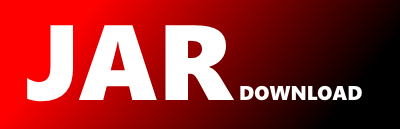
com.amazonaws.services.simplesystemsmanagement.model.PutParameterRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutParameterRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The fully qualified name of the parameter that you want to add to the system.
*
*
*
* You can't enter the Amazon Resource Name (ARN) for a parameter, only the parameter name itself.
*
*
*
* The fully qualified name includes the complete hierarchy of the parameter path and name. For parameters in a
* hierarchy, you must include a leading forward slash character (/) when you create or reference a parameter. For
* example: /Dev/DBServer/MySQL/db-string13
*
*
* Naming Constraints:
*
*
* -
*
* Parameter names are case sensitive.
*
*
* -
*
* A parameter name must be unique within an Amazon Web Services Region
*
*
* -
*
* A parameter name can't be prefixed with "aws
" or "ssm
" (case-insensitive).
*
*
* -
*
* Parameter names can include only the following symbols and letters: a-zA-Z0-9_.-
*
*
* In addition, the slash character ( / ) is used to delineate hierarchies in parameter names. For example:
* /Dev/Production/East/Project-ABC/MyParameter
*
*
* -
*
* A parameter name can't include spaces.
*
*
* -
*
* Parameter hierarchies are limited to a maximum depth of fifteen levels.
*
*
*
*
* For additional information about valid values for parameter names, see Creating
* Systems Manager parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
* The maximum length constraint of 2048 characters listed below includes 1037 characters reserved for internal use
* by Systems Manager. The maximum length for a parameter name that you create is 1011 characters. This includes the
* characters in the ARN that precede the name you specify, such as
* arn:aws:ssm:us-east-2:111122223333:parameter/
.
*
*
*/
private String name;
/**
*
* Information about the parameter that you want to add to the system. Optional but recommended.
*
*
*
* Don't enter personally identifiable information in this field.
*
*
*/
private String description;
/**
*
* The parameter value that you want to add to the system. Standard parameters have a value limit of 4 KB. Advanced
* parameters have a value limit of 8 KB.
*
*
*
* Parameters can't be referenced or nested in the values of other parameters. You can't include {{}}
* or {{ssm:parameter-name}}
in a parameter value.
*
*
*/
private String value;
/**
*
* The type of parameter that you want to add to the system.
*
*
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or special
* character to escape items in the list. If you have a parameter value that requires a comma, then use the
* String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type when
* creating a parameter.
*
*
*/
private String type;
/**
*
* The Key Management Service (KMS) ID that you want to use to encrypt a parameter. Use a custom key for better
* security. Required for parameters that use the SecureString
data type.
*
*
* If you don't specify a key ID, the system uses the default key associated with your Amazon Web Services account
* which is not as secure as using a custom key.
*
*
* -
*
* To use a custom KMS key, choose the SecureString
data type with the Key ID
parameter.
*
*
*
*/
private String keyId;
/**
*
* Overwrite an existing parameter. The default value is false
.
*
*/
private Boolean overwrite;
/**
*
* A regular expression used to validate the parameter value. For example, for String types with values restricted
* to numbers, you can specify the following: AllowedPattern=^\d+$
*
*/
private String allowedPattern;
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a Systems Manager parameter to identify
* the type of resource to which it applies, the environment, or the type of configuration data referenced by the
* parameter. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Resource,Value=S3bucket
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=ParameterType,Value=LicenseKey
*
*
*
*
*
* To add tags to an existing Systems Manager parameter, use the AddTagsToResource operation.
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The parameter tier to assign to a parameter.
*
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a content
* size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of 10,000 standard
* parameters for each Region in an Amazon Web Services account. Standard parameters are offered at no additional
* cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You can
* create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account. Advanced
* parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced parameter
* to a standard parameter. Reverting an advanced parameter to a standard parameter would result in data loss
* because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would also remove any
* policies attached to the parameter. Lastly, advanced parameters use a different form of encryption than standard
* parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced parameter,
* you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you specify
* a tier in the request, Parameter Store creates or updates the parameter according to that request. However, if
* you don't specify a tier in a request, Parameter Store assigns the tier based on the current Parameter Store
* default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in the
* standard-parameter tier. If one or more options requiring an advanced parameter are included in the request,
* Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web Services
* Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
*
*/
private String tier;
/**
*
* One or more policies to apply to a parameter. This operation takes a JSON array. Parameter Store, a capability of
* Amazon Web Services Systems Manager supports the following policy types:
*
*
* Expiration: This policy deletes the parameter after it expires. When you create the policy, you specify the
* expiration date. You can update the expiration date and time by updating the policy. Updating the
* parameter doesn't affect the expiration date and time. When the expiration time is reached, Parameter
* Store deletes the parameter.
*
*
* ExpirationNotification: This policy initiates an event in Amazon CloudWatch Events that notifies you about the
* expiration. By using this policy, you can receive notification before or after the expiration time is reached, in
* units of days or hours.
*
*
* NoChangeNotification: This policy initiates a CloudWatch Events event if a parameter hasn't been modified for a
* specified period of time. This policy type is useful when, for example, a secret needs to be changed within a
* period of time, but it hasn't been changed.
*
*
* All existing policies are preserved until you send new policies or an empty policy. For more information about
* parameter policies, see Assigning
* parameter policies.
*
*/
private String policies;
/**
*
* The data type for a String
parameter. Supported data types include plain text and Amazon Machine
* Image (AMI) IDs.
*
*
* The following data type values are supported.
*
*
* -
*
* text
*
*
* -
*
* aws:ec2:image
*
*
* -
*
* aws:ssm:integration
*
*
*
*
* When you create a String
parameter and specify aws:ec2:image
, Amazon Web Services
* Systems Manager validates the parameter value is in the required format, such as
* ami-12345abcdeEXAMPLE
, and that the specified AMI is available in your Amazon Web Services account.
*
*
*
* If the action is successful, the service sends back an HTTP 200 response which indicates a successful
* PutParameter
call for all cases except for data type aws:ec2:image
. If you call
* PutParameter
with aws:ec2:image
data type, a successful HTTP 200 response does not
* guarantee that your parameter was successfully created or updated. The aws:ec2:image
value is
* validated asynchronously, and the PutParameter
call returns before the validation is complete. If
* you submit an invalid AMI value, the PutParameter operation will return success, but the asynchronous validation
* will fail and the parameter will not be created or updated. To monitor whether your aws:ec2:image
* parameters are created successfully, see Setting up
* notifications or trigger actions based on Parameter Store events. For more information about AMI format
* validation , see Native
* parameter support for Amazon Machine Image IDs.
*
*
*/
private String dataType;
/**
*
* The fully qualified name of the parameter that you want to add to the system.
*
*
*
* You can't enter the Amazon Resource Name (ARN) for a parameter, only the parameter name itself.
