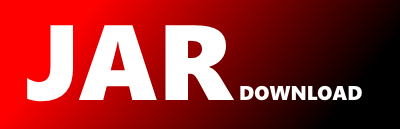
com.amazonaws.services.simplesystemsmanagement.model.GetDocumentResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetDocumentResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the SSM document.
*
*/
private String name;
/**
*
* The date the SSM document was created.
*
*/
private java.util.Date createdDate;
/**
*
* The friendly name of the SSM document. This value can differ for each version of the document. If you want to
* update this value, see UpdateDocument.
*
*/
private String displayName;
/**
*
* The version of the artifact associated with the document. For example, 12.6. This value is unique across all
* versions of a document, and can't be changed.
*
*/
private String versionName;
/**
*
* The document version.
*
*/
private String documentVersion;
/**
*
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
*
*/
private String status;
/**
*
* A message returned by Amazon Web Services Systems Manager that explains the Status
value. For
* example, a Failed
status might be explained by the StatusInformation
message,
* "The specified S3 bucket doesn't exist. Verify that the URL of the S3 bucket is correct."
*
*/
private String statusInformation;
/**
*
* The contents of the SSM document.
*
*/
private String content;
/**
*
* The document type.
*
*/
private String documentType;
/**
*
* The document format, either JSON or YAML.
*
*/
private String documentFormat;
/**
*
* A list of SSM documents required by a document. For example, an ApplicationConfiguration
document
* requires an ApplicationConfigurationSchema
document.
*
*/
private com.amazonaws.internal.SdkInternalList requires;
/**
*
* A description of the document attachments, including names, locations, sizes, and so on.
*
*/
private com.amazonaws.internal.SdkInternalList attachmentsContent;
/**
*
* The current review status of a new custom Systems Manager document (SSM document) created by a member of your
* organization, or of the latest version of an existing SSM document.
*
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is approved, the
* status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
*
*/
private String reviewStatus;
/**
*
* The name of the SSM document.
*
*
* @param name
* The name of the SSM document.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the SSM document.
*
*
* @return The name of the SSM document.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the SSM document.
*
*
* @param name
* The name of the SSM document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withName(String name) {
setName(name);
return this;
}
/**
*
* The date the SSM document was created.
*
*
* @param createdDate
* The date the SSM document was created.
*/
public void setCreatedDate(java.util.Date createdDate) {
this.createdDate = createdDate;
}
/**
*
* The date the SSM document was created.
*
*
* @return The date the SSM document was created.
*/
public java.util.Date getCreatedDate() {
return this.createdDate;
}
/**
*
* The date the SSM document was created.
*
*
* @param createdDate
* The date the SSM document was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withCreatedDate(java.util.Date createdDate) {
setCreatedDate(createdDate);
return this;
}
/**
*
* The friendly name of the SSM document. This value can differ for each version of the document. If you want to
* update this value, see UpdateDocument.
*
*
* @param displayName
* The friendly name of the SSM document. This value can differ for each version of the document. If you want
* to update this value, see UpdateDocument.
*/
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
*
* The friendly name of the SSM document. This value can differ for each version of the document. If you want to
* update this value, see UpdateDocument.
*
*
* @return The friendly name of the SSM document. This value can differ for each version of the document. If you
* want to update this value, see UpdateDocument.
*/
public String getDisplayName() {
return this.displayName;
}
/**
*
* The friendly name of the SSM document. This value can differ for each version of the document. If you want to
* update this value, see UpdateDocument.
*
*
* @param displayName
* The friendly name of the SSM document. This value can differ for each version of the document. If you want
* to update this value, see UpdateDocument.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withDisplayName(String displayName) {
setDisplayName(displayName);
return this;
}
/**
*
* The version of the artifact associated with the document. For example, 12.6. This value is unique across all
* versions of a document, and can't be changed.
*
*
* @param versionName
* The version of the artifact associated with the document. For example, 12.6. This value is unique across
* all versions of a document, and can't be changed.
