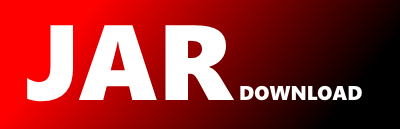
com.amazonaws.services.simplesystemsmanagement.model.AutomationExecutionMetadata Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Details about a specific Automation execution.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AutomationExecutionMetadata implements Serializable, Cloneable, StructuredPojo {
/**
*
* The execution ID.
*
*/
private String automationExecutionId;
/**
*
* The name of the Automation runbook used during execution.
*
*/
private String documentName;
/**
*
* The document version used during the execution.
*
*/
private String documentVersion;
/**
*
* The status of the execution.
*
*/
private String automationExecutionStatus;
/**
*
* The time the execution started.
*
*/
private java.util.Date executionStartTime;
/**
*
* The time the execution finished. This isn't populated if the execution is still in progress.
*
*/
private java.util.Date executionEndTime;
/**
*
* The IAM role ARN of the user who ran the automation.
*
*/
private String executedBy;
/**
*
* An S3 bucket where execution information is stored.
*
*/
private String logFile;
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*/
private java.util.Map> outputs;
/**
*
* The Automation execution mode.
*
*/
private String mode;
/**
*
* The execution ID of the parent automation.
*
*/
private String parentAutomationExecutionId;
/**
*
* The name of the step that is currently running.
*
*/
private String currentStepName;
/**
*
* The action of the step that is currently running.
*
*/
private String currentAction;
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*/
private String failureMessage;
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*/
private String targetParameterName;
/**
*
* The targets defined by the user when starting the automation.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*/
private com.amazonaws.internal.SdkInternalList>> targetMaps;
/**
*
* A list of targets that resolved during the execution.
*
*/
private ResolvedTargets resolvedTargets;
/**
*
* The MaxConcurrency
value specified by the user when starting the automation.
*
*/
private String maxConcurrency;
/**
*
* The MaxErrors
value specified by the user when starting the automation.
*
*/
private String maxErrors;
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*/
private String target;
/**
*
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount. CrossAccount is
* an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services accounts. For more
* information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon Web
* Services Systems Manager User Guide.
*
*/
private String automationType;
/**
*
* The details for the CloudWatch alarm applied to your automation.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*/
private com.amazonaws.internal.SdkInternalList triggeredAlarms;
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*/
private String automationSubtype;
/**
*
* The date and time the Automation operation is scheduled to start.
*
*/
private java.util.Date scheduledTime;
/**
*
* Information about the Automation runbooks that are run during a runbook workflow in Change Manager.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*/
private com.amazonaws.internal.SdkInternalList runbooks;
/**
*
* The ID of an OpsItem that is created to represent a Change Manager change request.
*
*/
private String opsItemId;
/**
*
* The ID of a State Manager association used in the Automation operation.
*
*/
private String associationId;
/**
*
* The name of the Change Manager change request.
*
*/
private String changeRequestName;
/**
*
* The execution ID.
*
*
* @param automationExecutionId
* The execution ID.
*/
public void setAutomationExecutionId(String automationExecutionId) {
this.automationExecutionId = automationExecutionId;
}
/**
*
* The execution ID.
*
*
* @return The execution ID.
*/
public String getAutomationExecutionId() {
return this.automationExecutionId;
}
/**
*
* The execution ID.
*
*
* @param automationExecutionId
* The execution ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withAutomationExecutionId(String automationExecutionId) {
setAutomationExecutionId(automationExecutionId);
return this;
}
/**
*
* The name of the Automation runbook used during execution.
*
*
* @param documentName
* The name of the Automation runbook used during execution.
*/
public void setDocumentName(String documentName) {
this.documentName = documentName;
}
/**
*
* The name of the Automation runbook used during execution.
*
*
* @return The name of the Automation runbook used during execution.
*/
public String getDocumentName() {
return this.documentName;
}
/**
*
* The name of the Automation runbook used during execution.
*
*
* @param documentName
* The name of the Automation runbook used during execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withDocumentName(String documentName) {
setDocumentName(documentName);
return this;
}
/**
*
* The document version used during the execution.
*
*
* @param documentVersion
* The document version used during the execution.
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The document version used during the execution.
*
*
* @return The document version used during the execution.
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The document version used during the execution.
*
*
* @param documentVersion
* The document version used during the execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* The status of the execution.
*
*
* @param automationExecutionStatus
* The status of the execution.
* @see AutomationExecutionStatus
*/
public void setAutomationExecutionStatus(String automationExecutionStatus) {
this.automationExecutionStatus = automationExecutionStatus;
}
/**
*
* The status of the execution.
