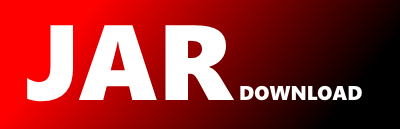
com.amazonaws.services.simplesystemsmanagement.model.CreateAssociationRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAssociationRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the SSM Command document or Automation runbook that contains the configuration information for the
* managed node.
*
*
* You can specify Amazon Web Services-predefined documents, documents you created, or a document that is shared
* with you from another Amazon Web Services account.
*
*
* For Systems Manager documents (SSM documents) that are shared with you from other Amazon Web Services accounts,
* you must specify the complete SSM document ARN, in the following format:
*
*
* arn:partition:ssm:region:account-id:document/document-name
*
*
* For example:
*
*
* arn:aws:ssm:us-east-2:12345678912:document/My-Shared-Document
*
*
* For Amazon Web Services-predefined documents and SSM documents you created in your account, you only need to
* specify the document name. For example, AWS-ApplyPatchBaseline
or My-Document
.
*
*/
private String name;
/**
*
* The document version you want to associate with the targets. Can be a specific version or the default version.
*
*
*
* State Manager doesn't support running associations that use a new version of a document if that document is
* shared from another account. State Manager always runs the default
version of a document if shared
* from another account, even though the Systems Manager console shows that a new version was processed. If you want
* to run an association using a new version of a document shared form another account, you must set the document
* version to default
.
*
*
*/
private String documentVersion;
/**
*
* The managed node ID.
*
*
*
* InstanceId
has been deprecated. To specify a managed node ID for an association, use the
* Targets
parameter. Requests that include the parameter InstanceID
with Systems Manager
* documents (SSM documents) that use schema version 2.0 or later will fail. In addition, if you use the parameter
* InstanceId
, you can't use the parameters AssociationName
, DocumentVersion
,
* MaxErrors
, MaxConcurrency
, OutputLocation
, or
* ScheduleExpression
. To use these parameters, you must use the Targets
parameter.
*
*
*/
private String instanceId;
/**
*
* The parameters for the runtime configuration of the document.
*
*/
private java.util.Map> parameters;
/**
*
* The targets for the association. You can target managed nodes by using tags, Amazon Web Services resource groups,
* all managed nodes in an Amazon Web Services account, or individual managed node IDs. You can target all managed
* nodes in an Amazon Web Services account by specifying the InstanceIds
key with a value of
* *
. For more information about choosing targets for an association, see About targets and rate controls in State Manager associations in the Amazon Web Services Systems Manager
* User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* A cron expression when the association will be applied to the targets.
*
*/
private String scheduleExpression;
/**
*
* An Amazon Simple Storage Service (Amazon S3) bucket where you want to store the output details of the request.
*
*/
private InstanceAssociationOutputLocation outputLocation;
/**
*
* Specify a descriptive name for the association.
*
*/
private String associationName;
/**
*
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*
*/
private String automationTargetParameterName;
/**
*
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops sending requests when the fourth
* error is received. If you specify 0, then the system stops sending requests after the first error is returned. If
* you run an association on 50 managed nodes and set MaxError
to 10%, then the system stops sending
* the request when the sixth error is received.
*
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more than
* max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at a time.
*
*/
private String maxErrors;
/**
*
* The maximum number of targets allowed to run the association at the same time. You can specify a number, for
* example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means all
* targets run the association at the same time.
*
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*
*/
private String maxConcurrency;
/**
*
* The severity level to assign to the association.
*
*/
private String complianceSeverity;
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager. It is
* managed by your direct call to the PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*/
private String syncCompliance;
/**
*
* By default, when you create a new association, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*/
private Boolean applyOnlyAtCronInterval;
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents you want to gate your
* associations under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*/
private com.amazonaws.internal.SdkInternalList calendarNames;
/**
*
* A location is a combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run
* the association. Use this action to create an association in multiple Regions and multiple accounts.
*
*/
private com.amazonaws.internal.SdkInternalList targetLocations;
/**
*
* Number of days to wait after the scheduled day to run an association. For example, if you specified a cron
* schedule of cron(0 0 ? * THU#2 *)
, you could specify an offset of 3 to run the association each
* Sunday after the second Thursday of the month. For more information about cron schedules for associations, see
* Reference: Cron and rate expressions for Systems Manager in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* To use offsets, you must specify the ApplyOnlyAtCronInterval
parameter. This option tells the system
* not to run an association immediately after you create it.
*
*
*/
private Integer scheduleOffset;
/**
*
* The number of hours the association can run before it is canceled. Duration applies to associations that are
* currently running, and any pending and in progress commands on all targets. If a target was taken offline for the
* association to run, it is made available again immediately, without a reboot.
*
*
* The Duration
parameter applies only when both these conditions are true:
*
*
* -
*
* The association for which you specify a duration is cancelable according to the parameters of the SSM command
* document or Automation runbook associated with this execution.
*
*
* -
*
* The command specifies the
* ApplyOnlyAtCronInterval
* parameter, which means that the association doesn't run immediately after it is created, but only according to
* the specified schedule.
*
*
*
*/
private Integer duration;
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*/
private com.amazonaws.internal.SdkInternalList>> targetMaps;
/**
*
* Adds or overwrites one or more tags for a State Manager association. Tags are metadata that you can assign
* to your Amazon Web Services resources. Tags enable you to categorize your resources in different ways, for
* example, by purpose, owner, or environment. Each tag consists of a key and an optional value, both of which you
* define.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
private AlarmConfiguration alarmConfiguration;
/**
*
* The name of the SSM Command document or Automation runbook that contains the configuration information for the
* managed node.
*
*
* You can specify Amazon Web Services-predefined documents, documents you created, or a document that is shared
* with you from another Amazon Web Services account.
*
*
* For Systems Manager documents (SSM documents) that are shared with you from other Amazon Web Services accounts,
* you must specify the complete SSM document ARN, in the following format:
*
*
* arn:partition:ssm:region:account-id:document/document-name
*
*
* For example:
*
*
* arn:aws:ssm:us-east-2:12345678912:document/My-Shared-Document
*
*
* For Amazon Web Services-predefined documents and SSM documents you created in your account, you only need to
* specify the document name. For example, AWS-ApplyPatchBaseline
or My-Document
.
*
*
* @param name
* The name of the SSM Command document or Automation runbook that contains the configuration information for
* the managed node.
*
* You can specify Amazon Web Services-predefined documents, documents you created, or a document that is
* shared with you from another Amazon Web Services account.
