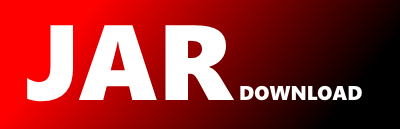
com.amazonaws.services.simplesystemsmanagement.model.MaintenanceWindowTask Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about a task defined for a maintenance window.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MaintenanceWindowTask implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the maintenance window where the task is registered.
*
*/
private String windowId;
/**
*
* The task ID.
*
*/
private String windowTaskId;
/**
*
* The resource that the task uses during execution. For RUN_COMMAND
and AUTOMATION
task
* types, TaskArn
is the Amazon Web Services Systems Manager (SSM document) name or ARN. For
* LAMBDA
tasks, it's the function name or ARN. For STEP_FUNCTIONS
tasks, it's the state
* machine ARN.
*
*/
private String taskArn;
/**
*
* The type of task.
*
*/
private String type;
/**
*
* The targets (either managed nodes or tags). Managed nodes are specified using
* Key=instanceids,Values=<instanceid1>,<instanceid2>
. Tags are specified using
* Key=<tag name>,Values=<tag value>
.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The parameters that should be passed to the task when it is run.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*/
private java.util.Map taskParameters;
/**
*
* The priority of the task in the maintenance window. The lower the number, the higher the priority. Tasks that
* have the same priority are scheduled in parallel.
*
*/
private Integer priority;
/**
*
* Information about an S3 bucket to write task-level logs to.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*/
private LoggingInfo loggingInfo;
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*/
private String serviceRoleArn;
/**
*
* The maximum number of targets this task can be run for, in parallel.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*/
private String maxConcurrency;
/**
*
* The maximum number of errors allowed before this task stops being scheduled.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*/
private String maxErrors;
/**
*
* The task name.
*
*/
private String name;
/**
*
* A description of the task.
*
*/
private String description;
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*/
private String cutoffBehavior;
/**
*
* The details for the CloudWatch alarm applied to your maintenance window task.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The ID of the maintenance window where the task is registered.
*
*
* @param windowId
* The ID of the maintenance window where the task is registered.
*/
public void setWindowId(String windowId) {
this.windowId = windowId;
}
/**
*
* The ID of the maintenance window where the task is registered.
*
*
* @return The ID of the maintenance window where the task is registered.
*/
public String getWindowId() {
return this.windowId;
}
/**
*
* The ID of the maintenance window where the task is registered.
*
*
* @param windowId
* The ID of the maintenance window where the task is registered.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withWindowId(String windowId) {
setWindowId(windowId);
return this;
}
/**
*
* The task ID.
*
*
* @param windowTaskId
* The task ID.
*/
public void setWindowTaskId(String windowTaskId) {
this.windowTaskId = windowTaskId;
}
/**
*
* The task ID.
*
*
* @return The task ID.
*/
public String getWindowTaskId() {
return this.windowTaskId;
}
/**
*
* The task ID.
*
*
* @param windowTaskId
* The task ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withWindowTaskId(String windowTaskId) {
setWindowTaskId(windowTaskId);
return this;
}
/**
*
* The resource that the task uses during execution. For RUN_COMMAND
and AUTOMATION
task
* types, TaskArn
is the Amazon Web Services Systems Manager (SSM document) name or ARN. For
* LAMBDA
tasks, it's the function name or ARN. For STEP_FUNCTIONS
tasks, it's the state
* machine ARN.
*
*
* @param taskArn
* The resource that the task uses during execution. For RUN_COMMAND
and AUTOMATION
* task types, TaskArn
is the Amazon Web Services Systems Manager (SSM document) name or ARN.
* For LAMBDA
tasks, it's the function name or ARN. For STEP_FUNCTIONS
tasks, it's
* the state machine ARN.
*/
public void setTaskArn(String taskArn) {
this.taskArn = taskArn;
}
/**
*
* The resource that the task uses during execution. For RUN_COMMAND
and AUTOMATION
task
* types, TaskArn
is the Amazon Web Services Systems Manager (SSM document) name or ARN. For
* LAMBDA
tasks, it's the function name or ARN. For STEP_FUNCTIONS
tasks, it's the state
* machine ARN.
