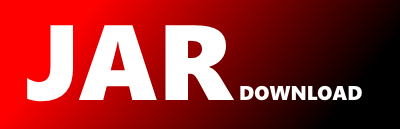
com.amazonaws.services.simplesystemsmanagement.model.Command Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a command request.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Command implements Serializable, Cloneable, StructuredPojo {
/**
*
* A unique identifier for this command.
*
*/
private String commandId;
/**
*
* The name of the document requested for execution.
*
*/
private String documentName;
/**
*
* The Systems Manager document (SSM document) version.
*
*/
private String documentVersion;
/**
*
* User-specified information about the command, such as a brief description of what the command should do.
*
*/
private String comment;
/**
*
* If a command expires, it changes status to DeliveryTimedOut
for all invocations that have the status
* InProgress
, Pending
, or Delayed
. ExpiresAfter
is calculated
* based on the total timeout for the overall command. For more information, see Understanding command timeout values in the Amazon Web Services Systems Manager User Guide.
*
*/
private java.util.Date expiresAfter;
/**
*
* The parameter values to be inserted in the document when running the command.
*
*/
private java.util.Map> parameters;
/**
*
* The managed node IDs against which this command was requested.
*
*/
private com.amazonaws.internal.SdkInternalList instanceIds;
/**
*
* An array of search criteria that targets managed nodes using a Key,Value combination that you specify. Targets is
* required if you don't provide one or more managed node IDs in the call.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The date and time the command was requested.
*
*/
private java.util.Date requestedDateTime;
/**
*
* The status of the command.
*
*/
private String status;
/**
*
* A detailed status of the command execution. StatusDetails
includes more information than
* Status
because it includes states resulting from error and concurrency control parameters.
* StatusDetails
can show different results than Status. For more information about these statuses, see
* Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be one of
* the following values:
*
*
* -
*
* Pending: The command hasn't been sent to any managed nodes.
*
*
* -
*
* In Progress: The command has been sent to at least one managed node but hasn't reached a final state on all
* managed nodes.
*
*
* -
*
* Success: The command successfully ran on all invocations. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The value of MaxErrors or more command invocations shows a status of Delivery Timed Out. This
* is a terminal state.
*
*
* -
*
* Execution Timed Out: The value of MaxErrors or more command invocations shows a status of Execution Timed Out.
* This is a terminal state.
*
*
* -
*
* Failed: The value of MaxErrors or more command invocations shows a status of Failed. This is a terminal state.
*
*
* -
*
* Incomplete: The command was attempted on all managed nodes and one or more invocations doesn't have a value of
* Success but not enough invocations failed for the status to be Failed. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Rate Exceeded: The number of managed nodes targeted by the command exceeded the account limit for pending
* invocations. The system has canceled the command before running it on any managed node. This is a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the managed node but wasn't successful. The system retries
* again.
*
*
*
*/
private String statusDetails;
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*
*/
private String outputS3Region;
/**
*
* The S3 bucket where the responses to the command executions should be stored. This was requested when issuing the
* command.
*
*/
private String outputS3BucketName;
/**
*
* The S3 directory path inside the bucket where the responses to the command executions should be stored. This was
* requested when issuing the command.
*
*/
private String outputS3KeyPrefix;
/**
*
* The maximum number of managed nodes that are allowed to run the command at the same time. You can specify a
* number of managed nodes, such as 10, or a percentage of nodes, such as 10%. The default value is 50. For more
* information about how to use MaxConcurrency
, see Amazon Web Services Systems
* Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*
*/
private String maxConcurrency;
/**
*
* The maximum number of errors allowed before the system stops sending the command to additional targets. You can
* specify a number of errors, such as 10, or a percentage or errors, such as 10%. The default value is
* 0
. For more information about how to use MaxErrors
, see Amazon Web Services Systems
* Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*
*/
private String maxErrors;
/**
*
* The number of targets for the command.
*
*/
private Integer targetCount;
/**
*
* The number of targets for which the command invocation reached a terminal state. Terminal states include the
* following: Success, Failed, Execution Timed Out, Delivery Timed Out, Cancelled, Terminated, or Undeliverable.
*
*/
private Integer completedCount;
/**
*
* The number of targets for which the status is Failed or Execution Timed Out.
*
*/
private Integer errorCount;
/**
*
* The number of targets for which the status is Delivery Timed Out.
*
*/
private Integer deliveryTimedOutCount;
/**
*
* The Identity and Access Management (IAM) service role that Run Command, a capability of Amazon Web Services
* Systems Manager, uses to act on your behalf when sending notifications about command status changes.
*
*/
private String serviceRole;
/**
*
* Configurations for sending notifications about command status changes.
*
*/
private NotificationConfig notificationConfig;
/**
*
* Amazon CloudWatch Logs information where you want Amazon Web Services Systems Manager to send the command output.
*
*/
private CloudWatchOutputConfig cloudWatchOutputConfig;
/**
*
* The TimeoutSeconds
value specified for a command.
*
*/
private Integer timeoutSeconds;
/**
*
* The details for the CloudWatch alarm applied to your command.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The CloudWatch alarm that was invoked by the command.
*
*/
private com.amazonaws.internal.SdkInternalList triggeredAlarms;
/**
*
* A unique identifier for this command.
*
*
* @param commandId
* A unique identifier for this command.
*/
public void setCommandId(String commandId) {
this.commandId = commandId;
}
/**
*
* A unique identifier for this command.
*
*
* @return A unique identifier for this command.
*/
public String getCommandId() {
return this.commandId;
}
/**
*
* A unique identifier for this command.
*
*
* @param commandId
* A unique identifier for this command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withCommandId(String commandId) {
setCommandId(commandId);
return this;
}
/**
*
* The name of the document requested for execution.
