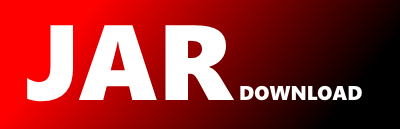
com.amazonaws.services.simplesystemsmanagement.model.SendCommandRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SendCommandRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The IDs of the managed nodes where the command should run. Specifying managed node IDs is most useful when you
* are targeting a limited number of managed nodes, though you can specify up to 50 IDs.
*
*
* To target a larger number of managed nodes, or if you prefer not to list individual node IDs, we recommend using
* the Targets
option instead. Using Targets
, which accepts tag key-value pairs to
* identify the managed nodes to send commands to, you can a send command to tens, hundreds, or thousands of nodes
* at once.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList instanceIds;
/**
*
* An array of search criteria that targets managed nodes using a Key,Value
combination that you
* specify. Specifying targets is most useful when you want to send a command to a large number of managed nodes at
* once. Using Targets
, which accepts tag key-value pairs to identify managed nodes, you can send a
* command to tens, hundreds, or thousands of nodes at once.
*
*
* To send a command to a smaller number of managed nodes, you can use the InstanceIds
option instead.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The name of the Amazon Web Services Systems Manager document (SSM document) to run. This can be a public document
* or a custom document. To run a shared document belonging to another account, specify the document Amazon Resource
* Name (ARN). For more information about how to use shared documents, see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
*
*
* If you specify a document name or ARN that hasn't been shared with your account, you receive an
* InvalidDocument
error.
*
*
*/
private String documentName;
/**
*
* The SSM document version to use in the request. You can specify $DEFAULT, $LATEST, or a specific version number.
* If you run commands by using the Command Line Interface (Amazon Web Services CLI), then you must escape the first
* two options by using a backslash. If you specify a version number, then you don't need to use the backslash. For
* example:
*
*
* --document-version "\$DEFAULT"
*
*
* --document-version "\$LATEST"
*
*
* --document-version "3"
*
*/
private String documentVersion;
/**
*
* The Sha256 or Sha1 hash created by the system when the document was created.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*/
private String documentHash;
/**
*
* Sha256 or Sha1.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*/
private String documentHashType;
/**
*
* If this time is reached and the command hasn't already started running, it won't run.
*
*/
private Integer timeoutSeconds;
/**
*
* User-specified information about the command, such as a brief description of what the command should do.
*
*/
private String comment;
/**
*
* The required and optional parameters specified in the document being run.
*
*/
private java.util.Map> parameters;
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*
*/
private String outputS3Region;
/**
*
* The name of the S3 bucket where command execution responses should be stored.
*
*/
private String outputS3BucketName;
/**
*
* The directory structure within the S3 bucket where the responses should be stored.
*
*/
private String outputS3KeyPrefix;
/**
*
* (Optional) The maximum number of managed nodes that are allowed to run the command at the same time. You can
* specify a number such as 10 or a percentage such as 10%. The default value is 50
. For more
* information about how to use MaxConcurrency
, see Using concurrency controls in the Amazon Web Services Systems Manager User Guide.
*
*/
private String maxConcurrency;
/**
*
* The maximum number of errors allowed without the command failing. When the command fails one more time beyond the
* value of MaxErrors
, the systems stops sending the command to additional targets. You can specify a
* number like 10 or a percentage like 10%. The default value is 0
. For more information about how to
* use MaxErrors
, see Using error controls in the Amazon Web Services Systems Manager User Guide.
*
*/
private String maxErrors;
/**
*
* The ARN of the Identity and Access Management (IAM) service role to use to publish Amazon Simple Notification
* Service (Amazon SNS) notifications for Run Command commands.
*
*
* This role must provide the sns:Publish
permission for your notification topic. For information about
* creating and using this service role, see Monitoring
* Systems Manager status changes using Amazon SNS notifications in the Amazon Web Services Systems Manager
* User Guide.
*
*/
private String serviceRoleArn;
/**
*
* Configurations for sending notifications.
*
*/
private NotificationConfig notificationConfig;
/**
*
* Enables Amazon Web Services Systems Manager to send Run Command output to Amazon CloudWatch Logs. Run Command is
* a capability of Amazon Web Services Systems Manager.
*
*/
private CloudWatchOutputConfig cloudWatchOutputConfig;
/**
*
* The CloudWatch alarm you want to apply to your command.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The IDs of the managed nodes where the command should run. Specifying managed node IDs is most useful when you
* are targeting a limited number of managed nodes, though you can specify up to 50 IDs.
*
*
* To target a larger number of managed nodes, or if you prefer not to list individual node IDs, we recommend using
* the Targets
option instead. Using Targets
, which accepts tag key-value pairs to
* identify the managed nodes to send commands to, you can a send command to tens, hundreds, or thousands of nodes
* at once.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*
* @return The IDs of the managed nodes where the command should run. Specifying managed node IDs is most useful
* when you are targeting a limited number of managed nodes, though you can specify up to 50 IDs.