*/
public void setVersionName(String versionName) {
this.versionName = versionName;
}
/**
*
* The version of the artifact associated with the document. For example, 12.6. This value is unique across all
* versions of a document, and can't be changed.
*
*
* @return The version of the artifact associated with the document. For example, 12.6. This value is unique across
* all versions of a document, and can't be changed.
*/
public String getVersionName() {
return this.versionName;
}
/**
*
* The version of the artifact associated with the document. For example, 12.6. This value is unique across all
* versions of a document, and can't be changed.
*
*
* @param versionName
* The version of the artifact associated with the document. For example, 12.6. This value is unique across
* all versions of a document, and can't be changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withVersionName(String versionName) {
setVersionName(versionName);
return this;
}
/**
*
* The document version.
*
*
* @param documentVersion
* The document version.
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The document version.
*
*
* @return The document version.
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The document version.
*
*
* @param documentVersion
* The document version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
*
*
* @param status
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
* @see DocumentStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
*
*
* @return The status of the SSM document, such as Creating
, Active
, Updating
* , Failed
, and Deleting
.
* @see DocumentStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
*
*
* @param status
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentStatus
*/
public GetDocumentResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
*
*
* @param status
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
* @see DocumentStatus
*/
public void setStatus(DocumentStatus status) {
withStatus(status);
}
/**
*
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
*
*
* @param status
* The status of the SSM document, such as Creating
, Active
, Updating
,
* Failed
, and Deleting
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentStatus
*/
public GetDocumentResult withStatus(DocumentStatus status) {
this.status = status.toString();
return this;
}
/**
*
* A message returned by Amazon Web Services Systems Manager that explains the Status
value. For
* example, a Failed
status might be explained by the StatusInformation
message,
* "The specified S3 bucket doesn't exist. Verify that the URL of the S3 bucket is correct."
*
*
* @param statusInformation
* A message returned by Amazon Web Services Systems Manager that explains the Status
value. For
* example, a Failed
status might be explained by the StatusInformation
message,
* "The specified S3 bucket doesn't exist. Verify that the URL of the S3 bucket is correct."
*/
public void setStatusInformation(String statusInformation) {
this.statusInformation = statusInformation;
}
/**
*
* A message returned by Amazon Web Services Systems Manager that explains the Status
value. For
* example, a Failed
status might be explained by the StatusInformation
message,
* "The specified S3 bucket doesn't exist. Verify that the URL of the S3 bucket is correct."
*
*
* @return A message returned by Amazon Web Services Systems Manager that explains the Status
value.
* For example, a Failed
status might be explained by the StatusInformation
* message, "The specified S3 bucket doesn't exist. Verify that the URL of the S3 bucket is correct."
*/
public String getStatusInformation() {
return this.statusInformation;
}
/**
*
* A message returned by Amazon Web Services Systems Manager that explains the Status
value. For
* example, a Failed
status might be explained by the StatusInformation
message,
* "The specified S3 bucket doesn't exist. Verify that the URL of the S3 bucket is correct."
*
*
* @param statusInformation
* A message returned by Amazon Web Services Systems Manager that explains the Status
value. For
* example, a Failed
status might be explained by the StatusInformation
message,
* "The specified S3 bucket doesn't exist. Verify that the URL of the S3 bucket is correct."
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withStatusInformation(String statusInformation) {
setStatusInformation(statusInformation);
return this;
}
/**
*
* The contents of the SSM document.
*
*
* @param content
* The contents of the SSM document.
*/
public void setContent(String content) {
this.content = content;
}
/**
*
* The contents of the SSM document.
*
*
* @return The contents of the SSM document.
*/
public String getContent() {
return this.content;
}
/**
*
* The contents of the SSM document.
*
*
* @param content
* The contents of the SSM document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withContent(String content) {
setContent(content);
return this;
}
/**
*
* The document type.
*
*
* @param documentType
* The document type.
* @see DocumentType
*/
public void setDocumentType(String documentType) {
this.documentType = documentType;
}
/**
*
* The document type.
*
*
* @return The document type.
* @see DocumentType
*/
public String getDocumentType() {
return this.documentType;
}
/**
*
* The document type.