*
*
* @param documentName
* The name of the document requested for execution.
*/
public void setDocumentName(String documentName) {
this.documentName = documentName;
}
/**
*
* The name of the document requested for execution.
*
*
* @return The name of the document requested for execution.
*/
public String getDocumentName() {
return this.documentName;
}
/**
*
* The name of the document requested for execution.
*
*
* @param documentName
* The name of the document requested for execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withDocumentName(String documentName) {
setDocumentName(documentName);
return this;
}
/**
*
* The Systems Manager document (SSM document) version.
*
*
* @param documentVersion
* The Systems Manager document (SSM document) version.
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The Systems Manager document (SSM document) version.
*
*
* @return The Systems Manager document (SSM document) version.
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The Systems Manager document (SSM document) version.
*
*
* @param documentVersion
* The Systems Manager document (SSM document) version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* User-specified information about the command, such as a brief description of what the command should do.
*
*
* @param comment
* User-specified information about the command, such as a brief description of what the command should do.
*/
public void setComment(String comment) {
this.comment = comment;
}
/**
*
* User-specified information about the command, such as a brief description of what the command should do.
*
*
* @return User-specified information about the command, such as a brief description of what the command should do.
*/
public String getComment() {
return this.comment;
}
/**
*
* User-specified information about the command, such as a brief description of what the command should do.
*
*
* @param comment
* User-specified information about the command, such as a brief description of what the command should do.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withComment(String comment) {
setComment(comment);
return this;
}
/**
*
* If a command expires, it changes status to DeliveryTimedOut
for all invocations that have the status
* InProgress
, Pending
, or Delayed
. ExpiresAfter
is calculated
* based on the total timeout for the overall command. For more information, see Understanding command timeout values in the Amazon Web Services Systems Manager User Guide.
*
*
* @param expiresAfter
* If a command expires, it changes status to DeliveryTimedOut
for all invocations that have the
* status InProgress
, Pending
, or Delayed
. ExpiresAfter
* is calculated based on the total timeout for the overall command. For more information, see Understanding command timeout values in the Amazon Web Services Systems Manager User Guide.
*/
public void setExpiresAfter(java.util.Date expiresAfter) {
this.expiresAfter = expiresAfter;
}
/**
*
* If a command expires, it changes status to DeliveryTimedOut
for all invocations that have the status
* InProgress
, Pending
, or Delayed
. ExpiresAfter
is calculated
* based on the total timeout for the overall command. For more information, see Understanding command timeout values in the Amazon Web Services Systems Manager User Guide.
*
*
* @return If a command expires, it changes status to DeliveryTimedOut
for all invocations that have
* the status InProgress
, Pending
, or Delayed
.
* ExpiresAfter
is calculated based on the total timeout for the overall command. For more
* information, see Understanding command timeout values in the Amazon Web Services Systems Manager User Guide.
*/
public java.util.Date getExpiresAfter() {
return this.expiresAfter;
}
/**
*
* If a command expires, it changes status to DeliveryTimedOut
for all invocations that have the status
* InProgress
, Pending
, or Delayed
. ExpiresAfter
is calculated
* based on the total timeout for the overall command. For more information, see Understanding command timeout values in the Amazon Web Services Systems Manager User Guide.
*
*
* @param expiresAfter
* If a command expires, it changes status to DeliveryTimedOut
for all invocations that have the
* status InProgress
, Pending
, or Delayed
. ExpiresAfter
* is calculated based on the total timeout for the overall command. For more information, see Understanding command timeout values in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withExpiresAfter(java.util.Date expiresAfter) {
setExpiresAfter(expiresAfter);
return this;
}
/**
*
* The parameter values to be inserted in the document when running the command.
*
*
* @return The parameter values to be inserted in the document when running the command.
*/
public java.util.Map> getParameters() {
return parameters;
}
/**
*
* The parameter values to be inserted in the document when running the command.
*
*
* @param parameters
* The parameter values to be inserted in the document when running the command.
*/
public void setParameters(java.util.Map> parameters) {
this.parameters = parameters;
}
/**
*
* The parameter values to be inserted in the document when running the command.
*
*
* @param parameters
* The parameter values to be inserted in the document when running the command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withParameters(java.util.Map> parameters) {
setParameters(parameters);
return this;
}
/**
* Add a single Parameters entry
*
* @see Command#withParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public Command addParametersEntry(String key, java.util.List value) {
if (null == this.parameters) {
this.parameters = new java.util.HashMap>();
}
if (this.parameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.parameters.put(key, value);
return this;
}
/**
* Removes all the entries added into Parameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command clearParametersEntries() {
this.parameters = null;
return this;
}
/**
*
* The managed node IDs against which this command was requested.
*
*
* @return The managed node IDs against which this command was requested.
*/
public java.util.List getInstanceIds() {
if (instanceIds == null) {
instanceIds = new com.amazonaws.internal.SdkInternalList();
}
return instanceIds;
}
/**
*
* The managed node IDs against which this command was requested.
*
*
* @param instanceIds
* The managed node IDs against which this command was requested.
*/
public void setInstanceIds(java.util.Collection instanceIds) {
if (instanceIds == null) {
this.instanceIds = null;
return;
}
this.instanceIds = new com.amazonaws.internal.SdkInternalList(instanceIds);
}
/**
*
* The managed node IDs against which this command was requested.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInstanceIds(java.util.Collection)} or {@link #withInstanceIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param instanceIds
* The managed node IDs against which this command was requested.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withInstanceIds(String... instanceIds) {
if (this.instanceIds == null) {
setInstanceIds(new com.amazonaws.internal.SdkInternalList(instanceIds.length));
}
for (String ele : instanceIds) {
this.instanceIds.add(ele);
}
return this;
}
/**
*
* The managed node IDs against which this command was requested.
