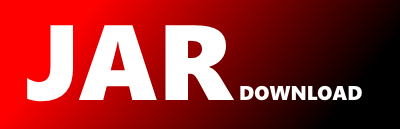
com.amazonaws.services.simplesystemsmanagement.model.ResourceDataSyncItem Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about a resource data sync configuration, including its current status and last successful sync.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ResourceDataSyncItem implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the resource data sync.
*
*/
private String syncName;
/**
*
* The type of resource data sync. If SyncType
is SyncToDestination
, then the resource
* data sync synchronizes data to an S3 bucket. If the SyncType
is SyncFromSource
then the
* resource data sync synchronizes data from Organizations or from multiple Amazon Web Services Regions.
*
*/
private String syncType;
/**
*
* Information about the source where the data was synchronized.
*
*/
private ResourceDataSyncSourceWithState syncSource;
/**
*
* Configuration information for the target S3 bucket.
*
*/
private ResourceDataSyncS3Destination s3Destination;
/**
*
* The last time the configuration attempted to sync (UTC).
*
*/
private java.util.Date lastSyncTime;
/**
*
* The last time the sync operations returned a status of SUCCESSFUL
(UTC).
*
*/
private java.util.Date lastSuccessfulSyncTime;
/**
*
* The date and time the resource data sync was changed.
*
*/
private java.util.Date syncLastModifiedTime;
/**
*
* The status reported by the last sync.
*
*/
private String lastStatus;
/**
*
* The date and time the configuration was created (UTC).
*
*/
private java.util.Date syncCreatedTime;
/**
*
* The status message details reported by the last sync.
*
*/
private String lastSyncStatusMessage;
/**
*
* The name of the resource data sync.
*
*
* @param syncName
* The name of the resource data sync.
*/
public void setSyncName(String syncName) {
this.syncName = syncName;
}
/**
*
* The name of the resource data sync.
*
*
* @return The name of the resource data sync.
*/
public String getSyncName() {
return this.syncName;
}
/**
*
* The name of the resource data sync.
*
*
* @param syncName
* The name of the resource data sync.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncItem withSyncName(String syncName) {
setSyncName(syncName);
return this;
}
/**
*
* The type of resource data sync. If SyncType
is SyncToDestination
, then the resource
* data sync synchronizes data to an S3 bucket. If the SyncType
is SyncFromSource
then the
* resource data sync synchronizes data from Organizations or from multiple Amazon Web Services Regions.
*
*
* @param syncType
* The type of resource data sync. If SyncType
is SyncToDestination
, then the
* resource data sync synchronizes data to an S3 bucket. If the SyncType
is
* SyncFromSource
then the resource data sync synchronizes data from Organizations or from
* multiple Amazon Web Services Regions.
*/
public void setSyncType(String syncType) {
this.syncType = syncType;
}
/**
*
* The type of resource data sync. If SyncType
is SyncToDestination
, then the resource
* data sync synchronizes data to an S3 bucket. If the SyncType
is SyncFromSource
then the
* resource data sync synchronizes data from Organizations or from multiple Amazon Web Services Regions.
*
*
* @return The type of resource data sync. If SyncType
is SyncToDestination
, then the
* resource data sync synchronizes data to an S3 bucket. If the SyncType
is
* SyncFromSource
then the resource data sync synchronizes data from Organizations or from
* multiple Amazon Web Services Regions.
*/
public String getSyncType() {
return this.syncType;
}
/**
*
* The type of resource data sync. If SyncType
is SyncToDestination
, then the resource
* data sync synchronizes data to an S3 bucket. If the SyncType
is SyncFromSource
then the
* resource data sync synchronizes data from Organizations or from multiple Amazon Web Services Regions.
*
*
* @param syncType
* The type of resource data sync. If SyncType
is SyncToDestination
, then the
* resource data sync synchronizes data to an S3 bucket. If the SyncType
is
* SyncFromSource
then the resource data sync synchronizes data from Organizations or from
* multiple Amazon Web Services Regions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncItem withSyncType(String syncType) {
setSyncType(syncType);
return this;
}
/**
*
* Information about the source where the data was synchronized.