*
*
* For Systems Manager documents (SSM documents) that are shared with you from other Amazon Web Services
* accounts, you must specify the complete SSM document ARN, in the following format:
*
*
* arn:partition:ssm:region:account-id:document/document-name
*
*
* For example:
*
*
* arn:aws:ssm:us-east-2:12345678912:document/My-Shared-Document
*
*
* For Amazon Web Services-predefined documents and SSM documents you created in your account, you only need
* to specify the document name. For example, AWS-ApplyPatchBaseline
or My-Document
.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the SSM Command document or Automation runbook that contains the configuration information for the
* managed node.
*
*
* You can specify Amazon Web Services-predefined documents, documents you created, or a document that is shared
* with you from another Amazon Web Services account.
*
*
* For Systems Manager documents (SSM documents) that are shared with you from other Amazon Web Services accounts,
* you must specify the complete SSM document ARN, in the following format:
*
*
* arn:partition:ssm:region:account-id:document/document-name
*
*
* For example:
*
*
* arn:aws:ssm:us-east-2:12345678912:document/My-Shared-Document
*
*
* For Amazon Web Services-predefined documents and SSM documents you created in your account, you only need to
* specify the document name. For example, AWS-ApplyPatchBaseline
or My-Document
.
*
*
* @return The name of the SSM Command document or Automation runbook that contains the configuration information
* for the managed node.
*
* You can specify Amazon Web Services-predefined documents, documents you created, or a document that is
* shared with you from another Amazon Web Services account.
*
*
* For Systems Manager documents (SSM documents) that are shared with you from other Amazon Web Services
* accounts, you must specify the complete SSM document ARN, in the following format:
*
*
* arn:partition:ssm:region:account-id:document/document-name
*
*
* For example:
*
*
* arn:aws:ssm:us-east-2:12345678912:document/My-Shared-Document
*
*
* For Amazon Web Services-predefined documents and SSM documents you created in your account, you only need
* to specify the document name. For example, AWS-ApplyPatchBaseline
or
* My-Document
.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the SSM Command document or Automation runbook that contains the configuration information for the
* managed node.
*
*
* You can specify Amazon Web Services-predefined documents, documents you created, or a document that is shared
* with you from another Amazon Web Services account.
*
*
* For Systems Manager documents (SSM documents) that are shared with you from other Amazon Web Services accounts,
* you must specify the complete SSM document ARN, in the following format:
*
*
* arn:partition:ssm:region:account-id:document/document-name
*
*
* For example:
*
*
* arn:aws:ssm:us-east-2:12345678912:document/My-Shared-Document
*
*
* For Amazon Web Services-predefined documents and SSM documents you created in your account, you only need to
* specify the document name. For example, AWS-ApplyPatchBaseline
or My-Document
.
*
*
* @param name
* The name of the SSM Command document or Automation runbook that contains the configuration information for
* the managed node.
*
* You can specify Amazon Web Services-predefined documents, documents you created, or a document that is
* shared with you from another Amazon Web Services account.
*
*
* For Systems Manager documents (SSM documents) that are shared with you from other Amazon Web Services
* accounts, you must specify the complete SSM document ARN, in the following format:
*
*
* arn:partition:ssm:region:account-id:document/document-name
*
*
* For example:
*
*
* arn:aws:ssm:us-east-2:12345678912:document/My-Shared-Document
*
*
* For Amazon Web Services-predefined documents and SSM documents you created in your account, you only need
* to specify the document name. For example, AWS-ApplyPatchBaseline
or My-Document
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The document version you want to associate with the targets. Can be a specific version or the default version.
*
*
*
* State Manager doesn't support running associations that use a new version of a document if that document is
* shared from another account. State Manager always runs the default
version of a document if shared
* from another account, even though the Systems Manager console shows that a new version was processed. If you want
* to run an association using a new version of a document shared form another account, you must set the document
* version to default
.
*
*
*
* @param documentVersion
* The document version you want to associate with the targets. Can be a specific version or the default
* version.
*
* State Manager doesn't support running associations that use a new version of a document if that document
* is shared from another account. State Manager always runs the default
version of a document
* if shared from another account, even though the Systems Manager console shows that a new version was
* processed. If you want to run an association using a new version of a document shared form another
* account, you must set the document version to default
.
*
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The document version you want to associate with the targets. Can be a specific version or the default version.
*
*
*
* State Manager doesn't support running associations that use a new version of a document if that document is
* shared from another account. State Manager always runs the default
version of a document if shared
* from another account, even though the Systems Manager console shows that a new version was processed. If you want
* to run an association using a new version of a document shared form another account, you must set the document
* version to default
.
*
*
*
* @return The document version you want to associate with the targets. Can be a specific version or the default
* version.
*
* State Manager doesn't support running associations that use a new version of a document if that document
* is shared from another account. State Manager always runs the default
version of a document
* if shared from another account, even though the Systems Manager console shows that a new version was
* processed. If you want to run an association using a new version of a document shared form another
* account, you must set the document version to default
.
*
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The document version you want to associate with the targets. Can be a specific version or the default version.
*
*
*
* State Manager doesn't support running associations that use a new version of a document if that document is
* shared from another account. State Manager always runs the default
version of a document if shared
* from another account, even though the Systems Manager console shows that a new version was processed. If you want
* to run an association using a new version of a document shared form another account, you must set the document
* version to default
.
*
*
*
* @param documentVersion
* The document version you want to associate with the targets. Can be a specific version or the default
* version.
*
* State Manager doesn't support running associations that use a new version of a document if that document
* is shared from another account. State Manager always runs the default
version of a document
* if shared from another account, even though the Systems Manager console shows that a new version was
* processed. If you want to run an association using a new version of a document shared form another
* account, you must set the document version to default
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* The managed node ID.
*
*
*
* InstanceId
has been deprecated. To specify a managed node ID for an association, use the
* Targets
parameter. Requests that include the parameter InstanceID
with Systems Manager
* documents (SSM documents) that use schema version 2.0 or later will fail. In addition, if you use the parameter
* InstanceId
, you can't use the parameters AssociationName
, DocumentVersion
,
* MaxErrors
, MaxConcurrency
, OutputLocation
, or
* ScheduleExpression
. To use these parameters, you must use the Targets
parameter.
*
*
*
* @param instanceId
* The managed node ID.
