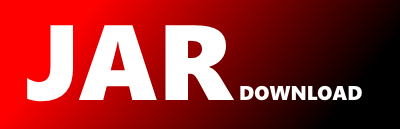
com.amazonaws.services.simplesystemsmanagement.model.CreateDocumentRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateDocumentRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The content for the new SSM document in JSON or YAML format. The content of the document must not exceed 64KB.
* This quota also includes the content specified for input parameters at runtime. We recommend storing the contents
* for your new document in an external JSON or YAML file and referencing the file in a command.
*
*
* For examples, see the following topics in the Amazon Web Services Systems Manager User Guide.
*
*
* -
*
*
* -
*
*
* -
*
*
*
*/
private String content;
/**
*
* A list of SSM documents required by a document. This parameter is used exclusively by AppConfig. When a user
* creates an AppConfig configuration in an SSM document, the user must also specify a required document for
* validation purposes. In this case, an ApplicationConfiguration
document requires an
* ApplicationConfigurationSchema
document for validation purposes. For more information, see What is AppConfig? in
* the AppConfig User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList requires;
/**
*
* A list of key-value pairs that describe attachments to a version of a document.
*
*/
private com.amazonaws.internal.SdkInternalList attachments;
/**
*
* A name for the SSM document.
*
*
*
* You can't use the following strings as document name prefixes. These are reserved by Amazon Web Services for use
* as document name prefixes:
*
*
* -
*
* aws
*
*
* -
*
* amazon
*
*
* -
*
* amzn
*
*
* -
*
* AWSEC2
*
*
* -
*
* AWSConfigRemediation
*
*
* -
*
* AWSSupport
*
*
*
*
*/
private String name;
/**
*
* An optional field where you can specify a friendly name for the SSM document. This value can differ for each
* version of the document. You can update this value at a later time using the UpdateDocument operation.
*
*/
private String displayName;
/**
*
* An optional field specifying the version of the artifact you are creating with the document. For example,
* Release12.1
. This value is unique across all versions of a document, and can't be changed.
*
*/
private String versionName;
/**
*
* The type of document to create.
*
*
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for AppConfig.
*
*
*/
private String documentType;
/**
*
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the default
* format.
*
*/
private String documentFormat;
/**
*
* Specify a target type to define the kinds of resources the document can run on. For example, to run a document on
* EC2 instances, specify the following value: /AWS::EC2::Instance
. If you specify a value of '/' the
* document can run on all types of resources. If you don't specify a value, the document can't run on any
* resources. For a list of valid resource types, see Amazon
* Web Services resource and property types reference in the CloudFormation User Guide.
*
*/
private String targetType;
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag an SSM document to identify the types of
* targets or the environment where it will run. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing SSM document, use the AddTagsToResource operation.
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The content for the new SSM document in JSON or YAML format. The content of the document must not exceed 64KB.
* This quota also includes the content specified for input parameters at runtime. We recommend storing the contents
* for your new document in an external JSON or YAML file and referencing the file in a command.
*
*
* For examples, see the following topics in the Amazon Web Services Systems Manager User Guide.
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param content
* The content for the new SSM document in JSON or YAML format. The content of the document must not exceed
* 64KB. This quota also includes the content specified for input parameters at runtime. We recommend storing
* the contents for your new document in an external JSON or YAML file and referencing the file in a
* command.
*
* For examples, see the following topics in the Amazon Web Services Systems Manager User Guide.
*
*
* -
*
*
* -
*
*
* -
*
*
*/
public void setContent(String content) {
this.content = content;
}
/**
*
* The content for the new SSM document in JSON or YAML format. The content of the document must not exceed 64KB.
* This quota also includes the content specified for input parameters at runtime. We recommend storing the contents
* for your new document in an external JSON or YAML file and referencing the file in a command.
*
*
* For examples, see the following topics in the Amazon Web Services Systems Manager User Guide.
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @return The content for the new SSM document in JSON or YAML format. The content of the document must not exceed
* 64KB. This quota also includes the content specified for input parameters at runtime. We recommend
* storing the contents for your new document in an external JSON or YAML file and referencing the file in a
* command.
