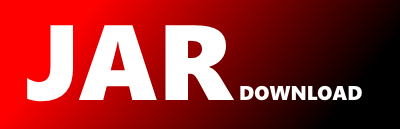
com.amazonaws.services.simplesystemsmanagement.model.ParameterMetadata Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Metadata includes information like the Amazon Resource Name (ARN) of the last user to update the parameter and the
* date and time the parameter was last used.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ParameterMetadata implements Serializable, Cloneable, StructuredPojo {
/**
*
* The parameter name.
*
*/
private String name;
/**
*
* The (ARN) of the last user to update the parameter.
*
*/
private String aRN;
/**
*
* The type of parameter. Valid parameter types include the following: String
, StringList
,
* and SecureString
.
*
*/
private String type;
/**
*
* The alias of the Key Management Service (KMS) key used to encrypt the parameter. Applies to
* SecureString
parameters only.
*
*/
private String keyId;
/**
*
* Date the parameter was last changed or updated.
*
*/
private java.util.Date lastModifiedDate;
/**
*
* Amazon Resource Name (ARN) of the Amazon Web Services user who last changed the parameter.
*
*/
private String lastModifiedUser;
/**
*
* Description of the parameter actions.
*
*/
private String description;
/**
*
* A parameter name can include only the following letters and symbols.
*
*
* a-zA-Z0-9_.-
*
*/
private String allowedPattern;
/**
*
* The parameter version.
*
*/
private Long version;
/**
*
* The parameter tier.
*
*/
private String tier;
/**
*
* A list of policies associated with a parameter.
*
*/
private com.amazonaws.internal.SdkInternalList policies;
/**
*
* The data type of the parameter, such as text
or aws:ec2:image
. The default is
* text
.
*
*/
private String dataType;
/**
*
* The parameter name.
*
*
* @param name
* The parameter name.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The parameter name.
*
*
* @return The parameter name.
*/
public String getName() {
return this.name;
}
/**
*
* The parameter name.
*
*
* @param name
* The parameter name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withName(String name) {
setName(name);
return this;
}
/**
*
* The (ARN) of the last user to update the parameter.
*
*
* @param aRN
* The (ARN) of the last user to update the parameter.
*/
public void setARN(String aRN) {
this.aRN = aRN;
}
/**
*
* The (ARN) of the last user to update the parameter.
*
*
* @return The (ARN) of the last user to update the parameter.
*/
public String getARN() {
return this.aRN;
}
/**
*
* The (ARN) of the last user to update the parameter.
*
*
* @param aRN
* The (ARN) of the last user to update the parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withARN(String aRN) {
setARN(aRN);
return this;
}
/**
*
* The type of parameter. Valid parameter types include the following: String
, StringList
,
* and SecureString
.
*
*
* @param type
* The type of parameter. Valid parameter types include the following: String
,
* StringList
, and SecureString
.
* @see ParameterType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of parameter. Valid parameter types include the following: String
, StringList
,
* and SecureString
.
*
*
* @return The type of parameter. Valid parameter types include the following: String
,
* StringList
, and SecureString
.
* @see ParameterType
*/
public String getType() {
return this.type;
}
/**
*
* The type of parameter. Valid parameter types include the following: String
, StringList
,
* and SecureString
.
*
*
* @param type
* The type of parameter. Valid parameter types include the following: String
,
* StringList
, and SecureString
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParameterType
*/
public ParameterMetadata withType(String type) {
setType(type);
return this;
}
/**
*
* The type of parameter. Valid parameter types include the following: String
, StringList
,
* and SecureString
.
*
*
* @param type
* The type of parameter. Valid parameter types include the following: String
,
* StringList
, and SecureString
.
* @see ParameterType
*/
public void setType(ParameterType type) {
withType(type);
}
/**
*
* The type of parameter. Valid parameter types include the following: String
, StringList
,
* and SecureString
.
*
*
* @param type
* The type of parameter. Valid parameter types include the following: String
,
* StringList
, and SecureString
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParameterType
*/
public ParameterMetadata withType(ParameterType type) {
this.type = type.toString();
return this;
}
/**
*
* The alias of the Key Management Service (KMS) key used to encrypt the parameter. Applies to
* SecureString
parameters only.
*
*
* @param keyId
* The alias of the Key Management Service (KMS) key used to encrypt the parameter. Applies to
* SecureString
parameters only.
*/
public void setKeyId(String keyId) {
this.keyId = keyId;
}
/**
*
* The alias of the Key Management Service (KMS) key used to encrypt the parameter. Applies to
* SecureString
parameters only.
*
*
* @return The alias of the Key Management Service (KMS) key used to encrypt the parameter. Applies to
* SecureString
parameters only.
*/
public String getKeyId() {
return this.keyId;
}
/**
*
* The alias of the Key Management Service (KMS) key used to encrypt the parameter. Applies to
* SecureString
parameters only.
