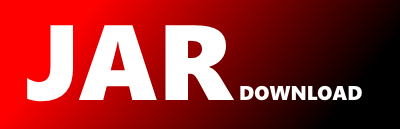
com.amazonaws.services.simplesystemsmanagement.model.CreateOpsItemRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateOpsItemRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* User-defined text that contains information about the OpsItem, in Markdown format.
*
*
*
* Provide enough information so that users viewing this OpsItem for the first time understand the issue.
*
*
*/
private String description;
/**
*
* The type of OpsItem to create. Systems Manager supports the following types of OpsItems:
*
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*
*/
private String opsItemType;
/**
*
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you can
* specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You enter
* operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a maximum size
* of 20 KB.
*
*
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search access.
* Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that isn't
* searchable is only viewable by users who have access to the OpsItem (as provided by the GetOpsItem API
* operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request. Use the
* /aws/automations
key in OperationalData to associate an Automation runbook with the OpsItem. To view
* Amazon Web Services CLI example commands that use these keys, see Create OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*
*/
private java.util.Map operationalData;
/**
*
* The Amazon Resource Name (ARN) of an SNS topic where notifications are sent when this OpsItem is edited or
* changed.
*
*/
private com.amazonaws.internal.SdkInternalList notifications;
/**
*
* The importance of this OpsItem in relation to other OpsItems in the system.
*
*/
private Integer priority;
/**
*
* One or more OpsItems that share something in common with the current OpsItems. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*/
private com.amazonaws.internal.SdkInternalList relatedOpsItems;
/**
*
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager.
*
*
*
* The source name can't contain the following strings: aws
, amazon
, and amzn
* .
*
*
*/
private String source;
/**
*
* A short heading that describes the nature of the OpsItem and the impacted resource.
*
*/
private String title;
/**
*
* Optional metadata that you assign to a resource.
*
*
* Tags use a key-value pair. For example:
*
*
* Key=Department,Value=Finance
*
*
*
* To add tags to a new OpsItem, a user must have IAM permissions for both the ssm:CreateOpsItems
* operation and the ssm:AddTagsToResource
operation. To add tags to an existing OpsItem, use the
* AddTagsToResource operation.
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* Specify a category to assign to an OpsItem.
*
*/
private String category;
/**
*
* Specify a severity to assign to an OpsItem.
*
*/
private String severity;
/**
*
* The time a runbook workflow started. Currently reported only for the OpsItem type /aws/changerequest
* .
*
*/
private java.util.Date actualStartTime;
/**
*
* The time a runbook workflow ended. Currently reported only for the OpsItem type /aws/changerequest
.
*
*/
private java.util.Date actualEndTime;
/**
*
* The time specified in a change request for a runbook workflow to start. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*/
private java.util.Date plannedStartTime;
/**
*
* The time specified in a change request for a runbook workflow to end. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*/
private java.util.Date plannedEndTime;
/**
*
* The target Amazon Web Services account where you want to create an OpsItem. To make this call, your account must
* be configured to work with OpsItems across accounts. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*/
private String accountId;
/**
*
* User-defined text that contains information about the OpsItem, in Markdown format.
*
*
*
* Provide enough information so that users viewing this OpsItem for the first time understand the issue.
*
*
*
* @param description
* User-defined text that contains information about the OpsItem, in Markdown format.
*
* Provide enough information so that users viewing this OpsItem for the first time understand the issue.
*
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* User-defined text that contains information about the OpsItem, in Markdown format.
*
*
*
* Provide enough information so that users viewing this OpsItem for the first time understand the issue.
*
*
*
* @return User-defined text that contains information about the OpsItem, in Markdown format.
*
* Provide enough information so that users viewing this OpsItem for the first time understand the issue.
*
*/
public String getDescription() {
return this.description;
}
/**
*
* User-defined text that contains information about the OpsItem, in Markdown format.
*
*
*
* Provide enough information so that users viewing this OpsItem for the first time understand the issue.
*
*
*
* @param description
* User-defined text that contains information about the OpsItem, in Markdown format.