*
*
* @return The status of the execution.
* @see AutomationExecutionStatus
*/
public String getAutomationExecutionStatus() {
return this.automationExecutionStatus;
}
/**
*
* The status of the execution.
*
*
* @param automationExecutionStatus
* The status of the execution.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationExecutionStatus
*/
public AutomationExecutionMetadata withAutomationExecutionStatus(String automationExecutionStatus) {
setAutomationExecutionStatus(automationExecutionStatus);
return this;
}
/**
*
* The status of the execution.
*
*
* @param automationExecutionStatus
* The status of the execution.
* @see AutomationExecutionStatus
*/
public void setAutomationExecutionStatus(AutomationExecutionStatus automationExecutionStatus) {
withAutomationExecutionStatus(automationExecutionStatus);
}
/**
*
* The status of the execution.
*
*
* @param automationExecutionStatus
* The status of the execution.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationExecutionStatus
*/
public AutomationExecutionMetadata withAutomationExecutionStatus(AutomationExecutionStatus automationExecutionStatus) {
this.automationExecutionStatus = automationExecutionStatus.toString();
return this;
}
/**
*
* The time the execution started.
*
*
* @param executionStartTime
* The time the execution started.
*/
public void setExecutionStartTime(java.util.Date executionStartTime) {
this.executionStartTime = executionStartTime;
}
/**
*
* The time the execution started.
*
*
* @return The time the execution started.
*/
public java.util.Date getExecutionStartTime() {
return this.executionStartTime;
}
/**
*
* The time the execution started.
*
*
* @param executionStartTime
* The time the execution started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withExecutionStartTime(java.util.Date executionStartTime) {
setExecutionStartTime(executionStartTime);
return this;
}
/**
*
* The time the execution finished. This isn't populated if the execution is still in progress.
*
*
* @param executionEndTime
* The time the execution finished. This isn't populated if the execution is still in progress.
*/
public void setExecutionEndTime(java.util.Date executionEndTime) {
this.executionEndTime = executionEndTime;
}
/**
*
* The time the execution finished. This isn't populated if the execution is still in progress.
*
*
* @return The time the execution finished. This isn't populated if the execution is still in progress.
*/
public java.util.Date getExecutionEndTime() {
return this.executionEndTime;
}
/**
*
* The time the execution finished. This isn't populated if the execution is still in progress.
*
*
* @param executionEndTime
* The time the execution finished. This isn't populated if the execution is still in progress.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withExecutionEndTime(java.util.Date executionEndTime) {
setExecutionEndTime(executionEndTime);
return this;
}
/**
*
* The IAM role ARN of the user who ran the automation.
*
*
* @param executedBy
* The IAM role ARN of the user who ran the automation.
*/
public void setExecutedBy(String executedBy) {
this.executedBy = executedBy;
}
/**
*
* The IAM role ARN of the user who ran the automation.
*
*
* @return The IAM role ARN of the user who ran the automation.
*/
public String getExecutedBy() {
return this.executedBy;
}
/**
*
* The IAM role ARN of the user who ran the automation.
*
*
* @param executedBy
* The IAM role ARN of the user who ran the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withExecutedBy(String executedBy) {
setExecutedBy(executedBy);
return this;
}
/**
*
* An S3 bucket where execution information is stored.
*
*
* @param logFile
* An S3 bucket where execution information is stored.
*/
public void setLogFile(String logFile) {
this.logFile = logFile;
}
/**
*
* An S3 bucket where execution information is stored.
*
*
* @return An S3 bucket where execution information is stored.
*/
public String getLogFile() {
return this.logFile;
}
/**
*
* An S3 bucket where execution information is stored.
*
*
* @param logFile
* An S3 bucket where execution information is stored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withLogFile(String logFile) {
setLogFile(logFile);
return this;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @return The list of execution outputs as defined in the Automation runbook.
*/
public java.util.Map> getOutputs() {
return outputs;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param outputs
* The list of execution outputs as defined in the Automation runbook.
*/
public void setOutputs(java.util.Map> outputs) {
this.outputs = outputs;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param outputs
* The list of execution outputs as defined in the Automation runbook.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withOutputs(java.util.Map> outputs) {
setOutputs(outputs);
return this;
}
/**
* Add a single Outputs entry
*
* @see AutomationExecutionMetadata#withOutputs
* @returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata addOutputsEntry(String key, java.util.List value) {
if (null == this.outputs) {
this.outputs = new java.util.HashMap>();
}
if (this.outputs.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.outputs.put(key, value);
return this;
}
/**
* Removes all the entries added into Outputs.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata clearOutputsEntries() {
this.outputs = null;
return this;
}
/**
*
* The Automation execution mode.
