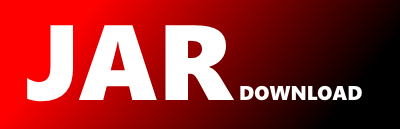
com.amazonaws.services.simplesystemsmanagement.model.CreateMaintenanceWindowRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateMaintenanceWindowRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the maintenance window.
*
*/
private String name;
/**
*
* An optional description for the maintenance window. We recommend specifying a description to help you organize
* your maintenance windows.
*
*/
private String description;
/**
*
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become active.
* StartDate
allows you to delay activation of the maintenance window until the specified future date.
*
*
*
* When using a rate schedule, if you provide a start date that occurs in the past, the current date and time are
* used as the start date.
*
*
*/
private String startDate;
/**
*
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become inactive.
* EndDate
allows you to set a date and time in the future when the maintenance window will no longer
* run.
*
*/
private String endDate;
/**
*
* The schedule of the maintenance window in the form of a cron or rate expression.
*
*/
private String schedule;
/**
*
* The time zone that the scheduled maintenance window executions are based on, in Internet Assigned Numbers
* Authority (IANA) format. For example: "America/Los_Angeles", "UTC", or "Asia/Seoul". For more information, see
* the Time Zone Database on the IANA website.
*
*/
private String scheduleTimezone;
/**
*
* The number of days to wait after the date and time specified by a cron expression before running the maintenance
* window.
*
*
* For example, the following cron expression schedules a maintenance window to run on the third Tuesday of every
* month at 11:30 PM.
*
*
* cron(30 23 ? * TUE#3 *)
*
*
* If the schedule offset is 2
, the maintenance window won't run until two days later.
*
*/
private Integer scheduleOffset;
/**
*
* The duration of the maintenance window in hours.
*
*/
private Integer duration;
/**
*
* The number of hours before the end of the maintenance window that Amazon Web Services Systems Manager stops
* scheduling new tasks for execution.
*
*/
private Integer cutoff;
/**
*
* Enables a maintenance window task to run on managed nodes, even if you haven't registered those nodes as targets.
* If enabled, then you must specify the unregistered managed nodes (by node ID) when you register a task with the
* maintenance window.
*
*
* If you don't enable this option, then you must specify previously-registered targets when you register a task
* with the maintenance window.
*
*/
private Boolean allowUnassociatedTargets;
/**
*
* User-provided idempotency token.
*
*/
private String clientToken;
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a maintenance window to identify the
* type of tasks it will run, the types of targets, and the environment it will run in. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=TaskType,Value=AgentUpdate
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing maintenance window, use the AddTagsToResource operation.
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The name of the maintenance window.
*
*
* @param name
* The name of the maintenance window.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the maintenance window.
*
*
* @return The name of the maintenance window.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the maintenance window.
*
*
* @param name
* The name of the maintenance window.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withName(String name) {
setName(name);
return this;
}
/**
*
* An optional description for the maintenance window. We recommend specifying a description to help you organize
* your maintenance windows.
*
*
* @param description
* An optional description for the maintenance window. We recommend specifying a description to help you
* organize your maintenance windows.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* An optional description for the maintenance window. We recommend specifying a description to help you organize
* your maintenance windows.
*
*
* @return An optional description for the maintenance window. We recommend specifying a description to help you
* organize your maintenance windows.
*/
public String getDescription() {
return this.description;
}
/**
*
* An optional description for the maintenance window. We recommend specifying a description to help you organize
* your maintenance windows.
*
*
* @param description
* An optional description for the maintenance window. We recommend specifying a description to help you
* organize your maintenance windows.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become active.
* StartDate
allows you to delay activation of the maintenance window until the specified future date.
*
*
*
* When using a rate schedule, if you provide a start date that occurs in the past, the current date and time are
* used as the start date.
*
*
*
* @param startDate
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become active.
* StartDate
allows you to delay activation of the maintenance window until the specified future
* date.
*
* When using a rate schedule, if you provide a start date that occurs in the past, the current date and time
* are used as the start date.
*
*/
public void setStartDate(String startDate) {
this.startDate = startDate;
}
/**
*
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become active.
* StartDate
allows you to delay activation of the maintenance window until the specified future date.
*
*
*
* When using a rate schedule, if you provide a start date that occurs in the past, the current date and time are
* used as the start date.
