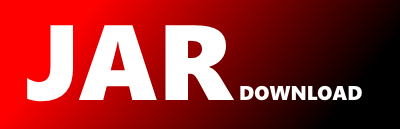
com.amazonaws.services.simplesystemsmanagement.model.StartAutomationExecutionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartAutomationExecutionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the SSM document to run. This can be a public document or a custom document. To run a shared document
* belonging to another account, specify the document ARN. For more information about how to use shared documents,
* see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
*/
private String documentName;
/**
*
* The version of the Automation runbook to use for this execution.
*
*/
private String documentVersion;
/**
*
* A key-value map of execution parameters, which match the declared parameters in the Automation runbook.
*
*/
private java.util.Map> parameters;
/**
*
* User-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format, and
* can't be reused.
*
*/
private String clientToken;
/**
*
* The execution mode of the automation. Valid modes include the following: Auto and Interactive. The default mode
* is Auto.
*
*/
private String mode;
/**
*
* The name of the parameter used as the target resource for the rate-controlled execution. Required if you specify
* targets.
*
*/
private String targetParameterName;
/**
*
* A key-value mapping to target resources. Required if you specify TargetParameterName.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*/
private com.amazonaws.internal.SdkInternalList>> targetMaps;
/**
*
* The maximum number of targets allowed to run this task in parallel. You can specify a number, such as 10, or a
* percentage, such as 10%. The default value is 10
.
*
*/
private String maxConcurrency;
/**
*
* The number of errors that are allowed before the system stops running the automation on additional targets. You
* can specify either an absolute number of errors, for example 10, or a percentage of the target set, for example
* 10%. If you specify 3, for example, the system stops running the automation when the fourth error is received. If
* you specify 0, then the system stops running the automation on additional targets after the first error result is
* returned. If you run an automation on 50 resources and set max-errors to 10%, then the system stops running the
* automation on additional targets when the sixth error is received.
*
*
* Executions that are already running an automation when max-errors is reached are allowed to complete, but some of
* these executions may fail as well. If you need to ensure that there won't be more than max-errors failed
* executions, set max-concurrency to 1 so the executions proceed one at a time.
*
*/
private String maxErrors;
/**
*
* A location is a combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to
* run the automation. Use this operation to start an automation in multiple Amazon Web Services Regions and
* multiple Amazon Web Services accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and Amazon Web Services accounts in the
* Amazon Web Services Systems Manager User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList targetLocations;
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for an automation. Tags
* enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For example,
* you might want to tag an automation to identify an environment or operating system. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=environment,Value=test
*
*
* -
*
* Key=OS,Value=Windows
*
*
*
*
*
* To add tags to an existing automation, use the AddTagsToResource operation.
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The CloudWatch alarm you want to apply to your automation.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The name of the SSM document to run. This can be a public document or a custom document. To run a shared document
* belonging to another account, specify the document ARN. For more information about how to use shared documents,
* see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
*
* @param documentName
* The name of the SSM document to run. This can be a public document or a custom document. To run a shared
* document belonging to another account, specify the document ARN. For more information about how to use
* shared documents, see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*/
public void setDocumentName(String documentName) {
this.documentName = documentName;
}
/**
*
* The name of the SSM document to run. This can be a public document or a custom document. To run a shared document
* belonging to another account, specify the document ARN. For more information about how to use shared documents,
* see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
*
* @return The name of the SSM document to run. This can be a public document or a custom document. To run a shared
* document belonging to another account, specify the document ARN. For more information about how to use
* shared documents, see Sharing
* SSM documents in the Amazon Web Services Systems Manager User Guide.
*/
public String getDocumentName() {
return this.documentName;
}
/**
*
* The name of the SSM document to run. This can be a public document or a custom document. To run a shared document
* belonging to another account, specify the document ARN. For more information about how to use shared documents,
* see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
*
*
* @param documentName
* The name of the SSM document to run. This can be a public document or a custom document. To run a shared
* document belonging to another account, specify the document ARN. For more information about how to use
* shared documents, see Sharing SSM
* documents in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withDocumentName(String documentName) {
setDocumentName(documentName);
return this;
}
/**
*
* The version of the Automation runbook to use for this execution.
*
*
* @param documentVersion
* The version of the Automation runbook to use for this execution.
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The version of the Automation runbook to use for this execution.
*
*
* @return The version of the Automation runbook to use for this execution.
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The version of the Automation runbook to use for this execution.
*
*
* @param documentVersion
* The version of the Automation runbook to use for this execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* A key-value map of execution parameters, which match the declared parameters in the Automation runbook.
