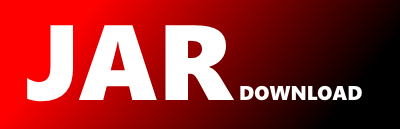
com.amazonaws.services.simplesystemsmanagement.model.OpsItem Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Operations engineers and IT professionals use Amazon Web Services Systems Manager OpsCenter to view, investigate, and
* remediate operational work items (OpsItems) impacting the performance and health of their Amazon Web Services
* resources. OpsCenter is integrated with Amazon EventBridge and Amazon CloudWatch. This means you can configure these
* services to automatically create an OpsItem in OpsCenter when a CloudWatch alarm enters the ALARM state or when
* EventBridge processes an event from any Amazon Web Services service that publishes events. Configuring Amazon
* CloudWatch alarms and EventBridge events to automatically create OpsItems allows you to quickly diagnose and
* remediate issues with Amazon Web Services resources from a single console.
*
*
* To help you diagnose issues, each OpsItem includes contextually relevant information such as the name and ID of the
* Amazon Web Services resource that generated the OpsItem, alarm or event details, alarm history, and an alarm timeline
* graph. For the Amazon Web Services resource, OpsCenter aggregates information from Config, CloudTrail logs, and
* EventBridge, so you don't have to navigate across multiple console pages during your investigation. For more
* information, see Amazon Web
* Services Systems Manager OpsCenter in the Amazon Web Services Systems Manager User Guide.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class OpsItem implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ARN of the Amazon Web Services account that created the OpsItem.
*
*/
private String createdBy;
/**
*
* The type of OpsItem. Systems Manager supports the following types of OpsItems:
*
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*
*/
private String opsItemType;
/**
*
* The date and time the OpsItem was created.
*
*/
private java.util.Date createdTime;
/**
*
* The OpsItem description.
*
*/
private String description;
/**
*
* The ARN of the Amazon Web Services account that last updated the OpsItem.
*
*/
private String lastModifiedBy;
/**
*
* The date and time the OpsItem was last updated.
*
*/
private java.util.Date lastModifiedTime;
/**
*
* The Amazon Resource Name (ARN) of an Amazon Simple Notification Service (Amazon SNS) topic where notifications
* are sent when this OpsItem is edited or changed.
*
*/
private com.amazonaws.internal.SdkInternalList notifications;
/**
*
* The importance of this OpsItem in relation to other OpsItems in the system.
*
*/
private Integer priority;
/**
*
* One or more OpsItems that share something in common with the current OpsItem. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*/
private com.amazonaws.internal.SdkInternalList relatedOpsItems;
/**
*
* The OpsItem status. Status can be Open
, In Progress
, or Resolved
. For more
* information, see Editing OpsItem details in the Amazon Web Services Systems Manager User Guide.
*
*/
private String status;
/**
*
* The ID of the OpsItem.
*
*/
private String opsItemId;
/**
*
* The version of this OpsItem. Each time the OpsItem is edited the version number increments by one.
*
*/
private String version;
/**
*
* A short heading that describes the nature of the OpsItem and the impacted resource.
*
*/
private String title;
/**
*
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager. The impacted resource is a subset of source.
*
*/
private String source;
/**
*
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you can
* specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You enter
* operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a maximum size
* of 20 KB.
*
*
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search access.
* Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that isn't
* searchable is only viewable by users who have access to the OpsItem (as provided by the GetOpsItem API
* operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request. Use the
* /aws/automations
key in OperationalData to associate an Automation runbook with the OpsItem. To view
* Amazon Web Services CLI example commands that use these keys, see Creating OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*
*/
private java.util.Map operationalData;
/**
*
* An OpsItem category. Category options include: Availability, Cost, Performance, Recovery, Security.
*
*/
private String category;
/**
*
* The severity of the OpsItem. Severity options range from 1 to 4.
*
*/
private String severity;
/**
*
* The time a runbook workflow started. Currently reported only for the OpsItem type /aws/changerequest
* .
*
*/
private java.util.Date actualStartTime;
/**
*
* The time a runbook workflow ended. Currently reported only for the OpsItem type /aws/changerequest
.
*
*/
private java.util.Date actualEndTime;
/**
*
* The time specified in a change request for a runbook workflow to start. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*/
private java.util.Date plannedStartTime;
/**
*
* The time specified in a change request for a runbook workflow to end. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*/
private java.util.Date plannedEndTime;
/**
*
* The OpsItem Amazon Resource Name (ARN).