*
*
*
* The fully qualified name includes the complete hierarchy of the parameter path and name. For parameters in a
* hierarchy, you must include a leading forward slash character (/) when you create or reference a parameter. For
* example: /Dev/DBServer/MySQL/db-string13
*
*
* Naming Constraints:
*
*
* -
*
* Parameter names are case sensitive.
*
*
* -
*
* A parameter name must be unique within an Amazon Web Services Region
*
*
* -
*
* A parameter name can't be prefixed with "aws
" or "ssm
" (case-insensitive).
*
*
* -
*
* Parameter names can include only the following symbols and letters: a-zA-Z0-9_.-
*
*
* In addition, the slash character ( / ) is used to delineate hierarchies in parameter names. For example:
* /Dev/Production/East/Project-ABC/MyParameter
*
*
* -
*
* A parameter name can't include spaces.
*
*
* -
*
* Parameter hierarchies are limited to a maximum depth of fifteen levels.
*
*
*
*
* For additional information about valid values for parameter names, see Creating
* Systems Manager parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
* The maximum length constraint of 2048 characters listed below includes 1037 characters reserved for internal use
* by Systems Manager. The maximum length for a parameter name that you create is 1011 characters. This includes the
* characters in the ARN that precede the name you specify, such as
* arn:aws:ssm:us-east-2:111122223333:parameter/
.
*
*
*
* @param name
* The fully qualified name of the parameter that you want to add to the system.
*
* You can't enter the Amazon Resource Name (ARN) for a parameter, only the parameter name itself.
*
*
*
* The fully qualified name includes the complete hierarchy of the parameter path and name. For parameters in
* a hierarchy, you must include a leading forward slash character (/) when you create or reference a
* parameter. For example: /Dev/DBServer/MySQL/db-string13
*
*
* Naming Constraints:
*
*
* -
*
* Parameter names are case sensitive.
*
*
* -
*
* A parameter name must be unique within an Amazon Web Services Region
*
*
* -
*
* A parameter name can't be prefixed with "aws
" or "ssm
" (case-insensitive).
*
*
* -
*
* Parameter names can include only the following symbols and letters: a-zA-Z0-9_.-
*
*
* In addition, the slash character ( / ) is used to delineate hierarchies in parameter names. For example:
* /Dev/Production/East/Project-ABC/MyParameter
*
*
* -
*
* A parameter name can't include spaces.
*
*
* -
*
* Parameter hierarchies are limited to a maximum depth of fifteen levels.
*
*
*
*
* For additional information about valid values for parameter names, see Creating Systems Manager parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
* The maximum length constraint of 2048 characters listed below includes 1037 characters reserved for
* internal use by Systems Manager. The maximum length for a parameter name that you create is 1011
* characters. This includes the characters in the ARN that precede the name you specify, such as
* arn:aws:ssm:us-east-2:111122223333:parameter/
.
*
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The fully qualified name of the parameter that you want to add to the system.
*
*
*
* You can't enter the Amazon Resource Name (ARN) for a parameter, only the parameter name itself.
*
*
*
* The fully qualified name includes the complete hierarchy of the parameter path and name. For parameters in a
* hierarchy, you must include a leading forward slash character (/) when you create or reference a parameter. For
* example: /Dev/DBServer/MySQL/db-string13
*
*
* Naming Constraints:
*
*
* -
*
* Parameter names are case sensitive.
*
*
* -
*
* A parameter name must be unique within an Amazon Web Services Region
*
*
* -
*
* A parameter name can't be prefixed with "aws
" or "ssm
" (case-insensitive).
*
*
* -
*
* Parameter names can include only the following symbols and letters: a-zA-Z0-9_.-
*
*
* In addition, the slash character ( / ) is used to delineate hierarchies in parameter names. For example:
* /Dev/Production/East/Project-ABC/MyParameter
*
*
* -
*
* A parameter name can't include spaces.
*
*
* -
*
* Parameter hierarchies are limited to a maximum depth of fifteen levels.
*
*
*
*
* For additional information about valid values for parameter names, see Creating
* Systems Manager parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
* The maximum length constraint of 2048 characters listed below includes 1037 characters reserved for internal use
* by Systems Manager. The maximum length for a parameter name that you create is 1011 characters. This includes the
* characters in the ARN that precede the name you specify, such as
* arn:aws:ssm:us-east-2:111122223333:parameter/
.
*
*
*
* @return The fully qualified name of the parameter that you want to add to the system.
*
* You can't enter the Amazon Resource Name (ARN) for a parameter, only the parameter name itself.
*
*
*
* The fully qualified name includes the complete hierarchy of the parameter path and name. For parameters
* in a hierarchy, you must include a leading forward slash character (/) when you create or reference a
* parameter. For example: /Dev/DBServer/MySQL/db-string13
*
*
* Naming Constraints:
*
*
* -
*
* Parameter names are case sensitive.
*
*
* -
*
* A parameter name must be unique within an Amazon Web Services Region
*
*
* -
*
* A parameter name can't be prefixed with "aws
" or "ssm
" (case-insensitive).
*
*
* -
*
* Parameter names can include only the following symbols and letters: a-zA-Z0-9_.-
*
*
* In addition, the slash character ( / ) is used to delineate hierarchies in parameter names. For example:
* /Dev/Production/East/Project-ABC/MyParameter
*
*
* -
*
* A parameter name can't include spaces.
*
*
* -
*
* Parameter hierarchies are limited to a maximum depth of fifteen levels.
*
*
*
*
* For additional information about valid values for parameter names, see Creating Systems Manager parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
* The maximum length constraint of 2048 characters listed below includes 1037 characters reserved for
* internal use by Systems Manager. The maximum length for a parameter name that you create is 1011
* characters. This includes the characters in the ARN that precede the name you specify, such as
* arn:aws:ssm:us-east-2:111122223333:parameter/
.
*
*/
public String getName() {
return this.name;
}
/**
*
* The fully qualified name of the parameter that you want to add to the system.
*
*
*
* You can't enter the Amazon Resource Name (ARN) for a parameter, only the parameter name itself.
*
*
*
* The fully qualified name includes the complete hierarchy of the parameter path and name. For parameters in a
* hierarchy, you must include a leading forward slash character (/) when you create or reference a parameter. For
* example: /Dev/DBServer/MySQL/db-string13
*
*
* Naming Constraints:
*
*
* -
*
* Parameter names are case sensitive.
*
*
* -
*
* A parameter name must be unique within an Amazon Web Services Region
*
*
* -
*
* A parameter name can't be prefixed with "aws
" or "ssm
" (case-insensitive).
*
*
* -
*
* Parameter names can include only the following symbols and letters: a-zA-Z0-9_.-
*
*
* In addition, the slash character ( / ) is used to delineate hierarchies in parameter names. For example:
* /Dev/Production/East/Project-ABC/MyParameter
*
*
* -
*
* A parameter name can't include spaces.
*
*
* -
*
* Parameter hierarchies are limited to a maximum depth of fifteen levels.
