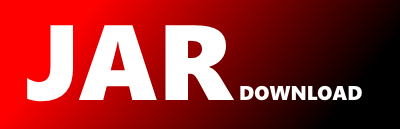
com.amazonaws.services.simplesystemsmanagement.model.PutComplianceItemsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutComplianceItemsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Specify an ID for this resource. For a managed node, this is the node ID.
*
*/
private String resourceId;
/**
*
* Specify the type of resource. ManagedInstance
is currently the only supported resource type.
*
*/
private String resourceType;
/**
*
* Specify the compliance type. For example, specify Association (for a State Manager association), Patch, or
* Custom:string
.
*
*/
private String complianceType;
/**
*
* A summary of the call execution that includes an execution ID, the type of execution (for example,
* Command
), and the date/time of the execution using a datetime object that is saved in the following
* format: yyyy-MM-dd'T'HH:mm:ss'Z'
*
*/
private ComplianceExecutionSummary executionSummary;
/**
*
* Information about the compliance as defined by the resource type. For example, for a patch compliance type,
* Items
includes information about the PatchSeverity, Classification, and so on.
*
*/
private com.amazonaws.internal.SdkInternalList items;
/**
*
* MD5 or SHA-256 content hash. The content hash is used to determine if existing information should be overwritten
* or ignored. If the content hashes match, the request to put compliance information is ignored.
*
*/
private String itemContentHash;
/**
*
* The mode for uploading compliance items. You can specify COMPLETE
or PARTIAL
. In
* COMPLETE
mode, the system overwrites all existing compliance information for the resource. You must
* provide a full list of compliance items each time you send the request.
*
*
* In PARTIAL
mode, the system overwrites compliance information for a specific association. The
* association must be configured with SyncCompliance
set to MANUAL
. By default, all
* requests use COMPLETE
mode.
*
*
*
* This attribute is only valid for association compliance.
*
*
*/
private String uploadType;
/**
*
* Specify an ID for this resource. For a managed node, this is the node ID.
*
*
* @param resourceId
* Specify an ID for this resource. For a managed node, this is the node ID.
*/
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
/**
*
* Specify an ID for this resource. For a managed node, this is the node ID.
*
*
* @return Specify an ID for this resource. For a managed node, this is the node ID.
*/
public String getResourceId() {
return this.resourceId;
}
/**
*
* Specify an ID for this resource. For a managed node, this is the node ID.
*
*
* @param resourceId
* Specify an ID for this resource. For a managed node, this is the node ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutComplianceItemsRequest withResourceId(String resourceId) {
setResourceId(resourceId);
return this;
}
/**
*
* Specify the type of resource. ManagedInstance
is currently the only supported resource type.
*
*
* @param resourceType
* Specify the type of resource. ManagedInstance
is currently the only supported resource type.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* Specify the type of resource. ManagedInstance
is currently the only supported resource type.
*
*
* @return Specify the type of resource. ManagedInstance
is currently the only supported resource type.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* Specify the type of resource. ManagedInstance
is currently the only supported resource type.
*
*
* @param resourceType
* Specify the type of resource. ManagedInstance
is currently the only supported resource type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutComplianceItemsRequest withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* Specify the compliance type. For example, specify Association (for a State Manager association), Patch, or
* Custom:string
.
*
*
* @param complianceType
* Specify the compliance type. For example, specify Association (for a State Manager association), Patch, or
* Custom:string
.
*/
public void setComplianceType(String complianceType) {
this.complianceType = complianceType;
}
/**
*
* Specify the compliance type. For example, specify Association (for a State Manager association), Patch, or
* Custom:string
.
*
*
* @return Specify the compliance type. For example, specify Association (for a State Manager association), Patch,
* or Custom:string
.
*/
public String getComplianceType() {
return this.complianceType;
}
/**
*
* Specify the compliance type. For example, specify Association (for a State Manager association), Patch, or
* Custom:string
.