*
*
* @param instanceIds
* The managed node IDs against which this command was requested.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withInstanceIds(java.util.Collection instanceIds) {
setInstanceIds(instanceIds);
return this;
}
/**
*
* An array of search criteria that targets managed nodes using a Key,Value combination that you specify. Targets is
* required if you don't provide one or more managed node IDs in the call.
*
*
* @return An array of search criteria that targets managed nodes using a Key,Value combination that you specify.
* Targets is required if you don't provide one or more managed node IDs in the call.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* An array of search criteria that targets managed nodes using a Key,Value combination that you specify. Targets is
* required if you don't provide one or more managed node IDs in the call.
*
*
* @param targets
* An array of search criteria that targets managed nodes using a Key,Value combination that you specify.
* Targets is required if you don't provide one or more managed node IDs in the call.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* An array of search criteria that targets managed nodes using a Key,Value combination that you specify. Targets is
* required if you don't provide one or more managed node IDs in the call.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* An array of search criteria that targets managed nodes using a Key,Value combination that you specify.
* Targets is required if you don't provide one or more managed node IDs in the call.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* An array of search criteria that targets managed nodes using a Key,Value combination that you specify. Targets is
* required if you don't provide one or more managed node IDs in the call.
*
*
* @param targets
* An array of search criteria that targets managed nodes using a Key,Value combination that you specify.
* Targets is required if you don't provide one or more managed node IDs in the call.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The date and time the command was requested.
*
*
* @param requestedDateTime
* The date and time the command was requested.
*/
public void setRequestedDateTime(java.util.Date requestedDateTime) {
this.requestedDateTime = requestedDateTime;
}
/**
*
* The date and time the command was requested.
*
*
* @return The date and time the command was requested.
*/
public java.util.Date getRequestedDateTime() {
return this.requestedDateTime;
}
/**
*
* The date and time the command was requested.
*
*
* @param requestedDateTime
* The date and time the command was requested.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withRequestedDateTime(java.util.Date requestedDateTime) {
setRequestedDateTime(requestedDateTime);
return this;
}
/**
*
* The status of the command.
*
*
* @param status
* The status of the command.
* @see CommandStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the command.
*
*
* @return The status of the command.
* @see CommandStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the command.
*
*
* @param status
* The status of the command.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CommandStatus
*/
public Command withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the command.
*
*
* @param status
* The status of the command.
* @see CommandStatus
*/
public void setStatus(CommandStatus status) {
withStatus(status);
}
/**
*
* The status of the command.
*
*
* @param status
* The status of the command.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CommandStatus
*/
public Command withStatus(CommandStatus status) {
this.status = status.toString();
return this;
}
/**
*
* A detailed status of the command execution. StatusDetails
includes more information than
* Status
because it includes states resulting from error and concurrency control parameters.
* StatusDetails
can show different results than Status. For more information about these statuses, see
* Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be one of
* the following values:
*
*
* -
*
* Pending: The command hasn't been sent to any managed nodes.
*
*
* -
*
* In Progress: The command has been sent to at least one managed node but hasn't reached a final state on all
* managed nodes.
*
*
* -
*
* Success: The command successfully ran on all invocations. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The value of MaxErrors or more command invocations shows a status of Delivery Timed Out. This
* is a terminal state.
*
*
* -
*
* Execution Timed Out: The value of MaxErrors or more command invocations shows a status of Execution Timed Out.
* This is a terminal state.
*
*
* -
*
* Failed: The value of MaxErrors or more command invocations shows a status of Failed. This is a terminal state.
*
*
* -
*
* Incomplete: The command was attempted on all managed nodes and one or more invocations doesn't have a value of
* Success but not enough invocations failed for the status to be Failed. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Rate Exceeded: The number of managed nodes targeted by the command exceeded the account limit for pending
* invocations. The system has canceled the command before running it on any managed node. This is a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the managed node but wasn't successful. The system retries
* again.
*
*
*
*
* @param statusDetails
* A detailed status of the command execution. StatusDetails
includes more information than
* Status
because it includes states resulting from error and concurrency control parameters.
* StatusDetails
can show different results than Status. For more information about these
* statuses, see Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be
* one of the following values:
*
* -
*
* Pending: The command hasn't been sent to any managed nodes.
*
*
* -
*
* In Progress: The command has been sent to at least one managed node but hasn't reached a final state on
* all managed nodes.
*
*
* -
*
* Success: The command successfully ran on all invocations. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The value of MaxErrors or more command invocations shows a status of Delivery Timed
* Out. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The value of MaxErrors or more command invocations shows a status of Execution Timed
* Out. This is a terminal state.
*
*
* -
*
* Failed: The value of MaxErrors or more command invocations shows a status of Failed. This is a terminal
* state.
*
*
* -
*
* Incomplete: The command was attempted on all managed nodes and one or more invocations doesn't have a
* value of Success but not enough invocations failed for the status to be Failed. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Rate Exceeded: The number of managed nodes targeted by the command exceeded the account limit for pending
* invocations. The system has canceled the command before running it on any managed node. This is a terminal
* state.
*
*
* -
*
* Delayed: The system attempted to send the command to the managed node but wasn't successful. The system
* retries again.
*
*
*/
public void setStatusDetails(String statusDetails) {
this.statusDetails = statusDetails;
}
/**
*
* A detailed status of the command execution. StatusDetails
includes more information than
* Status
because it includes states resulting from error and concurrency control parameters.
* StatusDetails
can show different results than Status. For more information about these statuses, see
* Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be one of
* the following values:
*
*
* -
*
* Pending: The command hasn't been sent to any managed nodes.