*
* Provide enough information so that users viewing this OpsItem for the first time understand the issue.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The type of OpsItem to create. Systems Manager supports the following types of OpsItems:
*
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*
*
* @param opsItemType
* The type of OpsItem to create. Systems Manager supports the following types of OpsItems:
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*/
public void setOpsItemType(String opsItemType) {
this.opsItemType = opsItemType;
}
/**
*
* The type of OpsItem to create. Systems Manager supports the following types of OpsItems:
*
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*
*
* @return The type of OpsItem to create. Systems Manager supports the following types of OpsItems:
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*/
public String getOpsItemType() {
return this.opsItemType;
}
/**
*
* The type of OpsItem to create. Systems Manager supports the following types of OpsItems:
*
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*
*
* @param opsItemType
* The type of OpsItem to create. Systems Manager supports the following types of OpsItems:
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withOpsItemType(String opsItemType) {
setOpsItemType(opsItemType);
return this;
}
/**
*
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you can
* specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You enter
* operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a maximum size
* of 20 KB.
*
*
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search access.
* Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that isn't
* searchable is only viewable by users who have access to the OpsItem (as provided by the GetOpsItem API
* operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request. Use the
* /aws/automations
key in OperationalData to associate an Automation runbook with the OpsItem. To view
* Amazon Web Services CLI example commands that use these keys, see Create OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*
*
* @return Operational data is custom data that provides useful reference details about the OpsItem. For example,
* you can specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You
* enter operational data as key-value pairs. The key has a maximum length of 128 characters. The value has
* a maximum size of 20 KB.
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search
* access. Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that
* isn't searchable is only viewable by users who have access to the OpsItem (as provided by the
* GetOpsItem API operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request.
* Use the /aws/automations
key in OperationalData to associate an Automation runbook with the
* OpsItem. To view Amazon Web Services CLI example commands that use these keys, see Create OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*/
public java.util.Map getOperationalData() {
return operationalData;
}
/**
*
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you can
* specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You enter
* operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a maximum size
* of 20 KB.
*
*
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search access.
* Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that isn't
* searchable is only viewable by users who have access to the OpsItem (as provided by the GetOpsItem API
* operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request. Use the
* /aws/automations
key in OperationalData to associate an Automation runbook with the OpsItem. To view
* Amazon Web Services CLI example commands that use these keys, see Create OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*
*
* @param operationalData
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you
* can specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You
* enter operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a
* maximum size of 20 KB.
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search
* access. Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that
* isn't searchable is only viewable by users who have access to the OpsItem (as provided by the
* GetOpsItem API operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request.
* Use the /aws/automations
key in OperationalData to associate an Automation runbook with the
* OpsItem. To view Amazon Web Services CLI example commands that use these keys, see Create OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*/
public void setOperationalData(java.util.Map operationalData) {
this.operationalData = operationalData;
}
/**
*
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you can
* specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You enter
* operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a maximum size
* of 20 KB.
*
*
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search access.
* Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that isn't
* searchable is only viewable by users who have access to the OpsItem (as provided by the GetOpsItem API
* operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request. Use the
* /aws/automations
key in OperationalData to associate an Automation runbook with the OpsItem. To view
* Amazon Web Services CLI example commands that use these keys, see Create OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*
*
* @param operationalData
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you
* can specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You
* enter operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a
* maximum size of 20 KB.
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search
* access. Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that
* isn't searchable is only viewable by users who have access to the OpsItem (as provided by the
* GetOpsItem API operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request.
* Use the /aws/automations
key in OperationalData to associate an Automation runbook with the
* OpsItem. To view Amazon Web Services CLI example commands that use these keys, see Create OpsItems manually in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withOperationalData(java.util.Map operationalData) {
setOperationalData(operationalData);
return this;
}
/**
* Add a single OperationalData entry
*
* @see CreateOpsItemRequest#withOperationalData
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest addOperationalDataEntry(String key, OpsItemDataValue value) {
if (null == this.operationalData) {
this.operationalData = new java.util.HashMap();
}
if (this.operationalData.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.operationalData.put(key, value);
return this;
}
/**
* Removes all the entries added into OperationalData.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest clearOperationalDataEntries() {
this.operationalData = null;
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an SNS topic where notifications are sent when this OpsItem is edited or
* changed.