*
* InstanceId
has been deprecated. To specify a managed node ID for an association, use the
* Targets
parameter. Requests that include the parameter InstanceID
with Systems
* Manager documents (SSM documents) that use schema version 2.0 or later will fail. In addition, if you use
* the parameter InstanceId
, you can't use the parameters AssociationName
,
* DocumentVersion
, MaxErrors
, MaxConcurrency
,
* OutputLocation
, or ScheduleExpression
. To use these parameters, you must use the
* Targets
parameter.
*
*/
public void setInstanceId(String instanceId) {
this.instanceId = instanceId;
}
/**
*
* The managed node ID.
*
*
*
* InstanceId
has been deprecated. To specify a managed node ID for an association, use the
* Targets
parameter. Requests that include the parameter InstanceID
with Systems Manager
* documents (SSM documents) that use schema version 2.0 or later will fail. In addition, if you use the parameter
* InstanceId
, you can't use the parameters AssociationName
, DocumentVersion
,
* MaxErrors
, MaxConcurrency
, OutputLocation
, or
* ScheduleExpression
. To use these parameters, you must use the Targets
parameter.
*
*
*
* @return The managed node ID.
*
* InstanceId
has been deprecated. To specify a managed node ID for an association, use the
* Targets
parameter. Requests that include the parameter InstanceID
with Systems
* Manager documents (SSM documents) that use schema version 2.0 or later will fail. In addition, if you use
* the parameter InstanceId
, you can't use the parameters AssociationName
,
* DocumentVersion
, MaxErrors
, MaxConcurrency
,
* OutputLocation
, or ScheduleExpression
. To use these parameters, you must use
* the Targets
parameter.
*
*/
public String getInstanceId() {
return this.instanceId;
}
/**
*
* The managed node ID.
*
*
*
* InstanceId
has been deprecated. To specify a managed node ID for an association, use the
* Targets
parameter. Requests that include the parameter InstanceID
with Systems Manager
* documents (SSM documents) that use schema version 2.0 or later will fail. In addition, if you use the parameter
* InstanceId
, you can't use the parameters AssociationName
, DocumentVersion
,
* MaxErrors
, MaxConcurrency
, OutputLocation
, or
* ScheduleExpression
. To use these parameters, you must use the Targets
parameter.
*
*
*
* @param instanceId
* The managed node ID.
*
* InstanceId
has been deprecated. To specify a managed node ID for an association, use the
* Targets
parameter. Requests that include the parameter InstanceID
with Systems
* Manager documents (SSM documents) that use schema version 2.0 or later will fail. In addition, if you use
* the parameter InstanceId
, you can't use the parameters AssociationName
,
* DocumentVersion
, MaxErrors
, MaxConcurrency
,
* OutputLocation
, or ScheduleExpression
. To use these parameters, you must use the
* Targets
parameter.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withInstanceId(String instanceId) {
setInstanceId(instanceId);
return this;
}
/**
*
* The parameters for the runtime configuration of the document.
*
*
* @return The parameters for the runtime configuration of the document.
*/
public java.util.Map> getParameters() {
return parameters;
}
/**
*
* The parameters for the runtime configuration of the document.
*
*
* @param parameters
* The parameters for the runtime configuration of the document.
*/
public void setParameters(java.util.Map> parameters) {
this.parameters = parameters;
}
/**
*
* The parameters for the runtime configuration of the document.
*
*
* @param parameters
* The parameters for the runtime configuration of the document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withParameters(java.util.Map> parameters) {
setParameters(parameters);
return this;
}
/**
* Add a single Parameters entry
*
* @see CreateAssociationRequest#withParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest addParametersEntry(String key, java.util.List value) {
if (null == this.parameters) {
this.parameters = new java.util.HashMap>();
}
if (this.parameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.parameters.put(key, value);
return this;
}
/**
* Removes all the entries added into Parameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest clearParametersEntries() {
this.parameters = null;
return this;
}
/**
*
* The targets for the association. You can target managed nodes by using tags, Amazon Web Services resource groups,
* all managed nodes in an Amazon Web Services account, or individual managed node IDs. You can target all managed
* nodes in an Amazon Web Services account by specifying the InstanceIds
key with a value of
* *
. For more information about choosing targets for an association, see About targets and rate controls in State Manager associations in the Amazon Web Services Systems Manager
* User Guide.
*
*
* @return The targets for the association. You can target managed nodes by using tags, Amazon Web Services resource
* groups, all managed nodes in an Amazon Web Services account, or individual managed node IDs. You can
* target all managed nodes in an Amazon Web Services account by specifying the InstanceIds
key
* with a value of *
. For more information about choosing targets for an association, see About targets and rate controls in State Manager associations in the Amazon Web Services Systems
* Manager User Guide.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The targets for the association. You can target managed nodes by using tags, Amazon Web Services resource groups,
* all managed nodes in an Amazon Web Services account, or individual managed node IDs. You can target all managed
* nodes in an Amazon Web Services account by specifying the InstanceIds
key with a value of
* *
. For more information about choosing targets for an association, see About targets and rate controls in State Manager associations in the Amazon Web Services Systems Manager
* User Guide.
*
*
* @param targets
* The targets for the association. You can target managed nodes by using tags, Amazon Web Services resource
* groups, all managed nodes in an Amazon Web Services account, or individual managed node IDs. You can
* target all managed nodes in an Amazon Web Services account by specifying the InstanceIds
key
* with a value of *
. For more information about choosing targets for an association, see About targets and rate controls in State Manager associations in the Amazon Web Services Systems
* Manager User Guide.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The targets for the association. You can target managed nodes by using tags, Amazon Web Services resource groups,
* all managed nodes in an Amazon Web Services account, or individual managed node IDs. You can target all managed
* nodes in an Amazon Web Services account by specifying the InstanceIds
key with a value of
* *
. For more information about choosing targets for an association, see About targets and rate controls in State Manager associations in the Amazon Web Services Systems Manager
* User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The targets for the association. You can target managed nodes by using tags, Amazon Web Services resource
* groups, all managed nodes in an Amazon Web Services account, or individual managed node IDs. You can
* target all managed nodes in an Amazon Web Services account by specifying the InstanceIds
key
* with a value of *
. For more information about choosing targets for an association, see About targets and rate controls in State Manager associations in the Amazon Web Services Systems
* Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The targets for the association. You can target managed nodes by using tags, Amazon Web Services resource groups,
* all managed nodes in an Amazon Web Services account, or individual managed node IDs. You can target all managed
* nodes in an Amazon Web Services account by specifying the InstanceIds
key with a value of
* *
. For more information about choosing targets for an association, see About targets and rate controls in State Manager associations in the Amazon Web Services Systems Manager
* User Guide.