*
*
* @param mode
* The Automation execution mode.
* @see ExecutionMode
*/
public void setMode(String mode) {
this.mode = mode;
}
/**
*
* The Automation execution mode.
*
*
* @return The Automation execution mode.
* @see ExecutionMode
*/
public String getMode() {
return this.mode;
}
/**
*
* The Automation execution mode.
*
*
* @param mode
* The Automation execution mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionMode
*/
public AutomationExecutionMetadata withMode(String mode) {
setMode(mode);
return this;
}
/**
*
* The Automation execution mode.
*
*
* @param mode
* The Automation execution mode.
* @see ExecutionMode
*/
public void setMode(ExecutionMode mode) {
withMode(mode);
}
/**
*
* The Automation execution mode.
*
*
* @param mode
* The Automation execution mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionMode
*/
public AutomationExecutionMetadata withMode(ExecutionMode mode) {
this.mode = mode.toString();
return this;
}
/**
*
* The execution ID of the parent automation.
*
*
* @param parentAutomationExecutionId
* The execution ID of the parent automation.
*/
public void setParentAutomationExecutionId(String parentAutomationExecutionId) {
this.parentAutomationExecutionId = parentAutomationExecutionId;
}
/**
*
* The execution ID of the parent automation.
*
*
* @return The execution ID of the parent automation.
*/
public String getParentAutomationExecutionId() {
return this.parentAutomationExecutionId;
}
/**
*
* The execution ID of the parent automation.
*
*
* @param parentAutomationExecutionId
* The execution ID of the parent automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withParentAutomationExecutionId(String parentAutomationExecutionId) {
setParentAutomationExecutionId(parentAutomationExecutionId);
return this;
}
/**
*
* The name of the step that is currently running.
*
*
* @param currentStepName
* The name of the step that is currently running.
*/
public void setCurrentStepName(String currentStepName) {
this.currentStepName = currentStepName;
}
/**
*
* The name of the step that is currently running.
*
*
* @return The name of the step that is currently running.
*/
public String getCurrentStepName() {
return this.currentStepName;
}
/**
*
* The name of the step that is currently running.
*
*
* @param currentStepName
* The name of the step that is currently running.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withCurrentStepName(String currentStepName) {
setCurrentStepName(currentStepName);
return this;
}
/**
*
* The action of the step that is currently running.
*
*
* @param currentAction
* The action of the step that is currently running.
*/
public void setCurrentAction(String currentAction) {
this.currentAction = currentAction;
}
/**
*
* The action of the step that is currently running.
*
*
* @return The action of the step that is currently running.
*/
public String getCurrentAction() {
return this.currentAction;
}
/**
*
* The action of the step that is currently running.
*
*
* @param currentAction
* The action of the step that is currently running.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withCurrentAction(String currentAction) {
setCurrentAction(currentAction);
return this;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param failureMessage
* The list of execution outputs as defined in the Automation runbook.
*/
public void setFailureMessage(String failureMessage) {
this.failureMessage = failureMessage;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @return The list of execution outputs as defined in the Automation runbook.
*/
public String getFailureMessage() {
return this.failureMessage;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param failureMessage
* The list of execution outputs as defined in the Automation runbook.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withFailureMessage(String failureMessage) {
setFailureMessage(failureMessage);
return this;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param targetParameterName
* The list of execution outputs as defined in the Automation runbook.
*/
public void setTargetParameterName(String targetParameterName) {
this.targetParameterName = targetParameterName;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @return The list of execution outputs as defined in the Automation runbook.
*/
public String getTargetParameterName() {
return this.targetParameterName;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param targetParameterName
* The list of execution outputs as defined in the Automation runbook.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withTargetParameterName(String targetParameterName) {
setTargetParameterName(targetParameterName);
return this;
}
/**
*
* The targets defined by the user when starting the automation.
*
*
* @return The targets defined by the user when starting the automation.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The targets defined by the user when starting the automation.
*
*
* @param targets
* The targets defined by the user when starting the automation.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The targets defined by the user when starting the automation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The targets defined by the user when starting the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The targets defined by the user when starting the automation.
*
*
* @param targets
* The targets defined by the user when starting the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*
* @return The specified key-value mapping of document parameters to target resources.
*/
public java.util.List>> getTargetMaps() {
if (targetMaps == null) {
targetMaps = new com.amazonaws.internal.SdkInternalList>>();
}
return targetMaps;
}
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*
* @param targetMaps
* The specified key-value mapping of document parameters to target resources.