*
*
* @param keyId
* The alias of the Key Management Service (KMS) key used to encrypt the parameter. Applies to
* SecureString
parameters only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withKeyId(String keyId) {
setKeyId(keyId);
return this;
}
/**
*
* Date the parameter was last changed or updated.
*
*
* @param lastModifiedDate
* Date the parameter was last changed or updated.
*/
public void setLastModifiedDate(java.util.Date lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
/**
*
* Date the parameter was last changed or updated.
*
*
* @return Date the parameter was last changed or updated.
*/
public java.util.Date getLastModifiedDate() {
return this.lastModifiedDate;
}
/**
*
* Date the parameter was last changed or updated.
*
*
* @param lastModifiedDate
* Date the parameter was last changed or updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withLastModifiedDate(java.util.Date lastModifiedDate) {
setLastModifiedDate(lastModifiedDate);
return this;
}
/**
*
* Amazon Resource Name (ARN) of the Amazon Web Services user who last changed the parameter.
*
*
* @param lastModifiedUser
* Amazon Resource Name (ARN) of the Amazon Web Services user who last changed the parameter.
*/
public void setLastModifiedUser(String lastModifiedUser) {
this.lastModifiedUser = lastModifiedUser;
}
/**
*
* Amazon Resource Name (ARN) of the Amazon Web Services user who last changed the parameter.
*
*
* @return Amazon Resource Name (ARN) of the Amazon Web Services user who last changed the parameter.
*/
public String getLastModifiedUser() {
return this.lastModifiedUser;
}
/**
*
* Amazon Resource Name (ARN) of the Amazon Web Services user who last changed the parameter.
*
*
* @param lastModifiedUser
* Amazon Resource Name (ARN) of the Amazon Web Services user who last changed the parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withLastModifiedUser(String lastModifiedUser) {
setLastModifiedUser(lastModifiedUser);
return this;
}
/**
*
* Description of the parameter actions.
*
*
* @param description
* Description of the parameter actions.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* Description of the parameter actions.
*
*
* @return Description of the parameter actions.
*/
public String getDescription() {
return this.description;
}
/**
*
* Description of the parameter actions.
*
*
* @param description
* Description of the parameter actions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* A parameter name can include only the following letters and symbols.
*
*
* a-zA-Z0-9_.-
*
*
* @param allowedPattern
* A parameter name can include only the following letters and symbols.
*
* a-zA-Z0-9_.-
*/
public void setAllowedPattern(String allowedPattern) {
this.allowedPattern = allowedPattern;
}
/**
*
* A parameter name can include only the following letters and symbols.
*
*
* a-zA-Z0-9_.-
*
*
* @return A parameter name can include only the following letters and symbols.
*
* a-zA-Z0-9_.-
*/
public String getAllowedPattern() {
return this.allowedPattern;
}
/**
*
* A parameter name can include only the following letters and symbols.
*
*
* a-zA-Z0-9_.-
*
*
* @param allowedPattern
* A parameter name can include only the following letters and symbols.
*
* a-zA-Z0-9_.-
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withAllowedPattern(String allowedPattern) {
setAllowedPattern(allowedPattern);
return this;
}
/**
*
* The parameter version.
*
*
* @param version
* The parameter version.
*/
public void setVersion(Long version) {
this.version = version;
}
/**
*
* The parameter version.
*
*
* @return The parameter version.
*/
public Long getVersion() {
return this.version;
}
/**
*
* The parameter version.
*
*
* @param version
* The parameter version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withVersion(Long version) {
setVersion(version);
return this;
}
/**
*
* The parameter tier.
*
*
* @param tier
* The parameter tier.
* @see ParameterTier
*/
public void setTier(String tier) {
this.tier = tier;
}
/**
*
* The parameter tier.
*
*
* @return The parameter tier.
* @see ParameterTier
*/
public String getTier() {
return this.tier;
}
/**
*
* The parameter tier.
*
*
* @param tier
* The parameter tier.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParameterTier
*/
public ParameterMetadata withTier(String tier) {
setTier(tier);
return this;
}
/**
*
* The parameter tier.
*
*
* @param tier
* The parameter tier.
* @see ParameterTier
*/
public void setTier(ParameterTier tier) {
withTier(tier);
}
/**
*
* The parameter tier.
*
*
* @param tier
* The parameter tier.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParameterTier
*/
public ParameterMetadata withTier(ParameterTier tier) {
this.tier = tier.toString();
return this;
}
/**
*
* A list of policies associated with a parameter.
*
*
* @return A list of policies associated with a parameter.
*/
public java.util.List getPolicies() {
if (policies == null) {
policies = new com.amazonaws.internal.SdkInternalList();
}
return policies;
}
/**
*
* A list of policies associated with a parameter.