*
*
* @param targets
* The targets for the association. You can target managed nodes by using tags, Amazon Web Services resource
* groups, all managed nodes in an Amazon Web Services account, or individual managed node IDs. You can
* target all managed nodes in an Amazon Web Services account by specifying the InstanceIds
key
* with a value of *
. For more information about choosing targets for an association, see About targets and rate controls in State Manager associations in the Amazon Web Services Systems
* Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* A cron expression when the association will be applied to the targets.
*
*
* @param scheduleExpression
* A cron expression when the association will be applied to the targets.
*/
public void setScheduleExpression(String scheduleExpression) {
this.scheduleExpression = scheduleExpression;
}
/**
*
* A cron expression when the association will be applied to the targets.
*
*
* @return A cron expression when the association will be applied to the targets.
*/
public String getScheduleExpression() {
return this.scheduleExpression;
}
/**
*
* A cron expression when the association will be applied to the targets.
*
*
* @param scheduleExpression
* A cron expression when the association will be applied to the targets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withScheduleExpression(String scheduleExpression) {
setScheduleExpression(scheduleExpression);
return this;
}
/**
*
* An Amazon Simple Storage Service (Amazon S3) bucket where you want to store the output details of the request.
*
*
* @param outputLocation
* An Amazon Simple Storage Service (Amazon S3) bucket where you want to store the output details of the
* request.
*/
public void setOutputLocation(InstanceAssociationOutputLocation outputLocation) {
this.outputLocation = outputLocation;
}
/**
*
* An Amazon Simple Storage Service (Amazon S3) bucket where you want to store the output details of the request.
*
*
* @return An Amazon Simple Storage Service (Amazon S3) bucket where you want to store the output details of the
* request.
*/
public InstanceAssociationOutputLocation getOutputLocation() {
return this.outputLocation;
}
/**
*
* An Amazon Simple Storage Service (Amazon S3) bucket where you want to store the output details of the request.
*
*
* @param outputLocation
* An Amazon Simple Storage Service (Amazon S3) bucket where you want to store the output details of the
* request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withOutputLocation(InstanceAssociationOutputLocation outputLocation) {
setOutputLocation(outputLocation);
return this;
}
/**
*
* Specify a descriptive name for the association.
*
*
* @param associationName
* Specify a descriptive name for the association.
*/
public void setAssociationName(String associationName) {
this.associationName = associationName;
}
/**
*
* Specify a descriptive name for the association.
*
*
* @return Specify a descriptive name for the association.
*/
public String getAssociationName() {
return this.associationName;
}
/**
*
* Specify a descriptive name for the association.
*
*
* @param associationName
* Specify a descriptive name for the association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withAssociationName(String associationName) {
setAssociationName(associationName);
return this;
}
/**
*
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*
*
* @param automationTargetParameterName
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*/
public void setAutomationTargetParameterName(String automationTargetParameterName) {
this.automationTargetParameterName = automationTargetParameterName;
}
/**
*
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*
*
* @return Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*/
public String getAutomationTargetParameterName() {
return this.automationTargetParameterName;
}
/**
*
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
*
*
* @param automationTargetParameterName
* Choose the parameter that will define how your automation will branch out. This target is required for
* associations that use an Automation runbook and target resources by using rate controls. Automation is a
* capability of Amazon Web Services Systems Manager.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withAutomationTargetParameterName(String automationTargetParameterName) {
setAutomationTargetParameterName(automationTargetParameterName);
return this;
}
/**
*
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops sending requests when the fourth
* error is received. If you specify 0, then the system stops sending requests after the first error is returned. If
* you run an association on 50 managed nodes and set MaxError
to 10%, then the system stops sending
* the request when the sixth error is received.
*
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more than
* max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at a time.
*
*
* @param maxErrors
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage
* of the target set, for example 10%. If you specify 3, for example, the system stops sending requests when
* the fourth error is received. If you specify 0, then the system stops sending requests after the first
* error is returned. If you run an association on 50 managed nodes and set MaxError
to 10%,
* then the system stops sending the request when the sixth error is received.
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more
* than max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at
* a time.
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops sending requests when the fourth
* error is received. If you specify 0, then the system stops sending requests after the first error is returned. If
* you run an association on 50 managed nodes and set MaxError
to 10%, then the system stops sending
* the request when the sixth error is received.
*
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more than
* max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at a time.
*
*
* @return The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage
* of the target set, for example 10%. If you specify 3, for example, the system stops sending requests when
* the fourth error is received. If you specify 0, then the system stops sending requests after the first
* error is returned. If you run an association on 50 managed nodes and set MaxError
to 10%,
* then the system stops sending the request when the sixth error is received.
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more
* than max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at
* a time.
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops sending requests when the fourth
* error is received. If you specify 0, then the system stops sending requests after the first error is returned. If
* you run an association on 50 managed nodes and set MaxError
to 10%, then the system stops sending
* the request when the sixth error is received.
*
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more than
* max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at a time.
*
*
* @param maxErrors
* The number of errors that are allowed before the system stops sending requests to run the association on
* additional targets. You can specify either an absolute number of errors, for example 10, or a percentage
* of the target set, for example 10%. If you specify 3, for example, the system stops sending requests when
* the fourth error is received. If you specify 0, then the system stops sending requests after the first
* error is returned. If you run an association on 50 managed nodes and set MaxError
to 10%,
* then the system stops sending the request when the sixth error is received.
*
* Executions that are already running an association when MaxErrors
is reached are allowed to
* complete, but some of these executions may fail as well. If you need to ensure that there won't be more
* than max-errors failed executions, set MaxConcurrency
to 1 so that executions proceed one at
* a time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The maximum number of targets allowed to run the association at the same time. You can specify a number, for
* example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means all
* targets run the association at the same time.
*
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*
*
* @param maxConcurrency
* The maximum number of targets allowed to run the association at the same time. You can specify a number,
* for example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means
* all targets run the association at the same time.
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The maximum number of targets allowed to run the association at the same time. You can specify a number, for
* example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means all
* targets run the association at the same time.
*
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*
*
* @return The maximum number of targets allowed to run the association at the same time. You can specify a number,
* for example 10, or a percentage of the target set, for example 10%. The default value is 100%, which
* means all targets run the association at the same time.
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The maximum number of targets allowed to run the association at the same time. You can specify a number, for
* example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means all
* targets run the association at the same time.
*
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
*
*
* @param maxConcurrency
* The maximum number of targets allowed to run the association at the same time. You can specify a number,
* for example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means
* all targets run the association at the same time.