*/
public void setTargetMaps(java.util.Collection>> targetMaps) {
if (targetMaps == null) {
this.targetMaps = null;
return;
}
this.targetMaps = new com.amazonaws.internal.SdkInternalList>>(targetMaps);
}
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetMaps(java.util.Collection)} or {@link #withTargetMaps(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param targetMaps
* The specified key-value mapping of document parameters to target resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withTargetMaps(java.util.Map>... targetMaps) {
if (this.targetMaps == null) {
setTargetMaps(new com.amazonaws.internal.SdkInternalList>>(targetMaps.length));
}
for (java.util.Map> ele : targetMaps) {
this.targetMaps.add(ele);
}
return this;
}
/**
*
* The specified key-value mapping of document parameters to target resources.
*
*
* @param targetMaps
* The specified key-value mapping of document parameters to target resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withTargetMaps(java.util.Collection>> targetMaps) {
setTargetMaps(targetMaps);
return this;
}
/**
*
* A list of targets that resolved during the execution.
*
*
* @param resolvedTargets
* A list of targets that resolved during the execution.
*/
public void setResolvedTargets(ResolvedTargets resolvedTargets) {
this.resolvedTargets = resolvedTargets;
}
/**
*
* A list of targets that resolved during the execution.
*
*
* @return A list of targets that resolved during the execution.
*/
public ResolvedTargets getResolvedTargets() {
return this.resolvedTargets;
}
/**
*
* A list of targets that resolved during the execution.
*
*
* @param resolvedTargets
* A list of targets that resolved during the execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withResolvedTargets(ResolvedTargets resolvedTargets) {
setResolvedTargets(resolvedTargets);
return this;
}
/**
*
* The MaxConcurrency
value specified by the user when starting the automation.
*
*
* @param maxConcurrency
* The MaxConcurrency
value specified by the user when starting the automation.
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The MaxConcurrency
value specified by the user when starting the automation.
*
*
* @return The MaxConcurrency
value specified by the user when starting the automation.
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The MaxConcurrency
value specified by the user when starting the automation.
*
*
* @param maxConcurrency
* The MaxConcurrency
value specified by the user when starting the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The MaxErrors
value specified by the user when starting the automation.
*
*
* @param maxErrors
* The MaxErrors
value specified by the user when starting the automation.
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The MaxErrors
value specified by the user when starting the automation.
*
*
* @return The MaxErrors
value specified by the user when starting the automation.
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The MaxErrors
value specified by the user when starting the automation.
*
*
* @param maxErrors
* The MaxErrors
value specified by the user when starting the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param target
* The list of execution outputs as defined in the Automation runbook.
*/
public void setTarget(String target) {
this.target = target;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @return The list of execution outputs as defined in the Automation runbook.
*/
public String getTarget() {
return this.target;
}
/**
*
* The list of execution outputs as defined in the Automation runbook.
*
*
* @param target
* The list of execution outputs as defined in the Automation runbook.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withTarget(String target) {
setTarget(target);
return this;
}
/**
*
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount. CrossAccount is
* an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services accounts. For more
* information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon Web
* Services Systems Manager User Guide.
*
*
* @param automationType
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount.
* CrossAccount is an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services
* accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon
* Web Services Systems Manager User Guide.
* @see AutomationType
*/
public void setAutomationType(String automationType) {
this.automationType = automationType;
}
/**
*
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount. CrossAccount is
* an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services accounts. For more
* information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon Web
* Services Systems Manager User Guide.
*
*
* @return Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount.
* CrossAccount is an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services
* accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon
* Web Services Systems Manager User Guide.
* @see AutomationType
*/
public String getAutomationType() {
return this.automationType;
}
/**
*
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount. CrossAccount is
* an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services accounts. For more
* information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon Web
* Services Systems Manager User Guide.
*
*
* @param automationType
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount.
* CrossAccount is an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services
* accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon
* Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationType
*/
public AutomationExecutionMetadata withAutomationType(String automationType) {
setAutomationType(automationType);
return this;
}
/**
*
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount. CrossAccount is
* an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services accounts. For more
* information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon Web
* Services Systems Manager User Guide.
*
*
* @param automationType
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount.
* CrossAccount is an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services
* accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon
* Web Services Systems Manager User Guide.
* @see AutomationType
*/
public void setAutomationType(AutomationType automationType) {
withAutomationType(automationType);
}
/**
*
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount. CrossAccount is
* an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services accounts. For more
* information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon Web
* Services Systems Manager User Guide.