*
*
* @param policies
* A list of policies associated with a parameter.
*/
public void setPolicies(java.util.Collection policies) {
if (policies == null) {
this.policies = null;
return;
}
this.policies = new com.amazonaws.internal.SdkInternalList(policies);
}
/**
*
* A list of policies associated with a parameter.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPolicies(java.util.Collection)} or {@link #withPolicies(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param policies
* A list of policies associated with a parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withPolicies(ParameterInlinePolicy... policies) {
if (this.policies == null) {
setPolicies(new com.amazonaws.internal.SdkInternalList(policies.length));
}
for (ParameterInlinePolicy ele : policies) {
this.policies.add(ele);
}
return this;
}
/**
*
* A list of policies associated with a parameter.
*
*
* @param policies
* A list of policies associated with a parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withPolicies(java.util.Collection policies) {
setPolicies(policies);
return this;
}
/**
*
* The data type of the parameter, such as text
or aws:ec2:image
. The default is
* text
.
*
*
* @param dataType
* The data type of the parameter, such as text
or aws:ec2:image
. The default is
* text
.
*/
public void setDataType(String dataType) {
this.dataType = dataType;
}
/**
*
* The data type of the parameter, such as text
or aws:ec2:image
. The default is
* text
.
*
*
* @return The data type of the parameter, such as text
or aws:ec2:image
. The default is
* text
.
*/
public String getDataType() {
return this.dataType;
}
/**
*
* The data type of the parameter, such as text
or aws:ec2:image
. The default is
* text
.
*
*
* @param dataType
* The data type of the parameter, such as text
or aws:ec2:image
. The default is
* text
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParameterMetadata withDataType(String dataType) {
setDataType(dataType);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getARN() != null)
sb.append("ARN: ").append(getARN()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getKeyId() != null)
sb.append("KeyId: ").append(getKeyId()).append(",");
if (getLastModifiedDate() != null)
sb.append("LastModifiedDate: ").append(getLastModifiedDate()).append(",");
if (getLastModifiedUser() != null)
sb.append("LastModifiedUser: ").append(getLastModifiedUser()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getAllowedPattern() != null)
sb.append("AllowedPattern: ").append(getAllowedPattern()).append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion()).append(",");
if (getTier() != null)
sb.append("Tier: ").append(getTier()).append(",");
if (getPolicies() != null)
sb.append("Policies: ").append(getPolicies()).append(",");
if (getDataType() != null)
sb.append("DataType: ").append(getDataType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ParameterMetadata == false)
return false;
ParameterMetadata other = (ParameterMetadata) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getARN() == null ^ this.getARN() == null)
return false;
if (other.getARN() != null && other.getARN().equals(this.getARN()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getKeyId() == null ^ this.getKeyId() == null)
return false;
if (other.getKeyId() != null && other.getKeyId().equals(this.getKeyId()) == false)
return false;
if (other.getLastModifiedDate() == null ^ this.getLastModifiedDate() == null)
return false;
if (other.getLastModifiedDate() != null && other.getLastModifiedDate().equals(this.getLastModifiedDate()) == false)
return false;
if (other.getLastModifiedUser() == null ^ this.getLastModifiedUser() == null)
return false;
if (other.getLastModifiedUser() != null && other.getLastModifiedUser().equals(this.getLastModifiedUser()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getAllowedPattern() == null ^ this.getAllowedPattern() == null)
return false;
if (other.getAllowedPattern() != null && other.getAllowedPattern().equals(this.getAllowedPattern()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
if (other.getTier() == null ^ this.getTier() == null)
return false;
if (other.getTier() != null && other.getTier().equals(this.getTier()) == false)
return false;
if (other.getPolicies() == null ^ this.getPolicies() == null)
return false;
if (other.getPolicies() != null && other.getPolicies().equals(this.getPolicies()) == false)
return false;
if (other.getDataType() == null ^ this.getDataType() == null)
return false;
if (other.getDataType() != null && other.getDataType().equals(this.getDataType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getARN() == null) ? 0 : getARN().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getKeyId() == null) ? 0 : getKeyId().hashCode());
hashCode = prime * hashCode + ((getLastModifiedDate() == null) ? 0 : getLastModifiedDate().hashCode());
hashCode = prime * hashCode + ((getLastModifiedUser() == null) ? 0 : getLastModifiedUser().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getAllowedPattern() == null) ? 0 : getAllowedPattern().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
hashCode = prime * hashCode + ((getTier() == null) ? 0 : getTier().hashCode());
hashCode = prime * hashCode + ((getPolicies() == null) ? 0 : getPolicies().hashCode());
hashCode = prime * hashCode + ((getDataType() == null) ? 0 : getDataType().hashCode());
return hashCode;
}
@Override
public ParameterMetadata clone() {
try {
return (ParameterMetadata) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.ParameterMetadataMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}