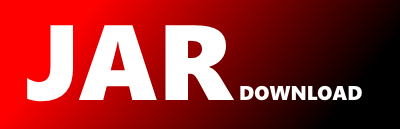
com.amazonaws.services.simplesystemsmanagement.model.AutomationExecution Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Detailed information about the current state of an individual Automation execution.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AutomationExecution implements Serializable, Cloneable, StructuredPojo {
/**
*
* The execution ID.
*
*/
private String automationExecutionId;
/**
*
* The name of the Automation runbook used during the execution.
*
*/
private String documentName;
/**
*
* The version of the document to use during execution.
*
*/
private String documentVersion;
/**
*
* The time the execution started.
*
*/
private java.util.Date executionStartTime;
/**
*
* The time the execution finished.
*
*/
private java.util.Date executionEndTime;
/**
*
* The execution status of the Automation.
*
*/
private String automationExecutionStatus;
/**
*
* A list of details about the current state of all steps that comprise an execution. An Automation runbook contains
* a list of steps that are run in order.
*
*/
private com.amazonaws.internal.SdkInternalList stepExecutions;
/**
*
* A boolean value that indicates if the response contains the full list of the Automation step executions. If true,
* use the DescribeAutomationStepExecutions API operation to get the full list of step executions.
*
*/
private Boolean stepExecutionsTruncated;
/**
*
* The key-value map of execution parameters, which were supplied when calling StartAutomationExecution.
*
*/
private java.util.Map> parameters;
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*/
private java.util.Map> outputs;
/**
*
* A message describing why an execution has failed, if the status is set to Failed.
*
*/
private String failureMessage;
/**
*
* The automation execution mode.
*
*/
private String mode;
/**
*
* The AutomationExecutionId of the parent automation.
*
*/
private String parentAutomationExecutionId;
/**
*
* The Amazon Resource Name (ARN) of the user who ran the automation.
*
*/
private String executedBy;
/**
*
* The name of the step that is currently running.
*
*/
private String currentStepName;
/**
*
* The action of the step that is currently running.
*
*/
private String currentAction;
/**
*
* The parameter name.
*
*/
private String targetParameterName;
/**
*
* The specified targets.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*/
private com.amazonaws.internal.SdkInternalList>> targetMaps;
/**
*
* A list of resolved targets in the rate control execution.
*
*/
private ResolvedTargets resolvedTargets;
/**
*
* The MaxConcurrency
value specified by the user when the execution started.
*
*/
private String maxConcurrency;
/**
*
* The MaxErrors value specified by the user when the execution started.
*
*/
private String maxErrors;
/**
*
* The target of the execution.
*
*/
private String target;
/**
*
* The combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to run the
* Automation.
*
*/
private com.amazonaws.internal.SdkInternalList targetLocations;
/**
*
* An aggregate of step execution statuses displayed in the Amazon Web Services Systems Manager console for a
* multi-Region and multi-account Automation execution.
*
*/
private ProgressCounters progressCounters;
/**
*
* The details for the CloudWatch alarm applied to your automation.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*/
private com.amazonaws.internal.SdkInternalList triggeredAlarms;
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*/
private String automationSubtype;
/**
*
* The date and time the Automation operation is scheduled to start.
*
*/
private java.util.Date scheduledTime;
/**
*
* Information about the Automation runbooks that are run as part of a runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*/
private com.amazonaws.internal.SdkInternalList runbooks;
/**
*
* The ID of an OpsItem that is created to represent a Change Manager change request.
*
*/
private String opsItemId;
/**
*
* The ID of a State Manager association used in the Automation operation.
*
*/
private String associationId;
/**
*
* The name of the Change Manager change request.
*
*/
private String changeRequestName;
/**
*
* Variables defined for the automation.
*
*/
private java.util.Map> variables;
/**
*
* The execution ID.
*
*
* @param automationExecutionId
* The execution ID.
*/
public void setAutomationExecutionId(String automationExecutionId) {
this.automationExecutionId = automationExecutionId;
}
/**
*
* The execution ID.
*
*
* @return The execution ID.
*/
public String getAutomationExecutionId() {
return this.automationExecutionId;
}
/**
*
* The execution ID.
*
*
* @param automationExecutionId
* The execution ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withAutomationExecutionId(String automationExecutionId) {
setAutomationExecutionId(automationExecutionId);
return this;
}
/**
*
* The name of the Automation runbook used during the execution.
*
*
* @param documentName
* The name of the Automation runbook used during the execution.
*/
public void setDocumentName(String documentName) {
this.documentName = documentName;
}
/**
*
* The name of the Automation runbook used during the execution.
*
*
* @return The name of the Automation runbook used during the execution.
*/
public String getDocumentName() {
return this.documentName;
}
/**
*
* The name of the Automation runbook used during the execution.
*
*
* @param documentName
* The name of the Automation runbook used during the execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withDocumentName(String documentName) {
setDocumentName(documentName);
return this;
}
/**
*
* The version of the document to use during execution.
*
*
* @param documentVersion
* The version of the document to use during execution.
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The version of the document to use during execution.
*
*
* @return The version of the document to use during execution.
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The version of the document to use during execution.
*
*
* @param documentVersion
* The version of the document to use during execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* The time the execution started.
*
*
* @param executionStartTime
* The time the execution started.
*/
public void setExecutionStartTime(java.util.Date executionStartTime) {
this.executionStartTime = executionStartTime;
}
/**
*
* The time the execution started.
*
*
* @return The time the execution started.