*
* To target a larger number of managed nodes, or if you prefer not to list individual node IDs, we
* recommend using the Targets
option instead. Using Targets
, which accepts tag
* key-value pairs to identify the managed nodes to send commands to, you can a send command to tens,
* hundreds, or thousands of nodes at once.
*
*
* For more information about how to use targets, see Run
* commands at scale in the Amazon Web Services Systems Manager User Guide.
*/
public java.util.List getInstanceIds() {
if (instanceIds == null) {
instanceIds = new com.amazonaws.internal.SdkInternalList();
}
return instanceIds;
}
/**
*
* The IDs of the managed nodes where the command should run. Specifying managed node IDs is most useful when you
* are targeting a limited number of managed nodes, though you can specify up to 50 IDs.
*
*
* To target a larger number of managed nodes, or if you prefer not to list individual node IDs, we recommend using
* the Targets
option instead. Using Targets
, which accepts tag key-value pairs to
* identify the managed nodes to send commands to, you can a send command to tens, hundreds, or thousands of nodes
* at once.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*
* @param instanceIds
* The IDs of the managed nodes where the command should run. Specifying managed node IDs is most useful when
* you are targeting a limited number of managed nodes, though you can specify up to 50 IDs.
*
* To target a larger number of managed nodes, or if you prefer not to list individual node IDs, we recommend
* using the Targets
option instead. Using Targets
, which accepts tag key-value
* pairs to identify the managed nodes to send commands to, you can a send command to tens, hundreds, or
* thousands of nodes at once.
*
*
* For more information about how to use targets, see Run
* commands at scale in the Amazon Web Services Systems Manager User Guide.
*/
public void setInstanceIds(java.util.Collection instanceIds) {
if (instanceIds == null) {
this.instanceIds = null;
return;
}
this.instanceIds = new com.amazonaws.internal.SdkInternalList(instanceIds);
}
/**
*
* The IDs of the managed nodes where the command should run. Specifying managed node IDs is most useful when you
* are targeting a limited number of managed nodes, though you can specify up to 50 IDs.
*
*
* To target a larger number of managed nodes, or if you prefer not to list individual node IDs, we recommend using
* the Targets
option instead. Using Targets
, which accepts tag key-value pairs to
* identify the managed nodes to send commands to, you can a send command to tens, hundreds, or thousands of nodes
* at once.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInstanceIds(java.util.Collection)} or {@link #withInstanceIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param instanceIds
* The IDs of the managed nodes where the command should run. Specifying managed node IDs is most useful when
* you are targeting a limited number of managed nodes, though you can specify up to 50 IDs.
*
* To target a larger number of managed nodes, or if you prefer not to list individual node IDs, we recommend
* using the Targets
option instead. Using Targets
, which accepts tag key-value
* pairs to identify the managed nodes to send commands to, you can a send command to tens, hundreds, or
* thousands of nodes at once.
*
*
* For more information about how to use targets, see Run
* commands at scale in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withInstanceIds(String... instanceIds) {
if (this.instanceIds == null) {
setInstanceIds(new com.amazonaws.internal.SdkInternalList(instanceIds.length));
}
for (String ele : instanceIds) {
this.instanceIds.add(ele);
}
return this;
}
/**
*
* The IDs of the managed nodes where the command should run. Specifying managed node IDs is most useful when you
* are targeting a limited number of managed nodes, though you can specify up to 50 IDs.
*
*
* To target a larger number of managed nodes, or if you prefer not to list individual node IDs, we recommend using
* the Targets
option instead. Using Targets
, which accepts tag key-value pairs to
* identify the managed nodes to send commands to, you can a send command to tens, hundreds, or thousands of nodes
* at once.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*
* @param instanceIds
* The IDs of the managed nodes where the command should run. Specifying managed node IDs is most useful when
* you are targeting a limited number of managed nodes, though you can specify up to 50 IDs.
*
* To target a larger number of managed nodes, or if you prefer not to list individual node IDs, we recommend
* using the Targets
option instead. Using Targets
, which accepts tag key-value
* pairs to identify the managed nodes to send commands to, you can a send command to tens, hundreds, or
* thousands of nodes at once.
*
*
* For more information about how to use targets, see Run
* commands at scale in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withInstanceIds(java.util.Collection instanceIds) {
setInstanceIds(instanceIds);
return this;
}
/**
*
* An array of search criteria that targets managed nodes using a Key,Value
combination that you
* specify. Specifying targets is most useful when you want to send a command to a large number of managed nodes at
* once. Using Targets
, which accepts tag key-value pairs to identify managed nodes, you can send a
* command to tens, hundreds, or thousands of nodes at once.
*
*
* To send a command to a smaller number of managed nodes, you can use the InstanceIds
option instead.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*
* @return An array of search criteria that targets managed nodes using a Key,Value
combination that
* you specify. Specifying targets is most useful when you want to send a command to a large number of
* managed nodes at once. Using Targets
, which accepts tag key-value pairs to identify managed
* nodes, you can send a command to tens, hundreds, or thousands of nodes at once.