*
*/
private String opsItemArn;
/**
*
* The ARN of the Amazon Web Services account that created the OpsItem.
*
*
* @param createdBy
* The ARN of the Amazon Web Services account that created the OpsItem.
*/
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
/**
*
* The ARN of the Amazon Web Services account that created the OpsItem.
*
*
* @return The ARN of the Amazon Web Services account that created the OpsItem.
*/
public String getCreatedBy() {
return this.createdBy;
}
/**
*
* The ARN of the Amazon Web Services account that created the OpsItem.
*
*
* @param createdBy
* The ARN of the Amazon Web Services account that created the OpsItem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withCreatedBy(String createdBy) {
setCreatedBy(createdBy);
return this;
}
/**
*
* The type of OpsItem. Systems Manager supports the following types of OpsItems:
*
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*
*
* @param opsItemType
* The type of OpsItem. Systems Manager supports the following types of OpsItems:
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*/
public void setOpsItemType(String opsItemType) {
this.opsItemType = opsItemType;
}
/**
*
* The type of OpsItem. Systems Manager supports the following types of OpsItems:
*
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*
*
* @return The type of OpsItem. Systems Manager supports the following types of OpsItems:
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*/
public String getOpsItemType() {
return this.opsItemType;
}
/**
*
* The type of OpsItem. Systems Manager supports the following types of OpsItems:
*
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
*
*
* @param opsItemType
* The type of OpsItem. Systems Manager supports the following types of OpsItems:
*
* -
*
* /aws/issue
*
*
* This type of OpsItem is used for default OpsItems created by OpsCenter.
*
*
* -
*
* /aws/changerequest
*
*
* This type of OpsItem is used by Change Manager for reviewing and approving or rejecting change requests.
*
*
* -
*
* /aws/insight
*
*
* This type of OpsItem is used by OpsCenter for aggregating and reporting on duplicate OpsItems.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withOpsItemType(String opsItemType) {
setOpsItemType(opsItemType);
return this;
}
/**
*
* The date and time the OpsItem was created.
*
*
* @param createdTime
* The date and time the OpsItem was created.
*/
public void setCreatedTime(java.util.Date createdTime) {
this.createdTime = createdTime;
}
/**
*
* The date and time the OpsItem was created.
*
*
* @return The date and time the OpsItem was created.
*/
public java.util.Date getCreatedTime() {
return this.createdTime;
}
/**
*
* The date and time the OpsItem was created.
*
*
* @param createdTime
* The date and time the OpsItem was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withCreatedTime(java.util.Date createdTime) {
setCreatedTime(createdTime);
return this;
}
/**
*
* The OpsItem description.
*
*
* @param description
* The OpsItem description.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The OpsItem description.
*
*
* @return The OpsItem description.
*/
public String getDescription() {
return this.description;
}
/**
*
* The OpsItem description.
*
*
* @param description
* The OpsItem description.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The ARN of the Amazon Web Services account that last updated the OpsItem.
*
*
* @param lastModifiedBy
* The ARN of the Amazon Web Services account that last updated the OpsItem.
*/
public void setLastModifiedBy(String lastModifiedBy) {
this.lastModifiedBy = lastModifiedBy;
}
/**
*
* The ARN of the Amazon Web Services account that last updated the OpsItem.
*
*
* @return The ARN of the Amazon Web Services account that last updated the OpsItem.
*/
public String getLastModifiedBy() {
return this.lastModifiedBy;
}
/**
*
* The ARN of the Amazon Web Services account that last updated the OpsItem.
*
*
* @param lastModifiedBy
* The ARN of the Amazon Web Services account that last updated the OpsItem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withLastModifiedBy(String lastModifiedBy) {
setLastModifiedBy(lastModifiedBy);
return this;
}
/**
*
* The date and time the OpsItem was last updated.
*
*
* @param lastModifiedTime
* The date and time the OpsItem was last updated.
*/
public void setLastModifiedTime(java.util.Date lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
/**
*
* The date and time the OpsItem was last updated.
*
*
* @return The date and time the OpsItem was last updated.
*/
public java.util.Date getLastModifiedTime() {
return this.lastModifiedTime;
}
/**
*
* The date and time the OpsItem was last updated.
*
*
* @param lastModifiedTime
* The date and time the OpsItem was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withLastModifiedTime(java.util.Date lastModifiedTime) {
setLastModifiedTime(lastModifiedTime);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an Amazon Simple Notification Service (Amazon SNS) topic where notifications
* are sent when this OpsItem is edited or changed.