*
* If a new managed node starts and attempts to run an association while Systems Manager is running
* MaxConcurrency
associations, the association is allowed to run. During the next association
* interval, the new managed node will process its association within the limit specified for
* MaxConcurrency
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The severity level to assign to the association.
*
*
* @param complianceSeverity
* The severity level to assign to the association.
* @see AssociationComplianceSeverity
*/
public void setComplianceSeverity(String complianceSeverity) {
this.complianceSeverity = complianceSeverity;
}
/**
*
* The severity level to assign to the association.
*
*
* @return The severity level to assign to the association.
* @see AssociationComplianceSeverity
*/
public String getComplianceSeverity() {
return this.complianceSeverity;
}
/**
*
* The severity level to assign to the association.
*
*
* @param complianceSeverity
* The severity level to assign to the association.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationComplianceSeverity
*/
public CreateAssociationRequest withComplianceSeverity(String complianceSeverity) {
setComplianceSeverity(complianceSeverity);
return this;
}
/**
*
* The severity level to assign to the association.
*
*
* @param complianceSeverity
* The severity level to assign to the association.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationComplianceSeverity
*/
public CreateAssociationRequest withComplianceSeverity(AssociationComplianceSeverity complianceSeverity) {
this.complianceSeverity = complianceSeverity.toString();
return this;
}
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager. It is
* managed by your direct call to the PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*
* @param syncCompliance
* The mode for generating association compliance. You can specify AUTO
or MANUAL
.
* In AUTO
mode, the system uses the status of the association execution to determine the
* compliance status. If the association execution runs successfully, then the association is
* COMPLIANT
. If the association execution doesn't run successfully, the association is
* NON-COMPLIANT
.
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager. It
* is managed by your direct call to the PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
* @see AssociationSyncCompliance
*/
public void setSyncCompliance(String syncCompliance) {
this.syncCompliance = syncCompliance;
}
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager. It is
* managed by your direct call to the PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*
* @return The mode for generating association compliance. You can specify AUTO
or MANUAL
.
* In AUTO
mode, the system uses the status of the association execution to determine the
* compliance status. If the association execution runs successfully, then the association is
* COMPLIANT
. If the association execution doesn't run successfully, the association is
* NON-COMPLIANT
.
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager. It
* is managed by your direct call to the PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
* @see AssociationSyncCompliance
*/
public String getSyncCompliance() {
return this.syncCompliance;
}
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager. It is
* managed by your direct call to the PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*
* @param syncCompliance
* The mode for generating association compliance. You can specify AUTO
or MANUAL
.
* In AUTO
mode, the system uses the status of the association execution to determine the
* compliance status. If the association execution runs successfully, then the association is
* COMPLIANT
. If the association execution doesn't run successfully, the association is
* NON-COMPLIANT
.
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager. It
* is managed by your direct call to the PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationSyncCompliance
*/
public CreateAssociationRequest withSyncCompliance(String syncCompliance) {
setSyncCompliance(syncCompliance);
return this;
}
/**
*
* The mode for generating association compliance. You can specify AUTO
or MANUAL
. In
* AUTO
mode, the system uses the status of the association execution to determine the compliance
* status. If the association execution runs successfully, then the association is COMPLIANT
. If the
* association execution doesn't run successfully, the association is NON-COMPLIANT
.
*
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager. It is
* managed by your direct call to the PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
*
*
* @param syncCompliance
* The mode for generating association compliance. You can specify AUTO
or MANUAL
.
* In AUTO
mode, the system uses the status of the association execution to determine the
* compliance status. If the association execution runs successfully, then the association is
* COMPLIANT
. If the association execution doesn't run successfully, the association is
* NON-COMPLIANT
.
*
* In MANUAL
mode, you must specify the AssociationId
as a parameter for the
* PutComplianceItems API operation. In this case, compliance data isn't managed by State Manager. It
* is managed by your direct call to the PutComplianceItems API operation.
*
*
* By default, all associations use AUTO
mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationSyncCompliance
*/
public CreateAssociationRequest withSyncCompliance(AssociationSyncCompliance syncCompliance) {
this.syncCompliance = syncCompliance.toString();
return this;
}
/**
*
* By default, when you create a new association, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*
* @param applyOnlyAtCronInterval
* By default, when you create a new association, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run
* immediately after you create it. This parameter isn't supported for rate expressions.
*/
public void setApplyOnlyAtCronInterval(Boolean applyOnlyAtCronInterval) {
this.applyOnlyAtCronInterval = applyOnlyAtCronInterval;
}
/**
*
* By default, when you create a new association, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*
* @return By default, when you create a new association, the system runs it immediately after it is created and
* then according to the schedule you specified. Specify this option if you don't want an association to run
* immediately after you create it. This parameter isn't supported for rate expressions.
*/
public Boolean getApplyOnlyAtCronInterval() {
return this.applyOnlyAtCronInterval;
}
/**
*
* By default, when you create a new association, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*
* @param applyOnlyAtCronInterval
* By default, when you create a new association, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run
* immediately after you create it. This parameter isn't supported for rate expressions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withApplyOnlyAtCronInterval(Boolean applyOnlyAtCronInterval) {
setApplyOnlyAtCronInterval(applyOnlyAtCronInterval);
return this;
}
/**
*
* By default, when you create a new association, the system runs it immediately after it is created and then
* according to the schedule you specified. Specify this option if you don't want an association to run immediately
* after you create it. This parameter isn't supported for rate expressions.
*
*
* @return By default, when you create a new association, the system runs it immediately after it is created and
* then according to the schedule you specified. Specify this option if you don't want an association to run
* immediately after you create it. This parameter isn't supported for rate expressions.
*/
public Boolean isApplyOnlyAtCronInterval() {
return this.applyOnlyAtCronInterval;
}
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents you want to gate your
* associations under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*
* @return The names or Amazon Resource Names (ARNs) of the Change Calendar type documents you want to gate your
* associations under. The associations only run when that change calendar is open. For more information,
* see Amazon Web Services Systems Manager Change Calendar.
*/
public java.util.List getCalendarNames() {
if (calendarNames == null) {
calendarNames = new com.amazonaws.internal.SdkInternalList();
}
return calendarNames;
}
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents you want to gate your
* associations under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*
* @param calendarNames
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents you want to gate your
* associations under. The associations only run when that change calendar is open. For more information, see
*
* Amazon Web Services Systems Manager Change Calendar.