*
* For examples, see the following topics in the Amazon Web Services Systems Manager User Guide.
*
*
* -
*
*
* -
*
*
* -
*
*
*/
public String getContent() {
return this.content;
}
/**
*
* The content for the new SSM document in JSON or YAML format. The content of the document must not exceed 64KB.
* This quota also includes the content specified for input parameters at runtime. We recommend storing the contents
* for your new document in an external JSON or YAML file and referencing the file in a command.
*
*
* For examples, see the following topics in the Amazon Web Services Systems Manager User Guide.
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param content
* The content for the new SSM document in JSON or YAML format. The content of the document must not exceed
* 64KB. This quota also includes the content specified for input parameters at runtime. We recommend storing
* the contents for your new document in an external JSON or YAML file and referencing the file in a
* command.
*
* For examples, see the following topics in the Amazon Web Services Systems Manager User Guide.
*
*
* -
*
*
* -
*
*
* -
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withContent(String content) {
setContent(content);
return this;
}
/**
*
* A list of SSM documents required by a document. This parameter is used exclusively by AppConfig. When a user
* creates an AppConfig configuration in an SSM document, the user must also specify a required document for
* validation purposes. In this case, an ApplicationConfiguration
document requires an
* ApplicationConfigurationSchema
document for validation purposes. For more information, see What is AppConfig? in
* the AppConfig User Guide.
*
*
* @return A list of SSM documents required by a document. This parameter is used exclusively by AppConfig. When a
* user creates an AppConfig configuration in an SSM document, the user must also specify a required
* document for validation purposes. In this case, an ApplicationConfiguration
document
* requires an ApplicationConfigurationSchema
document for validation purposes. For more
* information, see What is
* AppConfig? in the AppConfig User Guide.
*/
public java.util.List getRequires() {
if (requires == null) {
requires = new com.amazonaws.internal.SdkInternalList();
}
return requires;
}
/**
*
* A list of SSM documents required by a document. This parameter is used exclusively by AppConfig. When a user
* creates an AppConfig configuration in an SSM document, the user must also specify a required document for
* validation purposes. In this case, an ApplicationConfiguration
document requires an
* ApplicationConfigurationSchema
document for validation purposes. For more information, see What is AppConfig? in
* the AppConfig User Guide.
*
*
* @param requires
* A list of SSM documents required by a document. This parameter is used exclusively by AppConfig. When a
* user creates an AppConfig configuration in an SSM document, the user must also specify a required document
* for validation purposes. In this case, an ApplicationConfiguration
document requires an
* ApplicationConfigurationSchema
document for validation purposes. For more information, see What is
* AppConfig? in the AppConfig User Guide.
*/
public void setRequires(java.util.Collection requires) {
if (requires == null) {
this.requires = null;
return;
}
this.requires = new com.amazonaws.internal.SdkInternalList(requires);
}
/**
*
* A list of SSM documents required by a document. This parameter is used exclusively by AppConfig. When a user
* creates an AppConfig configuration in an SSM document, the user must also specify a required document for
* validation purposes. In this case, an ApplicationConfiguration
document requires an
* ApplicationConfigurationSchema
document for validation purposes. For more information, see What is AppConfig? in
* the AppConfig User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRequires(java.util.Collection)} or {@link #withRequires(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param requires
* A list of SSM documents required by a document. This parameter is used exclusively by AppConfig. When a
* user creates an AppConfig configuration in an SSM document, the user must also specify a required document
* for validation purposes. In this case, an ApplicationConfiguration
document requires an
* ApplicationConfigurationSchema
document for validation purposes. For more information, see What is
* AppConfig? in the AppConfig User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withRequires(DocumentRequires... requires) {
if (this.requires == null) {
setRequires(new com.amazonaws.internal.SdkInternalList(requires.length));
}
for (DocumentRequires ele : requires) {
this.requires.add(ele);
}
return this;
}
/**
*
* A list of SSM documents required by a document. This parameter is used exclusively by AppConfig. When a user
* creates an AppConfig configuration in an SSM document, the user must also specify a required document for
* validation purposes. In this case, an ApplicationConfiguration
document requires an
* ApplicationConfigurationSchema
document for validation purposes. For more information, see What is AppConfig? in
* the AppConfig User Guide.
