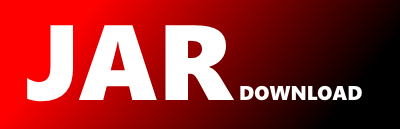
com.amazonaws.services.simplesystemsmanagement.model.AssociationExecution Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Includes information about the specified association.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AssociationExecution implements Serializable, Cloneable, StructuredPojo {
/**
*
* The association ID.
*
*/
private String associationId;
/**
*
* The association version.
*
*/
private String associationVersion;
/**
*
* The execution ID for the association.
*
*/
private String executionId;
/**
*
* The status of the association execution.
*
*/
private String status;
/**
*
* Detailed status information about the execution.
*
*/
private String detailedStatus;
/**
*
* The time the execution started.
*
*/
private java.util.Date createdTime;
/**
*
* The date of the last execution.
*
*/
private java.util.Date lastExecutionDate;
/**
*
* An aggregate status of the resources in the execution based on the status type.
*
*/
private String resourceCountByStatus;
private AlarmConfiguration alarmConfiguration;
/**
*
* The CloudWatch alarms that were invoked by the association.
*
*/
private com.amazonaws.internal.SdkInternalList triggeredAlarms;
/**
*
* The association ID.
*
*
* @param associationId
* The association ID.
*/
public void setAssociationId(String associationId) {
this.associationId = associationId;
}
/**
*
* The association ID.
*
*
* @return The association ID.
*/
public String getAssociationId() {
return this.associationId;
}
/**
*
* The association ID.
*
*
* @param associationId
* The association ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withAssociationId(String associationId) {
setAssociationId(associationId);
return this;
}
/**
*
* The association version.
*
*
* @param associationVersion
* The association version.
*/
public void setAssociationVersion(String associationVersion) {
this.associationVersion = associationVersion;
}
/**
*
* The association version.
*
*
* @return The association version.
*/
public String getAssociationVersion() {
return this.associationVersion;
}
/**
*
* The association version.
*
*
* @param associationVersion
* The association version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withAssociationVersion(String associationVersion) {
setAssociationVersion(associationVersion);
return this;
}
/**
*
* The execution ID for the association.
*
*
* @param executionId
* The execution ID for the association.
*/
public void setExecutionId(String executionId) {
this.executionId = executionId;
}
/**
*
* The execution ID for the association.
*
*
* @return The execution ID for the association.
*/
public String getExecutionId() {
return this.executionId;
}
/**
*
* The execution ID for the association.
*
*
* @param executionId
* The execution ID for the association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withExecutionId(String executionId) {
setExecutionId(executionId);
return this;
}
/**
*
* The status of the association execution.
*
*
* @param status
* The status of the association execution.
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the association execution.
*
*
* @return The status of the association execution.
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the association execution.
*
*
* @param status
* The status of the association execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Detailed status information about the execution.
*
*
* @param detailedStatus
* Detailed status information about the execution.
*/
public void setDetailedStatus(String detailedStatus) {
this.detailedStatus = detailedStatus;
}
/**
*
* Detailed status information about the execution.
*
*
* @return Detailed status information about the execution.
*/
public String getDetailedStatus() {
return this.detailedStatus;
}
/**
*
* Detailed status information about the execution.
*
*
* @param detailedStatus
* Detailed status information about the execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withDetailedStatus(String detailedStatus) {
setDetailedStatus(detailedStatus);
return this;
}
/**
*
* The time the execution started.
*
*
* @param createdTime
* The time the execution started.
*/
public void setCreatedTime(java.util.Date createdTime) {
this.createdTime = createdTime;
}
/**
*
* The time the execution started.
*
*
* @return The time the execution started.
*/
public java.util.Date getCreatedTime() {
return this.createdTime;
}
/**
*
* The time the execution started.
*
*
* @param createdTime
* The time the execution started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withCreatedTime(java.util.Date createdTime) {
setCreatedTime(createdTime);
return this;
}
/**
*
* The date of the last execution.
*
*
* @param lastExecutionDate
* The date of the last execution.
*/
public void setLastExecutionDate(java.util.Date lastExecutionDate) {
this.lastExecutionDate = lastExecutionDate;
}
/**
*
* The date of the last execution.
*
*
* @return The date of the last execution.
*/
public java.util.Date getLastExecutionDate() {
return this.lastExecutionDate;
}
/**
*
* The date of the last execution.
*
*
* @param lastExecutionDate
* The date of the last execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withLastExecutionDate(java.util.Date lastExecutionDate) {
setLastExecutionDate(lastExecutionDate);
return this;
}
/**
*
* An aggregate status of the resources in the execution based on the status type.
*
*
* @param resourceCountByStatus
* An aggregate status of the resources in the execution based on the status type.
*/
public void setResourceCountByStatus(String resourceCountByStatus) {
this.resourceCountByStatus = resourceCountByStatus;
}
/**
*
* An aggregate status of the resources in the execution based on the status type.
*
*
* @return An aggregate status of the resources in the execution based on the status type.
*/
public String getResourceCountByStatus() {
return this.resourceCountByStatus;
}
/**
*
* An aggregate status of the resources in the execution based on the status type.
*
*
* @param resourceCountByStatus
* An aggregate status of the resources in the execution based on the status type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withResourceCountByStatus(String resourceCountByStatus) {
setResourceCountByStatus(resourceCountByStatus);
return this;
}
/**
* @param alarmConfiguration
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
* @return
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
* @param alarmConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
*
* The CloudWatch alarms that were invoked by the association.