*
*
* @return A key-value map of execution parameters, which match the declared parameters in the Automation runbook.
*/
public java.util.Map> getParameters() {
return parameters;
}
/**
*
* A key-value map of execution parameters, which match the declared parameters in the Automation runbook.
*
*
* @param parameters
* A key-value map of execution parameters, which match the declared parameters in the Automation runbook.
*/
public void setParameters(java.util.Map> parameters) {
this.parameters = parameters;
}
/**
*
* A key-value map of execution parameters, which match the declared parameters in the Automation runbook.
*
*
* @param parameters
* A key-value map of execution parameters, which match the declared parameters in the Automation runbook.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withParameters(java.util.Map> parameters) {
setParameters(parameters);
return this;
}
/**
* Add a single Parameters entry
*
* @see StartAutomationExecutionRequest#withParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest addParametersEntry(String key, java.util.List value) {
if (null == this.parameters) {
this.parameters = new java.util.HashMap>();
}
if (this.parameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.parameters.put(key, value);
return this;
}
/**
* Removes all the entries added into Parameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest clearParametersEntries() {
this.parameters = null;
return this;
}
/**
*
* User-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format, and
* can't be reused.
*
*
* @param clientToken
* User-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format,
* and can't be reused.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* User-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format, and
* can't be reused.
*
*
* @return User-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format,
* and can't be reused.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* User-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format, and
* can't be reused.
*
*
* @param clientToken
* User-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format,
* and can't be reused.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The execution mode of the automation. Valid modes include the following: Auto and Interactive. The default mode
* is Auto.
*
*
* @param mode
* The execution mode of the automation. Valid modes include the following: Auto and Interactive. The default
* mode is Auto.
* @see ExecutionMode
*/
public void setMode(String mode) {
this.mode = mode;
}
/**
*
* The execution mode of the automation. Valid modes include the following: Auto and Interactive. The default mode
* is Auto.
*
*
* @return The execution mode of the automation. Valid modes include the following: Auto and Interactive. The
* default mode is Auto.
* @see ExecutionMode
*/
public String getMode() {
return this.mode;
}
/**
*
* The execution mode of the automation. Valid modes include the following: Auto and Interactive. The default mode
* is Auto.
*
*
* @param mode
* The execution mode of the automation. Valid modes include the following: Auto and Interactive. The default
* mode is Auto.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionMode
*/
public StartAutomationExecutionRequest withMode(String mode) {
setMode(mode);
return this;
}
/**
*
* The execution mode of the automation. Valid modes include the following: Auto and Interactive. The default mode
* is Auto.
*
*
* @param mode
* The execution mode of the automation. Valid modes include the following: Auto and Interactive. The default
* mode is Auto.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionMode
*/
public StartAutomationExecutionRequest withMode(ExecutionMode mode) {
this.mode = mode.toString();
return this;
}
/**
*
* The name of the parameter used as the target resource for the rate-controlled execution. Required if you specify
* targets.
*
*
* @param targetParameterName
* The name of the parameter used as the target resource for the rate-controlled execution. Required if you
* specify targets.
*/
public void setTargetParameterName(String targetParameterName) {
this.targetParameterName = targetParameterName;
}
/**
*
* The name of the parameter used as the target resource for the rate-controlled execution. Required if you specify
* targets.
*
*
* @return The name of the parameter used as the target resource for the rate-controlled execution. Required if you
* specify targets.
*/
public String getTargetParameterName() {
return this.targetParameterName;
}
/**
*
* The name of the parameter used as the target resource for the rate-controlled execution. Required if you specify
* targets.
*
*
* @param targetParameterName
* The name of the parameter used as the target resource for the rate-controlled execution. Required if you
* specify targets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withTargetParameterName(String targetParameterName) {
setTargetParameterName(targetParameterName);
return this;
}
/**
*
* A key-value mapping to target resources. Required if you specify TargetParameterName.
*
*
* @return A key-value mapping to target resources. Required if you specify TargetParameterName.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* A key-value mapping to target resources. Required if you specify TargetParameterName.
*
*
* @param targets
* A key-value mapping to target resources. Required if you specify TargetParameterName.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* A key-value mapping to target resources. Required if you specify TargetParameterName.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* A key-value mapping to target resources. Required if you specify TargetParameterName.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* A key-value mapping to target resources. Required if you specify TargetParameterName.