*
*
* @param requires
* A list of SSM documents required by a document. This parameter is used exclusively by AppConfig. When a
* user creates an AppConfig configuration in an SSM document, the user must also specify a required document
* for validation purposes. In this case, an ApplicationConfiguration
document requires an
* ApplicationConfigurationSchema
document for validation purposes. For more information, see What is
* AppConfig? in the AppConfig User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withRequires(java.util.Collection requires) {
setRequires(requires);
return this;
}
/**
*
* A list of key-value pairs that describe attachments to a version of a document.
*
*
* @return A list of key-value pairs that describe attachments to a version of a document.
*/
public java.util.List getAttachments() {
if (attachments == null) {
attachments = new com.amazonaws.internal.SdkInternalList();
}
return attachments;
}
/**
*
* A list of key-value pairs that describe attachments to a version of a document.
*
*
* @param attachments
* A list of key-value pairs that describe attachments to a version of a document.
*/
public void setAttachments(java.util.Collection attachments) {
if (attachments == null) {
this.attachments = null;
return;
}
this.attachments = new com.amazonaws.internal.SdkInternalList(attachments);
}
/**
*
* A list of key-value pairs that describe attachments to a version of a document.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAttachments(java.util.Collection)} or {@link #withAttachments(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param attachments
* A list of key-value pairs that describe attachments to a version of a document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withAttachments(AttachmentsSource... attachments) {
if (this.attachments == null) {
setAttachments(new com.amazonaws.internal.SdkInternalList(attachments.length));
}
for (AttachmentsSource ele : attachments) {
this.attachments.add(ele);
}
return this;
}
/**
*
* A list of key-value pairs that describe attachments to a version of a document.
*
*
* @param attachments
* A list of key-value pairs that describe attachments to a version of a document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withAttachments(java.util.Collection attachments) {
setAttachments(attachments);
return this;
}
/**
*
* A name for the SSM document.
*
*
*
* You can't use the following strings as document name prefixes. These are reserved by Amazon Web Services for use
* as document name prefixes:
*
*
* -
*
* aws
*
*
* -
*
* amazon
*
*
* -
*
* amzn
*
*
* -
*
* AWSEC2
*
*
* -
*
* AWSConfigRemediation
*
*
* -
*
* AWSSupport
*
*
*
*
*
* @param name
* A name for the SSM document.
*
* You can't use the following strings as document name prefixes. These are reserved by Amazon Web Services
* for use as document name prefixes:
*
*
* -
*
* aws
*
*
* -
*
* amazon
*
*
* -
*
* amzn
*
*
* -
*
* AWSEC2
*
*
* -
*
* AWSConfigRemediation
*
*
* -
*
* AWSSupport
*
*
*
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A name for the SSM document.
*
*
*
* You can't use the following strings as document name prefixes. These are reserved by Amazon Web Services for use
* as document name prefixes:
*
*
* -
*
* aws
*
*
* -
*
* amazon
*
*
* -
*
* amzn
*
*
* -
*
* AWSEC2
*
*
* -
*
* AWSConfigRemediation
*
*
* -
*
* AWSSupport
*
*
*
*
*
* @return A name for the SSM document.
*
* You can't use the following strings as document name prefixes. These are reserved by Amazon Web Services
* for use as document name prefixes:
*
*
* -
*
* aws
*
*
* -
*
* amazon
*
*
* -
*
* amzn
*
*
* -
*
* AWSEC2
*
*
* -
*
* AWSConfigRemediation
*
*
* -
*
* AWSSupport
*
*
*
*/
public String getName() {
return this.name;
}
/**
*
* A name for the SSM document.
