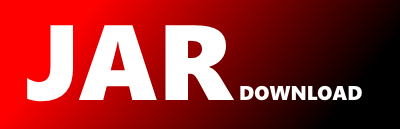
com.amazonaws.services.simplesystemsmanagement.model.GetMaintenanceWindowExecutionTaskResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetMaintenanceWindowExecutionTaskResult extends com.amazonaws.AmazonWebServiceResult implements Serializable,
Cloneable {
/**
*
* The ID of the maintenance window execution that includes the task.
*
*/
private String windowExecutionId;
/**
*
* The ID of the specific task execution in the maintenance window task that was retrieved.
*
*/
private String taskExecutionId;
/**
*
* The Amazon Resource Name (ARN) of the task that ran.
*
*/
private String taskArn;
/**
*
* The role that was assumed when running the task.
*
*/
private String serviceRole;
/**
*
* The type of task that was run.
*
*/
private String type;
/**
*
* The parameters passed to the task when it was run.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* -
*
* Key
: string, between 1 and 255 characters
*
*
* -
*
* Value
: an array of strings, each between 1 and 255 characters
*
*
*
*/
private com.amazonaws.internal.SdkInternalList> taskParameters;
/**
*
* The priority of the task.
*
*/
private Integer priority;
/**
*
* The defined maximum number of task executions that could be run in parallel.
*
*/
private String maxConcurrency;
/**
*
* The defined maximum number of task execution errors allowed before scheduling of the task execution would have
* been stopped.
*
*/
private String maxErrors;
/**
*
* The status of the task.
*
*/
private String status;
/**
*
* The details explaining the status. Not available for all status values.
*
*/
private String statusDetails;
/**
*
* The time the task execution started.
*
*/
private java.util.Date startTime;
/**
*
* The time the task execution completed.
*
*/
private java.util.Date endTime;
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The CloudWatch alarms that were invoked by the maintenance window task.
*
*/
private com.amazonaws.internal.SdkInternalList triggeredAlarms;
/**
*
* The ID of the maintenance window execution that includes the task.
*
*
* @param windowExecutionId
* The ID of the maintenance window execution that includes the task.
*/
public void setWindowExecutionId(String windowExecutionId) {
this.windowExecutionId = windowExecutionId;
}
/**
*
* The ID of the maintenance window execution that includes the task.
*
*
* @return The ID of the maintenance window execution that includes the task.
*/
public String getWindowExecutionId() {
return this.windowExecutionId;
}
/**
*
* The ID of the maintenance window execution that includes the task.
*
*
* @param windowExecutionId
* The ID of the maintenance window execution that includes the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withWindowExecutionId(String windowExecutionId) {
setWindowExecutionId(windowExecutionId);
return this;
}
/**
*
* The ID of the specific task execution in the maintenance window task that was retrieved.
*
*
* @param taskExecutionId
* The ID of the specific task execution in the maintenance window task that was retrieved.
*/
public void setTaskExecutionId(String taskExecutionId) {
this.taskExecutionId = taskExecutionId;
}
/**
*
* The ID of the specific task execution in the maintenance window task that was retrieved.
*
*
* @return The ID of the specific task execution in the maintenance window task that was retrieved.
*/
public String getTaskExecutionId() {
return this.taskExecutionId;
}
/**
*
* The ID of the specific task execution in the maintenance window task that was retrieved.
*
*
* @param taskExecutionId
* The ID of the specific task execution in the maintenance window task that was retrieved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withTaskExecutionId(String taskExecutionId) {
setTaskExecutionId(taskExecutionId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the task that ran.
*
*
* @param taskArn
* The Amazon Resource Name (ARN) of the task that ran.
*/
public void setTaskArn(String taskArn) {
this.taskArn = taskArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task that ran.
*
*
* @return The Amazon Resource Name (ARN) of the task that ran.
*/
public String getTaskArn() {
return this.taskArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task that ran.
*
*
* @param taskArn
* The Amazon Resource Name (ARN) of the task that ran.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withTaskArn(String taskArn) {
setTaskArn(taskArn);
return this;
}
/**
*
* The role that was assumed when running the task.
