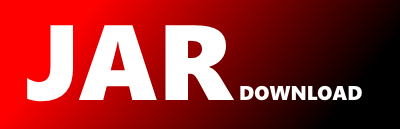
com.amazonaws.services.simplesystemsmanagement.model.GetCommandInvocationResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetCommandInvocationResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The parent command ID of the invocation plugin.
*
*/
private String commandId;
/**
*
* The ID of the managed node targeted by the command. A managed node can be an Amazon Elastic Compute Cloud
* (Amazon EC2) instance, edge device, or on-premises server or VM in your hybrid environment that is configured for
* Amazon Web Services Systems Manager.
*
*/
private String instanceId;
/**
*
* The comment text for the command.
*
*/
private String comment;
/**
*
* The name of the document that was run. For example, AWS-RunShellScript
.
*
*/
private String documentName;
/**
*
* The Systems Manager document (SSM document) version used in the request.
*
*/
private String documentVersion;
/**
*
* The name of the plugin, or step name, for which details are reported. For example,
* aws:RunShellScript
is a plugin.
*
*/
private String pluginName;
/**
*
* The error level response code for the plugin script. If the response code is -1
, then the command
* hasn't started running on the managed node, or it wasn't received by the node.
*
*/
private Integer responseCode;
/**
*
* The date and time the plugin started running. Date and time are written in ISO 8601 format. For example, June 7,
* 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedBefore
filter.
*
*
* aws ssm list-commands --filters key=InvokedBefore,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*
*/
private String executionStartDateTime;
/**
*
* Duration since ExecutionStartDateTime
.
*
*/
private String executionElapsedTime;
/**
*
* The date and time the plugin finished running. Date and time are written in ISO 8601 format. For example, June 7,
* 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedAfter
filter.
*
*
* aws ssm list-commands --filters key=InvokedAfter,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*
*/
private String executionEndDateTime;
/**
*
* The status of this invocation plugin. This status can be different than StatusDetails
.
*
*/
private String status;
/**
*
* A detailed status of the command execution for an invocation. StatusDetails
includes more
* information than Status
because it includes states resulting from error and concurrency control
* parameters. StatusDetails
can show different results than Status
. For more information
* about these statuses, see Understanding command
* statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails
can be one
* of the following values:
*
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the target, but the target wasn't available. The managed
* node might not be available because of network issues, because the node was stopped, or for similar reasons. The
* system will try to send the command again.
*
*
* -
*
* Success: The command or plugin ran successfully. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do contribute
* to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The command started to run on the managed node, but the execution wasn't complete before the
* timeout expired. Execution timeouts count against the MaxErrors
limit of the parent command. This is
* a terminal state.
*
*
* -
*
* Failed: The command wasn't run successfully on the managed node. For a plugin, this indicates that the result
* code wasn't zero. For a command invocation, this indicates that the result code for one or more plugins wasn't
* zero. Invocation failures count against the MaxErrors
limit of the parent command. This is a
* terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The node might not exist or might not be
* responding. Undeliverable invocations don't count against the parent command's MaxErrors
limit and
* don't contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors
limit and subsequent command invocations were
* canceled by the system. This is a terminal state.
*
*
*
*/
private String statusDetails;
/**
*
* The first 24,000 characters written by the plugin to stdout
. If the command hasn't finished running,
* if ExecutionStatus
is neither Succeeded nor Failed, then this string is empty.
*
*/
private String standardOutputContent;
/**
*
* The URL for the complete text written by the plugin to stdout
in Amazon Simple Storage Service
* (Amazon S3). If an S3 bucket wasn't specified, then this string is empty.
*
*/
private String standardOutputUrl;
/**
*
* The first 8,000 characters written by the plugin to stderr
. If the command hasn't finished running,
* then this string is empty.
*
*/
private String standardErrorContent;
/**
*
* The URL for the complete text written by the plugin to stderr
. If the command hasn't finished
* running, then this string is empty.
*
*/
private String standardErrorUrl;
/**
*
* Amazon CloudWatch Logs information where Systems Manager sent the command output.
*
*/
private CloudWatchOutputConfig cloudWatchOutputConfig;
/**
*
* The parent command ID of the invocation plugin.
*
*
* @param commandId
* The parent command ID of the invocation plugin.
*/
public void setCommandId(String commandId) {
this.commandId = commandId;
}
/**
*
* The parent command ID of the invocation plugin.
*
*
* @return The parent command ID of the invocation plugin.
*/
public String getCommandId() {
return this.commandId;
}
/**
*
* The parent command ID of the invocation plugin.