*
*
* @param automationType
* Use this filter with DescribeAutomationExecutions. Specify either Local or CrossAccount.
* CrossAccount is an Automation that runs in multiple Amazon Web Services Regions and Amazon Web Services
* accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and accounts in the Amazon
* Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationType
*/
public AutomationExecutionMetadata withAutomationType(AutomationType automationType) {
this.automationType = automationType.toString();
return this;
}
/**
*
* The details for the CloudWatch alarm applied to your automation.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm applied to your automation.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm applied to your automation.
*
*
* @return The details for the CloudWatch alarm applied to your automation.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm applied to your automation.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm applied to your automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*
* @return The CloudWatch alarm that was invoked by the automation.
*/
public java.util.List getTriggeredAlarms() {
if (triggeredAlarms == null) {
triggeredAlarms = new com.amazonaws.internal.SdkInternalList();
}
return triggeredAlarms;
}
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked by the automation.
*/
public void setTriggeredAlarms(java.util.Collection triggeredAlarms) {
if (triggeredAlarms == null) {
this.triggeredAlarms = null;
return;
}
this.triggeredAlarms = new com.amazonaws.internal.SdkInternalList(triggeredAlarms);
}
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTriggeredAlarms(java.util.Collection)} or {@link #withTriggeredAlarms(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked by the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withTriggeredAlarms(AlarmStateInformation... triggeredAlarms) {
if (this.triggeredAlarms == null) {
setTriggeredAlarms(new com.amazonaws.internal.SdkInternalList(triggeredAlarms.length));
}
for (AlarmStateInformation ele : triggeredAlarms) {
this.triggeredAlarms.add(ele);
}
return this;
}
/**
*
* The CloudWatch alarm that was invoked by the automation.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked by the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withTriggeredAlarms(java.util.Collection triggeredAlarms) {
setTriggeredAlarms(triggeredAlarms);
return this;
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @param automationSubtype
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
* .
* @see AutomationSubtype
*/
public void setAutomationSubtype(String automationSubtype) {
this.automationSubtype = automationSubtype;
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @return The subtype of the Automation operation. Currently, the only supported value is
* ChangeRequest
.
* @see AutomationSubtype
*/
public String getAutomationSubtype() {
return this.automationSubtype;
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @param automationSubtype
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
* .
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationSubtype
*/
public AutomationExecutionMetadata withAutomationSubtype(String automationSubtype) {
setAutomationSubtype(automationSubtype);
return this;
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @param automationSubtype
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
* .
* @see AutomationSubtype
*/
public void setAutomationSubtype(AutomationSubtype automationSubtype) {
withAutomationSubtype(automationSubtype);
}
/**
*
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
.
*
*
* @param automationSubtype
* The subtype of the Automation operation. Currently, the only supported value is ChangeRequest
* .
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationSubtype
*/
public AutomationExecutionMetadata withAutomationSubtype(AutomationSubtype automationSubtype) {
this.automationSubtype = automationSubtype.toString();
return this;
}
/**
*
* The date and time the Automation operation is scheduled to start.
*
*
* @param scheduledTime
* The date and time the Automation operation is scheduled to start.
*/
public void setScheduledTime(java.util.Date scheduledTime) {
this.scheduledTime = scheduledTime;
}
/**
*
* The date and time the Automation operation is scheduled to start.
*
*
* @return The date and time the Automation operation is scheduled to start.
*/
public java.util.Date getScheduledTime() {
return this.scheduledTime;
}
/**
*
* The date and time the Automation operation is scheduled to start.
*
*
* @param scheduledTime
* The date and time the Automation operation is scheduled to start.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withScheduledTime(java.util.Date scheduledTime) {
setScheduledTime(scheduledTime);
return this;
}
/**
*
* Information about the Automation runbooks that are run during a runbook workflow in Change Manager.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @return Information about the Automation runbooks that are run during a runbook workflow in Change Manager.
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
*/
public java.util.List getRunbooks() {
if (runbooks == null) {
runbooks = new com.amazonaws.internal.SdkInternalList();
}
return runbooks;
}
/**
*
* Information about the Automation runbooks that are run during a runbook workflow in Change Manager.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @param runbooks
* Information about the Automation runbooks that are run during a runbook workflow in Change Manager.
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
*/
public void setRunbooks(java.util.Collection runbooks) {
if (runbooks == null) {
this.runbooks = null;
return;
}
this.runbooks = new com.amazonaws.internal.SdkInternalList(runbooks);
}
/**
*
* Information about the Automation runbooks that are run during a runbook workflow in Change Manager.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRunbooks(java.util.Collection)} or {@link #withRunbooks(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param runbooks
* Information about the Automation runbooks that are run during a runbook workflow in Change Manager.