*
*
* @return The Amazon Resource Name (ARN) of an Amazon Simple Notification Service (Amazon SNS) topic where
* notifications are sent when this OpsItem is edited or changed.
*/
public java.util.List getNotifications() {
if (notifications == null) {
notifications = new com.amazonaws.internal.SdkInternalList();
}
return notifications;
}
/**
*
* The Amazon Resource Name (ARN) of an Amazon Simple Notification Service (Amazon SNS) topic where notifications
* are sent when this OpsItem is edited or changed.
*
*
* @param notifications
* The Amazon Resource Name (ARN) of an Amazon Simple Notification Service (Amazon SNS) topic where
* notifications are sent when this OpsItem is edited or changed.
*/
public void setNotifications(java.util.Collection notifications) {
if (notifications == null) {
this.notifications = null;
return;
}
this.notifications = new com.amazonaws.internal.SdkInternalList(notifications);
}
/**
*
* The Amazon Resource Name (ARN) of an Amazon Simple Notification Service (Amazon SNS) topic where notifications
* are sent when this OpsItem is edited or changed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNotifications(java.util.Collection)} or {@link #withNotifications(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param notifications
* The Amazon Resource Name (ARN) of an Amazon Simple Notification Service (Amazon SNS) topic where
* notifications are sent when this OpsItem is edited or changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withNotifications(OpsItemNotification... notifications) {
if (this.notifications == null) {
setNotifications(new com.amazonaws.internal.SdkInternalList(notifications.length));
}
for (OpsItemNotification ele : notifications) {
this.notifications.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an Amazon Simple Notification Service (Amazon SNS) topic where notifications
* are sent when this OpsItem is edited or changed.
*
*
* @param notifications
* The Amazon Resource Name (ARN) of an Amazon Simple Notification Service (Amazon SNS) topic where
* notifications are sent when this OpsItem is edited or changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withNotifications(java.util.Collection notifications) {
setNotifications(notifications);
return this;
}
/**
*
* The importance of this OpsItem in relation to other OpsItems in the system.
*
*
* @param priority
* The importance of this OpsItem in relation to other OpsItems in the system.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* The importance of this OpsItem in relation to other OpsItems in the system.
*
*
* @return The importance of this OpsItem in relation to other OpsItems in the system.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* The importance of this OpsItem in relation to other OpsItems in the system.
*
*
* @param priority
* The importance of this OpsItem in relation to other OpsItems in the system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* One or more OpsItems that share something in common with the current OpsItem. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*
* @return One or more OpsItems that share something in common with the current OpsItem. For example, related
* OpsItems can include OpsItems with similar error messages, impacted resources, or statuses for the
* impacted resource.
*/
public java.util.List getRelatedOpsItems() {
if (relatedOpsItems == null) {
relatedOpsItems = new com.amazonaws.internal.SdkInternalList();
}
return relatedOpsItems;
}
/**
*
* One or more OpsItems that share something in common with the current OpsItem. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*
* @param relatedOpsItems
* One or more OpsItems that share something in common with the current OpsItem. For example, related
* OpsItems can include OpsItems with similar error messages, impacted resources, or statuses for the
* impacted resource.
*/
public void setRelatedOpsItems(java.util.Collection relatedOpsItems) {
if (relatedOpsItems == null) {
this.relatedOpsItems = null;
return;
}
this.relatedOpsItems = new com.amazonaws.internal.SdkInternalList(relatedOpsItems);
}
/**
*
* One or more OpsItems that share something in common with the current OpsItem. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRelatedOpsItems(java.util.Collection)} or {@link #withRelatedOpsItems(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param relatedOpsItems
* One or more OpsItems that share something in common with the current OpsItem. For example, related
* OpsItems can include OpsItems with similar error messages, impacted resources, or statuses for the
* impacted resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withRelatedOpsItems(RelatedOpsItem... relatedOpsItems) {
if (this.relatedOpsItems == null) {
setRelatedOpsItems(new com.amazonaws.internal.SdkInternalList(relatedOpsItems.length));
}
for (RelatedOpsItem ele : relatedOpsItems) {
this.relatedOpsItems.add(ele);
}
return this;
}
/**
*
* One or more OpsItems that share something in common with the current OpsItem. For example, related OpsItems can
* include OpsItems with similar error messages, impacted resources, or statuses for the impacted resource.