*/
public java.util.Date getExecutionStartTime() {
return this.executionStartTime;
}
/**
*
* The time the execution started.
*
*
* @param executionStartTime
* The time the execution started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withExecutionStartTime(java.util.Date executionStartTime) {
setExecutionStartTime(executionStartTime);
return this;
}
/**
*
* The time the execution finished.
*
*
* @param executionEndTime
* The time the execution finished.
*/
public void setExecutionEndTime(java.util.Date executionEndTime) {
this.executionEndTime = executionEndTime;
}
/**
*
* The time the execution finished.
*
*
* @return The time the execution finished.
*/
public java.util.Date getExecutionEndTime() {
return this.executionEndTime;
}
/**
*
* The time the execution finished.
*
*
* @param executionEndTime
* The time the execution finished.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withExecutionEndTime(java.util.Date executionEndTime) {
setExecutionEndTime(executionEndTime);
return this;
}
/**
*
* The execution status of the Automation.
*
*
* @param automationExecutionStatus
* The execution status of the Automation.
* @see AutomationExecutionStatus
*/
public void setAutomationExecutionStatus(String automationExecutionStatus) {
this.automationExecutionStatus = automationExecutionStatus;
}
/**
*
* The execution status of the Automation.
*
*
* @return The execution status of the Automation.
* @see AutomationExecutionStatus
*/
public String getAutomationExecutionStatus() {
return this.automationExecutionStatus;
}
/**
*
* The execution status of the Automation.
*
*
* @param automationExecutionStatus
* The execution status of the Automation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationExecutionStatus
*/
public AutomationExecution withAutomationExecutionStatus(String automationExecutionStatus) {
setAutomationExecutionStatus(automationExecutionStatus);
return this;
}
/**
*
* The execution status of the Automation.
*
*
* @param automationExecutionStatus
* The execution status of the Automation.
* @see AutomationExecutionStatus
*/
public void setAutomationExecutionStatus(AutomationExecutionStatus automationExecutionStatus) {
withAutomationExecutionStatus(automationExecutionStatus);
}
/**
*
* The execution status of the Automation.
*
*
* @param automationExecutionStatus
* The execution status of the Automation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationExecutionStatus
*/
public AutomationExecution withAutomationExecutionStatus(AutomationExecutionStatus automationExecutionStatus) {
this.automationExecutionStatus = automationExecutionStatus.toString();
return this;
}
/**
*
* A list of details about the current state of all steps that comprise an execution. An Automation runbook contains
* a list of steps that are run in order.
*
*
* @return A list of details about the current state of all steps that comprise an execution. An Automation runbook
* contains a list of steps that are run in order.
*/
public java.util.List getStepExecutions() {
if (stepExecutions == null) {
stepExecutions = new com.amazonaws.internal.SdkInternalList();
}
return stepExecutions;
}
/**
*
* A list of details about the current state of all steps that comprise an execution. An Automation runbook contains
* a list of steps that are run in order.
*
*
* @param stepExecutions
* A list of details about the current state of all steps that comprise an execution. An Automation runbook
* contains a list of steps that are run in order.
*/
public void setStepExecutions(java.util.Collection stepExecutions) {
if (stepExecutions == null) {
this.stepExecutions = null;
return;
}
this.stepExecutions = new com.amazonaws.internal.SdkInternalList(stepExecutions);
}
/**
*
* A list of details about the current state of all steps that comprise an execution. An Automation runbook contains
* a list of steps that are run in order.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStepExecutions(java.util.Collection)} or {@link #withStepExecutions(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param stepExecutions
* A list of details about the current state of all steps that comprise an execution. An Automation runbook
* contains a list of steps that are run in order.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withStepExecutions(StepExecution... stepExecutions) {
if (this.stepExecutions == null) {
setStepExecutions(new com.amazonaws.internal.SdkInternalList(stepExecutions.length));
}
for (StepExecution ele : stepExecutions) {
this.stepExecutions.add(ele);
}
return this;
}
/**
*
* A list of details about the current state of all steps that comprise an execution. An Automation runbook contains
* a list of steps that are run in order.
*
*
* @param stepExecutions
* A list of details about the current state of all steps that comprise an execution. An Automation runbook
* contains a list of steps that are run in order.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withStepExecutions(java.util.Collection stepExecutions) {
setStepExecutions(stepExecutions);
return this;
}
/**
*
* A boolean value that indicates if the response contains the full list of the Automation step executions. If true,
* use the DescribeAutomationStepExecutions API operation to get the full list of step executions.
*
*
* @param stepExecutionsTruncated
* A boolean value that indicates if the response contains the full list of the Automation step executions.
* If true, use the DescribeAutomationStepExecutions API operation to get the full list of step executions.
*/
public void setStepExecutionsTruncated(Boolean stepExecutionsTruncated) {
this.stepExecutionsTruncated = stepExecutionsTruncated;
}
/**
*
* A boolean value that indicates if the response contains the full list of the Automation step executions. If true,
* use the DescribeAutomationStepExecutions API operation to get the full list of step executions.
*
*
* @return A boolean value that indicates if the response contains the full list of the Automation step executions.
* If true, use the DescribeAutomationStepExecutions API operation to get the full list of step executions.
*/
public Boolean getStepExecutionsTruncated() {
return this.stepExecutionsTruncated;
}
/**
*
* A boolean value that indicates if the response contains the full list of the Automation step executions. If true,
* use the DescribeAutomationStepExecutions API operation to get the full list of step executions.
*
*
* @param stepExecutionsTruncated
* A boolean value that indicates if the response contains the full list of the Automation step executions.