*
*
* @return The Amazon Resource Name (ARN) of an SNS topic where notifications are sent when this OpsItem is edited
* or changed.
*/
public java.util.List getNotifications() {
if (notifications == null) {
notifications = new com.amazonaws.internal.SdkInternalList();
}
return notifications;
}
/**
*
* The Amazon Resource Name (ARN) of an SNS topic where notifications are sent when this OpsItem is edited or
* changed.
*
*
* @param notifications
* The Amazon Resource Name (ARN) of an SNS topic where notifications are sent when this OpsItem is edited or
* changed.
*/
public void setNotifications(java.util.Collection notifications) {
if (notifications == null) {
this.notifications = null;
return;
}
this.notifications = new com.amazonaws.internal.SdkInternalList(notifications);
}
/**
*
* The Amazon Resource Name (ARN) of an SNS topic where notifications are sent when this OpsItem is edited or
* changed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNotifications(java.util.Collection)} or {@link #withNotifications(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param notifications
* The Amazon Resource Name (ARN) of an SNS topic where notifications are sent when this OpsItem is edited or
* changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withNotifications(OpsItemNotification... notifications) {
if (this.notifications == null) {
setNotifications(new com.amazonaws.internal.SdkInternalList(notifications.length));
}
for (OpsItemNotification ele : notifications) {
this.notifications.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an SNS topic where notifications are sent when this OpsItem is edited or
* changed.
*
*
* @param notifications
* The Amazon Resource Name (ARN) of an SNS topic where notifications are sent when this OpsItem is edited or
* changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withNotifications(java.util.Collection notifications) {
setNotifications(notifications);
return this;
}
/**
*
* The importance of this OpsItem in relation to other OpsItems in the system.
*
*
* @param priority
* The importance of this OpsItem in relation to other OpsItems in the system.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* The importance of this OpsItem in relation to other OpsItems in the system.
*
*
* @return The importance of this OpsItem in relation to other OpsItems in the system.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* The importance of this OpsItem in relation to other OpsItems in the system.
*
*
* @param priority
* The importance of this OpsItem in relation to other OpsItems in the system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* One or more OpsItems that share something in common with the current OpsItems. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*
* @return One or more OpsItems that share something in common with the current OpsItems. For example, related
* OpsItems can include OpsItems with similar error messages, impacted resources, or statuses for the
* impacted resource.
*/
public java.util.List getRelatedOpsItems() {
if (relatedOpsItems == null) {
relatedOpsItems = new com.amazonaws.internal.SdkInternalList();
}
return relatedOpsItems;
}
/**
*
* One or more OpsItems that share something in common with the current OpsItems. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*
* @param relatedOpsItems
* One or more OpsItems that share something in common with the current OpsItems. For example, related
* OpsItems can include OpsItems with similar error messages, impacted resources, or statuses for the
* impacted resource.
*/
public void setRelatedOpsItems(java.util.Collection relatedOpsItems) {
if (relatedOpsItems == null) {
this.relatedOpsItems = null;
return;
}
this.relatedOpsItems = new com.amazonaws.internal.SdkInternalList(relatedOpsItems);
}
/**
*
* One or more OpsItems that share something in common with the current OpsItems. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRelatedOpsItems(java.util.Collection)} or {@link #withRelatedOpsItems(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param relatedOpsItems
* One or more OpsItems that share something in common with the current OpsItems. For example, related
* OpsItems can include OpsItems with similar error messages, impacted resources, or statuses for the
* impacted resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withRelatedOpsItems(RelatedOpsItem... relatedOpsItems) {
if (this.relatedOpsItems == null) {
setRelatedOpsItems(new com.amazonaws.internal.SdkInternalList(relatedOpsItems.length));
}
for (RelatedOpsItem ele : relatedOpsItems) {
this.relatedOpsItems.add(ele);
}
return this;
}
/**
*
* One or more OpsItems that share something in common with the current OpsItems. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*
* @param relatedOpsItems
* One or more OpsItems that share something in common with the current OpsItems. For example, related
* OpsItems can include OpsItems with similar error messages, impacted resources, or statuses for the
* impacted resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withRelatedOpsItems(java.util.Collection relatedOpsItems) {
setRelatedOpsItems(relatedOpsItems);
return this;
}
/**
*
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager.