*
*
* @param commandId
* The parent command ID of the invocation plugin.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withCommandId(String commandId) {
setCommandId(commandId);
return this;
}
/**
*
* The ID of the managed node targeted by the command. A managed node can be an Amazon Elastic Compute Cloud
* (Amazon EC2) instance, edge device, or on-premises server or VM in your hybrid environment that is configured for
* Amazon Web Services Systems Manager.
*
*
* @param instanceId
* The ID of the managed node targeted by the command. A managed node can be an Amazon Elastic Compute
* Cloud (Amazon EC2) instance, edge device, or on-premises server or VM in your hybrid environment that is
* configured for Amazon Web Services Systems Manager.
*/
public void setInstanceId(String instanceId) {
this.instanceId = instanceId;
}
/**
*
* The ID of the managed node targeted by the command. A managed node can be an Amazon Elastic Compute Cloud
* (Amazon EC2) instance, edge device, or on-premises server or VM in your hybrid environment that is configured for
* Amazon Web Services Systems Manager.
*
*
* @return The ID of the managed node targeted by the command. A managed node can be an Amazon Elastic
* Compute Cloud (Amazon EC2) instance, edge device, or on-premises server or VM in your hybrid environment
* that is configured for Amazon Web Services Systems Manager.
*/
public String getInstanceId() {
return this.instanceId;
}
/**
*
* The ID of the managed node targeted by the command. A managed node can be an Amazon Elastic Compute Cloud
* (Amazon EC2) instance, edge device, or on-premises server or VM in your hybrid environment that is configured for
* Amazon Web Services Systems Manager.
*
*
* @param instanceId
* The ID of the managed node targeted by the command. A managed node can be an Amazon Elastic Compute
* Cloud (Amazon EC2) instance, edge device, or on-premises server or VM in your hybrid environment that is
* configured for Amazon Web Services Systems Manager.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withInstanceId(String instanceId) {
setInstanceId(instanceId);
return this;
}
/**
*
* The comment text for the command.
*
*
* @param comment
* The comment text for the command.
*/
public void setComment(String comment) {
this.comment = comment;
}
/**
*
* The comment text for the command.
*
*
* @return The comment text for the command.
*/
public String getComment() {
return this.comment;
}
/**
*
* The comment text for the command.
*
*
* @param comment
* The comment text for the command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withComment(String comment) {
setComment(comment);
return this;
}
/**
*
* The name of the document that was run. For example, AWS-RunShellScript
.
*
*
* @param documentName
* The name of the document that was run. For example, AWS-RunShellScript
.
*/
public void setDocumentName(String documentName) {
this.documentName = documentName;
}
/**
*
* The name of the document that was run. For example, AWS-RunShellScript
.
*
*
* @return The name of the document that was run. For example, AWS-RunShellScript
.
*/
public String getDocumentName() {
return this.documentName;
}
/**
*
* The name of the document that was run. For example, AWS-RunShellScript
.
*
*
* @param documentName
* The name of the document that was run. For example, AWS-RunShellScript
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withDocumentName(String documentName) {
setDocumentName(documentName);
return this;
}
/**
*
* The Systems Manager document (SSM document) version used in the request.
*
*
* @param documentVersion
* The Systems Manager document (SSM document) version used in the request.
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The Systems Manager document (SSM document) version used in the request.
*
*
* @return The Systems Manager document (SSM document) version used in the request.
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The Systems Manager document (SSM document) version used in the request.
*
*
* @param documentVersion
* The Systems Manager document (SSM document) version used in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* The name of the plugin, or step name, for which details are reported. For example,
* aws:RunShellScript
is a plugin.
*
*
* @param pluginName
* The name of the plugin, or step name, for which details are reported. For example,
* aws:RunShellScript
is a plugin.
*/
public void setPluginName(String pluginName) {
this.pluginName = pluginName;
}
/**
*
* The name of the plugin, or step name, for which details are reported. For example,
* aws:RunShellScript
is a plugin.
*
*
* @return The name of the plugin, or step name, for which details are reported. For example,
* aws:RunShellScript
is a plugin.
*/
public String getPluginName() {
return this.pluginName;
}
/**
*
* The name of the plugin, or step name, for which details are reported. For example,
* aws:RunShellScript
is a plugin.
*
*
* @param pluginName
* The name of the plugin, or step name, for which details are reported. For example,
* aws:RunShellScript
is a plugin.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withPluginName(String pluginName) {
setPluginName(pluginName);
return this;
}
/**
*
* The error level response code for the plugin script. If the response code is -1
, then the command
* hasn't started running on the managed node, or it wasn't received by the node.