* If true, use the DescribeAutomationStepExecutions API operation to get the full list of step executions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withStepExecutionsTruncated(Boolean stepExecutionsTruncated) {
setStepExecutionsTruncated(stepExecutionsTruncated);
return this;
}
/**
*
* A boolean value that indicates if the response contains the full list of the Automation step executions. If true,
* use the DescribeAutomationStepExecutions API operation to get the full list of step executions.
*
*
* @return A boolean value that indicates if the response contains the full list of the Automation step executions.
* If true, use the DescribeAutomationStepExecutions API operation to get the full list of step executions.
*/
public Boolean isStepExecutionsTruncated() {
return this.stepExecutionsTruncated;
}
/**
*
* The key-value map of execution parameters, which were supplied when calling StartAutomationExecution.
*
*
* @return The key-value map of execution parameters, which were supplied when calling
* StartAutomationExecution.
*/
public java.util.Map> getParameters() {
return parameters;
}
/**
*
* The key-value map of execution parameters, which were supplied when calling StartAutomationExecution.
*
*
* @param parameters
* The key-value map of execution parameters, which were supplied when calling
* StartAutomationExecution.
*/
public void setParameters(java.util.Map> parameters) {
this.parameters = parameters;
}
/**
*
* The key-value map of execution parameters, which were supplied when calling StartAutomationExecution.
*
*
* @param parameters
* The key-value map of execution parameters, which were supplied when calling
* StartAutomationExecution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withParameters(java.util.Map> parameters) {
setParameters(parameters);
return this;
}
/**
* Add a single Parameters entry
*
* @see AutomationExecution#withParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution addParametersEntry(String key, java.util.List value) {
if (null == this.parameters) {
this.parameters = new java.util.HashMap>();
}
if (this.parameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.parameters.put(key, value);
return this;
}
/**
* Removes all the entries added into Parameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution clearParametersEntries() {
this.parameters = null;
return this;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @return The list of execution outputs as defined in the Automation runbook.
*/
public java.util.Map> getOutputs() {
return outputs;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param outputs
* The list of execution outputs as defined in the Automation runbook.
*/
public void setOutputs(java.util.Map> outputs) {
this.outputs = outputs;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param outputs
* The list of execution outputs as defined in the Automation runbook.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withOutputs(java.util.Map> outputs) {
setOutputs(outputs);
return this;
}
/**
* Add a single Outputs entry
*
* @see AutomationExecution#withOutputs
* @returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution addOutputsEntry(String key, java.util.List value) {
if (null == this.outputs) {
this.outputs = new java.util.HashMap>();
}
if (this.outputs.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.outputs.put(key, value);
return this;
}
/**
* Removes all the entries added into Outputs.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution clearOutputsEntries() {
this.outputs = null;
return this;
}
/**
*
* A message describing why an execution has failed, if the status is set to Failed.
*
*
* @param failureMessage
* A message describing why an execution has failed, if the status is set to Failed.
*/
public void setFailureMessage(String failureMessage) {
this.failureMessage = failureMessage;
}
/**
*
* A message describing why an execution has failed, if the status is set to Failed.
*
*
* @return A message describing why an execution has failed, if the status is set to Failed.
*/
public String getFailureMessage() {
return this.failureMessage;
}
/**
*
* A message describing why an execution has failed, if the status is set to Failed.
*
*
* @param failureMessage
* A message describing why an execution has failed, if the status is set to Failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withFailureMessage(String failureMessage) {
setFailureMessage(failureMessage);
return this;
}
/**
*
* The automation execution mode.
*
*
* @param mode
* The automation execution mode.
* @see ExecutionMode
*/
public void setMode(String mode) {
this.mode = mode;
}
/**
*
* The automation execution mode.
*
*
* @return The automation execution mode.
* @see ExecutionMode
*/
public String getMode() {
return this.mode;
}
/**
*
* The automation execution mode.
*
*
* @param mode
* The automation execution mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionMode
*/
public AutomationExecution withMode(String mode) {
setMode(mode);
return this;
}
/**
*
* The automation execution mode.
*
*
* @param mode
* The automation execution mode.
* @see ExecutionMode
*/
public void setMode(ExecutionMode mode) {
withMode(mode);
}
/**
*
* The automation execution mode.
*
*
* @param mode
* The automation execution mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionMode
*/
public AutomationExecution withMode(ExecutionMode mode) {
this.mode = mode.toString();
return this;
}
/**
*
* The AutomationExecutionId of the parent automation.
*
*
* @param parentAutomationExecutionId
* The AutomationExecutionId of the parent automation.
*/
public void setParentAutomationExecutionId(String parentAutomationExecutionId) {
this.parentAutomationExecutionId = parentAutomationExecutionId;
}
/**
*
* The AutomationExecutionId of the parent automation.
*
*
* @return The AutomationExecutionId of the parent automation.
*/
public String getParentAutomationExecutionId() {
return this.parentAutomationExecutionId;
}
/**
*
* The AutomationExecutionId of the parent automation.
*
*
* @param parentAutomationExecutionId
* The AutomationExecutionId of the parent automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withParentAutomationExecutionId(String parentAutomationExecutionId) {
setParentAutomationExecutionId(parentAutomationExecutionId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the user who ran the automation.
*
*
* @param executedBy
* The Amazon Resource Name (ARN) of the user who ran the automation.
*/
public void setExecutedBy(String executedBy) {
this.executedBy = executedBy;
}
/**
*
* The Amazon Resource Name (ARN) of the user who ran the automation.