*
*
*
* The source name can't contain the following strings: aws
, amazon
, and amzn
* .
*
*
*
* @param source
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager.
*
* The source name can't contain the following strings: aws
, amazon
, and
* amzn
.
*
*/
public void setSource(String source) {
this.source = source;
}
/**
*
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager.
*
*
*
* The source name can't contain the following strings: aws
, amazon
, and amzn
* .
*
*
*
* @return The origin of the OpsItem, such as Amazon EC2 or Systems Manager.
*
* The source name can't contain the following strings: aws
, amazon
, and
* amzn
.
*
*/
public String getSource() {
return this.source;
}
/**
*
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager.
*
*
*
* The source name can't contain the following strings: aws
, amazon
, and amzn
* .
*
*
*
* @param source
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager.
*
* The source name can't contain the following strings: aws
, amazon
, and
* amzn
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withSource(String source) {
setSource(source);
return this;
}
/**
*
* A short heading that describes the nature of the OpsItem and the impacted resource.
*
*
* @param title
* A short heading that describes the nature of the OpsItem and the impacted resource.
*/
public void setTitle(String title) {
this.title = title;
}
/**
*
* A short heading that describes the nature of the OpsItem and the impacted resource.
*
*
* @return A short heading that describes the nature of the OpsItem and the impacted resource.
*/
public String getTitle() {
return this.title;
}
/**
*
* A short heading that describes the nature of the OpsItem and the impacted resource.
*
*
* @param title
* A short heading that describes the nature of the OpsItem and the impacted resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withTitle(String title) {
setTitle(title);
return this;
}
/**
*
* Optional metadata that you assign to a resource.
*
*
* Tags use a key-value pair. For example:
*
*
* Key=Department,Value=Finance
*
*
*
* To add tags to a new OpsItem, a user must have IAM permissions for both the ssm:CreateOpsItems
* operation and the ssm:AddTagsToResource
operation. To add tags to an existing OpsItem, use the
* AddTagsToResource operation.
*
*
*
* @return Optional metadata that you assign to a resource.
*
* Tags use a key-value pair. For example:
*
*
* Key=Department,Value=Finance
*
*
*
* To add tags to a new OpsItem, a user must have IAM permissions for both the
* ssm:CreateOpsItems
operation and the ssm:AddTagsToResource
operation. To add
* tags to an existing OpsItem, use the AddTagsToResource operation.
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* Optional metadata that you assign to a resource.
*
*
* Tags use a key-value pair. For example:
*
*
* Key=Department,Value=Finance
*
*
*
* To add tags to a new OpsItem, a user must have IAM permissions for both the ssm:CreateOpsItems
* operation and the ssm:AddTagsToResource
operation. To add tags to an existing OpsItem, use the
* AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource.
*
* Tags use a key-value pair. For example:
*
*
* Key=Department,Value=Finance
*
*
*
* To add tags to a new OpsItem, a user must have IAM permissions for both the
* ssm:CreateOpsItems
operation and the ssm:AddTagsToResource
operation. To add
* tags to an existing OpsItem, use the AddTagsToResource operation.
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* Optional metadata that you assign to a resource.
*
*
* Tags use a key-value pair. For example:
*
*
* Key=Department,Value=Finance
*
*
*
* To add tags to a new OpsItem, a user must have IAM permissions for both the ssm:CreateOpsItems
* operation and the ssm:AddTagsToResource
operation. To add tags to an existing OpsItem, use the
* AddTagsToResource operation.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Optional metadata that you assign to a resource.
