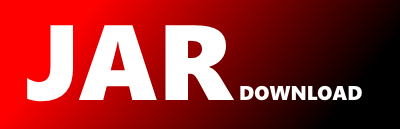
com.amazonaws.services.simplesystemsmanagement.model.CommandPlugin Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes plugin details.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CommandPlugin implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the plugin. Must be one of the following: aws:updateAgent
, aws:domainjoin
,
* aws:applications
, aws:runPowerShellScript
, aws:psmodule
,
* aws:cloudWatch
, aws:runShellScript
, or aws:updateSSMAgent
.
*
*/
private String name;
/**
*
* The status of this plugin. You can run a document with multiple plugins.
*
*/
private String status;
/**
*
* A detailed status of the plugin execution. StatusDetails
includes more information than Status
* because it includes states resulting from error and concurrency control parameters. StatusDetails can show
* different results than Status. For more information about these statuses, see Understanding command
* statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be one of the
* following values:
*
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Success: The execution of the command or plugin was successfully completed. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do contribute
* to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: Command execution started on the managed node, but the execution wasn't complete before the
* execution timeout expired. Execution timeouts count against the MaxErrors
limit of the parent
* command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't successful on the managed node. For a plugin, this indicates that the result code
* wasn't zero. For a command invocation, this indicates that the result code for one or more plugins wasn't zero.
* Invocation failures count against the MaxErrors limit of the parent command. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The managed node might not exist, or it might
* not be responding. Undeliverable invocations don't count against the parent command's MaxErrors limit, and they
* don't contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors limit and subsequent command invocations were canceled by
* the system. This is a terminal state.
*
*
*
*/
private String statusDetails;
/**
*
* A numeric response code generated after running the plugin.
*
*/
private Integer responseCode;
/**
*
* The time the plugin started running.
*
*/
private java.util.Date responseStartDateTime;
/**
*
* The time the plugin stopped running. Could stop prematurely if, for example, a cancel command was sent.
*
*/
private java.util.Date responseFinishDateTime;
/**
*
* Output of the plugin execution.
*
*/
private String output;
/**
*
* The URL for the complete text written by the plugin to stdout in Amazon S3. If the S3 bucket for the command
* wasn't specified, then this string is empty.
*
*/
private String standardOutputUrl;
/**
*
* The URL for the complete text written by the plugin to stderr. If execution isn't yet complete, then this string
* is empty.
*
*/
private String standardErrorUrl;
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Amazon Web Services
* Systems Manager automatically determines the S3 bucket region.
*
*/
private String outputS3Region;
/**
*
* The S3 bucket where the responses to the command executions should be stored. This was requested when issuing the
* command. For example, in the following response:
*
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*
*/
private String outputS3BucketName;
/**
*
* The S3 directory path inside the bucket where the responses to the command executions should be stored. This was
* requested when issuing the command. For example, in the following response:
*
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*
*/
private String outputS3KeyPrefix;
/**
*
* The name of the plugin. Must be one of the following: aws:updateAgent
, aws:domainjoin
,
* aws:applications
, aws:runPowerShellScript
, aws:psmodule
,
* aws:cloudWatch
, aws:runShellScript
, or aws:updateSSMAgent
.
*
*
* @param name
* The name of the plugin. Must be one of the following: aws:updateAgent
,
* aws:domainjoin
, aws:applications
, aws:runPowerShellScript
,
* aws:psmodule
, aws:cloudWatch
, aws:runShellScript
, or
* aws:updateSSMAgent
.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the plugin. Must be one of the following: aws:updateAgent
, aws:domainjoin
,
* aws:applications
, aws:runPowerShellScript
, aws:psmodule
,
* aws:cloudWatch
, aws:runShellScript
, or aws:updateSSMAgent
.
*
*
* @return The name of the plugin. Must be one of the following: aws:updateAgent
,
* aws:domainjoin
, aws:applications
, aws:runPowerShellScript
,
* aws:psmodule
, aws:cloudWatch
, aws:runShellScript
, or
* aws:updateSSMAgent
.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the plugin. Must be one of the following: aws:updateAgent
, aws:domainjoin
,
* aws:applications
, aws:runPowerShellScript
, aws:psmodule
,
* aws:cloudWatch
, aws:runShellScript
, or aws:updateSSMAgent
.