*
*
* @param serviceRole
* The role that was assumed when running the task.
*/
public void setServiceRole(String serviceRole) {
this.serviceRole = serviceRole;
}
/**
*
* The role that was assumed when running the task.
*
*
* @return The role that was assumed when running the task.
*/
public String getServiceRole() {
return this.serviceRole;
}
/**
*
* The role that was assumed when running the task.
*
*
* @param serviceRole
* The role that was assumed when running the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withServiceRole(String serviceRole) {
setServiceRole(serviceRole);
return this;
}
/**
*
* The type of task that was run.
*
*
* @param type
* The type of task that was run.
* @see MaintenanceWindowTaskType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of task that was run.
*
*
* @return The type of task that was run.
* @see MaintenanceWindowTaskType
*/
public String getType() {
return this.type;
}
/**
*
* The type of task that was run.
*
*
* @param type
* The type of task that was run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskType
*/
public GetMaintenanceWindowExecutionTaskResult withType(String type) {
setType(type);
return this;
}
/**
*
* The type of task that was run.
*
*
* @param type
* The type of task that was run.
* @see MaintenanceWindowTaskType
*/
public void setType(MaintenanceWindowTaskType type) {
withType(type);
}
/**
*
* The type of task that was run.
*
*
* @param type
* The type of task that was run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskType
*/
public GetMaintenanceWindowExecutionTaskResult withType(MaintenanceWindowTaskType type) {
this.type = type.toString();
return this;
}
/**
*
* The parameters passed to the task when it was run.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* -
*
* Key
: string, between 1 and 255 characters
*
*
* -
*
* Value
: an array of strings, each between 1 and 255 characters
*
*
*
*
* @return The parameters passed to the task when it was run.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure.
* For information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* -
*
* Key
: string, between 1 and 255 characters
*
*
* -
*
* Value
: an array of strings, each between 1 and 255 characters
*
*
*/
public java.util.List> getTaskParameters() {
if (taskParameters == null) {
taskParameters = new com.amazonaws.internal.SdkInternalList>();
}
return taskParameters;
}
/**
*
* The parameters passed to the task when it was run.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* -
*
* Key
: string, between 1 and 255 characters
*
*
* -
*
* Value
: an array of strings, each between 1 and 255 characters
*
*
*
*
* @param taskParameters
* The parameters passed to the task when it was run.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* -
*
* Key
: string, between 1 and 255 characters
*
*
* -
*
* Value
: an array of strings, each between 1 and 255 characters
*
*
*/
public void setTaskParameters(java.util.Collection> taskParameters) {
if (taskParameters == null) {
this.taskParameters = null;
return;
}
this.taskParameters = new com.amazonaws.internal.SdkInternalList>(taskParameters);
}
/**
*
* The parameters passed to the task when it was run.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* -
*
* Key
: string, between 1 and 255 characters
*
*
* -
*
* Value
: an array of strings, each between 1 and 255 characters
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTaskParameters(java.util.Collection)} or {@link #withTaskParameters(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param taskParameters
* The parameters passed to the task when it was run.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* -
*
* Key
: string, between 1 and 255 characters
*
*
* -
*
* Value
: an array of strings, each between 1 and 255 characters
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withTaskParameters(java.util.Map... taskParameters) {
if (this.taskParameters == null) {
setTaskParameters(new com.amazonaws.internal.SdkInternalList>(
taskParameters.length));
}
for (java.util.Map ele : taskParameters) {
this.taskParameters.add(ele);
}
return this;
}
/**
*
* The parameters passed to the task when it was run.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* -
*
* Key
: string, between 1 and 255 characters
*
*
* -
*
* Value
: an array of strings, each between 1 and 255 characters
*
*
*
*
* @param taskParameters
* The parameters passed to the task when it was run.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* -
*
* Key
: string, between 1 and 255 characters
*
*
* -
*
* Value
: an array of strings, each between 1 and 255 characters
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withTaskParameters(
java.util.Collection> taskParameters) {
setTaskParameters(taskParameters);
return this;
}
/**
*
* The priority of the task.