*
*
* @param syncSource
* Information about the source where the data was synchronized.
*/
public void setSyncSource(ResourceDataSyncSourceWithState syncSource) {
this.syncSource = syncSource;
}
/**
*
* Information about the source where the data was synchronized.
*
*
* @return Information about the source where the data was synchronized.
*/
public ResourceDataSyncSourceWithState getSyncSource() {
return this.syncSource;
}
/**
*
* Information about the source where the data was synchronized.
*
*
* @param syncSource
* Information about the source where the data was synchronized.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncItem withSyncSource(ResourceDataSyncSourceWithState syncSource) {
setSyncSource(syncSource);
return this;
}
/**
*
* Configuration information for the target S3 bucket.
*
*
* @param s3Destination
* Configuration information for the target S3 bucket.
*/
public void setS3Destination(ResourceDataSyncS3Destination s3Destination) {
this.s3Destination = s3Destination;
}
/**
*
* Configuration information for the target S3 bucket.
*
*
* @return Configuration information for the target S3 bucket.
*/
public ResourceDataSyncS3Destination getS3Destination() {
return this.s3Destination;
}
/**
*
* Configuration information for the target S3 bucket.
*
*
* @param s3Destination
* Configuration information for the target S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncItem withS3Destination(ResourceDataSyncS3Destination s3Destination) {
setS3Destination(s3Destination);
return this;
}
/**
*
* The last time the configuration attempted to sync (UTC).
*
*
* @param lastSyncTime
* The last time the configuration attempted to sync (UTC).
*/
public void setLastSyncTime(java.util.Date lastSyncTime) {
this.lastSyncTime = lastSyncTime;
}
/**
*
* The last time the configuration attempted to sync (UTC).
*
*
* @return The last time the configuration attempted to sync (UTC).
*/
public java.util.Date getLastSyncTime() {
return this.lastSyncTime;
}
/**
*
* The last time the configuration attempted to sync (UTC).
*
*
* @param lastSyncTime
* The last time the configuration attempted to sync (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncItem withLastSyncTime(java.util.Date lastSyncTime) {
setLastSyncTime(lastSyncTime);
return this;
}
/**
*
* The last time the sync operations returned a status of SUCCESSFUL
(UTC).
*
*
* @param lastSuccessfulSyncTime
* The last time the sync operations returned a status of SUCCESSFUL
(UTC).
*/
public void setLastSuccessfulSyncTime(java.util.Date lastSuccessfulSyncTime) {
this.lastSuccessfulSyncTime = lastSuccessfulSyncTime;
}
/**
*
* The last time the sync operations returned a status of SUCCESSFUL
(UTC).
*
*
* @return The last time the sync operations returned a status of SUCCESSFUL
(UTC).
*/
public java.util.Date getLastSuccessfulSyncTime() {
return this.lastSuccessfulSyncTime;
}
/**
*
* The last time the sync operations returned a status of SUCCESSFUL
(UTC).
*
*
* @param lastSuccessfulSyncTime
* The last time the sync operations returned a status of SUCCESSFUL
(UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncItem withLastSuccessfulSyncTime(java.util.Date lastSuccessfulSyncTime) {
setLastSuccessfulSyncTime(lastSuccessfulSyncTime);
return this;
}
/**
*
* The date and time the resource data sync was changed.
*
*
* @param syncLastModifiedTime
* The date and time the resource data sync was changed.
*/
public void setSyncLastModifiedTime(java.util.Date syncLastModifiedTime) {
this.syncLastModifiedTime = syncLastModifiedTime;
}
/**
*
* The date and time the resource data sync was changed.
*
*
* @return The date and time the resource data sync was changed.
*/
public java.util.Date getSyncLastModifiedTime() {
return this.syncLastModifiedTime;
}
/**
*
* The date and time the resource data sync was changed.
