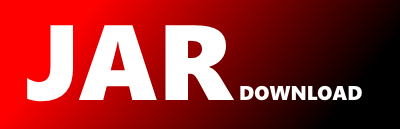
com.amazonaws.services.simplesystemsmanagement.model.StartChangeRequestExecutionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartChangeRequestExecutionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The date and time specified in the change request to run the Automation runbooks.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*/
private java.util.Date scheduledTime;
/**
*
* The name of the change template document to run during the runbook workflow.
*
*/
private String documentName;
/**
*
* The version of the change template document to run during the runbook workflow.
*
*/
private String documentVersion;
/**
*
* A key-value map of parameters that match the declared parameters in the change template document.
*
*/
private java.util.Map> parameters;
/**
*
* The name of the change request associated with the runbook workflow to be run.
*
*/
private String changeRequestName;
/**
*
* The user-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format, and
* can't be reused.
*
*/
private String clientToken;
/**
*
* Indicates whether the change request can be approved automatically without the need for manual approvals.
*
*
* If AutoApprovable
is enabled in a change template, then setting AutoApprove
to
* true
in StartChangeRequestExecution
creates a change request that bypasses approver
* review.
*
*
*
* Change Calendar restrictions are not bypassed in this scenario. If the state of an associated calendar is
* CLOSED
, change freeze approvers must still grant permission for this change request to run. If they
* don't, the change won't be processed until the calendar state is again OPEN
.
*
*
*/
private Boolean autoApprove;
/**
*
* Information about the Automation runbooks that are run during the runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*/
private com.amazonaws.internal.SdkInternalList runbooks;
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for a change request.
* Tags enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For
* example, you might want to tag a change request to identify an environment or target Amazon Web Services Region.
* In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Environment,Value=Production
*
*
* -
*
* Key=Region,Value=us-east-2
*
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The time that the requester expects the runbook workflow related to the change request to complete. The time is
* an estimate only that the requester provides for reviewers.
*
*/
private java.util.Date scheduledEndTime;
/**
*
* User-provided details about the change. If no details are provided, content specified in the Template
* information section of the associated change template is added.
*
*/
private String changeDetails;
/**
*
* The date and time specified in the change request to run the Automation runbooks.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @param scheduledTime
* The date and time specified in the change request to run the Automation runbooks.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
*/
public void setScheduledTime(java.util.Date scheduledTime) {
this.scheduledTime = scheduledTime;
}
/**
*
* The date and time specified in the change request to run the Automation runbooks.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @return The date and time specified in the change request to run the Automation runbooks.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
*/
public java.util.Date getScheduledTime() {
return this.scheduledTime;
}
/**
*
* The date and time specified in the change request to run the Automation runbooks.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @param scheduledTime
* The date and time specified in the change request to run the Automation runbooks.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withScheduledTime(java.util.Date scheduledTime) {
setScheduledTime(scheduledTime);
return this;
}
/**
*
* The name of the change template document to run during the runbook workflow.
*
*
* @param documentName
* The name of the change template document to run during the runbook workflow.
*/
public void setDocumentName(String documentName) {
this.documentName = documentName;
}
/**
*
* The name of the change template document to run during the runbook workflow.
*
*
* @return The name of the change template document to run during the runbook workflow.
*/
public String getDocumentName() {
return this.documentName;
}
/**
*
* The name of the change template document to run during the runbook workflow.
*
*
* @param documentName
* The name of the change template document to run during the runbook workflow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withDocumentName(String documentName) {
setDocumentName(documentName);
return this;
}
/**
*
* The version of the change template document to run during the runbook workflow.
*
*
* @param documentVersion
* The version of the change template document to run during the runbook workflow.
*/
public void setDocumentVersion(String documentVersion) {
this.documentVersion = documentVersion;
}
/**
*
* The version of the change template document to run during the runbook workflow.
*
*
* @return The version of the change template document to run during the runbook workflow.
*/
public String getDocumentVersion() {
return this.documentVersion;
}
/**
*
* The version of the change template document to run during the runbook workflow.
*
*
* @param documentVersion
* The version of the change template document to run during the runbook workflow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withDocumentVersion(String documentVersion) {
setDocumentVersion(documentVersion);
return this;
}
/**
*
* A key-value map of parameters that match the declared parameters in the change template document.
