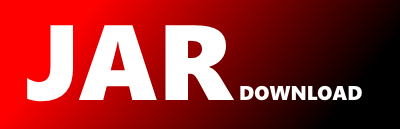
com.amazonaws.services.simplesystemsmanagement.model.StepExecution Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Detailed information about an the execution state of an Automation step.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StepExecution implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of this execution step.
*
*/
private String stepName;
/**
*
* The action this step performs. The action determines the behavior of the step.
*
*/
private String action;
/**
*
* The timeout seconds of the step.
*
*/
private Long timeoutSeconds;
/**
*
* The action to take if the step fails. The default value is Abort
.
*
*/
private String onFailure;
/**
*
* The maximum number of tries to run the action of the step. The default value is 1
.
*
*/
private Integer maxAttempts;
/**
*
* If a step has begun execution, this contains the time the step started. If the step is in Pending status, this
* field isn't populated.
*
*/
private java.util.Date executionStartTime;
/**
*
* If a step has finished execution, this contains the time the execution ended. If the step hasn't yet concluded,
* this field isn't populated.
*
*/
private java.util.Date executionEndTime;
/**
*
* The execution status for this step.
*
*/
private String stepStatus;
/**
*
* The response code returned by the execution of the step.
*
*/
private String responseCode;
/**
*
* Fully-resolved values passed into the step before execution.
*
*/
private java.util.Map inputs;
/**
*
* Returned values from the execution of the step.
*
*/
private java.util.Map> outputs;
/**
*
* A message associated with the response code for an execution.
*
*/
private String response;
/**
*
* If a step failed, this message explains why the execution failed.
*
*/
private String failureMessage;
/**
*
* Information about the Automation failure.
*
*/
private FailureDetails failureDetails;
/**
*
* The unique ID of a step execution.
*
*/
private String stepExecutionId;
/**
*
* A user-specified list of parameters to override when running a step.
*
*/
private java.util.Map> overriddenParameters;
/**
*
* The flag which can be used to end automation no matter whether the step succeeds or fails.
*
*/
private Boolean isEnd;
/**
*
* The next step after the step succeeds.
*
*/
private String nextStep;
/**
*
* The flag which can be used to help decide whether the failure of current step leads to the Automation failure.
*
*/
private Boolean isCritical;
/**
*
* Strategies used when step fails, we support Continue and Abort. Abort will fail the automation when the step
* fails. Continue will ignore the failure of current step and allow automation to run the next step. With
* conditional branching, we add step:stepName to support the automation to go to another specific step.
*
*/
private com.amazonaws.internal.SdkInternalList validNextSteps;
/**
*
* The targets for the step execution.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The combination of Amazon Web Services Regions and Amazon Web Services accounts targeted by the current
* Automation execution.
*
*/
private TargetLocation targetLocation;
/**
*
* The CloudWatch alarms that were invoked by the automation.
*
*/
private com.amazonaws.internal.SdkInternalList triggeredAlarms;
/**
*
* Information about the parent step.
*
*/
private ParentStepDetails parentStepDetails;
/**
*
* The name of this execution step.
*
*
* @param stepName
* The name of this execution step.
*/
public void setStepName(String stepName) {
this.stepName = stepName;
}
/**
*
* The name of this execution step.
*
*
* @return The name of this execution step.
*/
public String getStepName() {
return this.stepName;
}
/**
*
* The name of this execution step.
*
*
* @param stepName
* The name of this execution step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withStepName(String stepName) {
setStepName(stepName);
return this;
}
/**
*
* The action this step performs. The action determines the behavior of the step.
*
*
* @param action
* The action this step performs. The action determines the behavior of the step.
*/
public void setAction(String action) {
this.action = action;
}
/**
*
* The action this step performs. The action determines the behavior of the step.
*
*
* @return The action this step performs. The action determines the behavior of the step.
*/
public String getAction() {
return this.action;
}
/**
*
* The action this step performs. The action determines the behavior of the step.
*
*
* @param action
* The action this step performs. The action determines the behavior of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withAction(String action) {
setAction(action);
return this;
}
/**
*
* The timeout seconds of the step.
*
*
* @param timeoutSeconds
* The timeout seconds of the step.
*/
public void setTimeoutSeconds(Long timeoutSeconds) {
this.timeoutSeconds = timeoutSeconds;
}
/**
*
* The timeout seconds of the step.