*
*
* @param relatedOpsItems
* One or more OpsItems that share something in common with the current OpsItem. For example, related
* OpsItems can include OpsItems with similar error messages, impacted resources, or statuses for the
* impacted resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withRelatedOpsItems(java.util.Collection relatedOpsItems) {
setRelatedOpsItems(relatedOpsItems);
return this;
}
/**
*
* The OpsItem status. Status can be Open
, In Progress
, or Resolved
. For more
* information, see Editing OpsItem details in the Amazon Web Services Systems Manager User Guide.
*
*
* @param status
* The OpsItem status. Status can be Open
, In Progress
, or Resolved
.
* For more information, see Editing OpsItem details in the Amazon Web Services Systems Manager User Guide.
* @see OpsItemStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The OpsItem status. Status can be Open
, In Progress
, or Resolved
. For more
* information, see Editing OpsItem details in the Amazon Web Services Systems Manager User Guide.
*
*
* @return The OpsItem status. Status can be Open
, In Progress
, or Resolved
.
* For more information, see Editing OpsItem details in the Amazon Web Services Systems Manager User Guide.
* @see OpsItemStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The OpsItem status. Status can be Open
, In Progress
, or Resolved
. For more
* information, see Editing OpsItem details in the Amazon Web Services Systems Manager User Guide.
*
*
* @param status
* The OpsItem status. Status can be Open
, In Progress
, or Resolved
.
* For more information, see Editing OpsItem details in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OpsItemStatus
*/
public OpsItem withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The OpsItem status. Status can be Open
, In Progress
, or Resolved
. For more
* information, see Editing OpsItem details in the Amazon Web Services Systems Manager User Guide.
*
*
* @param status
* The OpsItem status. Status can be Open
, In Progress
, or Resolved
.
* For more information, see Editing OpsItem details in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OpsItemStatus
*/
public OpsItem withStatus(OpsItemStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The ID of the OpsItem.
*
*
* @param opsItemId
* The ID of the OpsItem.
*/
public void setOpsItemId(String opsItemId) {
this.opsItemId = opsItemId;
}
/**
*
* The ID of the OpsItem.
*
*
* @return The ID of the OpsItem.
*/
public String getOpsItemId() {
return this.opsItemId;
}
/**
*
* The ID of the OpsItem.
*
*
* @param opsItemId
* The ID of the OpsItem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withOpsItemId(String opsItemId) {
setOpsItemId(opsItemId);
return this;
}
/**
*
* The version of this OpsItem. Each time the OpsItem is edited the version number increments by one.
*
*
* @param version
* The version of this OpsItem. Each time the OpsItem is edited the version number increments by one.
*/
public void setVersion(String version) {
this.version = version;
}
/**
*
* The version of this OpsItem. Each time the OpsItem is edited the version number increments by one.
*
*
* @return The version of this OpsItem. Each time the OpsItem is edited the version number increments by one.
*/
public String getVersion() {
return this.version;
}
/**
*
* The version of this OpsItem. Each time the OpsItem is edited the version number increments by one.
*
*
* @param version
* The version of this OpsItem. Each time the OpsItem is edited the version number increments by one.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withVersion(String version) {
setVersion(version);
return this;
}
/**
*
* A short heading that describes the nature of the OpsItem and the impacted resource.
*
*
* @param title
* A short heading that describes the nature of the OpsItem and the impacted resource.
*/
public void setTitle(String title) {
this.title = title;
}
/**
*
* A short heading that describes the nature of the OpsItem and the impacted resource.
*
*
* @return A short heading that describes the nature of the OpsItem and the impacted resource.
*/
public String getTitle() {
return this.title;
}
/**
*
* A short heading that describes the nature of the OpsItem and the impacted resource.
*
*
* @param title
* A short heading that describes the nature of the OpsItem and the impacted resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withTitle(String title) {
setTitle(title);
return this;
}
/**
*
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager. The impacted resource is a subset of source.
*
*
* @param source
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager. The impacted resource is a subset of
* source.
*/
public void setSource(String source) {
this.source = source;
}
/**
*
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager. The impacted resource is a subset of source.
*
*
* @return The origin of the OpsItem, such as Amazon EC2 or Systems Manager. The impacted resource is a subset of
* source.
*/
public String getSource() {
return this.source;
}
/**
*
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager. The impacted resource is a subset of source.