*
*
* @return The resource that the task uses during execution. For RUN_COMMAND
and
* AUTOMATION
task types, TaskArn
is the Amazon Web Services Systems Manager (SSM
* document) name or ARN. For LAMBDA
tasks, it's the function name or ARN. For
* STEP_FUNCTIONS
tasks, it's the state machine ARN.
*/
public String getTaskArn() {
return this.taskArn;
}
/**
*
* The resource that the task uses during execution. For RUN_COMMAND
and AUTOMATION
task
* types, TaskArn
is the Amazon Web Services Systems Manager (SSM document) name or ARN. For
* LAMBDA
tasks, it's the function name or ARN. For STEP_FUNCTIONS
tasks, it's the state
* machine ARN.
*
*
* @param taskArn
* The resource that the task uses during execution. For RUN_COMMAND
and AUTOMATION
* task types, TaskArn
is the Amazon Web Services Systems Manager (SSM document) name or ARN.
* For LAMBDA
tasks, it's the function name or ARN. For STEP_FUNCTIONS
tasks, it's
* the state machine ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withTaskArn(String taskArn) {
setTaskArn(taskArn);
return this;
}
/**
*
* The type of task.
*
*
* @param type
* The type of task.
* @see MaintenanceWindowTaskType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of task.
*
*
* @return The type of task.
* @see MaintenanceWindowTaskType
*/
public String getType() {
return this.type;
}
/**
*
* The type of task.
*
*
* @param type
* The type of task.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskType
*/
public MaintenanceWindowTask withType(String type) {
setType(type);
return this;
}
/**
*
* The type of task.
*
*
* @param type
* The type of task.
* @see MaintenanceWindowTaskType
*/
public void setType(MaintenanceWindowTaskType type) {
withType(type);
}
/**
*
* The type of task.
*
*
* @param type
* The type of task.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskType
*/
public MaintenanceWindowTask withType(MaintenanceWindowTaskType type) {
this.type = type.toString();
return this;
}
/**
*
* The targets (either managed nodes or tags). Managed nodes are specified using
* Key=instanceids,Values=<instanceid1>,<instanceid2>
. Tags are specified using
* Key=<tag name>,Values=<tag value>
.
*
*
* @return The targets (either managed nodes or tags). Managed nodes are specified using
* Key=instanceids,Values=<instanceid1>,<instanceid2>
. Tags are specified using
* Key=<tag name>,Values=<tag value>
.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The targets (either managed nodes or tags). Managed nodes are specified using
* Key=instanceids,Values=<instanceid1>,<instanceid2>
. Tags are specified using
* Key=<tag name>,Values=<tag value>
.
*
*
* @param targets
* The targets (either managed nodes or tags). Managed nodes are specified using
* Key=instanceids,Values=<instanceid1>,<instanceid2>
. Tags are specified using
* Key=<tag name>,Values=<tag value>
.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The targets (either managed nodes or tags). Managed nodes are specified using
* Key=instanceids,Values=<instanceid1>,<instanceid2>
. Tags are specified using
* Key=<tag name>,Values=<tag value>
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The targets (either managed nodes or tags). Managed nodes are specified using
* Key=instanceids,Values=<instanceid1>,<instanceid2>
. Tags are specified using
* Key=<tag name>,Values=<tag value>
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The targets (either managed nodes or tags). Managed nodes are specified using
* Key=instanceids,Values=<instanceid1>,<instanceid2>
. Tags are specified using
* Key=<tag name>,Values=<tag value>
.
*
*
* @param targets
* The targets (either managed nodes or tags). Managed nodes are specified using
* Key=instanceids,Values=<instanceid1>,<instanceid2>
. Tags are specified using
* Key=<tag name>,Values=<tag value>
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The parameters that should be passed to the task when it is run.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @return The parameters that should be passed to the task when it is run.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure.
* For information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public java.util.Map getTaskParameters() {
return taskParameters;
}
/**
*
* The parameters that should be passed to the task when it is run.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param taskParameters
* The parameters that should be passed to the task when it is run.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public void setTaskParameters(java.util.Map taskParameters) {
this.taskParameters = taskParameters;
}
/**
*
* The parameters that should be passed to the task when it is run.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param taskParameters
* The parameters that should be passed to the task when it is run.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withTaskParameters(java.util.Map taskParameters) {
setTaskParameters(taskParameters);
return this;
}
/**
* Add a single TaskParameters entry
*
* @see MaintenanceWindowTask#withTaskParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask addTaskParametersEntry(String key, MaintenanceWindowTaskParameterValueExpression value) {
if (null == this.taskParameters) {
this.taskParameters = new java.util.HashMap();
}
if (this.taskParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.taskParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into TaskParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask clearTaskParametersEntries() {
this.taskParameters = null;
return this;
}
/**
*
* The priority of the task in the maintenance window. The lower the number, the higher the priority. Tasks that
* have the same priority are scheduled in parallel.