*
*
* @param name
* The name of the plugin. Must be one of the following: aws:updateAgent
,
* aws:domainjoin
, aws:applications
, aws:runPowerShellScript
,
* aws:psmodule
, aws:cloudWatch
, aws:runShellScript
, or
* aws:updateSSMAgent
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withName(String name) {
setName(name);
return this;
}
/**
*
* The status of this plugin. You can run a document with multiple plugins.
*
*
* @param status
* The status of this plugin. You can run a document with multiple plugins.
* @see CommandPluginStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of this plugin. You can run a document with multiple plugins.
*
*
* @return The status of this plugin. You can run a document with multiple plugins.
* @see CommandPluginStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of this plugin. You can run a document with multiple plugins.
*
*
* @param status
* The status of this plugin. You can run a document with multiple plugins.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CommandPluginStatus
*/
public CommandPlugin withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of this plugin. You can run a document with multiple plugins.
*
*
* @param status
* The status of this plugin. You can run a document with multiple plugins.
* @see CommandPluginStatus
*/
public void setStatus(CommandPluginStatus status) {
withStatus(status);
}
/**
*
* The status of this plugin. You can run a document with multiple plugins.
*
*
* @param status
* The status of this plugin. You can run a document with multiple plugins.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CommandPluginStatus
*/
public CommandPlugin withStatus(CommandPluginStatus status) {
this.status = status.toString();
return this;
}
/**
*
* A detailed status of the plugin execution. StatusDetails
includes more information than Status
* because it includes states resulting from error and concurrency control parameters. StatusDetails can show
* different results than Status. For more information about these statuses, see Understanding command
* statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be one of the
* following values:
*
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Success: The execution of the command or plugin was successfully completed. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do contribute
* to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: Command execution started on the managed node, but the execution wasn't complete before the
* execution timeout expired. Execution timeouts count against the MaxErrors
limit of the parent
* command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't successful on the managed node. For a plugin, this indicates that the result code
* wasn't zero. For a command invocation, this indicates that the result code for one or more plugins wasn't zero.
* Invocation failures count against the MaxErrors limit of the parent command. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The managed node might not exist, or it might
* not be responding. Undeliverable invocations don't count against the parent command's MaxErrors limit, and they
* don't contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors limit and subsequent command invocations were canceled by
* the system. This is a terminal state.
*
*
*
*
* @param statusDetails
* A detailed status of the plugin execution. StatusDetails
includes more information than
* Status because it includes states resulting from error and concurrency control parameters. StatusDetails
* can show different results than Status. For more information about these statuses, see Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be
* one of the following values:
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Success: The execution of the command or plugin was successfully completed. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do
* contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: Command execution started on the managed node, but the execution wasn't complete
* before the execution timeout expired. Execution timeouts count against the MaxErrors
limit of
* the parent command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't successful on the managed node. For a plugin, this indicates that the result
* code wasn't zero. For a command invocation, this indicates that the result code for one or more plugins
* wasn't zero. Invocation failures count against the MaxErrors limit of the parent command. This is a
* terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The managed node might not exist, or it
* might not be responding. Undeliverable invocations don't count against the parent command's MaxErrors
* limit, and they don't contribute to whether the parent command status is Success or Incomplete. This is a
* terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors limit and subsequent command invocations were
* canceled by the system. This is a terminal state.
*
*
*/
public void setStatusDetails(String statusDetails) {
this.statusDetails = statusDetails;
}
/**
*
* A detailed status of the plugin execution. StatusDetails
includes more information than Status
* because it includes states resulting from error and concurrency control parameters. StatusDetails can show
* different results than Status. For more information about these statuses, see Understanding command
* statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be one of the
* following values:
*
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Success: The execution of the command or plugin was successfully completed. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do contribute
* to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: Command execution started on the managed node, but the execution wasn't complete before the
* execution timeout expired. Execution timeouts count against the MaxErrors
limit of the parent
* command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't successful on the managed node. For a plugin, this indicates that the result code
* wasn't zero. For a command invocation, this indicates that the result code for one or more plugins wasn't zero.
* Invocation failures count against the MaxErrors limit of the parent command. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The managed node might not exist, or it might
* not be responding. Undeliverable invocations don't count against the parent command's MaxErrors limit, and they
* don't contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors limit and subsequent command invocations were canceled by
* the system. This is a terminal state.