*/
public void setCalendarNames(java.util.Collection calendarNames) {
if (calendarNames == null) {
this.calendarNames = null;
return;
}
this.calendarNames = new com.amazonaws.internal.SdkInternalList(calendarNames);
}
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents you want to gate your
* associations under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCalendarNames(java.util.Collection)} or {@link #withCalendarNames(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param calendarNames
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents you want to gate your
* associations under. The associations only run when that change calendar is open. For more information, see
*
* Amazon Web Services Systems Manager Change Calendar.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withCalendarNames(String... calendarNames) {
if (this.calendarNames == null) {
setCalendarNames(new com.amazonaws.internal.SdkInternalList(calendarNames.length));
}
for (String ele : calendarNames) {
this.calendarNames.add(ele);
}
return this;
}
/**
*
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents you want to gate your
* associations under. The associations only run when that change calendar is open. For more information, see Amazon Web
* Services Systems Manager Change Calendar.
*
*
* @param calendarNames
* The names or Amazon Resource Names (ARNs) of the Change Calendar type documents you want to gate your
* associations under. The associations only run when that change calendar is open. For more information, see
*
* Amazon Web Services Systems Manager Change Calendar.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withCalendarNames(java.util.Collection calendarNames) {
setCalendarNames(calendarNames);
return this;
}
/**
*
* A location is a combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run
* the association. Use this action to create an association in multiple Regions and multiple accounts.
*
*
* @return A location is a combination of Amazon Web Services Regions and Amazon Web Services accounts where you
* want to run the association. Use this action to create an association in multiple Regions and multiple
* accounts.
*/
public java.util.List getTargetLocations() {
if (targetLocations == null) {
targetLocations = new com.amazonaws.internal.SdkInternalList();
}
return targetLocations;
}
/**
*
* A location is a combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run
* the association. Use this action to create an association in multiple Regions and multiple accounts.
*
*
* @param targetLocations
* A location is a combination of Amazon Web Services Regions and Amazon Web Services accounts where you want
* to run the association. Use this action to create an association in multiple Regions and multiple
* accounts.
*/
public void setTargetLocations(java.util.Collection targetLocations) {
if (targetLocations == null) {
this.targetLocations = null;
return;
}
this.targetLocations = new com.amazonaws.internal.SdkInternalList(targetLocations);
}
/**
*
* A location is a combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run
* the association. Use this action to create an association in multiple Regions and multiple accounts.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetLocations(java.util.Collection)} or {@link #withTargetLocations(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param targetLocations
* A location is a combination of Amazon Web Services Regions and Amazon Web Services accounts where you want
* to run the association. Use this action to create an association in multiple Regions and multiple
* accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withTargetLocations(TargetLocation... targetLocations) {
if (this.targetLocations == null) {
setTargetLocations(new com.amazonaws.internal.SdkInternalList(targetLocations.length));
}
for (TargetLocation ele : targetLocations) {
this.targetLocations.add(ele);
}
return this;
}
/**
*
* A location is a combination of Amazon Web Services Regions and Amazon Web Services accounts where you want to run
* the association. Use this action to create an association in multiple Regions and multiple accounts.
*
*
* @param targetLocations
* A location is a combination of Amazon Web Services Regions and Amazon Web Services accounts where you want
* to run the association. Use this action to create an association in multiple Regions and multiple
* accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withTargetLocations(java.util.Collection targetLocations) {
setTargetLocations(targetLocations);
return this;
}
/**
*
* Number of days to wait after the scheduled day to run an association. For example, if you specified a cron
* schedule of cron(0 0 ? * THU#2 *)
, you could specify an offset of 3 to run the association each
* Sunday after the second Thursday of the month. For more information about cron schedules for associations, see
* Reference: Cron and rate expressions for Systems Manager in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* To use offsets, you must specify the ApplyOnlyAtCronInterval
parameter. This option tells the system
* not to run an association immediately after you create it.
*
*
*
* @param scheduleOffset
* Number of days to wait after the scheduled day to run an association. For example, if you specified a cron
* schedule of cron(0 0 ? * THU#2 *)
, you could specify an offset of 3 to run the association
* each Sunday after the second Thursday of the month. For more information about cron schedules for
* associations, see Reference: Cron and rate expressions for Systems Manager in the Amazon Web Services Systems
* Manager User Guide.
*
* To use offsets, you must specify the ApplyOnlyAtCronInterval
parameter. This option tells the
* system not to run an association immediately after you create it.
*
*/
public void setScheduleOffset(Integer scheduleOffset) {
this.scheduleOffset = scheduleOffset;
}
/**
*
* Number of days to wait after the scheduled day to run an association. For example, if you specified a cron
* schedule of cron(0 0 ? * THU#2 *)
, you could specify an offset of 3 to run the association each
* Sunday after the second Thursday of the month. For more information about cron schedules for associations, see
* Reference: Cron and rate expressions for Systems Manager in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* To use offsets, you must specify the ApplyOnlyAtCronInterval
parameter. This option tells the system
* not to run an association immediately after you create it.
*
*
*
* @return Number of days to wait after the scheduled day to run an association. For example, if you specified a
* cron schedule of cron(0 0 ? * THU#2 *)
, you could specify an offset of 3 to run the
* association each Sunday after the second Thursday of the month. For more information about cron schedules
* for associations, see Reference: Cron and rate expressions for Systems Manager in the Amazon Web Services Systems
* Manager User Guide.
*
* To use offsets, you must specify the ApplyOnlyAtCronInterval
parameter. This option tells
* the system not to run an association immediately after you create it.
*
*/
public Integer getScheduleOffset() {
return this.scheduleOffset;
}
/**
*
* Number of days to wait after the scheduled day to run an association. For example, if you specified a cron
* schedule of cron(0 0 ? * THU#2 *)
, you could specify an offset of 3 to run the association each
* Sunday after the second Thursday of the month. For more information about cron schedules for associations, see
* Reference: Cron and rate expressions for Systems Manager in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* To use offsets, you must specify the ApplyOnlyAtCronInterval
parameter. This option tells the system
* not to run an association immediately after you create it.
*
*
*
* @param scheduleOffset
* Number of days to wait after the scheduled day to run an association. For example, if you specified a cron
* schedule of cron(0 0 ? * THU#2 *)
, you could specify an offset of 3 to run the association
* each Sunday after the second Thursday of the month. For more information about cron schedules for
* associations, see Reference: Cron and rate expressions for Systems Manager in the Amazon Web Services Systems
* Manager User Guide.