*
*
*
* @return The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become
* active. StartDate
allows you to delay activation of the maintenance window until the
* specified future date.
*
* When using a rate schedule, if you provide a start date that occurs in the past, the current date and
* time are used as the start date.
*
*/
public String getStartDate() {
return this.startDate;
}
/**
*
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become active.
* StartDate
allows you to delay activation of the maintenance window until the specified future date.
*
*
*
* When using a rate schedule, if you provide a start date that occurs in the past, the current date and time are
* used as the start date.
*
*
*
* @param startDate
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become active.
* StartDate
allows you to delay activation of the maintenance window until the specified future
* date.
*
* When using a rate schedule, if you provide a start date that occurs in the past, the current date and time
* are used as the start date.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withStartDate(String startDate) {
setStartDate(startDate);
return this;
}
/**
*
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become inactive.
* EndDate
allows you to set a date and time in the future when the maintenance window will no longer
* run.
*
*
* @param endDate
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become
* inactive. EndDate
allows you to set a date and time in the future when the maintenance window
* will no longer run.
*/
public void setEndDate(String endDate) {
this.endDate = endDate;
}
/**
*
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become inactive.
* EndDate
allows you to set a date and time in the future when the maintenance window will no longer
* run.
*
*
* @return The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become
* inactive. EndDate
allows you to set a date and time in the future when the maintenance
* window will no longer run.
*/
public String getEndDate() {
return this.endDate;
}
/**
*
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become inactive.
* EndDate
allows you to set a date and time in the future when the maintenance window will no longer
* run.
*
*
* @param endDate
* The date and time, in ISO-8601 Extended format, for when you want the maintenance window to become
* inactive. EndDate
allows you to set a date and time in the future when the maintenance window
* will no longer run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withEndDate(String endDate) {
setEndDate(endDate);
return this;
}
/**
*
* The schedule of the maintenance window in the form of a cron or rate expression.
*
*
* @param schedule
* The schedule of the maintenance window in the form of a cron or rate expression.
*/
public void setSchedule(String schedule) {
this.schedule = schedule;
}
/**
*
* The schedule of the maintenance window in the form of a cron or rate expression.
*
*
* @return The schedule of the maintenance window in the form of a cron or rate expression.
*/
public String getSchedule() {
return this.schedule;
}
/**
*
* The schedule of the maintenance window in the form of a cron or rate expression.
*
*
* @param schedule
* The schedule of the maintenance window in the form of a cron or rate expression.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withSchedule(String schedule) {
setSchedule(schedule);
return this;
}
/**
*
* The time zone that the scheduled maintenance window executions are based on, in Internet Assigned Numbers
* Authority (IANA) format. For example: "America/Los_Angeles", "UTC", or "Asia/Seoul". For more information, see
* the Time Zone Database on the IANA website.
*
*
* @param scheduleTimezone
* The time zone that the scheduled maintenance window executions are based on, in Internet Assigned Numbers
* Authority (IANA) format. For example: "America/Los_Angeles", "UTC", or "Asia/Seoul". For more information,
* see the Time Zone Database on the IANA website.
*/
public void setScheduleTimezone(String scheduleTimezone) {
this.scheduleTimezone = scheduleTimezone;
}
/**
*
* The time zone that the scheduled maintenance window executions are based on, in Internet Assigned Numbers
* Authority (IANA) format. For example: "America/Los_Angeles", "UTC", or "Asia/Seoul". For more information, see
* the Time Zone Database on the IANA website.
*
*
* @return The time zone that the scheduled maintenance window executions are based on, in Internet Assigned Numbers
* Authority (IANA) format. For example: "America/Los_Angeles", "UTC", or "Asia/Seoul". For more
* information, see the Time Zone Database on the IANA
* website.
*/
public String getScheduleTimezone() {
return this.scheduleTimezone;
}
/**
*
* The time zone that the scheduled maintenance window executions are based on, in Internet Assigned Numbers
* Authority (IANA) format. For example: "America/Los_Angeles", "UTC", or "Asia/Seoul". For more information, see
* the Time Zone Database on the IANA website.
*
*
* @param scheduleTimezone
* The time zone that the scheduled maintenance window executions are based on, in Internet Assigned Numbers
* Authority (IANA) format. For example: "America/Los_Angeles", "UTC", or "Asia/Seoul". For more information,
* see the Time Zone Database on the IANA website.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withScheduleTimezone(String scheduleTimezone) {
setScheduleTimezone(scheduleTimezone);
return this;
}
/**
*
* The number of days to wait after the date and time specified by a cron expression before running the maintenance
* window.