*
*
* @return A key-value map of parameters that match the declared parameters in the change template document.
*/
public java.util.Map> getParameters() {
return parameters;
}
/**
*
* A key-value map of parameters that match the declared parameters in the change template document.
*
*
* @param parameters
* A key-value map of parameters that match the declared parameters in the change template document.
*/
public void setParameters(java.util.Map> parameters) {
this.parameters = parameters;
}
/**
*
* A key-value map of parameters that match the declared parameters in the change template document.
*
*
* @param parameters
* A key-value map of parameters that match the declared parameters in the change template document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withParameters(java.util.Map> parameters) {
setParameters(parameters);
return this;
}
/**
* Add a single Parameters entry
*
* @see StartChangeRequestExecutionRequest#withParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest addParametersEntry(String key, java.util.List value) {
if (null == this.parameters) {
this.parameters = new java.util.HashMap>();
}
if (this.parameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.parameters.put(key, value);
return this;
}
/**
* Removes all the entries added into Parameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest clearParametersEntries() {
this.parameters = null;
return this;
}
/**
*
* The name of the change request associated with the runbook workflow to be run.
*
*
* @param changeRequestName
* The name of the change request associated with the runbook workflow to be run.
*/
public void setChangeRequestName(String changeRequestName) {
this.changeRequestName = changeRequestName;
}
/**
*
* The name of the change request associated with the runbook workflow to be run.
*
*
* @return The name of the change request associated with the runbook workflow to be run.
*/
public String getChangeRequestName() {
return this.changeRequestName;
}
/**
*
* The name of the change request associated with the runbook workflow to be run.
*
*
* @param changeRequestName
* The name of the change request associated with the runbook workflow to be run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withChangeRequestName(String changeRequestName) {
setChangeRequestName(changeRequestName);
return this;
}
/**
*
* The user-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format, and
* can't be reused.
*
*
* @param clientToken
* The user-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID
* format, and can't be reused.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* The user-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format, and
* can't be reused.
*
*
* @return The user-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID
* format, and can't be reused.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* The user-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID format, and
* can't be reused.
*
*
* @param clientToken
* The user-provided idempotency token. The token must be unique, is case insensitive, enforces the UUID
* format, and can't be reused.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* Indicates whether the change request can be approved automatically without the need for manual approvals.
*
*
* If AutoApprovable
is enabled in a change template, then setting AutoApprove
to
* true
in StartChangeRequestExecution
creates a change request that bypasses approver
* review.
*
*
*
* Change Calendar restrictions are not bypassed in this scenario. If the state of an associated calendar is
* CLOSED
, change freeze approvers must still grant permission for this change request to run. If they
* don't, the change won't be processed until the calendar state is again OPEN
.
*
*
*
* @param autoApprove
* Indicates whether the change request can be approved automatically without the need for manual
* approvals.
*
* If AutoApprovable
is enabled in a change template, then setting AutoApprove
to
* true
in StartChangeRequestExecution
creates a change request that bypasses
* approver review.
*
*
*
* Change Calendar restrictions are not bypassed in this scenario. If the state of an associated calendar is
* CLOSED
, change freeze approvers must still grant permission for this change request to run.
* If they don't, the change won't be processed until the calendar state is again OPEN
.
*
*/
public void setAutoApprove(Boolean autoApprove) {
this.autoApprove = autoApprove;
}
/**
*
* Indicates whether the change request can be approved automatically without the need for manual approvals.
*
*
* If AutoApprovable
is enabled in a change template, then setting AutoApprove
to
* true
in StartChangeRequestExecution
creates a change request that bypasses approver
* review.
*
*
*
* Change Calendar restrictions are not bypassed in this scenario. If the state of an associated calendar is
* CLOSED
, change freeze approvers must still grant permission for this change request to run. If they
* don't, the change won't be processed until the calendar state is again OPEN
.
*
*
*
* @return Indicates whether the change request can be approved automatically without the need for manual
* approvals.
*
* If AutoApprovable
is enabled in a change template, then setting AutoApprove
to
* true
in StartChangeRequestExecution
creates a change request that bypasses
* approver review.