*
*
*
*
* For additional information about valid values for parameter names, see Creating
* Systems Manager parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
* The maximum length constraint of 2048 characters listed below includes 1037 characters reserved for internal use
* by Systems Manager. The maximum length for a parameter name that you create is 1011 characters. This includes the
* characters in the ARN that precede the name you specify, such as
* arn:aws:ssm:us-east-2:111122223333:parameter/
.
*
*
*
* @param name
* The fully qualified name of the parameter that you want to add to the system.
*
* You can't enter the Amazon Resource Name (ARN) for a parameter, only the parameter name itself.
*
*
*
* The fully qualified name includes the complete hierarchy of the parameter path and name. For parameters in
* a hierarchy, you must include a leading forward slash character (/) when you create or reference a
* parameter. For example: /Dev/DBServer/MySQL/db-string13
*
*
* Naming Constraints:
*
*
* -
*
* Parameter names are case sensitive.
*
*
* -
*
* A parameter name must be unique within an Amazon Web Services Region
*
*
* -
*
* A parameter name can't be prefixed with "aws
" or "ssm
" (case-insensitive).
*
*
* -
*
* Parameter names can include only the following symbols and letters: a-zA-Z0-9_.-
*
*
* In addition, the slash character ( / ) is used to delineate hierarchies in parameter names. For example:
* /Dev/Production/East/Project-ABC/MyParameter
*
*
* -
*
* A parameter name can't include spaces.
*
*
* -
*
* Parameter hierarchies are limited to a maximum depth of fifteen levels.
*
*
*
*
* For additional information about valid values for parameter names, see Creating Systems Manager parameters in the Amazon Web Services Systems Manager User Guide.
*
*
*
* The maximum length constraint of 2048 characters listed below includes 1037 characters reserved for
* internal use by Systems Manager. The maximum length for a parameter name that you create is 1011
* characters. This includes the characters in the ARN that precede the name you specify, such as
* arn:aws:ssm:us-east-2:111122223333:parameter/
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withName(String name) {
setName(name);
return this;
}
/**
*
* Information about the parameter that you want to add to the system. Optional but recommended.
*
*
*
* Don't enter personally identifiable information in this field.
*
*
*
* @param description
* Information about the parameter that you want to add to the system. Optional but recommended.
*
*
* Don't enter personally identifiable information in this field.
*
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* Information about the parameter that you want to add to the system. Optional but recommended.
*
*
*
* Don't enter personally identifiable information in this field.
*
*
*
* @return Information about the parameter that you want to add to the system. Optional but recommended.
*
*
* Don't enter personally identifiable information in this field.
*
*/
public String getDescription() {
return this.description;
}
/**
*
* Information about the parameter that you want to add to the system. Optional but recommended.
*
*
*
* Don't enter personally identifiable information in this field.
*
*
*
* @param description
* Information about the parameter that you want to add to the system. Optional but recommended.
*
*
* Don't enter personally identifiable information in this field.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The parameter value that you want to add to the system. Standard parameters have a value limit of 4 KB. Advanced
* parameters have a value limit of 8 KB.
*
*
*
* Parameters can't be referenced or nested in the values of other parameters. You can't include {{}}
* or {{ssm:parameter-name}}
in a parameter value.
*
*
*
* @param value
* The parameter value that you want to add to the system. Standard parameters have a value limit of 4 KB.
* Advanced parameters have a value limit of 8 KB.
*
* Parameters can't be referenced or nested in the values of other parameters. You can't include
* {{}}
or {{ssm:parameter-name}}
in a parameter value.
*
*/
public void setValue(String value) {
this.value = value;
}
/**
*
* The parameter value that you want to add to the system. Standard parameters have a value limit of 4 KB. Advanced
* parameters have a value limit of 8 KB.
*
*
*
* Parameters can't be referenced or nested in the values of other parameters. You can't include {{}}
* or {{ssm:parameter-name}}
in a parameter value.
*
*
*
* @return The parameter value that you want to add to the system. Standard parameters have a value limit of 4 KB.
* Advanced parameters have a value limit of 8 KB.
*
* Parameters can't be referenced or nested in the values of other parameters. You can't include
* {{}}
or {{ssm:parameter-name}}
in a parameter value.
*
*/
public String getValue() {
return this.value;
}
/**
*
* The parameter value that you want to add to the system. Standard parameters have a value limit of 4 KB. Advanced
* parameters have a value limit of 8 KB.
*
*
*
* Parameters can't be referenced or nested in the values of other parameters. You can't include {{}}
* or {{ssm:parameter-name}}
in a parameter value.
*
*
*
* @param value
* The parameter value that you want to add to the system. Standard parameters have a value limit of 4 KB.
* Advanced parameters have a value limit of 8 KB.
*
* Parameters can't be referenced or nested in the values of other parameters. You can't include
* {{}}
or {{ssm:parameter-name}}
in a parameter value.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withValue(String value) {
setValue(value);
return this;
}
/**
*
* The type of parameter that you want to add to the system.
*
*
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or special
* character to escape items in the list. If you have a parameter value that requires a comma, then use the
* String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type when
* creating a parameter.
*
*
*
* @param type
* The type of parameter that you want to add to the system.
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or
* special character to escape items in the list. If you have a parameter value that requires a comma, then
* use the String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type
* when creating a parameter.
*
* @see ParameterType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of parameter that you want to add to the system.
*
*
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or special
* character to escape items in the list. If you have a parameter value that requires a comma, then use the
* String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type when
* creating a parameter.
*
*
*
* @return The type of parameter that you want to add to the system.
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or
* special character to escape items in the list. If you have a parameter value that requires a comma, then
* use the String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type
* when creating a parameter.
*
* @see ParameterType
*/
public String getType() {
return this.type;
}
/**
*
* The type of parameter that you want to add to the system.
*
*
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or special
* character to escape items in the list. If you have a parameter value that requires a comma, then use the
* String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type when
* creating a parameter.
*
*
*
* @param type
* The type of parameter that you want to add to the system.
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or
* special character to escape items in the list. If you have a parameter value that requires a comma, then
* use the String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type
* when creating a parameter.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParameterType
*/
public PutParameterRequest withType(String type) {
setType(type);
return this;
}
/**
*
* The type of parameter that you want to add to the system.
*
*
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or special
* character to escape items in the list. If you have a parameter value that requires a comma, then use the
* String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type when
* creating a parameter.
*
*
*
* @param type
* The type of parameter that you want to add to the system.
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or
* special character to escape items in the list. If you have a parameter value that requires a comma, then
* use the String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type
* when creating a parameter.
*
* @see ParameterType
*/
public void setType(ParameterType type) {
withType(type);
}
/**
*
* The type of parameter that you want to add to the system.
*
*
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or special
* character to escape items in the list. If you have a parameter value that requires a comma, then use the
* String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type when
* creating a parameter.
*
*
*
* @param type
* The type of parameter that you want to add to the system.
*
* SecureString
isn't currently supported for CloudFormation templates.
*
*
*
* Items in a StringList
must be separated by a comma (,). You can't use other punctuation or
* special character to escape items in the list. If you have a parameter value that requires a comma, then
* use the String
data type.