*
*
* @return The timeout seconds of the step.
*/
public Long getTimeoutSeconds() {
return this.timeoutSeconds;
}
/**
*
* The timeout seconds of the step.
*
*
* @param timeoutSeconds
* The timeout seconds of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withTimeoutSeconds(Long timeoutSeconds) {
setTimeoutSeconds(timeoutSeconds);
return this;
}
/**
*
* The action to take if the step fails. The default value is Abort
.
*
*
* @param onFailure
* The action to take if the step fails. The default value is Abort
.
*/
public void setOnFailure(String onFailure) {
this.onFailure = onFailure;
}
/**
*
* The action to take if the step fails. The default value is Abort
.
*
*
* @return The action to take if the step fails. The default value is Abort
.
*/
public String getOnFailure() {
return this.onFailure;
}
/**
*
* The action to take if the step fails. The default value is Abort
.
*
*
* @param onFailure
* The action to take if the step fails. The default value is Abort
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withOnFailure(String onFailure) {
setOnFailure(onFailure);
return this;
}
/**
*
* The maximum number of tries to run the action of the step. The default value is 1
.
*
*
* @param maxAttempts
* The maximum number of tries to run the action of the step. The default value is 1
.
*/
public void setMaxAttempts(Integer maxAttempts) {
this.maxAttempts = maxAttempts;
}
/**
*
* The maximum number of tries to run the action of the step. The default value is 1
.
*
*
* @return The maximum number of tries to run the action of the step. The default value is 1
.
*/
public Integer getMaxAttempts() {
return this.maxAttempts;
}
/**
*
* The maximum number of tries to run the action of the step. The default value is 1
.
*
*
* @param maxAttempts
* The maximum number of tries to run the action of the step. The default value is 1
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withMaxAttempts(Integer maxAttempts) {
setMaxAttempts(maxAttempts);
return this;
}
/**
*
* If a step has begun execution, this contains the time the step started. If the step is in Pending status, this
* field isn't populated.
*
*
* @param executionStartTime
* If a step has begun execution, this contains the time the step started. If the step is in Pending status,
* this field isn't populated.
*/
public void setExecutionStartTime(java.util.Date executionStartTime) {
this.executionStartTime = executionStartTime;
}
/**
*
* If a step has begun execution, this contains the time the step started. If the step is in Pending status, this
* field isn't populated.
*
*
* @return If a step has begun execution, this contains the time the step started. If the step is in Pending status,
* this field isn't populated.
*/
public java.util.Date getExecutionStartTime() {
return this.executionStartTime;
}
/**
*
* If a step has begun execution, this contains the time the step started. If the step is in Pending status, this
* field isn't populated.
*
*
* @param executionStartTime
* If a step has begun execution, this contains the time the step started. If the step is in Pending status,
* this field isn't populated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withExecutionStartTime(java.util.Date executionStartTime) {
setExecutionStartTime(executionStartTime);
return this;
}
/**
*
* If a step has finished execution, this contains the time the execution ended. If the step hasn't yet concluded,
* this field isn't populated.
*
*
* @param executionEndTime
* If a step has finished execution, this contains the time the execution ended. If the step hasn't yet
* concluded, this field isn't populated.
*/
public void setExecutionEndTime(java.util.Date executionEndTime) {
this.executionEndTime = executionEndTime;
}
/**
*
* If a step has finished execution, this contains the time the execution ended. If the step hasn't yet concluded,
* this field isn't populated.
*
*
* @return If a step has finished execution, this contains the time the execution ended. If the step hasn't yet
* concluded, this field isn't populated.
*/
public java.util.Date getExecutionEndTime() {
return this.executionEndTime;
}
/**
*
* If a step has finished execution, this contains the time the execution ended. If the step hasn't yet concluded,
* this field isn't populated.
*
*
* @param executionEndTime
* If a step has finished execution, this contains the time the execution ended. If the step hasn't yet
* concluded, this field isn't populated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withExecutionEndTime(java.util.Date executionEndTime) {
setExecutionEndTime(executionEndTime);
return this;
}
/**
*
* The execution status for this step.
*
*
* @param stepStatus
* The execution status for this step.
* @see AutomationExecutionStatus
*/
public void setStepStatus(String stepStatus) {
this.stepStatus = stepStatus;
}
/**
*
* The execution status for this step.
*
*
* @return The execution status for this step.