*
*
* @param documentType
* The document type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentType
*/
public GetDocumentResult withDocumentType(String documentType) {
setDocumentType(documentType);
return this;
}
/**
*
* The document type.
*
*
* @param documentType
* The document type.
* @see DocumentType
*/
public void setDocumentType(DocumentType documentType) {
withDocumentType(documentType);
}
/**
*
* The document type.
*
*
* @param documentType
* The document type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentType
*/
public GetDocumentResult withDocumentType(DocumentType documentType) {
this.documentType = documentType.toString();
return this;
}
/**
*
* The document format, either JSON or YAML.
*
*
* @param documentFormat
* The document format, either JSON or YAML.
* @see DocumentFormat
*/
public void setDocumentFormat(String documentFormat) {
this.documentFormat = documentFormat;
}
/**
*
* The document format, either JSON or YAML.
*
*
* @return The document format, either JSON or YAML.
* @see DocumentFormat
*/
public String getDocumentFormat() {
return this.documentFormat;
}
/**
*
* The document format, either JSON or YAML.
*
*
* @param documentFormat
* The document format, either JSON or YAML.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentFormat
*/
public GetDocumentResult withDocumentFormat(String documentFormat) {
setDocumentFormat(documentFormat);
return this;
}
/**
*
* The document format, either JSON or YAML.
*
*
* @param documentFormat
* The document format, either JSON or YAML.
* @see DocumentFormat
*/
public void setDocumentFormat(DocumentFormat documentFormat) {
withDocumentFormat(documentFormat);
}
/**
*
* The document format, either JSON or YAML.
*
*
* @param documentFormat
* The document format, either JSON or YAML.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentFormat
*/
public GetDocumentResult withDocumentFormat(DocumentFormat documentFormat) {
this.documentFormat = documentFormat.toString();
return this;
}
/**
*
* A list of SSM documents required by a document. For example, an ApplicationConfiguration
document
* requires an ApplicationConfigurationSchema
document.
*
*
* @return A list of SSM documents required by a document. For example, an ApplicationConfiguration
* document requires an ApplicationConfigurationSchema
document.
*/
public java.util.List getRequires() {
if (requires == null) {
requires = new com.amazonaws.internal.SdkInternalList();
}
return requires;
}
/**
*
* A list of SSM documents required by a document. For example, an ApplicationConfiguration
document
* requires an ApplicationConfigurationSchema
document.
*
*
* @param requires
* A list of SSM documents required by a document. For example, an ApplicationConfiguration
* document requires an ApplicationConfigurationSchema
document.
*/
public void setRequires(java.util.Collection requires) {
if (requires == null) {
this.requires = null;
return;
}
this.requires = new com.amazonaws.internal.SdkInternalList(requires);
}
/**
*
* A list of SSM documents required by a document. For example, an ApplicationConfiguration
document
* requires an ApplicationConfigurationSchema
document.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRequires(java.util.Collection)} or {@link #withRequires(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param requires
* A list of SSM documents required by a document. For example, an ApplicationConfiguration
* document requires an ApplicationConfigurationSchema
document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withRequires(DocumentRequires... requires) {
if (this.requires == null) {
setRequires(new com.amazonaws.internal.SdkInternalList(requires.length));
}
for (DocumentRequires ele : requires) {
this.requires.add(ele);
}
return this;
}
/**
*
* A list of SSM documents required by a document. For example, an ApplicationConfiguration
document
* requires an ApplicationConfigurationSchema
document.
*
*
* @param requires
* A list of SSM documents required by a document. For example, an ApplicationConfiguration
* document requires an ApplicationConfigurationSchema
document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withRequires(java.util.Collection requires) {
setRequires(requires);
return this;
}
/**
*
* A description of the document attachments, including names, locations, sizes, and so on.
*
*
* @return A description of the document attachments, including names, locations, sizes, and so on.
*/
public java.util.List getAttachmentsContent() {
if (attachmentsContent == null) {
attachmentsContent = new com.amazonaws.internal.SdkInternalList();
}
return attachmentsContent;
}
/**
*
* A description of the document attachments, including names, locations, sizes, and so on.