*
*
* @param priority
* The priority of the task in the maintenance window. The lower the number, the higher the priority. Tasks
* that have the same priority are scheduled in parallel.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* The priority of the task in the maintenance window. The lower the number, the higher the priority. Tasks that
* have the same priority are scheduled in parallel.
*
*
* @return The priority of the task in the maintenance window. The lower the number, the higher the priority. Tasks
* that have the same priority are scheduled in parallel.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* The priority of the task in the maintenance window. The lower the number, the higher the priority. Tasks that
* have the same priority are scheduled in parallel.
*
*
* @param priority
* The priority of the task in the maintenance window. The lower the number, the higher the priority. Tasks
* that have the same priority are scheduled in parallel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* Information about an S3 bucket to write task-level logs to.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param loggingInfo
* Information about an S3 bucket to write task-level logs to.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
* options in the TaskInvocationParameters
structure. For information about how Amazon Web
* Services Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*/
public void setLoggingInfo(LoggingInfo loggingInfo) {
this.loggingInfo = loggingInfo;
}
/**
*
* Information about an S3 bucket to write task-level logs to.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @return Information about an S3 bucket to write task-level logs to.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and
* OutputS3KeyPrefix
options in the TaskInvocationParameters
structure. For
* information about how Amazon Web Services Systems Manager handles these options for the supported
* maintenance window task types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public LoggingInfo getLoggingInfo() {
return this.loggingInfo;
}
/**
*
* Information about an S3 bucket to write task-level logs to.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param loggingInfo
* Information about an S3 bucket to write task-level logs to.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
* options in the TaskInvocationParameters
structure. For information about how Amazon Web
* Services Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withLoggingInfo(LoggingInfo loggingInfo) {
setLoggingInfo(loggingInfo);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*
* @param serviceRoleArn
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish
* Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*/
public void setServiceRoleArn(String serviceRoleArn) {
this.serviceRoleArn = serviceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*
* @return The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish
* Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*/
public String getServiceRoleArn() {
return this.serviceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*
* @param serviceRoleArn
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish
* Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withServiceRoleArn(String serviceRoleArn) {
setServiceRoleArn(serviceRoleArn);
return this;
}
/**
*
* The maximum number of targets this task can be run for, in parallel.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @param maxConcurrency
* The maximum number of targets this task can be run for, in parallel.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The maximum number of targets this task can be run for, in parallel.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @return The maximum number of targets this task can be run for, in parallel.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering
* or updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The maximum number of targets this task can be run for, in parallel.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @param maxConcurrency
* The maximum number of targets this task can be run for, in parallel.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The maximum number of errors allowed before this task stops being scheduled.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @param maxErrors
* The maximum number of errors allowed before this task stops being scheduled.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The maximum number of errors allowed before this task stops being scheduled.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @return The maximum number of errors allowed before this task stops being scheduled.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering
* or updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The maximum number of errors allowed before this task stops being scheduled.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @param maxErrors
* The maximum number of errors allowed before this task stops being scheduled.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The task name.
*
*
* @param name
* The task name.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The task name.
*
*
* @return The task name.
*/
public String getName() {
return this.name;
}
/**
*
* The task name.
*
*
* @param name
* The task name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withName(String name) {
setName(name);
return this;
}
/**
*
* A description of the task.
*
*
* @param description
* A description of the task.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the task.
*
*
* @return A description of the task.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the task.