* @see AutomationExecutionStatus
*/
public String getStepStatus() {
return this.stepStatus;
}
/**
*
* The execution status for this step.
*
*
* @param stepStatus
* The execution status for this step.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationExecutionStatus
*/
public StepExecution withStepStatus(String stepStatus) {
setStepStatus(stepStatus);
return this;
}
/**
*
* The execution status for this step.
*
*
* @param stepStatus
* The execution status for this step.
* @see AutomationExecutionStatus
*/
public void setStepStatus(AutomationExecutionStatus stepStatus) {
withStepStatus(stepStatus);
}
/**
*
* The execution status for this step.
*
*
* @param stepStatus
* The execution status for this step.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationExecutionStatus
*/
public StepExecution withStepStatus(AutomationExecutionStatus stepStatus) {
this.stepStatus = stepStatus.toString();
return this;
}
/**
*
* The response code returned by the execution of the step.
*
*
* @param responseCode
* The response code returned by the execution of the step.
*/
public void setResponseCode(String responseCode) {
this.responseCode = responseCode;
}
/**
*
* The response code returned by the execution of the step.
*
*
* @return The response code returned by the execution of the step.
*/
public String getResponseCode() {
return this.responseCode;
}
/**
*
* The response code returned by the execution of the step.
*
*
* @param responseCode
* The response code returned by the execution of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withResponseCode(String responseCode) {
setResponseCode(responseCode);
return this;
}
/**
*
* Fully-resolved values passed into the step before execution.
*
*
* @return Fully-resolved values passed into the step before execution.
*/
public java.util.Map getInputs() {
return inputs;
}
/**
*
* Fully-resolved values passed into the step before execution.
*
*
* @param inputs
* Fully-resolved values passed into the step before execution.
*/
public void setInputs(java.util.Map inputs) {
this.inputs = inputs;
}
/**
*
* Fully-resolved values passed into the step before execution.
*
*
* @param inputs
* Fully-resolved values passed into the step before execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withInputs(java.util.Map inputs) {
setInputs(inputs);
return this;
}
/**
* Add a single Inputs entry
*
* @see StepExecution#withInputs
* @returns a reference to this object so that method calls can be chained together.
*/
public StepExecution addInputsEntry(String key, String value) {
if (null == this.inputs) {
this.inputs = new java.util.HashMap();
}
if (this.inputs.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.inputs.put(key, value);
return this;
}
/**
* Removes all the entries added into Inputs.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution clearInputsEntries() {
this.inputs = null;
return this;
}
/**
*
* Returned values from the execution of the step.
*
*
* @return Returned values from the execution of the step.
*/
public java.util.Map> getOutputs() {
return outputs;
}
/**
*
* Returned values from the execution of the step.
*
*
* @param outputs
* Returned values from the execution of the step.
*/
public void setOutputs(java.util.Map> outputs) {
this.outputs = outputs;
}
/**
*
* Returned values from the execution of the step.
*
*
* @param outputs
* Returned values from the execution of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withOutputs(java.util.Map> outputs) {
setOutputs(outputs);
return this;
}
/**
* Add a single Outputs entry
*
* @see StepExecution#withOutputs
* @returns a reference to this object so that method calls can be chained together.
*/
public StepExecution addOutputsEntry(String key, java.util.List value) {
if (null == this.outputs) {
this.outputs = new java.util.HashMap>();
}
if (this.outputs.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.outputs.put(key, value);
return this;
}
/**
* Removes all the entries added into Outputs.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution clearOutputsEntries() {
this.outputs = null;
return this;
}
/**
*
* A message associated with the response code for an execution.
*
*
* @param response
* A message associated with the response code for an execution.
*/
public void setResponse(String response) {
this.response = response;
}
/**
*
* A message associated with the response code for an execution.
*
*
* @return A message associated with the response code for an execution.
*/
public String getResponse() {
return this.response;
}
/**
*
* A message associated with the response code for an execution.
*
*
* @param response
* A message associated with the response code for an execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withResponse(String response) {
setResponse(response);
return this;
}
/**
*
* If a step failed, this message explains why the execution failed.
*
*
* @param failureMessage
* If a step failed, this message explains why the execution failed.
*/
public void setFailureMessage(String failureMessage) {
this.failureMessage = failureMessage;
}
/**
*
* If a step failed, this message explains why the execution failed.
*
*
* @return If a step failed, this message explains why the execution failed.