*
* To send a command to a smaller number of managed nodes, you can use the InstanceIds
option
* instead.
*
*
* For more information about how to use targets, see Run
* commands at scale in the Amazon Web Services Systems Manager User Guide.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* An array of search criteria that targets managed nodes using a Key,Value
combination that you
* specify. Specifying targets is most useful when you want to send a command to a large number of managed nodes at
* once. Using Targets
, which accepts tag key-value pairs to identify managed nodes, you can send a
* command to tens, hundreds, or thousands of nodes at once.
*
*
* To send a command to a smaller number of managed nodes, you can use the InstanceIds
option instead.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*
* @param targets
* An array of search criteria that targets managed nodes using a Key,Value
combination that you
* specify. Specifying targets is most useful when you want to send a command to a large number of managed
* nodes at once. Using Targets
, which accepts tag key-value pairs to identify managed nodes,
* you can send a command to tens, hundreds, or thousands of nodes at once.
*
* To send a command to a smaller number of managed nodes, you can use the InstanceIds
option
* instead.
*
*
* For more information about how to use targets, see Run
* commands at scale in the Amazon Web Services Systems Manager User Guide.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* An array of search criteria that targets managed nodes using a Key,Value
combination that you
* specify. Specifying targets is most useful when you want to send a command to a large number of managed nodes at
* once. Using Targets
, which accepts tag key-value pairs to identify managed nodes, you can send a
* command to tens, hundreds, or thousands of nodes at once.
*
*
* To send a command to a smaller number of managed nodes, you can use the InstanceIds
option instead.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* An array of search criteria that targets managed nodes using a Key,Value
combination that you
* specify. Specifying targets is most useful when you want to send a command to a large number of managed
* nodes at once. Using Targets
, which accepts tag key-value pairs to identify managed nodes,
* you can send a command to tens, hundreds, or thousands of nodes at once.
*
* To send a command to a smaller number of managed nodes, you can use the InstanceIds
option
* instead.
*
*
* For more information about how to use targets, see Run
* commands at scale in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* An array of search criteria that targets managed nodes using a Key,Value
combination that you
* specify. Specifying targets is most useful when you want to send a command to a large number of managed nodes at
* once. Using Targets
, which accepts tag key-value pairs to identify managed nodes, you can send a
* command to tens, hundreds, or thousands of nodes at once.
*
*
* To send a command to a smaller number of managed nodes, you can use the InstanceIds
option instead.
*
*
* For more information about how to use targets, see Run commands at
* scale in the Amazon Web Services Systems Manager User Guide.
*
*
* @param targets
* An array of search criteria that targets managed nodes using a Key,Value
combination that you
* specify. Specifying targets is most useful when you want to send a command to a large number of managed
* nodes at once. Using Targets
, which accepts tag key-value pairs to identify managed nodes,
* you can send a command to tens, hundreds, or thousands of nodes at once.
*
* To send a command to a smaller number of managed nodes, you can use the InstanceIds
option
* instead.
*
*
* For more information about how to use targets, see Run
* commands at scale in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The name of the Amazon Web Services Systems Manager document (SSM document) to run. This can be a public document
* or a custom document. To run a shared document belonging to another account, specify the document Amazon Resource
* Name (ARN). For more information about how to use shared documents, see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
*
*
* If you specify a document name or ARN that hasn't been shared with your account, you receive an
* InvalidDocument
error.
*
*
*
* @param documentName
* The name of the Amazon Web Services Systems Manager document (SSM document) to run. This can be a public
* document or a custom document. To run a shared document belonging to another account, specify the document
* Amazon Resource Name (ARN). For more information about how to use shared documents, see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
* If you specify a document name or ARN that hasn't been shared with your account, you receive an
* InvalidDocument
error.
*
*/
public void setDocumentName(String documentName) {
this.documentName = documentName;
}
/**
*
* The name of the Amazon Web Services Systems Manager document (SSM document) to run. This can be a public document
* or a custom document. To run a shared document belonging to another account, specify the document Amazon Resource
* Name (ARN). For more information about how to use shared documents, see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
*
*
* If you specify a document name or ARN that hasn't been shared with your account, you receive an
* InvalidDocument
error.
*
*
*
* @return The name of the Amazon Web Services Systems Manager document (SSM document) to run. This can be a public
* document or a custom document. To run a shared document belonging to another account, specify the
* document Amazon Resource Name (ARN). For more information about how to use shared documents, see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
* If you specify a document name or ARN that hasn't been shared with your account, you receive an
* InvalidDocument
error.
*
*/
public String getDocumentName() {
return this.documentName;
}
/**
*
* The name of the Amazon Web Services Systems Manager document (SSM document) to run. This can be a public document
* or a custom document. To run a shared document belonging to another account, specify the document Amazon Resource
* Name (ARN). For more information about how to use shared documents, see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
*
*
* If you specify a document name or ARN that hasn't been shared with your account, you receive an
* InvalidDocument
error.