*
*
* For example, the following cron expression schedules a maintenance window to run on the third Tuesday of every
* month at 11:30 PM.
*
*
* cron(30 23 ? * TUE#3 *)
*
*
* If the schedule offset is 2
, the maintenance window won't run until two days later.
*
*
* @param scheduleOffset
* The number of days to wait after the date and time specified by a cron expression before running the
* maintenance window.
*
* For example, the following cron expression schedules a maintenance window to run on the third Tuesday of
* every month at 11:30 PM.
*
*
* cron(30 23 ? * TUE#3 *)
*
*
* If the schedule offset is 2
, the maintenance window won't run until two days later.
*/
public void setScheduleOffset(Integer scheduleOffset) {
this.scheduleOffset = scheduleOffset;
}
/**
*
* The number of days to wait after the date and time specified by a cron expression before running the maintenance
* window.
*
*
* For example, the following cron expression schedules a maintenance window to run on the third Tuesday of every
* month at 11:30 PM.
*
*
* cron(30 23 ? * TUE#3 *)
*
*
* If the schedule offset is 2
, the maintenance window won't run until two days later.
*
*
* @return The number of days to wait after the date and time specified by a cron expression before running the
* maintenance window.
*
* For example, the following cron expression schedules a maintenance window to run on the third Tuesday of
* every month at 11:30 PM.
*
*
* cron(30 23 ? * TUE#3 *)
*
*
* If the schedule offset is 2
, the maintenance window won't run until two days later.
*/
public Integer getScheduleOffset() {
return this.scheduleOffset;
}
/**
*
* The number of days to wait after the date and time specified by a cron expression before running the maintenance
* window.
*
*
* For example, the following cron expression schedules a maintenance window to run on the third Tuesday of every
* month at 11:30 PM.
*
*
* cron(30 23 ? * TUE#3 *)
*
*
* If the schedule offset is 2
, the maintenance window won't run until two days later.
*
*
* @param scheduleOffset
* The number of days to wait after the date and time specified by a cron expression before running the
* maintenance window.
*
* For example, the following cron expression schedules a maintenance window to run on the third Tuesday of
* every month at 11:30 PM.
*
*
* cron(30 23 ? * TUE#3 *)
*
*
* If the schedule offset is 2
, the maintenance window won't run until two days later.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withScheduleOffset(Integer scheduleOffset) {
setScheduleOffset(scheduleOffset);
return this;
}
/**
*
* The duration of the maintenance window in hours.
*
*
* @param duration
* The duration of the maintenance window in hours.
*/
public void setDuration(Integer duration) {
this.duration = duration;
}
/**
*
* The duration of the maintenance window in hours.
*
*
* @return The duration of the maintenance window in hours.
*/
public Integer getDuration() {
return this.duration;
}
/**
*
* The duration of the maintenance window in hours.
*
*
* @param duration
* The duration of the maintenance window in hours.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withDuration(Integer duration) {
setDuration(duration);
return this;
}
/**
*
* The number of hours before the end of the maintenance window that Amazon Web Services Systems Manager stops
* scheduling new tasks for execution.
*
*
* @param cutoff
* The number of hours before the end of the maintenance window that Amazon Web Services Systems Manager
* stops scheduling new tasks for execution.
*/
public void setCutoff(Integer cutoff) {
this.cutoff = cutoff;
}
/**
*
* The number of hours before the end of the maintenance window that Amazon Web Services Systems Manager stops
* scheduling new tasks for execution.
*
*
* @return The number of hours before the end of the maintenance window that Amazon Web Services Systems Manager
* stops scheduling new tasks for execution.
*/
public Integer getCutoff() {
return this.cutoff;
}
/**
*
* The number of hours before the end of the maintenance window that Amazon Web Services Systems Manager stops
* scheduling new tasks for execution.
*
*
* @param cutoff
* The number of hours before the end of the maintenance window that Amazon Web Services Systems Manager
* stops scheduling new tasks for execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withCutoff(Integer cutoff) {
setCutoff(cutoff);
return this;
}
/**
*
* Enables a maintenance window task to run on managed nodes, even if you haven't registered those nodes as targets.