*/
public String getFailureMessage() {
return this.failureMessage;
}
/**
*
* If a step failed, this message explains why the execution failed.
*
*
* @param failureMessage
* If a step failed, this message explains why the execution failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withFailureMessage(String failureMessage) {
setFailureMessage(failureMessage);
return this;
}
/**
*
* Information about the Automation failure.
*
*
* @param failureDetails
* Information about the Automation failure.
*/
public void setFailureDetails(FailureDetails failureDetails) {
this.failureDetails = failureDetails;
}
/**
*
* Information about the Automation failure.
*
*
* @return Information about the Automation failure.
*/
public FailureDetails getFailureDetails() {
return this.failureDetails;
}
/**
*
* Information about the Automation failure.
*
*
* @param failureDetails
* Information about the Automation failure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withFailureDetails(FailureDetails failureDetails) {
setFailureDetails(failureDetails);
return this;
}
/**
*
* The unique ID of a step execution.
*
*
* @param stepExecutionId
* The unique ID of a step execution.
*/
public void setStepExecutionId(String stepExecutionId) {
this.stepExecutionId = stepExecutionId;
}
/**
*
* The unique ID of a step execution.
*
*
* @return The unique ID of a step execution.
*/
public String getStepExecutionId() {
return this.stepExecutionId;
}
/**
*
* The unique ID of a step execution.
*
*
* @param stepExecutionId
* The unique ID of a step execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withStepExecutionId(String stepExecutionId) {
setStepExecutionId(stepExecutionId);
return this;
}
/**
*
* A user-specified list of parameters to override when running a step.
*
*
* @return A user-specified list of parameters to override when running a step.
*/
public java.util.Map> getOverriddenParameters() {
return overriddenParameters;
}
/**
*
* A user-specified list of parameters to override when running a step.
*
*
* @param overriddenParameters
* A user-specified list of parameters to override when running a step.
*/
public void setOverriddenParameters(java.util.Map> overriddenParameters) {
this.overriddenParameters = overriddenParameters;
}
/**
*
* A user-specified list of parameters to override when running a step.
*
*
* @param overriddenParameters
* A user-specified list of parameters to override when running a step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withOverriddenParameters(java.util.Map> overriddenParameters) {
setOverriddenParameters(overriddenParameters);
return this;
}
/**
* Add a single OverriddenParameters entry
*
* @see StepExecution#withOverriddenParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public StepExecution addOverriddenParametersEntry(String key, java.util.List value) {
if (null == this.overriddenParameters) {
this.overriddenParameters = new java.util.HashMap>();
}
if (this.overriddenParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.overriddenParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into OverriddenParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution clearOverriddenParametersEntries() {
this.overriddenParameters = null;
return this;
}
/**
*
* The flag which can be used to end automation no matter whether the step succeeds or fails.
*
*
* @param isEnd
* The flag which can be used to end automation no matter whether the step succeeds or fails.
*/
public void setIsEnd(Boolean isEnd) {
this.isEnd = isEnd;
}
/**
*
* The flag which can be used to end automation no matter whether the step succeeds or fails.
*
*
* @return The flag which can be used to end automation no matter whether the step succeeds or fails.
*/
public Boolean getIsEnd() {
return this.isEnd;
}
/**
*
* The flag which can be used to end automation no matter whether the step succeeds or fails.
*
*
* @param isEnd
* The flag which can be used to end automation no matter whether the step succeeds or fails.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withIsEnd(Boolean isEnd) {
setIsEnd(isEnd);
return this;
}
/**
*
* The flag which can be used to end automation no matter whether the step succeeds or fails.
*
*
* @return The flag which can be used to end automation no matter whether the step succeeds or fails.
*/
public Boolean isEnd() {
return this.isEnd;
}
/**
*
* The next step after the step succeeds.
*
*
* @param nextStep
* The next step after the step succeeds.
*/
public void setNextStep(String nextStep) {
this.nextStep = nextStep;
}
/**
*
* The next step after the step succeeds.
*
*
* @return The next step after the step succeeds.
*/
public String getNextStep() {
return this.nextStep;
}
/**
*
* The next step after the step succeeds.
*
*
* @param nextStep
* The next step after the step succeeds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withNextStep(String nextStep) {
setNextStep(nextStep);
return this;
}
/**
*
* The flag which can be used to help decide whether the failure of current step leads to the Automation failure.