*
*
* -
*
* In Progress: The command has been sent to at least one managed node but hasn't reached a final state on all
* managed nodes.
*
*
* -
*
* Success: The command successfully ran on all invocations. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The value of MaxErrors or more command invocations shows a status of Delivery Timed Out. This
* is a terminal state.
*
*
* -
*
* Execution Timed Out: The value of MaxErrors or more command invocations shows a status of Execution Timed Out.
* This is a terminal state.
*
*
* -
*
* Failed: The value of MaxErrors or more command invocations shows a status of Failed. This is a terminal state.
*
*
* -
*
* Incomplete: The command was attempted on all managed nodes and one or more invocations doesn't have a value of
* Success but not enough invocations failed for the status to be Failed. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Rate Exceeded: The number of managed nodes targeted by the command exceeded the account limit for pending
* invocations. The system has canceled the command before running it on any managed node. This is a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the managed node but wasn't successful. The system retries
* again.
*
*
*
*
* @return A detailed status of the command execution. StatusDetails
includes more information than
* Status
because it includes states resulting from error and concurrency control parameters.
* StatusDetails
can show different results than Status. For more information about these
* statuses, see Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be
* one of the following values:
*
* -
*
* Pending: The command hasn't been sent to any managed nodes.
*
*
* -
*
* In Progress: The command has been sent to at least one managed node but hasn't reached a final state on
* all managed nodes.
*
*
* -
*
* Success: The command successfully ran on all invocations. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The value of MaxErrors or more command invocations shows a status of Delivery Timed
* Out. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The value of MaxErrors or more command invocations shows a status of Execution Timed
* Out. This is a terminal state.
*
*
* -
*
* Failed: The value of MaxErrors or more command invocations shows a status of Failed. This is a terminal
* state.
*
*
* -
*
* Incomplete: The command was attempted on all managed nodes and one or more invocations doesn't have a
* value of Success but not enough invocations failed for the status to be Failed. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Rate Exceeded: The number of managed nodes targeted by the command exceeded the account limit for pending
* invocations. The system has canceled the command before running it on any managed node. This is a
* terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the managed node but wasn't successful. The system
* retries again.
*
*
*/
public String getStatusDetails() {
return this.statusDetails;
}
/**
*
* A detailed status of the command execution. StatusDetails
includes more information than
* Status
because it includes states resulting from error and concurrency control parameters.
* StatusDetails
can show different results than Status. For more information about these statuses, see
* Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be one of
* the following values:
*
*
* -
*
* Pending: The command hasn't been sent to any managed nodes.
*
*
* -
*
* In Progress: The command has been sent to at least one managed node but hasn't reached a final state on all
* managed nodes.
*
*
* -
*
* Success: The command successfully ran on all invocations. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The value of MaxErrors or more command invocations shows a status of Delivery Timed Out. This
* is a terminal state.
*
*
* -
*
* Execution Timed Out: The value of MaxErrors or more command invocations shows a status of Execution Timed Out.
* This is a terminal state.
*
*
* -
*
* Failed: The value of MaxErrors or more command invocations shows a status of Failed. This is a terminal state.
*
*
* -
*
* Incomplete: The command was attempted on all managed nodes and one or more invocations doesn't have a value of
* Success but not enough invocations failed for the status to be Failed. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Rate Exceeded: The number of managed nodes targeted by the command exceeded the account limit for pending
* invocations. The system has canceled the command before running it on any managed node. This is a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the managed node but wasn't successful. The system retries
* again.
*
*
*
*
* @param statusDetails
* A detailed status of the command execution. StatusDetails
includes more information than
* Status
because it includes states resulting from error and concurrency control parameters.
* StatusDetails
can show different results than Status. For more information about these
* statuses, see Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be
* one of the following values:
*
* -
*
* Pending: The command hasn't been sent to any managed nodes.
*
*
* -
*
* In Progress: The command has been sent to at least one managed node but hasn't reached a final state on
* all managed nodes.
*
*
* -
*
* Success: The command successfully ran on all invocations. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The value of MaxErrors or more command invocations shows a status of Delivery Timed
* Out. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The value of MaxErrors or more command invocations shows a status of Execution Timed
* Out. This is a terminal state.
*
*
* -
*
* Failed: The value of MaxErrors or more command invocations shows a status of Failed. This is a terminal
* state.
*
*
* -
*
* Incomplete: The command was attempted on all managed nodes and one or more invocations doesn't have a
* value of Success but not enough invocations failed for the status to be Failed. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Rate Exceeded: The number of managed nodes targeted by the command exceeded the account limit for pending
* invocations. The system has canceled the command before running it on any managed node. This is a terminal
* state.
*
*
* -
*
* Delayed: The system attempted to send the command to the managed node but wasn't successful. The system
* retries again.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withStatusDetails(String statusDetails) {
setStatusDetails(statusDetails);
return this;
}
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*
*
* @param outputS3Region
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*/
public void setOutputS3Region(String outputS3Region) {
this.outputS3Region = outputS3Region;
}
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*
*
* @return (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*/
public String getOutputS3Region() {
return this.outputS3Region;
}
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*
*
* @param outputS3Region
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withOutputS3Region(String outputS3Region) {
setOutputS3Region(outputS3Region);
return this;
}
/**
*
* The S3 bucket where the responses to the command executions should be stored. This was requested when issuing the
* command.
*
*
* @param outputS3BucketName
* The S3 bucket where the responses to the command executions should be stored. This was requested when
* issuing the command.
*/
public void setOutputS3BucketName(String outputS3BucketName) {
this.outputS3BucketName = outputS3BucketName;
}
/**
*
* The S3 bucket where the responses to the command executions should be stored. This was requested when issuing the
* command.
*
*
* @return The S3 bucket where the responses to the command executions should be stored. This was requested when
* issuing the command.