* If enabled, then you must specify the unregistered managed nodes (by node ID) when you register a task with the
* maintenance window.
*
*
* If you don't enable this option, then you must specify previously-registered targets when you register a task
* with the maintenance window.
*
*
* @param allowUnassociatedTargets
* Enables a maintenance window task to run on managed nodes, even if you haven't registered those nodes as
* targets. If enabled, then you must specify the unregistered managed nodes (by node ID) when you register a
* task with the maintenance window.
*
* If you don't enable this option, then you must specify previously-registered targets when you register a
* task with the maintenance window.
*/
public void setAllowUnassociatedTargets(Boolean allowUnassociatedTargets) {
this.allowUnassociatedTargets = allowUnassociatedTargets;
}
/**
*
* Enables a maintenance window task to run on managed nodes, even if you haven't registered those nodes as targets.
* If enabled, then you must specify the unregistered managed nodes (by node ID) when you register a task with the
* maintenance window.
*
*
* If you don't enable this option, then you must specify previously-registered targets when you register a task
* with the maintenance window.
*
*
* @return Enables a maintenance window task to run on managed nodes, even if you haven't registered those nodes as
* targets. If enabled, then you must specify the unregistered managed nodes (by node ID) when you register
* a task with the maintenance window.
*
* If you don't enable this option, then you must specify previously-registered targets when you register a
* task with the maintenance window.
*/
public Boolean getAllowUnassociatedTargets() {
return this.allowUnassociatedTargets;
}
/**
*
* Enables a maintenance window task to run on managed nodes, even if you haven't registered those nodes as targets.
* If enabled, then you must specify the unregistered managed nodes (by node ID) when you register a task with the
* maintenance window.
*
*
* If you don't enable this option, then you must specify previously-registered targets when you register a task
* with the maintenance window.
*
*
* @param allowUnassociatedTargets
* Enables a maintenance window task to run on managed nodes, even if you haven't registered those nodes as
* targets. If enabled, then you must specify the unregistered managed nodes (by node ID) when you register a
* task with the maintenance window.
*
* If you don't enable this option, then you must specify previously-registered targets when you register a
* task with the maintenance window.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withAllowUnassociatedTargets(Boolean allowUnassociatedTargets) {
setAllowUnassociatedTargets(allowUnassociatedTargets);
return this;
}
/**
*
* Enables a maintenance window task to run on managed nodes, even if you haven't registered those nodes as targets.
* If enabled, then you must specify the unregistered managed nodes (by node ID) when you register a task with the
* maintenance window.
*
*
* If you don't enable this option, then you must specify previously-registered targets when you register a task
* with the maintenance window.
*
*
* @return Enables a maintenance window task to run on managed nodes, even if you haven't registered those nodes as
* targets. If enabled, then you must specify the unregistered managed nodes (by node ID) when you register
* a task with the maintenance window.
*
* If you don't enable this option, then you must specify previously-registered targets when you register a
* task with the maintenance window.
*/
public Boolean isAllowUnassociatedTargets() {
return this.allowUnassociatedTargets;
}
/**
*
* User-provided idempotency token.
*
*
* @param clientToken
* User-provided idempotency token.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* User-provided idempotency token.
*
*
* @return User-provided idempotency token.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* User-provided idempotency token.
*
*
* @param clientToken
* User-provided idempotency token.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a maintenance window to identify the
* type of tasks it will run, the types of targets, and the environment it will run in. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=TaskType,Value=AgentUpdate
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing maintenance window, use the AddTagsToResource operation.
*
*
*
* @return Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag a maintenance window
* to identify the type of tasks it will run, the types of targets, and the environment it will run in. In
* this case, you could specify the following key-value pairs:
*
* -
*
* Key=TaskType,Value=AgentUpdate
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing maintenance window, use the AddTagsToResource operation.
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a maintenance window to identify the
* type of tasks it will run, the types of targets, and the environment it will run in. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=TaskType,Value=AgentUpdate
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing maintenance window, use the AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag a maintenance window
* to identify the type of tasks it will run, the types of targets, and the environment it will run in. In
* this case, you could specify the following key-value pairs:
*
* -
*
* Key=TaskType,Value=AgentUpdate
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing maintenance window, use the AddTagsToResource operation.