*
*
* @param targets
* A key-value mapping to target resources. Required if you specify TargetParameterName.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* @return A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
*/
public java.util.List>> getTargetMaps() {
if (targetMaps == null) {
targetMaps = new com.amazonaws.internal.SdkInternalList>>();
}
return targetMaps;
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* @param targetMaps
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
*/
public void setTargetMaps(java.util.Collection>> targetMaps) {
if (targetMaps == null) {
this.targetMaps = null;
return;
}
this.targetMaps = new com.amazonaws.internal.SdkInternalList>>(targetMaps);
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetMaps(java.util.Collection)} or {@link #withTargetMaps(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param targetMaps
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withTargetMaps(java.util.Map>... targetMaps) {
if (this.targetMaps == null) {
setTargetMaps(new com.amazonaws.internal.SdkInternalList>>(targetMaps.length));
}
for (java.util.Map> ele : targetMaps) {
this.targetMaps.add(ele);
}
return this;
}
/**
*
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be specified
* together.
*
*
* @param targetMaps
* A key-value mapping of document parameters to target resources. Both Targets and TargetMaps can't be
* specified together.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withTargetMaps(java.util.Collection>> targetMaps) {
setTargetMaps(targetMaps);
return this;
}
/**
*
* The maximum number of targets allowed to run this task in parallel. You can specify a number, such as 10, or a
* percentage, such as 10%. The default value is 10
.
*
*
* @param maxConcurrency
* The maximum number of targets allowed to run this task in parallel. You can specify a number, such as 10,
* or a percentage, such as 10%. The default value is 10
.
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The maximum number of targets allowed to run this task in parallel. You can specify a number, such as 10, or a
* percentage, such as 10%. The default value is 10
.
*
*
* @return The maximum number of targets allowed to run this task in parallel. You can specify a number, such as 10,
* or a percentage, such as 10%. The default value is 10
.
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The maximum number of targets allowed to run this task in parallel. You can specify a number, such as 10, or a
* percentage, such as 10%. The default value is 10
.
*
*
* @param maxConcurrency
* The maximum number of targets allowed to run this task in parallel. You can specify a number, such as 10,
* or a percentage, such as 10%. The default value is 10
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The number of errors that are allowed before the system stops running the automation on additional targets. You
* can specify either an absolute number of errors, for example 10, or a percentage of the target set, for example
* 10%. If you specify 3, for example, the system stops running the automation when the fourth error is received. If
* you specify 0, then the system stops running the automation on additional targets after the first error result is
* returned. If you run an automation on 50 resources and set max-errors to 10%, then the system stops running the
* automation on additional targets when the sixth error is received.
*
*
* Executions that are already running an automation when max-errors is reached are allowed to complete, but some of
* these executions may fail as well. If you need to ensure that there won't be more than max-errors failed
* executions, set max-concurrency to 1 so the executions proceed one at a time.
*
*
* @param maxErrors
* The number of errors that are allowed before the system stops running the automation on additional
* targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops running the automation when
* the fourth error is received. If you specify 0, then the system stops running the automation on additional
* targets after the first error result is returned. If you run an automation on 50 resources and set
* max-errors to 10%, then the system stops running the automation on additional targets when the sixth error
* is received.
*
* Executions that are already running an automation when max-errors is reached are allowed to complete, but
* some of these executions may fail as well. If you need to ensure that there won't be more than max-errors
* failed executions, set max-concurrency to 1 so the executions proceed one at a time.
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The number of errors that are allowed before the system stops running the automation on additional targets. You
* can specify either an absolute number of errors, for example 10, or a percentage of the target set, for example
* 10%. If you specify 3, for example, the system stops running the automation when the fourth error is received. If
* you specify 0, then the system stops running the automation on additional targets after the first error result is
* returned. If you run an automation on 50 resources and set max-errors to 10%, then the system stops running the
* automation on additional targets when the sixth error is received.
*
*
* Executions that are already running an automation when max-errors is reached are allowed to complete, but some of
* these executions may fail as well. If you need to ensure that there won't be more than max-errors failed
* executions, set max-concurrency to 1 so the executions proceed one at a time.
*
*
* @return The number of errors that are allowed before the system stops running the automation on additional
* targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops running the automation when
* the fourth error is received. If you specify 0, then the system stops running the automation on
* additional targets after the first error result is returned. If you run an automation on 50 resources and
* set max-errors to 10%, then the system stops running the automation on additional targets when the sixth
* error is received.
*
* Executions that are already running an automation when max-errors is reached are allowed to complete, but
* some of these executions may fail as well. If you need to ensure that there won't be more than max-errors
* failed executions, set max-concurrency to 1 so the executions proceed one at a time.