*
*
*
* You can't use the following strings as document name prefixes. These are reserved by Amazon Web Services for use
* as document name prefixes:
*
*
* -
*
* aws
*
*
* -
*
* amazon
*
*
* -
*
* amzn
*
*
* -
*
* AWSEC2
*
*
* -
*
* AWSConfigRemediation
*
*
* -
*
* AWSSupport
*
*
*
*
*
* @param name
* A name for the SSM document.
*
* You can't use the following strings as document name prefixes. These are reserved by Amazon Web Services
* for use as document name prefixes:
*
*
* -
*
* aws
*
*
* -
*
* amazon
*
*
* -
*
* amzn
*
*
* -
*
* AWSEC2
*
*
* -
*
* AWSConfigRemediation
*
*
* -
*
* AWSSupport
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withName(String name) {
setName(name);
return this;
}
/**
*
* An optional field where you can specify a friendly name for the SSM document. This value can differ for each
* version of the document. You can update this value at a later time using the UpdateDocument operation.
*
*
* @param displayName
* An optional field where you can specify a friendly name for the SSM document. This value can differ for
* each version of the document. You can update this value at a later time using the UpdateDocument
* operation.
*/
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
*
* An optional field where you can specify a friendly name for the SSM document. This value can differ for each
* version of the document. You can update this value at a later time using the UpdateDocument operation.
*
*
* @return An optional field where you can specify a friendly name for the SSM document. This value can differ for
* each version of the document. You can update this value at a later time using the UpdateDocument
* operation.
*/
public String getDisplayName() {
return this.displayName;
}
/**
*
* An optional field where you can specify a friendly name for the SSM document. This value can differ for each
* version of the document. You can update this value at a later time using the UpdateDocument operation.
*
*
* @param displayName
* An optional field where you can specify a friendly name for the SSM document. This value can differ for
* each version of the document. You can update this value at a later time using the UpdateDocument
* operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withDisplayName(String displayName) {
setDisplayName(displayName);
return this;
}
/**
*
* An optional field specifying the version of the artifact you are creating with the document. For example,
* Release12.1
. This value is unique across all versions of a document, and can't be changed.
*
*
* @param versionName
* An optional field specifying the version of the artifact you are creating with the document. For example,
* Release12.1
. This value is unique across all versions of a document, and can't be changed.
*/
public void setVersionName(String versionName) {
this.versionName = versionName;
}
/**
*
* An optional field specifying the version of the artifact you are creating with the document. For example,
* Release12.1
. This value is unique across all versions of a document, and can't be changed.
*
*
* @return An optional field specifying the version of the artifact you are creating with the document. For example,
* Release12.1
. This value is unique across all versions of a document, and can't be changed.
*/
public String getVersionName() {
return this.versionName;
}
/**
*
* An optional field specifying the version of the artifact you are creating with the document. For example,
* Release12.1
. This value is unique across all versions of a document, and can't be changed.
*
*
* @param versionName
* An optional field specifying the version of the artifact you are creating with the document. For example,
* Release12.1
. This value is unique across all versions of a document, and can't be changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withVersionName(String versionName) {
setVersionName(versionName);
return this;
}
/**
*
* The type of document to create.
*
*
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for AppConfig.
*
*
*
* @param documentType
* The type of document to create.
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for
* AppConfig.
*
* @see DocumentType
*/
public void setDocumentType(String documentType) {
this.documentType = documentType;
}
/**
*
* The type of document to create.
*
*
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for AppConfig.
*
*
*
* @return The type of document to create.
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for
* AppConfig.
*
* @see DocumentType
*/
public String getDocumentType() {
return this.documentType;
}
/**
*
* The type of document to create.
*
*
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for AppConfig.
*
*
*
* @param documentType
* The type of document to create.
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for
* AppConfig.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentType
*/
public CreateDocumentRequest withDocumentType(String documentType) {
setDocumentType(documentType);
return this;
}
/**
*
* The type of document to create.
*
*
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for AppConfig.
*
*
*
* @param documentType
* The type of document to create.
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for
* AppConfig.
*
* @see DocumentType
*/
public void setDocumentType(DocumentType documentType) {
withDocumentType(documentType);
}
/**
*
* The type of document to create.
*
*
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for AppConfig.
*
*
*
* @param documentType
* The type of document to create.
