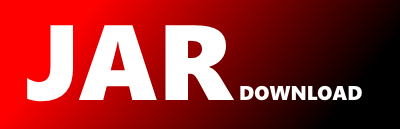
com.amazonaws.services.simplesystemsmanagement.model.GetMaintenanceWindowTaskResult Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetMaintenanceWindowTaskResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The retrieved maintenance window ID.
*
*/
private String windowId;
/**
*
* The retrieved maintenance window task ID.
*
*/
private String windowTaskId;
/**
*
* The targets where the task should run.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The resource that the task used during execution. For RUN_COMMAND
and AUTOMATION
task
* types, the value of TaskArn
is the SSM document name/ARN. For LAMBDA
tasks, the value
* is the function name/ARN. For STEP_FUNCTIONS
tasks, the value is the state machine ARN.
*
*/
private String taskArn;
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*/
private String serviceRoleArn;
/**
*
* The type of task to run.
*
*/
private String taskType;
/**
*
* The parameters to pass to the task when it runs.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*/
private java.util.Map taskParameters;
/**
*
* The parameters to pass to the task when it runs.
*
*/
private MaintenanceWindowTaskInvocationParameters taskInvocationParameters;
/**
*
* The priority of the task when it runs. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*
*/
private Integer priority;
/**
*
* The maximum number of targets allowed to run this task in parallel.
*
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
, which may be reported in the response to this command. This
* value doesn't affect the running of your task and can be ignored.
*
*
*/
private String maxConcurrency;
/**
*
* The maximum number of errors allowed before the task stops being scheduled.
*
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
, which may be reported in the response to this command. This
* value doesn't affect the running of your task and can be ignored.
*
*
*/
private String maxErrors;
/**
*
* The location in Amazon Simple Storage Service (Amazon S3) where the task results are logged.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*/
private LoggingInfo loggingInfo;
/**
*
* The retrieved task name.
*
*/
private String name;
/**
*
* The retrieved task description.
*
*/
private String description;
/**
*
* The action to take on tasks when the maintenance window cutoff time is reached. CONTINUE_TASK
means
* that tasks continue to run. For Automation, Lambda, Step Functions tasks, CANCEL_TASK
means that
* currently running task invocations continue, but no new task invocations are started. For Run Command tasks,
* CANCEL_TASK
means the system attempts to stop the task by sending a CancelCommand
* operation.
*
*/
private String cutoffBehavior;
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The retrieved maintenance window ID.
*
*
* @param windowId
* The retrieved maintenance window ID.
*/
public void setWindowId(String windowId) {
this.windowId = windowId;
}
/**
*
* The retrieved maintenance window ID.
*
*
* @return The retrieved maintenance window ID.
*/
public String getWindowId() {
return this.windowId;
}
/**
*
* The retrieved maintenance window ID.
*
*
* @param windowId
* The retrieved maintenance window ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withWindowId(String windowId) {
setWindowId(windowId);
return this;
}
/**
*
* The retrieved maintenance window task ID.
*
*
* @param windowTaskId
* The retrieved maintenance window task ID.
*/
public void setWindowTaskId(String windowTaskId) {
this.windowTaskId = windowTaskId;
}
/**
*
* The retrieved maintenance window task ID.
*
*
* @return The retrieved maintenance window task ID.
*/
public String getWindowTaskId() {
return this.windowTaskId;
}
/**
*
* The retrieved maintenance window task ID.
*
*
* @param windowTaskId
* The retrieved maintenance window task ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withWindowTaskId(String windowTaskId) {
setWindowTaskId(windowTaskId);
return this;
}
/**
*
* The targets where the task should run.
*
*
* @return The targets where the task should run.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The targets where the task should run.
*
*
* @param targets
* The targets where the task should run.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The targets where the task should run.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The targets where the task should run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The targets where the task should run.
*
*
* @param targets
* The targets where the task should run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The resource that the task used during execution. For RUN_COMMAND
and AUTOMATION
task
* types, the value of TaskArn
is the SSM document name/ARN. For LAMBDA
tasks, the value
* is the function name/ARN. For STEP_FUNCTIONS
tasks, the value is the state machine ARN.