*
*
* @param complianceType
* Specify the compliance type. For example, specify Association (for a State Manager association), Patch, or
* Custom:string
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutComplianceItemsRequest withComplianceType(String complianceType) {
setComplianceType(complianceType);
return this;
}
/**
*
* A summary of the call execution that includes an execution ID, the type of execution (for example,
* Command
), and the date/time of the execution using a datetime object that is saved in the following
* format: yyyy-MM-dd'T'HH:mm:ss'Z'
*
*
* @param executionSummary
* A summary of the call execution that includes an execution ID, the type of execution (for example,
* Command
), and the date/time of the execution using a datetime object that is saved in the
* following format: yyyy-MM-dd'T'HH:mm:ss'Z'
*/
public void setExecutionSummary(ComplianceExecutionSummary executionSummary) {
this.executionSummary = executionSummary;
}
/**
*
* A summary of the call execution that includes an execution ID, the type of execution (for example,
* Command
), and the date/time of the execution using a datetime object that is saved in the following
* format: yyyy-MM-dd'T'HH:mm:ss'Z'
*
*
* @return A summary of the call execution that includes an execution ID, the type of execution (for example,
* Command
), and the date/time of the execution using a datetime object that is saved in the
* following format: yyyy-MM-dd'T'HH:mm:ss'Z'
*/
public ComplianceExecutionSummary getExecutionSummary() {
return this.executionSummary;
}
/**
*
* A summary of the call execution that includes an execution ID, the type of execution (for example,
* Command
), and the date/time of the execution using a datetime object that is saved in the following
* format: yyyy-MM-dd'T'HH:mm:ss'Z'
*
*
* @param executionSummary
* A summary of the call execution that includes an execution ID, the type of execution (for example,
* Command
), and the date/time of the execution using a datetime object that is saved in the
* following format: yyyy-MM-dd'T'HH:mm:ss'Z'
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutComplianceItemsRequest withExecutionSummary(ComplianceExecutionSummary executionSummary) {
setExecutionSummary(executionSummary);
return this;
}
/**
*
* Information about the compliance as defined by the resource type. For example, for a patch compliance type,
* Items
includes information about the PatchSeverity, Classification, and so on.
*
*
* @return Information about the compliance as defined by the resource type. For example, for a patch compliance
* type, Items
includes information about the PatchSeverity, Classification, and so on.
*/
public java.util.List getItems() {
if (items == null) {
items = new com.amazonaws.internal.SdkInternalList();
}
return items;
}
/**
*
* Information about the compliance as defined by the resource type. For example, for a patch compliance type,
* Items
includes information about the PatchSeverity, Classification, and so on.
*
*
* @param items
* Information about the compliance as defined by the resource type. For example, for a patch compliance
* type, Items
includes information about the PatchSeverity, Classification, and so on.
*/
public void setItems(java.util.Collection items) {
if (items == null) {
this.items = null;
return;
}
this.items = new com.amazonaws.internal.SdkInternalList(items);
}
/**
*
* Information about the compliance as defined by the resource type. For example, for a patch compliance type,
* Items
includes information about the PatchSeverity, Classification, and so on.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setItems(java.util.Collection)} or {@link #withItems(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param items
* Information about the compliance as defined by the resource type. For example, for a patch compliance
* type, Items
includes information about the PatchSeverity, Classification, and so on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutComplianceItemsRequest withItems(ComplianceItemEntry... items) {
if (this.items == null) {
setItems(new com.amazonaws.internal.SdkInternalList(items.length));
}
for (ComplianceItemEntry ele : items) {
this.items.add(ele);
}
return this;
}
/**
*
* Information about the compliance as defined by the resource type. For example, for a patch compliance type,
* Items
includes information about the PatchSeverity, Classification, and so on.
*
*
* @param items
* Information about the compliance as defined by the resource type. For example, for a patch compliance
* type, Items
includes information about the PatchSeverity, Classification, and so on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutComplianceItemsRequest withItems(java.util.Collection items) {
setItems(items);
return this;
}
/**
*
* MD5 or SHA-256 content hash. The content hash is used to determine if existing information should be overwritten
* or ignored. If the content hashes match, the request to put compliance information is ignored.