*
*
*
* Change Calendar restrictions are not bypassed in this scenario. If the state of an associated calendar is
* CLOSED
, change freeze approvers must still grant permission for this change request to run.
* If they don't, the change won't be processed until the calendar state is again OPEN
.
*
*/
public Boolean getAutoApprove() {
return this.autoApprove;
}
/**
*
* Indicates whether the change request can be approved automatically without the need for manual approvals.
*
*
* If AutoApprovable
is enabled in a change template, then setting AutoApprove
to
* true
in StartChangeRequestExecution
creates a change request that bypasses approver
* review.
*
*
*
* Change Calendar restrictions are not bypassed in this scenario. If the state of an associated calendar is
* CLOSED
, change freeze approvers must still grant permission for this change request to run. If they
* don't, the change won't be processed until the calendar state is again OPEN
.
*
*
*
* @param autoApprove
* Indicates whether the change request can be approved automatically without the need for manual
* approvals.
*
* If AutoApprovable
is enabled in a change template, then setting AutoApprove
to
* true
in StartChangeRequestExecution
creates a change request that bypasses
* approver review.
*
*
*
* Change Calendar restrictions are not bypassed in this scenario. If the state of an associated calendar is
* CLOSED
, change freeze approvers must still grant permission for this change request to run.
* If they don't, the change won't be processed until the calendar state is again OPEN
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withAutoApprove(Boolean autoApprove) {
setAutoApprove(autoApprove);
return this;
}
/**
*
* Indicates whether the change request can be approved automatically without the need for manual approvals.
*
*
* If AutoApprovable
is enabled in a change template, then setting AutoApprove
to
* true
in StartChangeRequestExecution
creates a change request that bypasses approver
* review.
*
*
*
* Change Calendar restrictions are not bypassed in this scenario. If the state of an associated calendar is
* CLOSED
, change freeze approvers must still grant permission for this change request to run. If they
* don't, the change won't be processed until the calendar state is again OPEN
.
*
*
*
* @return Indicates whether the change request can be approved automatically without the need for manual
* approvals.
*
* If AutoApprovable
is enabled in a change template, then setting AutoApprove
to
* true
in StartChangeRequestExecution
creates a change request that bypasses
* approver review.
*
*
*
* Change Calendar restrictions are not bypassed in this scenario. If the state of an associated calendar is
* CLOSED
, change freeze approvers must still grant permission for this change request to run.
* If they don't, the change won't be processed until the calendar state is again OPEN
.
*
*/
public Boolean isAutoApprove() {
return this.autoApprove;
}
/**
*
* Information about the Automation runbooks that are run during the runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @return Information about the Automation runbooks that are run during the runbook workflow.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
*/
public java.util.List getRunbooks() {
if (runbooks == null) {
runbooks = new com.amazonaws.internal.SdkInternalList();
}
return runbooks;
}
/**
*
* Information about the Automation runbooks that are run during the runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @param runbooks
* Information about the Automation runbooks that are run during the runbook workflow.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
*/
public void setRunbooks(java.util.Collection runbooks) {
if (runbooks == null) {
this.runbooks = null;
return;
}
this.runbooks = new com.amazonaws.internal.SdkInternalList(runbooks);
}
/**
*
* Information about the Automation runbooks that are run during the runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRunbooks(java.util.Collection)} or {@link #withRunbooks(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param runbooks
* Information about the Automation runbooks that are run during the runbook workflow.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withRunbooks(Runbook... runbooks) {
if (this.runbooks == null) {
setRunbooks(new com.amazonaws.internal.SdkInternalList(runbooks.length));
}
for (Runbook ele : runbooks) {
this.runbooks.add(ele);
}
return this;
}
/**
*
* Information about the Automation runbooks that are run during the runbook workflow.
*
*
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the change
* request have been received.
*
*
*
* @param runbooks
* Information about the Automation runbooks that are run during the runbook workflow.
*
* The Automation runbooks specified for the runbook workflow can't run until all required approvals for the
* change request have been received.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withRunbooks(java.util.Collection runbooks) {
setRunbooks(runbooks);
return this;
}
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for a change request.