*
*
* @param taskArn
* The resource that the task used during execution. For RUN_COMMAND
and AUTOMATION
* task types, the value of TaskArn
is the SSM document name/ARN. For LAMBDA
tasks,
* the value is the function name/ARN. For STEP_FUNCTIONS
tasks, the value is the state machine
* ARN.
*/
public void setTaskArn(String taskArn) {
this.taskArn = taskArn;
}
/**
*
* The resource that the task used during execution. For RUN_COMMAND
and AUTOMATION
task
* types, the value of TaskArn
is the SSM document name/ARN. For LAMBDA
tasks, the value
* is the function name/ARN. For STEP_FUNCTIONS
tasks, the value is the state machine ARN.
*
*
* @return The resource that the task used during execution. For RUN_COMMAND
and
* AUTOMATION
task types, the value of TaskArn
is the SSM document name/ARN. For
* LAMBDA
tasks, the value is the function name/ARN. For STEP_FUNCTIONS
tasks, the
* value is the state machine ARN.
*/
public String getTaskArn() {
return this.taskArn;
}
/**
*
* The resource that the task used during execution. For RUN_COMMAND
and AUTOMATION
task
* types, the value of TaskArn
is the SSM document name/ARN. For LAMBDA
tasks, the value
* is the function name/ARN. For STEP_FUNCTIONS
tasks, the value is the state machine ARN.
*
*
* @param taskArn
* The resource that the task used during execution. For RUN_COMMAND
and AUTOMATION
* task types, the value of TaskArn
is the SSM document name/ARN. For LAMBDA
tasks,
* the value is the function name/ARN. For STEP_FUNCTIONS
tasks, the value is the state machine
* ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withTaskArn(String taskArn) {
setTaskArn(taskArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*
* @param serviceRoleArn
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish
* Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*/
public void setServiceRoleArn(String serviceRoleArn) {
this.serviceRoleArn = serviceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*
* @return The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish
* Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*/
public String getServiceRoleArn() {
return this.serviceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*
* @param serviceRoleArn
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish
* Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withServiceRoleArn(String serviceRoleArn) {
setServiceRoleArn(serviceRoleArn);
return this;
}
/**
*
* The type of task to run.
*
*
* @param taskType
* The type of task to run.
* @see MaintenanceWindowTaskType
*/
public void setTaskType(String taskType) {
this.taskType = taskType;
}
/**
*
* The type of task to run.
*
*
* @return The type of task to run.
* @see MaintenanceWindowTaskType
*/
public String getTaskType() {
return this.taskType;
}
/**
*
* The type of task to run.
*
*
* @param taskType
* The type of task to run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskType
*/
public GetMaintenanceWindowTaskResult withTaskType(String taskType) {
setTaskType(taskType);
return this;
}
/**
*
* The type of task to run.
*
*
* @param taskType
* The type of task to run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskType
*/
public GetMaintenanceWindowTaskResult withTaskType(MaintenanceWindowTaskType taskType) {
this.taskType = taskType.toString();
return this;
}
/**
*
* The parameters to pass to the task when it runs.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @return The parameters to pass to the task when it runs.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure.
* For information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public java.util.Map getTaskParameters() {
return taskParameters;
}
/**
*
* The parameters to pass to the task when it runs.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param taskParameters
* The parameters to pass to the task when it runs.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public void setTaskParameters(java.util.Map taskParameters) {
this.taskParameters = taskParameters;
}
/**
*
* The parameters to pass to the task when it runs.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param taskParameters
* The parameters to pass to the task when it runs.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withTaskParameters(java.util.Map taskParameters) {
setTaskParameters(taskParameters);
return this;
}
/**
* Add a single TaskParameters entry
*
* @see GetMaintenanceWindowTaskResult#withTaskParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult addTaskParametersEntry(String key, MaintenanceWindowTaskParameterValueExpression value) {
if (null == this.taskParameters) {
this.taskParameters = new java.util.HashMap();
}
if (this.taskParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.taskParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into TaskParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult clearTaskParametersEntries() {
this.taskParameters = null;
return this;
}
/**
*
* The parameters to pass to the task when it runs.
*
*
* @param taskInvocationParameters
* The parameters to pass to the task when it runs.
*/
public void setTaskInvocationParameters(MaintenanceWindowTaskInvocationParameters taskInvocationParameters) {
this.taskInvocationParameters = taskInvocationParameters;
}
/**
*
* The parameters to pass to the task when it runs.