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withRunbooks(Runbook... runbooks) {
if (this.runbooks == null) {
setRunbooks(new com.amazonaws.internal.SdkInternalList(runbooks.length));
}
for (Runbook ele : runbooks) {
this.runbooks.add(ele);
}
return this;
}
/**
*
* Information about the Automation runbooks that are run during a runbook workflow in Change Manager.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @param runbooks
* Information about the Automation runbooks that are run during a runbook workflow in Change Manager.
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withRunbooks(java.util.Collection runbooks) {
setRunbooks(runbooks);
return this;
}
/**
*
* The ID of an OpsItem that is created to represent a Change Manager change request.
*
*
* @param opsItemId
* The ID of an OpsItem that is created to represent a Change Manager change request.
*/
public void setOpsItemId(String opsItemId) {
this.opsItemId = opsItemId;
}
/**
*
* The ID of an OpsItem that is created to represent a Change Manager change request.
*
*
* @return The ID of an OpsItem that is created to represent a Change Manager change request.
*/
public String getOpsItemId() {
return this.opsItemId;
}
/**
*
* The ID of an OpsItem that is created to represent a Change Manager change request.
*
*
* @param opsItemId
* The ID of an OpsItem that is created to represent a Change Manager change request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withOpsItemId(String opsItemId) {
setOpsItemId(opsItemId);
return this;
}
/**
*
* The ID of a State Manager association used in the Automation operation.
*
*
* @param associationId
* The ID of a State Manager association used in the Automation operation.
*/
public void setAssociationId(String associationId) {
this.associationId = associationId;
}
/**
*
* The ID of a State Manager association used in the Automation operation.
*
*
* @return The ID of a State Manager association used in the Automation operation.
*/
public String getAssociationId() {
return this.associationId;
}
/**
*
* The ID of a State Manager association used in the Automation operation.
*
*
* @param associationId
* The ID of a State Manager association used in the Automation operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withAssociationId(String associationId) {
setAssociationId(associationId);
return this;
}
/**
*
* The name of the Change Manager change request.
*
*
* @param changeRequestName
* The name of the Change Manager change request.
*/
public void setChangeRequestName(String changeRequestName) {
this.changeRequestName = changeRequestName;
}
/**
*
* The name of the Change Manager change request.
*
*
* @return The name of the Change Manager change request.
*/
public String getChangeRequestName() {
return this.changeRequestName;
}
/**
*
* The name of the Change Manager change request.
*
*
* @param changeRequestName
* The name of the Change Manager change request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutomationExecutionMetadata withChangeRequestName(String changeRequestName) {
setChangeRequestName(changeRequestName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAutomationExecutionId() != null)
sb.append("AutomationExecutionId: ").append(getAutomationExecutionId()).append(",");
if (getDocumentName() != null)
sb.append("DocumentName: ").append(getDocumentName()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getAutomationExecutionStatus() != null)
sb.append("AutomationExecutionStatus: ").append(getAutomationExecutionStatus()).append(",");
if (getExecutionStartTime() != null)
sb.append("ExecutionStartTime: ").append(getExecutionStartTime()).append(",");
if (getExecutionEndTime() != null)
sb.append("ExecutionEndTime: ").append(getExecutionEndTime()).append(",");
if (getExecutedBy() != null)
sb.append("ExecutedBy: ").append(getExecutedBy()).append(",");
if (getLogFile() != null)
sb.append("LogFile: ").append(getLogFile()).append(",");
if (getOutputs() != null)
sb.append("Outputs: ").append(getOutputs()).append(",");
if (getMode() != null)
sb.append("Mode: ").append(getMode()).append(",");
if (getParentAutomationExecutionId() != null)
sb.append("ParentAutomationExecutionId: ").append(getParentAutomationExecutionId()).append(",");
if (getCurrentStepName() != null)
sb.append("CurrentStepName: ").append(getCurrentStepName()).append(",");
if (getCurrentAction() != null)
sb.append("CurrentAction: ").append(getCurrentAction()).append(",");
if (getFailureMessage() != null)
sb.append("FailureMessage: ").append(getFailureMessage()).append(",");
if (getTargetParameterName() != null)
sb.append("TargetParameterName: ").append(getTargetParameterName()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getTargetMaps() != null)