*
*
*
* Specifying a parameter type isn't required when updating a parameter. You must specify a parameter type
* when creating a parameter.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParameterType
*/
public PutParameterRequest withType(ParameterType type) {
this.type = type.toString();
return this;
}
/**
*
* The Key Management Service (KMS) ID that you want to use to encrypt a parameter. Use a custom key for better
* security. Required for parameters that use the SecureString
data type.
*
*
* If you don't specify a key ID, the system uses the default key associated with your Amazon Web Services account
* which is not as secure as using a custom key.
*
*
* -
*
* To use a custom KMS key, choose the SecureString
data type with the Key ID
parameter.
*
*
*
*
* @param keyId
* The Key Management Service (KMS) ID that you want to use to encrypt a parameter. Use a custom key for
* better security. Required for parameters that use the SecureString
data type.
*
* If you don't specify a key ID, the system uses the default key associated with your Amazon Web Services
* account which is not as secure as using a custom key.
*
*
* -
*
* To use a custom KMS key, choose the SecureString
data type with the Key ID
* parameter.
*
*
*/
public void setKeyId(String keyId) {
this.keyId = keyId;
}
/**
*
* The Key Management Service (KMS) ID that you want to use to encrypt a parameter. Use a custom key for better
* security. Required for parameters that use the SecureString
data type.
*
*
* If you don't specify a key ID, the system uses the default key associated with your Amazon Web Services account
* which is not as secure as using a custom key.
*
*
* -
*
* To use a custom KMS key, choose the SecureString
data type with the Key ID
parameter.
*
*
*
*
* @return The Key Management Service (KMS) ID that you want to use to encrypt a parameter. Use a custom key for
* better security. Required for parameters that use the SecureString
data type.
*
* If you don't specify a key ID, the system uses the default key associated with your Amazon Web Services
* account which is not as secure as using a custom key.
*
*
* -
*
* To use a custom KMS key, choose the SecureString
data type with the Key ID
* parameter.
*
*
*/
public String getKeyId() {
return this.keyId;
}
/**
*
* The Key Management Service (KMS) ID that you want to use to encrypt a parameter. Use a custom key for better
* security. Required for parameters that use the SecureString
data type.
*
*
* If you don't specify a key ID, the system uses the default key associated with your Amazon Web Services account
* which is not as secure as using a custom key.
*
*
* -
*
* To use a custom KMS key, choose the SecureString
data type with the Key ID
parameter.
*
*
*
*
* @param keyId
* The Key Management Service (KMS) ID that you want to use to encrypt a parameter. Use a custom key for
* better security. Required for parameters that use the SecureString
data type.
*
* If you don't specify a key ID, the system uses the default key associated with your Amazon Web Services
* account which is not as secure as using a custom key.
*
*
* -
*
* To use a custom KMS key, choose the SecureString
data type with the Key ID
* parameter.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withKeyId(String keyId) {
setKeyId(keyId);
return this;
}
/**
*
* Overwrite an existing parameter. The default value is false
.
*
*
* @param overwrite
* Overwrite an existing parameter. The default value is false
.
*/
public void setOverwrite(Boolean overwrite) {
this.overwrite = overwrite;
}
/**
*
* Overwrite an existing parameter. The default value is false
.
*
*
* @return Overwrite an existing parameter. The default value is false
.
*/
public Boolean getOverwrite() {
return this.overwrite;
}
/**
*
* Overwrite an existing parameter. The default value is false
.
*
*
* @param overwrite
* Overwrite an existing parameter. The default value is false
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withOverwrite(Boolean overwrite) {
setOverwrite(overwrite);
return this;
}
/**
*
* Overwrite an existing parameter. The default value is false
.
*
*
* @return Overwrite an existing parameter. The default value is false
.
*/
public Boolean isOverwrite() {
return this.overwrite;
}
/**
*
* A regular expression used to validate the parameter value. For example, for String types with values restricted
* to numbers, you can specify the following: AllowedPattern=^\d+$
*
*
* @param allowedPattern
* A regular expression used to validate the parameter value. For example, for String types with values
* restricted to numbers, you can specify the following: AllowedPattern=^\d+$
*/
public void setAllowedPattern(String allowedPattern) {
this.allowedPattern = allowedPattern;
}
/**
*
* A regular expression used to validate the parameter value. For example, for String types with values restricted
* to numbers, you can specify the following: AllowedPattern=^\d+$
*
*
* @return A regular expression used to validate the parameter value. For example, for String types with values
* restricted to numbers, you can specify the following: AllowedPattern=^\d+$
*/
public String getAllowedPattern() {
return this.allowedPattern;
}
/**
*
* A regular expression used to validate the parameter value. For example, for String types with values restricted
* to numbers, you can specify the following: AllowedPattern=^\d+$
*
*
* @param allowedPattern
* A regular expression used to validate the parameter value. For example, for String types with values
* restricted to numbers, you can specify the following: AllowedPattern=^\d+$
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withAllowedPattern(String allowedPattern) {
setAllowedPattern(allowedPattern);
return this;
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a Systems Manager parameter to identify
* the type of resource to which it applies, the environment, or the type of configuration data referenced by the
* parameter. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Resource,Value=S3bucket
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=ParameterType,Value=LicenseKey
*
*
*
*
*
* To add tags to an existing Systems Manager parameter, use the AddTagsToResource operation.
*
*
*
* @return Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag a Systems Manager
* parameter to identify the type of resource to which it applies, the environment, or the type of
* configuration data referenced by the parameter. In this case, you could specify the following key-value
* pairs:
*
* -
*
* Key=Resource,Value=S3bucket
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=ParameterType,Value=LicenseKey
*
*
*
*
*
* To add tags to an existing Systems Manager parameter, use the AddTagsToResource operation.
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a Systems Manager parameter to identify
* the type of resource to which it applies, the environment, or the type of configuration data referenced by the
* parameter. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Resource,Value=S3bucket
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=ParameterType,Value=LicenseKey
*
*
*
*
*
* To add tags to an existing Systems Manager parameter, use the AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag a Systems Manager
* parameter to identify the type of resource to which it applies, the environment, or the type of
* configuration data referenced by the parameter. In this case, you could specify the following key-value
* pairs:
*
* -
*
* Key=Resource,Value=S3bucket
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=ParameterType,Value=LicenseKey
*
*
*
*
*
* To add tags to an existing Systems Manager parameter, use the AddTagsToResource operation.
