetMaps);
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetMaps(java.util.Collection)} or {@link #withTargetMaps(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param targetMaps
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withTargetMaps(java.util.Map>... targetMaps) {
if (this.targetMaps == null) {
setTargetMaps(new com.amazonaws.internal.SdkInternalList>>(targetMaps.length));
}
for (java.util.Map> ele : targetMaps) {
this.targetMaps.add(ele);
}
return this;
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* @param targetMaps
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withTargetMaps(java.util.Collection>> targetMaps) {
setTargetMaps(targetMaps);
return this;
}
/**
* @param alarmConfiguration
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
* @return
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
* @param alarmConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
*
* The CloudWatch alarm that was invoked during the association.
*
*
* @return The CloudWatch alarm that was invoked during the association.
*/
public java.util.List getTriggeredAlarms() {
if (triggeredAlarms == null) {
triggeredAlarms = new com.amazonaws.internal.SdkInternalList();
}
return triggeredAlarms;
}
/**
*
* The CloudWatch alarm that was invoked during the association.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked during the association.
*/
public void setTriggeredAlarms(java.util.Collection triggeredAlarms) {
if (triggeredAlarms == null) {
this.triggeredAlarms = null;
return;
}
this.triggeredAlarms = new com.amazonaws.internal.SdkInternalList(triggeredAlarms);
}
/**
*
* The CloudWatch alarm that was invoked during the association.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTriggeredAlarms(java.util.Collection)} or {@link #withTriggeredAlarms(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked during the association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withTriggeredAlarms(AlarmStateInformation... triggeredAlarms) {
if (this.triggeredAlarms == null) {
setTriggeredAlarms(new com.amazonaws.internal.SdkInternalList(triggeredAlarms.length));
}
for (AlarmStateInformation ele : triggeredAlarms) {
this.triggeredAlarms.add(ele);
}
return this;
}
/**
*
* The CloudWatch alarm that was invoked during the association.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked during the association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationDescription withTriggeredAlarms(java.util.Collection triggeredAlarms) {
setTriggeredAlarms(triggeredAlarms);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getInstanceId() != null)
sb.append("InstanceId: ").append(getInstanceId()).append(",");
if (getAssociationVersion() != null)
sb.append("AssociationVersion: ").append(getAssociationVersion()).append(",");
if (getDate() != null)
sb.append("Date: ").append(getDate()).append(",");
if (getLastUpdateAssociationDate() != null)
sb.append("LastUpdateAssociationDate: ").append(getLastUpdateAssociationDate()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getOverview() != null)
sb.append("Overview: ").append(getOverview()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getAutomationTargetParameterName() != null)
sb.append("AutomationTargetParameterName: ").append(getAutomationTargetParameterName()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append("***Sensitive Data Redacted***").append(",");
if (getAssociationId() != null)
sb.append("AssociationId: ").append(getAssociationId()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getScheduleExpression() != null)
sb.append("ScheduleExpression: ").append(getScheduleExpression()).append(",");
if (getOutputLocation() != null)
sb.append("OutputLocation: ").append(getOutputLocation()).append(",");
if (getLastExecutionDate() != null)
sb.append("LastExecutionDate: ").append(getLastExecutionDate()).append(",");
if (getLastSuccessfulExecutionDate() != null)
sb.append("LastSuccessfulExecutionDate: ").append(getLastSuccessfulExecutionDate()).append(",");
if (getAssociationName() != null)
sb.append("AssociationName: ").append(getAssociationName()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getComplianceSeverity() != null)
sb.append("ComplianceSeverity: ").append(getComplianceSeverity()).append(",");
if (getSyncCompliance() != null)
sb.append("SyncCompliance: ").append(getSyncCompliance()).append(",");
if (getApplyOnlyAtCronInterval() != null)
sb.append("ApplyOnlyAtCronInterval: ").append(getApplyOnlyAtCronInterval()).append(",");
if (getCalendarNames() != null)
sb.append("CalendarNames: ").append(getCalendarNames()).append(",");
if (getTargetLocations() != null)
sb.append("TargetLocations: ").append(getTargetLocations()).append(",");
if (getScheduleOffset() != null)
sb.append("ScheduleOffset: ").append(getScheduleOffset()).append(",");
if (getDuration() != null)
sb.append("Duration: ").append(getDuration()).append(",");
if (getTargetMaps() != null)
sb.append("TargetMaps: ").append(getTargetMaps()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration()).append(",");
if (getTriggeredAlarms() != null)
sb.append("TriggeredAlarms: ").append(getTriggeredAlarms());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AssociationDescription == false)
return false;
AssociationDescription other = (AssociationDescription) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getInstanceId() == null ^ this.getInstanceId() == null)
return false;
if (other.getInstanceId() != null && other.getInstanceId().equals(this.getInstanceId()) == false)
return false;
if (other.getAssociationVersion() == null ^ this.getAssociationVersion() == null)
return false;
if (other.getAssociationVersion() != null && other.getAssociationVersion().equals(this.getAssociationVersion()) == false)
return false;
if (other.getDate() == null ^ this.getDate() == null)
return false;
if (other.getDate() != null && other.getDate().equals(this.getDate()) == false)
return false;
if (other.getLastUpdateAssociationDate() == null ^ this.getLastUpdateAssociationDate() == null)
return false;
if (other.getLastUpdateAssociationDate() != null && other.getLastUpdateAssociationDate().equals(this.getLastUpdateAssociationDate()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getOverview() == null ^ this.getOverview() == null)