sb.append("TargetMaps: ").append(getTargetMaps()).append(",");
if (getResolvedTargets() != null)
sb.append("ResolvedTargets: ").append(getResolvedTargets()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getTarget() != null)
sb.append("Target: ").append(getTarget()).append(",");
if (getAutomationType() != null)
sb.append("AutomationType: ").append(getAutomationType()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration()).append(",");
if (getTriggeredAlarms() != null)
sb.append("TriggeredAlarms: ").append(getTriggeredAlarms()).append(",");
if (getAutomationSubtype() != null)
sb.append("AutomationSubtype: ").append(getAutomationSubtype()).append(",");
if (getScheduledTime() != null)
sb.append("ScheduledTime: ").append(getScheduledTime()).append(",");
if (getRunbooks() != null)
sb.append("Runbooks: ").append(getRunbooks()).append(",");
if (getOpsItemId() != null)
sb.append("OpsItemId: ").append(getOpsItemId()).append(",");
if (getAssociationId() != null)
sb.append("AssociationId: ").append(getAssociationId()).append(",");
if (getChangeRequestName() != null)
sb.append("ChangeRequestName: ").append(getChangeRequestName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AutomationExecutionMetadata == false)
return false;
AutomationExecutionMetadata other = (AutomationExecutionMetadata) obj;
if (other.getAutomationExecutionId() == null ^ this.getAutomationExecutionId() == null)
return false;
if (other.getAutomationExecutionId() != null && other.getAutomationExecutionId().equals(this.getAutomationExecutionId()) == false)
return false;
if (other.getDocumentName() == null ^ this.getDocumentName() == null)
return false;
if (other.getDocumentName() != null && other.getDocumentName().equals(this.getDocumentName()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getAutomationExecutionStatus() == null ^ this.getAutomationExecutionStatus() == null)
return false;
if (other.getAutomationExecutionStatus() != null && other.getAutomationExecutionStatus().equals(this.getAutomationExecutionStatus()) == false)
return false;
if (other.getExecutionStartTime() == null ^ this.getExecutionStartTime() == null)
return false;
if (other.getExecutionStartTime() != null && other.getExecutionStartTime().equals(this.getExecutionStartTime()) == false)
return false;
if (other.getExecutionEndTime() == null ^ this.getExecutionEndTime() == null)
return false;
if (other.getExecutionEndTime() != null && other.getExecutionEndTime().equals(this.getExecutionEndTime()) == false)
return false;
if (other.getExecutedBy() == null ^ this.getExecutedBy() == null)
return false;
if (other.getExecutedBy() != null && other.getExecutedBy().equals(this.getExecutedBy()) == false)
return false;
if (other.getLogFile() == null ^ this.getLogFile() == null)
return false;
if (other.getLogFile() != null && other.getLogFile().equals(this.getLogFile()) == false)
return false;
if (other.getOutputs() == null ^ this.getOutputs() == null)
return false;
if (other.getOutputs() != null && other.getOutputs().equals(this.getOutputs()) == false)
return false;
if (other.getMode() == null ^ this.getMode() == null)
return false;
if (other.getMode() != null && other.getMode().equals(this.getMode()) == false)
return false;
if (other.getParentAutomationExecutionId() == null ^ this.getParentAutomationExecutionId() == null)
return false;
if (other.getParentAutomationExecutionId() != null && other.getParentAutomationExecutionId().equals(this.getParentAutomationExecutionId()) == false)
return false;
if (other.getCurrentStepName() == null ^ this.getCurrentStepName() == null)
return false;
if (other.getCurrentStepName() != null && other.getCurrentStepName().equals(this.getCurrentStepName()) == false)
return false;
if (other.getCurrentAction() == null ^ this.getCurrentAction() == null)
return false;
if (other.getCurrentAction() != null && other.getCurrentAction().equals(this.getCurrentAction()) == false)
return false;
if (other.getFailureMessage() == null ^ this.getFailureMessage() == null)
return false;
if (other.getFailureMessage() != null && other.getFailureMessage().equals(this.getFailureMessage()) == false)
return false;
if (other.getTargetParameterName() == null ^ this.getTargetParameterName() == null)
return false;
if (other.getTargetParameterName() != null && other.getTargetParameterName().equals(this.getTargetParameterName()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getTargetMaps() == null ^ this.getTargetMaps() == null)
return false;
if (other.getTargetMaps() != null && other.getTargetMaps().equals(this.getTargetMaps()) == false)
return false;
if (other.getResolvedTargets() == null ^ this.getResolvedTargets() == null)
return false;