* Tags enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For
* example, you might want to tag a change request to identify an environment or target Amazon Web Services Region.
* In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Environment,Value=Production
*
*
* -
*
* Key=Region,Value=us-east-2
*
*
*
*
* @return Optional metadata that you assign to a resource. You can specify a maximum of five tags for a change
* request. Tags enable you to categorize a resource in different ways, such as by purpose, owner, or
* environment. For example, you might want to tag a change request to identify an environment or target
* Amazon Web Services Region. In this case, you could specify the following key-value pairs:
*
* -
*
* Key=Environment,Value=Production
*
*
* -
*
* Key=Region,Value=us-east-2
*
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for a change request.
* Tags enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For
* example, you might want to tag a change request to identify an environment or target Amazon Web Services Region.
* In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Environment,Value=Production
*
*
* -
*
* Key=Region,Value=us-east-2
*
*
*
*
* @param tags
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for a change
* request. Tags enable you to categorize a resource in different ways, such as by purpose, owner, or
* environment. For example, you might want to tag a change request to identify an environment or target
* Amazon Web Services Region. In this case, you could specify the following key-value pairs:
*
* -
*
* Key=Environment,Value=Production
*
*
* -
*
* Key=Region,Value=us-east-2
*
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for a change request.
* Tags enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For
* example, you might want to tag a change request to identify an environment or target Amazon Web Services Region.
* In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Environment,Value=Production
*
*
* -
*
* Key=Region,Value=us-east-2
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for a change
* request. Tags enable you to categorize a resource in different ways, such as by purpose, owner, or
* environment. For example, you might want to tag a change request to identify an environment or target
* Amazon Web Services Region. In this case, you could specify the following key-value pairs:
*
* -
*
* Key=Environment,Value=Production
*
*
* -
*
* Key=Region,Value=us-east-2
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for a change request.
* Tags enable you to categorize a resource in different ways, such as by purpose, owner, or environment. For
* example, you might want to tag a change request to identify an environment or target Amazon Web Services Region.
* In this case, you could specify the following key-value pairs:
*
*
* -
*
* Key=Environment,Value=Production
*
*
* -
*
* Key=Region,Value=us-east-2
*
*
*
*
* @param tags
* Optional metadata that you assign to a resource. You can specify a maximum of five tags for a change
* request. Tags enable you to categorize a resource in different ways, such as by purpose, owner, or
* environment. For example, you might want to tag a change request to identify an environment or target
* Amazon Web Services Region. In this case, you could specify the following key-value pairs:
*
* -
*
* Key=Environment,Value=Production
*
*
* -
*
* Key=Region,Value=us-east-2
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The time that the requester expects the runbook workflow related to the change request to complete. The time is
* an estimate only that the requester provides for reviewers.
*
*
* @param scheduledEndTime
* The time that the requester expects the runbook workflow related to the change request to complete. The
* time is an estimate only that the requester provides for reviewers.
*/
public void setScheduledEndTime(java.util.Date scheduledEndTime) {
this.scheduledEndTime = scheduledEndTime;
}
/**
*
* The time that the requester expects the runbook workflow related to the change request to complete. The time is
* an estimate only that the requester provides for reviewers.
*
*
* @return The time that the requester expects the runbook workflow related to the change request to complete. The
* time is an estimate only that the requester provides for reviewers.
*/
public java.util.Date getScheduledEndTime() {
return this.scheduledEndTime;
}
/**
*
* The time that the requester expects the runbook workflow related to the change request to complete. The time is
* an estimate only that the requester provides for reviewers.
*
*
* @param scheduledEndTime
* The time that the requester expects the runbook workflow related to the change request to complete. The
* time is an estimate only that the requester provides for reviewers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withScheduledEndTime(java.util.Date scheduledEndTime) {
setScheduledEndTime(scheduledEndTime);
return this;
}
/**
*
* User-provided details about the change. If no details are provided, content specified in the Template
* information section of the associated change template is added.
*
*
* @param changeDetails
* User-provided details about the change. If no details are provided, content specified in the Template
* information section of the associated change template is added.