*
*
* @param responseCode
* The error level response code for the plugin script. If the response code is -1
, then the
* command hasn't started running on the managed node, or it wasn't received by the node.
*/
public void setResponseCode(Integer responseCode) {
this.responseCode = responseCode;
}
/**
*
* The error level response code for the plugin script. If the response code is -1
, then the command
* hasn't started running on the managed node, or it wasn't received by the node.
*
*
* @return The error level response code for the plugin script. If the response code is -1
, then the
* command hasn't started running on the managed node, or it wasn't received by the node.
*/
public Integer getResponseCode() {
return this.responseCode;
}
/**
*
* The error level response code for the plugin script. If the response code is -1
, then the command
* hasn't started running on the managed node, or it wasn't received by the node.
*
*
* @param responseCode
* The error level response code for the plugin script. If the response code is -1
, then the
* command hasn't started running on the managed node, or it wasn't received by the node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withResponseCode(Integer responseCode) {
setResponseCode(responseCode);
return this;
}
/**
*
* The date and time the plugin started running. Date and time are written in ISO 8601 format. For example, June 7,
* 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedBefore
filter.
*
*
* aws ssm list-commands --filters key=InvokedBefore,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*
*
* @param executionStartDateTime
* The date and time the plugin started running. Date and time are written in ISO 8601 format. For example,
* June 7, 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedBefore
filter.
*
* aws ssm list-commands --filters key=InvokedBefore,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*/
public void setExecutionStartDateTime(String executionStartDateTime) {
this.executionStartDateTime = executionStartDateTime;
}
/**
*
* The date and time the plugin started running. Date and time are written in ISO 8601 format. For example, June 7,
* 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedBefore
filter.
*
*
* aws ssm list-commands --filters key=InvokedBefore,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*
*
* @return The date and time the plugin started running. Date and time are written in ISO 8601 format. For example,
* June 7, 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedBefore
filter.
*
* aws ssm list-commands --filters key=InvokedBefore,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*/
public String getExecutionStartDateTime() {
return this.executionStartDateTime;
}
/**
*
* The date and time the plugin started running. Date and time are written in ISO 8601 format. For example, June 7,
* 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedBefore
filter.
*
*
* aws ssm list-commands --filters key=InvokedBefore,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*
*
* @param executionStartDateTime
* The date and time the plugin started running. Date and time are written in ISO 8601 format. For example,
* June 7, 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedBefore
filter.
*
* aws ssm list-commands --filters key=InvokedBefore,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withExecutionStartDateTime(String executionStartDateTime) {
setExecutionStartDateTime(executionStartDateTime);
return this;
}
/**
*
* Duration since ExecutionStartDateTime
.
*
*
* @param executionElapsedTime
* Duration since ExecutionStartDateTime
.
*/
public void setExecutionElapsedTime(String executionElapsedTime) {
this.executionElapsedTime = executionElapsedTime;
}
/**
*
* Duration since ExecutionStartDateTime
.
*
*
* @return Duration since ExecutionStartDateTime
.
*/
public String getExecutionElapsedTime() {
return this.executionElapsedTime;
}
/**
*
* Duration since ExecutionStartDateTime
.
*
*
* @param executionElapsedTime
* Duration since ExecutionStartDateTime
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withExecutionElapsedTime(String executionElapsedTime) {
setExecutionElapsedTime(executionElapsedTime);
return this;
}
/**
*
* The date and time the plugin finished running. Date and time are written in ISO 8601 format. For example, June 7,
* 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedAfter
filter.
*
*
* aws ssm list-commands --filters key=InvokedAfter,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*
*
* @param executionEndDateTime
* The date and time the plugin finished running. Date and time are written in ISO 8601 format. For example,
* June 7, 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedAfter
filter.
*
* aws ssm list-commands --filters key=InvokedAfter,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*/
public void setExecutionEndDateTime(String executionEndDateTime) {
this.executionEndDateTime = executionEndDateTime;
}
/**
*
* The date and time the plugin finished running. Date and time are written in ISO 8601 format. For example, June 7,
* 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedAfter
filter.
*
*
* aws ssm list-commands --filters key=InvokedAfter,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*
*
* @return The date and time the plugin finished running. Date and time are written in ISO 8601 format. For example,
* June 7, 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedAfter
filter.
*
* aws ssm list-commands --filters key=InvokedAfter,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*/
public String getExecutionEndDateTime() {
return this.executionEndDateTime;
}
/**
*
* The date and time the plugin finished running. Date and time are written in ISO 8601 format. For example, June 7,
* 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedAfter
filter.