if (other.getResolvedTargets() != null && other.getResolvedTargets().equals(this.getResolvedTargets()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getTarget() == null ^ this.getTarget() == null)
return false;
if (other.getTarget() != null && other.getTarget().equals(this.getTarget()) == false)
return false;
if (other.getAutomationType() == null ^ this.getAutomationType() == null)
return false;
if (other.getAutomationType() != null && other.getAutomationType().equals(this.getAutomationType()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
if (other.getTriggeredAlarms() == null ^ this.getTriggeredAlarms() == null)
return false;
if (other.getTriggeredAlarms() != null && other.getTriggeredAlarms().equals(this.getTriggeredAlarms()) == false)
return false;
if (other.getAutomationSubtype() == null ^ this.getAutomationSubtype() == null)
return false;
if (other.getAutomationSubtype() != null && other.getAutomationSubtype().equals(this.getAutomationSubtype()) == false)
return false;
if (other.getScheduledTime() == null ^ this.getScheduledTime() == null)
return false;
if (other.getScheduledTime() != null && other.getScheduledTime().equals(this.getScheduledTime()) == false)
return false;
if (other.getRunbooks() == null ^ this.getRunbooks() == null)
return false;
if (other.getRunbooks() != null && other.getRunbooks().equals(this.getRunbooks()) == false)
return false;
if (other.getOpsItemId() == null ^ this.getOpsItemId() == null)
return false;
if (other.getOpsItemId() != null && other.getOpsItemId().equals(this.getOpsItemId()) == false)
return false;
if (other.getAssociationId() == null ^ this.getAssociationId() == null)
return false;
if (other.getAssociationId() != null && other.getAssociationId().equals(this.getAssociationId()) == false)
return false;
if (other.getChangeRequestName() == null ^ this.getChangeRequestName() == null)
return false;
if (other.getChangeRequestName() != null && other.getChangeRequestName().equals(this.getChangeRequestName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAutomationExecutionId() == null) ? 0 : getAutomationExecutionId().hashCode());
hashCode = prime * hashCode + ((getDocumentName() == null) ? 0 : getDocumentName().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getAutomationExecutionStatus() == null) ? 0 : getAutomationExecutionStatus().hashCode());
hashCode = prime * hashCode + ((getExecutionStartTime() == null) ? 0 : getExecutionStartTime().hashCode());
hashCode = prime * hashCode + ((getExecutionEndTime() == null) ? 0 : getExecutionEndTime().hashCode());
hashCode = prime * hashCode + ((getExecutedBy() == null) ? 0 : getExecutedBy().hashCode());
hashCode = prime * hashCode + ((getLogFile() == null) ? 0 : getLogFile().hashCode());
hashCode = prime * hashCode + ((getOutputs() == null) ? 0 : getOutputs().hashCode());
hashCode = prime * hashCode + ((getMode() == null) ? 0 : getMode().hashCode());
hashCode = prime * hashCode + ((getParentAutomationExecutionId() == null) ? 0 : getParentAutomationExecutionId().hashCode());
hashCode = prime * hashCode + ((getCurrentStepName() == null) ? 0 : getCurrentStepName().hashCode());
hashCode = prime * hashCode + ((getCurrentAction() == null) ? 0 : getCurrentAction().hashCode());
hashCode = prime * hashCode + ((getFailureMessage() == null) ? 0 : getFailureMessage().hashCode());
hashCode = prime * hashCode + ((getTargetParameterName() == null) ? 0 : getTargetParameterName().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getTargetMaps() == null) ? 0 : getTargetMaps().hashCode());
hashCode = prime * hashCode + ((getResolvedTargets() == null) ? 0 : getResolvedTargets().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getTarget() == null) ? 0 : getTarget().hashCode());
hashCode = prime * hashCode + ((getAutomationType() == null) ? 0 : getAutomationType().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
hashCode = prime * hashCode + ((getTriggeredAlarms() == null) ? 0 : getTriggeredAlarms().hashCode());
hashCode = prime * hashCode + ((getAutomationSubtype() == null) ? 0 : getAutomationSubtype().hashCode());
hashCode = prime * hashCode + ((getScheduledTime() == null) ? 0 : getScheduledTime().hashCode());
hashCode = prime * hashCode + ((getRunbooks() == null) ? 0 : getRunbooks().hashCode());
hashCode = prime * hashCode + ((getOpsItemId() == null) ? 0 : getOpsItemId().hashCode());
hashCode = prime * hashCode + ((getAssociationId() == null) ? 0 : getAssociationId().hashCode());
hashCode = prime * hashCode + ((getChangeRequestName() == null) ? 0 : getChangeRequestName().hashCode());
return hashCode;
}
@Override
public AutomationExecutionMetadata clone() {
try {
return (AutomationExecutionMetadata) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.AutomationExecutionMetadataMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}