*
*
*
* @param documentName
* The name of the Amazon Web Services Systems Manager document (SSM document) to run. This can be a public
* document or a custom document. To run a shared document belonging to another account, specify the document
* Amazon Resource Name (ARN). For more information about how to use shared documents, see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
* If you specify a document name or ARN that hasn't been shared with your account, you receive an
* InvalidDocument
error.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withDocumentName(String documentName) {
setDocumentName(documentName);
return this;
}
/**
*
* The SSM document version to use in the request. You can specify $DEFAULT, $LATEST, or a specific version number.
* If you run commands by using the Command Line Interface (Amazon Web Services CLI), then you must escape the first
* two options by using a backslash. If you specify a version number, then you don't need to use the backslash. For
* example:
*
*
* --document-version "\$DEFAULT"
*
*
* --document-version "\$LATEST"
*
*
* --document-version "3"
*
*
* @param documentVersion
* The SSM document version to use in the request. You can specify $DEFAULT, $LATEST, or a specific version
* number. If you run commands by using the Command Line Interface (Amazon Web Services CLI), then you must
* escape the first two options by using a backslash. If you specify a version number, then you don't need to
* use the backslash. For example:
*
* --document-version "\$DEFAULT"
*
*
* --document-version "\$LATEST"
*
*
* --document-version "3"
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The SSM document version to use in the request. You can specify $DEFAULT, $LATEST, or a specific version number.
* If you run commands by using the Command Line Interface (Amazon Web Services CLI), then you must escape the first
* two options by using a backslash. If you specify a version number, then you don't need to use the backslash. For
* example:
*
*
* --document-version "\$DEFAULT"
*
*
* --document-version "\$LATEST"
*
*
* --document-version "3"
*
*
* @return The SSM document version to use in the request. You can specify $DEFAULT, $LATEST, or a specific version
* number. If you run commands by using the Command Line Interface (Amazon Web Services CLI), then you must
* escape the first two options by using a backslash. If you specify a version number, then you don't need
* to use the backslash. For example:
*
* --document-version "\$DEFAULT"
*
*
* --document-version "\$LATEST"
*
*
* --document-version "3"
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The SSM document version to use in the request. You can specify $DEFAULT, $LATEST, or a specific version number.
* If you run commands by using the Command Line Interface (Amazon Web Services CLI), then you must escape the first
* two options by using a backslash. If you specify a version number, then you don't need to use the backslash. For
* example:
*
*
* --document-version "\$DEFAULT"
*
*
* --document-version "\$LATEST"
*
*
* --document-version "3"
*
*
* @param documentVersion
* The SSM document version to use in the request. You can specify $DEFAULT, $LATEST, or a specific version
* number. If you run commands by using the Command Line Interface (Amazon Web Services CLI), then you must
* escape the first two options by using a backslash. If you specify a version number, then you don't need to
* use the backslash. For example:
*
* --document-version "\$DEFAULT"
*
*
* --document-version "\$LATEST"
*
*
* --document-version "3"
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* The Sha256 or Sha1 hash created by the system when the document was created.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*
* @param documentHash
* The Sha256 or Sha1 hash created by the system when the document was created.
*
* Sha1 hashes have been deprecated.
*
*/
public void setDocumentHash(String documentHash) {
this.documentHash = documentHash;
}
/**
*
* The Sha256 or Sha1 hash created by the system when the document was created.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*
* @return The Sha256 or Sha1 hash created by the system when the document was created.
*
* Sha1 hashes have been deprecated.
*
*/
public String getDocumentHash() {
return this.documentHash;
}
/**
*
* The Sha256 or Sha1 hash created by the system when the document was created.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*
* @param documentHash
* The Sha256 or Sha1 hash created by the system when the document was created.
*
* Sha1 hashes have been deprecated.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withDocumentHash(String documentHash) {
setDocumentHash(documentHash);
return this;
}
/**
*
* Sha256 or Sha1.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*
* @param documentHashType
* Sha256 or Sha1.
*
* Sha1 hashes have been deprecated.
*
* @see DocumentHashType
*/
public void setDocumentHashType(String documentHashType) {
this.documentHashType = documentHashType;
}
/**
*
* Sha256 or Sha1.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*
* @return Sha256 or Sha1.
*
* Sha1 hashes have been deprecated.
*
* @see DocumentHashType
*/
public String getDocumentHashType() {
return this.documentHashType;
}
/**
*
* Sha256 or Sha1.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*
* @param documentHashType
* Sha256 or Sha1.
*
* Sha1 hashes have been deprecated.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentHashType
*/
public SendCommandRequest withDocumentHashType(String documentHashType) {
setDocumentHashType(documentHashType);
return this;
}
/**
*
* Sha256 or Sha1.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*
* @param documentHashType
* Sha256 or Sha1.
*
* Sha1 hashes have been deprecated.