*
*
* @return The parameters to pass to the task when it runs.
*/
public MaintenanceWindowTaskInvocationParameters getTaskInvocationParameters() {
return this.taskInvocationParameters;
}
/**
*
* The parameters to pass to the task when it runs.
*
*
* @param taskInvocationParameters
* The parameters to pass to the task when it runs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withTaskInvocationParameters(MaintenanceWindowTaskInvocationParameters taskInvocationParameters) {
setTaskInvocationParameters(taskInvocationParameters);
return this;
}
/**
*
* The priority of the task when it runs. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*
*
* @param priority
* The priority of the task when it runs. The lower the number, the higher the priority. Tasks that have the
* same priority are scheduled in parallel.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* The priority of the task when it runs. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*
*
* @return The priority of the task when it runs. The lower the number, the higher the priority. Tasks that have the
* same priority are scheduled in parallel.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* The priority of the task when it runs. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*
*
* @param priority
* The priority of the task when it runs. The lower the number, the higher the priority. Tasks that have the
* same priority are scheduled in parallel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* The maximum number of targets allowed to run this task in parallel.
*
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
, which may be reported in the response to this command. This
* value doesn't affect the running of your task and can be ignored.
*
*
*
* @param maxConcurrency
* The maximum number of targets allowed to run this task in parallel.
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
, which may be reported in the response
* to this command. This value doesn't affect the running of your task and can be ignored.
*
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The maximum number of targets allowed to run this task in parallel.
*
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
, which may be reported in the response to this command. This
* value doesn't affect the running of your task and can be ignored.
*
*
*
* @return The maximum number of targets allowed to run this task in parallel.
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
, which may be reported in the response
* to this command. This value doesn't affect the running of your task and can be ignored.
*
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The maximum number of targets allowed to run this task in parallel.
*
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
, which may be reported in the response to this command. This
* value doesn't affect the running of your task and can be ignored.
*
*
*
* @param maxConcurrency
* The maximum number of targets allowed to run this task in parallel.
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
, which may be reported in the response
* to this command. This value doesn't affect the running of your task and can be ignored.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The maximum number of errors allowed before the task stops being scheduled.
*
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
, which may be reported in the response to this command. This
* value doesn't affect the running of your task and can be ignored.
*
*
*
* @param maxErrors
* The maximum number of errors allowed before the task stops being scheduled.
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
, which may be reported in the response
* to this command. This value doesn't affect the running of your task and can be ignored.
*
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The maximum number of errors allowed before the task stops being scheduled.
*
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
, which may be reported in the response to this command. This
* value doesn't affect the running of your task and can be ignored.
*
*
*
* @return The maximum number of errors allowed before the task stops being scheduled.
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
, which may be reported in the response
* to this command. This value doesn't affect the running of your task and can be ignored.
*
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The maximum number of errors allowed before the task stops being scheduled.
*
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
, which may be reported in the response to this command. This
* value doesn't affect the running of your task and can be ignored.
*
*
*
* @param maxErrors
* The maximum number of errors allowed before the task stops being scheduled.
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
, which may be reported in the response
* to this command. This value doesn't affect the running of your task and can be ignored.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The location in Amazon Simple Storage Service (Amazon S3) where the task results are logged.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param loggingInfo
* The location in Amazon Simple Storage Service (Amazon S3) where the task results are logged.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
* options in the TaskInvocationParameters
structure. For information about how Amazon Web
* Services Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*/
public void setLoggingInfo(LoggingInfo loggingInfo) {
this.loggingInfo = loggingInfo;
}
/**
*
* The location in Amazon Simple Storage Service (Amazon S3) where the task results are logged.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @return The location in Amazon Simple Storage Service (Amazon S3) where the task results are logged.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and
* OutputS3KeyPrefix
options in the TaskInvocationParameters
structure. For
* information about how Amazon Web Services Systems Manager handles these options for the supported
* maintenance window task types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public LoggingInfo getLoggingInfo() {
return this.loggingInfo;
}
/**
*
* The location in Amazon Simple Storage Service (Amazon S3) where the task results are logged.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param loggingInfo
* The location in Amazon Simple Storage Service (Amazon S3) where the task results are logged.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
* options in the TaskInvocationParameters
structure. For information about how Amazon Web
* Services Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withLoggingInfo(LoggingInfo loggingInfo) {
setLoggingInfo(loggingInfo);
return this;
}
/**
*
* The retrieved task name.