*
*
* @return The Amazon Resource Name (ARN) of the user who ran the automation.
*/
public String getExecutedBy() {
return this.executedBy;
}
/**
*
* The Amazon Resource Name (ARN) of the user who ran the automation.
*
*
* @param executedBy
* The Amazon Resource Name (ARN) of the user who ran the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withExecutedBy(String executedBy) {
setExecutedBy(executedBy);
return this;
}
/**
*
* The name of the step that is currently running.
*
*
* @param currentStepName
* The name of the step that is currently running.
*/
public void setCurrentStepName(String currentStepName) {
this.currentStepName = currentStepName;
}
/**
*
* The name of the step that is currently running.
*
*
* @return The name of the step that is currently running.
*/
public String getCurrentStepName() {
return this.currentStepName;
}
/**
*
* The name of the step that is currently running.
*
*
* @param currentStepName
* The name of the step that is currently running.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withCurrentStepName(String currentStepName) {
setCurrentStepName(currentStepName);
return this;
}
/**
*
* The action of the step that is currently running.
*
*
* @param currentAction
* The action of the step that is currently running.
*/
public void setCurrentAction(String currentAction) {
this.currentAction = currentAction;
}
/**
*
* The action of the step that is currently running.
*
*
* @return The action of the step that is currently running.
*/
public String getCurrentAction() {
return this.currentAction;
}
/**
*
* The action of the step that is currently running.
*
*
* @param currentAction
* The action of the step that is currently running.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withCurrentAction(String currentAction) {
setCurrentAction(currentAction);
return this;
}
/**
*
* The parameter name.
*
*
* @param targetParameterName
* The parameter name.
*/
public void setTargetParameterName(String targetParameterName) {
this.targetParameterName = targetParameterName;
}
/**
*
* The parameter name.
*
*
* @return The parameter name.
*/
public String getTargetParameterName() {
return this.targetParameterName;
}
/**
*
* The parameter name.
*
*
* @param targetParameterName
* The parameter name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTargetParameterName(String targetParameterName) {
setTargetParameterName(targetParameterName);
return this;
}
/**
*
* The specified targets.
*
*
* @return The specified targets.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The specified targets.
*
*
* @param targets
* The specified targets.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The specified targets.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The specified targets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The specified targets.
*
*
* @param targets
* The specified targets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*
* @return The specified key-value mapping of document parameters to target resources.
*/
public java.util.List>> getTargetMaps() {
if (targetMaps == null) {
targetMaps = new com.amazonaws.internal.SdkInternalList>>();
}
return targetMaps;
}
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*
* @param targetMaps
* The specified key-value mapping of document parameters to target resources.
*/
public void setTargetMaps(java.util.Collection>> targetMaps) {
if (targetMaps == null) {
this.targetMaps = null;
return;
}
this.targetMaps = new com.amazonaws.internal.SdkInternalList>>(targetMaps);
}
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetMaps(java.util.Collection)} or {@link #withTargetMaps(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param targetMaps
* The specified key-value mapping of document parameters to target resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTargetMaps(java.util.Map>... targetMaps) {
if (this.targetMaps == null) {
setTargetMaps(new com.amazonaws.internal.SdkInternalList>>(targetMaps.length));
}
for (java.util.Map> ele : targetMaps) {
this.targetMaps.add(ele);
}
return this;
}
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*
* @param targetMaps
* The specified key-value mapping of document parameters to target resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTargetMaps(java.util.Collection>> targetMaps) {
setTargetMaps(targetMaps);
return this;
}
/**
*
* A list of resolved targets in the rate control execution.
*
*
* @param resolvedTargets
* A list of resolved targets in the rate control execution.
*/
public void setResolvedTargets(ResolvedTargets resolvedTargets) {
this.resolvedTargets = resolvedTargets;
}
/**
*
* A list of resolved targets in the rate control execution.
*
*
* @return A list of resolved targets in the rate control execution.
*/
public ResolvedTargets getResolvedTargets() {
return this.resolvedTargets;
}
/**
*
* A list of resolved targets in the rate control execution.
*
*
* @param resolvedTargets
* A list of resolved targets in the rate control execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withResolvedTargets(ResolvedTargets resolvedTargets) {
setResolvedTargets(resolvedTargets);
return this;
}
/**
*
* The MaxConcurrency
value specified by the user when the execution started.
*
*
* @param maxConcurrency
* The MaxConcurrency
value specified by the user when the execution started.
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The MaxConcurrency
value specified by the user when the execution started.
*
*
* @return The MaxConcurrency
value specified by the user when the execution started.
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The MaxConcurrency
value specified by the user when the execution started.
*
*
* @param maxConcurrency
* The MaxConcurrency
value specified by the user when the execution started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The MaxErrors value specified by the user when the execution started.
*
*
* @param maxErrors
* The MaxErrors value specified by the user when the execution started.
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The MaxErrors value specified by the user when the execution started.
*
*
* @return The MaxErrors value specified by the user when the execution started.
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The MaxErrors value specified by the user when the execution started.
*
*
* @param maxErrors
* The MaxErrors value specified by the user when the execution started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The target of the execution.
*
*
* @param target
* The target of the execution.
*/
public void setTarget(String target) {
this.target = target;
}
/**
*
* The target of the execution.
*
*
* @return The target of the execution.
*/
public String getTarget() {
return this.target;
}
/**
*
* The target of the execution.
*
*
* @param target
* The target of the execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTarget(String target) {
setTarget(target);
return this;
}
/**
*
* The combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to run the
* Automation.
*
*
* @return The combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to run
* the Automation.