*
*
* @param syncLastModifiedTime
* The date and time the resource data sync was changed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncItem withSyncLastModifiedTime(java.util.Date syncLastModifiedTime) {
setSyncLastModifiedTime(syncLastModifiedTime);
return this;
}
/**
*
* The status reported by the last sync.
*
*
* @param lastStatus
* The status reported by the last sync.
* @see LastResourceDataSyncStatus
*/
public void setLastStatus(String lastStatus) {
this.lastStatus = lastStatus;
}
/**
*
* The status reported by the last sync.
*
*
* @return The status reported by the last sync.
* @see LastResourceDataSyncStatus
*/
public String getLastStatus() {
return this.lastStatus;
}
/**
*
* The status reported by the last sync.
*
*
* @param lastStatus
* The status reported by the last sync.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LastResourceDataSyncStatus
*/
public ResourceDataSyncItem withLastStatus(String lastStatus) {
setLastStatus(lastStatus);
return this;
}
/**
*
* The status reported by the last sync.
*
*
* @param lastStatus
* The status reported by the last sync.
* @see LastResourceDataSyncStatus
*/
public void setLastStatus(LastResourceDataSyncStatus lastStatus) {
withLastStatus(lastStatus);
}
/**
*
* The status reported by the last sync.
*
*
* @param lastStatus
* The status reported by the last sync.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LastResourceDataSyncStatus
*/
public ResourceDataSyncItem withLastStatus(LastResourceDataSyncStatus lastStatus) {
this.lastStatus = lastStatus.toString();
return this;
}
/**
*
* The date and time the configuration was created (UTC).
*
*
* @param syncCreatedTime
* The date and time the configuration was created (UTC).
*/
public void setSyncCreatedTime(java.util.Date syncCreatedTime) {
this.syncCreatedTime = syncCreatedTime;
}
/**
*
* The date and time the configuration was created (UTC).
*
*
* @return The date and time the configuration was created (UTC).
*/
public java.util.Date getSyncCreatedTime() {
return this.syncCreatedTime;
}
/**
*
* The date and time the configuration was created (UTC).
*
*
* @param syncCreatedTime
* The date and time the configuration was created (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncItem withSyncCreatedTime(java.util.Date syncCreatedTime) {
setSyncCreatedTime(syncCreatedTime);
return this;
}
/**
*
* The status message details reported by the last sync.
*
*
* @param lastSyncStatusMessage
* The status message details reported by the last sync.
*/
public void setLastSyncStatusMessage(String lastSyncStatusMessage) {
this.lastSyncStatusMessage = lastSyncStatusMessage;
}
/**
*
* The status message details reported by the last sync.
*
*
* @return The status message details reported by the last sync.
*/
public String getLastSyncStatusMessage() {
return this.lastSyncStatusMessage;
}
/**
*
* The status message details reported by the last sync.