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a Systems Manager parameter to identify
* the type of resource to which it applies, the environment, or the type of configuration data referenced by the
* parameter. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Resource,Value=S3bucket
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=ParameterType,Value=LicenseKey
*
*
*
*
*
* To add tags to an existing Systems Manager parameter, use the AddTagsToResource operation.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag a Systems Manager
* parameter to identify the type of resource to which it applies, the environment, or the type of
* configuration data referenced by the parameter. In this case, you could specify the following key-value
* pairs:
*
* -
*
* Key=Resource,Value=S3bucket
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=ParameterType,Value=LicenseKey
*
*
*
*
*
* To add tags to an existing Systems Manager parameter, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different ways, such
* as by purpose, owner, or environment. For example, you might want to tag a Systems Manager parameter to identify
* the type of resource to which it applies, the environment, or the type of configuration data referenced by the
* parameter. In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Resource,Value=S3bucket
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=ParameterType,Value=LicenseKey
*
*
*
*
*
* To add tags to an existing Systems Manager parameter, use the AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource. Tags enable you to categorize a resource in different
* ways, such as by purpose, owner, or environment. For example, you might want to tag a Systems Manager
* parameter to identify the type of resource to which it applies, the environment, or the type of
* configuration data referenced by the parameter. In this case, you could specify the following key-value
* pairs:
*
* -
*
* Key=Resource,Value=S3bucket
*
*
* -
*
* Key=OS,Value=Windows
*
*
* -
*
* Key=ParameterType,Value=LicenseKey
*
*
*
*
*
* To add tags to an existing Systems Manager parameter, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The parameter tier to assign to a parameter.
*
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a content
* size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of 10,000 standard
* parameters for each Region in an Amazon Web Services account. Standard parameters are offered at no additional
* cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You can
* create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account. Advanced
* parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced parameter
* to a standard parameter. Reverting an advanced parameter to a standard parameter would result in data loss
* because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would also remove any
* policies attached to the parameter. Lastly, advanced parameters use a different form of encryption than standard
* parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced parameter,
* you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you specify
* a tier in the request, Parameter Store creates or updates the parameter according to that request. However, if
* you don't specify a tier in a request, Parameter Store assigns the tier based on the current Parameter Store
* default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in the
* standard-parameter tier. If one or more options requiring an advanced parameter are included in the request,
* Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web Services
* Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
*
*
* @param tier
* The parameter tier to assign to a parameter.
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a
* content size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of
* 10,000 standard parameters for each Region in an Amazon Web Services account. Standard parameters are
* offered at no additional cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You
* can create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account.
* Advanced parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced
* parameter to a standard parameter. Reverting an advanced parameter to a standard parameter would result in
* data loss because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would
* also remove any policies attached to the parameter. Lastly, advanced parameters use a different form of
* encryption than standard parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced
* parameter, you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you
* specify a tier in the request, Parameter Store creates or updates the parameter according to that request.
* However, if you don't specify a tier in a request, Parameter Store assigns the tier based on the current
* Parameter Store default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in
* the standard-parameter tier. If one or more options requiring an advanced parameter are included in the
* request, Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web
* Services Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
* @see ParameterTier
*/
public void setTier(String tier) {
this.tier = tier;
}
/**
*
* The parameter tier to assign to a parameter.
*
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a content
* size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of 10,000 standard
* parameters for each Region in an Amazon Web Services account. Standard parameters are offered at no additional
* cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You can
* create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account. Advanced
* parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced parameter
* to a standard parameter. Reverting an advanced parameter to a standard parameter would result in data loss
* because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would also remove any
* policies attached to the parameter. Lastly, advanced parameters use a different form of encryption than standard
* parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced parameter,
* you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you specify
* a tier in the request, Parameter Store creates or updates the parameter according to that request. However, if
* you don't specify a tier in a request, Parameter Store assigns the tier based on the current Parameter Store
* default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in the
* standard-parameter tier. If one or more options requiring an advanced parameter are included in the request,
* Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web Services
* Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
*
*
* @return The parameter tier to assign to a parameter.
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a
* content size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of
* 10,000 standard parameters for each Region in an Amazon Web Services account. Standard parameters are
* offered at no additional cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies.
* You can create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services
* account. Advanced parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced
* parameter to a standard parameter. Reverting an advanced parameter to a standard parameter would result
* in data loss because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting
* would also remove any policies attached to the parameter. Lastly, advanced parameters use a different
* form of encryption than standard parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced
* parameter, you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you
* specify a tier in the request, Parameter Store creates or updates the parameter according to that
* request. However, if you don't specify a tier in a request, Parameter Store assigns the tier based on the
* current Parameter Store default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created
* in the standard-parameter tier. If one or more options requiring an advanced parameter are included in
* the request, Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web
* Services Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
* @see ParameterTier
*/
public String getTier() {
return this.tier;
}
/**
*
* The parameter tier to assign to a parameter.
*
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a content
* size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of 10,000 standard
* parameters for each Region in an Amazon Web Services account. Standard parameters are offered at no additional
* cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You can
* create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account. Advanced
* parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced parameter
* to a standard parameter. Reverting an advanced parameter to a standard parameter would result in data loss
* because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would also remove any
* policies attached to the parameter. Lastly, advanced parameters use a different form of encryption than standard
* parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced parameter,
* you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you specify
* a tier in the request, Parameter Store creates or updates the parameter according to that request. However, if
* you don't specify a tier in a request, Parameter Store assigns the tier based on the current Parameter Store
* default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in the
* standard-parameter tier. If one or more options requiring an advanced parameter are included in the request,
* Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web Services
* Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
*
*
* @param tier
* The parameter tier to assign to a parameter.
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a
* content size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of
* 10,000 standard parameters for each Region in an Amazon Web Services account. Standard parameters are
* offered at no additional cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You
* can create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account.
* Advanced parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced
* parameter to a standard parameter. Reverting an advanced parameter to a standard parameter would result in
* data loss because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would
* also remove any policies attached to the parameter. Lastly, advanced parameters use a different form of
* encryption than standard parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced
* parameter, you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you
* specify a tier in the request, Parameter Store creates or updates the parameter according to that request.
* However, if you don't specify a tier in a request, Parameter Store assigns the tier based on the current
* Parameter Store default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in
* the standard-parameter tier. If one or more options requiring an advanced parameter are included in the
* request, Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web
* Services Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParameterTier
*/
public PutParameterRequest withTier(String tier) {
setTier(tier);
return this;
}
/**
*
* The parameter tier to assign to a parameter.
*
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a content
* size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of 10,000 standard
* parameters for each Region in an Amazon Web Services account. Standard parameters are offered at no additional
* cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You can
* create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account. Advanced
* parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced parameter
* to a standard parameter. Reverting an advanced parameter to a standard parameter would result in data loss
* because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would also remove any
* policies attached to the parameter. Lastly, advanced parameters use a different form of encryption than standard
* parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced parameter,
* you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you specify
* a tier in the request, Parameter Store creates or updates the parameter according to that request. However, if
* you don't specify a tier in a request, Parameter Store assigns the tier based on the current Parameter Store
* default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in the
* standard-parameter tier. If one or more options requiring an advanced parameter are included in the request,
* Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web Services
* Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
*
*
* @param tier
* The parameter tier to assign to a parameter.
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a
* content size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of
* 10,000 standard parameters for each Region in an Amazon Web Services account. Standard parameters are
* offered at no additional cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You
* can create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account.