*/
public java.util.List getTargetLocations() {
if (targetLocations == null) {
targetLocations = new com.amazonaws.internal.SdkInternalList();
}
return targetLocations;
}
/**
*
* The combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to run the
* Automation.
*
*
* @param targetLocations
* The combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to run
* the Automation.
*/
public void setTargetLocations(java.util.Collection targetLocations) {
if (targetLocations == null) {
this.targetLocations = null;
return;
}
this.targetLocations = new com.amazonaws.internal.SdkInternalList(targetLocations);
}
/**
*
* The combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to run the
* Automation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetLocations(java.util.Collection)} or {@link #withTargetLocations(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param targetLocations
* The combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to run
* the Automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTargetLocations(TargetLocation... targetLocations) {
if (this.targetLocations == null) {
setTargetLocations(new com.amazonaws.internal.SdkInternalList(targetLocations.length));
}
for (TargetLocation ele : targetLocations) {
this.targetLocations.add(ele);
}
return this;
}
/**
*
* The combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to run the
* Automation.
*
*
* @param targetLocations
* The combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to run
* the Automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTargetLocations(java.util.Collection targetLocations) {
setTargetLocations(targetLocations);
return this;
}
/**
*
* An aggregate of step execution statuses displayed in the Amazon Web Services Systems Manager console for a
* multi-Region and multi-account Automation execution.
*
*
* @param progressCounters
* An aggregate of step execution statuses displayed in the Amazon Web Services Systems Manager console for a
* multi-Region and multi-account Automation execution.
*/
public void setProgressCounters(ProgressCounters progressCounters) {
this.progressCounters = progressCounters;
}
/**
*
* An aggregate of step execution statuses displayed in the Amazon Web Services Systems Manager console for a
* multi-Region and multi-account Automation execution.
*
*
* @return An aggregate of step execution statuses displayed in the Amazon Web Services Systems Manager console for
* a multi-Region and multi-account Automation execution.
*/
public ProgressCounters getProgressCounters() {
return this.progressCounters;
}
/**
*
* An aggregate of step execution statuses displayed in the Amazon Web Services Systems Manager console for a
* multi-Region and multi-account Automation execution.
*
*
* @param progressCounters
* An aggregate of step execution statuses displayed in the Amazon Web Services Systems Manager console for a
* multi-Region and multi-account Automation execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withProgressCounters(ProgressCounters progressCounters) {
setProgressCounters(progressCounters);
return this;
}
/**
*
* The details for the CloudWatch alarm applied to your automation.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm applied to your automation.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm applied to your automation.
*
*
* @return The details for the CloudWatch alarm applied to your automation.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm applied to your automation.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm applied to your automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*
* @return The CloudWatch alarm that was invoked by the automation.
*/
public java.util.List getTriggeredAlarms() {
if (triggeredAlarms == null) {
triggeredAlarms = new com.amazonaws.internal.SdkInternalList();
}
return triggeredAlarms;
}
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked by the automation.
*/
public void setTriggeredAlarms(java.util.Collection triggeredAlarms) {
if (triggeredAlarms == null) {
this.triggeredAlarms = null;
return;
}
this.triggeredAlarms = new com.amazonaws.internal.SdkInternalList(triggeredAlarms);
}
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTriggeredAlarms(java.util.Collection)} or {@link #withTriggeredAlarms(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked by the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTriggeredAlarms(AlarmStateInformation... triggeredAlarms) {
if (this.triggeredAlarms == null) {
setTriggeredAlarms(new com.amazonaws.internal.SdkInternalList(triggeredAlarms.length));
}
for (AlarmStateInformation ele : triggeredAlarms) {
this.triggeredAlarms.add(ele);
}
return this;
}
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked by the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withTriggeredAlarms(java.util.Collection triggeredAlarms) {
setTriggeredAlarms(triggeredAlarms);
return this;
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @param automationSubtype
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
* .
* @see AutomationSubtype
*/
public void setAutomationSubtype(String automationSubtype) {
this.automationSubtype = automationSubtype;
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @return The subtype of the Automation operation. Currently, the only supported value is
* ChangeRequest
.
* @see AutomationSubtype
*/
public String getAutomationSubtype() {
return this.automationSubtype;
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @param automationSubtype
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
* .
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationSubtype
*/
public AutomationExecution withAutomationSubtype(String automationSubtype) {
setAutomationSubtype(automationSubtype);
return this;
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @param automationSubtype
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
* .
* @see AutomationSubtype
*/
public void setAutomationSubtype(AutomationSubtype automationSubtype) {
withAutomationSubtype(automationSubtype);
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @param automationSubtype
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
* .
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationSubtype
*/
public AutomationExecution withAutomationSubtype(AutomationSubtype automationSubtype) {
this.automationSubtype = automationSubtype.toString();
return this;
}
/**
*
* The date and time the Automation operation is scheduled to start.
*
*
* @param scheduledTime
* The date and time the Automation operation is scheduled to start.
*/
public void setScheduledTime(java.util.Date scheduledTime) {
this.scheduledTime = scheduledTime;
}
/**
*
* The date and time the Automation operation is scheduled to start.
*
*
* @return The date and time the Automation operation is scheduled to start.
*/
public java.util.Date getScheduledTime() {
return this.scheduledTime;
}
/**
*
* The date and time the Automation operation is scheduled to start.