return false;
if (other.getOverview() != null && other.getOverview().equals(this.getOverview()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getAutomationTargetParameterName() == null ^ this.getAutomationTargetParameterName() == null)
return false;
if (other.getAutomationTargetParameterName() != null
&& other.getAutomationTargetParameterName().equals(this.getAutomationTargetParameterName()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getAssociationId() == null ^ this.getAssociationId() == null)
return false;
if (other.getAssociationId() != null && other.getAssociationId().equals(this.getAssociationId()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getScheduleExpression() == null ^ this.getScheduleExpression() == null)
return false;
if (other.getScheduleExpression() != null && other.getScheduleExpression().equals(this.getScheduleExpression()) == false)
return false;
if (other.getOutputLocation() == null ^ this.getOutputLocation() == null)
return false;
if (other.getOutputLocation() != null && other.getOutputLocation().equals(this.getOutputLocation()) == false)
return false;
if (other.getLastExecutionDate() == null ^ this.getLastExecutionDate() == null)
return false;
if (other.getLastExecutionDate() != null && other.getLastExecutionDate().equals(this.getLastExecutionDate()) == false)
return false;
if (other.getLastSuccessfulExecutionDate() == null ^ this.getLastSuccessfulExecutionDate() == null)
return false;
if (other.getLastSuccessfulExecutionDate() != null && other.getLastSuccessfulExecutionDate().equals(this.getLastSuccessfulExecutionDate()) == false)
return false;
if (other.getAssociationName() == null ^ this.getAssociationName() == null)
return false;
if (other.getAssociationName() != null && other.getAssociationName().equals(this.getAssociationName()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getComplianceSeverity() == null ^ this.getComplianceSeverity() == null)
return false;
if (other.getComplianceSeverity() != null && other.getComplianceSeverity().equals(this.getComplianceSeverity()) == false)
return false;
if (other.getSyncCompliance() == null ^ this.getSyncCompliance() == null)
return false;
if (other.getSyncCompliance() != null && other.getSyncCompliance().equals(this.getSyncCompliance()) == false)
return false;
if (other.getApplyOnlyAtCronInterval() == null ^ this.getApplyOnlyAtCronInterval() == null)
return false;
if (other.getApplyOnlyAtCronInterval() != null && other.getApplyOnlyAtCronInterval().equals(this.getApplyOnlyAtCronInterval()) == false)
return false;
if (other.getCalendarNames() == null ^ this.getCalendarNames() == null)
return false;
if (other.getCalendarNames() != null && other.getCalendarNames().equals(this.getCalendarNames()) == false)
return false;
if (other.getTargetLocations() == null ^ this.getTargetLocations() == null)
return false;
if (other.getTargetLocations() != null && other.getTargetLocations().equals(this.getTargetLocations()) == false)
return false;
if (other.getScheduleOffset() == null ^ this.getScheduleOffset() == null)
return false;
if (other.getScheduleOffset() != null && other.getScheduleOffset().equals(this.getScheduleOffset()) == false)
return false;
if (other.getDuration() == null ^ this.getDuration() == null)
return false;
if (other.getDuration() != null && other.getDuration().equals(this.getDuration()) == false)
return false;
if (other.getTargetMaps() == null ^ this.getTargetMaps() == null)
return false;
if (other.getTargetMaps() != null && other.getTargetMaps().equals(this.getTargetMaps()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
if (other.getTriggeredAlarms() == null ^ this.getTriggeredAlarms() == null)
return false;
if (other.getTriggeredAlarms() != null && other.getTriggeredAlarms().equals(this.getTriggeredAlarms()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getInstanceId() == null) ? 0 : getInstanceId().hashCode());
hashCode = prime * hashCode + ((getAssociationVersion() == null) ? 0 : getAssociationVersion().hashCode());
hashCode = prime * hashCode + ((getDate() == null) ? 0 : getDate().hashCode());
hashCode = prime * hashCode + ((getLastUpdateAssociationDate() == null) ? 0 : getLastUpdateAssociationDate().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getOverview() == null) ? 0 : getOverview().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getAutomationTargetParameterName() == null) ? 0 : getAutomationTargetParameterName().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getAssociationId() == null) ? 0 : getAssociationId().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getScheduleExpression() == null) ? 0 : getScheduleExpression().hashCode());
hashCode = prime * hashCode + ((getOutputLocation() == null) ? 0 : getOutputLocation().hashCode());
hashCode = prime * hashCode + ((getLastExecutionDate() == null) ? 0 : getLastExecutionDate().hashCode());
hashCode = prime * hashCode + ((getLastSuccessfulExecutionDate() == null) ? 0 : getLastSuccessfulExecutionDate().hashCode());
hashCode = prime * hashCode + ((getAssociationName() == null) ? 0 : getAssociationName().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getComplianceSeverity() == null) ? 0 : getComplianceSeverity().hashCode());
hashCode = prime * hashCode + ((getSyncCompliance() == null) ? 0 : getSyncCompliance().hashCode());
hashCode = prime * hashCode + ((getApplyOnlyAtCronInterval() == null) ? 0 : getApplyOnlyAtCronInterval().hashCode());
hashCode = prime * hashCode + ((getCalendarNames() == null) ? 0 : getCalendarNames().hashCode());
hashCode = prime * hashCode + ((getTargetLocations() == null) ? 0 : getTargetLocations().hashCode());
hashCode = prime * hashCode + ((getScheduleOffset() == null) ? 0 : getScheduleOffset().hashCode());
hashCode = prime * hashCode + ((getDuration() == null) ? 0 : getDuration().hashCode());
hashCode = prime * hashCode + ((getTargetMaps() == null) ? 0 : getTargetMaps().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
hashCode = prime * hashCode + ((getTriggeredAlarms() == null) ? 0 : getTriggeredAlarms().hashCode());
return hashCode;
}
@Override
public AssociationDescription clone() {
try {
return (AssociationDescription) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.AssociationDescriptionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}