*/
public String getOutputS3BucketName() {
return this.outputS3BucketName;
}
/**
*
* The S3 bucket where the responses to the command executions should be stored. This was requested when issuing the
* command.
*
*
* @param outputS3BucketName
* The S3 bucket where the responses to the command executions should be stored. This was requested when
* issuing the command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withOutputS3BucketName(String outputS3BucketName) {
setOutputS3BucketName(outputS3BucketName);
return this;
}
/**
*
* The S3 directory path inside the bucket where the responses to the command executions should be stored. This was
* requested when issuing the command.
*
*
* @param outputS3KeyPrefix
* The S3 directory path inside the bucket where the responses to the command executions should be stored.
* This was requested when issuing the command.
*/
public void setOutputS3KeyPrefix(String outputS3KeyPrefix) {
this.outputS3KeyPrefix = outputS3KeyPrefix;
}
/**
*
* The S3 directory path inside the bucket where the responses to the command executions should be stored. This was
* requested when issuing the command.
*
*
* @return The S3 directory path inside the bucket where the responses to the command executions should be stored.
* This was requested when issuing the command.
*/
public String getOutputS3KeyPrefix() {
return this.outputS3KeyPrefix;
}
/**
*
* The S3 directory path inside the bucket where the responses to the command executions should be stored. This was
* requested when issuing the command.
*
*
* @param outputS3KeyPrefix
* The S3 directory path inside the bucket where the responses to the command executions should be stored.
* This was requested when issuing the command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withOutputS3KeyPrefix(String outputS3KeyPrefix) {
setOutputS3KeyPrefix(outputS3KeyPrefix);
return this;
}
/**
*
* The maximum number of managed nodes that are allowed to run the command at the same time. You can specify a
* number of managed nodes, such as 10, or a percentage of nodes, such as 10%. The default value is 50. For more
* information about how to use MaxConcurrency
, see Amazon Web Services Systems
* Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*
*
* @param maxConcurrency
* The maximum number of managed nodes that are allowed to run the command at the same time. You can specify
* a number of managed nodes, such as 10, or a percentage of nodes, such as 10%. The default value is 50. For
* more information about how to use MaxConcurrency
, see Amazon Web Services
* Systems Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The maximum number of managed nodes that are allowed to run the command at the same time. You can specify a
* number of managed nodes, such as 10, or a percentage of nodes, such as 10%. The default value is 50. For more
* information about how to use MaxConcurrency
, see Amazon Web Services Systems
* Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*
*
* @return The maximum number of managed nodes that are allowed to run the command at the same time. You can specify
* a number of managed nodes, such as 10, or a percentage of nodes, such as 10%. The default value is 50.
* For more information about how to use MaxConcurrency
, see Amazon Web Services
* Systems Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The maximum number of managed nodes that are allowed to run the command at the same time. You can specify a
* number of managed nodes, such as 10, or a percentage of nodes, such as 10%. The default value is 50. For more
* information about how to use MaxConcurrency
, see Amazon Web Services Systems
* Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*
*
* @param maxConcurrency
* The maximum number of managed nodes that are allowed to run the command at the same time. You can specify
* a number of managed nodes, such as 10, or a percentage of nodes, such as 10%. The default value is 50. For
* more information about how to use MaxConcurrency
, see Amazon Web Services
* Systems Manager Run Command in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The maximum number of errors allowed before the system stops sending the command to additional targets. You can
* specify a number of errors, such as 10, or a percentage or errors, such as 10%. The default value is
* 0
. For more information about how to use MaxErrors
, see Amazon Web Services Systems
* Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*
*
* @param maxErrors
* The maximum number of errors allowed before the system stops sending the command to additional targets.
* You can specify a number of errors, such as 10, or a percentage or errors, such as 10%. The default value
* is 0
. For more information about how to use MaxErrors
, see Amazon Web Services
* Systems Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The maximum number of errors allowed before the system stops sending the command to additional targets. You can
* specify a number of errors, such as 10, or a percentage or errors, such as 10%. The default value is
* 0
. For more information about how to use MaxErrors
, see Amazon Web Services Systems
* Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*
*
* @return The maximum number of errors allowed before the system stops sending the command to additional targets.
* You can specify a number of errors, such as 10, or a percentage or errors, such as 10%. The default value
* is 0
. For more information about how to use MaxErrors
, see Amazon Web Services
* Systems Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The maximum number of errors allowed before the system stops sending the command to additional targets. You can
* specify a number of errors, such as 10, or a percentage or errors, such as 10%. The default value is
* 0
. For more information about how to use MaxErrors
, see Amazon Web Services Systems
* Manager Run Command in the Amazon Web Services Systems Manager User Guide.
*
*
* @param maxErrors
* The maximum number of errors allowed before the system stops sending the command to additional targets.
* You can specify a number of errors, such as 10, or a percentage or errors, such as 10%. The default value
* is 0
. For more information about how to use MaxErrors
, see Amazon Web Services
* Systems Manager Run Command in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The number of targets for the command.
*
*
* @param targetCount
* The number of targets for the command.
*/
public void setTargetCount(Integer targetCount) {
this.targetCount = targetCount;
}
/**
*
* The number of targets for the command.
*
*
* @return The number of targets for the command.
*/
public Integer getTargetCount() {
return this.targetCount;
}
/**
*
* The number of targets for the command.
*
*
* @param targetCount
* The number of targets for the command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withTargetCount(Integer targetCount) {
setTargetCount(targetCount);
return this;
}
/**
*
* The number of targets for which the command invocation reached a terminal state. Terminal states include the
* following: Success, Failed, Execution Timed Out, Delivery Timed Out, Cancelled, Terminated, or Undeliverable.
