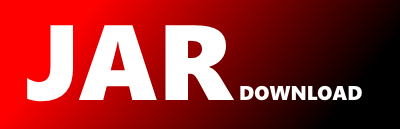
com.amazonaws.services.simplesystemsmanagement.model.ResourceDataSyncS3Destination Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about the target S3 bucket for the resource data sync.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ResourceDataSyncS3Destination implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the S3 bucket where the aggregated data is stored.
*
*/
private String bucketName;
/**
*
* An Amazon S3 prefix for the bucket.
*
*/
private String prefix;
/**
*
* A supported sync format. The following format is currently supported: JsonSerDe
*
*/
private String syncFormat;
/**
*
* The Amazon Web Services Region with the S3 bucket targeted by the resource data sync.
*
*/
private String region;
/**
*
* The ARN of an encryption key for a destination in Amazon S3. Must belong to the same Region as the destination S3
* bucket.
*
*/
private String aWSKMSKeyARN;
/**
*
* Enables destination data sharing. By default, this field is null
.
*
*/
private ResourceDataSyncDestinationDataSharing destinationDataSharing;
/**
*
* The name of the S3 bucket where the aggregated data is stored.
*
*
* @param bucketName
* The name of the S3 bucket where the aggregated data is stored.
*/
public void setBucketName(String bucketName) {
this.bucketName = bucketName;
}
/**
*
* The name of the S3 bucket where the aggregated data is stored.
*
*
* @return The name of the S3 bucket where the aggregated data is stored.
*/
public String getBucketName() {
return this.bucketName;
}
/**
*
* The name of the S3 bucket where the aggregated data is stored.
*
*
* @param bucketName
* The name of the S3 bucket where the aggregated data is stored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncS3Destination withBucketName(String bucketName) {
setBucketName(bucketName);
return this;
}
/**
*
* An Amazon S3 prefix for the bucket.
*
*
* @param prefix
* An Amazon S3 prefix for the bucket.
*/
public void setPrefix(String prefix) {
this.prefix = prefix;
}
/**
*
* An Amazon S3 prefix for the bucket.
*
*
* @return An Amazon S3 prefix for the bucket.
*/
public String getPrefix() {
return this.prefix;
}
/**
*
* An Amazon S3 prefix for the bucket.
*
*
* @param prefix
* An Amazon S3 prefix for the bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncS3Destination withPrefix(String prefix) {
setPrefix(prefix);
return this;
}
/**
*
* A supported sync format. The following format is currently supported: JsonSerDe
*
*
* @param syncFormat
* A supported sync format. The following format is currently supported: JsonSerDe
* @see ResourceDataSyncS3Format
*/
public void setSyncFormat(String syncFormat) {
this.syncFormat = syncFormat;
}
/**
*
* A supported sync format. The following format is currently supported: JsonSerDe
*
*
* @return A supported sync format. The following format is currently supported: JsonSerDe
* @see ResourceDataSyncS3Format
*/
public String getSyncFormat() {
return this.syncFormat;
}
/**
*
* A supported sync format. The following format is currently supported: JsonSerDe
*
*
* @param syncFormat
* A supported sync format. The following format is currently supported: JsonSerDe
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceDataSyncS3Format
*/
public ResourceDataSyncS3Destination withSyncFormat(String syncFormat) {
setSyncFormat(syncFormat);
return this;
}
/**
*
* A supported sync format. The following format is currently supported: JsonSerDe
*
*
* @param syncFormat
* A supported sync format. The following format is currently supported: JsonSerDe
* @see ResourceDataSyncS3Format
*/
public void setSyncFormat(ResourceDataSyncS3Format syncFormat) {
withSyncFormat(syncFormat);
}
/**
*
* A supported sync format. The following format is currently supported: JsonSerDe
*
*
* @param syncFormat
* A supported sync format. The following format is currently supported: JsonSerDe
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceDataSyncS3Format
*/
public ResourceDataSyncS3Destination withSyncFormat(ResourceDataSyncS3Format syncFormat) {
this.syncFormat = syncFormat.toString();
return this;
}
/**
*
* The Amazon Web Services Region with the S3 bucket targeted by the resource data sync.
*
*
* @param region
* The Amazon Web Services Region with the S3 bucket targeted by the resource data sync.
*/
public void setRegion(String region) {
this.region = region;
}
/**
*
* The Amazon Web Services Region with the S3 bucket targeted by the resource data sync.
*
*
* @return The Amazon Web Services Region with the S3 bucket targeted by the resource data sync.
*/
public String getRegion() {
return this.region;
}
/**
*
* The Amazon Web Services Region with the S3 bucket targeted by the resource data sync.
*
*
* @param region
* The Amazon Web Services Region with the S3 bucket targeted by the resource data sync.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncS3Destination withRegion(String region) {
setRegion(region);
return this;
}
/**
*
* The ARN of an encryption key for a destination in Amazon S3. Must belong to the same Region as the destination S3
* bucket.