*
*
* @param source
* The origin of the OpsItem, such as Amazon EC2 or Systems Manager. The impacted resource is a subset of
* source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withSource(String source) {
setSource(source);
return this;
}
/**
*
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you can
* specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You enter
* operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a maximum size
* of 20 KB.
*
*
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search access.
* Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that isn't
* searchable is only viewable by users who have access to the OpsItem (as provided by the GetOpsItem API
* operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request. Use the
* /aws/automations
key in OperationalData to associate an Automation runbook with the OpsItem. To view
* Amazon Web Services CLI example commands that use these keys, see Creating OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*
*
* @return Operational data is custom data that provides useful reference details about the OpsItem. For example,
* you can specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You
* enter operational data as key-value pairs. The key has a maximum length of 128 characters. The value has
* a maximum size of 20 KB.
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search
* access. Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that
* isn't searchable is only viewable by users who have access to the OpsItem (as provided by the
* GetOpsItem API operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request.
* Use the /aws/automations
key in OperationalData to associate an Automation runbook with the
* OpsItem. To view Amazon Web Services CLI example commands that use these keys, see Creating OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*/
public java.util.Map getOperationalData() {
return operationalData;
}
/**
*
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you can
* specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You enter
* operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a maximum size
* of 20 KB.
*
*
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search access.
* Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that isn't
* searchable is only viewable by users who have access to the OpsItem (as provided by the GetOpsItem API
* operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request. Use the
* /aws/automations
key in OperationalData to associate an Automation runbook with the OpsItem. To view
* Amazon Web Services CLI example commands that use these keys, see Creating OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*
*
* @param operationalData
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you
* can specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You
* enter operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a
* maximum size of 20 KB.
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search
* access. Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that
* isn't searchable is only viewable by users who have access to the OpsItem (as provided by the
* GetOpsItem API operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request.
* Use the /aws/automations
key in OperationalData to associate an Automation runbook with the
* OpsItem. To view Amazon Web Services CLI example commands that use these keys, see Creating OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*/
public void setOperationalData(java.util.Map operationalData) {
this.operationalData = operationalData;
}
/**
*
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you can
* specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You enter
* operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a maximum size
* of 20 KB.
*
*
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search access.
* Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that isn't
* searchable is only viewable by users who have access to the OpsItem (as provided by the GetOpsItem API
* operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request. Use the
* /aws/automations
key in OperationalData to associate an Automation runbook with the OpsItem. To view
* Amazon Web Services CLI example commands that use these keys, see Creating OpsItems manually in the Amazon Web Services Systems Manager User Guide.
*
*
* @param operationalData
* Operational data is custom data that provides useful reference details about the OpsItem. For example, you
* can specify log files, error strings, license keys, troubleshooting tips, or other relevant data. You
* enter operational data as key-value pairs. The key has a maximum length of 128 characters. The value has a
* maximum size of 20 KB.
*
* Operational data keys can't begin with the following: amazon
, aws
,
* amzn
, ssm
, /amazon
, /aws
, /amzn
,
* /ssm
.
*
*
*
* You can choose to make the data searchable by other users in the account or you can restrict search
* access. Searchable data means that all users with access to the OpsItem Overview page (as provided by the
* DescribeOpsItems API operation) can view and search on the specified data. Operational data that
* isn't searchable is only viewable by users who have access to the OpsItem (as provided by the
* GetOpsItem API operation).
*
*
* Use the /aws/resources
key in OperationalData to specify a related resource in the request.
* Use the /aws/automations
key in OperationalData to associate an Automation runbook with the
* OpsItem. To view Amazon Web Services CLI example commands that use these keys, see Creating OpsItems manually in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withOperationalData(java.util.Map operationalData) {
setOperationalData(operationalData);
return this;
}
/**
* Add a single OperationalData entry
*
* @see OpsItem#withOperationalData
* @returns a reference to this object so that method calls can be chained together.
*/
public OpsItem addOperationalDataEntry(String key, OpsItemDataValue value) {
if (null == this.operationalData) {
this.operationalData = new java.util.HashMap();
}
if (this.operationalData.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.operationalData.put(key, value);
return this;
}
/**
* Removes all the entries added into OperationalData.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem clearOperationalDataEntries() {
this.operationalData = null;
return this;
}
/**
*
* An OpsItem category. Category options include: Availability, Cost, Performance, Recovery, Security.
*
*
* @param category
* An OpsItem category. Category options include: Availability, Cost, Performance, Recovery, Security.
*/
public void setCategory(String category) {
this.category = category;
}
/**
*
* An OpsItem category. Category options include: Availability, Cost, Performance, Recovery, Security.