*
*
* @param itemContentHash
* MD5 or SHA-256 content hash. The content hash is used to determine if existing information should be
* overwritten or ignored. If the content hashes match, the request to put compliance information is ignored.
*/
public void setItemContentHash(String itemContentHash) {
this.itemContentHash = itemContentHash;
}
/**
*
* MD5 or SHA-256 content hash. The content hash is used to determine if existing information should be overwritten
* or ignored. If the content hashes match, the request to put compliance information is ignored.
*
*
* @return MD5 or SHA-256 content hash. The content hash is used to determine if existing information should be
* overwritten or ignored. If the content hashes match, the request to put compliance information is
* ignored.
*/
public String getItemContentHash() {
return this.itemContentHash;
}
/**
*
* MD5 or SHA-256 content hash. The content hash is used to determine if existing information should be overwritten
* or ignored. If the content hashes match, the request to put compliance information is ignored.
*
*
* @param itemContentHash
* MD5 or SHA-256 content hash. The content hash is used to determine if existing information should be
* overwritten or ignored. If the content hashes match, the request to put compliance information is ignored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutComplianceItemsRequest withItemContentHash(String itemContentHash) {
setItemContentHash(itemContentHash);
return this;
}
/**
*
* The mode for uploading compliance items. You can specify COMPLETE
or PARTIAL
. In
* COMPLETE
mode, the system overwrites all existing compliance information for the resource. You must
* provide a full list of compliance items each time you send the request.
*
*
* In PARTIAL
mode, the system overwrites compliance information for a specific association. The
* association must be configured with SyncCompliance
set to MANUAL
. By default, all
* requests use COMPLETE
mode.
*
*
*
* This attribute is only valid for association compliance.
*
*
*
* @param uploadType
* The mode for uploading compliance items. You can specify COMPLETE
or PARTIAL
. In
* COMPLETE
mode, the system overwrites all existing compliance information for the resource.
* You must provide a full list of compliance items each time you send the request.
*
* In PARTIAL
mode, the system overwrites compliance information for a specific association. The
* association must be configured with SyncCompliance
set to MANUAL
. By default,
* all requests use COMPLETE
mode.
*
*
*
* This attribute is only valid for association compliance.
*
* @see ComplianceUploadType
*/
public void setUploadType(String uploadType) {
this.uploadType = uploadType;
}
/**
*
* The mode for uploading compliance items. You can specify COMPLETE
or PARTIAL
. In
* COMPLETE
mode, the system overwrites all existing compliance information for the resource. You must
* provide a full list of compliance items each time you send the request.
*
*
* In PARTIAL
mode, the system overwrites compliance information for a specific association. The
* association must be configured with SyncCompliance
set to MANUAL
. By default, all
* requests use COMPLETE
mode.
*
*
*
* This attribute is only valid for association compliance.
*
*
*
* @return The mode for uploading compliance items. You can specify COMPLETE
or PARTIAL
.
* In COMPLETE
mode, the system overwrites all existing compliance information for the
* resource. You must provide a full list of compliance items each time you send the request.
*
* In PARTIAL
mode, the system overwrites compliance information for a specific association.
* The association must be configured with SyncCompliance
set to MANUAL
. By
* default, all requests use COMPLETE
mode.
*
*
*
* This attribute is only valid for association compliance.
*
* @see ComplianceUploadType
*/
public String getUploadType() {
return this.uploadType;
}
/**
*
* The mode for uploading compliance items. You can specify COMPLETE
or PARTIAL
. In
* COMPLETE
mode, the system overwrites all existing compliance information for the resource. You must
* provide a full list of compliance items each time you send the request.
*
*
* In PARTIAL
mode, the system overwrites compliance information for a specific association. The
* association must be configured with SyncCompliance
set to MANUAL
. By default, all
* requests use COMPLETE
mode.
*
*
*
* This attribute is only valid for association compliance.
*
*
*
* @param uploadType
* The mode for uploading compliance items. You can specify COMPLETE
or PARTIAL
. In
* COMPLETE
mode, the system overwrites all existing compliance information for the resource.
