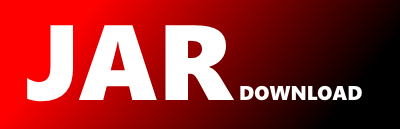
com.amazonaws.services.simplesystemsmanagement.model.PatchComplianceData Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about the state of a patch on a particular managed node as it relates to the patch baseline used to patch
* the node.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PatchComplianceData implements Serializable, Cloneable, StructuredPojo {
/**
*
* The title of the patch.
*
*/
private String title;
/**
*
* The operating system-specific ID of the patch.
*
*/
private String kBId;
/**
*
* The classification of the patch, such as SecurityUpdates
, Updates
, and
* CriticalUpdates
.
*
*/
private String classification;
/**
*
* The severity of the patch such as Critical
, Important
, and Moderate
.
*
*/
private String severity;
/**
*
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
*
*/
private String state;
/**
*
* The date/time the patch was installed on the managed node. Not all operating systems provide this level of
* information.
*
*/
private java.util.Date installedTime;
/**
*
* The IDs of one or more Common Vulnerabilities and Exposure (CVE) issues that are resolved by the patch.
*
*
*
* Currently, CVE ID values are reported only for patches with a status of Missing
or
* Failed
.
*
*
*/
private String cVEIds;
/**
*
* The title of the patch.
*
*
* @param title
* The title of the patch.
*/
public void setTitle(String title) {
this.title = title;
}
/**
*
* The title of the patch.
*
*
* @return The title of the patch.
*/
public String getTitle() {
return this.title;
}
/**
*
* The title of the patch.
*
*
* @param title
* The title of the patch.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PatchComplianceData withTitle(String title) {
setTitle(title);
return this;
}
/**
*
* The operating system-specific ID of the patch.
*
*
* @param kBId
* The operating system-specific ID of the patch.
*/
public void setKBId(String kBId) {
this.kBId = kBId;
}
/**
*
* The operating system-specific ID of the patch.
*
*
* @return The operating system-specific ID of the patch.
*/
public String getKBId() {
return this.kBId;
}
/**
*
* The operating system-specific ID of the patch.
*
*
* @param kBId
* The operating system-specific ID of the patch.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PatchComplianceData withKBId(String kBId) {
setKBId(kBId);
return this;
}
/**
*
* The classification of the patch, such as SecurityUpdates
, Updates
, and
* CriticalUpdates
.
*
*
* @param classification
* The classification of the patch, such as SecurityUpdates
, Updates
, and
* CriticalUpdates
.
*/
public void setClassification(String classification) {
this.classification = classification;
}
/**
*
* The classification of the patch, such as SecurityUpdates
, Updates
, and
* CriticalUpdates
.
*
*
* @return The classification of the patch, such as SecurityUpdates
, Updates
, and
* CriticalUpdates
.
*/
public String getClassification() {
return this.classification;
}
/**
*
* The classification of the patch, such as SecurityUpdates
, Updates
, and
* CriticalUpdates
.
*
*
* @param classification
* The classification of the patch, such as SecurityUpdates
, Updates
, and
* CriticalUpdates
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PatchComplianceData withClassification(String classification) {
setClassification(classification);
return this;
}
/**
*
* The severity of the patch such as Critical
, Important
, and Moderate
.
*
*
* @param severity
* The severity of the patch such as Critical
, Important
, and Moderate
* .
*/
public void setSeverity(String severity) {
this.severity = severity;
}
/**
*
* The severity of the patch such as Critical
, Important
, and Moderate
.
*
*
* @return The severity of the patch such as Critical
, Important
, and
* Moderate
.
*/
public String getSeverity() {
return this.severity;
}
/**
*
* The severity of the patch such as Critical
, Important
, and Moderate
.
*
*
* @param severity
* The severity of the patch such as Critical
, Important
, and Moderate
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PatchComplianceData withSeverity(String severity) {
setSeverity(severity);
return this;
}
/**
*
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
*
*
* @param state
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
* @see PatchComplianceDataState
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
*
*
* @return The state of the patch on the managed node, such as INSTALLED or FAILED.
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
* @see PatchComplianceDataState
*/
public String getState() {
return this.state;
}
/**
*
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
*
*
* @param state
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PatchComplianceDataState
*/
public PatchComplianceData withState(String state) {
setState(state);
return this;
}
/**
*
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
*
*
* @param state
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
* @see PatchComplianceDataState
*/
public void setState(PatchComplianceDataState state) {
withState(state);
}
/**
*
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
*
*
* @param state
* The state of the patch on the managed node, such as INSTALLED or FAILED.
*
* For descriptions of each patch state, see About patch compliance in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PatchComplianceDataState
*/
public PatchComplianceData withState(PatchComplianceDataState state) {
this.state = state.toString();
return this;
}
/**
*
* The date/time the patch was installed on the managed node. Not all operating systems provide this level of
* information.