*
*
* @return The CloudWatch alarms that were invoked by the association.
*/
public java.util.List getTriggeredAlarms() {
if (triggeredAlarms == null) {
triggeredAlarms = new com.amazonaws.internal.SdkInternalList();
}
return triggeredAlarms;
}
/**
*
* The CloudWatch alarms that were invoked by the association.
*
*
* @param triggeredAlarms
* The CloudWatch alarms that were invoked by the association.
*/
public void setTriggeredAlarms(java.util.Collection triggeredAlarms) {
if (triggeredAlarms == null) {
this.triggeredAlarms = null;
return;
}
this.triggeredAlarms = new com.amazonaws.internal.SdkInternalList(triggeredAlarms);
}
/**
*
* The CloudWatch alarms that were invoked by the association.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTriggeredAlarms(java.util.Collection)} or {@link #withTriggeredAlarms(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param triggeredAlarms
* The CloudWatch alarms that were invoked by the association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withTriggeredAlarms(AlarmStateInformation... triggeredAlarms) {
if (this.triggeredAlarms == null) {
setTriggeredAlarms(new com.amazonaws.internal.SdkInternalList(triggeredAlarms.length));
}
for (AlarmStateInformation ele : triggeredAlarms) {
this.triggeredAlarms.add(ele);
}
return this;
}
/**
*
* The CloudWatch alarms that were invoked by the association.
*
*
* @param triggeredAlarms
* The CloudWatch alarms that were invoked by the association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociationExecution withTriggeredAlarms(java.util.Collection triggeredAlarms) {
setTriggeredAlarms(triggeredAlarms);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAssociationId() != null)
sb.append("AssociationId: ").append(getAssociationId()).append(",");
if (getAssociationVersion() != null)
sb.append("AssociationVersion: ").append(getAssociationVersion()).append(",");
if (getExecutionId() != null)
sb.append("ExecutionId: ").append(getExecutionId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getDetailedStatus() != null)
sb.append("DetailedStatus: ").append(getDetailedStatus()).append(",");
if (getCreatedTime() != null)
sb.append("CreatedTime: ").append(getCreatedTime()).append(",");
if (getLastExecutionDate() != null)
sb.append("LastExecutionDate: ").append(getLastExecutionDate()).append(",");
if (getResourceCountByStatus() != null)
sb.append("ResourceCountByStatus: ").append(getResourceCountByStatus()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration()).append(",");
if (getTriggeredAlarms() != null)
sb.append("TriggeredAlarms: ").append(getTriggeredAlarms());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AssociationExecution == false)
return false;
AssociationExecution other = (AssociationExecution) obj;
if (other.getAssociationId() == null ^ this.getAssociationId() == null)
return false;
if (other.getAssociationId() != null && other.getAssociationId().equals(this.getAssociationId()) == false)
return false;
if (other.getAssociationVersion() == null ^ this.getAssociationVersion() == null)
return false;
if (other.getAssociationVersion() != null && other.getAssociationVersion().equals(this.getAssociationVersion()) == false)
return false;
if (other.getExecutionId() == null ^ this.getExecutionId() == null)
return false;
if (other.getExecutionId() != null && other.getExecutionId().equals(this.getExecutionId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getDetailedStatus() == null ^ this.getDetailedStatus() == null)
return false;
if (other.getDetailedStatus() != null && other.getDetailedStatus().equals(this.getDetailedStatus()) == false)
return false;
if (other.getCreatedTime() == null ^ this.getCreatedTime() == null)
return false;
if (other.getCreatedTime() != null && other.getCreatedTime().equals(this.getCreatedTime()) == false)
return false;
if (other.getLastExecutionDate() == null ^ this.getLastExecutionDate() == null)
return false;
if (other.getLastExecutionDate() != null && other.getLastExecutionDate().equals(this.getLastExecutionDate()) == false)
return false;
if (other.getResourceCountByStatus() == null ^ this.getResourceCountByStatus() == null)
return false;
if (other.getResourceCountByStatus() != null && other.getResourceCountByStatus().equals(this.getResourceCountByStatus()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
if (other.getTriggeredAlarms() == null ^ this.getTriggeredAlarms() == null)
return false;
if (other.getTriggeredAlarms() != null && other.getTriggeredAlarms().equals(this.getTriggeredAlarms()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAssociationId() == null) ? 0 : getAssociationId().hashCode());
hashCode = prime * hashCode + ((getAssociationVersion() == null) ? 0 : getAssociationVersion().hashCode());
hashCode = prime * hashCode + ((getExecutionId() == null) ? 0 : getExecutionId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getDetailedStatus() == null) ? 0 : getDetailedStatus().hashCode());
hashCode = prime * hashCode + ((getCreatedTime() == null) ? 0 : getCreatedTime().hashCode());
hashCode = prime * hashCode + ((getLastExecutionDate() == null) ? 0 : getLastExecutionDate().hashCode());
hashCode = prime * hashCode + ((getResourceCountByStatus() == null) ? 0 : getResourceCountByStatus().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
hashCode = prime * hashCode + ((getTriggeredAlarms() == null) ? 0 : getTriggeredAlarms().hashCode());
return hashCode;
}
@Override
public AssociationExecution clone() {
try {
return (AssociationExecution) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.AssociationExecutionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}