* You must provide a full list of compliance items each time you send the request.
*
* In PARTIAL
mode, the system overwrites compliance information for a specific association. The
* association must be configured with SyncCompliance
set to MANUAL
. By default,
* all requests use COMPLETE
mode.
*
*
*
* This attribute is only valid for association compliance.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComplianceUploadType
*/
public PutComplianceItemsRequest withUploadType(String uploadType) {
setUploadType(uploadType);
return this;
}
/**
*
* The mode for uploading compliance items. You can specify COMPLETE
or PARTIAL
. In
* COMPLETE
mode, the system overwrites all existing compliance information for the resource. You must
* provide a full list of compliance items each time you send the request.
*
*
* In PARTIAL
mode, the system overwrites compliance information for a specific association. The
* association must be configured with SyncCompliance
set to MANUAL
. By default, all
* requests use COMPLETE
mode.
*
*
*
* This attribute is only valid for association compliance.
*
*
*
* @param uploadType
* The mode for uploading compliance items. You can specify COMPLETE
or PARTIAL
. In
* COMPLETE
mode, the system overwrites all existing compliance information for the resource.
* You must provide a full list of compliance items each time you send the request.
*
* In PARTIAL
mode, the system overwrites compliance information for a specific association. The
* association must be configured with SyncCompliance
set to MANUAL
. By default,
* all requests use COMPLETE
mode.
*
*
*
* This attribute is only valid for association compliance.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComplianceUploadType
*/
public PutComplianceItemsRequest withUploadType(ComplianceUploadType uploadType) {
this.uploadType = uploadType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceId() != null)
sb.append("ResourceId: ").append(getResourceId()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getComplianceType() != null)
sb.append("ComplianceType: ").append(getComplianceType()).append(",");
if (getExecutionSummary() != null)
sb.append("ExecutionSummary: ").append(getExecutionSummary()).append(",");
if (getItems() != null)
sb.append("Items: ").append(getItems()).append(",");
if (getItemContentHash() != null)
sb.append("ItemContentHash: ").append(getItemContentHash()).append(",");
if (getUploadType() != null)
sb.append("UploadType: ").append(getUploadType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutComplianceItemsRequest == false)
return false;
PutComplianceItemsRequest other = (PutComplianceItemsRequest) obj;
if (other.getResourceId() == null ^ this.getResourceId() == null)
return false;
if (other.getResourceId() != null && other.getResourceId().equals(this.getResourceId()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getComplianceType() == null ^ this.getComplianceType() == null)
return false;
if (other.getComplianceType() != null && other.getComplianceType().equals(this.getComplianceType()) == false)
return false;
if (other.getExecutionSummary() == null ^ this.getExecutionSummary() == null)
return false;
if (other.getExecutionSummary() != null && other.getExecutionSummary().equals(this.getExecutionSummary()) == false)
return false;
if (other.getItems() == null ^ this.getItems() == null)
return false;
if (other.getItems() != null && other.getItems().equals(this.getItems()) == false)
return false;
if (other.getItemContentHash() == null ^ this.getItemContentHash() == null)
return false;
if (other.getItemContentHash() != null && other.getItemContentHash().equals(this.getItemContentHash()) == false)
return false;
if (other.getUploadType() == null ^ this.getUploadType() == null)
return false;
if (other.getUploadType() != null && other.getUploadType().equals(this.getUploadType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceId() == null) ? 0 : getResourceId().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getComplianceType() == null) ? 0 : getComplianceType().hashCode());
hashCode = prime * hashCode + ((getExecutionSummary() == null) ? 0 : getExecutionSummary().hashCode());
hashCode = prime * hashCode + ((getItems() == null) ? 0 : getItems().hashCode());
hashCode = prime * hashCode + ((getItemContentHash() == null) ? 0 : getItemContentHash().hashCode());
hashCode = prime * hashCode + ((getUploadType() == null) ? 0 : getUploadType().hashCode());
return hashCode;
}
@Override
public PutComplianceItemsRequest clone() {
return (PutComplianceItemsRequest) super.clone();
}
}