*
*
* @param installedTime
* The date/time the patch was installed on the managed node. Not all operating systems provide this level of
* information.
*/
public void setInstalledTime(java.util.Date installedTime) {
this.installedTime = installedTime;
}
/**
*
* The date/time the patch was installed on the managed node. Not all operating systems provide this level of
* information.
*
*
* @return The date/time the patch was installed on the managed node. Not all operating systems provide this level
* of information.
*/
public java.util.Date getInstalledTime() {
return this.installedTime;
}
/**
*
* The date/time the patch was installed on the managed node. Not all operating systems provide this level of
* information.
*
*
* @param installedTime
* The date/time the patch was installed on the managed node. Not all operating systems provide this level of
* information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PatchComplianceData withInstalledTime(java.util.Date installedTime) {
setInstalledTime(installedTime);
return this;
}
/**
*
* The IDs of one or more Common Vulnerabilities and Exposure (CVE) issues that are resolved by the patch.
*
*
*
* Currently, CVE ID values are reported only for patches with a status of Missing
or
* Failed
.
*
*
*
* @param cVEIds
* The IDs of one or more Common Vulnerabilities and Exposure (CVE) issues that are resolved by the
* patch.
*
* Currently, CVE ID values are reported only for patches with a status of Missing
or
* Failed
.
*
*/
public void setCVEIds(String cVEIds) {
this.cVEIds = cVEIds;
}
/**
*
* The IDs of one or more Common Vulnerabilities and Exposure (CVE) issues that are resolved by the patch.
*
*
*
* Currently, CVE ID values are reported only for patches with a status of Missing
or
* Failed
.
*
*
*
* @return The IDs of one or more Common Vulnerabilities and Exposure (CVE) issues that are resolved by the
* patch.
*
* Currently, CVE ID values are reported only for patches with a status of Missing
or
* Failed
.
*
*/
public String getCVEIds() {
return this.cVEIds;
}
/**
*
* The IDs of one or more Common Vulnerabilities and Exposure (CVE) issues that are resolved by the patch.
*
*
*
* Currently, CVE ID values are reported only for patches with a status of Missing
or
* Failed
.
*
*
*
* @param cVEIds
* The IDs of one or more Common Vulnerabilities and Exposure (CVE) issues that are resolved by the
* patch.
*
* Currently, CVE ID values are reported only for patches with a status of Missing
or
* Failed
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PatchComplianceData withCVEIds(String cVEIds) {
setCVEIds(cVEIds);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTitle() != null)
sb.append("Title: ").append(getTitle()).append(",");
if (getKBId() != null)
sb.append("KBId: ").append(getKBId()).append(",");
if (getClassification() != null)
sb.append("Classification: ").append(getClassification()).append(",");
if (getSeverity() != null)
sb.append("Severity: ").append(getSeverity()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getInstalledTime() != null)
sb.append("InstalledTime: ").append(getInstalledTime()).append(",");
if (getCVEIds() != null)
sb.append("CVEIds: ").append(getCVEIds());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PatchComplianceData == false)
return false;
PatchComplianceData other = (PatchComplianceData) obj;
if (other.getTitle() == null ^ this.getTitle() == null)
return false;
if (other.getTitle() != null && other.getTitle().equals(this.getTitle()) == false)
return false;
if (other.getKBId() == null ^ this.getKBId() == null)
return false;
if (other.getKBId() != null && other.getKBId().equals(this.getKBId()) == false)
return false;
if (other.getClassification() == null ^ this.getClassification() == null)
return false;
if (other.getClassification() != null && other.getClassification().equals(this.getClassification()) == false)
return false;
if (other.getSeverity() == null ^ this.getSeverity() == null)
return false;
if (other.getSeverity() != null && other.getSeverity().equals(this.getSeverity()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getInstalledTime() == null ^ this.getInstalledTime() == null)
return false;
if (other.getInstalledTime() != null && other.getInstalledTime().equals(this.getInstalledTime()) == false)
return false;
if (other.getCVEIds() == null ^ this.getCVEIds() == null)
return false;
if (other.getCVEIds() != null && other.getCVEIds().equals(this.getCVEIds()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTitle() == null) ? 0 : getTitle().hashCode());
hashCode = prime * hashCode + ((getKBId() == null) ? 0 : getKBId().hashCode());
hashCode = prime * hashCode + ((getClassification() == null) ? 0 : getClassification().hashCode());
hashCode = prime * hashCode + ((getSeverity() == null) ? 0 : getSeverity().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getInstalledTime() == null) ? 0 : getInstalledTime().hashCode());
hashCode = prime * hashCode + ((getCVEIds() == null) ? 0 : getCVEIds().hashCode());
return hashCode;
}
@Override
public PatchComplianceData clone() {
try {
return (PatchComplianceData) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.PatchComplianceDataMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}