*
*
*
*
* @return A detailed status of the plugin execution. StatusDetails
includes more information than
* Status because it includes states resulting from error and concurrency control parameters. StatusDetails
* can show different results than Status. For more information about these statuses, see Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be
* one of the following values:
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Success: The execution of the command or plugin was successfully completed. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do
* contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: Command execution started on the managed node, but the execution wasn't complete
* before the execution timeout expired. Execution timeouts count against the MaxErrors
limit
* of the parent command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't successful on the managed node. For a plugin, this indicates that the result
* code wasn't zero. For a command invocation, this indicates that the result code for one or more plugins
* wasn't zero. Invocation failures count against the MaxErrors limit of the parent command. This is a
* terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The managed node might not exist, or
* it might not be responding. Undeliverable invocations don't count against the parent command's MaxErrors
* limit, and they don't contribute to whether the parent command status is Success or Incomplete. This is a
* terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors limit and subsequent command invocations were
* canceled by the system. This is a terminal state.
*
*
*/
public String getStatusDetails() {
return this.statusDetails;
}
/**
*
* A detailed status of the plugin execution. StatusDetails
includes more information than Status
* because it includes states resulting from error and concurrency control parameters. StatusDetails can show
* different results than Status. For more information about these statuses, see Understanding command
* statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be one of the
* following values:
*
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Success: The execution of the command or plugin was successfully completed. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do contribute
* to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: Command execution started on the managed node, but the execution wasn't complete before the
* execution timeout expired. Execution timeouts count against the MaxErrors
limit of the parent
* command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't successful on the managed node. For a plugin, this indicates that the result code
* wasn't zero. For a command invocation, this indicates that the result code for one or more plugins wasn't zero.
* Invocation failures count against the MaxErrors limit of the parent command. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The managed node might not exist, or it might
* not be responding. Undeliverable invocations don't count against the parent command's MaxErrors limit, and they
* don't contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors limit and subsequent command invocations were canceled by
* the system. This is a terminal state.
*
*
*
*
* @param statusDetails
* A detailed status of the plugin execution. StatusDetails
includes more information than
* Status because it includes states resulting from error and concurrency control parameters. StatusDetails
* can show different results than Status. For more information about these statuses, see Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails can be
* one of the following values:
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Success: The execution of the command or plugin was successfully completed. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do
* contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: Command execution started on the managed node, but the execution wasn't complete
* before the execution timeout expired. Execution timeouts count against the MaxErrors
limit of
* the parent command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't successful on the managed node. For a plugin, this indicates that the result
* code wasn't zero. For a command invocation, this indicates that the result code for one or more plugins
* wasn't zero. Invocation failures count against the MaxErrors limit of the parent command. This is a
* terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The managed node might not exist, or it
* might not be responding. Undeliverable invocations don't count against the parent command's MaxErrors
* limit, and they don't contribute to whether the parent command status is Success or Incomplete. This is a
* terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors limit and subsequent command invocations were
* canceled by the system. This is a terminal state.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withStatusDetails(String statusDetails) {
setStatusDetails(statusDetails);
return this;
}
/**
*
* A numeric response code generated after running the plugin.
*
*
* @param responseCode
* A numeric response code generated after running the plugin.
*/
public void setResponseCode(Integer responseCode) {
this.responseCode = responseCode;
}
/**
*
* A numeric response code generated after running the plugin.
*
*
* @return A numeric response code generated after running the plugin.
*/
public Integer getResponseCode() {
return this.responseCode;
}
/**
*
* A numeric response code generated after running the plugin.
*
*
* @param responseCode
* A numeric response code generated after running the plugin.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withResponseCode(Integer responseCode) {
setResponseCode(responseCode);
return this;
}
/**
*
* The time the plugin started running.
*
*
* @param responseStartDateTime
* The time the plugin started running.
*/
public void setResponseStartDateTime(java.util.Date responseStartDateTime) {
this.responseStartDateTime = responseStartDateTime;
}
/**
*
* The time the plugin started running.
*
*
* @return The time the plugin started running.
*/
public java.util.Date getResponseStartDateTime() {
return this.responseStartDateTime;
}
/**
*
* The time the plugin started running.