* Advanced parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced
* parameter to a standard parameter. Reverting an advanced parameter to a standard parameter would result in
* data loss because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would
* also remove any policies attached to the parameter. Lastly, advanced parameters use a different form of
* encryption than standard parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced
* parameter, you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you
* specify a tier in the request, Parameter Store creates or updates the parameter according to that request.
* However, if you don't specify a tier in a request, Parameter Store assigns the tier based on the current
* Parameter Store default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in
* the standard-parameter tier. If one or more options requiring an advanced parameter are included in the
* request, Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web
* Services Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
* @see ParameterTier
*/
public void setTier(ParameterTier tier) {
withTier(tier);
}
/**
*
* The parameter tier to assign to a parameter.
*
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a content
* size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of 10,000 standard
* parameters for each Region in an Amazon Web Services account. Standard parameters are offered at no additional
* cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You can
* create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account. Advanced
* parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced parameter
* to a standard parameter. Reverting an advanced parameter to a standard parameter would result in data loss
* because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would also remove any
* policies attached to the parameter. Lastly, advanced parameters use a different form of encryption than standard
* parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced parameter,
* you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you specify
* a tier in the request, Parameter Store creates or updates the parameter according to that request. However, if
* you don't specify a tier in a request, Parameter Store assigns the tier based on the current Parameter Store
* default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in the
* standard-parameter tier. If one or more options requiring an advanced parameter are included in the request,
* Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web Services
* Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
*
*
* @param tier
* The parameter tier to assign to a parameter.
*
* Parameter Store offers a standard tier and an advanced tier for parameters. Standard parameters have a
* content size limit of 4 KB and can't be configured to use parameter policies. You can create a maximum of
* 10,000 standard parameters for each Region in an Amazon Web Services account. Standard parameters are
* offered at no additional cost.
*
*
* Advanced parameters have a content size limit of 8 KB and can be configured to use parameter policies. You
* can create a maximum of 100,000 advanced parameters for each Region in an Amazon Web Services account.
* Advanced parameters incur a charge. For more information, see Managing parameter tiers in the Amazon Web Services Systems Manager User Guide.
*
*
* You can change a standard parameter to an advanced parameter any time. But you can't revert an advanced
* parameter to a standard parameter. Reverting an advanced parameter to a standard parameter would result in
* data loss because the system would truncate the size of the parameter from 8 KB to 4 KB. Reverting would
* also remove any policies attached to the parameter. Lastly, advanced parameters use a different form of
* encryption than standard parameters.
*
*
* If you no longer need an advanced parameter, or if you no longer want to incur charges for an advanced
* parameter, you must delete it and recreate it as a new standard parameter.
*
*
* Using the Default Tier Configuration
*
*
* In PutParameter
requests, you can specify the tier to create the parameter in. Whenever you
* specify a tier in the request, Parameter Store creates or updates the parameter according to that request.
* However, if you don't specify a tier in a request, Parameter Store assigns the tier based on the current
* Parameter Store default tier configuration.
*
*
* The default tier when you begin using Parameter Store is the standard-parameter tier. If you use the
* advanced-parameter tier, you can specify one of the following as the default:
*
*
* -
*
* Advanced: With this option, Parameter Store evaluates all requests as advanced parameters.
*
*
* -
*
* Intelligent-Tiering: With this option, Parameter Store evaluates each request to determine if the
* parameter is standard or advanced.
*
*
* If the request doesn't include any options that require an advanced parameter, the parameter is created in
* the standard-parameter tier. If one or more options requiring an advanced parameter are included in the
* request, Parameter Store create a parameter in the advanced-parameter tier.
*
*
* This approach helps control your parameter-related costs by always creating standard parameters unless an
* advanced parameter is necessary.
*
*
*
*
* Options that require an advanced parameter include the following:
*
*
* -
*
* The content size of the parameter is more than 4 KB.
*
*
* -
*
* The parameter uses a parameter policy.
*
*
* -
*
* More than 10,000 parameters already exist in your Amazon Web Services account in the current Amazon Web
* Services Region.
*
*
*
*
* For more information about configuring the default tier option, see Specifying a default parameter tier in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParameterTier
*/
public PutParameterRequest withTier(ParameterTier tier) {
this.tier = tier.toString();
return this;
}
/**
*
* One or more policies to apply to a parameter. This operation takes a JSON array. Parameter Store, a capability of
* Amazon Web Services Systems Manager supports the following policy types:
*
*
* Expiration: This policy deletes the parameter after it expires. When you create the policy, you specify the
* expiration date. You can update the expiration date and time by updating the policy. Updating the
* parameter doesn't affect the expiration date and time. When the expiration time is reached, Parameter
* Store deletes the parameter.
*
*
* ExpirationNotification: This policy initiates an event in Amazon CloudWatch Events that notifies you about the
* expiration. By using this policy, you can receive notification before or after the expiration time is reached, in
* units of days or hours.
*
*
* NoChangeNotification: This policy initiates a CloudWatch Events event if a parameter hasn't been modified for a
* specified period of time. This policy type is useful when, for example, a secret needs to be changed within a
* period of time, but it hasn't been changed.
*
*
* All existing policies are preserved until you send new policies or an empty policy. For more information about
* parameter policies, see Assigning
* parameter policies.
*
*
* @param policies
* One or more policies to apply to a parameter. This operation takes a JSON array. Parameter Store, a
* capability of Amazon Web Services Systems Manager supports the following policy types:
*
* Expiration: This policy deletes the parameter after it expires. When you create the policy, you specify
* the expiration date. You can update the expiration date and time by updating the policy. Updating the
* parameter doesn't affect the expiration date and time. When the expiration time is reached,
* Parameter Store deletes the parameter.
*
*
* ExpirationNotification: This policy initiates an event in Amazon CloudWatch Events that notifies you about
* the expiration. By using this policy, you can receive notification before or after the expiration time is
* reached, in units of days or hours.
*
*
* NoChangeNotification: This policy initiates a CloudWatch Events event if a parameter hasn't been modified
* for a specified period of time. This policy type is useful when, for example, a secret needs to be changed
* within a period of time, but it hasn't been changed.
*
*
* All existing policies are preserved until you send new policies or an empty policy. For more information
* about parameter policies, see Assigning parameter policies.
*/
public void setPolicies(String policies) {
this.policies = policies;
}
/**
*
* One or more policies to apply to a parameter. This operation takes a JSON array. Parameter Store, a capability of
* Amazon Web Services Systems Manager supports the following policy types:
*
*
* Expiration: This policy deletes the parameter after it expires. When you create the policy, you specify the
* expiration date. You can update the expiration date and time by updating the policy. Updating the
* parameter doesn't affect the expiration date and time. When the expiration time is reached, Parameter
* Store deletes the parameter.
*
*
* ExpirationNotification: This policy initiates an event in Amazon CloudWatch Events that notifies you about the
* expiration. By using this policy, you can receive notification before or after the expiration time is reached, in
* units of days or hours.
*
*
* NoChangeNotification: This policy initiates a CloudWatch Events event if a parameter hasn't been modified for a
* specified period of time. This policy type is useful when, for example, a secret needs to be changed within a
* period of time, but it hasn't been changed.