*
* To use offsets, you must specify the ApplyOnlyAtCronInterval
parameter. This option tells the
* system not to run an association immediately after you create it.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withScheduleOffset(Integer scheduleOffset) {
setScheduleOffset(scheduleOffset);
return this;
}
/**
*
* The number of hours the association can run before it is canceled. Duration applies to associations that are
* currently running, and any pending and in progress commands on all targets. If a target was taken offline for the
* association to run, it is made available again immediately, without a reboot.
*
*
* The Duration
parameter applies only when both these conditions are true:
*
*
* -
*
* The association for which you specify a duration is cancelable according to the parameters of the SSM command
* document or Automation runbook associated with this execution.
*
*
* -
*
* The command specifies the
* ApplyOnlyAtCronInterval
* parameter, which means that the association doesn't run immediately after it is created, but only according to
* the specified schedule.
*
*
*
*
* @param duration
* The number of hours the association can run before it is canceled. Duration applies to associations that
* are currently running, and any pending and in progress commands on all targets. If a target was taken
* offline for the association to run, it is made available again immediately, without a reboot.
*
* The Duration
parameter applies only when both these conditions are true:
*
*
* -
*
* The association for which you specify a duration is cancelable according to the parameters of the SSM
* command document or Automation runbook associated with this execution.
*
*
* -
*
* The command specifies the
* ApplyOnlyAtCronInterval
* parameter, which means that the association doesn't run immediately after it is created, but only
* according to the specified schedule.
*
*
*/
public void setDuration(Integer duration) {
this.duration = duration;
}
/**
*
* The number of hours the association can run before it is canceled. Duration applies to associations that are
* currently running, and any pending and in progress commands on all targets. If a target was taken offline for the
* association to run, it is made available again immediately, without a reboot.
*
*
* The Duration
parameter applies only when both these conditions are true:
*
*
* -
*
* The association for which you specify a duration is cancelable according to the parameters of the SSM command
* document or Automation runbook associated with this execution.
*
*
* -
*
* The command specifies the
* ApplyOnlyAtCronInterval
* parameter, which means that the association doesn't run immediately after it is created, but only according to
* the specified schedule.
*
*
*
*
* @return The number of hours the association can run before it is canceled. Duration applies to associations that
* are currently running, and any pending and in progress commands on all targets. If a target was taken
* offline for the association to run, it is made available again immediately, without a reboot.
*
* The Duration
parameter applies only when both these conditions are true:
*
*
* -
*
* The association for which you specify a duration is cancelable according to the parameters of the SSM
* command document or Automation runbook associated with this execution.
*
*
* -
*
* The command specifies the
* ApplyOnlyAtCronInterval
* parameter, which means that the association doesn't run immediately after it is created, but only
* according to the specified schedule.
*
*
*/
public Integer getDuration() {
return this.duration;
}
/**
*
* The number of hours the association can run before it is canceled. Duration applies to associations that are
* currently running, and any pending and in progress commands on all targets. If a target was taken offline for the
* association to run, it is made available again immediately, without a reboot.
*
*
* The Duration
parameter applies only when both these conditions are true:
*
*
* -
*
* The association for which you specify a duration is cancelable according to the parameters of the SSM command
* document or Automation runbook associated with this execution.
*
*
* -
*
* The command specifies the
* ApplyOnlyAtCronInterval
* parameter, which means that the association doesn't run immediately after it is created, but only according to
* the specified schedule.
*
*
*
*
* @param duration
* The number of hours the association can run before it is canceled. Duration applies to associations that
* are currently running, and any pending and in progress commands on all targets. If a target was taken
* offline for the association to run, it is made available again immediately, without a reboot.
*
* The Duration
parameter applies only when both these conditions are true:
*
*
* -
*
* The association for which you specify a duration is cancelable according to the parameters of the SSM
* command document or Automation runbook associated with this execution.
*
*
* -
*
* The command specifies the
* ApplyOnlyAtCronInterval
* parameter, which means that the association doesn't run immediately after it is created, but only
* according to the specified schedule.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withDuration(Integer duration) {
setDuration(duration);
return this;
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* @return A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
*/
public java.util.List>> getTargetMaps() {
if (targetMaps == null) {
targetMaps = new com.amazonaws.internal.SdkInternalList>>();
}
return targetMaps;
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* @param targetMaps
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
*/
public void setTargetMaps(java.util.Collection>> targetMaps) {
if (targetMaps == null) {
this.targetMaps = null;
return;
}
this.targetMaps = new com.amazonaws.internal.SdkInternalList>>(targetMaps);
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetMaps(java.util.Collection)} or {@link #withTargetMaps(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param targetMaps
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withTargetMaps(java.util.Map>... targetMaps) {
if (this.targetMaps == null) {
setTargetMaps(new com.amazonaws.internal.SdkInternalList>>(targetMaps.length));
}
for (java.util.Map> ele : targetMaps) {
this.targetMaps.add(ele);
}
return this;
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* @param targetMaps
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withTargetMaps(java.util.Collection>> targetMaps) {
setTargetMaps(targetMaps);
return this;
}
/**
*
* Adds or overwrites one or more tags for a State Manager association. Tags are metadata that you can assign
* to your Amazon Web Services resources. Tags enable you to categorize your resources in different ways, for
* example, by purpose, owner, or environment. Each tag consists of a key and an optional value, both of which you
* define.
*
*
* @return Adds or overwrites one or more tags for a State Manager association. Tags are metadata that you
* can assign to your Amazon Web Services resources. Tags enable you to categorize your resources in
* different ways, for example, by purpose, owner, or environment. Each tag consists of a key and an
* optional value, both of which you define.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* Adds or overwrites one or more tags for a State Manager association. Tags are metadata that you can assign
* to your Amazon Web Services resources. Tags enable you to categorize your resources in different ways, for
* example, by purpose, owner, or environment. Each tag consists of a key and an optional value, both of which you
* define.
*
*
* @param tags
* Adds or overwrites one or more tags for a State Manager association. Tags are metadata that you can
* assign to your Amazon Web Services resources. Tags enable you to categorize your resources in different
* ways, for example, by purpose, owner, or environment. Each tag consists of a key and an optional value,
* both of which you define.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* Adds or overwrites one or more tags for a State Manager association. Tags are metadata that you can assign
* to your Amazon Web Services resources. Tags enable you to categorize your resources in different ways, for
* example, by purpose, owner, or environment. Each tag consists of a key and an optional value, both of which you
* define.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Adds or overwrites one or more tags for a State Manager association. Tags are metadata that you can
* assign to your Amazon Web Services resources. Tags enable you to categorize your resources in different
* ways, for example, by purpose, owner, or environment. Each tag consists of a key and an optional value,
* both of which you define.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Adds or overwrites one or more tags for a State Manager association. Tags are metadata that you can assign
* to your Amazon Web Services resources. Tags enable you to categorize your resources in different ways, for
* example, by purpose, owner, or environment. Each tag consists of a key and an optional value, both of which you
* define.