*
*
* @param priority
* The priority of the task.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* The priority of the task.
*
*
* @return The priority of the task.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* The priority of the task.
*
*
* @param priority
* The priority of the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* The defined maximum number of task executions that could be run in parallel.
*
*
* @param maxConcurrency
* The defined maximum number of task executions that could be run in parallel.
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The defined maximum number of task executions that could be run in parallel.
*
*
* @return The defined maximum number of task executions that could be run in parallel.
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The defined maximum number of task executions that could be run in parallel.
*
*
* @param maxConcurrency
* The defined maximum number of task executions that could be run in parallel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The defined maximum number of task execution errors allowed before scheduling of the task execution would have
* been stopped.
*
*
* @param maxErrors
* The defined maximum number of task execution errors allowed before scheduling of the task execution would
* have been stopped.
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The defined maximum number of task execution errors allowed before scheduling of the task execution would have
* been stopped.
*
*
* @return The defined maximum number of task execution errors allowed before scheduling of the task execution would
* have been stopped.
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The defined maximum number of task execution errors allowed before scheduling of the task execution would have
* been stopped.
*
*
* @param maxErrors
* The defined maximum number of task execution errors allowed before scheduling of the task execution would
* have been stopped.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The status of the task.
*
*
* @param status
* The status of the task.
* @see MaintenanceWindowExecutionStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the task.
*
*
* @return The status of the task.
* @see MaintenanceWindowExecutionStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the task.
*
*
* @param status
* The status of the task.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowExecutionStatus
*/
public GetMaintenanceWindowExecutionTaskResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the task.
*
*
* @param status
* The status of the task.
* @see MaintenanceWindowExecutionStatus
*/
public void setStatus(MaintenanceWindowExecutionStatus status) {
withStatus(status);
}
/**
*
* The status of the task.
*
*
* @param status
* The status of the task.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowExecutionStatus
*/
public GetMaintenanceWindowExecutionTaskResult withStatus(MaintenanceWindowExecutionStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The details explaining the status. Not available for all status values.
*
*
* @param statusDetails
* The details explaining the status. Not available for all status values.
*/
public void setStatusDetails(String statusDetails) {
this.statusDetails = statusDetails;
}
/**
*
* The details explaining the status. Not available for all status values.
*
*
* @return The details explaining the status. Not available for all status values.
*/
public String getStatusDetails() {
return this.statusDetails;
}
/**
*
* The details explaining the status. Not available for all status values.
*
*
* @param statusDetails
* The details explaining the status. Not available for all status values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withStatusDetails(String statusDetails) {
setStatusDetails(statusDetails);
return this;
}
/**
*
* The time the task execution started.
*
*
* @param startTime
* The time the task execution started.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The time the task execution started.
*
*
* @return The time the task execution started.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The time the task execution started.
*
*
* @param startTime
* The time the task execution started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The time the task execution completed.
*
*
* @param endTime
* The time the task execution completed.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The time the task execution completed.
*
*
* @return The time the task execution completed.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The time the task execution completed.
*
*
* @param endTime
* The time the task execution completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm you applied to your maintenance window task.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*
* @return The details for the CloudWatch alarm you applied to your maintenance window task.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm you applied to your maintenance window task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
*
* The CloudWatch alarms that were invoked by the maintenance window task.
*
*
* @return The CloudWatch alarms that were invoked by the maintenance window task.
*/
public java.util.List getTriggeredAlarms() {
if (triggeredAlarms == null) {
triggeredAlarms = new com.amazonaws.internal.SdkInternalList();
}
return triggeredAlarms;
}
/**
*
* The CloudWatch alarms that were invoked by the maintenance window task.
*
*
* @param triggeredAlarms
* The CloudWatch alarms that were invoked by the maintenance window task.