*
*
* aws ssm list-commands --filters key=InvokedAfter,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
*
*
* @param executionEndDateTime
* The date and time the plugin finished running. Date and time are written in ISO 8601 format. For example,
* June 7, 2017 is represented as 2017-06-7. The following sample Amazon Web Services CLI command uses the
* InvokedAfter
filter.
*
* aws ssm list-commands --filters key=InvokedAfter,value=2017-06-07T00:00:00Z
*
*
* If the plugin hasn't started to run, the string is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withExecutionEndDateTime(String executionEndDateTime) {
setExecutionEndDateTime(executionEndDateTime);
return this;
}
/**
*
* The status of this invocation plugin. This status can be different than StatusDetails
.
*
*
* @param status
* The status of this invocation plugin. This status can be different than StatusDetails
.
* @see CommandInvocationStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of this invocation plugin. This status can be different than StatusDetails
.
*
*
* @return The status of this invocation plugin. This status can be different than StatusDetails
.
* @see CommandInvocationStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of this invocation plugin. This status can be different than StatusDetails
.
*
*
* @param status
* The status of this invocation plugin. This status can be different than StatusDetails
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CommandInvocationStatus
*/
public GetCommandInvocationResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of this invocation plugin. This status can be different than StatusDetails
.
*
*
* @param status
* The status of this invocation plugin. This status can be different than StatusDetails
.
* @see CommandInvocationStatus
*/
public void setStatus(CommandInvocationStatus status) {
withStatus(status);
}
/**
*
* The status of this invocation plugin. This status can be different than StatusDetails
.
*
*
* @param status
* The status of this invocation plugin. This status can be different than StatusDetails
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CommandInvocationStatus
*/
public GetCommandInvocationResult withStatus(CommandInvocationStatus status) {
this.status = status.toString();
return this;
}
/**
*
* A detailed status of the command execution for an invocation. StatusDetails
includes more
* information than Status
because it includes states resulting from error and concurrency control
* parameters. StatusDetails
can show different results than Status
. For more information
* about these statuses, see Understanding command
* statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails
can be one
* of the following values:
*
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the target, but the target wasn't available. The managed
* node might not be available because of network issues, because the node was stopped, or for similar reasons. The
* system will try to send the command again.
*
*
* -
*
* Success: The command or plugin ran successfully. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do contribute
* to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The command started to run on the managed node, but the execution wasn't complete before the
* timeout expired. Execution timeouts count against the MaxErrors
limit of the parent command. This is
* a terminal state.
*
*
* -
*
* Failed: The command wasn't run successfully on the managed node. For a plugin, this indicates that the result
* code wasn't zero. For a command invocation, this indicates that the result code for one or more plugins wasn't
* zero. Invocation failures count against the MaxErrors
limit of the parent command. This is a
* terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The node might not exist or might not be
* responding. Undeliverable invocations don't count against the parent command's MaxErrors
limit and
* don't contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors
limit and subsequent command invocations were
* canceled by the system. This is a terminal state.
*
*
*
*
* @param statusDetails
* A detailed status of the command execution for an invocation. StatusDetails
includes more
* information than Status
because it includes states resulting from error and concurrency
* control parameters. StatusDetails
can show different results than Status
. For
* more information about these statuses, see Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide.
* StatusDetails
can be one of the following values:
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the target, but the target wasn't available. The
* managed node might not be available because of network issues, because the node was stopped, or for
* similar reasons. The system will try to send the command again.
*
*
* -
*
* Success: The command or plugin ran successfully. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do
* contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The command started to run on the managed node, but the execution wasn't complete
* before the timeout expired. Execution timeouts count against the MaxErrors
limit of the
* parent command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't run successfully on the managed node. For a plugin, this indicates that the
* result code wasn't zero. For a command invocation, this indicates that the result code for one or more
* plugins wasn't zero. Invocation failures count against the MaxErrors
limit of the parent
* command. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The node might not exist or might not
* be responding. Undeliverable invocations don't count against the parent command's MaxErrors
* limit and don't contribute to whether the parent command status is Success or Incomplete. This is a
* terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors
limit and subsequent command
* invocations were canceled by the system. This is a terminal state.
*
*
*/
public void setStatusDetails(String statusDetails) {
this.statusDetails = statusDetails;
}
/**
*
* A detailed status of the command execution for an invocation. StatusDetails
includes more
* information than Status
because it includes states resulting from error and concurrency control
* parameters. StatusDetails
can show different results than Status
. For more information
* about these statuses, see Understanding command
* statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails
can be one
* of the following values:
*
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the target, but the target wasn't available. The managed
* node might not be available because of network issues, because the node was stopped, or for similar reasons. The
* system will try to send the command again.