*
* Tags use a key-value pair. For example:
*
*
* Key=Department,Value=Finance
*
*
*
* To add tags to a new OpsItem, a user must have IAM permissions for both the
* ssm:CreateOpsItems
operation and the ssm:AddTagsToResource
operation. To add
* tags to an existing OpsItem, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Optional metadata that you assign to a resource.
*
*
* Tags use a key-value pair. For example:
*
*
* Key=Department,Value=Finance
*
*
*
* To add tags to a new OpsItem, a user must have IAM permissions for both the ssm:CreateOpsItems
* operation and the ssm:AddTagsToResource
operation. To add tags to an existing OpsItem, use the
* AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource.
*
* Tags use a key-value pair. For example:
*
*
* Key=Department,Value=Finance
*
*
*
* To add tags to a new OpsItem, a user must have IAM permissions for both the
* ssm:CreateOpsItems
operation and the ssm:AddTagsToResource
operation. To add
* tags to an existing OpsItem, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Specify a category to assign to an OpsItem.
*
*
* @param category
* Specify a category to assign to an OpsItem.
*/
public void setCategory(String category) {
this.category = category;
}
/**
*
* Specify a category to assign to an OpsItem.
*
*
* @return Specify a category to assign to an OpsItem.
*/
public String getCategory() {
return this.category;
}
/**
*
* Specify a category to assign to an OpsItem.
*
*
* @param category
* Specify a category to assign to an OpsItem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withCategory(String category) {
setCategory(category);
return this;
}
/**
*
* Specify a severity to assign to an OpsItem.
*
*
* @param severity
* Specify a severity to assign to an OpsItem.
*/
public void setSeverity(String severity) {
this.severity = severity;
}
/**
*
* Specify a severity to assign to an OpsItem.
*
*
* @return Specify a severity to assign to an OpsItem.
*/
public String getSeverity() {
return this.severity;
}
/**
*
* Specify a severity to assign to an OpsItem.
*
*
* @param severity
* Specify a severity to assign to an OpsItem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withSeverity(String severity) {
setSeverity(severity);
return this;
}
/**
*
* The time a runbook workflow started. Currently reported only for the OpsItem type /aws/changerequest
* .
*
*
* @param actualStartTime
* The time a runbook workflow started. Currently reported only for the OpsItem type
* /aws/changerequest
.
*/
public void setActualStartTime(java.util.Date actualStartTime) {
this.actualStartTime = actualStartTime;
}
/**
*
* The time a runbook workflow started. Currently reported only for the OpsItem type /aws/changerequest
* .
*
*
* @return The time a runbook workflow started. Currently reported only for the OpsItem type
* /aws/changerequest
.
*/
public java.util.Date getActualStartTime() {
return this.actualStartTime;
}
/**
*
* The time a runbook workflow started. Currently reported only for the OpsItem type /aws/changerequest
* .
*
*
* @param actualStartTime
* The time a runbook workflow started. Currently reported only for the OpsItem type
* /aws/changerequest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withActualStartTime(java.util.Date actualStartTime) {
setActualStartTime(actualStartTime);
return this;
}
/**
*
* The time a runbook workflow ended. Currently reported only for the OpsItem type /aws/changerequest
.
*
*
* @param actualEndTime
* The time a runbook workflow ended. Currently reported only for the OpsItem type
* /aws/changerequest
.
*/
public void setActualEndTime(java.util.Date actualEndTime) {
this.actualEndTime = actualEndTime;
}
/**
*
* The time a runbook workflow ended. Currently reported only for the OpsItem type /aws/changerequest
.
*
*
* @return The time a runbook workflow ended. Currently reported only for the OpsItem type
* /aws/changerequest
.
*/
public java.util.Date getActualEndTime() {
return this.actualEndTime;
}
/**
*
* The time a runbook workflow ended. Currently reported only for the OpsItem type /aws/changerequest
.