*
*
* @param scheduledTime
* The date and time the Automation operation is scheduled to start.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withScheduledTime(java.util.Date scheduledTime) {
setScheduledTime(scheduledTime);
return this;
}
/**
*
* Information about the Automation runbooks that are run as part of a runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @return Information about the Automation runbooks that are run as part of a runbook workflow.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
*/
public java.util.List getRunbooks() {
if (runbooks == null) {
runbooks = new com.amazonaws.internal.SdkInternalList();
}
return runbooks;
}
/**
*
* Information about the Automation runbooks that are run as part of a runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @param runbooks
* Information about the Automation runbooks that are run as part of a runbook workflow.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
*/
public void setRunbooks(java.util.Collection runbooks) {
if (runbooks == null) {
this.runbooks = null;
return;
}
this.runbooks = new com.amazonaws.internal.SdkInternalList(runbooks);
}
/**
*
* Information about the Automation runbooks that are run as part of a runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRunbooks(java.util.Collection)} or {@link #withRunbooks(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param runbooks
* Information about the Automation runbooks that are run as part of a runbook workflow.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withRunbooks(Runbook... runbooks) {
if (this.runbooks == null) {
setRunbooks(new com.amazonaws.internal.SdkInternalList(runbooks.length));
}
for (Runbook ele : runbooks) {
this.runbooks.add(ele);
}
return this;
}
/**
*
* Information about the Automation runbooks that are run as part of a runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @param runbooks
* Information about the Automation runbooks that are run as part of a runbook workflow.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withRunbooks(java.util.Collection runbooks) {
setRunbooks(runbooks);
return this;
}
/**
*
* The ID of an OpsItem that is created to represent a Change Manager change request.
*
*
* @param opsItemId
* The ID of an OpsItem that is created to represent a Change Manager change request.
*/
public void setOpsItemId(String opsItemId) {
this.opsItemId = opsItemId;
}
/**
*
* The ID of an OpsItem that is created to represent a Change Manager change request.
*
*
* @return The ID of an OpsItem that is created to represent a Change Manager change request.
*/
public String getOpsItemId() {
return this.opsItemId;
}
/**
*
* The ID of an OpsItem that is created to represent a Change Manager change request.
*
*
* @param opsItemId
* The ID of an OpsItem that is created to represent a Change Manager change request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withOpsItemId(String opsItemId) {
setOpsItemId(opsItemId);
return this;
}
/**
*
* The ID of a State Manager association used in the Automation operation.
*
*
* @param associationId
* The ID of a State Manager association used in the Automation operation.
*/
public void setAssociationId(String associationId) {
this.associationId = associationId;
}
/**
*
* The ID of a State Manager association used in the Automation operation.
*
*
* @return The ID of a State Manager association used in the Automation operation.
*/
public String getAssociationId() {
return this.associationId;
}
/**
*
* The ID of a State Manager association used in the Automation operation.
*
*
* @param associationId
* The ID of a State Manager association used in the Automation operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withAssociationId(String associationId) {
setAssociationId(associationId);
return this;
}
/**
*
* The name of the Change Manager change request.
*
*
* @param changeRequestName
* The name of the Change Manager change request.
*/
public void setChangeRequestName(String changeRequestName) {
this.changeRequestName = changeRequestName;
}
/**
*
* The name of the Change Manager change request.
*
*
* @return The name of the Change Manager change request.
*/
public String getChangeRequestName() {
return this.changeRequestName;
}
/**
*
* The name of the Change Manager change request.
*
*
* @param changeRequestName
* The name of the Change Manager change request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withChangeRequestName(String changeRequestName) {
setChangeRequestName(changeRequestName);
return this;
}
/**
*
* Variables defined for the automation.
*
*
* @return Variables defined for the automation.
*/
public java.util.Map> getVariables() {
return variables;
}
/**
*
* Variables defined for the automation.
*
*
* @param variables
* Variables defined for the automation.
*/
public void setVariables(java.util.Map> variables) {
this.variables = variables;
}
/**
*
* Variables defined for the automation.
*
*
* @param variables
* Variables defined for the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution withVariables(java.util.Map> variables) {
setVariables(variables);
return this;
}
/**
* Add a single Variables entry
*
* @see AutomationExecution#withVariables
* @returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution addVariablesEntry(String key, java.util.List value) {
if (null == this.variables) {
this.variables = new java.util.HashMap>();
}
if (this.variables.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.variables.put(key, value);
return this;
}
/**
* Removes all the entries added into Variables.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecution clearVariablesEntries() {
this.variables = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAutomationExecutionId() != null)
sb.append("AutomationExecutionId: ").append(getAutomationExecutionId()).append(",");
if (getDocumentName() != null)
sb.append("DocumentName: ").append(getDocumentName()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getExecutionStartTime() != null)
sb.append("ExecutionStartTime: ").append(getExecutionStartTime()).append(",");