*
*
* @param lastSyncStatusMessage
* The status message details reported by the last sync.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncItem withLastSyncStatusMessage(String lastSyncStatusMessage) {
setLastSyncStatusMessage(lastSyncStatusMessage);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSyncName() != null)
sb.append("SyncName: ").append(getSyncName()).append(",");
if (getSyncType() != null)
sb.append("SyncType: ").append(getSyncType()).append(",");
if (getSyncSource() != null)
sb.append("SyncSource: ").append(getSyncSource()).append(",");
if (getS3Destination() != null)
sb.append("S3Destination: ").append(getS3Destination()).append(",");
if (getLastSyncTime() != null)
sb.append("LastSyncTime: ").append(getLastSyncTime()).append(",");
if (getLastSuccessfulSyncTime() != null)
sb.append("LastSuccessfulSyncTime: ").append(getLastSuccessfulSyncTime()).append(",");
if (getSyncLastModifiedTime() != null)
sb.append("SyncLastModifiedTime: ").append(getSyncLastModifiedTime()).append(",");
if (getLastStatus() != null)
sb.append("LastStatus: ").append(getLastStatus()).append(",");
if (getSyncCreatedTime() != null)
sb.append("SyncCreatedTime: ").append(getSyncCreatedTime()).append(",");
if (getLastSyncStatusMessage() != null)
sb.append("LastSyncStatusMessage: ").append(getLastSyncStatusMessage());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResourceDataSyncItem == false)
return false;
ResourceDataSyncItem other = (ResourceDataSyncItem) obj;
if (other.getSyncName() == null ^ this.getSyncName() == null)
return false;
if (other.getSyncName() != null && other.getSyncName().equals(this.getSyncName()) == false)
return false;
if (other.getSyncType() == null ^ this.getSyncType() == null)
return false;
if (other.getSyncType() != null && other.getSyncType().equals(this.getSyncType()) == false)
return false;
if (other.getSyncSource() == null ^ this.getSyncSource() == null)
return false;
if (other.getSyncSource() != null && other.getSyncSource().equals(this.getSyncSource()) == false)
return false;
if (other.getS3Destination() == null ^ this.getS3Destination() == null)
return false;
if (other.getS3Destination() != null && other.getS3Destination().equals(this.getS3Destination()) == false)
return false;
if (other.getLastSyncTime() == null ^ this.getLastSyncTime() == null)
return false;
if (other.getLastSyncTime() != null && other.getLastSyncTime().equals(this.getLastSyncTime()) == false)
return false;
if (other.getLastSuccessfulSyncTime() == null ^ this.getLastSuccessfulSyncTime() == null)
return false;
if (other.getLastSuccessfulSyncTime() != null && other.getLastSuccessfulSyncTime().equals(this.getLastSuccessfulSyncTime()) == false)
return false;
if (other.getSyncLastModifiedTime() == null ^ this.getSyncLastModifiedTime() == null)
return false;
if (other.getSyncLastModifiedTime() != null && other.getSyncLastModifiedTime().equals(this.getSyncLastModifiedTime()) == false)
return false;
if (other.getLastStatus() == null ^ this.getLastStatus() == null)
return false;
if (other.getLastStatus() != null && other.getLastStatus().equals(this.getLastStatus()) == false)
return false;
if (other.getSyncCreatedTime() == null ^ this.getSyncCreatedTime() == null)
return false;
if (other.getSyncCreatedTime() != null && other.getSyncCreatedTime().equals(this.getSyncCreatedTime()) == false)
return false;
if (other.getLastSyncStatusMessage() == null ^ this.getLastSyncStatusMessage() == null)
return false;
if (other.getLastSyncStatusMessage() != null && other.getLastSyncStatusMessage().equals(this.getLastSyncStatusMessage()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSyncName() == null) ? 0 : getSyncName().hashCode());
hashCode = prime * hashCode + ((getSyncType() == null) ? 0 : getSyncType().hashCode());
hashCode = prime * hashCode + ((getSyncSource() == null) ? 0 : getSyncSource().hashCode());
hashCode = prime * hashCode + ((getS3Destination() == null) ? 0 : getS3Destination().hashCode());
hashCode = prime * hashCode + ((getLastSyncTime() == null) ? 0 : getLastSyncTime().hashCode());
hashCode = prime * hashCode + ((getLastSuccessfulSyncTime() == null) ? 0 : getLastSuccessfulSyncTime().hashCode());
hashCode = prime * hashCode + ((getSyncLastModifiedTime() == null) ? 0 : getSyncLastModifiedTime().hashCode());
hashCode = prime * hashCode + ((getLastStatus() == null) ? 0 : getLastStatus().hashCode());
hashCode = prime * hashCode + ((getSyncCreatedTime() == null) ? 0 : getSyncCreatedTime().hashCode());
hashCode = prime * hashCode + ((getLastSyncStatusMessage() == null) ? 0 : getLastSyncStatusMessage().hashCode());
return hashCode;
}
@Override
public ResourceDataSyncItem clone() {
try {
return (ResourceDataSyncItem) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.ResourceDataSyncItemMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}