*
* @see DocumentHashType
*/
public void setDocumentHashType(DocumentHashType documentHashType) {
withDocumentHashType(documentHashType);
}
/**
*
* Sha256 or Sha1.
*
*
*
* Sha1 hashes have been deprecated.
*
*
*
* @param documentHashType
* Sha256 or Sha1.
*
* Sha1 hashes have been deprecated.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DocumentHashType
*/
public SendCommandRequest withDocumentHashType(DocumentHashType documentHashType) {
this.documentHashType = documentHashType.toString();
return this;
}
/**
*
* If this time is reached and the command hasn't already started running, it won't run.
*
*
* @param timeoutSeconds
* If this time is reached and the command hasn't already started running, it won't run.
*/
public void setTimeoutSeconds(Integer timeoutSeconds) {
this.timeoutSeconds = timeoutSeconds;
}
/**
*
* If this time is reached and the command hasn't already started running, it won't run.
*
*
* @return If this time is reached and the command hasn't already started running, it won't run.
*/
public Integer getTimeoutSeconds() {
return this.timeoutSeconds;
}
/**
*
* If this time is reached and the command hasn't already started running, it won't run.
*
*
* @param timeoutSeconds
* If this time is reached and the command hasn't already started running, it won't run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withTimeoutSeconds(Integer timeoutSeconds) {
setTimeoutSeconds(timeoutSeconds);
return this;
}
/**
*
* User-specified information about the command, such as a brief description of what the command should do.
*
*
* @param comment
* User-specified information about the command, such as a brief description of what the command should do.
*/
public void setComment(String comment) {
this.comment = comment;
}
/**
*
* User-specified information about the command, such as a brief description of what the command should do.
*
*
* @return User-specified information about the command, such as a brief description of what the command should do.
*/
public String getComment() {
return this.comment;
}
/**
*
* User-specified information about the command, such as a brief description of what the command should do.
*
*
* @param comment
* User-specified information about the command, such as a brief description of what the command should do.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withComment(String comment) {
setComment(comment);
return this;
}
/**
*
* The required and optional parameters specified in the document being run.
*
*
* @return The required and optional parameters specified in the document being run.
*/
public java.util.Map> getParameters() {
return parameters;
}
/**
*
* The required and optional parameters specified in the document being run.
*
*
* @param parameters
* The required and optional parameters specified in the document being run.
*/
public void setParameters(java.util.Map> parameters) {
this.parameters = parameters;
}
/**
*
* The required and optional parameters specified in the document being run.
*
*
* @param parameters
* The required and optional parameters specified in the document being run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withParameters(java.util.Map> parameters) {
setParameters(parameters);
return this;
}
/**
* Add a single Parameters entry
*
* @see SendCommandRequest#withParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest addParametersEntry(String key, java.util.List value) {
if (null == this.parameters) {
this.parameters = new java.util.HashMap>();
}
if (this.parameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.parameters.put(key, value);
return this;
}
/**
* Removes all the entries added into Parameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest clearParametersEntries() {
this.parameters = null;
return this;
}
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*
*
* @param outputS3Region
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*/
public void setOutputS3Region(String outputS3Region) {
this.outputS3Region = outputS3Region;
}
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*
*
* @return (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*/
public String getOutputS3Region() {
return this.outputS3Region;
}
/**
*
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
*
*
* @param outputS3Region
* (Deprecated) You can no longer specify this parameter. The system ignores it. Instead, Systems Manager
* automatically determines the Amazon Web Services Region of the S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withOutputS3Region(String outputS3Region) {
setOutputS3Region(outputS3Region);
return this;
}
/**
*
* The name of the S3 bucket where command execution responses should be stored.
*
*
* @param outputS3BucketName
* The name of the S3 bucket where command execution responses should be stored.
*/
public void setOutputS3BucketName(String outputS3BucketName) {
this.outputS3BucketName = outputS3BucketName;
}
/**
*
* The name of the S3 bucket where command execution responses should be stored.
*
*
* @return The name of the S3 bucket where command execution responses should be stored.
*/
public String getOutputS3BucketName() {
return this.outputS3BucketName;
}
/**
*
* The name of the S3 bucket where command execution responses should be stored.
*
*
* @param outputS3BucketName
* The name of the S3 bucket where command execution responses should be stored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withOutputS3BucketName(String outputS3BucketName) {
setOutputS3BucketName(outputS3BucketName);
return this;
}
/**
*
* The directory structure within the S3 bucket where the responses should be stored.
*
*
* @param outputS3KeyPrefix
* The directory structure within the S3 bucket where the responses should be stored.
*/
public void setOutputS3KeyPrefix(String outputS3KeyPrefix) {
this.outputS3KeyPrefix = outputS3KeyPrefix;
}
/**
*
* The directory structure within the S3 bucket where the responses should be stored.
*
*
* @return The directory structure within the S3 bucket where the responses should be stored.
*/
public String getOutputS3KeyPrefix() {
return this.outputS3KeyPrefix;
}
/**
*
* The directory structure within the S3 bucket where the responses should be stored.