*
* The DeploymentStrategy
document type is an internal-use-only document type reserved for
* AppConfig.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentType
*/
public CreateDocumentRequest withDocumentType(DocumentType documentType) {
this.documentType = documentType.toString();
return this;
}
/**
*
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the default
* format.
*
*
* @param documentFormat
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the
* default format.
* @see DocumentFormat
*/
public void setDocumentFormat(String documentFormat) {
this.documentFormat = documentFormat;
}
/**
*
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the default
* format.
*
*
* @return Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the
* default format.
* @see DocumentFormat
*/
public String getDocumentFormat() {
return this.documentFormat;
}
/**
*
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the default
* format.
*
*
* @param documentFormat
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the
* default format.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentFormat
*/
public CreateDocumentRequest withDocumentFormat(String documentFormat) {
setDocumentFormat(documentFormat);
return this;
}
/**
*
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the default
* format.
*
*
* @param documentFormat
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the
* default format.
* @see DocumentFormat
*/
public void setDocumentFormat(DocumentFormat documentFormat) {
withDocumentFormat(documentFormat);
}
/**
*
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the default
* format.
*
*
* @param documentFormat
* Specify the document format for the request. The document format can be JSON, YAML, or TEXT. JSON is the
* default format.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentFormat
*/
public CreateDocumentRequest withDocumentFormat(DocumentFormat documentFormat) {
this.documentFormat = documentFormat.toString();
return this;
}
/**
*
* Specify a target type to define the kinds of resources the document can run on. For example, to run a document on
* EC2 instances, specify the following value: /AWS::EC2::Instance
. If you specify a value of '/' the
* document can run on all types of resources. If you don't specify a value, the document can't run on any
* resources. For a list of valid resource types, see Amazon
* Web Services resource and property types reference in the CloudFormation User Guide.
*
*
* @param targetType
* Specify a target type to define the kinds of resources the document can run on. For example, to run a
* document on EC2 instances, specify the following value: /AWS::EC2::Instance
. If you specify a
* value of '/' the document can run on all types of resources. If you don't specify a value, the document
* can't run on any resources. For a list of valid resource types, see Amazon Web Services resource and property types reference in the CloudFormation User Guide.
*/
public void setTargetType(String targetType) {
this.targetType = targetType;
}
/**
*
* Specify a target type to define the kinds of resources the document can run on. For example, to run a document on
* EC2 instances, specify the following value: /AWS::EC2::Instance
. If you specify a value of '/' the
* document can run on all types of resources. If you don't specify a value, the document can't run on any
* resources. For a list of valid resource types, see Amazon
* Web Services resource and property types reference in the CloudFormation User Guide.
*
*
* @return Specify a target type to define the kinds of resources the document can run on. For example, to run a
* document on EC2 instances, specify the following value: /AWS::EC2::Instance
. If you specify
* a value of '/' the document can run on all types of resources. If you don't specify a value, the document
* can't run on any resources. For a list of valid resource types, see Amazon Web Services resource and property types reference in the CloudFormation User Guide.
*/
public String getTargetType() {
return this.targetType;
}
/**
*
* Specify a target type to define the kinds of resources the document can run on. For example, to run a document on
* EC2 instances, specify the following value: /AWS::EC2::Instance
. If you specify a value of '/' the
* document can run on all types of resources. If you don't specify a value, the document can't run on any
* resources. For a list of valid resource types, see Amazon
* Web Services resource and property types reference in the CloudFormation User Guide.
*
*
* @param targetType
* Specify a target type to define the kinds of resources the document can run on. For example, to run a
* document on EC2 instances, specify the following value: /AWS::EC2::Instance
. If you specify a
* value of '/' the document can run on all types of resources. If you don't specify a value, the document
* can't run on any resources. For a list of valid resource types, see Amazon Web Services resource and property types reference in the CloudFormation User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withTargetType(String targetType) {
setTargetType(targetType);
return this;
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag an SSM document to identify the types of
* targets or the environment where it will run. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing SSM document, use the AddTagsToResource operation.