*
*
* -
*
* Success: The command or plugin ran successfully. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do contribute
* to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The command started to run on the managed node, but the execution wasn't complete before the
* timeout expired. Execution timeouts count against the MaxErrors
limit of the parent command. This is
* a terminal state.
*
*
* -
*
* Failed: The command wasn't run successfully on the managed node. For a plugin, this indicates that the result
* code wasn't zero. For a command invocation, this indicates that the result code for one or more plugins wasn't
* zero. Invocation failures count against the MaxErrors
limit of the parent command. This is a
* terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The node might not exist or might not be
* responding. Undeliverable invocations don't count against the parent command's MaxErrors
limit and
* don't contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors
limit and subsequent command invocations were
* canceled by the system. This is a terminal state.
*
*
*
*
* @return A detailed status of the command execution for an invocation. StatusDetails
includes more
* information than Status
because it includes states resulting from error and concurrency
* control parameters. StatusDetails
can show different results than Status
. For
* more information about these statuses, see Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide.
* StatusDetails
can be one of the following values:
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the target, but the target wasn't available. The
* managed node might not be available because of network issues, because the node was stopped, or for
* similar reasons. The system will try to send the command again.
*
*
* -
*
* Success: The command or plugin ran successfully. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do
* contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The command started to run on the managed node, but the execution wasn't complete
* before the timeout expired. Execution timeouts count against the MaxErrors
limit of the
* parent command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't run successfully on the managed node. For a plugin, this indicates that the
* result code wasn't zero. For a command invocation, this indicates that the result code for one or more
* plugins wasn't zero. Invocation failures count against the MaxErrors
limit of the parent
* command. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The node might not exist or might not
* be responding. Undeliverable invocations don't count against the parent command's MaxErrors
* limit and don't contribute to whether the parent command status is Success or Incomplete. This is a
* terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors
limit and subsequent command
* invocations were canceled by the system. This is a terminal state.
*
*
*/
public String getStatusDetails() {
return this.statusDetails;
}
/**
*
* A detailed status of the command execution for an invocation. StatusDetails
includes more
* information than Status
because it includes states resulting from error and concurrency control
* parameters. StatusDetails
can show different results than Status
. For more information
* about these statuses, see Understanding command
* statuses in the Amazon Web Services Systems Manager User Guide. StatusDetails
can be one
* of the following values:
*
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the target, but the target wasn't available. The managed
* node might not be available because of network issues, because the node was stopped, or for similar reasons. The
* system will try to send the command again.
*
*
* -
*
* Success: The command or plugin ran successfully. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do contribute
* to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The command started to run on the managed node, but the execution wasn't complete before the
* timeout expired. Execution timeouts count against the MaxErrors
limit of the parent command. This is
* a terminal state.
*
*
* -
*
* Failed: The command wasn't run successfully on the managed node. For a plugin, this indicates that the result
* code wasn't zero. For a command invocation, this indicates that the result code for one or more plugins wasn't
* zero. Invocation failures count against the MaxErrors
limit of the parent command. This is a
* terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The node might not exist or might not be
* responding. Undeliverable invocations don't count against the parent command's MaxErrors
limit and
* don't contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors
limit and subsequent command invocations were
* canceled by the system. This is a terminal state.
*
*
*
*
* @param statusDetails
* A detailed status of the command execution for an invocation. StatusDetails
includes more
* information than Status
because it includes states resulting from error and concurrency
* control parameters. StatusDetails
can show different results than Status
. For
* more information about these statuses, see Understanding
* command statuses in the Amazon Web Services Systems Manager User Guide.
* StatusDetails
can be one of the following values:
*
* -
*
* Pending: The command hasn't been sent to the managed node.
*
*
* -
*
* In Progress: The command has been sent to the managed node but hasn't reached a terminal state.
*
*
* -
*
* Delayed: The system attempted to send the command to the target, but the target wasn't available. The
* managed node might not be available because of network issues, because the node was stopped, or for
* similar reasons. The system will try to send the command again.
*
*
* -
*
* Success: The command or plugin ran successfully. This is a terminal state.
*
*
* -
*
* Delivery Timed Out: The command wasn't delivered to the managed node before the delivery timeout expired.
* Delivery timeouts don't count against the parent command's MaxErrors
limit, but they do
* contribute to whether the parent command status is Success or Incomplete. This is a terminal state.
*
*
* -
*
* Execution Timed Out: The command started to run on the managed node, but the execution wasn't complete
* before the timeout expired. Execution timeouts count against the MaxErrors
limit of the
* parent command. This is a terminal state.