*
*
* All existing policies are preserved until you send new policies or an empty policy. For more information about
* parameter policies, see Assigning
* parameter policies.
*
*
* @return One or more policies to apply to a parameter. This operation takes a JSON array. Parameter Store, a
* capability of Amazon Web Services Systems Manager supports the following policy types:
*
* Expiration: This policy deletes the parameter after it expires. When you create the policy, you specify
* the expiration date. You can update the expiration date and time by updating the policy. Updating the
* parameter doesn't affect the expiration date and time. When the expiration time is reached,
* Parameter Store deletes the parameter.
*
*
* ExpirationNotification: This policy initiates an event in Amazon CloudWatch Events that notifies you
* about the expiration. By using this policy, you can receive notification before or after the expiration
* time is reached, in units of days or hours.
*
*
* NoChangeNotification: This policy initiates a CloudWatch Events event if a parameter hasn't been modified
* for a specified period of time. This policy type is useful when, for example, a secret needs to be
* changed within a period of time, but it hasn't been changed.
*
*
* All existing policies are preserved until you send new policies or an empty policy. For more information
* about parameter policies, see Assigning parameter policies.
*/
public String getPolicies() {
return this.policies;
}
/**
*
* One or more policies to apply to a parameter. This operation takes a JSON array. Parameter Store, a capability of
* Amazon Web Services Systems Manager supports the following policy types:
*
*
* Expiration: This policy deletes the parameter after it expires. When you create the policy, you specify the
* expiration date. You can update the expiration date and time by updating the policy. Updating the
* parameter doesn't affect the expiration date and time. When the expiration time is reached, Parameter
* Store deletes the parameter.
*
*
* ExpirationNotification: This policy initiates an event in Amazon CloudWatch Events that notifies you about the
* expiration. By using this policy, you can receive notification before or after the expiration time is reached, in
* units of days or hours.
*
*
* NoChangeNotification: This policy initiates a CloudWatch Events event if a parameter hasn't been modified for a
* specified period of time. This policy type is useful when, for example, a secret needs to be changed within a
* period of time, but it hasn't been changed.
*
*
* All existing policies are preserved until you send new policies or an empty policy. For more information about
* parameter policies, see Assigning
* parameter policies.
*
*
* @param policies
* One or more policies to apply to a parameter. This operation takes a JSON array. Parameter Store, a
* capability of Amazon Web Services Systems Manager supports the following policy types:
*
* Expiration: This policy deletes the parameter after it expires. When you create the policy, you specify
* the expiration date. You can update the expiration date and time by updating the policy. Updating the
* parameter doesn't affect the expiration date and time. When the expiration time is reached,
* Parameter Store deletes the parameter.
*
*
* ExpirationNotification: This policy initiates an event in Amazon CloudWatch Events that notifies you about
* the expiration. By using this policy, you can receive notification before or after the expiration time is
* reached, in units of days or hours.
*
*
* NoChangeNotification: This policy initiates a CloudWatch Events event if a parameter hasn't been modified
* for a specified period of time. This policy type is useful when, for example, a secret needs to be changed
* within a period of time, but it hasn't been changed.
*
*
* All existing policies are preserved until you send new policies or an empty policy. For more information
* about parameter policies, see Assigning parameter policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withPolicies(String policies) {
setPolicies(policies);
return this;
}
/**
*
* The data type for a String
parameter. Supported data types include plain text and Amazon Machine
* Image (AMI) IDs.
*
*
* The following data type values are supported.
*
*
* -
*
* text
*
*
* -
*
* aws:ec2:image
*
*
* -
*
* aws:ssm:integration
*
*
*
*
* When you create a String
parameter and specify aws:ec2:image
, Amazon Web Services
* Systems Manager validates the parameter value is in the required format, such as
* ami-12345abcdeEXAMPLE
, and that the specified AMI is available in your Amazon Web Services account.
*
*
*
* If the action is successful, the service sends back an HTTP 200 response which indicates a successful
* PutParameter
call for all cases except for data type aws:ec2:image
. If you call
* PutParameter
with aws:ec2:image
data type, a successful HTTP 200 response does not
* guarantee that your parameter was successfully created or updated. The aws:ec2:image
value is
* validated asynchronously, and the PutParameter
call returns before the validation is complete. If
* you submit an invalid AMI value, the PutParameter operation will return success, but the asynchronous validation
* will fail and the parameter will not be created or updated. To monitor whether your aws:ec2:image
* parameters are created successfully, see Setting up
* notifications or trigger actions based on Parameter Store events. For more information about AMI format
* validation , see Native
* parameter support for Amazon Machine Image IDs.
*
*
*
* @param dataType
* The data type for a String
parameter. Supported data types include plain text and Amazon
* Machine Image (AMI) IDs.
*
* The following data type values are supported.
*
*
* -
*
* text
*
*
* -
*
* aws:ec2:image
*
*
* -
*
* aws:ssm:integration
*
*
*
*
* When you create a String
parameter and specify aws:ec2:image
, Amazon Web
* Services Systems Manager validates the parameter value is in the required format, such as
* ami-12345abcdeEXAMPLE
, and that the specified AMI is available in your Amazon Web Services
* account.
*
*
*
* If the action is successful, the service sends back an HTTP 200 response which indicates a successful
* PutParameter
call for all cases except for data type aws:ec2:image
. If you call
* PutParameter
with aws:ec2:image
data type, a successful HTTP 200 response does
* not guarantee that your parameter was successfully created or updated. The aws:ec2:image
* value is validated asynchronously, and the PutParameter
call returns before the validation is
* complete. If you submit an invalid AMI value, the PutParameter operation will return success, but the
* asynchronous validation will fail and the parameter will not be created or updated. To monitor whether
* your aws:ec2:image
parameters are created successfully, see Setting up
* notifications or trigger actions based on Parameter Store events. For more information about AMI
* format validation , see Native parameter support for Amazon Machine Image IDs.
*
*/
public void setDataType(String dataType) {
this.dataType = dataType;
}
/**
*
* The data type for a String
parameter. Supported data types include plain text and Amazon Machine
* Image (AMI) IDs.
*
*
* The following data type values are supported.
*
*
* -
*
* text
*
*
* -
*
* aws:ec2:image
*
*
* -
*
* aws:ssm:integration
*
*
*
*
* When you create a String
parameter and specify aws:ec2:image
, Amazon Web Services
* Systems Manager validates the parameter value is in the required format, such as
* ami-12345abcdeEXAMPLE
, and that the specified AMI is available in your Amazon Web Services account.
*
*
*
* If the action is successful, the service sends back an HTTP 200 response which indicates a successful
* PutParameter
call for all cases except for data type aws:ec2:image
. If you call
* PutParameter
with aws:ec2:image
data type, a successful HTTP 200 response does not
* guarantee that your parameter was successfully created or updated. The aws:ec2:image
value is
* validated asynchronously, and the PutParameter
call returns before the validation is complete. If
* you submit an invalid AMI value, the PutParameter operation will return success, but the asynchronous validation
* will fail and the parameter will not be created or updated. To monitor whether your aws:ec2:image
* parameters are created successfully, see Setting up
* notifications or trigger actions based on Parameter Store events. For more information about AMI format
* validation , see Native
* parameter support for Amazon Machine Image IDs.