if (getExecutionEndTime() != null)
sb.append("ExecutionEndTime: ").append(getExecutionEndTime()).append(",");
if (getAutomationExecutionStatus() != null)
sb.append("AutomationExecutionStatus: ").append(getAutomationExecutionStatus()).append(",");
if (getStepExecutions() != null)
sb.append("StepExecutions: ").append(getStepExecutions()).append(",");
if (getStepExecutionsTruncated() != null)
sb.append("StepExecutionsTruncated: ").append(getStepExecutionsTruncated()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters()).append(",");
if (getOutputs() != null)
sb.append("Outputs: ").append(getOutputs()).append(",");
if (getFailureMessage() != null)
sb.append("FailureMessage: ").append(getFailureMessage()).append(",");
if (getMode() != null)
sb.append("Mode: ").append(getMode()).append(",");
if (getParentAutomationExecutionId() != null)
sb.append("ParentAutomationExecutionId: ").append(getParentAutomationExecutionId()).append(",");
if (getExecutedBy() != null)
sb.append("ExecutedBy: ").append(getExecutedBy()).append(",");
if (getCurrentStepName() != null)
sb.append("CurrentStepName: ").append(getCurrentStepName()).append(",");
if (getCurrentAction() != null)
sb.append("CurrentAction: ").append(getCurrentAction()).append(",");
if (getTargetParameterName() != null)
sb.append("TargetParameterName: ").append(getTargetParameterName()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getTargetMaps() != null)
sb.append("TargetMaps: ").append(getTargetMaps()).append(",");
if (getResolvedTargets() != null)
sb.append("ResolvedTargets: ").append(getResolvedTargets()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getTarget() != null)
sb.append("Target: ").append(getTarget()).append(",");
if (getTargetLocations() != null)
sb.append("TargetLocations: ").append(getTargetLocations()).append(",");
if (getProgressCounters() != null)
sb.append("ProgressCounters: ").append(getProgressCounters()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration()).append(",");
if (getTriggeredAlarms() != null)
sb.append("TriggeredAlarms: ").append(getTriggeredAlarms()).append(",");
if (getAutomationSubtype() != null)
sb.append("AutomationSubtype: ").append(getAutomationSubtype()).append(",");
if (getScheduledTime() != null)
sb.append("ScheduledTime: ").append(getScheduledTime()).append(",");
if (getRunbooks() != null)
sb.append("Runbooks: ").append(getRunbooks()).append(",");
if (getOpsItemId() != null)
sb.append("OpsItemId: ").append(getOpsItemId()).append(",");
if (getAssociationId() != null)
sb.append("AssociationId: ").append(getAssociationId()).append(",");
if (getChangeRequestName() != null)
sb.append("ChangeRequestName: ").append(getChangeRequestName()).append(",");
if (getVariables() != null)
sb.append("Variables: ").append(getVariables());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AutomationExecution == false)
return false;
AutomationExecution other = (AutomationExecution) obj;
if (other.getAutomationExecutionId() == null ^ this.getAutomationExecutionId() == null)
return false;
if (other.getAutomationExecutionId() != null && other.getAutomationExecutionId().equals(this.getAutomationExecutionId()) == false)
return false;
if (other.getDocumentName() == null ^ this.getDocumentName() == null)
return false;
if (other.getDocumentName() != null && other.getDocumentName().equals(this.getDocumentName()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getExecutionStartTime() == null ^ this.getExecutionStartTime() == null)
return false;
if (other.getExecutionStartTime() != null && other.getExecutionStartTime().equals(this.getExecutionStartTime()) == false)
return false;
if (other.getExecutionEndTime() == null ^ this.getExecutionEndTime() == null)
return false;
if (other.getExecutionEndTime() != null && other.getExecutionEndTime().equals(this.getExecutionEndTime()) == false)
return false;
if (other.getAutomationExecutionStatus() == null ^ this.getAutomationExecutionStatus() == null)
return false;
if (other.getAutomationExecutionStatus() != null && other.getAutomationExecutionStatus().equals(this.getAutomationExecutionStatus()) == false)
return false;
if (other.getStepExecutions() == null ^ this.getStepExecutions() == null)
return false;
if (other.getStepExecutions() != null && other.getStepExecutions().equals(this.getStepExecutions()) == false)
return false;
if (other.getStepExecutionsTruncated() == null ^ this.getStepExecutionsTruncated() == null)
return false;
if (other.getStepExecutionsTruncated() != null && other.getStepExecutionsTruncated().equals(this.getStepExecutionsTruncated()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getOutputs() == null ^ this.getOutputs() == null)
return false;
if (other.getOutputs() != null && other.getOutputs().equals(this.getOutputs()) == false)
return false;
if (other.getFailureMessage() == null ^ this.getFailureMessage() == null)
return false;
if (other.getFailureMessage() != null && other.getFailureMessage().equals(this.getFailureMessage()) == false)
return false;
if (other.getMode() == null ^ this.getMode() == null)
return false;
if (other.getMode() != null && other.getMode().equals(this.getMode()) == false)
return false;
if (other.getParentAutomationExecutionId() == null ^ this.getParentAutomationExecutionId() == null)
return false;
if (other.getParentAutomationExecutionId() != null && other.getParentAutomationExecutionId().equals(this.getParentAutomationExecutionId()) == false)
return false;
if (other.getExecutedBy() == null ^ this.getExecutedBy() == null)
return false;
if (other.getExecutedBy() != null && other.getExecutedBy().equals(this.getExecutedBy()) == false)
return false;
if (other.getCurrentStepName() == null ^ this.getCurrentStepName() == null)
return false;
if (other.getCurrentStepName() != null && other.getCurrentStepName().equals(this.getCurrentStepName()) == false)
return false;
if (other.getCurrentAction() == null ^ this.getCurrentAction() == null)
return false;
if (other.getCurrentAction() != null && other.getCurrentAction().equals(this.getCurrentAction()) == false)
return false;