*
*
* @param completedCount
* The number of targets for which the command invocation reached a terminal state. Terminal states include
* the following: Success, Failed, Execution Timed Out, Delivery Timed Out, Cancelled, Terminated, or
* Undeliverable.
*/
public void setCompletedCount(Integer completedCount) {
this.completedCount = completedCount;
}
/**
*
* The number of targets for which the command invocation reached a terminal state. Terminal states include the
* following: Success, Failed, Execution Timed Out, Delivery Timed Out, Cancelled, Terminated, or Undeliverable.
*
*
* @return The number of targets for which the command invocation reached a terminal state. Terminal states include
* the following: Success, Failed, Execution Timed Out, Delivery Timed Out, Cancelled, Terminated, or
* Undeliverable.
*/
public Integer getCompletedCount() {
return this.completedCount;
}
/**
*
* The number of targets for which the command invocation reached a terminal state. Terminal states include the
* following: Success, Failed, Execution Timed Out, Delivery Timed Out, Cancelled, Terminated, or Undeliverable.
*
*
* @param completedCount
* The number of targets for which the command invocation reached a terminal state. Terminal states include
* the following: Success, Failed, Execution Timed Out, Delivery Timed Out, Cancelled, Terminated, or
* Undeliverable.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withCompletedCount(Integer completedCount) {
setCompletedCount(completedCount);
return this;
}
/**
*
* The number of targets for which the status is Failed or Execution Timed Out.
*
*
* @param errorCount
* The number of targets for which the status is Failed or Execution Timed Out.
*/
public void setErrorCount(Integer errorCount) {
this.errorCount = errorCount;
}
/**
*
* The number of targets for which the status is Failed or Execution Timed Out.
*
*
* @return The number of targets for which the status is Failed or Execution Timed Out.
*/
public Integer getErrorCount() {
return this.errorCount;
}
/**
*
* The number of targets for which the status is Failed or Execution Timed Out.
*
*
* @param errorCount
* The number of targets for which the status is Failed or Execution Timed Out.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withErrorCount(Integer errorCount) {
setErrorCount(errorCount);
return this;
}
/**
*
* The number of targets for which the status is Delivery Timed Out.
*
*
* @param deliveryTimedOutCount
* The number of targets for which the status is Delivery Timed Out.
*/
public void setDeliveryTimedOutCount(Integer deliveryTimedOutCount) {
this.deliveryTimedOutCount = deliveryTimedOutCount;
}
/**
*
* The number of targets for which the status is Delivery Timed Out.
*
*
* @return The number of targets for which the status is Delivery Timed Out.
*/
public Integer getDeliveryTimedOutCount() {
return this.deliveryTimedOutCount;
}
/**
*
* The number of targets for which the status is Delivery Timed Out.
*
*
* @param deliveryTimedOutCount
* The number of targets for which the status is Delivery Timed Out.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withDeliveryTimedOutCount(Integer deliveryTimedOutCount) {
setDeliveryTimedOutCount(deliveryTimedOutCount);
return this;
}
/**
*
* The Identity and Access Management (IAM) service role that Run Command, a capability of Amazon Web Services
* Systems Manager, uses to act on your behalf when sending notifications about command status changes.
*
*
* @param serviceRole
* The Identity and Access Management (IAM) service role that Run Command, a capability of Amazon Web
* Services Systems Manager, uses to act on your behalf when sending notifications about command status
* changes.
*/
public void setServiceRole(String serviceRole) {
this.serviceRole = serviceRole;
}
/**
*
* The Identity and Access Management (IAM) service role that Run Command, a capability of Amazon Web Services
* Systems Manager, uses to act on your behalf when sending notifications about command status changes.
*
*
* @return The Identity and Access Management (IAM) service role that Run Command, a capability of Amazon Web
* Services Systems Manager, uses to act on your behalf when sending notifications about command status
* changes.
*/
public String getServiceRole() {
return this.serviceRole;
}
/**
*
* The Identity and Access Management (IAM) service role that Run Command, a capability of Amazon Web Services
* Systems Manager, uses to act on your behalf when sending notifications about command status changes.
*
*
* @param serviceRole
* The Identity and Access Management (IAM) service role that Run Command, a capability of Amazon Web
* Services Systems Manager, uses to act on your behalf when sending notifications about command status
* changes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withServiceRole(String serviceRole) {
setServiceRole(serviceRole);
return this;
}
/**
*
* Configurations for sending notifications about command status changes.
*
*
* @param notificationConfig
* Configurations for sending notifications about command status changes.
*/
public void setNotificationConfig(NotificationConfig notificationConfig) {
this.notificationConfig = notificationConfig;
}
/**
*
* Configurations for sending notifications about command status changes.
*
*
* @return Configurations for sending notifications about command status changes.
*/
public NotificationConfig getNotificationConfig() {
return this.notificationConfig;
}
/**
*
* Configurations for sending notifications about command status changes.
*
*
* @param notificationConfig
* Configurations for sending notifications about command status changes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withNotificationConfig(NotificationConfig notificationConfig) {
setNotificationConfig(notificationConfig);
return this;
}
/**
*
* Amazon CloudWatch Logs information where you want Amazon Web Services Systems Manager to send the command output.
*
*
* @param cloudWatchOutputConfig
* Amazon CloudWatch Logs information where you want Amazon Web Services Systems Manager to send the command
* output.
*/
public void setCloudWatchOutputConfig(CloudWatchOutputConfig cloudWatchOutputConfig) {
this.cloudWatchOutputConfig = cloudWatchOutputConfig;
}
/**
*
* Amazon CloudWatch Logs information where you want Amazon Web Services Systems Manager to send the command output.
*
*
* @return Amazon CloudWatch Logs information where you want Amazon Web Services Systems Manager to send the command
* output.