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a maintenance window to identify the
* type of tasks it will run, the types of targets, and the environment it will run in. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=TaskType,Value=AgentUpdate
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing maintenance window, use the AddTagsToResource operation.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag a maintenance window
* to identify the type of tasks it will run, the types of targets, and the environment it will run in. In
* this case, you could specify the following key-value pairs:
*
* -
*
* Key=TaskType,Value=AgentUpdate
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing maintenance window, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a maintenance window to identify the
* type of tasks it will run, the types of targets, and the environment it will run in. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=TaskType,Value=AgentUpdate
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing maintenance window, use the AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag a maintenance window
* to identify the type of tasks it will run, the types of targets, and the environment it will run in. In
* this case, you could specify the following key-value pairs:
*
* -
*
* Key=TaskType,Value=AgentUpdate
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=Environment,Value=Production
*
*
*
*
*
* To add tags to an existing maintenance window, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMaintenanceWindowRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getStartDate() != null)
sb.append("StartDate: ").append(getStartDate()).append(",");
if (getEndDate() != null)
sb.append("EndDate: ").append(getEndDate()).append(",");
if (getSchedule() != null)
sb.append("Schedule: ").append(getSchedule()).append(",");
if (getScheduleTimezone() != null)
sb.append("ScheduleTimezone: ").append(getScheduleTimezone()).append(",");
if (getScheduleOffset() != null)
sb.append("ScheduleOffset: ").append(getScheduleOffset()).append(",");
if (getDuration() != null)
sb.append("Duration: ").append(getDuration()).append(",");
if (getCutoff() != null)
sb.append("Cutoff: ").append(getCutoff()).append(",");
if (getAllowUnassociatedTargets() != null)
sb.append("AllowUnassociatedTargets: ").append(getAllowUnassociatedTargets()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateMaintenanceWindowRequest == false)
return false;
CreateMaintenanceWindowRequest other = (CreateMaintenanceWindowRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getStartDate() == null ^ this.getStartDate() == null)
return false;
if (other.getStartDate() != null && other.getStartDate().equals(this.getStartDate()) == false)
return false;
if (other.getEndDate() == null ^ this.getEndDate() == null)
return false;
if (other.getEndDate() != null && other.getEndDate().equals(this.getEndDate()) == false)
return false;
if (other.getSchedule() == null ^ this.getSchedule() == null)
return false;
if (other.getSchedule() != null && other.getSchedule().equals(this.getSchedule()) == false)
return false;
if (other.getScheduleTimezone() == null ^ this.getScheduleTimezone() == null)
return false;
if (other.getScheduleTimezone() != null && other.getScheduleTimezone().equals(this.getScheduleTimezone()) == false)
return false;
if (other.getScheduleOffset() == null ^ this.getScheduleOffset() == null)
return false;
if (other.getScheduleOffset() != null && other.getScheduleOffset().equals(this.getScheduleOffset()) == false)
return false;
if (other.getDuration() == null ^ this.getDuration() == null)
return false;
if (other.getDuration() != null && other.getDuration().equals(this.getDuration()) == false)
return false;
if (other.getCutoff() == null ^ this.getCutoff() == null)
return false;
if (other.getCutoff() != null && other.getCutoff().equals(this.getCutoff()) == false)
return false;
if (other.getAllowUnassociatedTargets() == null ^ this.getAllowUnassociatedTargets() == null)
return false;
if (other.getAllowUnassociatedTargets() != null && other.getAllowUnassociatedTargets().equals(this.getAllowUnassociatedTargets()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getStartDate() == null) ? 0 : getStartDate().hashCode());
hashCode = prime * hashCode + ((getEndDate() == null) ? 0 : getEndDate().hashCode());
hashCode = prime * hashCode + ((getSchedule() == null) ? 0 : getSchedule().hashCode());
hashCode = prime * hashCode + ((getScheduleTimezone() == null) ? 0 : getScheduleTimezone().hashCode());
hashCode = prime * hashCode + ((getScheduleOffset() == null) ? 0 : getScheduleOffset().hashCode());
hashCode = prime * hashCode + ((getDuration() == null) ? 0 : getDuration().hashCode());
hashCode = prime * hashCode + ((getCutoff() == null) ? 0 : getCutoff().hashCode());
hashCode = prime * hashCode + ((getAllowUnassociatedTargets() == null) ? 0 : getAllowUnassociatedTargets().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateMaintenanceWindowRequest clone() {
return (CreateMaintenanceWindowRequest) super.clone();
}
}