*
*
* @param name
* The retrieved task name.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The retrieved task name.
*
*
* @return The retrieved task name.
*/
public String getName() {
return this.name;
}
/**
*
* The retrieved task name.
*
*
* @param name
* The retrieved task name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withName(String name) {
setName(name);
return this;
}
/**
*
* The retrieved task description.
*
*
* @param description
* The retrieved task description.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The retrieved task description.
*
*
* @return The retrieved task description.
*/
public String getDescription() {
return this.description;
}
/**
*
* The retrieved task description.
*
*
* @param description
* The retrieved task description.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The action to take on tasks when the maintenance window cutoff time is reached. CONTINUE_TASK
means
* that tasks continue to run. For Automation, Lambda, Step Functions tasks, CANCEL_TASK
means that
* currently running task invocations continue, but no new task invocations are started. For Run Command tasks,
* CANCEL_TASK
means the system attempts to stop the task by sending a CancelCommand
* operation.
*
*
* @param cutoffBehavior
* The action to take on tasks when the maintenance window cutoff time is reached. CONTINUE_TASK
* means that tasks continue to run. For Automation, Lambda, Step Functions tasks, CANCEL_TASK
* means that currently running task invocations continue, but no new task invocations are started. For Run
* Command tasks, CANCEL_TASK
means the system attempts to stop the task by sending a
* CancelCommand
operation.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public void setCutoffBehavior(String cutoffBehavior) {
this.cutoffBehavior = cutoffBehavior;
}
/**
*
* The action to take on tasks when the maintenance window cutoff time is reached. CONTINUE_TASK
means
* that tasks continue to run. For Automation, Lambda, Step Functions tasks, CANCEL_TASK
means that
* currently running task invocations continue, but no new task invocations are started. For Run Command tasks,
* CANCEL_TASK
means the system attempts to stop the task by sending a CancelCommand
* operation.
*
*
* @return The action to take on tasks when the maintenance window cutoff time is reached.
* CONTINUE_TASK
means that tasks continue to run. For Automation, Lambda, Step Functions
* tasks, CANCEL_TASK
means that currently running task invocations continue, but no new task
* invocations are started. For Run Command tasks, CANCEL_TASK
means the system attempts to
* stop the task by sending a CancelCommand
operation.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public String getCutoffBehavior() {
return this.cutoffBehavior;
}
/**
*
* The action to take on tasks when the maintenance window cutoff time is reached. CONTINUE_TASK
means
* that tasks continue to run. For Automation, Lambda, Step Functions tasks, CANCEL_TASK
means that
* currently running task invocations continue, but no new task invocations are started. For Run Command tasks,
* CANCEL_TASK
means the system attempts to stop the task by sending a CancelCommand
* operation.
*
*
* @param cutoffBehavior
* The action to take on tasks when the maintenance window cutoff time is reached. CONTINUE_TASK
* means that tasks continue to run. For Automation, Lambda, Step Functions tasks, CANCEL_TASK
* means that currently running task invocations continue, but no new task invocations are started. For Run
* Command tasks, CANCEL_TASK
means the system attempts to stop the task by sending a
* CancelCommand
operation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public GetMaintenanceWindowTaskResult withCutoffBehavior(String cutoffBehavior) {
setCutoffBehavior(cutoffBehavior);
return this;
}
/**
*
* The action to take on tasks when the maintenance window cutoff time is reached. CONTINUE_TASK
means
* that tasks continue to run. For Automation, Lambda, Step Functions tasks, CANCEL_TASK
means that
* currently running task invocations continue, but no new task invocations are started. For Run Command tasks,
* CANCEL_TASK
means the system attempts to stop the task by sending a CancelCommand
* operation.