*
*
* @param isCritical
* The flag which can be used to help decide whether the failure of current step leads to the Automation
* failure.
*/
public void setIsCritical(Boolean isCritical) {
this.isCritical = isCritical;
}
/**
*
* The flag which can be used to help decide whether the failure of current step leads to the Automation failure.
*
*
* @return The flag which can be used to help decide whether the failure of current step leads to the Automation
* failure.
*/
public Boolean getIsCritical() {
return this.isCritical;
}
/**
*
* The flag which can be used to help decide whether the failure of current step leads to the Automation failure.
*
*
* @param isCritical
* The flag which can be used to help decide whether the failure of current step leads to the Automation
* failure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withIsCritical(Boolean isCritical) {
setIsCritical(isCritical);
return this;
}
/**
*
* The flag which can be used to help decide whether the failure of current step leads to the Automation failure.
*
*
* @return The flag which can be used to help decide whether the failure of current step leads to the Automation
* failure.
*/
public Boolean isCritical() {
return this.isCritical;
}
/**
*
* Strategies used when step fails, we support Continue and Abort. Abort will fail the automation when the step
* fails. Continue will ignore the failure of current step and allow automation to run the next step. With
* conditional branching, we add step:stepName to support the automation to go to another specific step.
*
*
* @return Strategies used when step fails, we support Continue and Abort. Abort will fail the automation when the
* step fails. Continue will ignore the failure of current step and allow automation to run the next step.
* With conditional branching, we add step:stepName to support the automation to go to another specific
* step.
*/
public java.util.List getValidNextSteps() {
if (validNextSteps == null) {
validNextSteps = new com.amazonaws.internal.SdkInternalList();
}
return validNextSteps;
}
/**
*
* Strategies used when step fails, we support Continue and Abort. Abort will fail the automation when the step
* fails. Continue will ignore the failure of current step and allow automation to run the next step. With
* conditional branching, we add step:stepName to support the automation to go to another specific step.
*
*
* @param validNextSteps
* Strategies used when step fails, we support Continue and Abort. Abort will fail the automation when the
* step fails. Continue will ignore the failure of current step and allow automation to run the next step.
* With conditional branching, we add step:stepName to support the automation to go to another specific step.
*/
public void setValidNextSteps(java.util.Collection validNextSteps) {
if (validNextSteps == null) {
this.validNextSteps = null;
return;
}
this.validNextSteps = new com.amazonaws.internal.SdkInternalList(validNextSteps);
}
/**
*
* Strategies used when step fails, we support Continue and Abort. Abort will fail the automation when the step
* fails. Continue will ignore the failure of current step and allow automation to run the next step. With
* conditional branching, we add step:stepName to support the automation to go to another specific step.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setValidNextSteps(java.util.Collection)} or {@link #withValidNextSteps(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param validNextSteps
* Strategies used when step fails, we support Continue and Abort. Abort will fail the automation when the
* step fails. Continue will ignore the failure of current step and allow automation to run the next step.
* With conditional branching, we add step:stepName to support the automation to go to another specific step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withValidNextSteps(String... validNextSteps) {
if (this.validNextSteps == null) {
setValidNextSteps(new com.amazonaws.internal.SdkInternalList(validNextSteps.length));
}
for (String ele : validNextSteps) {
this.validNextSteps.add(ele);
}
return this;
}
/**
*
* Strategies used when step fails, we support Continue and Abort. Abort will fail the automation when the step
* fails. Continue will ignore the failure of current step and allow automation to run the next step. With
* conditional branching, we add step:stepName to support the automation to go to another specific step.
*
*
* @param validNextSteps
* Strategies used when step fails, we support Continue and Abort. Abort will fail the automation when the
* step fails. Continue will ignore the failure of current step and allow automation to run the next step.
* With conditional branching, we add step:stepName to support the automation to go to another specific step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withValidNextSteps(java.util.Collection validNextSteps) {
setValidNextSteps(validNextSteps);
return this;
}
/**
*
* The targets for the step execution.
*
*
* @return The targets for the step execution.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The targets for the step execution.
*
*
* @param targets
* The targets for the step execution.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The targets for the step execution.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The targets for the step execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The targets for the step execution.
*
*
* @param targets
* The targets for the step execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The combination of Amazon Web Services Regions and Amazon Web Services accounts targeted by the current
* Automation execution.