*/
public CloudWatchOutputConfig getCloudWatchOutputConfig() {
return this.cloudWatchOutputConfig;
}
/**
*
* Amazon CloudWatch Logs information where you want Amazon Web Services Systems Manager to send the command output.
*
*
* @param cloudWatchOutputConfig
* Amazon CloudWatch Logs information where you want Amazon Web Services Systems Manager to send the command
* output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withCloudWatchOutputConfig(CloudWatchOutputConfig cloudWatchOutputConfig) {
setCloudWatchOutputConfig(cloudWatchOutputConfig);
return this;
}
/**
*
* The TimeoutSeconds
value specified for a command.
*
*
* @param timeoutSeconds
* The TimeoutSeconds
value specified for a command.
*/
public void setTimeoutSeconds(Integer timeoutSeconds) {
this.timeoutSeconds = timeoutSeconds;
}
/**
*
* The TimeoutSeconds
value specified for a command.
*
*
* @return The TimeoutSeconds
value specified for a command.
*/
public Integer getTimeoutSeconds() {
return this.timeoutSeconds;
}
/**
*
* The TimeoutSeconds
value specified for a command.
*
*
* @param timeoutSeconds
* The TimeoutSeconds
value specified for a command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withTimeoutSeconds(Integer timeoutSeconds) {
setTimeoutSeconds(timeoutSeconds);
return this;
}
/**
*
* The details for the CloudWatch alarm applied to your command.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm applied to your command.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm applied to your command.
*
*
* @return The details for the CloudWatch alarm applied to your command.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm applied to your command.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm applied to your command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
*
* The CloudWatch alarm that was invoked by the command.
*
*
* @return The CloudWatch alarm that was invoked by the command.
*/
public java.util.List getTriggeredAlarms() {
if (triggeredAlarms == null) {
triggeredAlarms = new com.amazonaws.internal.SdkInternalList();
}
return triggeredAlarms;
}
/**
*
* The CloudWatch alarm that was invoked by the command.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked by the command.
*/
public void setTriggeredAlarms(java.util.Collection triggeredAlarms) {
if (triggeredAlarms == null) {
this.triggeredAlarms = null;
return;
}
this.triggeredAlarms = new com.amazonaws.internal.SdkInternalList(triggeredAlarms);
}
/**
*
* The CloudWatch alarm that was invoked by the command.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTriggeredAlarms(java.util.Collection)} or {@link #withTriggeredAlarms(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked by the command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withTriggeredAlarms(AlarmStateInformation... triggeredAlarms) {
if (this.triggeredAlarms == null) {
setTriggeredAlarms(new com.amazonaws.internal.SdkInternalList(triggeredAlarms.length));
}
for (AlarmStateInformation ele : triggeredAlarms) {
this.triggeredAlarms.add(ele);
}
return this;
}
/**
*
* The CloudWatch alarm that was invoked by the command.
*
*
* @param triggeredAlarms
* The CloudWatch alarm that was invoked by the command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Command withTriggeredAlarms(java.util.Collection triggeredAlarms) {
setTriggeredAlarms(triggeredAlarms);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCommandId() != null)
sb.append("CommandId: ").append(getCommandId()).append(",");
if (getDocumentName() != null)
sb.append("DocumentName: ").append(getDocumentName()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getComment() != null)
sb.append("Comment: ").append(getComment()).append(",");
if (getExpiresAfter() != null)
sb.append("ExpiresAfter: ").append(getExpiresAfter()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append("***Sensitive Data Redacted***").append(",");
if (getInstanceIds() != null)
sb.append("InstanceIds: ").append(getInstanceIds()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getRequestedDateTime() != null)
sb.append("RequestedDateTime: ").append(getRequestedDateTime()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusDetails() != null)
sb.append("StatusDetails: ").append(getStatusDetails()).append(",");
if (getOutputS3Region() != null)
sb.append("OutputS3Region: ").append(getOutputS3Region()).append(",");
if (getOutputS3BucketName() != null)
sb.append("OutputS3BucketName: ").append(getOutputS3BucketName()).append(",");
if (getOutputS3KeyPrefix() != null)
sb.append("OutputS3KeyPrefix: ").append(getOutputS3KeyPrefix()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getTargetCount() != null)
sb.append("TargetCount: ").append(getTargetCount()).append(",");
if (getCompletedCount() != null)
sb.append("CompletedCount: ").append(getCompletedCount()).append(",");
if (getErrorCount() != null)
sb.append("ErrorCount: ").append(getErrorCount()).append(",");
if (getDeliveryTimedOutCount() != null)
sb.append("DeliveryTimedOutCount: ").append(getDeliveryTimedOutCount()).append(",");
if (getServiceRole() != null)
sb.append("ServiceRole: ").append(getServiceRole()).append(",");
if (getNotificationConfig() != null)
sb.append("NotificationConfig: ").append(getNotificationConfig()).append(",");
if (getCloudWatchOutputConfig() != null)
sb.append("CloudWatchOutputConfig: ").append(getCloudWatchOutputConfig()).append(",");
if (getTimeoutSeconds() != null)
sb.append("TimeoutSeconds: ").append(getTimeoutSeconds()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration()).append(",");
if (getTriggeredAlarms() != null)
sb.append("TriggeredAlarms: ").append(getTriggeredAlarms());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Command == false)
return false;
Command other = (Command) obj;
if (other.getCommandId() == null ^ this.getCommandId() == null)
return false;
if (other.getCommandId() != null && other.getCommandId().equals(this.getCommandId()) == false)
return false;
if (other.getDocumentName() == null ^ this.getDocumentName() == null)