*
*
* @param aWSKMSKeyARN
* The ARN of an encryption key for a destination in Amazon S3. Must belong to the same Region as the
* destination S3 bucket.
*/
public void setAWSKMSKeyARN(String aWSKMSKeyARN) {
this.aWSKMSKeyARN = aWSKMSKeyARN;
}
/**
*
* The ARN of an encryption key for a destination in Amazon S3. Must belong to the same Region as the destination S3
* bucket.
*
*
* @return The ARN of an encryption key for a destination in Amazon S3. Must belong to the same Region as the
* destination S3 bucket.
*/
public String getAWSKMSKeyARN() {
return this.aWSKMSKeyARN;
}
/**
*
* The ARN of an encryption key for a destination in Amazon S3. Must belong to the same Region as the destination S3
* bucket.
*
*
* @param aWSKMSKeyARN
* The ARN of an encryption key for a destination in Amazon S3. Must belong to the same Region as the
* destination S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncS3Destination withAWSKMSKeyARN(String aWSKMSKeyARN) {
setAWSKMSKeyARN(aWSKMSKeyARN);
return this;
}
/**
*
* Enables destination data sharing. By default, this field is null
.
*
*
* @param destinationDataSharing
* Enables destination data sharing. By default, this field is null
.
*/
public void setDestinationDataSharing(ResourceDataSyncDestinationDataSharing destinationDataSharing) {
this.destinationDataSharing = destinationDataSharing;
}
/**
*
* Enables destination data sharing. By default, this field is null
.
*
*
* @return Enables destination data sharing. By default, this field is null
.
*/
public ResourceDataSyncDestinationDataSharing getDestinationDataSharing() {
return this.destinationDataSharing;
}
/**
*
* Enables destination data sharing. By default, this field is null
.
*
*
* @param destinationDataSharing
* Enables destination data sharing. By default, this field is null
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceDataSyncS3Destination withDestinationDataSharing(ResourceDataSyncDestinationDataSharing destinationDataSharing) {
setDestinationDataSharing(destinationDataSharing);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBucketName() != null)
sb.append("BucketName: ").append(getBucketName()).append(",");
if (getPrefix() != null)
sb.append("Prefix: ").append(getPrefix()).append(",");
if (getSyncFormat() != null)
sb.append("SyncFormat: ").append(getSyncFormat()).append(",");
if (getRegion() != null)
sb.append("Region: ").append(getRegion()).append(",");
if (getAWSKMSKeyARN() != null)
sb.append("AWSKMSKeyARN: ").append(getAWSKMSKeyARN()).append(",");
if (getDestinationDataSharing() != null)
sb.append("DestinationDataSharing: ").append(getDestinationDataSharing());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResourceDataSyncS3Destination == false)
return false;
ResourceDataSyncS3Destination other = (ResourceDataSyncS3Destination) obj;
if (other.getBucketName() == null ^ this.getBucketName() == null)
return false;
if (other.getBucketName() != null && other.getBucketName().equals(this.getBucketName()) == false)
return false;
if (other.getPrefix() == null ^ this.getPrefix() == null)
return false;
if (other.getPrefix() != null && other.getPrefix().equals(this.getPrefix()) == false)
return false;
if (other.getSyncFormat() == null ^ this.getSyncFormat() == null)
return false;
if (other.getSyncFormat() != null && other.getSyncFormat().equals(this.getSyncFormat()) == false)
return false;
if (other.getRegion() == null ^ this.getRegion() == null)
return false;
if (other.getRegion() != null && other.getRegion().equals(this.getRegion()) == false)
return false;
if (other.getAWSKMSKeyARN() == null ^ this.getAWSKMSKeyARN() == null)
return false;
if (other.getAWSKMSKeyARN() != null && other.getAWSKMSKeyARN().equals(this.getAWSKMSKeyARN()) == false)
return false;
if (other.getDestinationDataSharing() == null ^ this.getDestinationDataSharing() == null)
return false;
if (other.getDestinationDataSharing() != null && other.getDestinationDataSharing().equals(this.getDestinationDataSharing()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBucketName() == null) ? 0 : getBucketName().hashCode());
hashCode = prime * hashCode + ((getPrefix() == null) ? 0 : getPrefix().hashCode());
hashCode = prime * hashCode + ((getSyncFormat() == null) ? 0 : getSyncFormat().hashCode());
hashCode = prime * hashCode + ((getRegion() == null) ? 0 : getRegion().hashCode());
hashCode = prime * hashCode + ((getAWSKMSKeyARN() == null) ? 0 : getAWSKMSKeyARN().hashCode());
hashCode = prime * hashCode + ((getDestinationDataSharing() == null) ? 0 : getDestinationDataSharing().hashCode());
return hashCode;
}
@Override
public ResourceDataSyncS3Destination clone() {
try {
return (ResourceDataSyncS3Destination) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.ResourceDataSyncS3DestinationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}