*
*
*
* @return The data type for a String
parameter. Supported data types include plain text and Amazon
* Machine Image (AMI) IDs.
*
* The following data type values are supported.
*
*
* -
*
* text
*
*
* -
*
* aws:ec2:image
*
*
* -
*
* aws:ssm:integration
*
*
*
*
* When you create a String
parameter and specify aws:ec2:image
, Amazon Web
* Services Systems Manager validates the parameter value is in the required format, such as
* ami-12345abcdeEXAMPLE
, and that the specified AMI is available in your Amazon Web Services
* account.
*
*
*
* If the action is successful, the service sends back an HTTP 200 response which indicates a successful
* PutParameter
call for all cases except for data type aws:ec2:image
. If you call
* PutParameter
with aws:ec2:image
data type, a successful HTTP 200 response does
* not guarantee that your parameter was successfully created or updated. The aws:ec2:image
* value is validated asynchronously, and the PutParameter
call returns before the validation
* is complete. If you submit an invalid AMI value, the PutParameter operation will return success, but the
* asynchronous validation will fail and the parameter will not be created or updated. To monitor whether
* your aws:ec2:image
parameters are created successfully, see Setting up
* notifications or trigger actions based on Parameter Store events. For more information about AMI
* format validation , see Native parameter support for Amazon Machine Image IDs.
*
*/
public String getDataType() {
return this.dataType;
}
/**
*
* The data type for a String
parameter. Supported data types include plain text and Amazon Machine
* Image (AMI) IDs.
*
*
* The following data type values are supported.
*
*
* -
*
* text
*
*
* -
*
* aws:ec2:image
*
*
* -
*
* aws:ssm:integration
*
*
*
*
* When you create a String
parameter and specify aws:ec2:image
, Amazon Web Services
* Systems Manager validates the parameter value is in the required format, such as
* ami-12345abcdeEXAMPLE
, and that the specified AMI is available in your Amazon Web Services account.
*
*
*
* If the action is successful, the service sends back an HTTP 200 response which indicates a successful
* PutParameter
call for all cases except for data type aws:ec2:image
. If you call
* PutParameter
with aws:ec2:image
data type, a successful HTTP 200 response does not
* guarantee that your parameter was successfully created or updated. The aws:ec2:image
value is
* validated asynchronously, and the PutParameter
call returns before the validation is complete. If
* you submit an invalid AMI value, the PutParameter operation will return success, but the asynchronous validation
* will fail and the parameter will not be created or updated. To monitor whether your aws:ec2:image
* parameters are created successfully, see Setting up
* notifications or trigger actions based on Parameter Store events. For more information about AMI format
* validation , see Native
* parameter support for Amazon Machine Image IDs.
*
*
*
* @param dataType
* The data type for a String
parameter. Supported data types include plain text and Amazon
* Machine Image (AMI) IDs.
*
* The following data type values are supported.
*
*
* -
*
* text
*
*
* -
*
* aws:ec2:image
*
*
* -
*
* aws:ssm:integration
*
*
*
*
* When you create a String
parameter and specify aws:ec2:image
, Amazon Web
* Services Systems Manager validates the parameter value is in the required format, such as
* ami-12345abcdeEXAMPLE
, and that the specified AMI is available in your Amazon Web Services
* account.
*
*
*
* If the action is successful, the service sends back an HTTP 200 response which indicates a successful
* PutParameter
call for all cases except for data type aws:ec2:image
. If you call
* PutParameter
with aws:ec2:image
data type, a successful HTTP 200 response does
* not guarantee that your parameter was successfully created or updated. The aws:ec2:image
* value is validated asynchronously, and the PutParameter
call returns before the validation is
* complete. If you submit an invalid AMI value, the PutParameter operation will return success, but the
* asynchronous validation will fail and the parameter will not be created or updated. To monitor whether
* your aws:ec2:image
parameters are created successfully, see Setting up
* notifications or trigger actions based on Parameter Store events. For more information about AMI
* format validation , see Native parameter support for Amazon Machine Image IDs.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutParameterRequest withDataType(String dataType) {
setDataType(dataType);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getValue() != null)
sb.append("Value: ").append("***Sensitive Data Redacted***").append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getKeyId() != null)
sb.append("KeyId: ").append(getKeyId()).append(",");
if (getOverwrite() != null)
sb.append("Overwrite: ").append(getOverwrite()).append(",");
if (getAllowedPattern() != null)
sb.append("AllowedPattern: ").append(getAllowedPattern()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getTier() != null)
sb.append("Tier: ").append(getTier()).append(",");
if (getPolicies() != null)
sb.append("Policies: ").append(getPolicies()).append(",");
if (getDataType() != null)
sb.append("DataType: ").append(getDataType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutParameterRequest == false)
return false;
PutParameterRequest other = (PutParameterRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getValue() == null ^ this.getValue() == null)
return false;
if (other.getValue() != null && other.getValue().equals(this.getValue()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getKeyId() == null ^ this.getKeyId() == null)
return false;
if (other.getKeyId() != null && other.getKeyId().equals(this.getKeyId()) == false)
return false;
if (other.getOverwrite() == null ^ this.getOverwrite() == null)
return false;
if (other.getOverwrite() != null && other.getOverwrite().equals(this.getOverwrite()) == false)
return false;
if (other.getAllowedPattern() == null ^ this.getAllowedPattern() == null)
return false;
if (other.getAllowedPattern() != null && other.getAllowedPattern().equals(this.getAllowedPattern()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getTier() == null ^ this.getTier() == null)
return false;
if (other.getTier() != null && other.getTier().equals(this.getTier()) == false)
return false;
if (other.getPolicies() == null ^ this.getPolicies() == null)
return false;
if (other.getPolicies() != null && other.getPolicies().equals(this.getPolicies()) == false)
return false;
if (other.getDataType() == null ^ this.getDataType() == null)
return false;
if (other.getDataType() != null && other.getDataType().equals(this.getDataType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getValue() == null) ? 0 : getValue().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getKeyId() == null) ? 0 : getKeyId().hashCode());
hashCode = prime * hashCode + ((getOverwrite() == null) ? 0 : getOverwrite().hashCode());
hashCode = prime * hashCode + ((getAllowedPattern() == null) ? 0 : getAllowedPattern().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getTier() == null) ? 0 : getTier().hashCode());
hashCode = prime * hashCode + ((getPolicies() == null) ? 0 : getPolicies().hashCode());
hashCode = prime * hashCode + ((getDataType() == null) ? 0 : getDataType().hashCode());
return hashCode;
}
@Override
public PutParameterRequest clone() {
return (PutParameterRequest) super.clone();
}
}