*
*
* @param actualEndTime
* The time a runbook workflow ended. Currently reported only for the OpsItem type
* /aws/changerequest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withActualEndTime(java.util.Date actualEndTime) {
setActualEndTime(actualEndTime);
return this;
}
/**
*
* The time specified in a change request for a runbook workflow to start. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @param plannedStartTime
* The time specified in a change request for a runbook workflow to start. Currently supported only for the
* OpsItem type /aws/changerequest
.
*/
public void setPlannedStartTime(java.util.Date plannedStartTime) {
this.plannedStartTime = plannedStartTime;
}
/**
*
* The time specified in a change request for a runbook workflow to start. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @return The time specified in a change request for a runbook workflow to start. Currently supported only for the
* OpsItem type /aws/changerequest
.
*/
public java.util.Date getPlannedStartTime() {
return this.plannedStartTime;
}
/**
*
* The time specified in a change request for a runbook workflow to start. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @param plannedStartTime
* The time specified in a change request for a runbook workflow to start. Currently supported only for the
* OpsItem type /aws/changerequest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withPlannedStartTime(java.util.Date plannedStartTime) {
setPlannedStartTime(plannedStartTime);
return this;
}
/**
*
* The time specified in a change request for a runbook workflow to end. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @param plannedEndTime
* The time specified in a change request for a runbook workflow to end. Currently supported only for the
* OpsItem type /aws/changerequest
.
*/
public void setPlannedEndTime(java.util.Date plannedEndTime) {
this.plannedEndTime = plannedEndTime;
}
/**
*
* The time specified in a change request for a runbook workflow to end. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @return The time specified in a change request for a runbook workflow to end. Currently supported only for the
* OpsItem type /aws/changerequest
.
*/
public java.util.Date getPlannedEndTime() {
return this.plannedEndTime;
}
/**
*
* The time specified in a change request for a runbook workflow to end. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @param plannedEndTime
* The time specified in a change request for a runbook workflow to end. Currently supported only for the
* OpsItem type /aws/changerequest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withPlannedEndTime(java.util.Date plannedEndTime) {
setPlannedEndTime(plannedEndTime);
return this;
}
/**
*
* The target Amazon Web Services account where you want to create an OpsItem. To make this call, your account must
* be configured to work with OpsItems across accounts. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* @param accountId
* The target Amazon Web Services account where you want to create an OpsItem. To make this call, your
* account must be configured to work with OpsItems across accounts. For more information, see Set up
* OpsCenter in the Amazon Web Services Systems Manager User Guide.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The target Amazon Web Services account where you want to create an OpsItem. To make this call, your account must
* be configured to work with OpsItems across accounts. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* @return The target Amazon Web Services account where you want to create an OpsItem. To make this call, your
* account must be configured to work with OpsItems across accounts. For more information, see Set up
* OpsCenter in the Amazon Web Services Systems Manager User Guide.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The target Amazon Web Services account where you want to create an OpsItem. To make this call, your account must
* be configured to work with OpsItems across accounts. For more information, see Set up OpsCenter in
* the Amazon Web Services Systems Manager User Guide.