*
*
* @param targetLocation
* The combination of Amazon Web Services Regions and Amazon Web Services accounts targeted by the current
* Automation execution.
*/
public void setTargetLocation(TargetLocation targetLocation) {
this.targetLocation = targetLocation;
}
/**
*
* The combination of Amazon Web Services Regions and Amazon Web Services accounts targeted by the current
* Automation execution.
*
*
* @return The combination of Amazon Web Services Regions and Amazon Web Services accounts targeted by the current
* Automation execution.
*/
public TargetLocation getTargetLocation() {
return this.targetLocation;
}
/**
*
* The combination of Amazon Web Services Regions and Amazon Web Services accounts targeted by the current
* Automation execution.
*
*
* @param targetLocation
* The combination of Amazon Web Services Regions and Amazon Web Services accounts targeted by the current
* Automation execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withTargetLocation(TargetLocation targetLocation) {
setTargetLocation(targetLocation);
return this;
}
/**
*
* The CloudWatch alarms that were invoked by the automation.
*
*
* @return The CloudWatch alarms that were invoked by the automation.
*/
public java.util.List getTriggeredAlarms() {
if (triggeredAlarms == null) {
triggeredAlarms = new com.amazonaws.internal.SdkInternalList();
}
return triggeredAlarms;
}
/**
*
* The CloudWatch alarms that were invoked by the automation.
*
*
* @param triggeredAlarms
* The CloudWatch alarms that were invoked by the automation.
*/
public void setTriggeredAlarms(java.util.Collection triggeredAlarms) {
if (triggeredAlarms == null) {
this.triggeredAlarms = null;
return;
}
this.triggeredAlarms = new com.amazonaws.internal.SdkInternalList(triggeredAlarms);
}
/**
*
* The CloudWatch alarms that were invoked by the automation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTriggeredAlarms(java.util.Collection)} or {@link #withTriggeredAlarms(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param triggeredAlarms
* The CloudWatch alarms that were invoked by the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withTriggeredAlarms(AlarmStateInformation... triggeredAlarms) {
if (this.triggeredAlarms == null) {
setTriggeredAlarms(new com.amazonaws.internal.SdkInternalList(triggeredAlarms.length));
}
for (AlarmStateInformation ele : triggeredAlarms) {
this.triggeredAlarms.add(ele);
}
return this;
}
/**
*
* The CloudWatch alarms that were invoked by the automation.
*
*
* @param triggeredAlarms
* The CloudWatch alarms that were invoked by the automation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withTriggeredAlarms(java.util.Collection triggeredAlarms) {
setTriggeredAlarms(triggeredAlarms);
return this;
}
/**
*
* Information about the parent step.
*
*
* @param parentStepDetails
* Information about the parent step.
*/
public void setParentStepDetails(ParentStepDetails parentStepDetails) {
this.parentStepDetails = parentStepDetails;
}
/**
*
* Information about the parent step.
*
*
* @return Information about the parent step.
*/
public ParentStepDetails getParentStepDetails() {
return this.parentStepDetails;
}
/**
*
* Information about the parent step.