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The number of errors that are allowed before the system stops running the automation on additional targets. You
* can specify either an absolute number of errors, for example 10, or a percentage of the target set, for example
* 10%. If you specify 3, for example, the system stops running the automation when the fourth error is received. If
* you specify 0, then the system stops running the automation on additional targets after the first error result is
* returned. If you run an automation on 50 resources and set max-errors to 10%, then the system stops running the
* automation on additional targets when the sixth error is received.
*
*
* Executions that are already running an automation when max-errors is reached are allowed to complete, but some of
* these executions may fail as well. If you need to ensure that there won't be more than max-errors failed
* executions, set max-concurrency to 1 so the executions proceed one at a time.
*
*
* @param maxErrors
* The number of errors that are allowed before the system stops running the automation on additional
* targets. You can specify either an absolute number of errors, for example 10, or a percentage of the
* target set, for example 10%. If you specify 3, for example, the system stops running the automation when
* the fourth error is received. If you specify 0, then the system stops running the automation on additional
* targets after the first error result is returned. If you run an automation on 50 resources and set
* max-errors to 10%, then the system stops running the automation on additional targets when the sixth error
* is received.
*
* Executions that are already running an automation when max-errors is reached are allowed to complete, but
* some of these executions may fail as well. If you need to ensure that there won't be more than max-errors
* failed executions, set max-concurrency to 1 so the executions proceed one at a time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* A location is a combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to
* run the automation. Use this operation to start an automation in multiple Amazon Web Services Regions and
* multiple Amazon Web Services accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and Amazon Web Services accounts in the
* Amazon Web Services Systems Manager User Guide.
*
*
* @return A location is a combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you
* want to run the automation. Use this operation to start an automation in multiple Amazon Web Services
* Regions and multiple Amazon Web Services accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and Amazon Web Services
* accounts in the Amazon Web Services Systems Manager User Guide.
*/
public java.util.List getTargetLocations() {
if (targetLocations == null) {
targetLocations = new com.amazonaws.internal.SdkInternalList();
}
return targetLocations;
}
/**
*
* A location is a combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to
* run the automation. Use this operation to start an automation in multiple Amazon Web Services Regions and
* multiple Amazon Web Services accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and Amazon Web Services accounts in the
* Amazon Web Services Systems Manager User Guide.
*
*
* @param targetLocations
* A location is a combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you
* want to run the automation. Use this operation to start an automation in multiple Amazon Web Services
* Regions and multiple Amazon Web Services accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and Amazon Web Services accounts
* in the Amazon Web Services Systems Manager User Guide.
*/
public void setTargetLocations(java.util.Collection targetLocations) {
if (targetLocations == null) {
this.targetLocations = null;
return;
}
this.targetLocations = new com.amazonaws.internal.SdkInternalList(targetLocations);
}
/**
*
* A location is a combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to
* run the automation. Use this operation to start an automation in multiple Amazon Web Services Regions and
* multiple Amazon Web Services accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and Amazon Web Services accounts in the
* Amazon Web Services Systems Manager User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetLocations(java.util.Collection)} or {@link #withTargetLocations(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param targetLocations
* A location is a combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you
* want to run the automation. Use this operation to start an automation in multiple Amazon Web Services
* Regions and multiple Amazon Web Services accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and Amazon Web Services accounts
* in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withTargetLocations(TargetLocation... targetLocations) {
if (this.targetLocations == null) {
setTargetLocations(new com.amazonaws.internal.SdkInternalList(targetLocations.length));
}
for (TargetLocation ele : targetLocations) {
this.targetLocations.add(ele);
}
return this;
}
/**
*
* A location is a combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you want to
* run the automation. Use this operation to start an automation in multiple Amazon Web Services Regions and
* multiple Amazon Web Services accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and Amazon Web Services accounts in the
* Amazon Web Services Systems Manager User Guide.
*
*
* @param targetLocations
* A location is a combination of Amazon Web Services Regions and/or Amazon Web Services accounts where you
* want to run the automation. Use this operation to start an automation in multiple Amazon Web Services
* Regions and multiple Amazon Web Services accounts. For more information, see Running Automation workflows in multiple Amazon Web Services Regions and Amazon Web Services accounts
* in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withTargetLocations(java.util.Collection targetLocations) {
setTargetLocations(targetLocations);
return this;
}
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for an automation. Tags
* enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For example,
* you might want to tag an automation to identify an environment or operating system. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=environment,Value=test
*
*
* -
*
* Key=OS,Value=Windows
*
*
*
*
*
* To add tags to an existing automation, use the AddTagsToResource operation.