*
*
* @param outputS3KeyPrefix
* The directory structure within the S3 bucket where the responses should be stored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withOutputS3KeyPrefix(String outputS3KeyPrefix) {
setOutputS3KeyPrefix(outputS3KeyPrefix);
return this;
}
/**
*
* (Optional) The maximum number of managed nodes that are allowed to run the command at the same time. You can
* specify a number such as 10 or a percentage such as 10%. The default value is 50
. For more
* information about how to use MaxConcurrency
, see Using concurrency controls in the Amazon Web Services Systems Manager User Guide.
*
*
* @param maxConcurrency
* (Optional) The maximum number of managed nodes that are allowed to run the command at the same time. You
* can specify a number such as 10 or a percentage such as 10%. The default value is 50
. For
* more information about how to use MaxConcurrency
, see Using concurrency controls in the Amazon Web Services Systems Manager User Guide.
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* (Optional) The maximum number of managed nodes that are allowed to run the command at the same time. You can
* specify a number such as 10 or a percentage such as 10%. The default value is 50
. For more
* information about how to use MaxConcurrency
, see Using concurrency controls in the Amazon Web Services Systems Manager User Guide.
*
*
* @return (Optional) The maximum number of managed nodes that are allowed to run the command at the same time. You
* can specify a number such as 10 or a percentage such as 10%. The default value is 50
. For
* more information about how to use MaxConcurrency
, see Using concurrency controls in the Amazon Web Services Systems Manager User Guide.
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* (Optional) The maximum number of managed nodes that are allowed to run the command at the same time. You can
* specify a number such as 10 or a percentage such as 10%. The default value is 50
. For more
* information about how to use MaxConcurrency
, see Using concurrency controls in the Amazon Web Services Systems Manager User Guide.
*
*
* @param maxConcurrency
* (Optional) The maximum number of managed nodes that are allowed to run the command at the same time. You
* can specify a number such as 10 or a percentage such as 10%. The default value is 50
. For
* more information about how to use MaxConcurrency
, see Using concurrency controls in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The maximum number of errors allowed without the command failing. When the command fails one more time beyond the
* value of MaxErrors
, the systems stops sending the command to additional targets. You can specify a
* number like 10 or a percentage like 10%. The default value is 0
. For more information about how to
* use MaxErrors
, see Using error controls in the Amazon Web Services Systems Manager User Guide.
*
*
* @param maxErrors
* The maximum number of errors allowed without the command failing. When the command fails one more time
* beyond the value of MaxErrors
, the systems stops sending the command to additional targets.
* You can specify a number like 10 or a percentage like 10%. The default value is 0
. For more
* information about how to use MaxErrors
, see Using error controls in the Amazon Web Services Systems Manager User Guide.
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The maximum number of errors allowed without the command failing. When the command fails one more time beyond the
* value of MaxErrors
, the systems stops sending the command to additional targets. You can specify a
* number like 10 or a percentage like 10%. The default value is 0
. For more information about how to
* use MaxErrors
, see Using error controls in the Amazon Web Services Systems Manager User Guide.
*
*
* @return The maximum number of errors allowed without the command failing. When the command fails one more time
* beyond the value of MaxErrors
, the systems stops sending the command to additional targets.
* You can specify a number like 10 or a percentage like 10%. The default value is 0
. For more
* information about how to use MaxErrors
, see Using error controls in the Amazon Web Services Systems Manager User Guide.
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The maximum number of errors allowed without the command failing. When the command fails one more time beyond the
* value of MaxErrors
, the systems stops sending the command to additional targets. You can specify a
* number like 10 or a percentage like 10%. The default value is 0
. For more information about how to
* use MaxErrors
, see Using error controls in the Amazon Web Services Systems Manager User Guide.
*
*
* @param maxErrors
* The maximum number of errors allowed without the command failing. When the command fails one more time
* beyond the value of MaxErrors
, the systems stops sending the command to additional targets.
* You can specify a number like 10 or a percentage like 10%. The default value is 0
. For more
* information about how to use MaxErrors
, see Using error controls in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The ARN of the Identity and Access Management (IAM) service role to use to publish Amazon Simple Notification
* Service (Amazon SNS) notifications for Run Command commands.
*
*
* This role must provide the sns:Publish
permission for your notification topic. For information about
* creating and using this service role, see Monitoring
* Systems Manager status changes using Amazon SNS notifications in the Amazon Web Services Systems Manager
* User Guide.
*
*
* @param serviceRoleArn
* The ARN of the Identity and Access Management (IAM) service role to use to publish Amazon Simple
* Notification Service (Amazon SNS) notifications for Run Command commands.
*
* This role must provide the sns:Publish
permission for your notification topic. For
* information about creating and using this service role, see Monitoring Systems Manager status changes using Amazon SNS notifications in the Amazon Web
* Services Systems Manager User Guide.
*/
public void setServiceRoleArn(String serviceRoleArn) {
this.serviceRoleArn = serviceRoleArn;
}
/**
*
* The ARN of the Identity and Access Management (IAM) service role to use to publish Amazon Simple Notification
* Service (Amazon SNS) notifications for Run Command commands.