*
*
*
* @return Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag an SSM document to
* identify the types of targets or the environment where it will run. In this case, you could specify the
* following key-value pairs:
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing SSM document, use the AddTagsToResource operation.
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag an SSM document to identify the types of
* targets or the environment where it will run. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing SSM document, use the AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag an SSM document to
* identify the types of targets or the environment where it will run. In this case, you could specify the
* following key-value pairs:
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing SSM document, use the AddTagsToResource operation.
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag an SSM document to identify the types of
* targets or the environment where it will run. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing SSM document, use the AddTagsToResource operation.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag an SSM document to
* identify the types of targets or the environment where it will run. In this case, you could specify the
* following key-value pairs:
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing SSM document, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag an SSM document to identify the types of
* targets or the environment where it will run. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing SSM document, use the AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag an SSM document to
* identify the types of targets or the environment where it will run. In this case, you could specify the
* following key-value pairs:
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing SSM document, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDocumentRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getContent() != null)
sb.append("Content: ").append(getContent()).append(",");
if (getRequires() != null)
sb.append("Requires: ").append(getRequires()).append(",");
if (getAttachments() != null)
sb.append("Attachments: ").append(getAttachments()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDisplayName() != null)
sb.append("DisplayName: ").append(getDisplayName()).append(",");
if (getVersionName() != null)
sb.append("VersionName: ").append(getVersionName()).append(",");
if (getDocumentType() != null)
sb.append("DocumentType: ").append(getDocumentType()).append(",");
if (getDocumentFormat() != null)
sb.append("DocumentFormat: ").append(getDocumentFormat()).append(",");
if (getTargetType() != null)
sb.append("TargetType: ").append(getTargetType()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDocumentRequest == false)
return false;
CreateDocumentRequest other = (CreateDocumentRequest) obj;
if (other.getContent() == null ^ this.getContent() == null)
return false;
if (other.getContent() != null && other.getContent().equals(this.getContent()) == false)
return false;
if (other.getRequires() == null ^ this.getRequires() == null)
return false;
if (other.getRequires() != null && other.getRequires().equals(this.getRequires()) == false)
return false;
if (other.getAttachments() == null ^ this.getAttachments() == null)
return false;
if (other.getAttachments() != null && other.getAttachments().equals(this.getAttachments()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDisplayName() == null ^ this.getDisplayName() == null)
return false;
if (other.getDisplayName() != null && other.getDisplayName().equals(this.getDisplayName()) == false)
return false;
if (other.getVersionName() == null ^ this.getVersionName() == null)
return false;
if (other.getVersionName() != null && other.getVersionName().equals(this.getVersionName()) == false)
return false;
if (other.getDocumentType() == null ^ this.getDocumentType() == null)
return false;
if (other.getDocumentType() != null && other.getDocumentType().equals(this.getDocumentType()) == false)
return false;
if (other.getDocumentFormat() == null ^ this.getDocumentFormat() == null)
return false;
if (other.getDocumentFormat() != null && other.getDocumentFormat().equals(this.getDocumentFormat()) == false)
return false;
if (other.getTargetType() == null ^ this.getTargetType() == null)
return false;
if (other.getTargetType() != null && other.getTargetType().equals(this.getTargetType()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getContent() == null) ? 0 : getContent().hashCode());
hashCode = prime * hashCode + ((getRequires() == null) ? 0 : getRequires().hashCode());
hashCode = prime * hashCode + ((getAttachments() == null) ? 0 : getAttachments().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDisplayName() == null) ? 0 : getDisplayName().hashCode());
hashCode = prime * hashCode + ((getVersionName() == null) ? 0 : getVersionName().hashCode());
hashCode = prime * hashCode + ((getDocumentType() == null) ? 0 : getDocumentType().hashCode());
hashCode = prime * hashCode + ((getDocumentFormat() == null) ? 0 : getDocumentFormat().hashCode());
hashCode = prime * hashCode + ((getTargetType() == null) ? 0 : getTargetType().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateDocumentRequest clone() {
return (CreateDocumentRequest) super.clone();
}
}