*
*
* @return An OpsItem category. Category options include: Availability, Cost, Performance, Recovery, Security.
*/
public String getCategory() {
return this.category;
}
/**
*
* An OpsItem category. Category options include: Availability, Cost, Performance, Recovery, Security.
*
*
* @param category
* An OpsItem category. Category options include: Availability, Cost, Performance, Recovery, Security.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withCategory(String category) {
setCategory(category);
return this;
}
/**
*
* The severity of the OpsItem. Severity options range from 1 to 4.
*
*
* @param severity
* The severity of the OpsItem. Severity options range from 1 to 4.
*/
public void setSeverity(String severity) {
this.severity = severity;
}
/**
*
* The severity of the OpsItem. Severity options range from 1 to 4.
*
*
* @return The severity of the OpsItem. Severity options range from 1 to 4.
*/
public String getSeverity() {
return this.severity;
}
/**
*
* The severity of the OpsItem. Severity options range from 1 to 4.
*
*
* @param severity
* The severity of the OpsItem. Severity options range from 1 to 4.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withSeverity(String severity) {
setSeverity(severity);
return this;
}
/**
*
* The time a runbook workflow started. Currently reported only for the OpsItem type /aws/changerequest
* .
*
*
* @param actualStartTime
* The time a runbook workflow started. Currently reported only for the OpsItem type
* /aws/changerequest
.
*/
public void setActualStartTime(java.util.Date actualStartTime) {
this.actualStartTime = actualStartTime;
}
/**
*
* The time a runbook workflow started. Currently reported only for the OpsItem type /aws/changerequest
* .
*
*
* @return The time a runbook workflow started. Currently reported only for the OpsItem type
* /aws/changerequest
.
*/
public java.util.Date getActualStartTime() {
return this.actualStartTime;
}
/**
*
* The time a runbook workflow started. Currently reported only for the OpsItem type /aws/changerequest
* .
*
*
* @param actualStartTime
* The time a runbook workflow started. Currently reported only for the OpsItem type
* /aws/changerequest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withActualStartTime(java.util.Date actualStartTime) {
setActualStartTime(actualStartTime);
return this;
}
/**
*
* The time a runbook workflow ended. Currently reported only for the OpsItem type /aws/changerequest
.
*
*
* @param actualEndTime
* The time a runbook workflow ended. Currently reported only for the OpsItem type
* /aws/changerequest
.
*/
public void setActualEndTime(java.util.Date actualEndTime) {
this.actualEndTime = actualEndTime;
}
/**
*
* The time a runbook workflow ended. Currently reported only for the OpsItem type /aws/changerequest
.
*
*
* @return The time a runbook workflow ended. Currently reported only for the OpsItem type
* /aws/changerequest
.
*/
public java.util.Date getActualEndTime() {
return this.actualEndTime;
}
/**
*
* The time a runbook workflow ended. Currently reported only for the OpsItem type /aws/changerequest
.
*
*
* @param actualEndTime
* The time a runbook workflow ended. Currently reported only for the OpsItem type
* /aws/changerequest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withActualEndTime(java.util.Date actualEndTime) {
setActualEndTime(actualEndTime);
return this;
}
/**
*
* The time specified in a change request for a runbook workflow to start. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @param plannedStartTime
* The time specified in a change request for a runbook workflow to start. Currently supported only for the
* OpsItem type /aws/changerequest
.
*/
public void setPlannedStartTime(java.util.Date plannedStartTime) {
this.plannedStartTime = plannedStartTime;
}
/**
*
* The time specified in a change request for a runbook workflow to start. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @return The time specified in a change request for a runbook workflow to start. Currently supported only for the
* OpsItem type /aws/changerequest
.
*/
public java.util.Date getPlannedStartTime() {
return this.plannedStartTime;
}
/**
*
* The time specified in a change request for a runbook workflow to start. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @param plannedStartTime
* The time specified in a change request for a runbook workflow to start. Currently supported only for the
* OpsItem type /aws/changerequest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withPlannedStartTime(java.util.Date plannedStartTime) {
setPlannedStartTime(plannedStartTime);
return this;
}
/**
*
* The time specified in a change request for a runbook workflow to end. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @param plannedEndTime
* The time specified in a change request for a runbook workflow to end. Currently supported only for the
* OpsItem type /aws/changerequest
.