return false;
if (other.getDocumentName() != null && other.getDocumentName().equals(this.getDocumentName()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getComment() == null ^ this.getComment() == null)
return false;
if (other.getComment() != null && other.getComment().equals(this.getComment()) == false)
return false;
if (other.getExpiresAfter() == null ^ this.getExpiresAfter() == null)
return false;
if (other.getExpiresAfter() != null && other.getExpiresAfter().equals(this.getExpiresAfter()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getInstanceIds() == null ^ this.getInstanceIds() == null)
return false;
if (other.getInstanceIds() != null && other.getInstanceIds().equals(this.getInstanceIds()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getRequestedDateTime() == null ^ this.getRequestedDateTime() == null)
return false;
if (other.getRequestedDateTime() != null && other.getRequestedDateTime().equals(this.getRequestedDateTime()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusDetails() == null ^ this.getStatusDetails() == null)
return false;
if (other.getStatusDetails() != null && other.getStatusDetails().equals(this.getStatusDetails()) == false)
return false;
if (other.getOutputS3Region() == null ^ this.getOutputS3Region() == null)
return false;
if (other.getOutputS3Region() != null && other.getOutputS3Region().equals(this.getOutputS3Region()) == false)
return false;
if (other.getOutputS3BucketName() == null ^ this.getOutputS3BucketName() == null)
return false;
if (other.getOutputS3BucketName() != null && other.getOutputS3BucketName().equals(this.getOutputS3BucketName()) == false)
return false;
if (other.getOutputS3KeyPrefix() == null ^ this.getOutputS3KeyPrefix() == null)
return false;
if (other.getOutputS3KeyPrefix() != null && other.getOutputS3KeyPrefix().equals(this.getOutputS3KeyPrefix()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getTargetCount() == null ^ this.getTargetCount() == null)
return false;
if (other.getTargetCount() != null && other.getTargetCount().equals(this.getTargetCount()) == false)
return false;
if (other.getCompletedCount() == null ^ this.getCompletedCount() == null)
return false;
if (other.getCompletedCount() != null && other.getCompletedCount().equals(this.getCompletedCount()) == false)
return false;
if (other.getErrorCount() == null ^ this.getErrorCount() == null)
return false;
if (other.getErrorCount() != null && other.getErrorCount().equals(this.getErrorCount()) == false)
return false;
if (other.getDeliveryTimedOutCount() == null ^ this.getDeliveryTimedOutCount() == null)
return false;
if (other.getDeliveryTimedOutCount() != null && other.getDeliveryTimedOutCount().equals(this.getDeliveryTimedOutCount()) == false)
return false;
if (other.getServiceRole() == null ^ this.getServiceRole() == null)
return false;
if (other.getServiceRole() != null && other.getServiceRole().equals(this.getServiceRole()) == false)
return false;
if (other.getNotificationConfig() == null ^ this.getNotificationConfig() == null)
return false;
if (other.getNotificationConfig() != null && other.getNotificationConfig().equals(this.getNotificationConfig()) == false)
return false;
if (other.getCloudWatchOutputConfig() == null ^ this.getCloudWatchOutputConfig() == null)
return false;
if (other.getCloudWatchOutputConfig() != null && other.getCloudWatchOutputConfig().equals(this.getCloudWatchOutputConfig()) == false)
return false;
if (other.getTimeoutSeconds() == null ^ this.getTimeoutSeconds() == null)
return false;
if (other.getTimeoutSeconds() != null && other.getTimeoutSeconds().equals(this.getTimeoutSeconds()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
if (other.getTriggeredAlarms() == null ^ this.getTriggeredAlarms() == null)
return false;
if (other.getTriggeredAlarms() != null && other.getTriggeredAlarms().equals(this.getTriggeredAlarms()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCommandId() == null) ? 0 : getCommandId().hashCode());
hashCode = prime * hashCode + ((getDocumentName() == null) ? 0 : getDocumentName().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getComment() == null) ? 0 : getComment().hashCode());
hashCode = prime * hashCode + ((getExpiresAfter() == null) ? 0 : getExpiresAfter().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getInstanceIds() == null) ? 0 : getInstanceIds().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getRequestedDateTime() == null) ? 0 : getRequestedDateTime().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusDetails() == null) ? 0 : getStatusDetails().hashCode());
hashCode = prime * hashCode + ((getOutputS3Region() == null) ? 0 : getOutputS3Region().hashCode());
hashCode = prime * hashCode + ((getOutputS3BucketName() == null) ? 0 : getOutputS3BucketName().hashCode());
hashCode = prime * hashCode + ((getOutputS3KeyPrefix() == null) ? 0 : getOutputS3KeyPrefix().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getTargetCount() == null) ? 0 : getTargetCount().hashCode());
hashCode = prime * hashCode + ((getCompletedCount() == null) ? 0 : getCompletedCount().hashCode());
hashCode = prime * hashCode + ((getErrorCount() == null) ? 0 : getErrorCount().hashCode());
hashCode = prime * hashCode + ((getDeliveryTimedOutCount() == null) ? 0 : getDeliveryTimedOutCount().hashCode());
hashCode = prime * hashCode + ((getServiceRole() == null) ? 0 : getServiceRole().hashCode());
hashCode = prime * hashCode + ((getNotificationConfig() == null) ? 0 : getNotificationConfig().hashCode());
hashCode = prime * hashCode + ((getCloudWatchOutputConfig() == null) ? 0 : getCloudWatchOutputConfig().hashCode());
hashCode = prime * hashCode + ((getTimeoutSeconds() == null) ? 0 : getTimeoutSeconds().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
hashCode = prime * hashCode + ((getTriggeredAlarms() == null) ? 0 : getTriggeredAlarms().hashCode());
return hashCode;
}
@Override
public Command clone() {
try {
return (Command) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.CommandMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}