if (other.getTargetParameterName() == null ^ this.getTargetParameterName() == null)
return false;
if (other.getTargetParameterName() != null && other.getTargetParameterName().equals(this.getTargetParameterName()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getTargetMaps() == null ^ this.getTargetMaps() == null)
return false;
if (other.getTargetMaps() != null && other.getTargetMaps().equals(this.getTargetMaps()) == false)
return false;
if (other.getResolvedTargets() == null ^ this.getResolvedTargets() == null)
return false;
if (other.getResolvedTargets() != null && other.getResolvedTargets().equals(this.getResolvedTargets()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getTarget() == null ^ this.getTarget() == null)
return false;
if (other.getTarget() != null && other.getTarget().equals(this.getTarget()) == false)
return false;
if (other.getTargetLocations() == null ^ this.getTargetLocations() == null)
return false;
if (other.getTargetLocations() != null && other.getTargetLocations().equals(this.getTargetLocations()) == false)
return false;
if (other.getProgressCounters() == null ^ this.getProgressCounters() == null)
return false;
if (other.getProgressCounters() != null && other.getProgressCounters().equals(this.getProgressCounters()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
if (other.getTriggeredAlarms() == null ^ this.getTriggeredAlarms() == null)
return false;
if (other.getTriggeredAlarms() != null && other.getTriggeredAlarms().equals(this.getTriggeredAlarms()) == false)
return false;
if (other.getAutomationSubtype() == null ^ this.getAutomationSubtype() == null)
return false;
if (other.getAutomationSubtype() != null && other.getAutomationSubtype().equals(this.getAutomationSubtype()) == false)
return false;
if (other.getScheduledTime() == null ^ this.getScheduledTime() == null)
return false;
if (other.getScheduledTime() != null && other.getScheduledTime().equals(this.getScheduledTime()) == false)
return false;
if (other.getRunbooks() == null ^ this.getRunbooks() == null)
return false;
if (other.getRunbooks() != null && other.getRunbooks().equals(this.getRunbooks()) == false)
return false;
if (other.getOpsItemId() == null ^ this.getOpsItemId() == null)
return false;
if (other.getOpsItemId() != null && other.getOpsItemId().equals(this.getOpsItemId()) == false)
return false;
if (other.getAssociationId() == null ^ this.getAssociationId() == null)
return false;
if (other.getAssociationId() != null && other.getAssociationId().equals(this.getAssociationId()) == false)
return false;
if (other.getChangeRequestName() == null ^ this.getChangeRequestName() == null)
return false;
if (other.getChangeRequestName() != null && other.getChangeRequestName().equals(this.getChangeRequestName()) == false)
return false;
if (other.getVariables() == null ^ this.getVariables() == null)
return false;
if (other.getVariables() != null && other.getVariables().equals(this.getVariables()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAutomationExecutionId() == null) ? 0 : getAutomationExecutionId().hashCode());
hashCode = prime * hashCode + ((getDocumentName() == null) ? 0 : getDocumentName().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getExecutionStartTime() == null) ? 0 : getExecutionStartTime().hashCode());
hashCode = prime * hashCode + ((getExecutionEndTime() == null) ? 0 : getExecutionEndTime().hashCode());
hashCode = prime * hashCode + ((getAutomationExecutionStatus() == null) ? 0 : getAutomationExecutionStatus().hashCode());
hashCode = prime * hashCode + ((getStepExecutions() == null) ? 0 : getStepExecutions().hashCode());
hashCode = prime * hashCode + ((getStepExecutionsTruncated() == null) ? 0 : getStepExecutionsTruncated().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getOutputs() == null) ? 0 : getOutputs().hashCode());
hashCode = prime * hashCode + ((getFailureMessage() == null) ? 0 : getFailureMessage().hashCode());
hashCode = prime * hashCode + ((getMode() == null) ? 0 : getMode().hashCode());
hashCode = prime * hashCode + ((getParentAutomationExecutionId() == null) ? 0 : getParentAutomationExecutionId().hashCode());
hashCode = prime * hashCode + ((getExecutedBy() == null) ? 0 : getExecutedBy().hashCode());
hashCode = prime * hashCode + ((getCurrentStepName() == null) ? 0 : getCurrentStepName().hashCode());
hashCode = prime * hashCode + ((getCurrentAction() == null) ? 0 : getCurrentAction().hashCode());
hashCode = prime * hashCode + ((getTargetParameterName() == null) ? 0 : getTargetParameterName().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getTargetMaps() == null) ? 0 : getTargetMaps().hashCode());
hashCode = prime * hashCode + ((getResolvedTargets() == null) ? 0 : getResolvedTargets().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getTarget() == null) ? 0 : getTarget().hashCode());
hashCode = prime * hashCode + ((getTargetLocations() == null) ? 0 : getTargetLocations().hashCode());
hashCode = prime * hashCode + ((getProgressCounters() == null) ? 0 : getProgressCounters().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
hashCode = prime * hashCode + ((getTriggeredAlarms() == null) ? 0 : getTriggeredAlarms().hashCode());
hashCode = prime * hashCode + ((getAutomationSubtype() == null) ? 0 : getAutomationSubtype().hashCode());
hashCode = prime * hashCode + ((getScheduledTime() == null) ? 0 : getScheduledTime().hashCode());
hashCode = prime * hashCode + ((getRunbooks() == null) ? 0 : getRunbooks().hashCode());
hashCode = prime * hashCode + ((getOpsItemId() == null) ? 0 : getOpsItemId().hashCode());
hashCode = prime * hashCode + ((getAssociationId() == null) ? 0 : getAssociationId().hashCode());
hashCode = prime * hashCode + ((getChangeRequestName() == null) ? 0 : getChangeRequestName().hashCode());
hashCode = prime * hashCode + ((getVariables() == null) ? 0 : getVariables().hashCode());
return hashCode;
}
@Override
public AutomationExecution clone() {
try {
return (AutomationExecution) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.AutomationExecutionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}