*/
public void setPlannedEndTime(java.util.Date plannedEndTime) {
this.plannedEndTime = plannedEndTime;
}
/**
*
* The time specified in a change request for a runbook workflow to end. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @return The time specified in a change request for a runbook workflow to end. Currently supported only for the
* OpsItem type /aws/changerequest
.
*/
public java.util.Date getPlannedEndTime() {
return this.plannedEndTime;
}
/**
*
* The time specified in a change request for a runbook workflow to end. Currently supported only for the OpsItem
* type /aws/changerequest
.
*
*
* @param plannedEndTime
* The time specified in a change request for a runbook workflow to end. Currently supported only for the
* OpsItem type /aws/changerequest
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withPlannedEndTime(java.util.Date plannedEndTime) {
setPlannedEndTime(plannedEndTime);
return this;
}
/**
*
* The OpsItem Amazon Resource Name (ARN).
*
*
* @param opsItemArn
* The OpsItem Amazon Resource Name (ARN).
*/
public void setOpsItemArn(String opsItemArn) {
this.opsItemArn = opsItemArn;
}
/**
*
* The OpsItem Amazon Resource Name (ARN).
*
*
* @return The OpsItem Amazon Resource Name (ARN).
*/
public String getOpsItemArn() {
return this.opsItemArn;
}
/**
*
* The OpsItem Amazon Resource Name (ARN).
*
*
* @param opsItemArn
* The OpsItem Amazon Resource Name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpsItem withOpsItemArn(String opsItemArn) {
setOpsItemArn(opsItemArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCreatedBy() != null)
sb.append("CreatedBy: ").append(getCreatedBy()).append(",");
if (getOpsItemType() != null)
sb.append("OpsItemType: ").append(getOpsItemType()).append(",");
if (getCreatedTime() != null)
sb.append("CreatedTime: ").append(getCreatedTime()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getLastModifiedBy() != null)
sb.append("LastModifiedBy: ").append(getLastModifiedBy()).append(",");
if (getLastModifiedTime() != null)
sb.append("LastModifiedTime: ").append(getLastModifiedTime()).append(",");
if (getNotifications() != null)
sb.append("Notifications: ").append(getNotifications()).append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getRelatedOpsItems() != null)
sb.append("RelatedOpsItems: ").append(getRelatedOpsItems()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getOpsItemId() != null)
sb.append("OpsItemId: ").append(getOpsItemId()).append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion()).append(",");
if (getTitle() != null)
sb.append("Title: ").append(getTitle()).append(",");
if (getSource() != null)
sb.append("Source: ").append(getSource()).append(",");
if (getOperationalData() != null)
sb.append("OperationalData: ").append(getOperationalData()).append(",");
if (getCategory() != null)
sb.append("Category: ").append(getCategory()).append(",");
if (getSeverity() != null)
sb.append("Severity: ").append(getSeverity()).append(",");
if (getActualStartTime() != null)
sb.append("ActualStartTime: ").append(getActualStartTime()).append(",");
if (getActualEndTime() != null)
sb.append("ActualEndTime: ").append(getActualEndTime()).append(",");
if (getPlannedStartTime() != null)
sb.append("PlannedStartTime: ").append(getPlannedStartTime()).append(",");
if (getPlannedEndTime() != null)
sb.append("PlannedEndTime: ").append(getPlannedEndTime()).append(",");
if (getOpsItemArn() != null)
sb.append("OpsItemArn: ").append(getOpsItemArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof OpsItem == false)
return false;
OpsItem other = (OpsItem) obj;
if (other.getCreatedBy() == null ^ this.getCreatedBy() == null)
return false;
if (other.getCreatedBy() != null && other.getCreatedBy().equals(this.getCreatedBy()) == false)
return false;
if (other.getOpsItemType() == null ^ this.getOpsItemType() == null)
return false;
if (other.getOpsItemType() != null && other.getOpsItemType().equals(this.getOpsItemType()) == false)
return false;
if (other.getCreatedTime() == null ^ this.getCreatedTime() == null)
return false;
if (other.getCreatedTime() != null && other.getCreatedTime().equals(this.getCreatedTime()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getLastModifiedBy() == null ^ this.getLastModifiedBy() == null)
return false;
if (other.getLastModifiedBy() != null && other.getLastModifiedBy().equals(this.getLastModifiedBy()) == false)
return false;
if (other.getLastModifiedTime() == null ^ this.getLastModifiedTime() == null)
return false;
if (other.getLastModifiedTime() != null && other.getLastModifiedTime().equals(this.getLastModifiedTime()) == false)