*/
public void setTriggeredAlarms(java.util.Collection triggeredAlarms) {
if (triggeredAlarms == null) {
this.triggeredAlarms = null;
return;
}
this.triggeredAlarms = new com.amazonaws.internal.SdkInternalList(triggeredAlarms);
}
/**
*
* The CloudWatch alarms that were invoked by the maintenance window task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTriggeredAlarms(java.util.Collection)} or {@link #withTriggeredAlarms(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param triggeredAlarms
* The CloudWatch alarms that were invoked by the maintenance window task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withTriggeredAlarms(AlarmStateInformation... triggeredAlarms) {
if (this.triggeredAlarms == null) {
setTriggeredAlarms(new com.amazonaws.internal.SdkInternalList(triggeredAlarms.length));
}
for (AlarmStateInformation ele : triggeredAlarms) {
this.triggeredAlarms.add(ele);
}
return this;
}
/**
*
* The CloudWatch alarms that were invoked by the maintenance window task.
*
*
* @param triggeredAlarms
* The CloudWatch alarms that were invoked by the maintenance window task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowExecutionTaskResult withTriggeredAlarms(java.util.Collection triggeredAlarms) {
setTriggeredAlarms(triggeredAlarms);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWindowExecutionId() != null)
sb.append("WindowExecutionId: ").append(getWindowExecutionId()).append(",");
if (getTaskExecutionId() != null)
sb.append("TaskExecutionId: ").append(getTaskExecutionId()).append(",");
if (getTaskArn() != null)
sb.append("TaskArn: ").append(getTaskArn()).append(",");
if (getServiceRole() != null)
sb.append("ServiceRole: ").append(getServiceRole()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getTaskParameters() != null)
sb.append("TaskParameters: ").append("***Sensitive Data Redacted***").append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusDetails() != null)
sb.append("StatusDetails: ").append(getStatusDetails()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration()).append(",");
if (getTriggeredAlarms() != null)
sb.append("TriggeredAlarms: ").append(getTriggeredAlarms());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetMaintenanceWindowExecutionTaskResult == false)
return false;
GetMaintenanceWindowExecutionTaskResult other = (GetMaintenanceWindowExecutionTaskResult) obj;
if (other.getWindowExecutionId() == null ^ this.getWindowExecutionId() == null)
return false;
if (other.getWindowExecutionId() != null && other.getWindowExecutionId().equals(this.getWindowExecutionId()) == false)
return false;
if (other.getTaskExecutionId() == null ^ this.getTaskExecutionId() == null)
return false;
if (other.getTaskExecutionId() != null && other.getTaskExecutionId().equals(this.getTaskExecutionId()) == false)
return false;
if (other.getTaskArn() == null ^ this.getTaskArn() == null)
return false;
if (other.getTaskArn() != null && other.getTaskArn().equals(this.getTaskArn()) == false)
return false;
if (other.getServiceRole() == null ^ this.getServiceRole() == null)
return false;
if (other.getServiceRole() != null && other.getServiceRole().equals(this.getServiceRole()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getTaskParameters() == null ^ this.getTaskParameters() == null)
return false;
if (other.getTaskParameters() != null && other.getTaskParameters().equals(this.getTaskParameters()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusDetails() == null ^ this.getStatusDetails() == null)
return false;
if (other.getStatusDetails() != null && other.getStatusDetails().equals(this.getStatusDetails()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
if (other.getTriggeredAlarms() == null ^ this.getTriggeredAlarms() == null)
return false;
if (other.getTriggeredAlarms() != null && other.getTriggeredAlarms().equals(this.getTriggeredAlarms()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWindowExecutionId() == null) ? 0 : getWindowExecutionId().hashCode());
hashCode = prime * hashCode + ((getTaskExecutionId() == null) ? 0 : getTaskExecutionId().hashCode());
hashCode = prime * hashCode + ((getTaskArn() == null) ? 0 : getTaskArn().hashCode());
hashCode = prime * hashCode + ((getServiceRole() == null) ? 0 : getServiceRole().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getTaskParameters() == null) ? 0 : getTaskParameters().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusDetails() == null) ? 0 : getStatusDetails().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
hashCode = prime * hashCode + ((getTriggeredAlarms() == null) ? 0 : getTriggeredAlarms().hashCode());
return hashCode;
}
@Override
public GetMaintenanceWindowExecutionTaskResult clone() {
try {
return (GetMaintenanceWindowExecutionTaskResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}