*
*
* @param description
* A description of the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*
* @param cutoffBehavior
* The specification for whether tasks should continue to run after the cutoff time specified in the
* maintenance windows is reached.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public void setCutoffBehavior(String cutoffBehavior) {
this.cutoffBehavior = cutoffBehavior;
}
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*
* @return The specification for whether tasks should continue to run after the cutoff time specified in the
* maintenance windows is reached.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public String getCutoffBehavior() {
return this.cutoffBehavior;
}
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*
* @param cutoffBehavior
* The specification for whether tasks should continue to run after the cutoff time specified in the
* maintenance windows is reached.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public MaintenanceWindowTask withCutoffBehavior(String cutoffBehavior) {
setCutoffBehavior(cutoffBehavior);
return this;
}
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*
* @param cutoffBehavior
* The specification for whether tasks should continue to run after the cutoff time specified in the
* maintenance windows is reached.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public void setCutoffBehavior(MaintenanceWindowTaskCutoffBehavior cutoffBehavior) {
withCutoffBehavior(cutoffBehavior);
}
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*
* @param cutoffBehavior
* The specification for whether tasks should continue to run after the cutoff time specified in the
* maintenance windows is reached.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public MaintenanceWindowTask withCutoffBehavior(MaintenanceWindowTaskCutoffBehavior cutoffBehavior) {
this.cutoffBehavior = cutoffBehavior.toString();
return this;
}
/**
*
* The details for the CloudWatch alarm applied to your maintenance window task.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm applied to your maintenance window task.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm applied to your maintenance window task.
*
*
* @return The details for the CloudWatch alarm applied to your maintenance window task.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm applied to your maintenance window task.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm applied to your maintenance window task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MaintenanceWindowTask withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWindowId() != null)
sb.append("WindowId: ").append(getWindowId()).append(",");
if (getWindowTaskId() != null)
sb.append("WindowTaskId: ").append(getWindowTaskId()).append(",");
if (getTaskArn() != null)
sb.append("TaskArn: ").append(getTaskArn()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getTaskParameters() != null)
sb.append("TaskParameters: ").append("***Sensitive Data Redacted***").append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getLoggingInfo() != null)
sb.append("LoggingInfo: ").append(getLoggingInfo()).append(",");
if (getServiceRoleArn() != null)
sb.append("ServiceRoleArn: ").append(getServiceRoleArn()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getCutoffBehavior() != null)
sb.append("CutoffBehavior: ").append(getCutoffBehavior()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MaintenanceWindowTask == false)
return false;
MaintenanceWindowTask other = (MaintenanceWindowTask) obj;
if (other.getWindowId() == null ^ this.getWindowId() == null)
return false;
if (other.getWindowId() != null && other.getWindowId().equals(this.getWindowId()) == false)
return false;
if (other.getWindowTaskId() == null ^ this.getWindowTaskId() == null)
return false;
if (other.getWindowTaskId() != null && other.getWindowTaskId().equals(this.getWindowTaskId()) == false)
return false;
if (other.getTaskArn() == null ^ this.getTaskArn() == null)
return false;
if (other.getTaskArn() != null && other.getTaskArn().equals(this.getTaskArn()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getTaskParameters() == null ^ this.getTaskParameters() == null)
return false;
if (other.getTaskParameters() != null && other.getTaskParameters().equals(this.getTaskParameters()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getLoggingInfo() == null ^ this.getLoggingInfo() == null)
return false;
if (other.getLoggingInfo() != null && other.getLoggingInfo().equals(this.getLoggingInfo()) == false)
return false;
if (other.getServiceRoleArn() == null ^ this.getServiceRoleArn() == null)
return false;
if (other.getServiceRoleArn() != null && other.getServiceRoleArn().equals(this.getServiceRoleArn()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getCutoffBehavior() == null ^ this.getCutoffBehavior() == null)
return false;
if (other.getCutoffBehavior() != null && other.getCutoffBehavior().equals(this.getCutoffBehavior()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWindowId() == null) ? 0 : getWindowId().hashCode());
hashCode = prime * hashCode + ((getWindowTaskId() == null) ? 0 : getWindowTaskId().hashCode());
hashCode = prime * hashCode + ((getTaskArn() == null) ? 0 : getTaskArn().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getTaskParameters() == null) ? 0 : getTaskParameters().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getLoggingInfo() == null) ? 0 : getLoggingInfo().hashCode());
hashCode = prime * hashCode + ((getServiceRoleArn() == null) ? 0 : getServiceRoleArn().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getCutoffBehavior() == null) ? 0 : getCutoffBehavior().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
return hashCode;
}
@Override
public MaintenanceWindowTask clone() {
try {
return (MaintenanceWindowTask) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.MaintenanceWindowTaskMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}