*
*
* @param responseStartDateTime
* The time the plugin started running.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withResponseStartDateTime(java.util.Date responseStartDateTime) {
setResponseStartDateTime(responseStartDateTime);
return this;
}
/**
*
* The time the plugin stopped running. Could stop prematurely if, for example, a cancel command was sent.
*
*
* @param responseFinishDateTime
* The time the plugin stopped running. Could stop prematurely if, for example, a cancel command was sent.
*/
public void setResponseFinishDateTime(java.util.Date responseFinishDateTime) {
this.responseFinishDateTime = responseFinishDateTime;
}
/**
*
* The time the plugin stopped running. Could stop prematurely if, for example, a cancel command was sent.
*
*
* @return The time the plugin stopped running. Could stop prematurely if, for example, a cancel command was sent.
*/
public java.util.Date getResponseFinishDateTime() {
return this.responseFinishDateTime;
}
/**
*
* The time the plugin stopped running. Could stop prematurely if, for example, a cancel command was sent.
*
*
* @param responseFinishDateTime
* The time the plugin stopped running. Could stop prematurely if, for example, a cancel command was sent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withResponseFinishDateTime(java.util.Date responseFinishDateTime) {
setResponseFinishDateTime(responseFinishDateTime);
return this;
}
/**
*
* Output of the plugin execution.
*
*
* @param output
* Output of the plugin execution.
*/
public void setOutput(String output) {
this.output = output;
}
/**
*
* Output of the plugin execution.
*
*
* @return Output of the plugin execution.
*/
public String getOutput() {
return this.output;
}
/**
*
* Output of the plugin execution.
*
*
* @param output
* Output of the plugin execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withOutput(String output) {
setOutput(output);
return this;
}
/**
*
* The URL for the complete text written by the plugin to stdout in Amazon S3. If the S3 bucket for the command
* wasn't specified, then this string is empty.
*
*
* @param standardOutputUrl
* The URL for the complete text written by the plugin to stdout in Amazon S3. If the S3 bucket for the
* command wasn't specified, then this string is empty.
*/
public void setStandardOutputUrl(String standardOutputUrl) {
this.standardOutputUrl = standardOutputUrl;
}
/**
*
* The URL for the complete text written by the plugin to stdout in Amazon S3. If the S3 bucket for the command
* wasn't specified, then this string is empty.
*
*
* @return The URL for the complete text written by the plugin to stdout in Amazon S3. If the S3 bucket for the
* command wasn't specified, then this string is empty.
*/
public String getStandardOutputUrl() {
return this.standardOutputUrl;
}
/**
*
* The URL for the complete text written by the plugin to stdout in Amazon S3. If the S3 bucket for the command
* wasn't specified, then this string is empty.
*
*
* @param standardOutputUrl
* The URL for the complete text written by the plugin to stdout in Amazon S3. If the S3 bucket for the
* command wasn't specified, then this string is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withStandardOutputUrl(String standardOutputUrl) {
setStandardOutputUrl(standardOutputUrl);
return this;
}
/**
*
* The URL for the complete text written by the plugin to stderr. If execution isn't yet complete, then this string
* is empty.
*
*
* @param standardErrorUrl
* The URL for the complete text written by the plugin to stderr. If execution isn't yet complete, then this
* string is empty.
*/
public void setStandardErrorUrl(String standardErrorUrl) {
this.standardErrorUrl = standardErrorUrl;
}
/**
*
* The URL for the complete text written by the plugin to stderr. If execution isn't yet complete, then this string
* is empty.
*
*
* @return The URL for the complete text written by the plugin to stderr. If execution isn't yet complete, then this
* string is empty.
*/
public String getStandardErrorUrl() {
return this.standardErrorUrl;
}
/**
*
* The URL for the complete text written by the plugin to stderr. If execution isn't yet complete, then this string
* is empty.
*
*
* @param standardErrorUrl
* The URL for the complete text written by the plugin to stderr. If execution isn't yet complete, then this
* string is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withStandardErrorUrl(String standardErrorUrl) {
setStandardErrorUrl(standardErrorUrl);
return this;
}
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Amazon Web Services
* Systems Manager automatically determines the S3 bucket region.
*
*
* @param outputS3Region
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Amazon Web Services
* Systems Manager automatically determines the S3 bucket region.