*
*
* @param attachmentsContent
* A description of the document attachments, including names, locations, sizes, and so on.
*/
public void setAttachmentsContent(java.util.Collection attachmentsContent) {
if (attachmentsContent == null) {
this.attachmentsContent = null;
return;
}
this.attachmentsContent = new com.amazonaws.internal.SdkInternalList(attachmentsContent);
}
/**
*
* A description of the document attachments, including names, locations, sizes, and so on.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAttachmentsContent(java.util.Collection)} or {@link #withAttachmentsContent(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param attachmentsContent
* A description of the document attachments, including names, locations, sizes, and so on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withAttachmentsContent(AttachmentContent... attachmentsContent) {
if (this.attachmentsContent == null) {
setAttachmentsContent(new com.amazonaws.internal.SdkInternalList(attachmentsContent.length));
}
for (AttachmentContent ele : attachmentsContent) {
this.attachmentsContent.add(ele);
}
return this;
}
/**
*
* A description of the document attachments, including names, locations, sizes, and so on.
*
*
* @param attachmentsContent
* A description of the document attachments, including names, locations, sizes, and so on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDocumentResult withAttachmentsContent(java.util.Collection attachmentsContent) {
setAttachmentsContent(attachmentsContent);
return this;
}
/**
*
* The current review status of a new custom Systems Manager document (SSM document) created by a member of your
* organization, or of the latest version of an existing SSM document.
*
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is approved, the
* status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
*
*
* @param reviewStatus
* The current review status of a new custom Systems Manager document (SSM document) created by a member of
* your organization, or of the latest version of an existing SSM document.
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is
* approved, the status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
* @see ReviewStatus
*/
public void setReviewStatus(String reviewStatus) {
this.reviewStatus = reviewStatus;
}
/**
*
* The current review status of a new custom Systems Manager document (SSM document) created by a member of your
* organization, or of the latest version of an existing SSM document.
*
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is approved, the
* status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
*
*
* @return The current review status of a new custom Systems Manager document (SSM document) created by a member of
* your organization, or of the latest version of an existing SSM document.
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is
* approved, the status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
* @see ReviewStatus
*/
public String getReviewStatus() {
return this.reviewStatus;
}
/**
*
* The current review status of a new custom Systems Manager document (SSM document) created by a member of your
* organization, or of the latest version of an existing SSM document.
*
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is approved, the
* status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
*
*
* @param reviewStatus
* The current review status of a new custom Systems Manager document (SSM document) created by a member of
* your organization, or of the latest version of an existing SSM document.
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is
* approved, the status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReviewStatus
*/
public GetDocumentResult withReviewStatus(String reviewStatus) {
setReviewStatus(reviewStatus);
return this;
}
/**
*
* The current review status of a new custom Systems Manager document (SSM document) created by a member of your
* organization, or of the latest version of an existing SSM document.
*
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is approved, the
* status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
*
*
* @param reviewStatus
* The current review status of a new custom Systems Manager document (SSM document) created by a member of
* your organization, or of the latest version of an existing SSM document.
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is
* approved, the status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
* @see ReviewStatus
*/
public void setReviewStatus(ReviewStatus reviewStatus) {
withReviewStatus(reviewStatus);
}
/**
*
* The current review status of a new custom Systems Manager document (SSM document) created by a member of your
* organization, or of the latest version of an existing SSM document.
*
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is approved, the
* status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
*
*
* @param reviewStatus
* The current review status of a new custom Systems Manager document (SSM document) created by a member of
* your organization, or of the latest version of an existing SSM document.
*
* Only one version of an SSM document can be in the APPROVED state at a time. When a new version is
* approved, the status of the previous version changes to REJECTED.