return false;
if (other.getNotifications() == null ^ this.getNotifications() == null)
return false;
if (other.getNotifications() != null && other.getNotifications().equals(this.getNotifications()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getRelatedOpsItems() == null ^ this.getRelatedOpsItems() == null)
return false;
if (other.getRelatedOpsItems() != null && other.getRelatedOpsItems().equals(this.getRelatedOpsItems()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getOpsItemId() == null ^ this.getOpsItemId() == null)
return false;
if (other.getOpsItemId() != null && other.getOpsItemId().equals(this.getOpsItemId()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
if (other.getTitle() == null ^ this.getTitle() == null)
return false;
if (other.getTitle() != null && other.getTitle().equals(this.getTitle()) == false)
return false;
if (other.getSource() == null ^ this.getSource() == null)
return false;
if (other.getSource() != null && other.getSource().equals(this.getSource()) == false)
return false;
if (other.getOperationalData() == null ^ this.getOperationalData() == null)
return false;
if (other.getOperationalData() != null && other.getOperationalData().equals(this.getOperationalData()) == false)
return false;
if (other.getCategory() == null ^ this.getCategory() == null)
return false;
if (other.getCategory() != null && other.getCategory().equals(this.getCategory()) == false)
return false;
if (other.getSeverity() == null ^ this.getSeverity() == null)
return false;
if (other.getSeverity() != null && other.getSeverity().equals(this.getSeverity()) == false)
return false;
if (other.getActualStartTime() == null ^ this.getActualStartTime() == null)
return false;
if (other.getActualStartTime() != null && other.getActualStartTime().equals(this.getActualStartTime()) == false)
return false;
if (other.getActualEndTime() == null ^ this.getActualEndTime() == null)
return false;
if (other.getActualEndTime() != null && other.getActualEndTime().equals(this.getActualEndTime()) == false)
return false;
if (other.getPlannedStartTime() == null ^ this.getPlannedStartTime() == null)
return false;
if (other.getPlannedStartTime() != null && other.getPlannedStartTime().equals(this.getPlannedStartTime()) == false)
return false;
if (other.getPlannedEndTime() == null ^ this.getPlannedEndTime() == null)
return false;
if (other.getPlannedEndTime() != null && other.getPlannedEndTime().equals(this.getPlannedEndTime()) == false)
return false;
if (other.getOpsItemArn() == null ^ this.getOpsItemArn() == null)
return false;
if (other.getOpsItemArn() != null && other.getOpsItemArn().equals(this.getOpsItemArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCreatedBy() == null) ? 0 : getCreatedBy().hashCode());
hashCode = prime * hashCode + ((getOpsItemType() == null) ? 0 : getOpsItemType().hashCode());
hashCode = prime * hashCode + ((getCreatedTime() == null) ? 0 : getCreatedTime().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getLastModifiedBy() == null) ? 0 : getLastModifiedBy().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTime() == null) ? 0 : getLastModifiedTime().hashCode());
hashCode = prime * hashCode + ((getNotifications() == null) ? 0 : getNotifications().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getRelatedOpsItems() == null) ? 0 : getRelatedOpsItems().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getOpsItemId() == null) ? 0 : getOpsItemId().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
hashCode = prime * hashCode + ((getTitle() == null) ? 0 : getTitle().hashCode());
hashCode = prime * hashCode + ((getSource() == null) ? 0 : getSource().hashCode());
hashCode = prime * hashCode + ((getOperationalData() == null) ? 0 : getOperationalData().hashCode());
hashCode = prime * hashCode + ((getCategory() == null) ? 0 : getCategory().hashCode());
hashCode = prime * hashCode + ((getSeverity() == null) ? 0 : getSeverity().hashCode());
hashCode = prime * hashCode + ((getActualStartTime() == null) ? 0 : getActualStartTime().hashCode());
hashCode = prime * hashCode + ((getActualEndTime() == null) ? 0 : getActualEndTime().hashCode());
hashCode = prime * hashCode + ((getPlannedStartTime() == null) ? 0 : getPlannedStartTime().hashCode());
hashCode = prime * hashCode + ((getPlannedEndTime() == null) ? 0 : getPlannedEndTime().hashCode());
hashCode = prime * hashCode + ((getOpsItemArn() == null) ? 0 : getOpsItemArn().hashCode());
return hashCode;
}
@Override
public OpsItem clone() {
try {
return (OpsItem) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.OpsItemMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}