*
*
* @param tags
* Adds or overwrites one or more tags for a State Manager association. Tags are metadata that you can
* assign to your Amazon Web Services resources. Tags enable you to categorize your resources in different
* ways, for example, by purpose, owner, or environment. Each tag consists of a key and an optional value,
* both of which you define.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* @param alarmConfiguration
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
* @return
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
* @param alarmConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssociationRequest withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getInstanceId() != null)
sb.append("InstanceId: ").append(getInstanceId()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append("***Sensitive Data Redacted***").append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getScheduleExpression() != null)
sb.append("ScheduleExpression: ").append(getScheduleExpression()).append(",");
if (getOutputLocation() != null)
sb.append("OutputLocation: ").append(getOutputLocation()).append(",");
if (getAssociationName() != null)
sb.append("AssociationName: ").append(getAssociationName()).append(",");
if (getAutomationTargetParameterName() != null)
sb.append("AutomationTargetParameterName: ").append(getAutomationTargetParameterName()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getComplianceSeverity() != null)
sb.append("ComplianceSeverity: ").append(getComplianceSeverity()).append(",");
if (getSyncCompliance() != null)
sb.append("SyncCompliance: ").append(getSyncCompliance()).append(",");
if (getApplyOnlyAtCronInterval() != null)
sb.append("ApplyOnlyAtCronInterval: ").append(getApplyOnlyAtCronInterval()).append(",");
if (getCalendarNames() != null)
sb.append("CalendarNames: ").append(getCalendarNames()).append(",");
if (getTargetLocations() != null)
sb.append("TargetLocations: ").append(getTargetLocations()).append(",");
if (getScheduleOffset() != null)
sb.append("ScheduleOffset: ").append(getScheduleOffset()).append(",");
if (getDuration() != null)
sb.append("Duration: ").append(getDuration()).append(",");
if (getTargetMaps() != null)
sb.append("TargetMaps: ").append(getTargetMaps()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAssociationRequest == false)
return false;
CreateAssociationRequest other = (CreateAssociationRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getInstanceId() == null ^ this.getInstanceId() == null)
return false;
if (other.getInstanceId() != null && other.getInstanceId().equals(this.getInstanceId()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getScheduleExpression() == null ^ this.getScheduleExpression() == null)
return false;
if (other.getScheduleExpression() != null && other.getScheduleExpression().equals(this.getScheduleExpression()) == false)
return false;
if (other.getOutputLocation() == null ^ this.getOutputLocation() == null)
return false;
if (other.getOutputLocation() != null && other.getOutputLocation().equals(this.getOutputLocation()) == false)
return false;
if (other.getAssociationName() == null ^ this.getAssociationName() == null)
return false;
if (other.getAssociationName() != null && other.getAssociationName().equals(this.getAssociationName()) == false)
return false;
if (other.getAutomationTargetParameterName() == null ^ this.getAutomationTargetParameterName() == null)
return false;
if (other.getAutomationTargetParameterName() != null
&& other.getAutomationTargetParameterName().equals(this.getAutomationTargetParameterName()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getComplianceSeverity() == null ^ this.getComplianceSeverity() == null)
return false;
if (other.getComplianceSeverity() != null && other.getComplianceSeverity().equals(this.getComplianceSeverity()) == false)
return false;
if (other.getSyncCompliance() == null ^ this.getSyncCompliance() == null)
return false;
if (other.getSyncCompliance() != null && other.getSyncCompliance().equals(this.getSyncCompliance()) == false)
return false;
if (other.getApplyOnlyAtCronInterval() == null ^ this.getApplyOnlyAtCronInterval() == null)
return false;
if (other.getApplyOnlyAtCronInterval() != null && other.getApplyOnlyAtCronInterval().equals(this.getApplyOnlyAtCronInterval()) == false)
return false;
if (other.getCalendarNames() == null ^ this.getCalendarNames() == null)
return false;
if (other.getCalendarNames() != null && other.getCalendarNames().equals(this.getCalendarNames()) == false)
return false;
if (other.getTargetLocations() == null ^ this.getTargetLocations() == null)
return false;
if (other.getTargetLocations() != null && other.getTargetLocations().equals(this.getTargetLocations()) == false)
return false;
if (other.getScheduleOffset() == null ^ this.getScheduleOffset() == null)
return false;
if (other.getScheduleOffset() != null && other.getScheduleOffset().equals(this.getScheduleOffset()) == false)
return false;
if (other.getDuration() == null ^ this.getDuration() == null)
return false;
if (other.getDuration() != null && other.getDuration().equals(this.getDuration()) == false)
return false;
if (other.getTargetMaps() == null ^ this.getTargetMaps() == null)
return false;
if (other.getTargetMaps() != null && other.getTargetMaps().equals(this.getTargetMaps()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getInstanceId() == null) ? 0 : getInstanceId().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getScheduleExpression() == null) ? 0 : getScheduleExpression().hashCode());
hashCode = prime * hashCode + ((getOutputLocation() == null) ? 0 : getOutputLocation().hashCode());
hashCode = prime * hashCode + ((getAssociationName() == null) ? 0 : getAssociationName().hashCode());
hashCode = prime * hashCode + ((getAutomationTargetParameterName() == null) ? 0 : getAutomationTargetParameterName().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getComplianceSeverity() == null) ? 0 : getComplianceSeverity().hashCode());
hashCode = prime * hashCode + ((getSyncCompliance() == null) ? 0 : getSyncCompliance().hashCode());
hashCode = prime * hashCode + ((getApplyOnlyAtCronInterval() == null) ? 0 : getApplyOnlyAtCronInterval().hashCode());
hashCode = prime * hashCode + ((getCalendarNames() == null) ? 0 : getCalendarNames().hashCode());
hashCode = prime * hashCode + ((getTargetLocations() == null) ? 0 : getTargetLocations().hashCode());
hashCode = prime * hashCode + ((getScheduleOffset() == null) ? 0 : getScheduleOffset().hashCode());
hashCode = prime * hashCode + ((getDuration() == null) ? 0 : getDuration().hashCode());
hashCode = prime * hashCode + ((getTargetMaps() == null) ? 0 : getTargetMaps().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
return hashCode;
}
@Override
public CreateAssociationRequest clone() {
return (CreateAssociationRequest) super.clone();
}
}