*
*
*
* @return Optional metadata that you assign to a resource. You can specify a maximum of five tags for an
* automation. Tags enable you to categorize a resource in different ways, such as by purpose, owner, or
* environment. For example, you might want to tag an automation to identify an environment or operating
* system. In this case, you could specify the following key-value pairs:
*
* -
*
* Key=environment,Value=test
*
*
* -
*
* Key=OS,Value=Windows
*
*
*
*
*
* To add tags to an existing automation, use the AddTagsToResource operation.
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for an automation. Tags
* enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For example,
* you might want to tag an automation to identify an environment or operating system. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=environment,Value=test
*
*
* -
*
* Key=OS,Value=Windows
*
*
*
*
*
* To add tags to an existing automation, use the AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for an automation.
* Tags enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For
* example, you might want to tag an automation to identify an environment or operating system. In this case,
* you could specify the following key-value pairs:
*
* -
*
* Key=environment,Value=test
*
*
* -
*
* Key=OS,Value=Windows
*
*
*
*
*
* To add tags to an existing automation, use the AddTagsToResource operation.
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for an automation. Tags
* enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For example,
* you might want to tag an automation to identify an environment or operating system. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=environment,Value=test
*
*
* -
*
* Key=OS,Value=Windows
*
*
*
*
*
* To add tags to an existing automation, use the AddTagsToResource operation.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for an automation.
* Tags enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For
* example, you might want to tag an automation to identify an environment or operating system. In this case,
* you could specify the following key-value pairs:
*
* -
*
* Key=environment,Value=test
*
*
* -
*
* Key=OS,Value=Windows
*
*
*
*
*
* To add tags to an existing automation, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for an automation. Tags
* enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For example,
* you might want to tag an automation to identify an environment or operating system. In this case, you could
* specify the following key-value pairs:
*
*
* -
*
* Key=environment,Value=test
*
*
* -
*
* Key=OS,Value=Windows
*
*
*
*
*
* To add tags to an existing automation, use the AddTagsToResource operation.
*
*
*
* @param tags
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for an automation.
* Tags enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For
* example, you might want to tag an automation to identify an environment or operating system. In this case,
* you could specify the following key-value pairs:
*
* -
*
* Key=environment,Value=test
*
*
* -
*
* Key=OS,Value=Windows
*
*
*
*
*
* To add tags to an existing automation, use the AddTagsToResource operation.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The CloudWatch alarm you want to apply to your automation.
*
*
* @param alarmConfiguration
* The CloudWatch alarm you want to apply to your automation.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The CloudWatch alarm you want to apply to your automation.
*
*
* @return The CloudWatch alarm you want to apply to your automation.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The CloudWatch alarm you want to apply to your automation.
*
*
* @param alarmConfiguration
* The CloudWatch alarm you want to apply to your automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAutomationExecutionRequest withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDocumentName() != null)
sb.append("DocumentName: ").append(getDocumentName()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getMode() != null)
sb.append("Mode: ").append(getMode()).append(",");
if (getTargetParameterName() != null)
sb.append("TargetParameterName: ").append(getTargetParameterName()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getTargetMaps() != null)
sb.append("TargetMaps: ").append(getTargetMaps()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getTargetLocations() != null)
sb.append("TargetLocations: ").append(getTargetLocations()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartAutomationExecutionRequest == false)
return false;
StartAutomationExecutionRequest other = (StartAutomationExecutionRequest) obj;
if (other.getDocumentName() == null ^ this.getDocumentName() == null)
return false;
if (other.getDocumentName() != null && other.getDocumentName().equals(this.getDocumentName()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getMode() == null ^ this.getMode() == null)
return false;
if (other.getMode() != null && other.getMode().equals(this.getMode()) == false)
return false;
if (other.getTargetParameterName() == null ^ this.getTargetParameterName() == null)
return false;
if (other.getTargetParameterName() != null && other.getTargetParameterName().equals(this.getTargetParameterName()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getTargetMaps() == null ^ this.getTargetMaps() == null)
return false;
if (other.getTargetMaps() != null && other.getTargetMaps().equals(this.getTargetMaps()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getTargetLocations() == null ^ this.getTargetLocations() == null)
return false;
if (other.getTargetLocations() != null && other.getTargetLocations().equals(this.getTargetLocations()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDocumentName() == null) ? 0 : getDocumentName().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getMode() == null) ? 0 : getMode().hashCode());
hashCode = prime * hashCode + ((getTargetParameterName() == null) ? 0 : getTargetParameterName().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getTargetMaps() == null) ? 0 : getTargetMaps().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getTargetLocations() == null) ? 0 : getTargetLocations().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
return hashCode;
}
@Override
public StartAutomationExecutionRequest clone() {
return (StartAutomationExecutionRequest) super.clone();
}
}