*
*
* -
*
* Failed: The command wasn't run successfully on the managed node. For a plugin, this indicates that the
* result code wasn't zero. For a command invocation, this indicates that the result code for one or more
* plugins wasn't zero. Invocation failures count against the MaxErrors
limit of the parent
* command. This is a terminal state.
*
*
* -
*
* Cancelled: The command was terminated before it was completed. This is a terminal state.
*
*
* -
*
* Undeliverable: The command can't be delivered to the managed node. The node might not exist or might not
* be responding. Undeliverable invocations don't count against the parent command's MaxErrors
* limit and don't contribute to whether the parent command status is Success or Incomplete. This is a
* terminal state.
*
*
* -
*
* Terminated: The parent command exceeded its MaxErrors
limit and subsequent command
* invocations were canceled by the system. This is a terminal state.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withStatusDetails(String statusDetails) {
setStatusDetails(statusDetails);
return this;
}
/**
*
* The first 24,000 characters written by the plugin to stdout
. If the command hasn't finished running,
* if ExecutionStatus
is neither Succeeded nor Failed, then this string is empty.
*
*
* @param standardOutputContent
* The first 24,000 characters written by the plugin to stdout
. If the command hasn't finished
* running, if ExecutionStatus
is neither Succeeded nor Failed, then this string is empty.
*/
public void setStandardOutputContent(String standardOutputContent) {
this.standardOutputContent = standardOutputContent;
}
/**
*
* The first 24,000 characters written by the plugin to stdout
. If the command hasn't finished running,
* if ExecutionStatus
is neither Succeeded nor Failed, then this string is empty.
*
*
* @return The first 24,000 characters written by the plugin to stdout
. If the command hasn't finished
* running, if ExecutionStatus
is neither Succeeded nor Failed, then this string is empty.
*/
public String getStandardOutputContent() {
return this.standardOutputContent;
}
/**
*
* The first 24,000 characters written by the plugin to stdout
. If the command hasn't finished running,
* if ExecutionStatus
is neither Succeeded nor Failed, then this string is empty.
*
*
* @param standardOutputContent
* The first 24,000 characters written by the plugin to stdout
. If the command hasn't finished
* running, if ExecutionStatus
is neither Succeeded nor Failed, then this string is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withStandardOutputContent(String standardOutputContent) {
setStandardOutputContent(standardOutputContent);
return this;
}
/**
*
* The URL for the complete text written by the plugin to stdout
in Amazon Simple Storage Service
* (Amazon S3). If an S3 bucket wasn't specified, then this string is empty.
*
*
* @param standardOutputUrl
* The URL for the complete text written by the plugin to stdout
in Amazon Simple Storage
* Service (Amazon S3). If an S3 bucket wasn't specified, then this string is empty.
*/
public void setStandardOutputUrl(String standardOutputUrl) {
this.standardOutputUrl = standardOutputUrl;
}
/**
*
* The URL for the complete text written by the plugin to stdout
in Amazon Simple Storage Service
* (Amazon S3). If an S3 bucket wasn't specified, then this string is empty.
*
*
* @return The URL for the complete text written by the plugin to stdout
in Amazon Simple Storage
* Service (Amazon S3). If an S3 bucket wasn't specified, then this string is empty.
*/
public String getStandardOutputUrl() {
return this.standardOutputUrl;
}
/**
*
* The URL for the complete text written by the plugin to stdout
in Amazon Simple Storage Service
* (Amazon S3). If an S3 bucket wasn't specified, then this string is empty.
*
*
* @param standardOutputUrl
* The URL for the complete text written by the plugin to stdout
in Amazon Simple Storage
* Service (Amazon S3). If an S3 bucket wasn't specified, then this string is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withStandardOutputUrl(String standardOutputUrl) {
setStandardOutputUrl(standardOutputUrl);
return this;
}
/**
*
* The first 8,000 characters written by the plugin to stderr
. If the command hasn't finished running,
* then this string is empty.
*
*
* @param standardErrorContent
* The first 8,000 characters written by the plugin to stderr
. If the command hasn't finished
* running, then this string is empty.
*/
public void setStandardErrorContent(String standardErrorContent) {
this.standardErrorContent = standardErrorContent;
}
/**
*
* The first 8,000 characters written by the plugin to stderr
. If the command hasn't finished running,
* then this string is empty.
*
*
* @return The first 8,000 characters written by the plugin to stderr
. If the command hasn't finished
* running, then this string is empty.
*/
public String getStandardErrorContent() {
return this.standardErrorContent;
}
/**
*
* The first 8,000 characters written by the plugin to stderr
. If the command hasn't finished running,
* then this string is empty.