*
*
* @param cutoffBehavior
* The action to take on tasks when the maintenance window cutoff time is reached. CONTINUE_TASK
* means that tasks continue to run. For Automation, Lambda, Step Functions tasks, CANCEL_TASK
* means that currently running task invocations continue, but no new task invocations are started. For Run
* Command tasks, CANCEL_TASK
means the system attempts to stop the task by sending a
* CancelCommand
operation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public GetMaintenanceWindowTaskResult withCutoffBehavior(MaintenanceWindowTaskCutoffBehavior cutoffBehavior) {
this.cutoffBehavior = cutoffBehavior.toString();
return this;
}
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm you applied to your maintenance window task.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*
* @return The details for the CloudWatch alarm you applied to your maintenance window task.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm you applied to your maintenance window task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMaintenanceWindowTaskResult withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWindowId() != null)
sb.append("WindowId: ").append(getWindowId()).append(",");
if (getWindowTaskId() != null)
sb.append("WindowTaskId: ").append(getWindowTaskId()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getTaskArn() != null)
sb.append("TaskArn: ").append(getTaskArn()).append(",");
if (getServiceRoleArn() != null)
sb.append("ServiceRoleArn: ").append(getServiceRoleArn()).append(",");
if (getTaskType() != null)
sb.append("TaskType: ").append(getTaskType()).append(",");
if (getTaskParameters() != null)
sb.append("TaskParameters: ").append("***Sensitive Data Redacted***").append(",");
if (getTaskInvocationParameters() != null)
sb.append("TaskInvocationParameters: ").append(getTaskInvocationParameters()).append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getLoggingInfo() != null)
sb.append("LoggingInfo: ").append(getLoggingInfo()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getCutoffBehavior() != null)
sb.append("CutoffBehavior: ").append(getCutoffBehavior()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetMaintenanceWindowTaskResult == false)
return false;
GetMaintenanceWindowTaskResult other = (GetMaintenanceWindowTaskResult) obj;
if (other.getWindowId() == null ^ this.getWindowId() == null)
return false;
if (other.getWindowId() != null && other.getWindowId().equals(this.getWindowId()) == false)
return false;
if (other.getWindowTaskId() == null ^ this.getWindowTaskId() == null)
return false;
if (other.getWindowTaskId() != null && other.getWindowTaskId().equals(this.getWindowTaskId()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getTaskArn() == null ^ this.getTaskArn() == null)
return false;
if (other.getTaskArn() != null && other.getTaskArn().equals(this.getTaskArn()) == false)
return false;
if (other.getServiceRoleArn() == null ^ this.getServiceRoleArn() == null)
return false;
if (other.getServiceRoleArn() != null && other.getServiceRoleArn().equals(this.getServiceRoleArn()) == false)
return false;
if (other.getTaskType() == null ^ this.getTaskType() == null)
return false;
if (other.getTaskType() != null && other.getTaskType().equals(this.getTaskType()) == false)
return false;
if (other.getTaskParameters() == null ^ this.getTaskParameters() == null)
return false;
if (other.getTaskParameters() != null && other.getTaskParameters().equals(this.getTaskParameters()) == false)
return false;
if (other.getTaskInvocationParameters() == null ^ this.getTaskInvocationParameters() == null)
return false;
if (other.getTaskInvocationParameters() != null && other.getTaskInvocationParameters().equals(this.getTaskInvocationParameters()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getLoggingInfo() == null ^ this.getLoggingInfo() == null)
return false;
if (other.getLoggingInfo() != null && other.getLoggingInfo().equals(this.getLoggingInfo()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getCutoffBehavior() == null ^ this.getCutoffBehavior() == null)
return false;
if (other.getCutoffBehavior() != null && other.getCutoffBehavior().equals(this.getCutoffBehavior()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWindowId() == null) ? 0 : getWindowId().hashCode());
hashCode = prime * hashCode + ((getWindowTaskId() == null) ? 0 : getWindowTaskId().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getTaskArn() == null) ? 0 : getTaskArn().hashCode());
hashCode = prime * hashCode + ((getServiceRoleArn() == null) ? 0 : getServiceRoleArn().hashCode());
hashCode = prime * hashCode + ((getTaskType() == null) ? 0 : getTaskType().hashCode());
hashCode = prime * hashCode + ((getTaskParameters() == null) ? 0 : getTaskParameters().hashCode());
hashCode = prime * hashCode + ((getTaskInvocationParameters() == null) ? 0 : getTaskInvocationParameters().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getLoggingInfo() == null) ? 0 : getLoggingInfo().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getCutoffBehavior() == null) ? 0 : getCutoffBehavior().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
return hashCode;
}
@Override
public GetMaintenanceWindowTaskResult clone() {
try {
return (GetMaintenanceWindowTaskResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}