*
*
* @param accountId
* The target Amazon Web Services account where you want to create an OpsItem. To make this call, your
* account must be configured to work with OpsItems across accounts. For more information, see Set up
* OpsCenter in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateOpsItemRequest withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getOpsItemType() != null)
sb.append("OpsItemType: ").append(getOpsItemType()).append(",");
if (getOperationalData() != null)
sb.append("OperationalData: ").append(getOperationalData()).append(",");
if (getNotifications() != null)
sb.append("Notifications: ").append(getNotifications()).append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getRelatedOpsItems() != null)
sb.append("RelatedOpsItems: ").append(getRelatedOpsItems()).append(",");
if (getSource() != null)
sb.append("Source: ").append(getSource()).append(",");
if (getTitle() != null)
sb.append("Title: ").append(getTitle()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCategory() != null)
sb.append("Category: ").append(getCategory()).append(",");
if (getSeverity() != null)
sb.append("Severity: ").append(getSeverity()).append(",");
if (getActualStartTime() != null)
sb.append("ActualStartTime: ").append(getActualStartTime()).append(",");
if (getActualEndTime() != null)
sb.append("ActualEndTime: ").append(getActualEndTime()).append(",");
if (getPlannedStartTime() != null)
sb.append("PlannedStartTime: ").append(getPlannedStartTime()).append(",");
if (getPlannedEndTime() != null)
sb.append("PlannedEndTime: ").append(getPlannedEndTime()).append(",");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateOpsItemRequest == false)
return false;
CreateOpsItemRequest other = (CreateOpsItemRequest) obj;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getOpsItemType() == null ^ this.getOpsItemType() == null)
return false;
if (other.getOpsItemType() != null && other.getOpsItemType().equals(this.getOpsItemType()) == false)
return false;
if (other.getOperationalData() == null ^ this.getOperationalData() == null)
return false;
if (other.getOperationalData() != null && other.getOperationalData().equals(this.getOperationalData()) == false)
return false;
if (other.getNotifications() == null ^ this.getNotifications() == null)
return false;
if (other.getNotifications() != null && other.getNotifications().equals(this.getNotifications()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getRelatedOpsItems() == null ^ this.getRelatedOpsItems() == null)
return false;
if (other.getRelatedOpsItems() != null && other.getRelatedOpsItems().equals(this.getRelatedOpsItems()) == false)
return false;
if (other.getSource() == null ^ this.getSource() == null)
return false;
if (other.getSource() != null && other.getSource().equals(this.getSource()) == false)
return false;
if (other.getTitle() == null ^ this.getTitle() == null)
return false;
if (other.getTitle() != null && other.getTitle().equals(this.getTitle()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCategory() == null ^ this.getCategory() == null)
return false;
if (other.getCategory() != null && other.getCategory().equals(this.getCategory()) == false)
return false;
if (other.getSeverity() == null ^ this.getSeverity() == null)
return false;
if (other.getSeverity() != null && other.getSeverity().equals(this.getSeverity()) == false)
return false;
if (other.getActualStartTime() == null ^ this.getActualStartTime() == null)
return false;
if (other.getActualStartTime() != null && other.getActualStartTime().equals(this.getActualStartTime()) == false)
return false;
if (other.getActualEndTime() == null ^ this.getActualEndTime() == null)
return false;
if (other.getActualEndTime() != null && other.getActualEndTime().equals(this.getActualEndTime()) == false)
return false;
if (other.getPlannedStartTime() == null ^ this.getPlannedStartTime() == null)
return false;
if (other.getPlannedStartTime() != null && other.getPlannedStartTime().equals(this.getPlannedStartTime()) == false)
return false;
if (other.getPlannedEndTime() == null ^ this.getPlannedEndTime() == null)
return false;
if (other.getPlannedEndTime() != null && other.getPlannedEndTime().equals(this.getPlannedEndTime()) == false)
return false;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getOpsItemType() == null) ? 0 : getOpsItemType().hashCode());
hashCode = prime * hashCode + ((getOperationalData() == null) ? 0 : getOperationalData().hashCode());
hashCode = prime * hashCode + ((getNotifications() == null) ? 0 : getNotifications().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getRelatedOpsItems() == null) ? 0 : getRelatedOpsItems().hashCode());
hashCode = prime * hashCode + ((getSource() == null) ? 0 : getSource().hashCode());
hashCode = prime * hashCode + ((getTitle() == null) ? 0 : getTitle().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCategory() == null) ? 0 : getCategory().hashCode());
hashCode = prime * hashCode + ((getSeverity() == null) ? 0 : getSeverity().hashCode());
hashCode = prime * hashCode + ((getActualStartTime() == null) ? 0 : getActualStartTime().hashCode());
hashCode = prime * hashCode + ((getActualEndTime() == null) ? 0 : getActualEndTime().hashCode());
hashCode = prime * hashCode + ((getPlannedStartTime() == null) ? 0 : getPlannedStartTime().hashCode());
hashCode = prime * hashCode + ((getPlannedEndTime() == null) ? 0 : getPlannedEndTime().hashCode());
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
return hashCode;
}
@Override
public CreateOpsItemRequest clone() {
return (CreateOpsItemRequest) super.clone();
}
}