*/
public void setChangeDetails(String changeDetails) {
this.changeDetails = changeDetails;
}
/**
*
* User-provided details about the change. If no details are provided, content specified in the Template
* information section of the associated change template is added.
*
*
* @return User-provided details about the change. If no details are provided, content specified in the Template
* information section of the associated change template is added.
*/
public String getChangeDetails() {
return this.changeDetails;
}
/**
*
* User-provided details about the change. If no details are provided, content specified in the Template
* information section of the associated change template is added.
*
*
* @param changeDetails
* User-provided details about the change. If no details are provided, content specified in the Template
* information section of the associated change template is added.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartChangeRequestExecutionRequest withChangeDetails(String changeDetails) {
setChangeDetails(changeDetails);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getScheduledTime() != null)
sb.append("ScheduledTime: ").append(getScheduledTime()).append(",");
if (getDocumentName() != null)
sb.append("DocumentName: ").append(getDocumentName()).append(",");
if (getDocumentVersion() != null)
sb.append("DocumentVersion: ").append(getDocumentVersion()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters()).append(",");
if (getChangeRequestName() != null)
sb.append("ChangeRequestName: ").append(getChangeRequestName()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getAutoApprove() != null)
sb.append("AutoApprove: ").append(getAutoApprove()).append(",");
if (getRunbooks() != null)
sb.append("Runbooks: ").append(getRunbooks()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getScheduledEndTime() != null)
sb.append("ScheduledEndTime: ").append(getScheduledEndTime()).append(",");
if (getChangeDetails() != null)
sb.append("ChangeDetails: ").append(getChangeDetails());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartChangeRequestExecutionRequest == false)
return false;
StartChangeRequestExecutionRequest other = (StartChangeRequestExecutionRequest) obj;
if (other.getScheduledTime() == null ^ this.getScheduledTime() == null)
return false;
if (other.getScheduledTime() != null && other.getScheduledTime().equals(this.getScheduledTime()) == false)
return false;
if (other.getDocumentName() == null ^ this.getDocumentName() == null)
return false;
if (other.getDocumentName() != null && other.getDocumentName().equals(this.getDocumentName()) == false)
return false;
if (other.getDocumentVersion() == null ^ this.getDocumentVersion() == null)
return false;
if (other.getDocumentVersion() != null && other.getDocumentVersion().equals(this.getDocumentVersion()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getChangeRequestName() == null ^ this.getChangeRequestName() == null)
return false;
if (other.getChangeRequestName() != null && other.getChangeRequestName().equals(this.getChangeRequestName()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getAutoApprove() == null ^ this.getAutoApprove() == null)
return false;
if (other.getAutoApprove() != null && other.getAutoApprove().equals(this.getAutoApprove()) == false)
return false;
if (other.getRunbooks() == null ^ this.getRunbooks() == null)
return false;
if (other.getRunbooks() != null && other.getRunbooks().equals(this.getRunbooks()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getScheduledEndTime() == null ^ this.getScheduledEndTime() == null)
return false;
if (other.getScheduledEndTime() != null && other.getScheduledEndTime().equals(this.getScheduledEndTime()) == false)
return false;
if (other.getChangeDetails() == null ^ this.getChangeDetails() == null)
return false;
if (other.getChangeDetails() != null && other.getChangeDetails().equals(this.getChangeDetails()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getScheduledTime() == null) ? 0 : getScheduledTime().hashCode());
hashCode = prime * hashCode + ((getDocumentName() == null) ? 0 : getDocumentName().hashCode());
hashCode = prime * hashCode + ((getDocumentVersion() == null) ? 0 : getDocumentVersion().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getChangeRequestName() == null) ? 0 : getChangeRequestName().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getAutoApprove() == null) ? 0 : getAutoApprove().hashCode());
hashCode = prime * hashCode + ((getRunbooks() == null) ? 0 : getRunbooks().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getScheduledEndTime() == null) ? 0 : getScheduledEndTime().hashCode());
hashCode = prime * hashCode + ((getChangeDetails() == null) ? 0 : getChangeDetails().hashCode());
return hashCode;
}
@Override
public StartChangeRequestExecutionRequest clone() {
return (StartChangeRequestExecutionRequest) super.clone();
}
}