*
*
* This role must provide the sns:Publish
permission for your notification topic. For information about
* creating and using this service role, see Monitoring
* Systems Manager status changes using Amazon SNS notifications in the Amazon Web Services Systems Manager
* User Guide.
*
*
* @return The ARN of the Identity and Access Management (IAM) service role to use to publish Amazon Simple
* Notification Service (Amazon SNS) notifications for Run Command commands.
*
* This role must provide the sns:Publish
permission for your notification topic. For
* information about creating and using this service role, see Monitoring Systems Manager status changes using Amazon SNS notifications in the Amazon Web
* Services Systems Manager User Guide.
*/
public String getServiceRoleArn() {
return this.serviceRoleArn;
}
/**
*
* The ARN of the Identity and Access Management (IAM) service role to use to publish Amazon Simple Notification
* Service (Amazon SNS) notifications for Run Command commands.
*
*
* This role must provide the sns:Publish
permission for your notification topic. For information about
* creating and using this service role, see Monitoring
* Systems Manager status changes using Amazon SNS notifications in the Amazon Web Services Systems Manager
* User Guide.
*
*
* @param serviceRoleArn
* The ARN of the Identity and Access Management (IAM) service role to use to publish Amazon Simple
* Notification Service (Amazon SNS) notifications for Run Command commands.
*
* This role must provide the sns:Publish
permission for your notification topic. For
* information about creating and using this service role, see Monitoring Systems Manager status changes using Amazon SNS notifications in the Amazon Web
* Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withServiceRoleArn(String serviceRoleArn) {
setServiceRoleArn(serviceRoleArn);
return this;
}
/**
*
* Configurations for sending notifications.
*
*
* @param notificationConfig
* Configurations for sending notifications.
*/
public void setNotificationConfig(NotificationConfig notificationConfig) {
this.notificationConfig = notificationConfig;
}
/**
*
* Configurations for sending notifications.
*
*
* @return Configurations for sending notifications.
*/
public NotificationConfig getNotificationConfig() {
return this.notificationConfig;
}
/**
*
* Configurations for sending notifications.
*
*
* @param notificationConfig
* Configurations for sending notifications.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withNotificationConfig(NotificationConfig notificationConfig) {
setNotificationConfig(notificationConfig);
return this;
}
/**
*
* Enables Amazon Web Services Systems Manager to send Run Command output to Amazon CloudWatch Logs. Run Command is
* a capability of Amazon Web Services Systems Manager.
*
*
* @param cloudWatchOutputConfig
* Enables Amazon Web Services Systems Manager to send Run Command output to Amazon CloudWatch Logs. Run
* Command is a capability of Amazon Web Services Systems Manager.
*/
public void setCloudWatchOutputConfig(CloudWatchOutputConfig cloudWatchOutputConfig) {
this.cloudWatchOutputConfig = cloudWatchOutputConfig;
}
/**
*
* Enables Amazon Web Services Systems Manager to send Run Command output to Amazon CloudWatch Logs. Run Command is
* a capability of Amazon Web Services Systems Manager.
*
*
* @return Enables Amazon Web Services Systems Manager to send Run Command output to Amazon CloudWatch Logs. Run
* Command is a capability of Amazon Web Services Systems Manager.
*/
public CloudWatchOutputConfig getCloudWatchOutputConfig() {
return this.cloudWatchOutputConfig;
}
/**
*
* Enables Amazon Web Services Systems Manager to send Run Command output to Amazon CloudWatch Logs. Run Command is
* a capability of Amazon Web Services Systems Manager.
*
*
* @param cloudWatchOutputConfig
* Enables Amazon Web Services Systems Manager to send Run Command output to Amazon CloudWatch Logs. Run
* Command is a capability of Amazon Web Services Systems Manager.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withCloudWatchOutputConfig(CloudWatchOutputConfig cloudWatchOutputConfig) {
setCloudWatchOutputConfig(cloudWatchOutputConfig);
return this;
}
/**
*
* The CloudWatch alarm you want to apply to your command.
*
*
* @param alarmConfiguration
* The CloudWatch alarm you want to apply to your command.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The CloudWatch alarm you want to apply to your command.
*
*
* @return The CloudWatch alarm you want to apply to your command.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The CloudWatch alarm you want to apply to your command.