*
*
* @param parentStepDetails
* Information about the parent step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StepExecution withParentStepDetails(ParentStepDetails parentStepDetails) {
setParentStepDetails(parentStepDetails);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStepName() != null)
sb.append("StepName: ").append(getStepName()).append(",");
if (getAction() != null)
sb.append("Action: ").append(getAction()).append(",");
if (getTimeoutSeconds() != null)
sb.append("TimeoutSeconds: ").append(getTimeoutSeconds()).append(",");
if (getOnFailure() != null)
sb.append("OnFailure: ").append(getOnFailure()).append(",");
if (getMaxAttempts() != null)
sb.append("MaxAttempts: ").append(getMaxAttempts()).append(",");
if (getExecutionStartTime() != null)
sb.append("ExecutionStartTime: ").append(getExecutionStartTime()).append(",");
if (getExecutionEndTime() != null)
sb.append("ExecutionEndTime: ").append(getExecutionEndTime()).append(",");
if (getStepStatus() != null)
sb.append("StepStatus: ").append(getStepStatus()).append(",");
if (getResponseCode() != null)
sb.append("ResponseCode: ").append(getResponseCode()).append(",");
if (getInputs() != null)
sb.append("Inputs: ").append(getInputs()).append(",");
if (getOutputs() != null)
sb.append("Outputs: ").append(getOutputs()).append(",");
if (getResponse() != null)
sb.append("Response: ").append(getResponse()).append(",");
if (getFailureMessage() != null)
sb.append("FailureMessage: ").append(getFailureMessage()).append(",");
if (getFailureDetails() != null)
sb.append("FailureDetails: ").append(getFailureDetails()).append(",");
if (getStepExecutionId() != null)
sb.append("StepExecutionId: ").append(getStepExecutionId()).append(",");
if (getOverriddenParameters() != null)
sb.append("OverriddenParameters: ").append(getOverriddenParameters()).append(",");
if (getIsEnd() != null)
sb.append("IsEnd: ").append(getIsEnd()).append(",");
if (getNextStep() != null)
sb.append("NextStep: ").append(getNextStep()).append(",");
if (getIsCritical() != null)
sb.append("IsCritical: ").append(getIsCritical()).append(",");
if (getValidNextSteps() != null)
sb.append("ValidNextSteps: ").append(getValidNextSteps()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getTargetLocation() != null)
sb.append("TargetLocation: ").append(getTargetLocation()).append(",");
if (getTriggeredAlarms() != null)
sb.append("TriggeredAlarms: ").append(getTriggeredAlarms()).append(",");
if (getParentStepDetails() != null)
sb.append("ParentStepDetails: ").append(getParentStepDetails());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StepExecution == false)
return false;
StepExecution other = (StepExecution) obj;
if (other.getStepName() == null ^ this.getStepName() == null)
return false;
if (other.getStepName() != null && other.getStepName().equals(this.getStepName()) == false)
return false;
if (other.getAction() == null ^ this.getAction() == null)
return false;
if (other.getAction() != null && other.getAction().equals(this.getAction()) == false)
return false;
if (other.getTimeoutSeconds() == null ^ this.getTimeoutSeconds() == null)
return false;
if (other.getTimeoutSeconds() != null && other.getTimeoutSeconds().equals(this.getTimeoutSeconds()) == false)
return false;
if (other.getOnFailure() == null ^ this.getOnFailure() == null)
return false;
if (other.getOnFailure() != null && other.getOnFailure().equals(this.getOnFailure()) == false)
return false;
if (other.getMaxAttempts() == null ^ this.getMaxAttempts() == null)
return false;
if (other.getMaxAttempts() != null && other.getMaxAttempts().equals(this.getMaxAttempts()) == false)
return false;
if (other.getExecutionStartTime() == null ^ this.getExecutionStartTime() == null)
return false;
if (other.getExecutionStartTime() != null && other.getExecutionStartTime().equals(this.getExecutionStartTime()) == false)
return false;
if (other.getExecutionEndTime() == null ^ this.getExecutionEndTime() == null)
return false;
if (other.getExecutionEndTime() != null && other.getExecutionEndTime().equals(this.getExecutionEndTime()) == false)
return false;
if (other.getStepStatus() == null ^ this.getStepStatus() == null)
return false;
if (other.getStepStatus() != null && other.getStepStatus().equals(this.getStepStatus()) == false)
return false;
if (other.getResponseCode() == null ^ this.getResponseCode() == null)
return false;
if (other.getResponseCode() != null && other.getResponseCode().equals(this.getResponseCode()) == false)
return false;
if (other.getInputs() == null ^ this.getInputs() == null)
return false;
if (other.getInputs() != null && other.getInputs().equals(this.getInputs()) == false)
return false;
if (other.getOutputs() == null ^ this.getOutputs() == null)
return false;
if (other.getOutputs() != null && other.getOutputs().equals(this.getOutputs()) == false)
return false;
if (other.getResponse() == null ^ this.getResponse() == null)