*/
public void setOutputS3Region(String outputS3Region) {
this.outputS3Region = outputS3Region;
}
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Amazon Web Services
* Systems Manager automatically determines the S3 bucket region.
*
*
* @return (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Amazon Web
* Services Systems Manager automatically determines the S3 bucket region.
*/
public String getOutputS3Region() {
return this.outputS3Region;
}
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Amazon Web Services
* Systems Manager automatically determines the S3 bucket region.
*
*
* @param outputS3Region
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Amazon Web Services
* Systems Manager automatically determines the S3 bucket region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withOutputS3Region(String outputS3Region) {
setOutputS3Region(outputS3Region);
return this;
}
/**
*
* The S3 bucket where the responses to the command executions should be stored. This was requested when issuing the
* command. For example, in the following response:
*
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*
*
* @param outputS3BucketName
* The S3 bucket where the responses to the command executions should be stored. This was requested when
* issuing the command. For example, in the following response:
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*/
public void setOutputS3BucketName(String outputS3BucketName) {
this.outputS3BucketName = outputS3BucketName;
}
/**
*
* The S3 bucket where the responses to the command executions should be stored. This was requested when issuing the
* command. For example, in the following response:
*
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*
*
* @return The S3 bucket where the responses to the command executions should be stored. This was requested when
* issuing the command. For example, in the following response:
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*/
public String getOutputS3BucketName() {
return this.outputS3BucketName;
}
/**
*
* The S3 bucket where the responses to the command executions should be stored. This was requested when issuing the
* command. For example, in the following response:
*
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*
*
* @param outputS3BucketName
* The S3 bucket where the responses to the command executions should be stored. This was requested when
* issuing the command. For example, in the following response:
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withOutputS3BucketName(String outputS3BucketName) {
setOutputS3BucketName(outputS3BucketName);
return this;
}
/**
*
* The S3 directory path inside the bucket where the responses to the command executions should be stored. This was
* requested when issuing the command. For example, in the following response:
*
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*
*
* @param outputS3KeyPrefix
* The S3 directory path inside the bucket where the responses to the command executions should be stored.
* This was requested when issuing the command. For example, in the following response:
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*/
public void setOutputS3KeyPrefix(String outputS3KeyPrefix) {
this.outputS3KeyPrefix = outputS3KeyPrefix;
}
/**
*
* The S3 directory path inside the bucket where the responses to the command executions should be stored. This was
* requested when issuing the command. For example, in the following response:
*
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*
*
* @return The S3 directory path inside the bucket where the responses to the command executions should be stored.
* This was requested when issuing the command. For example, in the following response:
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*/
public String getOutputS3KeyPrefix() {
return this.outputS3KeyPrefix;
}
/**
*
* The S3 directory path inside the bucket where the responses to the command executions should be stored. This was
* requested when issuing the command. For example, in the following response:
*
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
*
*
* @param outputS3KeyPrefix
* The S3 directory path inside the bucket where the responses to the command executions should be stored.
* This was requested when issuing the command. For example, in the following response:
*
* doc-example-bucket/ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix/i-02573cafcfEXAMPLE/awsrunShellScript
*
*
* doc-example-bucket
is the name of the S3 bucket;
*
*
* ab19cb99-a030-46dd-9dfc-8eSAMPLEPre-Fix
is the name of the S3 prefix;
*
*
* i-02573cafcfEXAMPLE
is the managed node ID;
*
*
* awsrunShellScript
is the name of the plugin.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CommandPlugin withOutputS3KeyPrefix(String outputS3KeyPrefix) {