*
*
* @param alarmConfiguration
* The CloudWatch alarm you want to apply to your command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendCommandRequest withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getInstanceIds() != null)
sb.append("InstanceIds: ").append(getInstanceIds()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getDocumentName() != null)
sb.append("DocumentName: ").append(getDocumentName()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getDocumentHash() != null)
sb.append("DocumentHash: ").append(getDocumentHash()).append(",");
if (getDocumentHashType() != null)
sb.append("DocumentHashType: ").append(getDocumentHashType()).append(",");
if (getTimeoutSeconds() != null)
sb.append("TimeoutSeconds: ").append(getTimeoutSeconds()).append(",");
if (getComment() != null)
sb.append("Comment: ").append(getComment()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append("***Sensitive Data Redacted***").append(",");
if (getOutputS3Region() != null)
sb.append("OutputS3Region: ").append(getOutputS3Region()).append(",");
if (getOutputS3BucketName() != null)
sb.append("OutputS3BucketName: ").append(getOutputS3BucketName()).append(",");
if (getOutputS3KeyPrefix() != null)
sb.append("OutputS3KeyPrefix: ").append(getOutputS3KeyPrefix()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getServiceRoleArn() != null)
sb.append("ServiceRoleArn: ").append(getServiceRoleArn()).append(",");
if (getNotificationConfig() != null)
sb.append("NotificationConfig: ").append(getNotificationConfig()).append(",");
if (getCloudWatchOutputConfig() != null)
sb.append("CloudWatchOutputConfig: ").append(getCloudWatchOutputConfig()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SendCommandRequest == false)
return false;
SendCommandRequest other = (SendCommandRequest) obj;
if (other.getInstanceIds() == null ^ this.getInstanceIds() == null)
return false;
if (other.getInstanceIds() != null && other.getInstanceIds().equals(this.getInstanceIds()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getDocumentName() == null ^ this.getDocumentName() == null)
return false;
if (other.getDocumentName() != null && other.getDocumentName().equals(this.getDocumentName()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getDocumentHash() == null ^ this.getDocumentHash() == null)
return false;
if (other.getDocumentHash() != null && other.getDocumentHash().equals(this.getDocumentHash()) == false)
return false;
if (other.getDocumentHashType() == null ^ this.getDocumentHashType() == null)
return false;
if (other.getDocumentHashType() != null && other.getDocumentHashType().equals(this.getDocumentHashType()) == false)
return false;
if (other.getTimeoutSeconds() == null ^ this.getTimeoutSeconds() == null)
return false;
if (other.getTimeoutSeconds() != null && other.getTimeoutSeconds().equals(this.getTimeoutSeconds()) == false)
return false;
if (other.getComment() == null ^ this.getComment() == null)
return false;
if (other.getComment() != null && other.getComment().equals(this.getComment()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getOutputS3Region() == null ^ this.getOutputS3Region() == null)
return false;
if (other.getOutputS3Region() != null && other.getOutputS3Region().equals(this.getOutputS3Region()) == false)
return false;
if (other.getOutputS3BucketName() == null ^ this.getOutputS3BucketName() == null)
return false;
if (other.getOutputS3BucketName() != null && other.getOutputS3BucketName().equals(this.getOutputS3BucketName()) == false)
return false;
if (other.getOutputS3KeyPrefix() == null ^ this.getOutputS3KeyPrefix() == null)
return false;
if (other.getOutputS3KeyPrefix() != null && other.getOutputS3KeyPrefix().equals(this.getOutputS3KeyPrefix()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getServiceRoleArn() == null ^ this.getServiceRoleArn() == null)
return false;
if (other.getServiceRoleArn() != null && other.getServiceRoleArn().equals(this.getServiceRoleArn()) == false)
return false;
if (other.getNotificationConfig() == null ^ this.getNotificationConfig() == null)
return false;
if (other.getNotificationConfig() != null && other.getNotificationConfig().equals(this.getNotificationConfig()) == false)
return false;
if (other.getCloudWatchOutputConfig() == null ^ this.getCloudWatchOutputConfig() == null)
return false;
if (other.getCloudWatchOutputConfig() != null && other.getCloudWatchOutputConfig().equals(this.getCloudWatchOutputConfig()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getInstanceIds() == null) ? 0 : getInstanceIds().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getDocumentName() == null) ? 0 : getDocumentName().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getDocumentHash() == null) ? 0 : getDocumentHash().hashCode());
hashCode = prime * hashCode + ((getDocumentHashType() == null) ? 0 : getDocumentHashType().hashCode());
hashCode = prime * hashCode + ((getTimeoutSeconds() == null) ? 0 : getTimeoutSeconds().hashCode());
hashCode = prime * hashCode + ((getComment() == null) ? 0 : getComment().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getOutputS3Region() == null) ? 0 : getOutputS3Region().hashCode());
hashCode = prime * hashCode + ((getOutputS3BucketName() == null) ? 0 : getOutputS3BucketName().hashCode());
hashCode = prime * hashCode + ((getOutputS3KeyPrefix() == null) ? 0 : getOutputS3KeyPrefix().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getServiceRoleArn() == null) ? 0 : getServiceRoleArn().hashCode());
hashCode = prime * hashCode + ((getNotificationConfig() == null) ? 0 : getNotificationConfig().hashCode());
hashCode = prime * hashCode + ((getCloudWatchOutputConfig() == null) ? 0 : getCloudWatchOutputConfig().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
return hashCode;
}
@Override
public SendCommandRequest clone() {
return (SendCommandRequest) super.clone();
}
}