*
*
* Only one version of an SSM document can be in review, or PENDING, at a time.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReviewStatus
*/
public GetDocumentResult withReviewStatus(ReviewStatus reviewStatus) {
this.reviewStatus = reviewStatus.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getCreatedDate() != null)
sb.append("CreatedDate: ").append(getCreatedDate()).append(",");
if (getDisplayName() != null)
sb.append("DisplayName: ").append(getDisplayName()).append(",");
if (getVersionName() != null)
sb.append("VersionName: ").append(getVersionName()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusInformation() != null)
sb.append("StatusInformation: ").append(getStatusInformation()).append(",");
if (getContent() != null)
sb.append("Content: ").append(getContent()).append(",");
if (getDocumentType() != null)
sb.append("DocumentType: ").append(getDocumentType()).append(",");
if (getDocumentFormat() != null)
sb.append("DocumentFormat: ").append(getDocumentFormat()).append(",");
if (getRequires() != null)
sb.append("Requires: ").append(getRequires()).append(",");
if (getAttachmentsContent() != null)
sb.append("AttachmentsContent: ").append(getAttachmentsContent()).append(",");
if (getReviewStatus() != null)
sb.append("ReviewStatus: ").append(getReviewStatus());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetDocumentResult == false)
return false;
GetDocumentResult other = (GetDocumentResult) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getCreatedDate() == null ^ this.getCreatedDate() == null)
return false;
if (other.getCreatedDate() != null && other.getCreatedDate().equals(this.getCreatedDate()) == false)
return false;
if (other.getDisplayName() == null ^ this.getDisplayName() == null)
return false;
if (other.getDisplayName() != null && other.getDisplayName().equals(this.getDisplayName()) == false)
return false;
if (other.getVersionName() == null ^ this.getVersionName() == null)
return false;
if (other.getVersionName() != null && other.getVersionName().equals(this.getVersionName()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusInformation() == null ^ this.getStatusInformation() == null)
return false;
if (other.getStatusInformation() != null && other.getStatusInformation().equals(this.getStatusInformation()) == false)
return false;
if (other.getContent() == null ^ this.getContent() == null)
return false;
if (other.getContent() != null && other.getContent().equals(this.getContent()) == false)
return false;
if (other.getDocumentType() == null ^ this.getDocumentType() == null)
return false;
if (other.getDocumentType() != null && other.getDocumentType().equals(this.getDocumentType()) == false)
return false;
if (other.getDocumentFormat() == null ^ this.getDocumentFormat() == null)
return false;
if (other.getDocumentFormat() != null && other.getDocumentFormat().equals(this.getDocumentFormat()) == false)
return false;
if (other.getRequires() == null ^ this.getRequires() == null)
return false;
if (other.getRequires() != null && other.getRequires().equals(this.getRequires()) == false)
return false;
if (other.getAttachmentsContent() == null ^ this.getAttachmentsContent() == null)
return false;
if (other.getAttachmentsContent() != null && other.getAttachmentsContent().equals(this.getAttachmentsContent()) == false)
return false;
if (other.getReviewStatus() == null ^ this.getReviewStatus() == null)
return false;
if (other.getReviewStatus() != null && other.getReviewStatus().equals(this.getReviewStatus()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getCreatedDate() == null) ? 0 : getCreatedDate().hashCode());
hashCode = prime * hashCode + ((getDisplayName() == null) ? 0 : getDisplayName().hashCode());
hashCode = prime * hashCode + ((getVersionName() == null) ? 0 : getVersionName().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusInformation() == null) ? 0 : getStatusInformation().hashCode());
hashCode = prime * hashCode + ((getContent() == null) ? 0 : getContent().hashCode());
hashCode = prime * hashCode + ((getDocumentType() == null) ? 0 : getDocumentType().hashCode());
hashCode = prime * hashCode + ((getDocumentFormat() == null) ? 0 : getDocumentFormat().hashCode());
hashCode = prime * hashCode + ((getRequires() == null) ? 0 : getRequires().hashCode());
hashCode = prime * hashCode + ((getAttachmentsContent() == null) ? 0 : getAttachmentsContent().hashCode());
hashCode = prime * hashCode + ((getReviewStatus() == null) ? 0 : getReviewStatus().hashCode());
return hashCode;
}
@Override
public GetDocumentResult clone() {
try {
return (GetDocumentResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}