return false;
if (other.getResponse() != null && other.getResponse().equals(this.getResponse()) == false)
return false;
if (other.getFailureMessage() == null ^ this.getFailureMessage() == null)
return false;
if (other.getFailureMessage() != null && other.getFailureMessage().equals(this.getFailureMessage()) == false)
return false;
if (other.getFailureDetails() == null ^ this.getFailureDetails() == null)
return false;
if (other.getFailureDetails() != null && other.getFailureDetails().equals(this.getFailureDetails()) == false)
return false;
if (other.getStepExecutionId() == null ^ this.getStepExecutionId() == null)
return false;
if (other.getStepExecutionId() != null && other.getStepExecutionId().equals(this.getStepExecutionId()) == false)
return false;
if (other.getOverriddenParameters() == null ^ this.getOverriddenParameters() == null)
return false;
if (other.getOverriddenParameters() != null && other.getOverriddenParameters().equals(this.getOverriddenParameters()) == false)
return false;
if (other.getIsEnd() == null ^ this.getIsEnd() == null)
return false;
if (other.getIsEnd() != null && other.getIsEnd().equals(this.getIsEnd()) == false)
return false;
if (other.getNextStep() == null ^ this.getNextStep() == null)
return false;
if (other.getNextStep() != null && other.getNextStep().equals(this.getNextStep()) == false)
return false;
if (other.getIsCritical() == null ^ this.getIsCritical() == null)
return false;
if (other.getIsCritical() != null && other.getIsCritical().equals(this.getIsCritical()) == false)
return false;
if (other.getValidNextSteps() == null ^ this.getValidNextSteps() == null)
return false;
if (other.getValidNextSteps() != null && other.getValidNextSteps().equals(this.getValidNextSteps()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getTargetLocation() == null ^ this.getTargetLocation() == null)
return false;
if (other.getTargetLocation() != null && other.getTargetLocation().equals(this.getTargetLocation()) == false)
return false;
if (other.getTriggeredAlarms() == null ^ this.getTriggeredAlarms() == null)
return false;
if (other.getTriggeredAlarms() != null && other.getTriggeredAlarms().equals(this.getTriggeredAlarms()) == false)
return false;
if (other.getParentStepDetails() == null ^ this.getParentStepDetails() == null)
return false;
if (other.getParentStepDetails() != null && other.getParentStepDetails().equals(this.getParentStepDetails()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStepName() == null) ? 0 : getStepName().hashCode());
hashCode = prime * hashCode + ((getAction() == null) ? 0 : getAction().hashCode());
hashCode = prime * hashCode + ((getTimeoutSeconds() == null) ? 0 : getTimeoutSeconds().hashCode());
hashCode = prime * hashCode + ((getOnFailure() == null) ? 0 : getOnFailure().hashCode());
hashCode = prime * hashCode + ((getMaxAttempts() == null) ? 0 : getMaxAttempts().hashCode());
hashCode = prime * hashCode + ((getExecutionStartTime() == null) ? 0 : getExecutionStartTime().hashCode());
hashCode = prime * hashCode + ((getExecutionEndTime() == null) ? 0 : getExecutionEndTime().hashCode());
hashCode = prime * hashCode + ((getStepStatus() == null) ? 0 : getStepStatus().hashCode());
hashCode = prime * hashCode + ((getResponseCode() == null) ? 0 : getResponseCode().hashCode());
hashCode = prime * hashCode + ((getInputs() == null) ? 0 : getInputs().hashCode());
hashCode = prime * hashCode + ((getOutputs() == null) ? 0 : getOutputs().hashCode());
hashCode = prime * hashCode + ((getResponse() == null) ? 0 : getResponse().hashCode());
hashCode = prime * hashCode + ((getFailureMessage() == null) ? 0 : getFailureMessage().hashCode());
hashCode = prime * hashCode + ((getFailureDetails() == null) ? 0 : getFailureDetails().hashCode());
hashCode = prime * hashCode + ((getStepExecutionId() == null) ? 0 : getStepExecutionId().hashCode());
hashCode = prime * hashCode + ((getOverriddenParameters() == null) ? 0 : getOverriddenParameters().hashCode());
hashCode = prime * hashCode + ((getIsEnd() == null) ? 0 : getIsEnd().hashCode());
hashCode = prime * hashCode + ((getNextStep() == null) ? 0 : getNextStep().hashCode());
hashCode = prime * hashCode + ((getIsCritical() == null) ? 0 : getIsCritical().hashCode());
hashCode = prime * hashCode + ((getValidNextSteps() == null) ? 0 : getValidNextSteps().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getTargetLocation() == null) ? 0 : getTargetLocation().hashCode());
hashCode = prime * hashCode + ((getTriggeredAlarms() == null) ? 0 : getTriggeredAlarms().hashCode());
hashCode = prime * hashCode + ((getParentStepDetails() == null) ? 0 : getParentStepDetails().hashCode());
return hashCode;
}
@Override
public StepExecution clone() {
try {
return (StepExecution) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.StepExecutionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}