*
*
* @param standardErrorContent
* The first 8,000 characters written by the plugin to stderr
. If the command hasn't finished
* running, then this string is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withStandardErrorContent(String standardErrorContent) {
setStandardErrorContent(standardErrorContent);
return this;
}
/**
*
* The URL for the complete text written by the plugin to stderr
. If the command hasn't finished
* running, then this string is empty.
*
*
* @param standardErrorUrl
* The URL for the complete text written by the plugin to stderr
. If the command hasn't finished
* running, then this string is empty.
*/
public void setStandardErrorUrl(String standardErrorUrl) {
this.standardErrorUrl = standardErrorUrl;
}
/**
*
* The URL for the complete text written by the plugin to stderr
. If the command hasn't finished
* running, then this string is empty.
*
*
* @return The URL for the complete text written by the plugin to stderr
. If the command hasn't
* finished running, then this string is empty.
*/
public String getStandardErrorUrl() {
return this.standardErrorUrl;
}
/**
*
* The URL for the complete text written by the plugin to stderr
. If the command hasn't finished
* running, then this string is empty.
*
*
* @param standardErrorUrl
* The URL for the complete text written by the plugin to stderr
. If the command hasn't finished
* running, then this string is empty.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withStandardErrorUrl(String standardErrorUrl) {
setStandardErrorUrl(standardErrorUrl);
return this;
}
/**
*
* Amazon CloudWatch Logs information where Systems Manager sent the command output.
*
*
* @param cloudWatchOutputConfig
* Amazon CloudWatch Logs information where Systems Manager sent the command output.
*/
public void setCloudWatchOutputConfig(CloudWatchOutputConfig cloudWatchOutputConfig) {
this.cloudWatchOutputConfig = cloudWatchOutputConfig;
}
/**
*
* Amazon CloudWatch Logs information where Systems Manager sent the command output.
*
*
* @return Amazon CloudWatch Logs information where Systems Manager sent the command output.
*/
public CloudWatchOutputConfig getCloudWatchOutputConfig() {
return this.cloudWatchOutputConfig;
}
/**
*
* Amazon CloudWatch Logs information where Systems Manager sent the command output.
*
*
* @param cloudWatchOutputConfig
* Amazon CloudWatch Logs information where Systems Manager sent the command output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetCommandInvocationResult withCloudWatchOutputConfig(CloudWatchOutputConfig cloudWatchOutputConfig) {
setCloudWatchOutputConfig(cloudWatchOutputConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCommandId() != null)
sb.append("CommandId: ").append(getCommandId()).append(",");
if (getInstanceId() != null)
sb.append("InstanceId: ").append(getInstanceId()).append(",");
if (getComment() != null)
sb.append("Comment: ").append(getComment()).append(",");
if (getDocumentName() != null)
sb.append("DocumentName: ").append(getDocumentName()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getPluginName() != null)
sb.append("PluginName: ").append(getPluginName()).append(",");
if (getResponseCode() != null)
sb.append("ResponseCode: ").append(getResponseCode()).append(",");
if (getExecutionStartDateTime() != null)
sb.append("ExecutionStartDateTime: ").append(getExecutionStartDateTime()).append(",");
if (getExecutionElapsedTime() != null)
sb.append("ExecutionElapsedTime: ").append(getExecutionElapsedTime()).append(",");
if (getExecutionEndDateTime() != null)
sb.append("ExecutionEndDateTime: ").append(getExecutionEndDateTime()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusDetails() != null)
sb.append("StatusDetails: ").append(getStatusDetails()).append(",");
if (getStandardOutputContent() != null)
sb.append("StandardOutputContent: ").append(getStandardOutputContent()).append(",");
if (getStandardOutputUrl() != null)
sb.append("StandardOutputUrl: ").append(getStandardOutputUrl()).append(",");
if (getStandardErrorContent() != null)
sb.append("StandardErrorContent: ").append(getStandardErrorContent()).append(",");
if (getStandardErrorUrl() != null)
sb.append("StandardErrorUrl: ").append(getStandardErrorUrl()).append(",");
if (getCloudWatchOutputConfig() != null)
sb.append("CloudWatchOutputConfig: ").append(getCloudWatchOutputConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetCommandInvocationResult == false)
return false;
GetCommandInvocationResult other = (GetCommandInvocationResult) obj;
if (other.getCommandId() == null ^ this.getCommandId() == null)
return false;
if (other.getCommandId() != null && other.getCommandId().equals(this.getCommandId()) == false)
return false;
if (other.getInstanceId() == null ^ this.getInstanceId() == null)