setOutputS3KeyPrefix(outputS3KeyPrefix);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusDetails() != null)
sb.append("StatusDetails: ").append(getStatusDetails()).append(",");
if (getResponseCode() != null)
sb.append("ResponseCode: ").append(getResponseCode()).append(",");
if (getResponseStartDateTime() != null)
sb.append("ResponseStartDateTime: ").append(getResponseStartDateTime()).append(",");
if (getResponseFinishDateTime() != null)
sb.append("ResponseFinishDateTime: ").append(getResponseFinishDateTime()).append(",");
if (getOutput() != null)
sb.append("Output: ").append(getOutput()).append(",");
if (getStandardOutputUrl() != null)
sb.append("StandardOutputUrl: ").append(getStandardOutputUrl()).append(",");
if (getStandardErrorUrl() != null)
sb.append("StandardErrorUrl: ").append(getStandardErrorUrl()).append(",");
if (getOutputS3Region() != null)
sb.append("OutputS3Region: ").append(getOutputS3Region()).append(",");
if (getOutputS3BucketName() != null)
sb.append("OutputS3BucketName: ").append(getOutputS3BucketName()).append(",");
if (getOutputS3KeyPrefix() != null)
sb.append("OutputS3KeyPrefix: ").append(getOutputS3KeyPrefix());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CommandPlugin == false)
return false;
CommandPlugin other = (CommandPlugin) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusDetails() == null ^ this.getStatusDetails() == null)
return false;
if (other.getStatusDetails() != null && other.getStatusDetails().equals(this.getStatusDetails()) == false)
return false;
if (other.getResponseCode() == null ^ this.getResponseCode() == null)
return false;
if (other.getResponseCode() != null && other.getResponseCode().equals(this.getResponseCode()) == false)
return false;
if (other.getResponseStartDateTime() == null ^ this.getResponseStartDateTime() == null)
return false;
if (other.getResponseStartDateTime() != null && other.getResponseStartDateTime().equals(this.getResponseStartDateTime()) == false)
return false;
if (other.getResponseFinishDateTime() == null ^ this.getResponseFinishDateTime() == null)
return false;
if (other.getResponseFinishDateTime() != null && other.getResponseFinishDateTime().equals(this.getResponseFinishDateTime()) == false)
return false;
if (other.getOutput() == null ^ this.getOutput() == null)
return false;
if (other.getOutput() != null && other.getOutput().equals(this.getOutput()) == false)
return false;
if (other.getStandardOutputUrl() == null ^ this.getStandardOutputUrl() == null)
return false;
if (other.getStandardOutputUrl() != null && other.getStandardOutputUrl().equals(this.getStandardOutputUrl()) == false)
return false;
if (other.getStandardErrorUrl() == null ^ this.getStandardErrorUrl() == null)
return false;
if (other.getStandardErrorUrl() != null && other.getStandardErrorUrl().equals(this.getStandardErrorUrl()) == false)
return false;
if (other.getOutputS3Region() == null ^ this.getOutputS3Region() == null)
return false;
if (other.getOutputS3Region() != null && other.getOutputS3Region().equals(this.getOutputS3Region()) == false)
return false;
if (other.getOutputS3BucketName() == null ^ this.getOutputS3BucketName() == null)
return false;
if (other.getOutputS3BucketName() != null && other.getOutputS3BucketName().equals(this.getOutputS3BucketName()) == false)
return false;
if (other.getOutputS3KeyPrefix() == null ^ this.getOutputS3KeyPrefix() == null)
return false;
if (other.getOutputS3KeyPrefix() != null && other.getOutputS3KeyPrefix().equals(this.getOutputS3KeyPrefix()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusDetails() == null) ? 0 : getStatusDetails().hashCode());
hashCode = prime * hashCode + ((getResponseCode() == null) ? 0 : getResponseCode().hashCode());
hashCode = prime * hashCode + ((getResponseStartDateTime() == null) ? 0 : getResponseStartDateTime().hashCode());
hashCode = prime * hashCode + ((getResponseFinishDateTime() == null) ? 0 : getResponseFinishDateTime().hashCode());
hashCode = prime * hashCode + ((getOutput() == null) ? 0 : getOutput().hashCode());
hashCode = prime * hashCode + ((getStandardOutputUrl() == null) ? 0 : getStandardOutputUrl().hashCode());
hashCode = prime * hashCode + ((getStandardErrorUrl() == null) ? 0 : getStandardErrorUrl().hashCode());
hashCode = prime * hashCode + ((getOutputS3Region() == null) ? 0 : getOutputS3Region().hashCode());
hashCode = prime * hashCode + ((getOutputS3BucketName() == null) ? 0 : getOutputS3BucketName().hashCode());
hashCode = prime * hashCode + ((getOutputS3KeyPrefix() == null) ? 0 : getOutputS3KeyPrefix().hashCode());
return hashCode;
}
@Override
public CommandPlugin clone() {
try {
return (CommandPlugin) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.CommandPluginMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}