return false;
if (other.getInstanceId() != null && other.getInstanceId().equals(this.getInstanceId()) == false)
return false;
if (other.getComment() == null ^ this.getComment() == null)
return false;
if (other.getComment() != null && other.getComment().equals(this.getComment()) == false)
return false;
if (other.getDocumentName() == null ^ this.getDocumentName() == null)
return false;
if (other.getDocumentName() != null && other.getDocumentName().equals(this.getDocumentName()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getPluginName() == null ^ this.getPluginName() == null)
return false;
if (other.getPluginName() != null && other.getPluginName().equals(this.getPluginName()) == false)
return false;
if (other.getResponseCode() == null ^ this.getResponseCode() == null)
return false;
if (other.getResponseCode() != null && other.getResponseCode().equals(this.getResponseCode()) == false)
return false;
if (other.getExecutionStartDateTime() == null ^ this.getExecutionStartDateTime() == null)
return false;
if (other.getExecutionStartDateTime() != null && other.getExecutionStartDateTime().equals(this.getExecutionStartDateTime()) == false)
return false;
if (other.getExecutionElapsedTime() == null ^ this.getExecutionElapsedTime() == null)
return false;
if (other.getExecutionElapsedTime() != null && other.getExecutionElapsedTime().equals(this.getExecutionElapsedTime()) == false)
return false;
if (other.getExecutionEndDateTime() == null ^ this.getExecutionEndDateTime() == null)
return false;
if (other.getExecutionEndDateTime() != null && other.getExecutionEndDateTime().equals(this.getExecutionEndDateTime()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusDetails() == null ^ this.getStatusDetails() == null)
return false;
if (other.getStatusDetails() != null && other.getStatusDetails().equals(this.getStatusDetails()) == false)
return false;
if (other.getStandardOutputContent() == null ^ this.getStandardOutputContent() == null)
return false;
if (other.getStandardOutputContent() != null && other.getStandardOutputContent().equals(this.getStandardOutputContent()) == false)
return false;
if (other.getStandardOutputUrl() == null ^ this.getStandardOutputUrl() == null)
return false;
if (other.getStandardOutputUrl() != null && other.getStandardOutputUrl().equals(this.getStandardOutputUrl()) == false)
return false;
if (other.getStandardErrorContent() == null ^ this.getStandardErrorContent() == null)
return false;
if (other.getStandardErrorContent() != null && other.getStandardErrorContent().equals(this.getStandardErrorContent()) == false)
return false;
if (other.getStandardErrorUrl() == null ^ this.getStandardErrorUrl() == null)
return false;
if (other.getStandardErrorUrl() != null && other.getStandardErrorUrl().equals(this.getStandardErrorUrl()) == false)
return false;
if (other.getCloudWatchOutputConfig() == null ^ this.getCloudWatchOutputConfig() == null)
return false;
if (other.getCloudWatchOutputConfig() != null && other.getCloudWatchOutputConfig().equals(this.getCloudWatchOutputConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCommandId() == null) ? 0 : getCommandId().hashCode());
hashCode = prime * hashCode + ((getInstanceId() == null) ? 0 : getInstanceId().hashCode());
hashCode = prime * hashCode + ((getComment() == null) ? 0 : getComment().hashCode());
hashCode = prime * hashCode + ((getDocumentName() == null) ? 0 : getDocumentName().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getPluginName() == null) ? 0 : getPluginName().hashCode());
hashCode = prime * hashCode + ((getResponseCode() == null) ? 0 : getResponseCode().hashCode());
hashCode = prime * hashCode + ((getExecutionStartDateTime() == null) ? 0 : getExecutionStartDateTime().hashCode());
hashCode = prime * hashCode + ((getExecutionElapsedTime() == null) ? 0 : getExecutionElapsedTime().hashCode());
hashCode = prime * hashCode + ((getExecutionEndDateTime() == null) ? 0 : getExecutionEndDateTime().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusDetails() == null) ? 0 : getStatusDetails().hashCode());
hashCode = prime * hashCode + ((getStandardOutputContent() == null) ? 0 : getStandardOutputContent().hashCode());
hashCode = prime * hashCode + ((getStandardOutputUrl() == null) ? 0 : getStandardOutputUrl().hashCode());
hashCode = prime * hashCode + ((getStandardErrorContent() == null) ? 0 : getStandardErrorContent().hashCode());
hashCode = prime * hashCode + ((getStandardErrorUrl() == null) ? 0 : getStandardErrorUrl().hashCode());
hashCode = prime * hashCode + ((getCloudWatchOutputConfig() == null) ? 0 : getCloudWatchOutputConfig().hashCode());
return hashCode;
}
@Override
public GetCommandInvocationResult clone() {
try {
return (GetCommandInvocationResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}